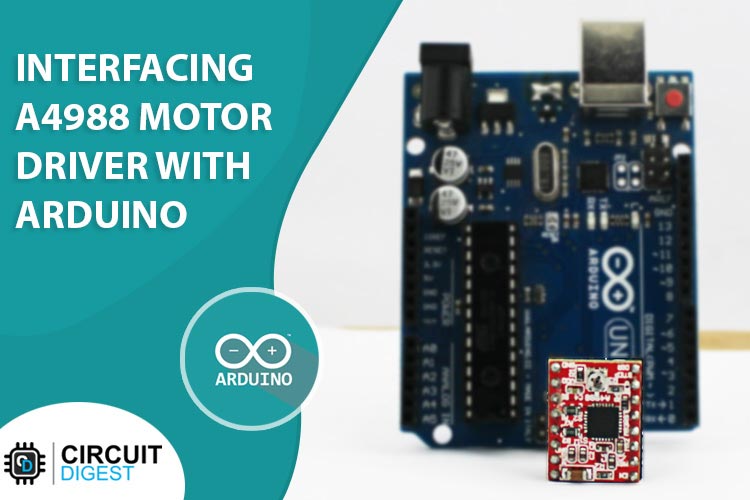
A stepper motor is a type of brushless DC motor that divides a full rotation into a number of steps, thus allowing for precise control of the motor's position. The A4988 driver is a versatile stepper driver for controlling stepper motors for various applications. When combined with an Arduino UNO microcontroller, the A4988 can be used to control a stepper motor in a highly precise and efficient manner. In this guide, we'll go over how to control a stepper motor with an A4988 driver and an Arduino UNO. We'll start by discussing the basic principles of stepper motors and the A4988 driver module. We'll then go over the wiring connections between the A4988, the stepper motor, and the Arduino. After that, we'll dive into the code, where we'll show you how to control the stepper motor's direction, speed, and number of steps. So without further ado let's get right into it.
How A4988 Stepper Motor Driver Module Works
Stepper motors use a cogged wheel and electromagnets to rotate the wheel one ‘step’ at a time. Each HIGH pulse energizes the coil, attracting the teeth closest to the cogged wheel and driving the motor one step forward.
The A4988 stepper motor driver has an output drive capacity of up to 35V and ±2A. This allows you to control a bipolar stepper motor, such as the NEMA 17, at up to 2A output current per coil. The gif above shows you how the motor works
A4988 Stepper Motor Driver Pinout
The A4988 Module has a total of 16 pins that connects to the stepper motor and the Arduino, The pinout is as follows
VDD This is the power pins of the Module, the VDD pin powers the internal logic circuit. That can range from 3.3V to 5.5V. It's pin 10 of the module.
GND The ground pin of the module connects to the ground pin of the Arduino. The A4988 module has two ground pins(9,16).
VMOT Motor Supply pin gives power to the motor, which can range from 8V to 35V. According to the datasheet, the motor driver requires a suitable decoupling capacitor close to the board.
MS1, MS2, MS3 Microstepping resolution select pins. The A4988 driver has three step size (resolution) selector inputs By setting the appropriate logic levels for these pins, we can set the motor to one of five step resolutions.
MS1 |
MS2 |
MS3 |
Microstep Resolution |
Low |
Low |
Low |
Full Step |
High |
Low |
Low |
Half Step |
Low |
High |
Low |
Quarter Step |
High |
High |
Low |
Eighth Step |
High |
High |
High |
Sixteenth Step |
These three microstep selection pins are pulled LOW by internal pull-down resistors, so if you leave them unconnected, the motor will operate in full step mode.
STEP input controls the microsteps of the motor. Each HIGH pulse sent to this pin drives the motor according to the number of microsteps determined by the microstep selection pins. The higher the pulse frequency, the faster the motor will spin.
DIR input controls the spinning direction of the motor. Pulling it HIGH turns the motor clockwise, while pulling it LOW turns it counterclockwise.
EN is an active low input pin. When this pin is pulled LOW, the A4988 driver is enabled. By default, this pin is pulled low, so unless you pull it high, the driver is always enabled. This pin is particularly useful when implementing an emergency stop or shutdown system.
SLP is an active low input pin. Pulling this pin LOW puts the driver into sleep mode, reducing power consumption to a minimum. You can use this to save power, especially when the motor is not in use.
RST is an active low input as well. When this pin is pulled LOW, all STEP inputs are ignored. It also resets the driver by setting the internal translator to a predefined “home” state. Home state is basically the initial position from which the motor starts, and it varies based on microstep resolution.
1B, 1A, 2A, 2B The output channels of the A4988 motor driver are broken out to the side of the module with pins. On the output pins small to medium size NEMA 17 motors can be connected Each output pin can supply up to 2A to the motor. However, the amount of current supplied to the motor is determined by the power supply, cooling system, and current limiting setting of the system.
A4988 Stepper Motor Driver Module Parts
The A4988 Stepper Motor Driver is a low cost high power motor driver IC that can be used for many applications. The parts marking of the A4988 is shown below.
As you can see from the above image the main component on the board is the A4988 stepper motor controller, other than that there are two more significant parts on the board
Commonly Asked Questions about A4988 Stepper Motor Driver
What is the specification of A4988?
The A4988 is a complete microstepping motor driver with built-in translator for easy operation. It is designed to operate bipolar stepper motors in full-, half-, quarter-, eighth-, and sixteenth-step modes, with an output drive capacity of up to 35 V and ±2 A.
What is the difference between A4988 and DRV8825?
The DRV8825 has a higher maximum supply voltage than the A4988 (45 V vs 35 V), which means the DRV8825 can be used more safely at higher voltages and is less susceptible to damage from LC voltage spikes.
What is the current limit of A4988?
The A4988 driver IC has a maximum current rating of 2 A per coil, but the actual current you can deliver depends on how well you can keep the IC cool.
Why does A4988 need a capacitor?
There are two purposes of such a capacitor: first it supplies power for short peaks in demand, so effectively enabling the 12V power source to supply much more current for short time, than it can support over long time and so the driver has more stable power and works generally better.
A4988 Stepper Motor Driver Circuit Diagram
The schematic diagram of the A4988 Stepper Driver module is shown above. The circuit is very simple and can be found in the datasheet of the A4899 module. But for the sake of simplicity, we will go through the circuit diagram and will try to figure out the most critical parts that need special attention.
Circuit Diagram of Interfacing A4988 with Arduino
The schematic diagram of the A4988 motor driver Module interfacing with Arduino is shown below, the schematic diagram is very simple and requires very few components to work with.
To power the logical part of the module we will be using a lab bench power supply and we will write the code so that the shaft of the motor will be moving back and forth so that we can see the working of the motor driver in action. In the image below we have shown you the hardware circuit of the motor driver.
If you are interested in other stepper motors including 28-BYJ48, then check our previous articles on their operation with different microcontrollers:
- Interfacing Stepper Motor with Arduino Uno
- Interfacing Stepper Motor with STM32F103C8
- Interfacing Stepper Motor with PIC Microcontroller
- Interfacing Stepper Motor with MSP430G2
- Stepper Motor Interfacing with 8051 Microcontroller
- Stepper Motor Control with Raspberry Pi
Arduino Code for interfacing A4988 Motor Driver IC with Arduino
The sample that follows demonstrates how to use the A4988 stepper motor driver to control the speed and spinning direction of a bipolar stepper motor may be used as the starting point for more realistic experiments and projects.
The code gets started by identifying the Arduino pins that are used to link the STEP and DIR pins of the A4988. StepsPerRevolution is a variable that is also defined. You may adjust it to your stepper motor's specifications.
const int dirPin = 2; const int stepPin = 3; const int stepsPerRevopinMode(stepPin, OUTPUT); pinMode(dirPin, OUTPUT);
In the setup section, all motor control pins are configured as digital OUTPUT.
pinMode(stepPin, OUTPUT); pinMode(dirPin, OUTPUT);
In the loop section, the motor is rotated slowly clockwise and then rapidly counterclockwise with one second intervals.
The spinning direction of the motor is controlled by,
digitalWrite(dirPin, HIGH);
The speed of the motor is controlled the following code
for(int x = 0; x < stepsPerRevolution; x++) { digitalWrite(stepPin, HIGH); delayMicroseconds(1000); digitalWrite(stepPin, LOW); delayMicroseconds(1000); }
Error in Interfacing A4988 4-Digit 7-Segment Display? - Here is what you Should do
- You see Stepper Drivers provide current to each coil of a Stepper Motor and those currents are switched back and forth to make the Stepper Motor rotate. So when a connection to one of the coils gets disconnected it creates a spike (current/voltage) that gets fed back to the Stepper Driver and can cause failure.
- For the stepper motor to work properly you need to connect a 100uF capacitor in parallel otherwise it can cause instability.
Projects Using Arduino and A4988 Stepper Motor Driver IC
If you are looking for a simple interfacing tutorial on A4988 motor driver module and Arduino, this project is for you, because in this project we not only interfaced the A4988 with Arduino we have also added an extra potentiometer to controller the rotation of the stepper.
If you have some of these cheap stepper drivers lying around and you are thinking about building projects with it, then this project might be for you, because as the name for this project goes we have built an automatic bottle filling system with it.
If you are looking for some really interesting projects to build and impress your friends then this projects is for you, in this project we have made a resistor cutting machine that will make your life a lot easier.
Complete Project Code
// Define pin connections & motor steps per revolution
const int dirPin = 2;
const int stepPin = 3;
const int stepsPerRevolution = 200;
void setup() {
// Declare pins as Outputs
pinMode(stepPin, OUTPUT);
pinMode(dirPin, OUTPUT);
}
void loop() {
// Set motor direction clockwise
digitalWrite(dirPin, HIGH);
// Spin motor slowly
for(int x = 0; x < stepsPerRevolution; x++) {
digitalWrite(stepPin, HIGH);
delayMicroseconds(2000);
digitalWrite(stepPin, LOW);
delayMicroseconds(2000);
}
delay(1000); // Wait a second
// Set motor direction counterclockwise
digitalWrite(dirPin, LOW);
// Spin motor quickly
for(int x = 0; x < stepsPerRevolution; x++) {
digitalWrite(stepPin, HIGH);
delayMicroseconds(1000);
digitalWrite(stepPin, LOW);
delayMicroseconds(1000);
}
delay(1000); // Wait a second
}