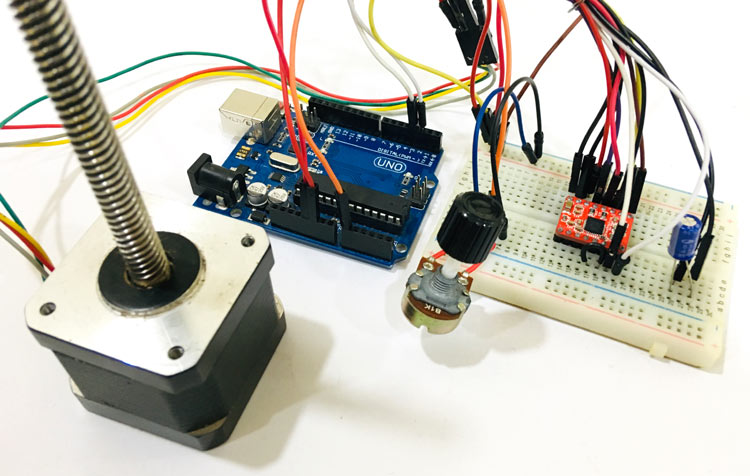
A stepper motor is a type of DC motor that works in discrete steps and used everywhere from a surveillance camera to sophisticated robots and machines. Stepper motors provide accurate controlling, and can be differentiated on the basis of torque, steps per revolution, and input voltage. In our previous project, we controlled 28-BYJ48 stepper motor using Arduino. 28-BYJ48 has relatively lower torque than the other stepper motors like NEMA 14, NEMA17.
In this tutorial, we are going to control NEMA17 stepper motor using Arduino Uno and A4988 stepper driver module. Nema17 stepper motor has higher torque and higher operating voltage than 28-BYJ48. Here a potentiometer will also be attached to control the direction of stepper motor.
Component Required
- Arduino UNO
- NEMA17 Stepper Motor
- A4988 Stepper Driver Module
- 47 µf Capacitor
- Potentiometer
NEMA17 Stepper Motor
Operation of Nema17 is similar to normal Stepper Motors. NEMA 17 stepper motor has a 1.7 x 1.7-inch faceplate, and it usually has more torque than the smaller variants, such as NEMA 14. This motor has six lead wires, and the rated voltage is 12 volt. It can be operated at a lower voltage, but torque will drop. Stepper motors do not rotate they step, and NEMA17 motor has a step angle of 1.8 deg. means it covers 1.8 degrees in every step. Wiring diagram for NEMA17 is given below.
As you can see that this motor has a Unipolar six-wire arrangement. These wire are connected in two split windings. Black, Yellow, Green wires are part of first winding where Black is center tap, and Yellow and Green are coil end while Red, White, and Blue is part of a second winding, in which White is center tap and Red and Blue are coil end wires. Normally center tap wires left disconnected.
Steps Per Revolution for NEMA17
Steps Per Revolution for a particular stepper motor is calculated using the step angle of that stepper motor. So in the case, NEMA 17 step angle is 1.8 deg.
Steps per Revolution = 360/ step angle 360/1.8 = 200 Steps Per Revolution
Specifications of NEMA17
- Rated Voltage: 12V DC
- Step Angle: 1.8 deg.
- No. of Phases: 4
- Motor Length: 1.54 inches
- 4-wire, 8-inch lead
- 200 steps per revolution, 1.8 degrees
- Operating Temperature: -10 to 40 °C
- Unipolar Holding Torque: 22.2 oz-in
Also check various stepper motor related projects here, which not only incudes basic interfacing with various microcontrollers but also have robotics projects which involves stepper motor.
A4988 Stepper Driver Module
A stepper driver module controls the working of a stepper motor. Stepper drivers send the current to stepper motor through various phases.
The A4988 Nema 17 stepper driver is a microstepping driver module that is used to control bipolar stepper motors. This driver module has a built-in translator that means that we can control the stepper motor using very few pins from our controller.
Using this Nema 17 motor driver module, we can control stepper motor by using only two pins, i.e., STEP and DIRECTION. STEP pin is used to control the steps while DIRECTION pin is used to control the direction of the motor. A4988 driver module provides five different step resolutions: full-step, haft-step, quarter-step, eight-step, and sixteenth-step. You can select the different step resolutions using the resolution selector pins ((MS1, MS2, and MS3). The truth table for these pins is given below:
MS1 | MS2 | MS3 | Microstep Resolution |
Low | Low | Low | Full Step |
High | Low | Low | ½ Step (Half Step) |
Low | High | Low | ¼ Step (Quarter Step) |
High | High | Low | 1/8 Step (Eighth Step) |
High | High | High | 1/16 Step (Sixteenth Step) |
Specifications of A4988
Max. Operating Voltage: 35V
Min. Operating Voltage: 8V
Max. Current Per Phase: 2A
Microstep resolution: Full step, ½ step, ¼ step, 1/8 and 1/16 step
Reverse voltage protection: No
Dimensions: 15.5 × 20.5 mm (0.6″ × 0.8″)
Circuit Diagram
Circuit diagram to control Nema 17 stepper motor with Arduino is given in the above image. As A4988 module has a built-in translator that means we only need to connect the Step and Direction pins to Arduino. Step pin is used for controlling the steps while the direction pin is used to control the direction. Stepper motor is powered using a 12V power source, and the A4988 module is powered via Arduino. Potentiometer is used to control the direction of the motor.
If you turn the potentiometer clockwise, then stepper will rotate clockwise, and if you turn potentiometer anticlockwise, then it will rotate anticlockwise. A 47 µf capacitor is used to protect the board from voltage spikes. MS1, MS2, and MS3 pins left disconnected, that means the driver will operate in full-step mode.
Complete connections for Arduino Nema 17 A4988 given in below table.
S.NO. |
A4988 Pin |
Connection |
1 |
VMOT |
+ve Of Battery |
2 |
GND |
-ve of Battery |
3 |
VDD |
5V of Arduino |
4 |
GND |
GND of Arduino |
5 |
STP |
Pin 3 of Arduino |
6 |
DIR |
Pin 2 of Arduino |
7 |
1A, 1B, 2A, 2B |
Stepper Motor |
Code Explanation
Complete code with working video control Nema 17 with Arduino is given at the end of this tutorial, here we are explaining the complete program to understand the working of the project.
First of all, add the stepper motor library to your Arduino IDE. You can download the stepper motor library from here.
After that define the no of steps for the NEMA 17. As we calculated, the no. of steps per revolution for NEMA 17 is 200.
#include <Stepper.h> #define STEPS 200
After that, specify the pins to which driver module is connected and define the motor interface type as Type1 because the motor is connected through the driver module.
Stepper stepper(STEPS, 2, 3); #define motorInterfaceType 1
Next set the speed for stepper motor using stepper.setSpeed function. Maximum motor speed for NEMA 17 is 4688 RPM but if we run it faster than 1000 RPM torque falls of quickly.
void setup() { stepper.setSpeed(1000);
Now in the main loop, we will read the potentiometer value from A0 pin. In this loop, there are two functions one is potVal, and the other is Pval. If the current value, i.e., potVal is higher than the previous value, i.e., Pval than it will move ten steps in the clockwise direction and if the current value is less than previous value than it will move ten steps in the counter-clockwise direction.
potVal = map(analogRead(A0),0,1024,0,500); if (potVal>Pval) stepper.step(10); if (potVal<Pval) stepper.step(-10); Pval = potVal;
Now connect the Arduino with your laptop and upload the code into your Arduino UNO board using Arduino IDE, select the Board and port no and then click on the upload button.
Now you can control the direction of Nema17 stepper motor using the potentiometer. The complete working of the project is shown in the video below. If you have any doubts regarding this project, post them in the comment section below.
#include <Stepper.h>
#define STEPS 200
// Define stepper motor connections and motor interface type. Motor interface type must be set to 1 when using a driver
Stepper stepper(STEPS, 2, 3); // Pin 2 connected to DIRECTION & Pin 3 connected to STEP Pin of Driver
#define motorInterfaceType 1
int Pval = 0;
int potVal = 0;
void setup() {
// Set the maximum speed in steps per second:
stepper.setSpeed(1000);
}
void loop() {
potVal = map(analogRead(A0),0,1024,0,500);
if (potVal>Pval)
stepper.step(10);
if (potVal<Pval)
stepper.step(-10);
Pval = potVal;
}
Controlling NEMA 17 Stepper Motor with Arduino and A4988 Stepper Driver Module
Hi
What changes to the code if any need to be made to make the stepper motor turn at 1/4 step and as slowly as possible. I am planning to use this circuit to control a HF magnetic loop antenna's variable capacitor so it needs to be slow , small steps for accuracy and it must stop where AND when I want it to stop.
cheers Mike