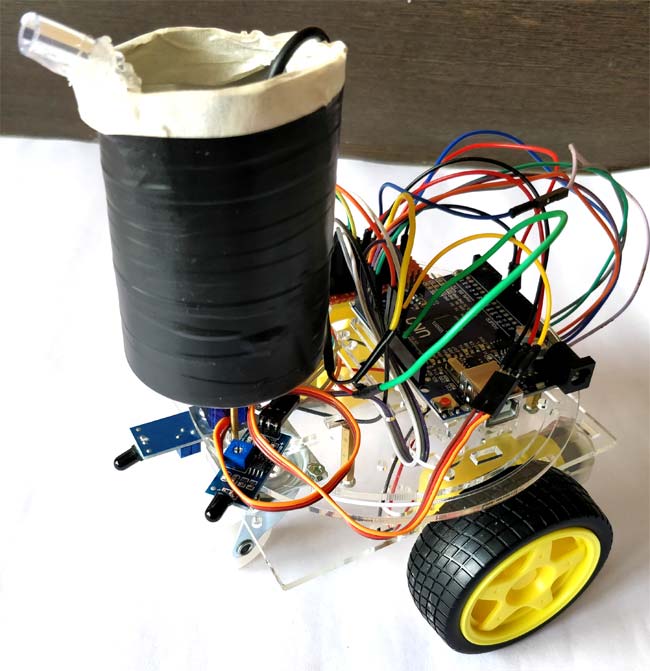
According to the latest data from the National Crime Records Bureau (NCRB), in 2022, India saw 7,435 deaths due to fire accidents. Even though there are a lot of precautions taken for Fire accidents, these natural/man-made disasters do occur now and then. In the event of a fire breakout, to rescue people and to put out the fire we are forced to use human resources which are not safe. With the advancement of technology especially in Robotics it is very much possible to replace humans with robots for fighting the fire. This would improve the efficiency of firefighters and would also prevent them from risking human lives. Today we are going to build a Fire Fighting Robot using Arduino, which will automatically sense the fire and start the water pump
In this project, we will learn how to build a simple robot using Arduino that could move towards the fire and pump out water around it to put down the fire. It is a very simple robot that would teach us the underlying concept of robotics; you would be able to build more sophisticated robots once you understand the following basics. So let’s get started...
Table of Contents
- What is a Fire Fighting Robot?
- Materials Required
- How to Make a Fire Fighting Robot
- Block Diagram
- Fire Fighting Robot Circuit Diagram
- Arduino Code Explanation
- Working Demonstration of Fire Fighting Robot
- Arduino Fire Fighting Robot Code - GitHub
- Common Issues and Solutions
- Conclusion and Future Developments
- Projects Similar to Fire Fighting Robot
What is a Fire Fighting Robot?
A fire fighting robot is an autonomous or remotely controlled machine designed to detect and extinguish fires without human intervention. These robots are typically equipped with advanced sensors to identify fire or smoke and carry water, CO2, or other suppression agents to control the flames. Integrated with onboard sensors and a microcontroller, some models of these robots can determine the fire's location, navigate toward it and efficiently extinguish it.
In this tutorial we will build a similar DIY fire fighting robot using Arduino, we will use IR sensors to detect the fire and BO motors with a motor driver to automatically move toward the fire. Our robot will also carry a small water tank and a pump to spray water on the fire and put it off.
Materials Required
- Arduino UNO
- Fire sensor or Flame sensor (3 Nos)
- Servo Motor (SG90)
- L293D motor Driver module
- Mini DC Submersible Pump
- Small Breadboard
- Robot chassis with motors (2) and wheels(2) (any type)
- A small can
- Connecting wires
How to Make a Fire Fighting Robot
The core of this project is the Arduino, which controls the fire detection and suppression system. To sense fire, we use a flame sensor module, as shown below.
These sensors feature an IR receiver (photodiode) that detects fire. When fire burns, it emits a small amount of infrared light, which is picked up by the IR receiver. An Op-Amp processes the signal and detects voltage changes across the IR receiver. If a fire is detected, the output pin (DO) goes LOW (0V), and if no fire is present, the output pin remains HIGH (5V).
To determine the fire's location, three sensors are placed in different directions on the robot. The below image shows our basic design of a fire fighting robot.
Once the fire's direction is identified, the robot moves toward it using motors controlled via an L293D motor driver module. To extinguish the fire, a small water container is mounted on the robot. A 5V water pump is placed inside the container, and the entire setup is positioned on a servo motor, allowing controlled water spraying in the required direction.
Block Diagram
The block diagram shown below illustrates the working of a fire-fighting robot using an Arduino UNO as the central controller.
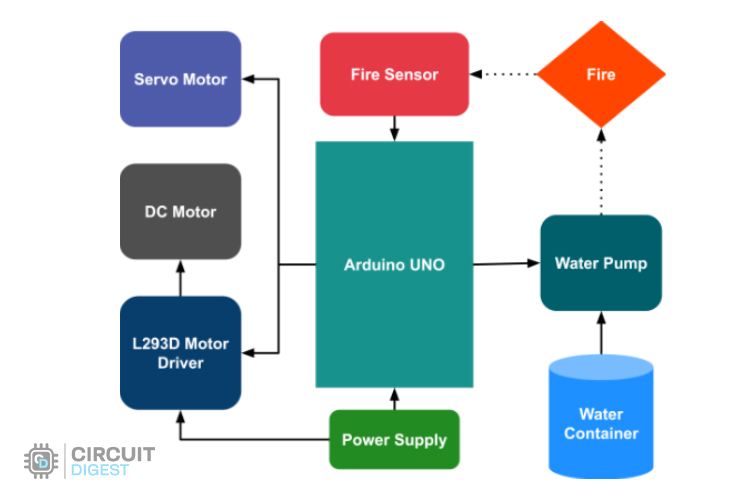
The fire sensor detects flames and sends signals to the Arduino, which processes the input and triggers necessary actions. Once fire is detected, the Arduino activates the water pump, drawing water from the container and spraying it onto the fire to extinguish it.
Simultaneously, the robot moves towards the fire using DC motors controlled by the L293D motor driver. A servo motor helps adjust the nozzle position for accurate water spraying. The entire system operates with a dedicated power supply, ensuring uninterrupted functionality.
Fire Fighting Robot Circuit Diagram
The complete circuit diagram for this Fire Fighting Robot is given below
You can either connect all the shown connections for uploading the program to check the working or you can assemble the bot completely and then proceed with the connections. Both ways the connections are very simple and you should be able to get it right.
Based on the robotic chassis that you are using you might not be able to use the same type of container that I am using. In that case use your own creativity to set up the pumping system. However the code will remain same. I used a small aluminium can (cool drinks can) to set the pump inside it and poured water inside it. I then assembled the whole can on top of a servo motor to control the direction of water. My robot looks something like this after assembly.
As you can see, I have fixed the servo fin to the bottom of the container using got glue and have fixed the servo motor with chassis using nuts and bolts. We can simply place the container on top of the motor and trigger the pump inside it to pump water outside through the tube. The whole container can then be rotated using the servo to control the direction of the water.
Arduino Code Explanation
Once you are ready with your hardware, you can upload the Arduino code for some action. The complete program is given at the end of this page. However, I have further explained few important bits and pieces here.
As we know the fire sensor will output a HIGH when there is fire and will output a LOW when there is fire. So we have to keep checking these sensor if any fire has occurred. If no fire is there we ask the motors to remain stop by making all the pins high as shown below
if (digitalRead(Left_S) ==1 && digitalRead(Right_S)==1 && digitalRead(Forward_S) ==1) //If Fire not detected all sensors are zero
{
//Do not move the robot
digitalWrite(LM1, HIGH);
digitalWrite(LM2, HIGH);
digitalWrite(RM1, HIGH);
digitalWrite(RM2, HIGH);
}
Similarly, if there is any fire we can ask the robot to move in that direction by rotating the respective motor. Once it reaches the fire the left and right sensor will not detect the fire as it would be standing straight ahead of the fire. Now we use the variable named “fire” that would execute the function to put off the fire.
else if (digitalRead(Forward_S) ==0) //If Fire is straight ahead
{
//Move the robot forward
digitalWrite(LM1, HIGH);
digitalWrite(LM2, LOW);
digitalWrite(RM1, HIGH);
digitalWrite(RM2, LOW);
fire = true;
}
Once the variable fire becomes true, the fire fighting robot arduino code will execute the put_off_fire function until the fire is put off. This is done using the code below.
while (fire == true)
{
put_off_fire();
}
Inside the put_off_fire() we just have to stop the robot by making all the pins high. Then turn on the pump to push water outside the container, while this is done we can also use the servo motor to rotate the container so that the water is split all over uniformly. This is done using the code below
void put_off_fire()
{
delay (500);
digitalWrite(LM1, HIGH);
digitalWrite(LM2, HIGH);
digitalWrite(RM1, HIGH);
digitalWrite(RM2, HIGH);
digitalWrite(pump, HIGH); delay(500);
for (pos = 50; pos <= 130; pos += 1) {
myservo.write(pos);
delay(10);
}
for (pos = 130; pos >= 50; pos -= 1) {
myservo.write(pos);
delay(10);
}
digitalWrite(pump,LOW);
myservo.write(90);
fire=false;
}
Working Demonstration of Fire Fighting Robot:
It is recommended to check the output of the robot in steps rather than running it all together for the first time. You can build the robot upto the servo motor and check if it is able to follow the fire successfully. Then you can check if the pump and the servo motor are working properly. You can also check out the working video of Fire Fighting Robot to see this robot in action, the video shows the robot automatically moving towards the flame and pumping water to extinguish the fire.
The maximum distance to which the fire can be detected depends on the size of the fire, for a small matchstick, the distance is relatively less. You can also use the potentiometers on top of the modules to control the sensitivity of the robot. I have used a power bank to power the robot you can use a battery or even power it with a 12V battery.
Hope you understood the project and would enjoy building something similar. If you have any problems in getting this build, use the comment section below to post your queries or use the forums for technical help.
Arduino Fire Fighting Robot Code - GitHub
You can find the complete code for the Arduino Fire Fighting Robot Project on the bottom of this page. Additionally, we have also created a GitHub repo for this project for people to easily access the code and other related files.
Common Issues and Solutions
If your line following robot is not working, do not worry it is common for people to face troubles especially if this is your first robot. Here are some common issues with their solutions, these issues were raised by our readers in the comment section below
If the flame sensor is not detecting fire, check its placement and ensure it is positioned correctly to detect flames without obstruction. Adjust the sensor’s sensitivity settings if necessary. Ambient light can sometimes interfere with detection, so test the sensor in different lighting conditions to rule out external interference. Also if you can check out our Arduino Flame Sensor tutorial to understand more about flame sensor and how to use it with arudino.
If the robot is not moving, inspect the motor driver wiring to confirm all connections are secure and properly soldered. The motor driver should be receiving the correct voltage, typically 12V, for optimal performance. Ensure the power source is stable and provides sufficient current to drive the motors.
If the water pump is not spraying, check the relay module and all wiring connections to verify they are intact and correctly configured. The pump should be receiving power when activated. Use a multimeter to check for voltage at the pump terminals and confirm that the relay is switching correctly. If the issue persists, inspect the pump for clogs or mechanical failures.
If you are still facing problems you can post them in the comment section below or write on our forums and we will help you out with troubleshooting.
Conclusion and Future Developments
In this project, we successfully built an Arduino-based Fire Fighting Robot that can detect and extinguish fires autonomously. By integrating flame sensors, a motorized water pump, and a servo-controlled water spray system, the robot efficiently locates and suppresses fires. This simple yet effective design demonstrates the potential of robotics in fire safety applications. With further enhancements, such as advanced sensors and AI-based navigation, this simple arduino based fire fighting robot project can be expanded for real world firefighting scenarios.
Projects Similar to Fire Fighting Robot
If you have successfully completed your firefighting robot project and are looking for more, we got you covered. Here is a list of DIY electronics projects with code and schematics that you build next. All these projects are built by us here in CircuitDigest and uses more or less the same components that is used in this project.
Obstacle Avoidance Robot using Arduino and Ultrasonic Sensor
Obstacle Avoiding Robot is an intelligent device that can automatically sense the obstacle in front of it and avoid them by turning itself in another direction. This design allows the robot to navigate in an unknown environment by avoiding collisions, which is a primary requirement for any autonomous mobile robot.
This robot car can be controlled wirelessly with Bluetooth and an android app, and other than that we have placed RGB Neopixel LEDs on the front, back, and bottom of the robot to make it look cool. We will also build a custom android app with the MIT app inventor so that we can send commands directly via Bluetooth.
Simple Arduino Line Following Robot
Line Follower Robot (LFR) is a simple autonomously guided robot that follows a line drawn on the ground to either detect a dark line on a white surface or a white line on a dark. The LFR is quite an interesting project to work on! In this tutorial, we will learn how to build a black line follower robot using Arduino Uno and some easily accessible components.
Gesture Controlled Robot Using Arduino
Let's build a cool hand gesture-controlled robot that'll amaze your friends and family—it's like magic! This step-by-step guide helps you build a hand gesture-controlled robot using simple components like Arduino, MPU6050 Accelerometer, nRF24L01 Transmitter-Receiver Pair, and L293D motor driver module. We'll split the robot into two parts: the Transmitter and the Receiver.
Light Following Robot using Arduino UNO
Today, we are building a simple Arduino-based project: a light-following robot. This project is perfect for beginners, and we'll use LDR sensor modules to detect light and an MX1508 motor driver module for control.
Complete Project Code
/*------ Arduino Fire Fighting Robot Code----- */
#include <Servo.h>
Servo myservo;
int pos = 0;
boolean fire = false;
/*-------defining Inputs------*/
#define Left_S 9 // left sensor
#define Right_S 10 // right sensor
#define Forward_S 8 //forward sensor
/*-------defining Outputs------*/
#define LM1 2 // left motor
#define LM2 3 // left motor
#define RM1 4 // right motor
#define RM2 5 // right motor
#define pump 6
void setup()
{
pinMode(Left_S, INPUT);
pinMode(Right_S, INPUT);
pinMode(Forward_S, INPUT);
pinMode(LM1, OUTPUT);
pinMode(LM2, OUTPUT);
pinMode(RM1, OUTPUT);
pinMode(RM2, OUTPUT);
pinMode(pump, OUTPUT);
myservo.attach(11);
myservo.write(90);
}
void put_off_fire()
{
delay (500);
digitalWrite(LM1, HIGH);
digitalWrite(LM2, HIGH);
digitalWrite(RM1, HIGH);
digitalWrite(RM2, HIGH);
digitalWrite(pump, HIGH); delay(500);
for (pos = 50; pos <= 130; pos += 1) {
myservo.write(pos);
delay(10);
}
for (pos = 130; pos >= 50; pos -= 1) {
myservo.write(pos);
delay(10);
}
digitalWrite(pump,LOW);
myservo.write(90);
fire=false;
}
void loop()
{
myservo.write(90); //Sweep_Servo();
if (digitalRead(Left_S) ==1 && digitalRead(Right_S)==1 && digitalRead(Forward_S) ==1) //If Fire not detected all sensors are zero
{
//Do not move the robot
digitalWrite(LM1, HIGH);
digitalWrite(LM2, HIGH);
digitalWrite(RM1, HIGH);
digitalWrite(RM2, HIGH);
}
else if (digitalRead(Forward_S) ==0) //If Fire is straight ahead
{
//Move the robot forward
digitalWrite(LM1, HIGH);
digitalWrite(LM2, LOW);
digitalWrite(RM1, HIGH);
digitalWrite(RM2, LOW);
fire = true;
}
else if (digitalRead(Left_S) ==0) //If Fire is to the left
{
//Move the robot left
digitalWrite(LM1, HIGH);
digitalWrite(LM2, LOW);
digitalWrite(RM1, HIGH);
digitalWrite(RM2, HIGH);
}
else if (digitalRead(Right_S) ==0) //If Fire is to the right
{
//Move the robot right
digitalWrite(LM1, HIGH);
digitalWrite(LM2, HIGH);
digitalWrite(RM1, HIGH);
digitalWrite(RM2, LOW);
}
delay(300); //Slow down the speed of robot
while (fire == true)
{
put_off_fire();
}
}
Comments
Hi, is there anybody else that knows what is the correct L293D motor driver module to buy. I want the same exact one that is shown in the project.If you do, do you know where I can buy it ? Please respond to me as soon as possible.
All module will work the same. Just make sure you do the connections right. Don't worry about getting the same module
Hi, thanks for the response
I have eliminated the previous issue
Now situation is like this
Robot is moving and stopping for a couple of seconds
Pump is working when wheels stops and synchronous motor is rotating according to pump
But the issue i am facing is :
It looks like arduino is giving command to pump.to spray water irrespective of flame sensor
I have disconnected the flame sensor still it is working the same
Can you kindly go through or match the code with ur working code so that if there is a bracket missing or something else, kindly guide me
Thanks
Hi, Thank you Hiro_Hamada for your help.
If i change l293d to l298n ..did have the same function ?
Hi Everyone,
I need help to get the right code to make the robot work properly. have used below code but is not working. what should I delete or add to below code?
/*------ Arduino Fire Fighting Robot Code----- */
#include <Servo.h>
Servo myservo;
int pos = 0;
boolean fire = false;
/*-------defining Inputs------*/
#define Left_S 9 // left sensor
#define Right_S 10 // right sensor
#define Forward_S 8 //forward sensor
/*-------defining Outputs------*/
#define LM1 2 // left motor
#define LM2 3 // left motor
#define RM1 4 // right motor
#define RM2 5 // right motor
#define pump 6
void setup()
{
pinMode(Left_S, INPUT);
pinMode(Right_S, INPUT);
pinMode(Forward_S, INPUT);
pinMode(LM1, OUTPUT);
pinMode(LM2, OUTPUT);
pinMode(RM1, OUTPUT);
pinMode(RM2, OUTPUT);
pinMode(pump, OUTPUT);
myservo.attach(11);
myservo.write(90);
}
void put_off_fire()
{
delay (500);
digitalWrite(LM1, HIGH);
digitalWrite(LM2, HIGH);
digitalWrite(RM1, HIGH);
digitalWrite(RM2, HIGH);
digitalWrite(pump, HIGH); delay(500);
for (pos = 50; pos <= 130; pos += 1) {
myservo.write(pos);
delay(10);
}
for (pos = 130; pos >= 50; pos -= 1) {
myservo.write(pos);
delay(10);
}
digitalWrite(pump,LOW);
myservo.write(90);
fire=false;
}
void loop()
{
myservo.write(90); //Sweep_Servo();
if (digitalRead(Left_S) ==1 && digitalRead(Right_S)==1 && digitalRead(Forward_S) ==1) //If Fire not detected all sensors are zero
{
//Do not move the robot
digitalWrite(LM1, HIGH);
digitalWrite(LM2, HIGH);
digitalWrite(RM1, HIGH);
digitalWrite(RM2, HIGH);
}
else if (digitalRead(Forward_S) ==0) //If Fire is straight ahead
{
//Move the robot forward
digitalWrite(LM1, HIGH);
digitalWrite(LM2, LOW);
digitalWrite(RM1, HIGH);
digitalWrite(RM2, LOW);
fire = true;
}
else if (digitalRead(Left_S) ==0) //If Fire is to the left
{
//Move the robot left
digitalWrite(LM1, HIGH);
digitalWrite(LM2, LOW);
digitalWrite(RM1, HIGH);
digitalWrite(RM2, HIGH);
}
else if (digitalRead(Right_S) ==0) //If Fire is to the right
{
//Move the robot right
digitalWrite(LM1, HIGH);
digitalWrite(LM2, HIGH);
digitalWrite(RM1, HIGH);
digitalWrite(RM2, LOW);
}
delay(300); //Slow down the speed of robot
while (fire == true)
{
put_off_fire();
}
}
I am not using water pump ...only wants the robot to follow fire when sensor detects fire please anybody can help the project is due this Monday 30th
When it detect flame, servo motor works but due to high current capacity of pump it does not work with arduino .. plz help me to run it ... Rest is working fine..
kindly sir, can you tell me, how you learn about the codding, which is used in this project, and which software is use for this codding.
Hi sir, please help me, when i try, the robot move forward 3 sec and stop 3 sec and continous
This means that the left and right flame sensors are not picking up any heat signals. Make sure they are wired correctly and also check for the sensitivity level by varying the pot on the sensor
ok sir thankyou for the answer, the trouble is on the sensor, sensor right and left same model, but sensor forward is different. sensor forward when read fire the output 5v but sensor right and left when read fire = 0v hmmm...so i modified the program...and the robot now can working
How to to connect 12V water pump instead of 5V?
I used a 5 channel flame sensor module, but it is highliy senstivie. It sends the signal to arduino (fire detected:spray water) in a normal place like having temperature greater than 30(outdoor). Can any one help me how to overcome this issue
The sensor does not react to heat, it reads to IR light. So if you are operating the bot in a place sunlight is high then the sensor by get false triggered.
If you want to reduce the sensitivity then check of there is a pot on the sensor module,if yes vary it else try using analog read if the sensor supports it
How to connect a 9V battery instead of connecting with arduino wire to laptop to power the robot
Hi Sir,
Can i get the correct code and connection, please help me!!
What kind of robot chassis and container is used here ?
i just want to run a water pump when sensor detect the fire what is the coding for that. i code by my self but when sensor detect the fire pump was not active but than i use led instead of pump led was on when sensor detect the fire.How the pump is going to work plz any one help.Thanks
What pump are you using? If the LED is working but not your pump then the problem is not with your code. Check your pump driver circuit
hi bro.may be your pump requires more voltages and your driver circuit in not providing the required voltages.try adding the required voltages by connecting the suitable batter in series
Hi sir!
Can I get the correct code? It is not work like you do.
I have used a 9 volt water pump but it is not working please help me in this matter as soon as possible.
can I connect a motor with a fan other than a water pumping motor pls helps me guys ASAP
thank you very much for providing such important information but i am facing a problem that when i compile the code i get a compilation error in the first line
which is expected unqualified-id.kindly guide me about it.
Post the complete Error from console
Can i use 12v water pump ?.. cause I can't fint 5v water pump easily .
Yes you can and for this puropose u need to connect you pump with mosfet
thank you for sharing .the robot works when the supplied connections are made, but the 5v water pump does not work . I would appreciate if you could help me with this.
Please give me the circuit diagram
Hello OP. I need some advice and insight related to this project. Is it possible to connect four of these sensors in parallel with two servos and get them to perform a Top-right, bottom-right, top-left and bottom-left motion? And how will this affect the original code if it were to be implemented. A quick reply would be deeply appreciated
Can I control my motors using L298n Motor driver ? could you please elaborate how ?
Sir please explain circuit diagram
Sir please explain circuit diagram
Sir please explain circuit diagram
How to connect the water tank movement controlling motor
How to connect the water tank movement controlling motor in to the arduino board
How to connect the water tank movement controlling motor in to the arduino board
What is the name of that motor (water tank movement controlling motor)
What should I do if water pump is not working when connected to 6 number pin of arduino.but it is working when it is connected between +5V and ground. I guess the pump is not receiving enough voltage when connected to arduino.Is there any solution to solve this problem. Please reply as soon as possible.
can i use camera and connect it with my smart phone... plusIR US MQ sensors..will microcontroller of arduino hold that much power or shoul i go for rasberry pi
something code is not correct!!
there is anyone have a correct code?
Hello sir can I know more about motor driver because it's not clear in the diagramme.
Hi,this is my first time to join with you ,i am so happy becuse you are helping each others,thanks for all
Hey admin!!
I have done all the connections correctly but my robot is only to its left and the wheels are also moving in the opposite direction.. servo motor is also not working.. i have not tested the water pump yet I will test that after these issues are solved
Looking forward to your assistance
thanks
Will you please post a video for assembly of fire fighting robot ?
hello sir can you give the access of your simulation so that i can check whats wrong
I think you should elaborate the problem on the forum. That way you can get quick results. Also post some picture so as to help you