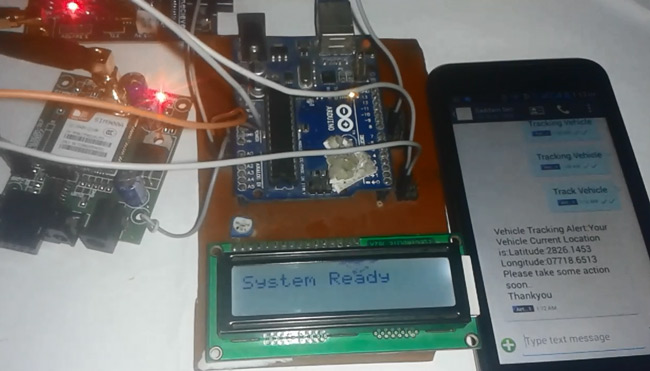
Vehicle Tracking systems are very commonly used in fleet management and asset tracking applications. Today these systems can not only track the location of the vehicle but can also report the speed and even control it remotely. In general, tracking of vehicles is a process in which we track the vehicle location in form of Latitude and Longitude (GPS coordinates). GPS Coordinates are the value of a location. This system is very efficient for outdoor application purposes. This kind of Vehicle Tracking System Project is widely in tracking Cabs/Taxis, stolen vehicles, school/college buses, etc.In this project, we are going one step ahead with GPS building a GSM and GPS based vehicle tracking system using Arduino. This Vehicle Tracking System can also be used to track a vehicle using GPS and GSM and can also be used as Accident Detection Alert System, Soldier Tracking System and many more, by just making few changes in hardware and software.
We have also build many other types of vehicle tracking systems previously, you can check them out if interested
- GPS Vehicle Tracking and Accident Alert using Arduino
- Vehicle Tracking with Google Maps using Arduino and ESP8266
- GPS Vehicle Tracking and Accident Alert using MSP430
- LoRa based GPS Vehicle Tracking using Arduino
- Location Tracker without GPS using SIM800 and Arduino
Components Required for Arduino based Vehicle Tracking system:
To build a simple vehicle tracking system suing Arduino we will need the following components.
- Arduino UNO
- GSM Module
- GPS Module
- 16x2 LCD
- Power Supply
- Connecting Wires
- 10 K POT
How can GSM Module be used to track location:
GPS stands for Global Positioning System and used to detect the Latitude and Longitude of any location on the Earth, with exact UTC time (Universal Time Coordinated). GPS module is the main component in our vehicle tracking system project. This device receives the coordinates from the satellite for each and every second, with time and date.
GPS module sends the data related to tracking position in real time, and it sends so many data in NMEA format (see the screenshot below). NMEA format consist several sentences, in which we only need one sentence. This sentence starts from $GPGGA and contains the coordinates, time and other useful information. This GPGGA is referred to Global Positioning System Fix Data. Know more about Reading GPS data and its strings here.
We can extract coordinate from $GPGGA string by counting the commas in the string. Suppose you find $GPGGA string and stores it in an array, then Latitude can be found after two commas and Longitude can be found after four commas. Now these latitude and longitude can be put in other arrays.
Below is the $GPGGA String, along with its description:
$GPGGA,104534.000,7791.0381,N,06727.4434,E,1,08,0.9,510.4,M,43.9,M,,*47
$GPGGA,HHMMSS.SSS,latitude,N,longitude,E,FQ,NOS,HDP,altitude,M,height,M,,checksum data
Identifier |
Description |
$GPGGA |
Global Positioning system fix data |
HHMMSS.SSS |
Time in hour minute seconds and milliseconds format. |
Latitude |
Latitude (Coordinate) |
N |
Direction N=North, S=South |
Longitude |
Longitude(Coordinate) |
E |
Direction E= East, W=West |
FQ |
Fix Quality Data |
NOS |
No. of Satellites being Used |
HPD |
Horizontal Dilution of Precision |
Altitude |
Altitude from sea level |
M |
Meter |
Height |
Height |
Checksum |
Checksum Data |
Circuit Explanation for Interfacing GSM and GPS with Arduino:
Circuit Connections of this Vehicle Tracking System Project is simple and is shown in the image belwo. Here Tx pin of GPS module is directly connected to digital pin number 10 of Arduino. By using Software Serial Library here, we have allowed serial communication on pin 10 and 11, and made them Rx and Tx respectively and left the Rx pin of GPS Module open. By default Pin 0 and 1 of Arduino are used for serial communication but by using SoftwareSerial library, we can allow serial communication on other digital pins of the Arduino. 12 Volt supply is used to power the GPS Module.
GSM module’s Tx and Rx pins of are directly connected to pin Rx and Tx of Arduino. GSM module is also powered by 12v supply. An optional LCD’s data pins D4, D5, D6 and D7 are connected to pin number 5, 4, 3, and 2 of Arduino. Command pin RS and EN of LCD are connected with pin number 2 and 3 of Arduino and RW pin is directly connected with ground. A Potentiometer is also used for setting contrast or brightness of LCD.
GSM and GPS based Vehicle Tracking system using Arduino - Working
In this project, Arduino is used for controlling whole the process with a GPS Receiver and GSM module. GPS Receiver is used for detecting coordinates of the vehicle, GSM module is used for sending the coordinates to user by SMS. And an optional 16x2 LCD is also used for displaying status messages or coordinates. We have used GPS Module SKG13BL and GSM Module SIM900A.
When we ready with our hardware after programming, we can install it in our vehicle and power it up. Then we just need to send a SMS, “Track Vehicle”, to the system that is placed in our vehicle. We can also use some prefix (#) or suffix (*) like #Track Vehicle*, to properly identify the starting and ending of the string, like we did in these projects: GSM Based Home Automation and Wireless Notice Board
Sent message is received by GSM module which is connected to the system and sends message data to Arduino. Arduino reads it and extract main message from the whole message. And then compare it with predefined message in Arduino. If any match occurs then Arduino reads coordinates by extracting $GPGGA String from GPS module data (GPS working explained above) and send it to user by using GSM module. This message contains the coordinates of vehicle location.
GAM and GPS Interfacing with Arduino Code to Track Vehicle Location
In programming part first we include libraries and define pins for LCD & software serial communication. Also define some variable with arrays for storing data. Software Serial Library is used to allow serial communication on pin 10 and 11.
#include<LiquidCrystal.h> LiquidCrystal lcd(7, 6, 5, 4, 3, 2); #include <SoftwareSerial.h> SoftwareSerial gps(10,11); // RX, TX char str[70]; String gpsString=""; ... .... .... ....
Here array str[70] is used for storing received message from GSM module and gpsString is used for storing GPS string. char *test=”$GPGGA” is used to compare the right string that we need for coordinates.
After it we have initialized serial communication, LCD, GSM & GPS module in setup function and showed a welcome message on LCD.
void setup() { lcd.begin(16,2); Serial.begin(9600); gps.begin(9600); lcd.print("Vehicle Tracking"); lcd.setCursor(0,1); ... .... .... ....
In loop function we receive message and GPS string.
void loop() { serialEvent(); if(temp) { get_gps(); tracking(); } }
Functions void init_sms and void send_sms() are used to initialising and sending message. Use proper 10 digit Cell phone no, in init_sms function.
Function void get_gps() has been used to extract the coordinates from the received string.
Function void gpsEvent() is used for receiving GPS data into the Arduino.
Function void serialEvent() is used for receiving message from GSM and comparing the received message with predefined message (Track Vehicle).
void serialEvent() { while(Serial.available()) { if(Serial.find("Track Vehicle")) { temp=1; break; } ... .... .... ...
Initialization function ‘gsm_init()’ is used for initialising and configuring the GSM Module, where firstly, GSM module is checked whether it is connected or not by sending ‘AT’ command to GSM module. If response OK is received, means it is ready. System keeps checking for the module until it becomes ready or until ‘OK’ is received. Then ECHO is turned off by sending the ATE0 command, otherwise GSM module will echo all the commands. Then finally Network availability is checked through the ‘AT+CPIN?’ command, if inserted card is SIM card and PIN is present, it gives the response +CPIN: READY. This is also check repeatedly until the network is found. This can be clearly understood by the Video below.
Check all the above functions in Code Section below.
Complete Project Code
#include<LiquidCrystal.h>
LiquidCrystal lcd(7, 6, 5, 4, 3, 2);
#include <SoftwareSerial.h>
SoftwareSerial gps(10,11); // RX, TX
//String str="";
char str[70];
String gpsString="";
char *test="$GPGGA";
String latitude="No Range ";
String longitude="No Range ";
int temp=0,i;
boolean gps_status=0;
void setup()
{
lcd.begin(16,2);
Serial.begin(9600);
gps.begin(9600);
lcd.print("Vehicle Tracking");
lcd.setCursor(0,1);
lcd.print(" System ");
delay(2000);
gsm_init();
lcd.clear();
Serial.println("AT+CNMI=2,2,0,0,0");
lcd.print("GPS Initializing");
lcd.setCursor(0,1);
lcd.print(" No GPS Range ");
get_gps();
delay(2000);
lcd.clear();
lcd.print("GPS Range Found");
lcd.setCursor(0,1);
lcd.print("GPS is Ready");
delay(2000);
lcd.clear();
lcd.print("System Ready");
temp=0;
}
void loop()
{
serialEvent();
if(temp)
{
get_gps();
tracking();
}
}
void serialEvent()
{
while(Serial.available())
{
if(Serial.find("Track Vehicle"))
{
temp=1;
break;
}
else
temp=0;
}
}
void gpsEvent()
{
gpsString="";
while(1)
{
while (gps.available()>0) //checking serial data from GPS
{
char inChar = (char)gps.read();
gpsString+= inChar; //store data from GPS into gpsString
i++;
if (i < 7)
{
if(gpsString[i-1] != test[i-1]) //checking for $GPGGA sentence
{
i=0;
gpsString="";
}
}
if(inChar=='\r')
{
if(i>65)
{
gps_status=1;
break;
}
else
{
i=0;
}
}
}
if(gps_status)
break;
}
}
void gsm_init()
{
lcd.clear();
lcd.print("Finding Module..");
boolean at_flag=1;
while(at_flag)
{
Serial.println("AT");
while(Serial.available()>0)
{
if(Serial.find("OK"))
at_flag=0;
}
delay(1000);
}
lcd.clear();
lcd.print("Module Connected..");
delay(1000);
lcd.clear();
lcd.print("Disabling ECHO");
boolean echo_flag=1;
while(echo_flag)
{
Serial.println("ATE0");
while(Serial.available()>0)
{
if(Serial.find("OK"))
echo_flag=0;
}
delay(1000);
}
lcd.clear();
lcd.print("Echo OFF");
delay(1000);
lcd.clear();
lcd.print("Finding Network..");
boolean net_flag=1;
while(net_flag)
{
Serial.println("AT+CPIN?");
while(Serial.available()>0)
{
if(Serial.find("+CPIN: READY"))
net_flag=0;
}
delay(1000);
}
lcd.clear();
lcd.print("Network Found..");
delay(1000);
lcd.clear();
}
void get_gps()
{
gps_status=0;
int x=0;
while(gps_status==0)
{
gpsEvent();
int str_lenth=i;
latitude="";
longitude="";
int comma=0;
while(x<str_lenth)
{
if(gpsString[x]==',')
comma++;
if(comma==2) //extract latitude from string
latitude+=gpsString[x+1];
else if(comma==4) //extract longitude from string
longitude+=gpsString[x+1];
x++;
}
int l1=latitude.length();
latitude[l1-1]=' ';
l1=longitude.length();
longitude[l1-1]=' ';
lcd.clear();
lcd.print("Lat:");
lcd.print(latitude);
lcd.setCursor(0,1);
lcd.print("Long:");
lcd.print(longitude);
i=0;x=0;
str_lenth=0;
delay(2000);
}
}
void init_sms()
{
Serial.println("AT+CMGF=1");
delay(400);
Serial.println("AT+CMGS=\"+91**********\""); // use your 10 digit cell no. here
delay(400);
}
void send_data(String message)
{
Serial.println(message);
delay(200);
}
void send_sms()
{
Serial.write(26);
}
void lcd_status()
{
lcd.clear();
lcd.print("Message Sent");
delay(2000);
lcd.clear();
lcd.print("System Ready");
return;
}
void tracking()
{
init_sms();
send_data("Vehicle Tracking Alert:");
send_data("Your Vehicle Current Location is:");
Serial.print("Latitude:");
send_data(latitude);
Serial.print("Longitude:");
send_data(longitude);
send_data("Please take some action soon..\nThankyou");
send_sms();
delay(2000);
lcd_status();
}
Comments
How to give 12 volt DC power
How to give 12 volt DC power supply to GPS and GSM
yeah adil this type of
yeah adil this type of problem has occured with me too
I do not know what i have mistaken....
No GPS Range Means your GPS
No GPS Range Means your GPS is not in getting signal from satellite.
you have to place your GPS receiver or GPS antenna outdoor(on the roof, window)..
I wanted to extract only
I wanted to extract only latitude and longitude and display them on lcd so i used this part of the code posted
#include<LiquidCrystal.h>
LiquidCrystal lcd(7, 6, 5, 4, 3, 2);
#include <SoftwareSerial.h>
SoftwareSerial gps(10,11); // RX, TX
//String str="";
//char str[70];
String gpsString="";
char *test="$GPGGA";
String latitude="No Range ";
String longitude="No Range ";
int temp=0,i;
boolean gps_status=0;
void setup()
{
lcd.begin(16,2);
Serial.begin(9600);
gps.begin(9600);
lcd.print("GPS Tracking");
lcd.setCursor(0,1);
lcd.print(" System ");
delay(2000);
//gsm_init();
lcd.clear();
//Serial.println("AT+CNMI=2,2,0,0,0");
lcd.print("GPS Initializing");
lcd.setCursor(0,1);
lcd.print(" No GPS Range ");
get_gps();
delay(2000);
lcd.clear();
lcd.print("GPS Range Found");
lcd.setCursor(0,1);
lcd.print("GPS is Ready");
delay(2000);
lcd.clear();
lcd.print("System Ready");
temp=0;
}
void loop()
{
//serialEvent();
if(temp)
{
get_gps();
//tracking();
}
}
void gpsEvent()
{
gpsString="";
while(1)
{
while (gps.available()>0) //checking serial data from GPS
{
char inChar = (char)gps.read();
gpsString+= inChar; //store data from GPS into gpsString
i++;
if (i < 7)
{
if(gpsString[i-1] != test[i-1]) //checking for $GPGGA sentence
{
i=0;
gpsString="";
}
}
if(inChar=='\r')
{
if(i>65)
{
gps_status=1;
break;
}
else
{
i=0;
}
}
}
if(gps_status)
break;
}
}
void get_gps()
{
gps_status=0;
int x=0;
while(gps_status==0)
{
gpsEvent();
int str_lenth=i;
latitude="";
longitude="";
int comma=0;
while(x<str_lenth)
{
if(gpsString[x]==',')
comma++;
if(comma==2) //extract latitude from string
latitude+=gpsString[x+1];
else if(comma==4) //extract longitude from string
longitude+=gpsString[x+1];
x++;
}
int l1=latitude.length();
latitude[l1-1]=' ';
l1=longitude.length();
longitude[l1-1]=' ';
lcd.clear();
lcd.print("Lat:");
lcd.print(latitude);
lcd.setCursor(0,1);
lcd.print("Long:");
lcd.print(longitude);
i=0;x=0;
str_lenth=0;
delay(2000);
}
}
latitude gives the wrong position
hello
i have run your code my arduino but it wrong latitude position and longtitude give write position
please help
why my GPS not blinking
why my GPS not blinking(status). on my LCD only 'Finding Module..' appear. i really count on you
Is it necessary that we use
Is it necessary that we use the same gps module specified by you?or can we use any other gps available in the market?
No it is not necessary but it
No it is not necessary but it will be helpful to you if you use the same
I know but I connected gps
I know but I connected gps module in gateway mode and it worked
it displayed the desired output as discussed in other post
but when i tried to do the same thing displaying it on lcd it is just stuck i mean how is it possible that one time same place is in range and other time it is not.....
Code worked previously but now it is not working...
Please help
Hi, im in school project, and
Hi, im in school project, and i need to get de gps cordenates and send it to a cellphone, im useing, de arduino uno and the SIM808 module, and my question is, how to use this module, is too hard to found examples of code with this module. thanks!
i used sim808 module.
i used sim808 module.
GSM part is working fine but its GPS power is not turning on by the command.
and command give a error.
please help me my project is
please help me my project is not working all item and circuit are same
Project related
Is. The project working properly because i have to make this for final year project
Which is the. Gps and gsm model pls reply as early as possible
@Darshan @nikhil: We have
@Darshan @nikhil: We have used GPS Module SKG13BL and GSM Module SIM900A, it is already mentioned.
Do you need a data plan for
Do you need a data plan for the GPS to get the coordinates? or an internet access of some sorts?
No you dont need Data plan
No you dont need Data plan and Internet, check this one How to Use GPS with Arduino
"NO GPS range" is stuck on the lcd.
Am using arduino uno and NEO-6m-0-001 (Gps) does this codes work for these models ?
hlp me plz..........
Yes, it should work. Check
Yes, it should work. Check the Tx, Rx and other pins of your model and connect accordingly.
Thanks for Ur Support , en
Thanks for Ur Support , en another thing is that my GSM module is SIM900 is it oky to use them with the codes above ?, I hv tried but it seems the execution of method gsm_init() isn't working .........
How should I make it work ?
Plz I need Ur help.........
After configuration of
After configuration of everything my LCD only displays "Vehicle Tracking System "
Hi. I have tried out this
Hi. I have tried out this project with sim800l. The lcd works properly and displays that 'network has been found' plus the 'longitude' and 'latitude'.
But when I send the text Track Vehicle, I get no reply.
Can you please help me to solve this???
IF you have Joined together
IF you have Joined together the by putting a GSM Module ontop of Arduino Possible cause may be that the GSM Module has failed to send the message due to lower current since transmission requires much current , try using external power adaptor of 12V and connect it directly to the GSM.........,
In the initial codes try to replace the serialevent() method with this one
void serialEvent()
{
while(Serial.available())
{
if(Serial.find("Track"))
{
lcd.clear();
lcd.println("Message Received");
temp=1;
break;
}
else
temp=0;
}
}
if the "Track Vehicle" is received and read You will see the LCD , and it will prove that its a transmission problem (sendind of the SMS)
My LCD keeps displaying
My LCD keeps displaying Finding Module............. and doesn't go beyond that , what is the Problem actually ?
I have come to notice that
I have come to notice that after the configuartion of the circuit is compete and I uploaded the codes,
My GSM Module doesn't Give the response to the AT commands sent so I keep seeing ATATATATATATATA....... on my Serial monitor so I was asking whats wrong ?, is it the codes or the connection that has a problem ?
Help me Plz its my Final Year project and the days are numbered.......
From Your Video it shows Your
From Your Video it shows Your codes and connection works just fine.....how do I configure my Modem using Hardware Serial and how my codes should be to work fine like Yours ? because its like the Response that GSM module is required to provide are not available , when I open the Serial Monitor I keep seeing ATATATATAT.......
when I try to write OK it goes to the next execution ........,
I don't knw whats wrong en I hv stayed For days troubleshooting any one with the knowledge will You Please help me.........
Even if its by sending the working Codes I will greatly appreciate it
PROBLEM SOLVED.........!!!!!!
PROBLEM SOLVED.........!!!!!!!!!!!!!!!!! For all the People who has the same Problem as mine Just make Sure that your Hardware serial Communication is Configured Properly(Connection between GSM Module and ARDUINO)
i.e The serial monitor repeatedly dispays ATATATATATAT............. Because the GSM Module doesn't Respond meaning that thehardware Serial connection has failed ....
Man thanx 4 the codes .It realy Helped me...........
lcd screen only show "finding module"
how exactly i gonna retify it..please help me sir...
error in uploading to ardino
Initailly even I got the same message on serial monitor i.e ATATATA....
later the code isn't uploading to the ardino board!(avrdude..error relating to port 0)...Could you please tell the changes to be made in the code and the circuit connections.
Thanks in advance
hi amir
hi amir
i am finding same problem so can you help me how to configure gsm module properlly
mail me: makwanavijay10000@gmail.com
is it necesary to supply 12V
is it necesary to supply 12V to GPS module
@Amir Saidi..
@Amir Saidi..
I have replaced the serial event method with
this one
void serialEvent()
{
while(Serial.available())
{
if(Serial.find("Track"))
{
lcd.clear();
lcd.println("Message Received");
temp=1;
break;
}
else
temp=0;
}
}
But the lcd stil does not display Message Received nor gsm sending any text to my phone. What else can I try? Anybody who can help me solve this problem???
#Amir Said thank you for your
#Amir Said thank you for your reply. I managed to receive the message after increasing the voltage.
@Elvin and @Amir : Glad to
@Elvin and @Amir : Glad to know, that you finally resolved your issues with the Project.
Guys does anyone know how to
Guys does anyone know how to change the code so as to receive the longitude and latitude data in degrees format alone without the minutes?
You can easily do with some
You can easily do with some programming like using 'round' function round(longitude/100) or by applying some other logic to extract the first two digits (degrees).
in the same project i dont
in the same project i dont want LCD display . i want the latitude and longitude once in 5minutes periodically ,please help me to finish my project.
No GPS found: Problem solved
The Tx pin of GPS must connect to pin 10 of arduino owing to software serial (10,11) (Rx,Tx)
Erros
Hi,
I have these errors, can you please resolve them. I have used the same code as define by the author.
sketch\test.cpp:8:12: warning: deprecated conversion from string constant to 'char*' [-Wwrite-strings]
char *test="$GPGGA";
^
test.cpp:12: error: 'boolean' does not name a type
boolean gps_status=0;
^
sketch\test.cpp: In function 'void setup()':
test.cpp:16: error: 'Serial' was not declared in this scope
Serial.begin(9600);
^
test.cpp:21: error: 'delay' was not declared in this scope
delay(2000);
^
test.cpp:22: error: 'gsm_init' was not declared in this scope
gsm_init();
^
test.cpp:28: error: 'get_gps' was not declared in this scope
get_gps();
^
sketch\test.cpp: In function 'void loop()':
test.cpp:41: error: 'serialEvent' was not declared in this scope
serialEvent();
^
test.cpp:44: error: 'get_gps' was not declared in this scope
get_gps();
^
test.cpp:45: error: 'tracking' was not declared in this scope
tracking();
^
sketch\test.cpp: In function 'void serialEvent()':
test.cpp:50: error: 'Serial' was not declared in this scope
while(Serial.available())
^
sketch\test.cpp: In function 'void gpsEvent()':
test.cpp:83: error: 'gps_status' was not declared in this scope
gps_status=1;
^
test.cpp:92: error: 'gps_status' was not declared in this scope
if(gps_status)
^
sketch\test.cpp: In function 'void gsm_init()':
test.cpp:100: error: 'boolean' was not declared in this scope
boolean at_flag=1;
^
test.cpp:100: error: expected ';' before 'at_flag'
boolean at_flag=1;
^
test.cpp:101: error: 'at_flag' was not declared in this scope
while(at_flag)
^
test.cpp:103: error: 'Serial' was not declared in this scope
Serial.println("AT");
^
test.cpp:110: error: 'delay' was not declared in this scope
delay(1000);
^
test.cpp:114: error: 'delay' was not declared in this scope
delay(1000);
^
test.cpp:117: error: expected ';' before 'echo_flag'
boolean echo_flag=1;
^
test.cpp:118: error: 'echo_flag' was not declared in this scope
while(echo_flag)
^
test.cpp:120: error: 'Serial' was not declared in this scope
Serial.println("ATE0");
^
test.cpp:133: error: expected ';' before 'net_flag'
boolean net_flag=1;
^
test.cpp:134: error: 'net_flag' was not declared in this scope
while(net_flag)
^
test.cpp:136: error: 'Serial' was not declared in this scope
Serial.println("AT+CPIN?");
^
sketch\test.cpp: In function 'void get_gps()':
test.cpp:151: error: 'gps_status' was not declared in this scope
gps_status=0;
^
test.cpp:182: error: 'delay' was not declared in this scope
delay(2000);
^
sketch\test.cpp: In function 'void init_sms()':
test.cpp:187: error: 'Serial' was not declared in this scope
Serial.println("AT+CMGF=1");
^
test.cpp:188: error: 'delay' was not declared in this scope
delay(400);
^
sketch\test.cpp: In function 'void send_data(String)':
test.cpp:194: error: 'Serial' was not declared in this scope
Serial.println(message);
^
test.cpp:195: error: 'delay' was not declared in this scope
delay(200);
^
sketch\test.cpp: In function 'void send_sms()':
test.cpp:199: error: 'Serial' was not declared in this scope
Serial.write(26);
^
sketch\test.cpp: In function 'void lcd_status()':
test.cpp:205: error: 'delay' was not declared in this scope
delay(2000);
^
sketch\test.cpp: In function 'void tracking()':
test.cpp:215: error: 'Serial' was not declared in this scope
Serial.print("Latitude:");
^
test.cpp:221: error: 'delay' was not declared in this scope
delay(2000);
^
exit status 1
'boolean' does not name a type
Invalid library found in C:\Program Files (x86)\Arduino\libraries\test: C:\Program Files (x86)\Arduino\libraries\test
Invalid library found in C:\Program Files (x86)\Arduino\libraries\test: C:\Program Files (x86)\Arduino\libraries\test
This report would have more information with
"Show verbose output during compilation"
option enabled in File -> Preferences.
this will read gsm output
this will read gsm output string and find OK substring or word comming from GSM response. and if matched with OK then set at_flag
how can i do this my simulation
hello guys i wish to know how to do this using proteus and arduino
what are the libraries to included in proteus and arduino and incorporating the gsm mudule and the pgrs/gps mudules
i am getting problem in above project
my lcd stops on finding module..
and serial window shows AT AT AT...
hi I wanted to know how do to
hi I wanted to know how do to communicate with arduino wassap or so already in code
please help i power my sim
please help i power my sim 800l with arduino uno 5volt on board using a usb port of my laptop,everything went fine but when i send track vehicle, i got no respond.i read sim800l datasheet which says it draw up to 2A of current.my sim800l works with all AT command. but doesnt respond to sms command.please what kind of power should i use to power my sim800l? thanks in advance
please admin,please power a
please admin,please power a 12volt battery charger with auto cut off of with lcd display...no post of power supply,regulator and battery charger or solar...please see into that
after powering the sim800l
after powering the sim800l with 3.7volt lithium battery,i was able to dial the number in the module and its rings.but when i send message(Track Vehicle) to the module,it only receive the message but nothing was sent back to the number i put in the programming...pls how to i test if a sim800l is working with sms recieving and sending sms using arduino...please urgent feedback is needed..
for those having issue this
for those having issue this project and u have the same hardware as mine. here is the solution....
hardware: gps:NEO-6m-0-001
hardware: gps:NEO-6m-0-001 (Gps), and arduino(any type)....make sure your the gps TX is connected to pin10 of the arduino and the RX is left unconnected.... gsm sim800l...i power my sim800l with a full charger power bank battery......Lithium Ion 18600 3.7v Battery... since the sim800l data sheet says it need power of 3.7v-4.2volt and draw current of up to 2A.. after all connection are done.here is the code i used..
#include<LiquidCrystal.h>
#include<LiquidCrystal.h>
#include <SoftwareSerial.h>
LiquidCrystal lcd(7, 6, 5, 4, 3, 2);
SoftwareSerial gps(10, 11); // RX, Tx
int led = 13;
int l = 0, x = 0, k = 0;
char str1[100], msg[32];
//String str="";
char str[70];
String gpsString = "";
char *test = "$GPGGA";
String latitude = "No Range ";
String longitude = "No Range ";
int temp = 0, i;
boolean gps_status = 0;
void setup()
{
lcd.begin(16, 2);
Serial.begin(9600);
gps.begin(9600);
lcd.print("Vehicle Tracking");
lcd.setCursor(0, 1);
lcd.print(" System ");
delay(2000);
gsm_init();
lcd.clear();
Serial.println("AT+CNMI=2,2,0,0,0");
delay(500);
Serial.println("AT+CMGF=1");
lcd.print("GPS Initializing");
lcd.setCursor(0, 1);
lcd.print(" No GPS Range ");
get_gps();
delay(500);
lcd.clear();
lcd.print("GPS Range Found");
lcd.setCursor(0, 1);
lcd.print("GPS is Ready");
delay(500);
lcd.clear();
lcd.print("System Ready");
digitalWrite(led, LOW);
}
void loop()
{
for (unsigned int t = 0; t < 60000; t++)
{
serialEvent();
if (temp == 1)
{
x = 0, k = 0, temp = 0;
while (x < l)
{
while (str1[x] == '#')
{
x++;
while (str1[x] != '*')
{
msg[k++] = str1[x++];
}
}
x++;
}
get_gps();
tracking();
msg[k] = '\0';
lcd.clear();
lcd.print(msg);
delay(1000);
temp = 0;
l = 0;
x = 0;
k = 0;
}
}
lcd.scrollDisplayLeft();
Serial.println("AT+CMGD=1,4"); // delete all SMS
}
void serialEvent()
{
while (Serial.available())
{
char ch = (char)Serial.read();
str1[l++] = ch;
if (ch == '*')
{
temp = 1;
lcd.clear();
lcd.print("Message Received");
delay(1000);
}
}
}
void gsm_init()
{
lcd.clear();
lcd.print("Finding Module..");
boolean at_flag = 1;
while (at_flag)
{
Serial.println("AT");
while (Serial.available() > 0)
{
if (Serial.find("OK"))
at_flag = 0;
}
delay(1000);
}
lcd.clear();
lcd.print("Module Connected..");
delay(1000);
lcd.clear();
lcd.print("Disabling ECHO");
boolean echo_flag = 1;
while (echo_flag)
{
Serial.println("ATE0");
while (Serial.available() > 0)
{
if (Serial.find("OK"))
echo_flag = 0;
}
delay(1000);
}
lcd.clear();
lcd.print("Echo OFF");
delay(1000);
lcd.clear();
lcd.print("Finding Network..");
boolean net_flag = 1;
while (net_flag)
{
Serial.println("AT+CPIN?");
while (Serial.available() > 0)
{
if (Serial.find("+CPIN: READY"))
net_flag = 0;
}
delay(1000);
}
lcd.clear();
lcd.print("Network Found..");
delay(1000);
lcd.clear();
}
void gpsEvent()
{
gpsString = "";
while (1)
{
while (gps.available() > 0) //checking serial data from GPS
{
char inChar = (char)gps.read();
gpsString += inChar; //store data from GPS into gpsString
i++;
if (i < 7)
{
if (gpsString[i - 1] != test[i - 1]) //checking for $GPGGA sentence
{
i = 0;
gpsString = "";
}
}
if (inChar == '\r')
{
if (i > 65)
{
gps_status = 1;
break;
}
else
{
i = 0;
}
}
}
if (gps_status)
break;
}
}
void get_gps()
{
gps_status = 0;
int x = 0;
while (gps_status == 0)
{
gpsEvent();
int str_lenth = i;
latitude = "";
longitude = "";
int comma = 0;
while (x < str_lenth)
{
if (gpsString[x] == ',')
comma++;
if (comma == 2) //extract latitude from string
latitude += gpsString[x + 1];
else if (comma == 4) //extract longitude from string
longitude += gpsString[x + 1];
x++;
}
int l1 = latitude.length();
latitude[l1 - 1] = ' ';
l1 = longitude.length();
longitude[l1 - 1] = ' ';
lcd.clear();
lcd.print("Lat:");
lcd.print(latitude);
lcd.setCursor(0, 1);
lcd.print("Long:");
lcd.print(longitude);
i = 0; x = 0;
str_lenth = 0;
delay(10000);
}
}
void tracking()
{
Serial.println("AT+CMGF=1"); //Sets the GSM Module in Text Mode
delay(1000); // Delay of 1000 milli seconds or 1 second
Serial.println("AT+CMGS=\"+2349051146346\""); // Replace x with mobile number
delay(100);
delay(1000);
Serial.println("Vehicle Tracking Alert:");
Serial.print("Your Vehicle Current Location is:");
Serial.print("Latitude:");
Serial.print(latitude);
Serial.print("Longitude:");
Serial.print(longitude);
Serial.print("Please take some action soon..\nThankyou");
delay(100);
Serial.write(26);// ASCII code of CTRL+Z
delay(1000);
lcd.clear();
lcd.print("Message Sent");
delay(2000);
lcd.clear();
lcd.print("System Ready");
//return;
}
errors in code could u please help me
Arduino: 1.8.1 (Windows XP), Board: "Arduino/Genuino Uno"
C:\Documents and Settings\sys\My Documents\Arduino\sketch_feb20c\sketch_feb20c.ino:11:14: warning: deprecated conversion from string constant to 'char*' [-Wwrite-strings]
char *test = "$GPGGA";
^
C:\Documents and Settings\sys\My Documents\Arduino\sketch_feb20c\sketch_feb20c.ino: In function 'void gsm_init()':
C:\Documents and Settings\sys\My Documents\Arduino\sketch_feb20c\sketch_feb20c.ino:104:27: warning: deprecated conversion from string constant to 'char*' [-Wwrite-strings]
if (Serial.find("OK"))
^
C:\Documents and Settings\sys\My Documents\Arduino\sketch_feb20c\sketch_feb20c.ino:120:27: warning: deprecated conversion from string constant to 'char*' [-Wwrite-strings]
if (Serial.find("OK"))
^
C:\Documents and Settings\sys\My Documents\Arduino\sketch_feb20c\sketch_feb20c.ino:136:37: warning: deprecated conversion from string constant to 'char*' [-Wwrite-strings]
if (Serial.find("+CPIN: READY"))
^
Sketch uses 8162 bytes (25%) of program storage space. Maximum is 32256 bytes.
Global variables use 1003 bytes (48%) of dynamic memory, leaving 1045 bytes for local variables. Maximum is 2048 bytes.
avrdude: ser_open(): can't set com-state for "\\.\COM3"
An error occurred while uploading the sketch
This report would have more information with
"Show verbose output during compilation"
option enabled in File -> Preferences.
coding of gps and gsm
Dear sir
I need a code of gps and gsm in which the data of gps is send to the mobile number through gsm continously.
Can you please help me for that.
thank you
regards
sumant mishra
during my study of the gsm
during my study of the gsm module i use, i found that my sim800l shutdown when i use serial.find(''Track Vehicle''); so i use the wireless notices board code in the other post and it work fine, so i just have to swap and do some modification to the existing code and it work..command to send is
#anything u like* example;
#track*
#track vehicle*
could you please send the
could you please send the whole code that is working properly
after the connection my gps
after the connection my gps was blinking while my sim800L is nt showing any light and in my serial monitor it is showing ATATATATAT....... PLS what should i do nw
thanks to admin for the great
thanks to admin for the great work being done..
Hi Salufu,
Hi Salufu,
Glad to know that your setup finally worked!
Thanks
nature of reply message
hi everyone. please is it possible to make the reply message to your phone a link to google maps showing the location of the car? if possible please how? thanks a lot.
Yes, you can, you need to
Yes, you can, you need to append the Lat and Long (separated by comma) to this URL:http://maps.google.com/maps?&z=15&mrt=yp&t=k&q= and send it on Cellphone uisng GSM. Check this article for more info: Track A Vehicle on Google Maps using Arduino, ESP8266 & GPS
Hi everyone , I m sending
Hi everyone , I m sending message from mobile ....... but not getting response on the mobile from the GSM module i.e the value of " lat" . and "long"....please help me out
Try using the code provided
Try using the code provided by user 'salufu' in above comments, he has successfully done it.
hello , can i use other gps
hello , can i use other gps module ? anyone ?
Yes, check the datasheet of
Yes, check the datasheet of your GPS before connecting it.
information
hi .... everyone I have GPS-GSM Module (SARA-G350) i used the first code but it doesnt work thé module give not a réponse please can you help me
@Muhammad and @mark: Yes you
@Muhammad and @mark: Yes you can use any GPS but check the datasheet of it before connecting it. Also you can use this procedure to first properly interface it with Arduino: How to Use GPS with Arduino
can you help me please
hi i am from Ecuador use the GPS module neo Neo 6 - m and 900 sim Hield but I made the connection and load the code but it does not work me out on serial port only the command AT
Check your connections and
Check your connections and code again. Further echo is disabled otherwise it will print all the given AT commands on serial monitor.
please urgent project is to race
that such a shield for 900 gsm sim I need other libraries or if it works with libraries that are in the code
You dont need to install any
You dont need to install any Library for GSM, we just need to serially send and receive the data from the GSM module using the Rx and Tx pin. Further SoftwareSerial.h library is enough to change the Default Rx Tx pins of Arduino, which we have used.
Arduino Real Time Vehicle Tracking System Using GPRS & GSM
Sir, this is my final year project, I have got the Arduino UNO R3, GPRS/GSM Shield SIM900 Ver 2.0
I am little confused with my components, My following doubts are,
1. In my GPRS Shield SIM900 there is a in built antenna, what's the purpose of the antenna?
2. is it nesessary to add a GPS module for this project?, if so please recommend a suitable one?
3. But in my Shield i don't have no idea where to plug the GPS module?
Your help would be highly appreciated!
Thank you.
Antenna is there in all the
Antenna is there in all the GSM modules, and you can use any GPS module or can use GPS Module SKG13 which we have used in this project.
is it OK to use trimmers 10k
is it OK to use trimmers 10k or high precision 3296w 10 k ? because their is no available 10k pot
hello.....anyone can help me
hello.....anyone can help me to do this project for my final year project?
for those that still
for those that still encounter problem in setting up this circuit to work, double check your connection very well and if its failed to work check the previous page and use the modified code i use for my own circuit
nurul this project can be use
nurul this project can be use for final year project but use just have to make sure you are not having any issue with the connection between the hardware. also make sure you check your hardware datasheet expecially the gsm and the gps for proper power connection
the issue i had then was that
the issue i had then was that my sim800l doesnt work with ''serial.find''
while(Serial.available())
{
if(Serial.find("#A."))
{
digitalWrite(led, HIGH);
delay(1000);
digitalWrite(led, LOW);
while (Serial.available())
{
char inChar=Serial.read();
str[i++]=inChar;
if(inChar=='*')
{
temp=1;
return;
} so i tried use other method like the while loop
serialEvent();
if (temp == 1)
{
x = 0, k = 0, temp = 0;
while (x < l)
{
while (str1[x] == '#')
{
x++;
while (str1[x] != '*')
{
msg[k++] = str1[x++];
}
}
x++;
}
so check whether your gsm works with the serial.find or the while loop
#include<LiquidCrystal.h>
#include<LiquidCrystal.h>
#include <SoftwareSerial.h>
LiquidCrystal lcd(7, 6, 5, 4, 3, 2);
SoftwareSerial gps(10, 11); // RX, Tx connect the gps tx to pin10 and leave the gps RX unconnected
int led = 13;
int l = 0, x = 0, k = 0;
char str1[100], msg[32];
//String str="";
char str[70];
String gpsString = "";
char *test = "$GPGGA";
String latitude = "No Range ";
String longitude = "No Range ";
int temp = 0, i;
boolean gps_status = 0;
void setup()
{
lcd.begin(16, 2);
Serial.begin(9600);
gps.begin(9600);
lcd.print("Vehicle Tracking");
lcd.setCursor(0, 1);
lcd.print(" System ");
delay(2000);
gsm_init();
lcd.clear();
Serial.println("AT+CNMI=2,2,0,0,0");
delay(500);
Serial.println("AT+CMGF=1");
lcd.print("GPS Initializing");
lcd.setCursor(0, 1);
lcd.print(" No GPS Range ");
get_gps();
delay(500);
lcd.clear();
lcd.print("GPS Range Found");
lcd.setCursor(0, 1);
lcd.print("GPS is Ready");
delay(500);
lcd.clear();
lcd.print("System Ready");
digitalWrite(led, LOW);
}
void loop()
{
for (unsigned int t = 0; t < 60000; t++)
{
serialEvent();
if (temp == 1)
{
x = 0, k = 0, temp = 0;
while (x < l)
{
while (str1[x] == '#')
{
x++;
while (str1[x] != '*')
{
msg[k++] = str1[x++];
}
}
x++;
}
get_gps();
tracking();
msg[k] = '\0';
lcd.clear();
lcd.print(msg);
delay(1000);
temp = 0;
l = 0;
x = 0;
k = 0;
}
}
// lcd.scrollDisplayLeft();
Serial.println("AT+CMGD=1,4"); // delete all SMS
}
void serialEvent()
{
while (Serial.available())
{
char ch = (char)Serial.read();
str1[l++] = ch;
if (ch == '*')
{
temp = 1;
lcd.clear();
lcd.print("Message Received");
delay(1000);
}
}
}
void gsm_init()
{
lcd.clear();
lcd.print("Finding Module..");
boolean at_flag = 1;
while (at_flag)
{
Serial.println("AT");
while (Serial.available() > 0)
{
if (Serial.find("OK"))
at_flag = 0;
}
delay(1000);
}
lcd.clear();
lcd.print("Module Connected..");
delay(1000);
lcd.clear();
lcd.print("Disabling ECHO");
boolean echo_flag = 1;
while (echo_flag)
{
Serial.println("ATE0");
while (Serial.available() > 0)
{
if (Serial.find("OK"))
echo_flag = 0;
}
delay(1000);
}
lcd.clear();
lcd.print("Echo OFF");
delay(1000);
lcd.clear();
lcd.print("Finding Network..");
boolean net_flag = 1;
while (net_flag)
{
Serial.println("AT+CPIN?");
while (Serial.available() > 0)
{
if (Serial.find("+CPIN: READY"))
net_flag = 0;
}
delay(1000);
}
lcd.clear();
lcd.print("Network Found..");
delay(1000);
lcd.clear();
}
void gpsEvent()
{
gpsString = "";
while (1)
{
while (gps.available() > 0) //checking serial data from GPS
{
char inChar = (char)gps.read();
gpsString += inChar; //store data from GPS into gpsString
i++;
if (i < 7)
{
if (gpsString[i - 1] != test[i - 1]) //checking for $GPGGA sentence
{
i = 0;
gpsString = "";
}
}
if (inChar == '\r')
{
if (i > 65)
{
gps_status = 1;
break;
}
else
{
i = 0;
}
}
}
if (gps_status)
break;
}
}
void get_gps()
{
gps_status = 0;
int x = 0;
while (gps_status == 0)
{
gpsEvent();
int str_lenth = i;
latitude = "";
longitude = "";
int comma = 0;
while (x < str_lenth)
{
if (gpsString[x] == ',')
comma++;
if (comma == 2) //extract latitude from string
latitude += gpsString[x + 1];
else if (comma == 4) //extract longitude from string
longitude += gpsString[x + 1];
x++;
}
int l1 = latitude.length();
latitude[l1 - 1] = ' ';
l1 = longitude.length();
longitude[l1 - 1] = ' ';
lcd.clear();
lcd.print("Lat:");
lcd.print(latitude);
lcd.setCursor(0, 1);
lcd.print("Long:");
lcd.print(longitude);
i = 0; x = 0;
str_lenth = 0;
delay(10000);
}
}
void tracking()
{
Serial.println("AT+CMGF=1"); //Sets the GSM Module in Text Mode
delay(1000); // Delay of 1000 milli seconds or 1 second
Serial.println("AT+CMGS=\"+xxxxxxxxxxxxxx\""); // Replace x with your country mobile number
delay(100);
delay(1000);
Serial.println("Vehicle Tracking Alert:");
Serial.print("Your Vehicle Current Location is:");
Serial.print("Latitude:");
Serial.print(latitude);
Serial.print("Longitude:");
Serial.print(longitude);
Serial.print("Please take some action soon..\nThankyou");
delay(100);
Serial.write(26);// ASCII code of CTRL+Z
delay(1000);
lcd.clear();
lcd.print("Message Sent");
delay(2000);
lcd.clear();
lcd.print("System Ready");
//return;
}
how to simulate this design in proteus?