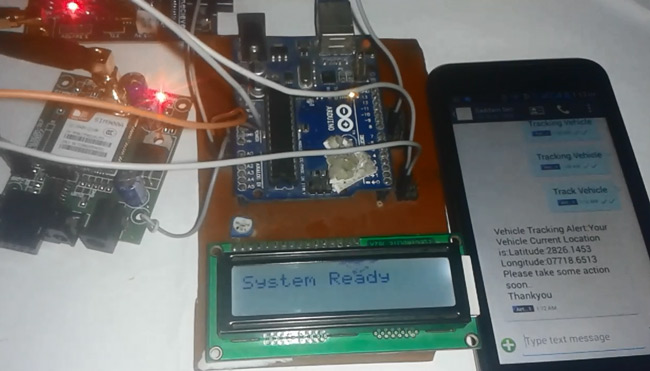
Vehicle Tracking systems are very commonly used in fleet management and asset tracking applications. Today these systems can not only track the location of the vehicle but can also report the speed and even control it remotely. In general, tracking of vehicles is a process in which we track the vehicle location in form of Latitude and Longitude (GPS coordinates). GPS Coordinates are the value of a location. This system is very efficient for outdoor application purposes. This kind of Vehicle Tracking System Project is widely in tracking Cabs/Taxis, stolen vehicles, school/college buses, etc.In this project, we are going one step ahead with GPS building a GSM and GPS based vehicle tracking system using Arduino. This Vehicle Tracking System can also be used to track a vehicle using GPS and GSM and can also be used as Accident Detection Alert System, Soldier Tracking System and many more, by just making few changes in hardware and software.
We have also build many other types of vehicle tracking systems previously, you can check them out if interested
- GPS Vehicle Tracking and Accident Alert using Arduino
- Vehicle Tracking with Google Maps using Arduino and ESP8266
- GPS Vehicle Tracking and Accident Alert using MSP430
- LoRa based GPS Vehicle Tracking using Arduino
- Location Tracker without GPS using SIM800 and Arduino
Components Required for Arduino based Vehicle Tracking system:
To build a simple vehicle tracking system suing Arduino we will need the following components.
- Arduino UNO
- GSM Module
- GPS Module
- 16x2 LCD
- Power Supply
- Connecting Wires
- 10 K POT
How can GSM Module be used to track location:
GPS stands for Global Positioning System and used to detect the Latitude and Longitude of any location on the Earth, with exact UTC time (Universal Time Coordinated). GPS module is the main component in our vehicle tracking system project. This device receives the coordinates from the satellite for each and every second, with time and date.
GPS module sends the data related to tracking position in real time, and it sends so many data in NMEA format (see the screenshot below). NMEA format consist several sentences, in which we only need one sentence. This sentence starts from $GPGGA and contains the coordinates, time and other useful information. This GPGGA is referred to Global Positioning System Fix Data. Know more about Reading GPS data and its strings here.
We can extract coordinate from $GPGGA string by counting the commas in the string. Suppose you find $GPGGA string and stores it in an array, then Latitude can be found after two commas and Longitude can be found after four commas. Now these latitude and longitude can be put in other arrays.
Below is the $GPGGA String, along with its description:
$GPGGA,104534.000,7791.0381,N,06727.4434,E,1,08,0.9,510.4,M,43.9,M,,*47
$GPGGA,HHMMSS.SSS,latitude,N,longitude,E,FQ,NOS,HDP,altitude,M,height,M,,checksum data
Identifier | Description |
$GPGGA | Global Positioning system fix data |
HHMMSS.SSS | Time in hour minute seconds and milliseconds format. |
Latitude | Latitude (Coordinate) |
N | Direction N=North, S=South |
Longitude | Longitude(Coordinate) |
E | Direction E= East, W=West |
FQ | Fix Quality Data |
NOS | No. of Satellites being Used |
HPD | Horizontal Dilution of Precision |
Altitude | Altitude from sea level |
M | Meter |
Height | Height |
Checksum | Checksum Data |
Circuit Explanation for Interfacing GSM and GPS with Arduino:
Circuit Connections of this Vehicle Tracking System Project is simple and is shown in the image belwo. Here Tx pin of GPS module is directly connected to digital pin number 10 of Arduino. By using Software Serial Library here, we have allowed serial communication on pin 10 and 11, and made them Rx and Tx respectively and left the Rx pin of GPS Module open. By default Pin 0 and 1 of Arduino are used for serial communication but by using SoftwareSerial library, we can allow serial communication on other digital pins of the Arduino. 12 Volt supply is used to power the GPS Module.
GSM module’s Tx and Rx pins of are directly connected to pin Rx and Tx of Arduino. GSM module is also powered by 12v supply. An optional LCD’s data pins D4, D5, D6 and D7 are connected to pin number 5, 4, 3, and 2 of Arduino. Command pin RS and EN of LCD are connected with pin number 2 and 3 of Arduino and RW pin is directly connected with ground. A Potentiometer is also used for setting contrast or brightness of LCD.
GSM and GPS based Vehicle Tracking system using Arduino - Working
In this project, Arduino is used for controlling whole the process with a GPS Receiver and GSM module. GPS Receiver is used for detecting coordinates of the vehicle, GSM module is used for sending the coordinates to user by SMS. And an optional 16x2 LCD is also used for displaying status messages or coordinates. We have used GPS Module SKG13BL and GSM Module SIM900A.
When we ready with our hardware after programming, we can install it in our vehicle and power it up. Then we just need to send a SMS, “Track Vehicle”, to the system that is placed in our vehicle. We can also use some prefix (#) or suffix (*) like #Track Vehicle*, to properly identify the starting and ending of the string, like we did in these projects: GSM Based Home Automation and Wireless Notice Board
Sent message is received by GSM module which is connected to the system and sends message data to Arduino. Arduino reads it and extract main message from the whole message. And then compare it with predefined message in Arduino. If any match occurs then Arduino reads coordinates by extracting $GPGGA String from GPS module data (GPS working explained above) and send it to user by using GSM module. This message contains the coordinates of vehicle location.
GAM and GPS Interfacing with Arduino Code to Track Vehicle Location
In programming part first we include libraries and define pins for LCD & software serial communication. Also define some variable with arrays for storing data. Software Serial Library is used to allow serial communication on pin 10 and 11.
#include<LiquidCrystal.h> LiquidCrystal lcd(7, 6, 5, 4, 3, 2); #include <SoftwareSerial.h> SoftwareSerial gps(10,11); // RX, TX char str[70]; String gpsString=""; ... .... .... ....
Here array str[70] is used for storing received message from GSM module and gpsString is used for storing GPS string. char *test=”$GPGGA” is used to compare the right string that we need for coordinates.
After it we have initialized serial communication, LCD, GSM & GPS module in setup function and showed a welcome message on LCD.
void setup() { lcd.begin(16,2); Serial.begin(9600); gps.begin(9600); lcd.print("Vehicle Tracking"); lcd.setCursor(0,1); ... .... .... ....
In loop function we receive message and GPS string.
void loop() { serialEvent(); if(temp) { get_gps(); tracking(); } }
Functions void init_sms and void send_sms() are used to initialising and sending message. Use proper 10 digit Cell phone no, in init_sms function.
Function void get_gps() has been used to extract the coordinates from the received string.
Function void gpsEvent() is used for receiving GPS data into the Arduino.
Function void serialEvent() is used for receiving message from GSM and comparing the received message with predefined message (Track Vehicle).
void serialEvent() { while(Serial.available()) { if(Serial.find("Track Vehicle")) { temp=1; break; } ... .... .... ...
Initialization function ‘gsm_init()’ is used for initialising and configuring the GSM Module, where firstly, GSM module is checked whether it is connected or not by sending ‘AT’ command to GSM module. If response OK is received, means it is ready. System keeps checking for the module until it becomes ready or until ‘OK’ is received. Then ECHO is turned off by sending the ATE0 command, otherwise GSM module will echo all the commands. Then finally Network availability is checked through the ‘AT+CPIN?’ command, if inserted card is SIM card and PIN is present, it gives the response +CPIN: READY. This is also check repeatedly until the network is found. This can be clearly understood by the Video below.
Check all the above functions in Code Section below.
Complete Project Code
#include<LiquidCrystal.h>
LiquidCrystal lcd(7, 6, 5, 4, 3, 2);
#include <SoftwareSerial.h>
SoftwareSerial gps(10,11); // RX, TX
//String str="";
char str[70];
String gpsString="";
char *test="$GPGGA";
String latitude="No Range ";
String longitude="No Range ";
int temp=0,i;
boolean gps_status=0;
void setup()
{
lcd.begin(16,2);
Serial.begin(9600);
gps.begin(9600);
lcd.print("Vehicle Tracking");
lcd.setCursor(0,1);
lcd.print(" System ");
delay(2000);
gsm_init();
lcd.clear();
Serial.println("AT+CNMI=2,2,0,0,0");
lcd.print("GPS Initializing");
lcd.setCursor(0,1);
lcd.print(" No GPS Range ");
get_gps();
delay(2000);
lcd.clear();
lcd.print("GPS Range Found");
lcd.setCursor(0,1);
lcd.print("GPS is Ready");
delay(2000);
lcd.clear();
lcd.print("System Ready");
temp=0;
}
void loop()
{
serialEvent();
if(temp)
{
get_gps();
tracking();
}
}
void serialEvent()
{
while(Serial.available())
{
if(Serial.find("Track Vehicle"))
{
temp=1;
break;
}
else
temp=0;
}
}
void gpsEvent()
{
gpsString="";
while(1)
{
while (gps.available()>0) //checking serial data from GPS
{
char inChar = (char)gps.read();
gpsString+= inChar; //store data from GPS into gpsString
i++;
if (i < 7)
{
if(gpsString[i-1] != test[i-1]) //checking for $GPGGA sentence
{
i=0;
gpsString="";
}
}
if(inChar=='\r')
{
if(i>65)
{
gps_status=1;
break;
}
else
{
i=0;
}
}
}
if(gps_status)
break;
}
}
void gsm_init()
{
lcd.clear();
lcd.print("Finding Module..");
boolean at_flag=1;
while(at_flag)
{
Serial.println("AT");
while(Serial.available()>0)
{
if(Serial.find("OK"))
at_flag=0;
}
delay(1000);
}
lcd.clear();
lcd.print("Module Connected..");
delay(1000);
lcd.clear();
lcd.print("Disabling ECHO");
boolean echo_flag=1;
while(echo_flag)
{
Serial.println("ATE0");
while(Serial.available()>0)
{
if(Serial.find("OK"))
echo_flag=0;
}
delay(1000);
}
lcd.clear();
lcd.print("Echo OFF");
delay(1000);
lcd.clear();
lcd.print("Finding Network..");
boolean net_flag=1;
while(net_flag)
{
Serial.println("AT+CPIN?");
while(Serial.available()>0)
{
if(Serial.find("+CPIN: READY"))
net_flag=0;
}
delay(1000);
}
lcd.clear();
lcd.print("Network Found..");
delay(1000);
lcd.clear();
}
void get_gps()
{
gps_status=0;
int x=0;
while(gps_status==0)
{
gpsEvent();
int str_lenth=i;
latitude="";
longitude="";
int comma=0;
while(x<str_lenth)
{
if(gpsString[x]==',')
comma++;
if(comma==2) //extract latitude from string
latitude+=gpsString[x+1];
else if(comma==4) //extract longitude from string
longitude+=gpsString[x+1];
x++;
}
int l1=latitude.length();
latitude[l1-1]=' ';
l1=longitude.length();
longitude[l1-1]=' ';
lcd.clear();
lcd.print("Lat:");
lcd.print(latitude);
lcd.setCursor(0,1);
lcd.print("Long:");
lcd.print(longitude);
i=0;x=0;
str_lenth=0;
delay(2000);
}
}
void init_sms()
{
Serial.println("AT+CMGF=1");
delay(400);
Serial.println("AT+CMGS=\"+91**********\""); // use your 10 digit cell no. here
delay(400);
}
void send_data(String message)
{
Serial.println(message);
delay(200);
}
void send_sms()
{
Serial.write(26);
}
void lcd_status()
{
lcd.clear();
lcd.print("Message Sent");
delay(2000);
lcd.clear();
lcd.print("System Ready");
return;
}
void tracking()
{
init_sms();
send_data("Vehicle Tracking Alert:");
send_data("Your Vehicle Current Location is:");
Serial.print("Latitude:");
send_data(latitude);
Serial.print("Longitude:");
send_data(longitude);
send_data("Please take some action soon..\nThankyou");
send_sms();
delay(2000);
lcd_status();
}
Comments
can you help me with the code.all i really want is for the Arduino+GPS+GSM to send me the coordInate to my phone
i have my final project. I used SIM800 and my gps is Skylab SKG25B . does it work for the same circuit and codes?.
hi, how do you simulate this design in proteus?
i have done this project with same components without using lcd.
i hav a litlle confusion with this..how to interface gps with uno... whether TTL pins hav to be interfaced with uno? is TTL pins used as transmitter?/ please suggest me how to interface gps with uno..
then i dont recieve any msg...
We have several circuits which use GPS with Arduino, check here.
I like this project to do for my self experiance
how can you simulate this project proteus? tq in advance .
it is showing finding module and that's it can someone say me a solution its urgent
what if i will not put lcd. what would i do? pls help me
LCD is optional to check the status messages for debugging purpose, you can remove it.
anyone can tell me how to create the source code for vehicle tracking system in java language in software
The LCD display says no GPS range. But the GPS works fine in the serial monitor.. guide me..
Hello, our project is a kid tracker that is coordinate system based and this has helped us know more what to do. I would like to ask if how can we possibly make a wearable for the kid? Help will be highly appreciated.
You should use either GPS shield with Arduino or use Arduino Pro mini, then instead of using GSM module, use wifi module to send data over internet. This will make the whole system quite small in size.
Hello sir , I have done made my project which is that I can receive message from arduino to sent coordinate . I want to addons googlemap , is that coding possible that i can use for this programming ?
Yes, check this one: Track A Vehicle on Google Maps using Arduino, ESP8266 & GPS
Very detail explanation..it's work .. thank you very much .
Using several GPS conversion modes and does not work, the coordinates it provides are not correct and lead nowhere.
hey can i design this tracking system without using gps becoz using gps is getting complicated and i dont want a perfect location for tracking but just want a simple tracking system
is this possible
hi..i tried the above code with several different gsm module only on time time i was able to get a feedback msg from gsm and later its not happening ...any one pls help me ...ty
my project is about Arduino based on vehicle tracking system using GPS and GSM
please help me with the programming /simulation /the whole operation i can not
Sir can u help me...i have project tracker vehicle using ublock NEO-V6, SIM900, arduino....please help me
Hi, how this system differ from the existing vehicle tracking system
AT+CMGF=1
AT+CMGS="+91**********"
Vehicle Tracking Alert:
Your Vehicle Current Location is:
Latitude:1914.8910
Longitude:07251.3482
Please take some action soon..
Thankyou
THIS IS PRINTED ON THE SERIAL MONITOR BUT I AM NOT GETTING IT AS A TEXT, CAN ANY PLEASE HELP ME OUT. I HAVE USED THE CODE IN THE TUTORIAL.
what is range of this tracking system...???
My final year project is to build a system to report the status of seat belt in a company vehicle with the cordunates over GSM.
PLS I would like to know how to configure this using sensors , GPS, gsm , arduino uno n LCD.
Thank you
In that gsm_init Block Serial.Println("AT") only running.in the seriala monitor this only runs.
Hello every one,I have tried out this project with sim300. The lcd works properly and displays that 'network has been found' plus the 'longitude' and 'latitude'. and System ready is displayed butt when I send the text Track Vehicle, I get no reply and in my mobile doesn't show msg received only sending is displayed
Can any one please help me to solve this?
i dont know which number we should use in the code .
either the sim which we insert on the gsm module or the sim number where we get the gsm details..
Enter no of the SIM which is in your Mobile phone, not in GSM module.
I bought L80 GPS module. I have a problem that the GPS status is not blinking, which means the power led is glowing and the status led is not blinking properly. Please give me a solution.
First learn using GPS with Arduino here: How to Use GPS with Arduino and check your GPS if it is faulty.
hi. Nyc explanation. I want to kow the teperature of the person who was driving the vehicle.
float temp;
int tempPin = 0;
void setup()
{
Serial.begin(9600);
}
void loop()
{
temp = analogRead(tempPin);
temp = temp * 0.48828125;
Serial.print("TEMPRATURE = ");
Serial.print(temp);
Serial.print("*C");
Serial.println();
delay(1000);
}
... I want this code to be attached with ur code such that i can get the sms of tarcking lat and long along with temperature .
could u plz help ?
:\Users\Maheswar Reddy\Documents\Arduino\sketch_mar13a\sketch_mar13a.ino: In function 'void serialEvent()':
C:\Users\Maheswar Reddy\Documents\Arduino\sketch_mar13a\sketch_mar13a.ino:52:42: warning: deprecated conversion from string constant to 'char*' [-Wwrite-strings]
if(Serial.find("Track Vaccum Cleaner"))
^
C:\Users\Maheswar Reddy\Documents\Arduino\sketch_mar13a\sketch_mar13a.ino: In function 'void gsm_init()':
C:\Users\Maheswar Reddy\Documents\Arduino\sketch_mar13a\sketch_mar13a.ino:106:26: warning: deprecated conversion from string constant to 'char*' [-Wwrite-strings]
if(Serial.find("OK"))
^
C:\Users\Maheswar Reddy\Documents\Arduino\sketch_mar13a\sketch_mar13a.ino:123:26: warning: deprecated conversion from string constant to 'char*' [-Wwrite-strings]
if(Serial.find("OK"))
^
C:\Users\Maheswar Reddy\Documents\Arduino\sketch_mar13a\sketch_mar13a.ino:139:36: warning: deprecated conversion from string constant to 'char*' [-Wwrite-strings]
if(Serial.find("+CPIN: READY"))
^
Sketch uses 8054 bytes (24%) of program storage space. Maximum is 32256 bytes.
Global variables use 863 bytes (42%) of dynamic memory, leaving 1185 bytes for local variables. Maximum is 2048 bytes.
I am using GSM800 module "AT" alone get printed on the serial monitor.but i have modifeis the given code by removing the lcd display commands.
do we need any library file for GPS in arduino library . do we need to add any additional library?
I'm trying to make this project without the lcd screen (everything else is same) . I'm not getting any output . Maybe my connections are wrong because i can't see them clearly in the demonstration video . Plz help
Follow the circuit diagram and debug using Serial Monitor or LCD.
Hello, what to do if I want to set my vehicle system within some specified coordinates and whenever the vehicle goes out of these specified coordinates the message to the mobile no. automatically sent. Thank you guys in advance
You can achieve this by modifying the Code, circuit will remain the same.
Please can some one help me. I have this error in the code given by the author.
C:\Users\Rap Okoro\Documents\Arduino\gpstracker\gpstracker.ino:11:14: warning: deprecated conversion from string constant to 'char*' [-Wwrite-strings]
char *test = "$GPGGA";
^
C:\Users\Rap Okoro\Documents\Arduino\gpstracker\gpstracker.ino: In function 'void gsm_init()':
C:\Users\Rap Okoro\Documents\Arduino\gpstracker\gpstracker.ino:104:27: warning: deprecated conversion from string constant to 'char*' [-Wwrite-strings]
if (Serial.find("OK"))
^
C:\Users\Rap Okoro\Documents\Arduino\gpstracker\gpstracker.ino:120:27: warning: deprecated conversion from string constant to 'char*' [-Wwrite-strings]
if (Serial.find("OK"))
^
C:\Users\Rap Okoro\Documents\Arduino\gpstracker\gpstracker.ino:136:37: warning: deprecated conversion from string constant to 'char*' [-Wwrite-strings]
if (Serial.find("+CPIN: READY"))
^
hey i wanted to know the meaning of two command used
one is temp=1
and the other is serialwrite(26)
a little urgent please reply asap
ALSO why are we using variable delay and not a fix one
Hey please i want to know regarding the commands usedin the progrAM
temp=1
and serialwrite(26)
please reply asap a little urgent.
thank you.
thank you so much guys. admin and all comment really successful. gsm not problem, u must know ur gsm condition. fast blinking means no network detected. heart beat blinking means already connected. gps problem- gps got small battery inside. maybe the battery has been drained. if ur gps not blinking fast continuously, that means ur gps are still not working properly. charge the gps with battery 9v. just put the vcc and gnd to battery until the gps blinking fast continuously (for my gps, it took 1-2 hour *for the first u open the packaging, then next just 10 -20 minutes), then u ready to execute the system. Dont forget to recharge ur gps everytime u want to reuse.
Hey
I have a SIM808, can I use it and what should be done in the software and hardware connections.
With great regards
I'm using sim808 module. after the upload this code LCD displayed No GPS Range .. what should i do..this is very important for me. please help me.
thank you!
Please read the previous comments before asking, your question might has already been answered.
hiii there...thanx for the helpful article.i am a engineering student nd my project is gsm based gps tracking system. i try to found such a article related" interfacing between mobile nd system but can't.just meant which android application to use or any other way. plz reply soon...
what is ARD1 and RV1 in the circuit diagram, and what is its use..
Hello, can I use the GPS module GY-NEO6MV2 and what should I do in the software I have already tried but the module is not found.
With kind regards
Hi. Thanks for the information.
I managed to have the system run. I can receive the latitude and longitude information on my phone as sms, but the information I received is different than what the standard is. The margin of error in the location information is shown to be approximately 40 kilometers.
Is there a way to fix this?
Thanks in advance.
I'm getting only
AT
AT
AT
in serial monitor and finding module in display but circuit connections are well enough good
I'.m trying since an week to resolve these error
First try interfacing GSM with Arduino, here are various articles on the same: https://circuitdigest.com/search/node/arduino%20gsm
SIM808 GSM + GPRS + GPS Cellular Module
pls is this code really working(although,have verified it) but am not sure if it will work
first of all thank you so much for your support
and my question is I need to use interface MQ3 alcohol sensor with GSM , GPS and Arduino uno so how to use it if some one knows it Pleas
for vehicle tracking using arduino project can we use SIM 808 Module with GSM+GPS+Bluuetooth model
hi Saddam khan, ive been try your code, but i get stuck on AT+CPIN loop and it keep looping and looping never ending. i was try on outdoor to test the gps network.
can you help me please, how to solved my issue?
thank you.
hi. I have tried doing this project. I used neo 6m as my gps module and an A6 mini GSM/GPRS module. My problem is that the output in the LCD screen got stuck in "Finding Network". Can you tell me what might be the problem?
Hi,
Were you able to resolve the "Finding Network" issue?. I too have this problem.
Thanks.
I am also doing same this project.
But I didn't use the LCD.
Can anyone help me....
pls post a circuit diagram
Its already given, kindly check
The circuit is already given
what coding i need to stop the looping?
i am really proud of sir..neega vera lvl...:)
can i use GY-NEO6MV2 NEO-6M gps module instead of SKG13
can it be connected with android appllication like open gps tracker
@ Salufu
What kind of "SMS" that you use is it still "Track Vehicle" just like the this web
You can use anything you want so far you use #as prefix and * as suffix ..Eg
#track*
/*
NOTE: to track the vechicle, send the commond on your phone message #TRACK
to OFF the engine send the command on your phone message #OFF ENGINE
to ON the engine send the command on your phone message #ON ENGINE
NOTE ALL THE MSG U ARE SENDING MUST BE IN CAPITAL LETTER
*/
#include<LiquidCrystal.h>
#include <SoftwareSerial.h>
LiquidCrystal lcd(7, 6, 5, 4, 3, 2);
SoftwareSerial gps(10, 11); // RX, Tx
int led = 13;
#define ENGINE 12 // PIN CONNECTED TO AN OUTPUT DEVICE. U CAN CONNECT A RESISTOR 330R OR 470R 0R 560R AND LED TO TEST THE OUTPUT,LETTER U CAN ADD A RELAY
int l = 0, x = 0, k = 0;
char str1[100], msg[32];
//String str="";
char str[70];
String gpsString = "";
char *test = "$GPGGA";
String latitude = "No Range ";
String longitude = "No Range ";
String location = "";
int temp = 0, i;
boolean gps_status = 0;
void setup()
{
lcd.begin(16, 2);
Serial.begin(9600);
gps.begin(9600);
lcd.print("Vehicle Tracking");
lcd.setCursor(0, 1);
lcd.print(" System ");
delay(2000);
gsm_init();
lcd.clear();
Serial.println("AT+CNMI=2,2,0,0,0");
delay(500);
Serial.println("AT+CMGF=1");
lcd.print("GPS Initializing");
lcd.setCursor(0, 1);
lcd.print(" No GPS Range ");
get_gps();
delay(500);
lcd.clear();
lcd.print("GPS Range Found");
lcd.setCursor(0, 1);
lcd.print("GPS is Ready");
delay(500);
lcd.clear();
lcd.print("System Ready");
pinMode(ENGINE, OUTPUT);
digitalWrite(ENGINE, LOW);
digitalWrite(led, LOW);
}
void loop()
{
serialEvent();
if (temp == 1)
{
x = 0, k = 0, temp = 0;
while (x < l)
{
while (str1[x] == '#')
{
x++;
while (str1[x] != '*')
{
msg[k++] = str1[x++];
}
}
x++;
}
//get_gps();
//tracking();
msg[k] = '\0';
check();
lcd.clear();
lcd.print(msg);
delay(1000);
temp = 0;
l = 0;
x = 0;
k = 0;
}
lcd.clear();
lcd.print(" System Ready ");
Serial.println("AT+CMGD=1,4"); // delete all SMS
}
void check()
{
if (!(strncmp(msg, "TRACK", 5)))
{
get_gps();
tracking();
delay(200);
}
else if (!(strncmp(msg, "OFF ENGINE", 10)))
{
digitalWrite(ENGINE, LOW);
//lcd.clear();
//lcd.setCursor(0, 0);
//lcd.print("ENGINE OFF");
delay(200);
}
else if (!(strncmp(msg, "ON ENGINE", 9)))
{
digitalWrite(ENGINE, HIGH);
//lcd.clear();
//lcd.setCursor(0, 0);
//lcd.print("ENGINE ON");
delay(200);
}
}
void serialEvent()
{
while (Serial.available())
{
char ch = (char)Serial.read();
str1[l++] = ch;
if (ch == '*')
{
temp = 1;
lcd.clear();
lcd.print("Message Received");
delay(1000);
}
}
}
void gsm_init()
{
lcd.clear();
lcd.print("Finding Module..");
boolean at_flag = 1;
while (at_flag)
{
Serial.println("AT");
while (Serial.available() > 0)
{
if (Serial.find("OK"))
at_flag = 0;
}
delay(1000);
}
lcd.clear();
lcd.print("Module Connected..");
delay(1000);
lcd.clear();
lcd.print("Disabling ECHO");
boolean echo_flag = 1;
while (echo_flag)
{
Serial.println("ATE0");
while (Serial.available() > 0)
{
if (Serial.find("OK"))
echo_flag = 0;
}
delay(1000);
}
lcd.clear();
lcd.print("Echo OFF");
delay(1000);
lcd.clear();
lcd.print("Finding Network..");
boolean net_flag = 1;
while (net_flag)
{
Serial.println("AT+CPIN?");
while (Serial.available() > 0)
{
if (Serial.find("+CPIN: READY"))
net_flag = 0;
}
delay(1000);
}
lcd.clear();
lcd.print("Network Found..");
delay(1000);
lcd.clear();
}
void gpsEvent()
{
gpsString = "";
while (1)
{
while (gps.available() > 0) //checking serial data from GPS
{
char inChar = (char)gps.read();
gpsString += inChar; //store data from GPS into gpsString
i++;
if (i < 7)
{
if (gpsString[i - 1] != test[i - 1]) //checking for $GPGGA sentence
{
i = 0;
gpsString = "";
}
}
if (inChar == '\r')
{
if (i > 65)
{
gps_status = 1;
break;
}
else
{
i = 0;
}
}
}
if (gps_status)
break;
}
}
void get_gps()
{
gps_status = 0;
int x = 0;
while (gps_status == 0)
{
gpsEvent();
int str_lenth = i;
latitude = "";
longitude = "";
int comma = 0;
while (x < str_lenth)
{
if (gpsString[x] == ',')
comma++;
if (comma == 2) //extract latitude from string
latitude += gpsString[x + 1];
else if (comma == 4) //extract longitude from string
longitude += gpsString[x + 1];
x++;
}
int l1 = latitude.length();
latitude[l1 - 1] = ' ';
l1 = longitude.length();
longitude[l1 - 1] = ' ';
lcd.clear();
lcd.print("Lat:");
lcd.print(latitude);
Serial.print(latitude);
lcd.setCursor(0, 1);
lcd.print("Long:");
lcd.print(longitude);
Serial.print(longitude);
i = 0; x = 0;
str_lenth = 0;
delay(10000);
}
}
void tracking()
{ float deg1;
float deg2;
//string::size_type sz;
float latstring;
float longstring;
latstring = latitude.toFloat();
longstring = longitude.toFloat();
float deg;
float degWhole;
float degDec;
degWhole = float(int(latstring / 100));
degDec = (latstring - degWhole * 100) / 60;
deg = degWhole + degDec;
deg1 = deg;
degWhole = float(int(longstring / 100));
degDec = (longstring - degWhole * 100) / 60;
deg = degWhole + degDec;
deg2 = deg;
Serial.print(deg1, 5);
Serial.print(deg2, 5);
location = "",
location += "Latitude:";
location += deg1;
location += "Longitude:";
location += deg2;
location += "http://maps.google.com/maps?&z=15&mrt=yp&t=k&q=";
location += (deg1, 5);
location += '+'; //28.612953, 77.231545 //28.612953,77.2293563
location += (deg2, 5);
location += "";
Serial.println(location);
Serial.println("AT+CMGF=1"); //Sets the GSM Module in Text Mode
delay(1000); // Delay of 1000 milli seconds or 1 second
Serial.println("AT+CMGS=\"+2348061171981\""); // Replace x with mobile number
delay(100);
delay(1000);
Serial.println("Vehicle Tracking Alert:");
Serial.print("Your Vehicle Current Location is:");
Serial.println(location);
Serial.print("Please take some action soon..\nThankyou");
delay(100);
Serial.write(26);// ASCII code of CTRL+Z
delay(1000);
lcd.clear();
lcd.print("Message Sent");
delay(2000);
lcd.clear();
lcd.print("System Ready");
//return;
}
i tried all your codes those are in comments . and all my circuit connections are correct :tx pin of gps to 10 of arduino, and tx of arduino to rx of gsm and rx to tx
in serial monitor it is showing
AT
AT
ATE0
AT+CPIN?
AT+CNMI = 2,2,0,0,0
AT+CMGF=1
This is i am getting in serial monitor(i did not used LCD)
BUT problem is if i send message to gsm ,i am not getting any replay message .so will help me where is the problem may possible.
Hi, Salufu, I'm using GSM A6 module and GPS NEO-6M-0-001, but when I compile the code , it comeout something like this
"
: warning: ISO C++ forbids converting a string constant to 'char*' [-Wwrite-strings]
char *test = "$GPGGA";
^
: In function 'void gsm_init()':
: warning: ISO C++ forbids converting a string constant to 'char*' [-Wwrite-strings]
if (Serial.find("OK"))
^
: warning: ISO C++ forbids converting a string constant to 'char*' [-Wwrite-strings]
if (Serial.find("OK"))
^
C:\Users\User\Documents\Arduino\g3\g3.ino:176:37: warning: ISO C++ forbids converting a string constant to 'char*' [-Wwrite-strings]
if (Serial.find("+CPIN: READY"))
When I run it, the LCD only stop at "Finding Network"....and Serial monitor always display " AT+SPIN?"
Can you help me? very urgent..I will very thank you for your help. please
i have my final project. I used SIM800 and my gps isRES52 NEO-6M UBLOX . does it work for the same circuit and codes?.
I have to a small change in this program that is when we send the message then these system find longitude value and latitude values and send these msg to our but instead of these condition when we push button press then these system should find latitude and longitude value and message us.
Then where we change in program?
guys i can connect gps and gsm modules with android application? if it is possible tell me how? and have a ready applications for this tracker?
Your phone already has a GSM and GPS.. why do you wanna use it external hardware
I have already done but i send msg than after i dont recive msg what to do .... and which mobile number is used in code i mean used in gsm module sim no. Or another we will get a msg......
Is there any specific module of GSM and GPS which is used in this project .If yes please specify the module name
GSM works with serial COM so any GAM module can be used. I am not sure about the GPS module though. Also the one used here is the most commonly available one
What exactly is RV1? How to connect it in the circuit?
RV1 is a potentiometer, you can connect it using the three leads as shown in the circuit diagram, the one with arrow is the center pin the other two can be connected anyhow
avrdude: stk500_getsync(): not in sync: resp=0x00
what does this mean??
plz help
hi salufu, could u send me the C code for this project. I have use arduino uno R3 , gsm module sim900A,gps module 6mv2
Serial.println("AT+CNMI=2,2,0,0,0"); why we use this i cant understand. particular 2,2,0,0,0 this nob.
"looks like to hard to use..D):
Can I used GPS module SIM28ML instead of GPS module SKG13BL.
Hi salufu hope u can help me with this problem i really need your help right for my final project this is the error in program
in my LCD it stock in Finding Module.. what is the problem>? and there are some problems below in arduino program says
C:\Users\ASUS\Documents\Arduino\GPS_tracker\GPS_tracker.ino:15:14: warning: deprecated conversion from string constant to 'char*' [-Wwrite-strings]
char *test = "$GPGGA";
^
C:\Users\ASUS\Documents\Arduino\GPS_tracker\GPS_tracker.ino: In function 'void gsm_init()':
C:\Users\ASUS\Documents\Arduino\GPS_tracker\GPS_tracker.ino:114:27: warning: deprecated conversion from string constant to 'char*' [-Wwrite-strings]
if (Serial.find("OK"))
^
C:\Users\ASUS\Documents\Arduino\GPS_tracker\GPS_tracker.ino:131:27: warning: deprecated conversion from string constant to 'char*' [-Wwrite-strings]
if (Serial.find("OK"))
^
C:\Users\ASUS\Documents\Arduino\GPS_tracker\GPS_tracker.ino:148:37: warning: deprecated conversion from string constant to 'char*' [-Wwrite-strings]
if (Serial.find("+CPIN: READY"))
Please help ASAP.. Thanks