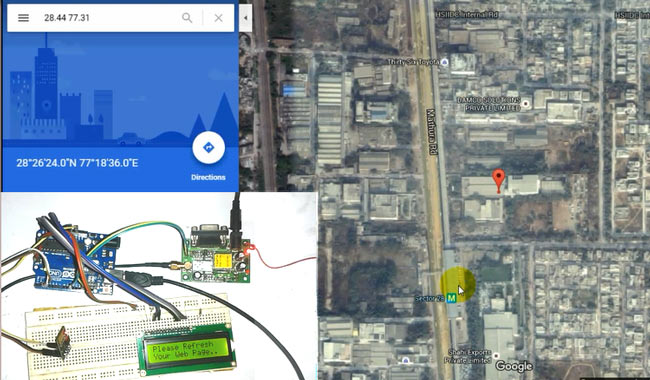
Vehicle Tracking System becomes very important now days, especially in case of stolen vehicles. If you have GPS system installed in your vehicle, you can track you Vehicle Location, and its helps police to track the Stolen Vehicles. Previously we have built similar project in which Location coordinates of Vehicle are sent on Cell Phone, check here ‘Arduino based Vehicle Tracker using GPS and GSM.
Here we are building more advanced version of Vehicle Tracking System in which you can Track your Vehicle on Google Maps. In this project, we will send the location coordinates to the Local Server and you just need to open a ‘webpage’ on your computer or mobile, where you will find a Link to Google Maps with your Vehicles Location Coordinates. When you click on that link, it takes you on Google Maps, showing your vehicles location. In this Vehicle Tracking System using Google Maps, GPS Module is used for getting the Location Coordinates, Wi-Fi module to keep send data to computer or mobile over Wi-Fi and Arduino is used to make GPS and Wi-Fi talk to each other.
How it Works:
To track the vehicle, we need to find the Coordinates of Vehicle by using GPS module. GPS module communicates continuously with the satellite for getting coordinates. Then we need to send these coordinates from GPS to our Arduino by using UART. And then Arduino extract the required data from received data by GPS.
Before this, Arduino sends command to Wi-Fi Module ESP8266 for configuring & connecting to the router and getting the IP address. After it Arduino initialize GPS for getting coordinates and the LCD shows a ‘Page Refresh message’. That means, user needs to refresh webpage. When user refreshes the webpage Arduino gets the GPS coordinates and sends the same to webpage (local server) over Wi-Fi, with some additional information and a Google maps link in it. Now by clicking this link user redirects to Google Maps with the coordinate and then he/she will get the Vehicle Current Location at the Red spot on the Google Maps. The whole process is properly shown in the Video at the end.
Components Required:
- Arduino UNO
- Wi-Fi Module ESP8266
- GPS module
- USB Cable
- Connecting wires
- Laptop
- Power supply
- 16x2 LCD
- Bread Board
- Wi-Fi router
Circuit Explanation:
Circuit for this ‘Vehicle Tracking using Google Maps project’ is very simple and we mainly need an Arduino UNO, GPS Module and ESP8266 Wi-Fi module. There is a 16x2 LCD optionally connected for displaying the status. This LCD is connected at 14-19 (A0-A5) Pins of Arduino.
Here Tx pin of GPS module is directly connected to digital pin number 10 of Arduino. By using Software Serial Library here, we have allowed serial communication on pin 10 and 11, and made them Rx and Tx respectively and left the Rx pin of GPS Module open. By default Pin 0 and 1 of Arduino are used for serial communication but by using SoftwareSerial library, we can allow serial communication on other digital pins of the Arduino. 12 Volt adaptor is used to power the GPS Module. Go through here to learn “How to Use GPS with Arduino” and get the coordinates.
Wi-Fi module ESP8266’s Vcc and GND pins are directly connected to 3.3V and GND of Arduino and CH_PD is also connected with 3.3V. Tx and Rx pins of ESP8266 are directly connected to pin 2 and 3 of Arduino. Software Serial Library is also used here to allow serial communication on pin 2 and 3 of Arduino. We have already covered the Interfacing of ESP8266 Wi-Fi module to Arduino in detail, also please go through “How to Send Data from Arduino to Webpage using WiFi” before doing this project. Below is the picture of ESP8266:
ESP8266 has two LEDs, one is Red, for indicating Power and second is Blue that is Data Communication LED. Blue LED blinks when ESP sends some data via its Tx pin. Also, do not connect ESP to +5 volt supply otherwise your device may damage. Here in this project, we have selected 9600 baud rate for all the UART communications.
User can also see the communication between Wi-Fi module ESP8266 and Arduino, on the Serial Monitor, at the baud rate of 9600:
Also check the Video at the end of this project, for detailed working process.
GPS Degree Minute to Decimal Degree Converison of Coordinates:
GPS Module receives coordinates from satellite in Degree Minute format (ddmm.mmmm) and here we need Decimal Degree format for search the location on Google Maps. So first we need to convert coordinates from Degree Minute Format to Decimal Degree Format by using given formula.
Suppose 2856.3465 (ddmm.mmmm) is the Latitude that we receive form the GPS Module. Now first two numbers are Degrees and remaining are Minutes.
So 28 is degree and 56.3465 is minute.
Now here, no need to convert Degree part (28), but only need to convert Minute part into Decimal Degree by dividing 60:
Decimal Degree Coordinate = Degree + Minute/60
Decimal Degree Coordinate = 28 + 56.3465/60
Decimal Degree Coordinate = 28 + 0.94
Decimal Degree Coordinate = 28.94
Same process will be done for Longitude Data. We have converted coordinates from Degree Minute to Decimal Degree by using above formulae in Arduino Sketch:
float minut= lat_minut.toFloat(); minut=minut/60; float degree=lat_degree.toFloat(); latitude=degree+minut; minut= long_minut.toFloat(); minut=minut/60; degree=long_degree.toFloat(); logitude=degree+minut;
Programming Explanation:
In this code, we have used SerialSoftware library to interface ESP8266 and GPS module with Arduino. Then we have defined different pins for both and initialize UART with 9600 baud rate. Also included LiquidCrystal Library for interface LCD with Arduino.
#include<SoftwareSerial.h> SoftwareSerial Serial1(2,3); //make RX arduino line is pin 2, make TX arduino line is pin 3. SoftwareSerial gps(10,11); #include<LiquidCrystal.h> LiquidCrystal lcd(14,15,16,17,18,19);
After it, we need to define or declare variable and string for different purpose.
String webpage=""; int i=0,k=0; int gps_status=0; String name="<p>1. Name: Your Name </p>"; //22 String dob="<p>2. DOB: 12 feb 1993</p>"; //21 String number="<p>4. Vehicle No.: RJ05 XY 4201</p>";//29 String cordinat="<p>Coordinates:</p>"; //17 String latitude=""; String logitude=""; String gpsString=""; char *test="$GPGGA";
Then we have made some functions for different purposes like:
Function for getting GPS data with coordinates:
void gpsEvent() { gpsString=""; while(1) { while (gps.available()>0) { char inChar = (char)gps.read(); gpsString+= inChar; if (i < 7) { if(gpsString[i-1] != test[i-1]) { i=0; ..... .... ..... .....
Function for extracting data from GPS string and convert that data to decimal degree format from the decimal minute format, as explained earliar.
void coordinate2dec() { String lat_degree=""; for(i=18;i<20;i++) lat_degree+=gpsString[i]; String lat_minut=""; for(i=20;i<28;i++) lat_minut+=gpsString[i]; ..... .... ..... .....
Function for sending commands to ESP8266 for configuring and connecting it with WIFI.
void connect_wifi(String cmd, int t) { int temp=0,i=0; while(1) { Serial.println(cmd); Serial1.println(cmd); while(Serial1.available()>0) ..... .... ..... .....
void show_coordinate() function for showing coordinate on the LCD and Serial Monitor and void get_ip() function for getting IP address.
Void Send() function for creating a String of information that is to be sent to webpage using ESP8266 and void sendwebdata() Function for sending information string to webpage using UART.
In void loop function Arduino continuously wait for request form webpage (Refreshing web page).
void loop() { k=0; Serial.println("Please Refresh Ur Page"); lcd.setCursor(0,0); lcd.print("Please Refresh "); lcd.setCursor(0,1); lcd.print("Your Web Page.. "); while(k<1000) ..... .... ..... .....
Check the Full Code Below.
Complete Project Code
#include<SoftwareSerial.h>
SoftwareSerial Serial1(2,3); //make RX arduino line is pin 2, make TX arduino line is pin 3.
SoftwareSerial gps(10,11);
#include<LiquidCrystal.h>
LiquidCrystal lcd(14,15,16,17,18,19);
boolean No_IP=false;
String IP="";
String webpage="";
int i=0,k=0;
int gps_status=0;
String name="<p>1. Name: Your Name </p>"; //22
String dob="<p>2. DOB: 12 feb 1993</p>"; //21
String number="<p>4. Vehicle No.: RJ05 XY 4201</p>";//29
String cordinat="<p>Coordinates:</p>"; //17
String latitude="";
String logitude="";
String gpsString="";
char *test="$GPGGA";
void check4IP(int t1)
{
int t2=millis();
while(t2+t1>millis())
{
while(Serial1.available()>0)
{
if(Serial1.find("WIFI GOT IP"))
{
No_IP=true;
}
}
}
}
void get_ip()
{
IP="";
char ch=0;
while(1)
{
Serial1.println("AT+CIFSR");
while(Serial1.available()>0)
{
if(Serial1.find("STAIP,"))
{
delay(1000);
Serial.print("IP Address:");
while(Serial1.available()>0)
{
ch=Serial1.read();
if(ch=='+')
break;
IP+=ch;
}
}
if(ch=='+')
break;
}
if(ch=='+')
break;
delay(1000);
}
lcd.clear();
lcd.print(IP);
lcd.setCursor(0,1);
lcd.print("Port: 80");
Serial.print(IP);
Serial.print("Port:");
Serial.println(80);
delay(1000);
}
void connect_wifi(String cmd, int t)
{
int temp=0,i=0;
while(1)
{
Serial.println(cmd);
Serial1.println(cmd);
while(Serial1.available()>0)
{
if(Serial1.find("OK"))
{
i=8;
}
}
delay(t);
if(i>5)
break;
i++;
}
if(i==8)
{
Serial.println("OK");
}
else
{
Serial.println("Error");
}
delay(1000);
}
void setup()
{
Serial1.begin(9600);
Serial.begin(9600);
lcd.begin(16,2);
lcd.print("Vehicle Tracking");
lcd.setCursor(0,1);
lcd.print(" System ");
delay(2000);
lcd.clear();
lcd.print("WIFI Connecting..");
// lcd.setCursor(0,1);
// lcd.print("Please Wait...");
delay(1000);
connect_wifi("AT",1000);
connect_wifi("AT+CWMODE=3",1000);
connect_wifi("AT+CWQAP",1000);
connect_wifi("AT+RST",5000);
check4IP(5000);
if(!No_IP)
{
Serial.println("Connecting Wifi....");
connect_wifi("AT+CWJAP=\"1st floor\",\"muda1884\"",7000); //AT+CWJAP=”wifi_username”,”wifi_password”
}
else
{
}
Serial.println("Wifi Connected");
lcd.clear();
lcd.print("WIFI Connected");
delay(2000);
lcd.clear();
lcd.print("Getting IP");
get_ip();
delay(2000);
connect_wifi("AT+CIPMUX=1",100);
connect_wifi("AT+CIPSERVER=1,80",100);
Serial1.end();
lcd.clear();
lcd.print("Waiting For GPS");
lcd.setCursor(0,1);
lcd.print(" Signal ");
delay(2000);
gps.begin(9600);
get_gps();
show_coordinate();
gps.end();
Serial1.begin(9600);
delay(2000);
lcd.clear();
lcd.print("GPS is Ready");
delay(1000);
lcd.clear();
lcd.print("System Ready");
Serial.println("System Ready..");
}
void loop()
{
k=0;
Serial.println("Please Refresh Ur Page");
lcd.setCursor(0,0);
lcd.print("Please Refresh ");
lcd.setCursor(0,1);
lcd.print("Your Web Page.. ");
while(k<1000)
{
k++;
while(Serial1.available())
{
if(Serial1.find("0,CONNECT"))
{
Serial1.end();
gps.begin(9600);
get_gps();
gps.end();
Serial1.begin(9600);
Serial1.flush();
/* lcd.clear();
lcd.print("Sending Data to ");
lcd.setCursor(0,1);
lcd.print(" Web Page ");*/
Serial.println("Start Printing");
Send();
show_coordinate();
Serial.println("Done Printing");
delay(5000);
lcd.clear();
lcd.print("System Ready");
delay(1000);
k=1200;
break;
}
}
delay(1);
}
}
void gpsEvent()
{
gpsString="";
while(1)
{
while (gps.available()>0) //Serial incoming data from GPS
{
char inChar = (char)gps.read();
gpsString+= inChar; //store incoming data from GPS to temporary string str[]
i++;
if (i < 7)
{
if(gpsString[i-1] != test[i-1]) //check for right string
{
i=0;
gpsString="";
}
}
if(inChar=='\r')
{
if(i>65)
{
gps_status=1;
break;
}
else
{
i=0;
}
}
}
if(gps_status)
break;
}
}
void get_gps()
{
gps_status=0;
int x=0;
while(gps_status==0)
{
gpsEvent();
int str_lenth=i;
latitude="";
logitude="";
coordinate2dec();
i=0;x=0;
str_lenth=0;
}
}
void show_coordinate()
{
lcd.clear();
lcd.print("Latitide:");
lcd.print(latitude);
lcd.setCursor(0,1);
lcd.print("Longitude:");
lcd.print(logitude);
Serial.print("Latitude:");
Serial.println(latitude);
Serial.print("Longitude:");
Serial.println(logitude);
}
void coordinate2dec()
{
//j=0;
String lat_degree="";
for(i=18;i<20;i++) //extract latitude from string
lat_degree+=gpsString[i];
String lat_minut="";
for(i=20;i<28;i++)
lat_minut+=gpsString[i];
String long_degree="";
for(i=30;i<33;i++) //extract longitude from string
long_degree+=gpsString[i];
String long_minut="";
for(i=33;i<41;i++)
long_minut+=gpsString[i];
float minut= lat_minut.toFloat();
minut=minut/60;
float degree=lat_degree.toFloat();
latitude=degree+minut;
minut= long_minut.toFloat();
minut=minut/60;
degree=long_degree.toFloat();
logitude=degree+minut;
}
void Send()
{
webpage = "<h1>Welcome to Saddam Khan's Page</h1><body bgcolor=f0f0f0>";
webpage+=name;
webpage+=dob;
webpage+=number;
webpage+=cordinat;
webpage+="<p>Latitude:";
webpage+=latitude;
webpage+="</p>";
webpage+="<p>Longitude:";
webpage+=logitude;
webpage+="</p>";
webpage+= "<a href=\"http://maps.google.com/maps?&z=15&mrt=yp&t=k&q=";
webpage+=latitude;
webpage+='+'; //28.612953, 77.231545 //28.612953,77.2293563
webpage+=logitude;
webpage+="\">Click Here for google map</a>";
sendwebdata();
webpage="";
while(1)
{
Serial.println("AT+CIPCLOSE=0");
Serial1.println("AT+CIPCLOSE=0");
while(Serial1.available())
{
//Serial.print(Serial1.read());
if(Serial1.find("0,CLOSE"))
{
return;
}
}
delay(500);
i++;
if(i>5)
{
i=0;
}
if(i==0)
break;
}
}
void sendwebdata()
{
i=0;
while(1)
{
unsigned int l=webpage.length();
Serial1.print("AT+CIPSEND=0,");
Serial1.println(l+2);
Serial.println(l+2);
Serial.println(webpage);
Serial1.println(webpage);
while(Serial1.available())
{
if(Serial1.find("OK"))
{
return;
}
}
i++;
if(i>5)
i=0;
if(i==0)
break;
delay(200);
}
}
Comments
yes we can.
Yes that's a valid point. We we can change it accordingly. thanks!
Did you the project to use
Did you the project to use gsm for the same purpose,and how can i add an alarm system,which i will use the gsm to trigger it??please help here
wat serious of gps u have used?
plz tell the gps serial name whether it is skg13bl or smethg else?
Gps tracker using arduino uno and gsm module...
Sir.... Pls help me.... I trusted u and completed the entire project and dumped source code. And i am able to reach to the LCD status.. That system is ready. But i am unable to get the msg to the mobile phone.... And i didn't get accurate latitude and longitude... Pls sir... Help me sir....
Sir we are waiting for the
Sir we are waiting for the upgrade of that project
U typed the IP address on the
U typed the IP address on the webpage ,which IP address is that?
This is the IP address of
This is the IP address of Arduino, allotted by router/modem.
I ran this code but in the
I ran this code but in the serial monitor it is showing error after each AT commands instead of showing OK ,can u help me with the problem
You can use ThingSpeak to
You can use ThingSpeak to upload and monitor your data online, check here to learn more.
error after each AT command
Sir, there is an error message popping after AT commands in the serial monitor. can you give some more detailed information regading this project
First try to interface GSM
First try to interface GSM with arduino, we have lot of projects with GSM and Arduino, check here.
how to connect esp 8266 d1 mini with this project
sir i am having problem while connecting esp 8266 d1 mini it doesnt have a ch pin and the serial monitor is giving error what to do???
didn't appear "Click Here for google map" link in the browser
hello,
I have run the code and viewed results on web browser using the assigned IP. but "Click Here for google map" link is not appeared in the browser.
can you please let me know the changes need to be done???
Thanks & regards,
shiva.
Please help me to improve the
Please help me to improve the accuracy of the coordinates since they only indicate me to two decimal places on the serial monitor and I want them to indicate me more decimals.
Thank you very much for your help
hello i had tried your code
hello i had tried your code bt is displaying as no gps range. when i was checking gps it is tracking.can u please give me the solution for it
Please tell what GPS module used in the project
Please tell what GPS module used in the project
Nice project
Here we have used "RoyalTek
Here we have used "RoyalTek REB-1315S4" GPS module, but you can use any.
You can use ThingSpeak to
You can use ThingSpeak to monitor the data over internet and you have to use various sensor to collect data like DHT11 Humidity & temperature sensor , BM180 pressure sensor etc. Distance and speed can be calculated using GPS data itself. Check here to use ThingSpeak Live Temperature and Humidity Monitoring over Internet using Arduino and ThingSpeak
gps didnt show any location
gps didnt show any location details.... if we put the gps code inside setup()...
code problem
please send me total code which doesnot have errors please urgent bro
this project is showing the
this project is showing the incorrect longitude and correct latitude. This may be due to some mistake in the code as my gps is working properly. So plz check the code and update it......
About lat and log decimal places
please can you put a code for the 4 decimal placers for the latitude and logitude
Not connecting to WiFi
I am getting AT+CWMODE=3 ERROR. My LCD got stuck on ' WIFI Connecting..........'.
Pls help me
I got the same error
Did you solve this, if yes? What is the solution? Can you please share it on the forum. Thanks in advance.
No output on web browser
Code successfully compiled and uploaded but problem is occuring at web browser. We are unable to connect to the web browser using 192.168.1.5:80 .... we are not getting reliable output while connecting to wifi . plz reply for this issue ...hopefull waiting for the solution .ty
luggage tracking instead of vehicle
hello
this is my final year project, am confused how all these works- my project is like exact same but need be luggage instead of vehicle.
can anyone help me please
hii..i'm rishi
hii..i'm rishi
i want this entire project
plz give any document or project report u have done
WI-Fi router
For what you used a Wi-Fi Router ?
how you use it ?
GPS Module
Hi, What GPS Module model did you use?
Thank you in advance.
tracking system
sir why we use wif module rather than gprs,,, what is the difference ....?
so you ar sending data from
so you ar sending data from arduino to local ip ! ok but you can not do the tracking if you do not send data to public ip ! maby we can just send data to email and read from there ..what you think saddam khan ?
Model Of Gps Model
Hi Sir,
My Name Is Shah Amar .And I would like to know about The Which Model Is Always Use for Gps Module?
HTTPS request
great work..!!
QQ: Is it possible to send the gps data to a cloud endpoint with authentication and bearer over https.?
if yes, can you please give some pointers..
TIA..
discussing about project
i am making a project which is almost same so can u tell me how you had made the android app in which we can track the locations
hello there, I am working on
hello there, I am working on this project can you send me the brief circuit (modules) connection, required stuffs and codes
hello.. can this gps be
hello.. can this gps be accesed from a website ...say if the vechile with the gps device is numbered 1 and if i want to knw its loctaion from a website are there any codings in such a way that if i enter 1 in my website i can access to the google map in which the location can be seen....If there is please tell me how to do it
Does this work for localhost
Hello, I am using XAMPP localhost as my web server. Does this work with localhost address 127.0.0.1?
Great Work!! But can u track
Great Work!! But can u track real time moving location of that vehicle or anything.
Thank you.
Sir can u tell me the gps module model number...?
I searched for gps modules in online for purchasing bet the module what u have used is not showing plz tell me the gps module number
Error in serial monitor, after each command AT, AT+CWMODE=3, etc
I have connected the hardware as shown in the article and uploaded the code as it is. I am getting compile time error as - can anybody help with this?
GPS_ArduinoUno_ESP8266.ino:22:12: warning: deprecated conversion from string constant to 'char*' [-Wwrite-strings]
char *test="$GPGGA";
^
GPS_ArduinoUno_ESP8266.ino: In function 'void check4IP(int)':
GPS_ArduinoUno_ESP8266.ino:27:14: warning: comparison between signed and unsigned integer expressions [-Wsign-compare]
while(t2+t1>millis())
^
GPS_ArduinoUno_ESP8266.ino:31:36: warning: deprecated conversion from string constant to 'char*' [-Wwrite-strings]
if(Serial1.find("WIFI GOT IP"))
^
GPS_ArduinoUno_ESP8266.ino: In function 'void get_ip()':
GPS_ArduinoUno_ESP8266.ino:48:31: warning: deprecated conversion from string constant to 'char*' [-Wwrite-strings]
if(Serial1.find("STAIP,"))
^
GPS_ArduinoUno_ESP8266.ino: In function 'void connect_wifi(String, int)':
GPS_ArduinoUno_ESP8266.ino:86:27: warning: deprecated conversion from string constant to 'char*' [-Wwrite-strings]
if(Serial1.find("OK"))
^
GPS_ArduinoUno_ESP8266.ino:79:7: warning: unused variable 'temp' [-Wunused-variable]
int temp=0,i=0;
^
GPS_ArduinoUno_ESP8266.ino: In function 'void loop()':
GPS_ArduinoUno_ESP8266.ino:179:32: warning: deprecated conversion from string constant to 'char*' [-Wwrite-strings]
if(Serial1.find("0,CONNECT"))
^
GPS_ArduinoUno_ESP8266.ino: In function 'void get_gps()':
GPS_ArduinoUno_ESP8266.ino:250:9: warning: variable 'str_lenth' set but not used [-Wunused-but-set-variable]
int str_lenth=i;
^
GPS_ArduinoUno_ESP8266.ino:246:8: warning: variable 'x' set but not used [-Wunused-but-set-variable]
int x=0;
^
GPS_ArduinoUno_ESP8266.ino: In function 'void Send()':
GPS_ArduinoUno_ESP8266.ino:333:34: warning: deprecated conversion from string constant to 'char*' [-Wwrite-strings]
if(Serial1.find("0,CLOSE"))
^
GPS_ArduinoUno_ESP8266.ino: In function 'void sendwebdata()':
GPS_ArduinoUno_ESP8266.ino:363:29: warning: deprecated conversion from string constant to 'char*' [-Wwrite-strings]
if(Serial1.find("OK"))
Do we have to keep ESP8266 in program mode?
While uploading code in Arduino, do we have to keep ESP8266 in program mode, or are there any other settings, I am getting errors in the serial monitor. ESP8266 is not getting connected. Getting stuck at GETTING IP address.
I'm not able to (calibrate to
I'm not able to (calibrate to) get accurate gps readings ...plz help
Same here,please help me if
Same here,please help me if you have resolved your issue.
thank you for the detailed
thank you for the detailed information..
and As per my obersevation in your code, your not using any AT commands for GPS with power ON of your GPS(AT+CGPSPWR=1) how that will work. it is my doubt please solve this, Thank you in advance.
Is wifi necessary for this
Is wifi necessary for this project.Can i use mobile`s data for that purpose?
Thanks
Turn on hotspot and connect
Turn on hotspot and connect your esp to it
Aswinth Raj.
Aswinth Raj.
I am really glad that you responded for my message,brother i am stuck in "getting iP" line.
I am not getting IP address of wifi module on serial monitor screen.
kindly help me,i am running exactly the same code provided here except the Baud rate which i changed to 115200 instead of 9600 because my wifi module does not connect to home wifi router at 9600 baud rate.
Thanks
DATA TO APRS WEB
HI
if it is possibal to send trackre data to aprs web in place fo googl map?
what changes to make for it
im getting no. of errors can anyone will help me???? please help
warning: ISO C++ forbids converting a string constant to 'char*' [-Wwrite-strings]
char *test="$GPGGA";
^
In function 'void check4IP(int)':
warning: ISO C++ forbids converting a string constant to 'char*' [-Wwrite-strings]
if(Serial1.find("WIFI GOT IP"))
^
In function 'void get_ip()':
warning: ISO C++ forbids converting a string constant to 'char*' [-Wwrite-strings]
if(Serial1.find("STAIP,"))
^
In function 'void connect_wifi(String, int)':
warning: ISO C++ forbids converting a string constant to 'char*' [-Wwrite-strings]
if(Serial1.find("OK"))
^
In function 'void loop()':
warning: ISO C++ forbids converting a string constant to 'char*' [-Wwrite-strings]
if(Serial1.find("0,CONNECT"))
^
In function 'void Send()':
warning: ISO C++ forbids converting a string constant to 'char*' [-Wwrite-strings]
if(Serial1.find("0,CLOSE"))
^
In function 'void sendwebdata()':
warning: ISO C++ forbids converting a string constant to 'char*' [-Wwrite-strings]
if(Serial1.find("OK"))
^
Location on Google Map
Hello Saddam,
Thanks for this useful tutorial. I have one question for you. If we need to contineously monitor the GPS location of a vehicle, we need to contineously feed the GPS coordinates to the google map right? As of now, I believe that we need to refresh the google map page to update the new location in the map, right? Do you have any idea how we can do this? If so pease post it.
Thanks in Advance..
Melvin
Great,
You have said data will be uploaded to web via wifi module, however i think currently not all cars have internet wifi included inside! i meant isn't it better to keep on the gsm shield to drop data to the web ?? since you don't need the thief to go to wifi AP and update where is he?