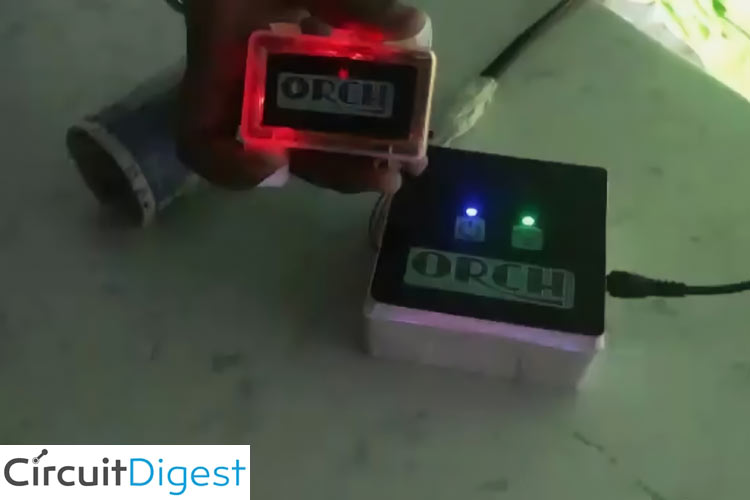
Do you feel disturbed when you need to adjust the temperature? The wait is over, the solution is here! We often feel uncomfortable while sleeping due to certain changes in room temperature, but we don’t want to get up from sleep and regulate fan/AC due to lethargy. This hampers our sleep and may put a negative effect on our health. We will use Arduino IDE to program ESP32. Check out our previously built ESP32 based projects.
Previously, we have worked on some smart fan projects,
- Build an Automatic Motion Controlled Smart Fan using ATmega328 and Motion Sensor
- IoT Based AC Fan Speed Control using Smart Phone with NodeMCU and Google Firebase
- Simple Fan Regulator Circuit to Control the Speed of AC Fan
- Temperature Controlled DC Fan using Thermistor
Component Required for Smart Fan Regulator
Project Used Hardware
ESP32, HC05, LM35, DHT11, TP4056, Arduino Nano, TRIAC, Optocoupler,3.7V Li-Po battery, 5V boost module, Bridge rectifier, LEDs, Resistors, Push to ON SPST, micro-USB type B female, Wires
Project Used Software
Kodular Creator, Google Firebase, Arduino IDE
Project Hardware/Software Selection
ESP32: ESP32 is used for its built in WIFI and Bluetooth feature along with its powerful circuit controlling ability.
HC05: HC05 is one of the most popular Bluetooth module out there and its easily available.
LM35: LM35 temperature sensor is being selected for its small and compact size.
DHT11: DHT11 is being used as room temperature and humidity sensor. We selected it due to its compact size and easy availability.
TP4056: TP4056 is used for charging the Li-Po battery inside the temperature tracker.
Arduino Nano: Arduino Nano is used as the medium for recording the body temperature and sending that data to control unit. We selected for its small and compact size so that we can fit it into the box (though Arduino Pro Mini could have been used).
TRIAC: TRIAC is used for controlling the AC motor of fan.
Optocoupler: Optocoupler is a part of TRIAC based dimming circuit.
3.7V Li-Po battery: This is used for small and compact size.
5V boost module: Boost module is being used for boosting the 3.7V of battery to 5V and to power the Arduino board.
Kodular creator: We selected Kodular for building the application, its free and popular.
Google Firebase: Google Firebase is one of the most popular and free database.
Smart Fan Regulator Circuit Diagram
We have made an automatic AC dimmer circuit following the zero cross detection technique. We have used LM35 as the temperature sensor for sensing body temperature and DHT11 for sensing both room temperature and humidity. Arduino Nano and HC05 is used for bluetooth communication between temperature tracker and main control unit(ESP32). Data collected from these sensors are processed by ESP32 and it controls the fan speed accordingly.
Complete Project Code
#include "BluetoothSerial.h"
#include <SimpleDHT.h>
BluetoothSerial SerialBT;
int pinDHT11 = 15;
SimpleDHT11 dht11(pinDHT11);
String MACadd = "00:21:06:08:4A:1C";
//uint8_t address[6] = {0x00, 0x21, 0x06, 0x08, 0x4A, 0x1C};
uint8_t address[6] = {0x00, 0x21, 0x06, 0x08, 0x4A, 0x1C};
String name = "ORCH";
char *pin = "1234"; //<- standard pin would be provided by default
bool connected;
unsigned long cm, pm;
int interval = 5000;
byte temperature = 0;
byte humidity = 0;
void setup() {
pinMode(2,OUTPUT); digitalWrite(2,0);
Serial.begin(115200);
SerialBT.begin("ORCH_MB", true);
Serial.println("The device started in master mode, make sure remote BT device is on!");
//connected = SerialBT.connect(name);
connected = SerialBT.connect(address);
if(connected)
{
Serial.println("Connected Succesfully!");
}
else
{
while(!SerialBT.connected(10000))
{
connected = SerialBT.connect(name);
Serial.println("Failed to connect. Make sure remote device is available and in range, then restart app.");
}
}
// this would reconnect to the name(will use address, if resolved) or address used with connect(name/address).
SerialBT.connect();
if(SerialBT.connected()){digitalWrite(2,1);}
}
void loop() {
if (SerialBT.available())
{
//Serial.print("Body temperature: ");
String C = String(SerialBT.read());
Serial.print("B1: ");Serial.write(SerialBT.read());
Serial.print(" B2: ");Serial.println(C);
//Serial.println(" *F");//delay(500);
}
//*******************************************************
int err = SimpleDHTErrSuccess;
if ((err = dht11.read(&temperature, &humidity, NULL)) != SimpleDHTErrSuccess)
{
Serial.print("Read DHT11 failed, err="); Serial.print(SimpleDHTErrCode(err));
Serial.print(","); Serial.println(SimpleDHTErrDuration(err)); delay(1000);
return;
}
//Serial.print((int)temperature); Serial.print(" *C, ");
//Serial.print((int)humidity); Serial.println(" H");
delay(1500);
//*******************************************************
cm=millis();
if ((cm-pm)>=interval)
{
//Serial.println(cm-pm);
if(SerialBT.connected())
{/*Serial.println("connected");*/ digitalWrite(2,1);}
else
{
/*Serial.println("not connected");*/ digitalWrite(2,0);
SerialBT.connect();
}
pm=millis();
}
}//loop
........................................
/*
Software serial multple serial test
Receives from the hardware serial, sends to software serial.
Receives from software serial, sends to hardware serial.
The circuit:
* RX is digital pin 10 (connect to TX of other device)
* TX is digital pin 11 (connect to RX of other device)
Note:
Not all pins on the Mega and Mega 2560 support change interrupts,
so only the following can be used for RX:
10, 11, 12, 13, 50, 51, 52, 53, 62, 63, 64, 65, 66, 67, 68, 69
Not all pins on the Leonardo and Micro support change interrupts,
so only the following can be used for RX:
8, 9, 10, 11, 14 (MISO), 15 (SCK), 16 (MOSI).
created back in the mists of time
modified 25 May 2012
by Tom Igoe
based on Mikal Hart's example
This example code is in the public domain.
*/
#include <SoftwareSerial.h>
SoftwareSerial mySerial(10, 11); // RX, TX
float temp, tempAvg, tf;
void setup() {
// Open serial communications and wait for port to open:
Serial.begin(115200);
Serial.println("Goodnight moon!");
// set the data rate for the SoftwareSerial port
mySerial.begin(9600);
pinMode(A0,INPUT);
}
void loop() { // run over and over
for (int i=0; i<100; i++)
{
temp = analogRead(A0);
temp = ( temp/1024.0)*5000;
temp = temp/10;
temp = ((9*temp)/5)+32;
Serial.println(temp);
tempAvg = tempAvg+temp;
delay(2);
}
tf=tempAvg/100;
Serial.println("------");
Serial.println(tf);
mySerial.println(String(tf));
delay(2500);
tempAvg=0;
}
........................................
int x,y;
const byte button_pin = 13;
const byte trig = 2;
int f=0;
boolean message = false;
unsigned long cm,pm;
void IRAM_ATTR got_function()
{
// if (f <= 300)
// {
Serial.print("lt");
Serial.println(micros()-pm);
cm = micros();
delayMicroseconds(x);
digitalWrite(trig,1);
delayMicroseconds(10);
digitalWrite(trig,0);
Serial.print("DT+TRIG");
Serial.println(micros()-cm);
pm = micros();
Serial.println(x);
//f++;
//}
}
void setup()
{
Serial.begin(115200);
pinMode(trig,OUTPUT);
digitalWrite(trig,0);
pinMode(button_pin,INPUT_PULLUP);
pinMode(A18,INPUT);
attachInterrupt(button_pin,got_function,FALLING);
}
void loop()
{
y = analogRead(A18);
x= map(y,0,4095, 200,19200);
}
...................................
#include "BluetoothSerial.h"
BluetoothSerial SerialBT;
String MACadd = "00:21:06:08:4A:1C";
//uint8_t address[6] = {0x00, 0x21, 0x06, 0x08, 0x4A, 0x1C};
uint8_t address[6] = {0x00, 0x21, 0x06, 0x08, 0x4A, 0x1C};
String name = "ORCH";
char *pin = "1234"; //<- standard pin would be provided by default
bool connected;
unsigned long cm, pm;
int interval = 5000;
void setup() {
pinMode(2,OUTPUT); digitalWrite(2,0);
Serial.begin(115200);
//SerialBT.setPin(pin);
SerialBT.begin("ORCH_MB", true);
//SerialBT.setPin(pin);
Serial.println("The device started in master mode, make sure remote BT device is on!");
// connect(address) is fast (upto 10 secs max), connect(name) is slow (upto 30 secs max) as it needs
// to resolve name to address first, but it allows to connect to different devices with the same name.
// Set CoreDebugLevel to Info to view devices bluetooth address and device names
//connected = SerialBT.connect(name);
connected = SerialBT.connect(address);
if(connected)
{
Serial.println("Connected Succesfully!");
}
else
{
while(!SerialBT.connected(10000))
{
connected = SerialBT.connect(name);
//Serial.println("Failed to connect. Make sure remote device is available and in range, then restart app.");
Serial.println("..");
}
}
// this would reconnect to the name(will use address, if resolved) or address used with connect(name/address).
SerialBT.connect();
if(SerialBT.connected()){digitalWrite(2,1);Serial.println("Connected."); }
}
void loop() {
if (SerialBT.available())
{Serial.write(SerialBT.read());}
cm=millis();
if ((cm-pm)>=interval)
{
//Serial.println(cm-pm);
if(SerialBT.connected())
{/*Serial.println("connected");*/ digitalWrite(2,1);}
else
{
/*Serial.println("not connected");*/ digitalWrite(2,0);
SerialBT.connect();
}
pm=millis();
}
}
....................................
#if defined(ESP32)
#include <WiFi.h>
#include <FirebaseESP32.h>
#elif defined(ESP8266)
#include <ESP8266WiFi.h>
#include <FirebaseESP8266.h>
#endif
//Provide the token generation process info.
#include <addons/TokenHelper.h>
//Provide the RTDB payload printing info and other helper functions.
#include <addons/RTDBHelper.h>
/* 1. Define the WiFi credentials */
#define WIFI_SSID "circuiTician"
#define WIFI_PASSWORD "Pneucis@202028"
#define led_wifi 15
//For the following credentials, see examples/Authentications/SignInAsUser/EmailPassword/EmailPassword.ino
/* 2. Define the API Key */
#define API_KEY "vSgAna1TJnANgkleTIUgvfId2i6odsGn4sEtT8AT"
/* 3. Define the RTDB URL */
#define DATABASE_URL "orch-48b91-default-rtdb.firebaseio.com/" //<databaseName>.firebaseio.com or <databaseName>.<region>.firebasedatabase.app
//Define Firebase Data object
FirebaseData fbdo;
FirebaseAuth auth;
FirebaseConfig config;
unsigned long sendDataPrevMillis = 0;
unsigned long count = 0;
void setup()
{
Serial.begin(115200);
pinMode(led_wifi, OUTPUT);
digitalWrite(led_wifi,0);
WiFi.begin(WIFI_SSID, WIFI_PASSWORD);
Serial.print("Connecting to Wi-Fi");
while (WiFi.status() != WL_CONNECTED)
{
Serial.print(".");
delay(300);
}
Serial.println();
Serial.print("Connected with IP: ");
Serial.println(WiFi.localIP());
Serial.println();
digitalWrite(led_wifi,1);
Serial.printf("Firebase Client v%s\n\n", FIREBASE_CLIENT_VERSION);
/* Assign the api key (required) */
config.api_key = API_KEY;
config.database_url = DATABASE_URL;
//////////////////////////////////////////////////////////////////////////////////////////////
//Please make sure the device free Heap is not lower than 80 k for ESP32 and 10 k for ESP8266,
//otherwise the SSL connection will fail.
//////////////////////////////////////////////////////////////////////////////////////////////
Firebase.begin(DATABASE_URL, API_KEY);
//Comment or pass false value when WiFi reconnection will control by your code or third party library
// Firebase.reconnectWiFi(true);
Firebase.setDoubleDigits(5);
}
void loop()
{
//Flash string (PROGMEM and (FPSTR), String, C/C++ string, const char, char array, string literal are supported
//in all Firebase and FirebaseJson functions, unless F() macro is not supported.
if (Firebase.ready() && (millis() - sendDataPrevMillis > 15000 || sendDataPrevMillis == 0))
{
sendDataPrevMillis = millis();
Firebase.setInt(fbdo, "/test/PM", sendDataPrevMillis);
//Serial.println(Firebase.getInt(fbdo, "/test/int"));
Serial.printf("Get int... %s\n", Firebase.getInt(fbdo, "/test/int") ? String(fbdo.to<int>()).c_str() : fbdo.errorReason().c_str());
// Serial.printf("Set bool... %s\n", Firebase.setBool(fbdo, "/test/bool", count % 2 == 0) ? "ok" : fbdo.errorReason().c_str());
//
// Serial.printf("Get bool... %s\n", Firebase.getBool(fbdo, "/test/bool") ? fbdo.to<bool>() ? "true" : "false" : fbdo.errorReason().c_str());
//
// bool bVal;
// Serial.printf("Get bool ref... %s\n", Firebase.getBool(fbdo, "/test/bool", &bVal) ? bVal ? "true" : "false" : fbdo.errorReason().c_str());
//
// Serial.printf("Set int... %s\n", Firebase.setInt(fbdo, "/test/int", count) ? "ok" : fbdo.errorReason().c_str());
//
// Serial.printf("Get int... %s\n", Firebase.getInt(fbdo, "/test/int") ? String(fbdo.to<int>()).c_str() : fbdo.errorReason().c_str());
//
// int iVal = 0;
// Serial.printf("Get int ref... %s\n", Firebase.getInt(fbdo, "/test/int", &iVal) ? String(iVal).c_str() : fbdo.errorReason().c_str());
//
// Serial.printf("Set float... %s\n", Firebase.setFloat(fbdo, "/test/float", count + 10.2) ? "ok" : fbdo.errorReason().c_str());
//
// Serial.printf("Get float... %s\n", Firebase.getFloat(fbdo, "/test/float") ? String(fbdo.to<float>()).c_str() : fbdo.errorReason().c_str());
//
// Serial.printf("Set double... %s\n", Firebase.setDouble(fbdo, "/test/double", count + 35.517549723765) ? "ok" : fbdo.errorReason().c_str());
//
// Serial.printf("Get double... %s\n", Firebase.getDouble(fbdo, "/test/double") ? String(fbdo.to<double>()).c_str() : fbdo.errorReason().c_str());
//
// Serial.printf("Set string... %s\n", Firebase.setString(fbdo, "/test/string", "Hello World!") ? "ok" : fbdo.errorReason().c_str());
//
// Serial.printf("Get string... %s\n", Firebase.getString(fbdo, "/test/string") ? fbdo.to<const char *>() : fbdo.errorReason().c_str());
//
// Serial.println();
count++;
}
}