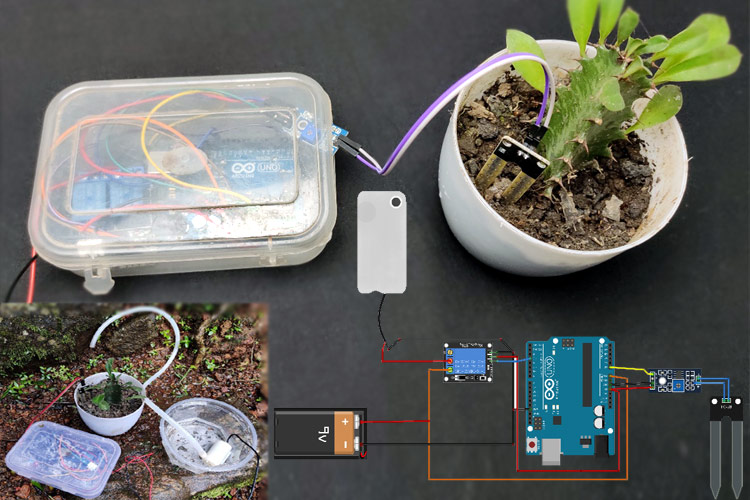
Everyone of us likes a little greenery in our houses, don’t we? Plants require really low maintenance and can be left for days without supervision but our long trips extending over a week or 2 can be detrimental for the health of plants due to the lack of moisture in the soil. In such situations, the plant may wither or die due to the absence of proper watering. In order to solve this problem, in this project, we are making an Automatic Irrigation System with an Arduino Uno which will irrigate your plants automatically and keep them healthy even when you are out of the town for weeks or months. In this project, a Moisture sensor will be used to maintain the optimum level of moisture for your plants. This system can be implemented, both for your garden or for your Indoor plants thus taking care of your leafy pets when you are away. If you are a beginner you can also read our Arduino Soil moisture sensor tutorial to understand the basics before you proceed with this tutorial.
We also build low cost IoT based Soil Moisture Monitoring Device, you can check out the demonstration video given below.
Working of the Automatic Irrigation System
The logic of this system is very simple. In this system, the moisture sensor senses the moisture level of the soil and when the sensor senses a low moisture level it automatically switches the water pump with the help of a microcontroller and irrigates the plant. After supplying sufficient water, the soil gets retains the moisture hence automatically stopping the pump.
Soil Moisture Sensor
The working of the soil moisture sensor is very easy to understand. It has 2 probes with exposed contacts that act like a variable resistor whose resistance varies according to the water content in the soil. This resistance is inversely proportional to the soil moisture which means that higher water in the soil means better conductivity and hence a lower resistance. While the lower water in the soil means poor conductivity and will result in higher resistance. The sensor produces an analog voltage output according to the resistance.
The sensor comes with an electronic module that connects the probe to the Arduino. The module has an LM393 High Precision Comparator which converts the analog signal to a Digital Output which is fed to the microcontroller. We have covered an in-depth Arduino soil moisture sensor tutorial which covers the working of soil moisture sensor module and how to use it with the Arduino. You can check the tutorial if you want to learn more about the soil moisture sensor.
Pump
We need a small pump to irrigate the plant, but in the case of a garden, we need to drive a larger pump that can provide a higher volume of water depending on the size of your garden which can’t be directly powered by an Arduino. So in case you need to operate a larger pump, a driver is necessary to provide enough current for the pump, to show that I am using a 5v relay. You can also use an AC-powered pump and use a suitable relay. The working will remain the same as shown in this project, you just have to replace the DC power input connected to the relay with an AC power input and have to power your Arduino with a separate DC power source.
Components Required for the Automatic Irrigation System
The project requires very few components and the connection is also very simple. The components are listed below:
- Arduino * 1
- moisture sensor * 1
- 5v relay module * 1
- 6v Mini water pump with small pipe * 1
- Connecting wires
- 5v battery * 1
Circuit Diagram of the Arduino Automatic irrigation system
The complete circuit diagram for the Arduino Automatic irrigation system is shown below:
In this section, I will explain all the details with the help of the schematic diagram. The Arduino UNO is the brain of this whole project. It controls the motor pump according to the moisture in the soil which is given by the moisture sensor.
To power the circuit, I am using an external Battery. You can use any 9v or 12-volt battery. The battery is connected to the Vin and ground pins of Arduino and we can also connect the motor to this battery via a relay. Moisture sensor output is connected to the analog pin of Arduino. Do remember to use the Arduino’s 5volt pin to power the sensor and relay module.
Assembling the Automatic Irrigation System
Let's start with connecting the relay to the Arduino board. Connect the VCC of the relay module to the 5v pin of the Arduino and connect the ground of the relay to the ground of Arduino. Now connect the relay signal pin to any digital pin of Arduino except pin 13. Here I have connected it to pin 3 as shown in the image below.
The next step is to connect the soil moisture sensor with the Arduino. Connect the VCC and gnd of the sensor to the 5volt and ground pin of the Arduino. The analogue output of the sensor connects to any analogue pin of the Arduino, here I’ve connected it to pin A0 (according to our program).
Finally, connect the pump to the relay module. A relay module has 3 connection points which are common, normally closed, and normally open. We have to connect the pump positive to common and connect the normally open pin to the positive of the battery. You have to select the battery as per your pump. The next step is to connect the ground of the pump to the ground of the Arduino and finally, connect the small hose to the water pump.
Now connect the battery to the circuit and if the pump starts working then your circuit is okay. Now let's upload code to Arduino.
Explanation of the code for The Automatic Irrigation System
For this project, we are not using any library we are just using the basic functions for programming. The code is very simple and easy to use. The explanation of the code is as follows.
We start by defining all the required integers here I used two integers for storing the soil moisture and the converted moisture percentage.
int soilMoistureValue = 0; int percentage=0;
Now, we define the pin mode, here I have used pin 3 as an output and in the next line, I have initialised Serial Monitor for debugging.
void setup() { pinMode(3,OUTPUT); Serial.begin(9600); }
I started the loop section by reading the soil moisture. I used the analogRead function of Arduino to read the soil moisture and I stored that in soilMoistureValue. This value varies from 0 to 1023
void loop() { soilMoistureValue = analogRead(A0);
In the below line, I have converted the sensor values from 0-100 percent for that we use the map function on Arduino. That means that if the soil is dry then the output moisture percentage is 0% and if the soil is extremely wet then the moisture percentage is 100%.
percentage = map(soilMoistureValue, 490, 1023, 0, 100); Serial.println(percentage);
Calibrating our Moisture Sensor
In the map function, we need to assign the dry value and wet value. To do that we need to monitor that values. You can read that values using the following code:
void setup() { Serial.begin(9600); } void loop() { int sensorValue = analogRead(A0); Serial.println(sensorValue); delay(1);
Upload the above code to your Arduino and open the serial monitor. Then place your soil moisture sensor in dry soil or just hold the sensor in the air and read the value. now put that value in place of 490(second term of map function).
The next step is to place the sensor in wet soil or submerge it in water and read the value and assign that value in place of 1023 (third term of map function). These values will calibrate your sensors correctly to get better results.
After converting the values we can control the pump according to the soil moisture percentage. With the help of ‘If condition’, I write the first condition, if the moisture percentage goes below 10, then the Arduino will turn pin 3 to LOW and the pump will turn on ( our relay module uses the active low signal to trigger) and the Arduino will print pump on message in the serial monitor.
If (percentage < 10) { Serial.println(" pump on"); digitalWrite(3,LOW); }
When the moisture percentage goes above 80 percent ( indicating soil is filled with water) the Arduino will turn off the pump and print the ‘pump off’ on the serial monitor.
if(percentage >80) { Serial.println("pump off"); digitalWrite(3,HIGH); } }
Testing the Automatic Irrigation System
After uploading the code to the Arduino, I placed the whole circuit except the pump and sensor probe in a plastic box as shown in the figure below.
Now place the moisture sensor into the soil. Place the sensor as close to the roots of the plants as possible for higher accuracy.
The final step is to place your motor in a container filled with water and your automatic irrigation is ready to take care of your lovely plants when you are not around.
You might have to change the moisture percentage to start and stop the pump as different plants have different water requirements. Hope you enjoyed the project and are ready to build your own automatic irrigation system using Arduino. If you have any questions, you can leave them in the comment section below.
int soilMoistureValue = 0;
int percentage=0;
void setup() {
pinMode(3,OUTPUT);
Serial.begin(9600);
}
void loop() {
soilMoistureValue = analogRead(A0);
Serial.println(percentage);
percentage = map(soilMoistureValue, 490, 1023, 100, 0);
if(percentage < 10)
{
Serial.println(" pump on");
digitalWrite(3,LOW);
}
if(percentage >80)
{
Serial.println("pump off");
digitalWrite(3,HIGH);
}
}