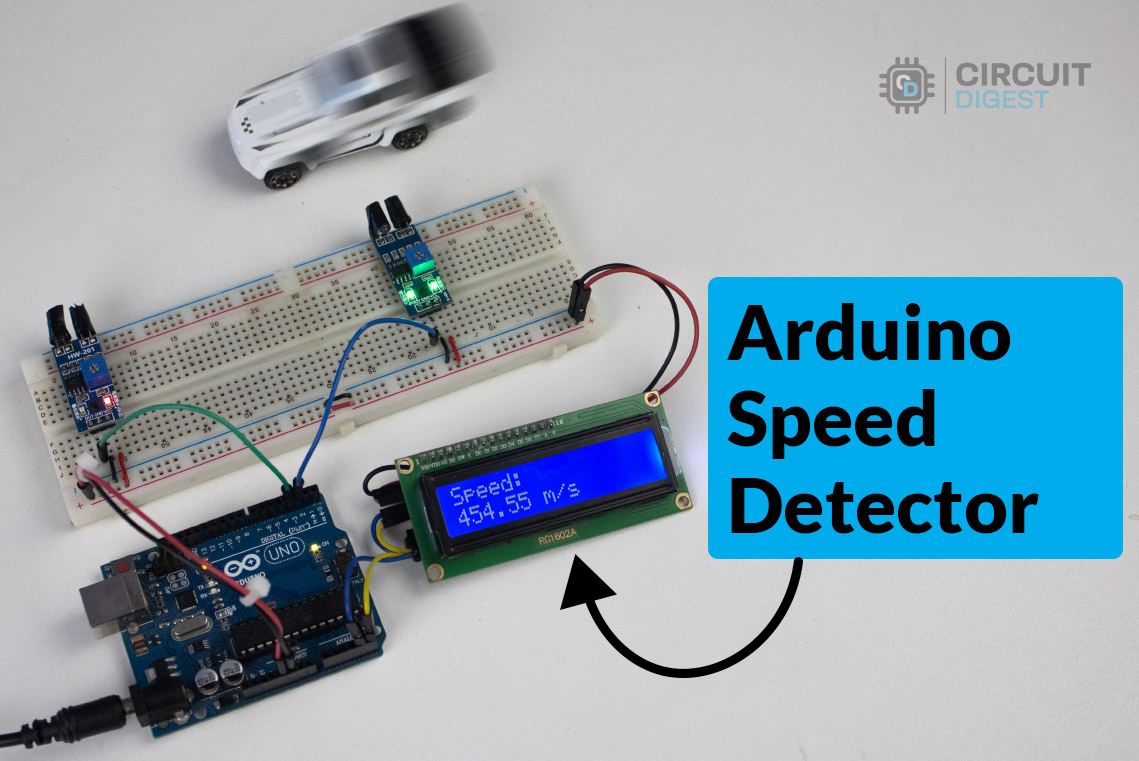
Have you ever wondered how speed guns measure the speed of moving vehicles or how an athlete's sprint speed is recorded? Normally these speed sensors work with radar or laser technology to bounce back radio waves or IR light from objects and calculate speed based on time taken for the light or wave to reflect. In this article, we will learn how to similar speed sensor using Arduino and IR sensor.
By setting up the two IR sensors at a fixed distance from each other, we can track the time it takes for the object to travel between them. With the recorded time and the known distance between them, we can accurately calculate the object's speed using a formula.
What makes this project even more interesting is its applicability. Not only we can measure the speed of moving cars or other objects, but we can also utilize this Arduino based speed sensor in scientific experiments to study motion, acceleration, or friction by measuring the speed of different objects in a controlled environments. So let's get started
Table of Contents
- What do you need to build an Arduino Speed Sensor
- Arduino Speed Detector Circuit Diagram
- How to Place the IR Sensor in the Right Position to Measure Speed
- Minimizing External Disturbance to IR sensor using Black Tape:
- How to Use IR Sensor Modules to Measure Speed?
- └ Basic Principle Behind IR Sensor Setup:
- └ Moving Object Actual Speed Calculation:
- └ Speed Calculation Formula:
- Arduino Code for Speed Sensor
- Car Speed Detector using Arduino and IR Sensor
- Working Video
- Other Arduino Projects Related to Speed Measuring
What do you need to build an Arduino Speed Sensor
Building an Arduino speed detector using IR technology to measure the speed of a moving object is much more cost-effective, and it is also very simple to build. All you need are a few basic parts, which are listed below.
Arduino UNO R3 development board
Two IR Sensors
16x2 LCD Display with I2C module.
Breadboard
Connecting Wires
12V Power Adaptor with DC Barrel Jack
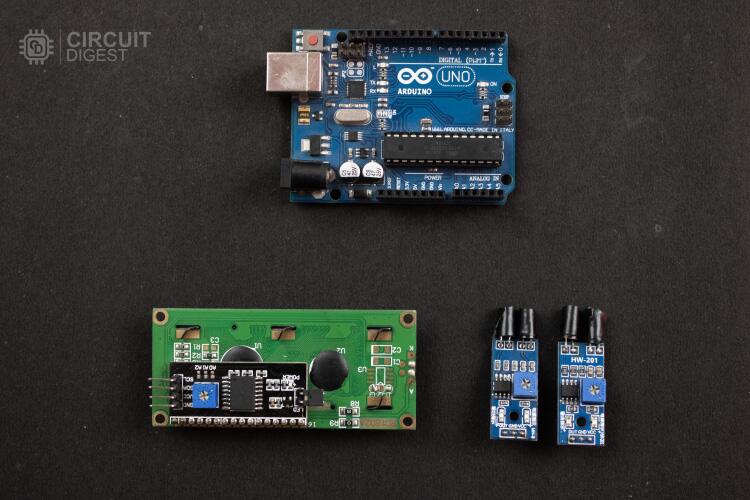
These simple components are essential for us to get started on building our own Arduino speed sensor system.
Arduino Speed Detector Circuit Diagram
A simple breadboard based circuit diagram is shown below to help you with making the connections. As you can see the circuit is very simple and only consists of two IR sensors, an Arduino Uno, LCD display and a breadboard.
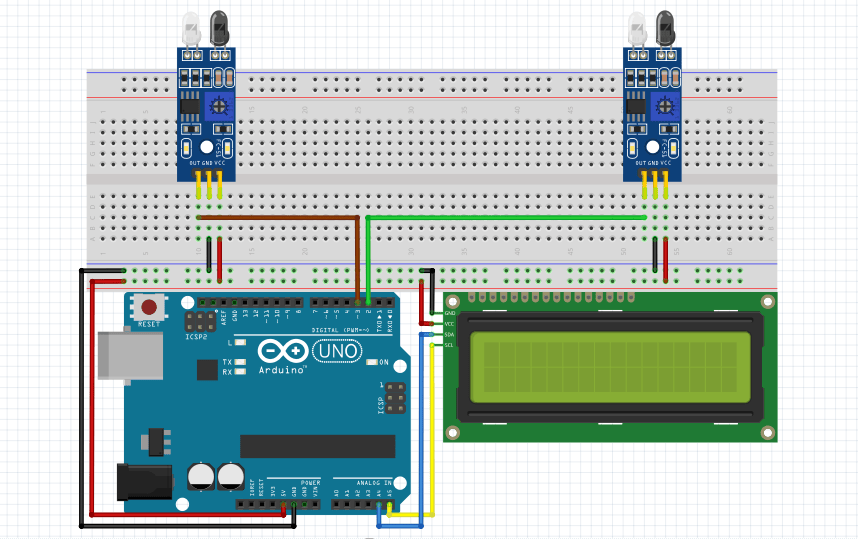
Also note that, to power this circuit you will need an external 12V power adapter. Because this setup consumes more current, and ordinary PC USB ports can’t deliver it. The power adapter simply has to be connected to Arduino UNO and is not shown in the above circuit diagram.
In the below image, you can see the actual hardware setup developed by using our Arduino sensor circuit diagram as a reference. Here you can see that I am powering this whole setup from the external power adapter through a 12V DC jack. We have also marled the parts to make the connections easy to understand. Also note that we have covered the IR LEDs on the sensor with a black tape to make it point only forward, we will discuss more about that later. If you are completely new to IR sensors and wish to learn more you can check our Arduino IR sensor tutorial, where we have explained the basic working of the IR sensor module and how to use it with Arduino.
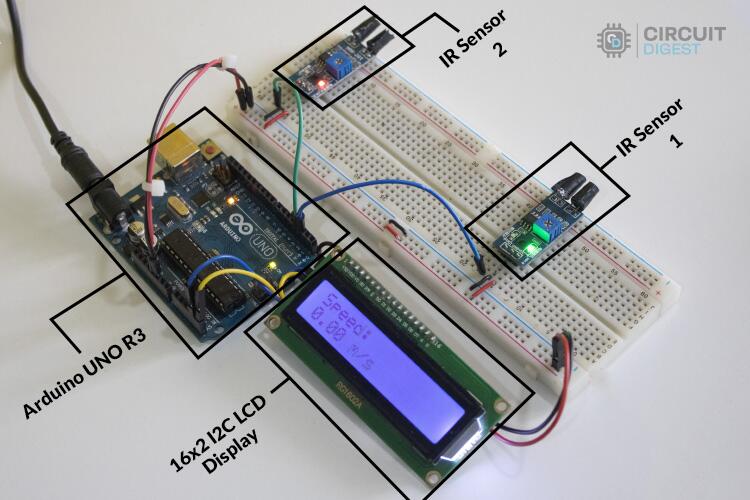
You can see our main controller, the “Arduino UNO R3”, is responsible for handling signals from IR sensors and doing some computation to calculate the speed of the object.
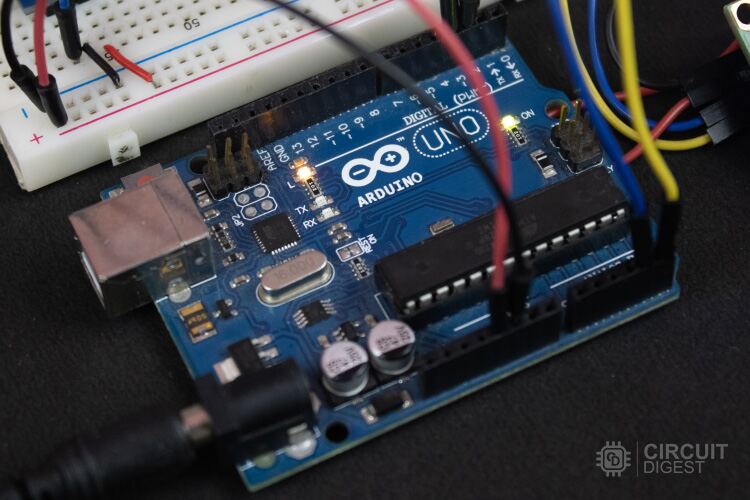
Here in the below picture, you can find our 16x2 LCD display unit, where users can able to see the speed of the moving object on it. If you are completely new to 16x2 LCD and wish to learn more about it you can check out our tutorial on Arduino 16x2 LCD display to understand the basic pinout layout and working of the 16x2 LCD display.
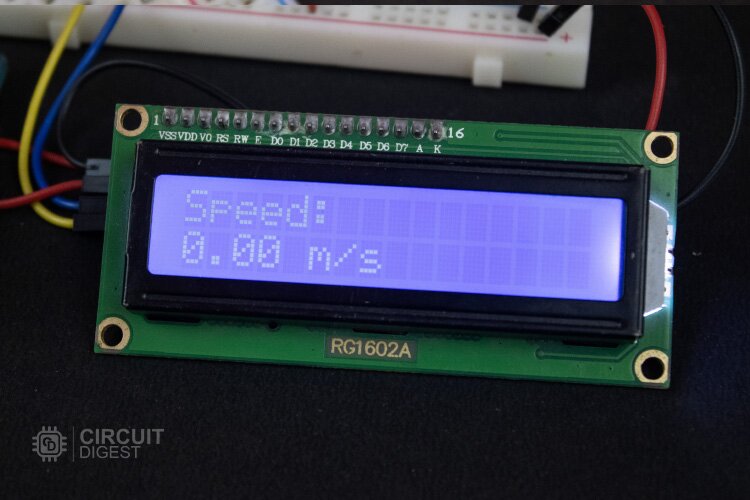
The below image shows the bottom view of our display unit, where you can see it uses an I2C IO Expansion module. It means we don’t need to rely on complex parallel communication; we can simply control the display through the I2C serial protocol.
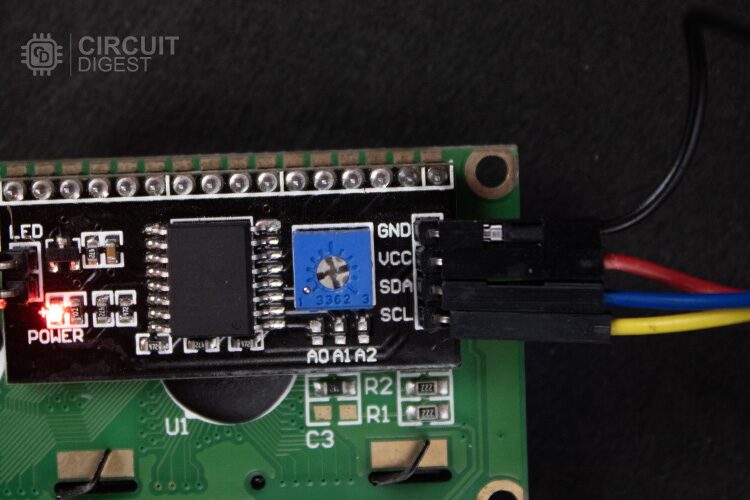
By using this kind of display, we can totally avoid the wiring complexity. This kind of I2C expansion boards for 16x2 LCD display is easily available in any electronics hardware store. It is recommended to use one to make the building processes easy.
How to Place the IR Sensor in the Right Position to Measure Speed
Next we want to ensure that the distance between the two IR sensors is in multiples of 10 or in such a way that the microcontroller does the division operation without any errors. Because in general, the Arduino UNO R3 doesn’t have sophisticated hardware support for floating-point operation. For that, I decided to keep a 10 cm spacing between two IR sensors.
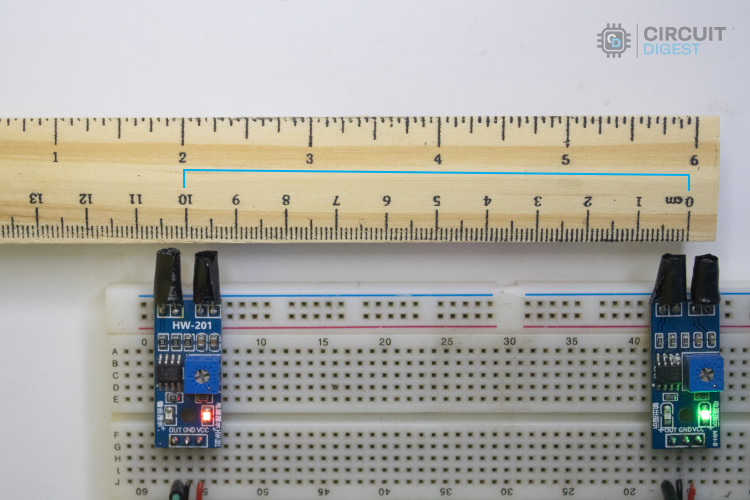
we can also make sure the spacing between the actual IR sensor should be bigger than the actual moving object’s size as shown in the below image. It’s not mandatory, but it helps in making our system work precisely.

In the next section, we are going to see some hardware hacks to overcome the IR sensor false trigger due to environmental factors.
Minimizing External Disturbance to IR sensor using Black Tape:
As we know, IR sensors are sensitive to external environmental conditions such as sunlight, room light, etc., which can significantly affect their accuracy and cause false triggers. To minimize that, I came up with an idea to cover the IR sensor’s LED using black tape by leaving its focus point alone uncovered.
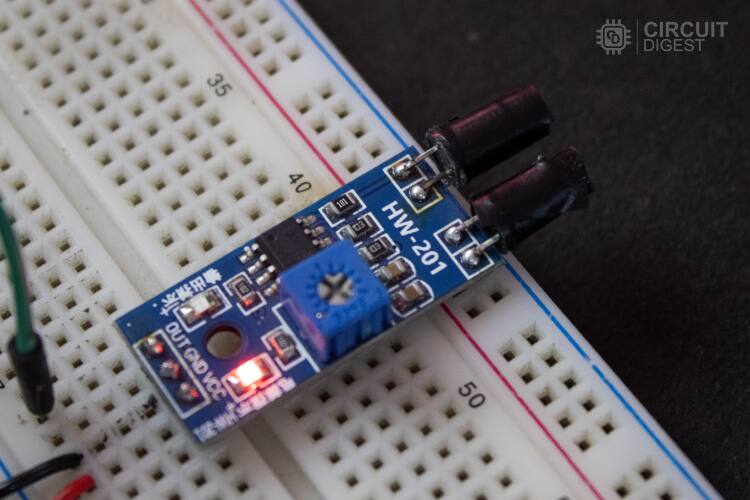
This precautionary measure makes our speed sensor work properly in ambient light conditions without any problem.
How to Use IR Sensor Modules to Measure Speed?
When measuring the speed of a moving object using two IR sensors, the process is based on calculating the time taken for a moving object to travel between those IR sensors. Later, speed can be calculated by dividing the distance traveled by the object by the time taken.
Basic Principle Behind IR Sensor Setup:
The two IR sensors are placed at a known distance apart from each other. When an object moves, it needs to pass the first IR sensor. While the object passes this first IR sensor, the first IR sensor signals Arduino to start the timer.
Later the object passes the second IR sensor, and while passing the second IR sensor signals Arduino to stop the timer; this finally helps us to calculate the time taken by the object to pass between the two IR sensors.
Moving Object Actual Speed Calculation:
We already know the existing distance between the two IR sensors. After calculating the time taken by the objects to pass between the IR sensors, we can easily able to calculate the speed of the moving object using the “Speed Formula.”.
Speed Calculation Formula:
The formula for calculating speed is given below.
Speed = Distance between Two IR sensors / Time taken by the object to travel between two IR sensors
For example, if the distance between the two IR sensors is 1 meter and the object takes 0.5 seconds to travel between them, then the speed would be
Speed = 1 meter / 0.4 seconds => 2.5 m/s
Arduino Code for Speed Sensor
In general, the following code makes Arduino to measure the speed of the moving object using two IR sensors and display the object speed in the I2C 16x2 LCD display. When the object triggers the first and second sensors, the respective sensor interrupts the Arduino to record the time for each event.
After that, Arduino calculates the speed using the distance between the two IR sensors and the time difference between the two IR sensors triggered time intervals. Later it displays the speed on the I2C LCD display.
#include <Wire.h>
#include <LiquidCrystal_I2C.h>
Here “Wire.h” and “LiquidCrystal_I2C.h” are included to make the Arduino UNO R3 board communicate with the I2C 16x2 LCD display and to control it easily without the need of writing complex hardware-specific libraries.
// Initialize the I2C LCD with the default address (usually 0x27 or 0x3F)
LiquidCrystal_I2C lcd(0x27, 16, 2);
This line sets the LCD object to communicate with a 16x2 LCD display module using the I2C protocol. Once initialized, we can use its object “lcd” object to interact with the display, such as showing text, clearing the screen, or adjusting the backlight, etc.
#define SENSOR_1_PIN 2 // Pin for first IR sensor (INT0)
#define SENSOR_2_PIN 3 // Pin for second IR sensor (INT1)
This line defines “SENSOR_1_PIN” as pin 2 and “SENSOR_2_PIN” as pin 3 on the Arduino board, where the two IR sensor signal pins get connected. Here Arduino’s pin 2 is associated with the external interrupt INT0, and pin 3 corresponds with the external interrupt INT1. By defining the pins using the macros instead of the variable, we can save certain memory space.
volatile unsigned long timeSensor1 = 0;
volatile unsigned long timeSensor2 = 0;
volatile bool sensor1Triggered = false;
volatile bool sensor2Triggered = false;
const float distanceBetweenSensors = 0.1; // Distance between sensors in meters
float speed = 0; // Speed in meters per second (m/s)
These variables store the timestamps when the IR sensors are triggered “timeSensor1” and “timeSensor2”. The variables “sensor1Triggered” and “sensor2Triggered” are used as a flag to track the sensor triggering status. The fixed distance between the two IR sensors is stored in the constant variable “distanceBetweenSensors,” and the calculated speed of the moving object gets stored in the “speed” variable.
void sensor1ISR() {
if (!sensor1Triggered) { // Ensure only the first trigger is recorded
timeSensor1 = micros(); // Record the time of sensor 1 trigger
sensor1Triggered = true;
}
}
void sensor2ISR() {
if (!sensor2Triggered) { // Ensure only the first trigger is recorded
timeSensor2 = micros(); // Record the time of sensor 2 trigger
sensor2Triggered = true;
}
}
This void sensor1ISR() and void sensor2ISR() both function as the interrupt service routines of the first IR sensor and the second IR sensor, respectively. These ISRs handle the detection of the IR sensor’s triggers. When an object crosses a sensor, the ISR records the time using the micros() function and sets a flag to avoid the multiple triggers getting processed.
void setup() {
// Initialize the LCD
lcd.init();
lcd.backlight();
lcd.setCursor(0, 0);
lcd.print("Speed Gun");
delay(2000); // Show the title for 2 seconds
lcd.clear();
pinMode(SENSOR_1_PIN, INPUT);
pinMode(SENSOR_2_PIN, INPUT);
// Attach interrupt for sensor 1 (INT0) and sensor 2 (INT1)
attachInterrupt(digitalPinToInterrupt(SENSOR_1_PIN), sensor1ISR, RISING);
attachInterrupt(digitalPinToInterrupt(SENSOR_2_PIN), sensor2ISR, RISING);
}
In this setup() function, the LCD gets initialized and displays the message “Speed Gun” for two seconds, then clears the screen using the predefined LCD libraries. The first and second sensor pins are set as inputs using the pinMode() function.
Later hardware interrupts are configured on the sensor pins using the “attachInterrupt()” function, so when a rising edge signal (IR sensor object detection signal) occurs, the respective interrupt service routine functions, namely “sensor1ISR()” and “sensor2ISR(),” are invoked. This setup() function ensures the system is ready to detect the object while it passes through both IR sensors without any latency in response time.
void loop() {
if (sensor1Triggered && sensor2Triggered) {
sensor1Triggered = false;
sensor2Triggered = false;
unsigned long timeDifference = abs(timeSensor2 - timeSensor1); // Time in microseconds
lcd.clear();
if (timeDifference > 0) {
speed = distanceBetweenSensors / (timeDifference / 1000000.0); // Convert µs to seconds
lcd.setCursor(0, 0);
lcd.print("Speed:");
lcd.setCursor(0, 1);
lcd.print(speed, 2); // Display speed with 2 decimal places
lcd.print(" m/s");
} else {
lcd.setCursor(0, 0);
lcd.print("Invalid Reading!");
}
}
}
In this loop() function, the code checks both IR sensors triggered flag status to know whether the object passes through it. If yes, then it starts to calculate the time difference using both IR sensors triggered time, and it computes the speed of the moving object using the known variables distanceBetweenSensors and timeDifference.
After that, the calculated speed is made to be displayed on the LCD screen with the two decimal places. If the time difference is invalid, it will print “Invalid Reading” on the display. The above process is repeated as long as both sensors are triggered.
That’s all about Arduino code. Let's feed this compiled binary code to the Arduino UNO R3 for converting the ordinary Arduino to a moving object speed-measuring device.
Car Speed Detector using Arduino and IR Sensor
That’s all; now we are in the final stage of testing our Arduino Speed Sensor project to measure the speed of moving objects. In the GIF below, you can see our project’s actual working.
Working Video
You can also find the complete project demonstration by clicking the video link below.
Other Arduino Projects Related to Speed Measuring
Previously we have built many Arduino Projects, I have listed a few projects that are related to measuring speed using Arduino. You can check them out if you want to build something similar next, all projects are well documented with circuit diagram and code for you to learn and build easily.
Analog Speedometer Using Arduino and IR Sensor
This project uses an IR sensor to measure the speed or RPM of a moving object, such as a motor or vehicle wheel. The speed is displayed in both analog and digital formats, making it useful for various applications.
This project utilizes an LM393 speed sensor module to track a robot’s speed, distance traveled, and turning angle. It helps in developing precise navigation systems for mobile robots.
DIY GPS Speedometer using Arduino and OLED
Using a GPS module, this project measures the speed of a moving vehicle and displays it on an OLED screen. It provides an easy way to create a digital speedometer without mechanical sensors.
DIY Speedometer using Arduino and Processing Android App
This project combines an Arduino, a hall sensor, and an Android app to measure and display vehicle speed. The data is sent to the app via Bluetooth, offering a modern and wireless speed monitoring solution.
Complete Project Code
#include <Wire.h>
#include <LiquidCrystal_I2C.h>
// Initialize the I2C LCD with the default address (usually 0x27 or 0x3F)
LiquidCrystal_I2C lcd(0x27, 16, 2);
#define SENSOR_1_PIN 2 // Pin for first IR sensor (INT0)
#define SENSOR_2_PIN 3 // Pin for second IR sensor (INT1)
volatile unsigned long timeSensor1 = 0;
volatile unsigned long timeSensor2 = 0;
volatile bool sensor1Triggered = false;
volatile bool sensor2Triggered = false;
const float distanceBetweenSensors = 0.1; // Distance between sensors in meters
float speed = 0; // Speed in meters per second (m/s)
void setup() {
// Initialize the LCD
lcd.init();
lcd.backlight();
lcd.setCursor(0, 0);
lcd.print("Speed Gun");
delay(2000); // Show the title for 2 seconds
lcd.clear();
pinMode(SENSOR_1_PIN, INPUT);
pinMode(SENSOR_2_PIN, INPUT);
// Attach interrupt for sensor 1 (INT0) and sensor 2 (INT1)
attachInterrupt(digitalPinToInterrupt(SENSOR_1_PIN), sensor1ISR, RISING);
attachInterrupt(digitalPinToInterrupt(SENSOR_2_PIN), sensor2ISR, RISING);
}
void loop() {
if (sensor1Triggered && sensor2Triggered) {
sensor1Triggered = false;
sensor2Triggered = false;
unsigned long timeDifference = abs(timeSensor2 - timeSensor1); // Time in microseconds
lcd.clear();
if (timeDifference > 0) {
speed = distanceBetweenSensors / (timeDifference / 1000000.0); // Convert µs to seconds
lcd.setCursor(0, 0);
lcd.print("Speed:");
lcd.setCursor(0, 1);
lcd.print(speed, 2); // Display speed with 2 decimal places
lcd.print(" m/s");
} else {
lcd.setCursor(0, 0);
lcd.print("Invalid Reading!");
}
}
}
void sensor1ISR() {
if (!sensor1Triggered) { // Ensure only the first trigger is recorded
timeSensor1 = micros(); // Record the time of sensor 1 trigger
sensor1Triggered = true;
}
}
void sensor2ISR() {
if (!sensor2Triggered) { // Ensure only the first trigger is recorded
timeSensor2 = micros(); // Record the time of sensor 2 trigger
sensor2Triggered = true;
}
}