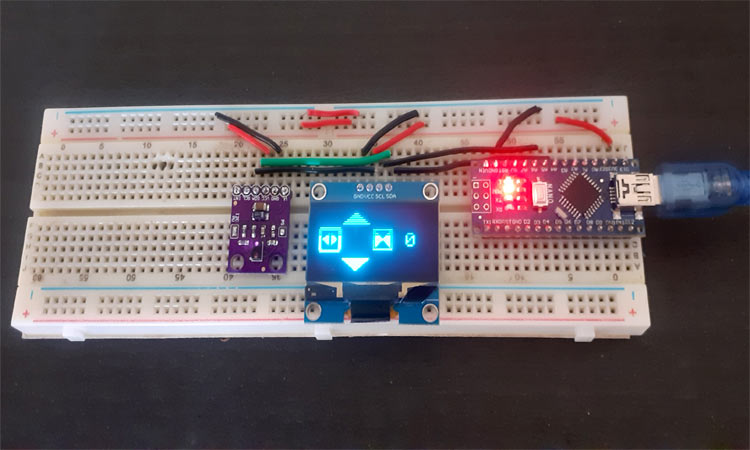
In this time of the Corona pandemic, elevators have become a high-risk place where everybody touches the same buttons. At many places, people have discovered tricks to prevent contact with elevator buttons like using a pumping paper, toothpicks, or sanitary tissues for pressing the lift buttons.
So in continuation of our previous Corona safety projects like Automatic sanitizer machine, contactless temperature monitoring, and Social distance detector, here we are going to build a Gesture controlled Elevator prototype using the Arduino Nano.
This Contactless Elevator is using Arduino Nano, an APDS9960 Gesture Sensor, and an OLED display module. With this gesture based control panel, you can easily control your Lift by making a hand gesture. The APDS9960 Sensor is used to read the gestures. UP and DOWN gestures are used to set the floor number, the left gesture is to close the lift door and move the lift according to the floor number and the Right gesture is used to open the door.
Components Required
- Arduino Nano
- OLED Display Module
- APDS9960 RGB & Gesture Sensor
- Breadboard
- Jumper Wires
APDS9960 RGB & Gesture Sensor
The APDS9960 RGB & Gesture Detection Module is a small breakout board that comes with a built-in APDS-9960 sensor, UV and IR blocking filters, four separate diodes sensitive to different directions, and an I2C compatible interface. This sensor can be used for ambient light and color measuring, proximity detection, and touchless gesture sensing. It has a gesture detection range of 10 to 20 cm and can be used to control a microcontroller, robot, and in many other projects.
Features:
- Operational Voltage: 2.4V to 3.6V
- Operating Range: 4-8in (10-20cm).
- I2C Interface (I2C Address: 0x39).
- Ambient Light and RGB Colour Sensing, Proximity
- Sensing, and Gesture Detection in an Optical Module
- I2C-bus Fast Mode Compatible Interface with Data Rates up to 400 kHz.
Circuit Diagram
Circuit Diagram for Contactless Elevator using APDS9960 is given below.
We are interfacing the Arduino Nano with APDS9960 Sensor and OLED Display. VCC and GND pins of both APDS9960 Sensor and OLED Display are connected to 3.3V and GND of Arduino. While SCL and SDA pins of APDS9960 Sensor and OLED Display are connected to A5 and A4 pins of Arduino Respectively.
OLED & APDS9960 Pin |
Arduino Nano Pin |
VCC |
3.3v |
GND |
GND |
SCL |
A5 |
SDA |
A4 |
This is how the complete setup for Gesture controlled Elevator using Arduino will look:
To learn more about OLED display and its interfacing with other microcontrollers, follow the link.
Code Explanation
The complete code for Contactless Elevator using APDS9960 is given at the end of the page. Here we are explaining some important parts of code. In this program, we are going to use the APDS9960 and Adafruit_SH1106 libraries. The APDS9960 library can be downloaded by from Arduino IDE. To download the library, go to Sketch > Library Manager > Search and then enter for Arduino APDS9960. While the Adafruit_SH1106 library can be downloaded from here.
So as usual start the code by including all the required libraries. Adafruit_SH1106.h is the modified version of the original Adafruit library.
#include <Wire.h> #include <Adafruit_GFX.h> #include <Adafruit_SH1106.h> #include <Arduino_APDS9960.h>
In the next lines, define the variables to store the current floor and floor number where the user wants to go.
int floornum=0; int currentfloor=0;
After that, enter the bitmaps for UP Arrow, Down Arrow, Open Door, and Close Door pictures. HEX code for an image can be generated by using a converter like Image2cpp. To learn more about how to use Image2cpp, follow this Arduino QR code generator tutorial.
const unsigned char up [] PROGMEM = { 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00,…………………………………………………………………………………….. }; const unsigned char down [] PROGMEM = { 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00,…………………………………………………………………………………….. }; const unsigned char dooropen [] PROGMEM = { 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x0f, 0xff, 0xff, 0xc0, 0x1f, 0xff, 0xff, 0xe0, ,…………………………………………………………………………………….. };
In the setup() function, initialize the Serial Monitor at a baud rate of 9600 for debugging purposes. Then in the next lines, initialize the OLED display and APDS9960 sensor with the begin() method as follows:
Serial.begin(9600); if (!APDS.begin()) { Serial.println("Error initializing APDS9960 sensor!"); } Serial.println("Detecting gestures ..."); display.begin(SH1106_SWITCHCAPVCC, 0x3C);
After initializing the display and sensor, clear the display buffer with the clearDisplay() method and set the font size and colour using the display.setTextSize() and display.setTextColor() methods.
display.setTextSize(2); display.setTextColor(WHITE); display.clearDisplay(); display.display();
Inside the void loop(), constantly check if any gesture was made. If yes, then read the gesture values and checks which gesture it is (UP, DOWN, RIGHT, LEFT) and prints the corresponding reading on the serial monitor. UP and DOWN gestures are used to set the floor number where the user wants to go to. The left gesture is to close the lift door and move the lift according to the floor number while the Right gesture is used to open the door.
if (APDS.gestureAvailable()) { int gesture = APDS.readGesture(); switch (gesture) { case GESTURE_UP: Serial.println("Detected UP gesture"); display.clearDisplay(); floornum ++; home1(); break; case GESTURE_DOWN: Serial.println("Detected DOWN gesture"); display.clearDisplay(); floornum --; home1(); break; case GESTURE_LEFT: Serial.println("Detected LEFT gesture"); display.clearDisplay(); start(); break; case GESTURE_RIGHT: Serial.println("Detected RIGHT gesture"); display.clearDisplay(); home1(); break; default: break; }
The home1() function is used to draw the home display for an elevator. This consists of an Up arrow, down arrow, Open Door, Close Door signs, and current floor number. drawBitmap() function is used to draw the images on the OLED display. The syntax for drawBitmap() function is given below:
drawBitmap(int16_t x, int16_t y, bitmap, int16_t w, int16_t h, colour);
Where:
int16_t x, int16_t y are X and Y coordinates of OLED display
the bitmap is the name of the bitmap
int16_t w, int16_t h are the height and weight of the image.
void home1() { display.setCursor(101,23); display.println(floornum); display.drawBitmap(23, 0, uparrow, 40, 18, WHITE); display.drawBitmap(26, 46, downarrow, 40, 18, WHITE); display.drawBitmap(0, 15, dooropen, 29, 30, WHITE); display.drawBitmap(60, 15, closedoor, 29, 30, WHITE); display.display(); }
The start() function is used to move the lift upwards or downwards. For that, the current floor no is compared with the floor number where the user wants to go. If the floor number is greater than the current floor no. then the lift will move upwards and if the floor number is lesser than the current floor no. then the lift will move downwards. The lift will stop when both the current floor no. and floor no. are the same.
void start() { while(floornum > currentfloor){ Serial.println("going UP "); currentfloor++; display.drawBitmap(0, 0, up, 100, 64, WHITE); display.setCursor(101,23); display.println(currentfloor); display.display(); display.clearDisplay(); delay(2000); } while(floornum < currentfloor){ Serial.println("going Down "); currentfloor--; display.drawBitmap(0, 0, down, 100, 64, WHITE); display.setCursor(101,23); display.println(currentfloor); display.display(); display.clearDisplay(); delay(2000); } if(floornum== currentfloor){ Serial.println("Reached"); display.clearDisplay(); home1(); Serial.print(currentfloor); } }
Testing the Gesture Controlled Touchless Lift
Once the hardware and code are ready, connect the Arduino Nano to the laptop and upload the complete code given below. As you can see by default OLED will display the Elevator UI.
Now wave off your hand up or down, like shown in the video below, to set the floor where you want to go. Then make the left gesture for acknowledging the lift to go to that floor. If you want to stop the lift, then make the right gesture with your hand.
The complete working video and code for this project are given below. Hope you enjoyed building this project. If you have any questions regarding this project, please leave them in the comment section.
Complete Project Code
#include <Wire.h>
#include <Adafruit_GFX.h>
#include <Adafruit_SH1106.h>
#include <Arduino_APDS9960.h>
#define OLED_RESET -1
Adafruit_SH1106 display(OLED_RESET);
int floornum=0;
int currentfloor=0;
//Paste your bitmap here
const unsigned char up [] PROGMEM = {
0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00,
0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00,
0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x38, 0x00, 0x00,
0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x7c, 0x00, 0x00, 0x00, 0x00, 0x00,
0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x01, 0xff, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00,
0x00, 0x00, 0x00, 0x03, 0xff, 0x80, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00,
0x0f, 0xff, 0xe0, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x1f, 0xff, 0xf0,
0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x7f, 0xff, 0xfc, 0x00, 0x00, 0x00,
0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0xff, 0xff, 0xfe, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00,
0x00, 0x00, 0x00, 0x03, 0xff, 0xc7, 0xff, 0x80, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00,
0x07, 0xff, 0x81, 0xff, 0xc0, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x1f, 0xfe, 0x00,
0xff, 0xf0, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x3f, 0xfc, 0x00, 0x3f, 0xf8, 0x00,
0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0xff, 0xf0, 0x00, 0x1f, 0xfe, 0x00, 0x00, 0x00, 0x00,
0x00, 0x00, 0x00, 0x01, 0xff, 0xe0, 0x00, 0x07, 0xff, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00,
0x07, 0xff, 0x80, 0x00, 0x03, 0xff, 0xc0, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x0f, 0xff, 0x00,
0x00, 0x00, 0xff, 0xe0, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x3f, 0xfc, 0x00, 0x00, 0x00, 0x7f,
0xf8, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x7f, 0xf8, 0x00, 0x00, 0x00, 0x1f, 0xfc, 0x00, 0x00,
0x00, 0x00, 0x00, 0x01, 0xff, 0xe0, 0x00, 0x00, 0x00, 0x0f, 0xff, 0x00, 0x00, 0x00, 0x00, 0x00,
0x03, 0xff, 0xc0, 0x00, 0x38, 0x00, 0x03, 0xff, 0x80, 0x00, 0x00, 0x00, 0x00, 0x0f, 0xff, 0x00,
0x00, 0x7c, 0x00, 0x01, 0xff, 0xe0, 0x00, 0x00, 0x00, 0x00, 0x1f, 0xfe, 0x00, 0x01, 0xff, 0x00,
0x00, 0x7f, 0xf0, 0x00, 0x00, 0x00, 0x00, 0x7f, 0xf8, 0x00, 0x03, 0xff, 0x80, 0x00, 0x3f, 0xfc,
0x00, 0x00, 0x00, 0x00, 0xff, 0xf0, 0x00, 0x0f, 0xff, 0xe0, 0x00, 0x0f, 0xfe, 0x00, 0x00, 0x00,
0x03, 0xff, 0xc0, 0x00, 0x1f, 0xff, 0xf0, 0x00, 0x07, 0xff, 0x80, 0x00, 0x00, 0x07, 0xff, 0x80,
0x00, 0x7f, 0xff, 0xfc, 0x00, 0x01, 0xff, 0xc0, 0x00, 0x00, 0x1f, 0xfe, 0x00, 0x00, 0xff, 0xff,
0xfe, 0x00, 0x00, 0xff, 0xf0, 0x00, 0x00, 0x3f, 0xfc, 0x00, 0x03, 0xff, 0xc7, 0xff, 0x80, 0x00,
0x3f, 0xf8, 0x00, 0x00, 0xff, 0xf0, 0x00, 0x07, 0xff, 0x81, 0xff, 0xc0, 0x00, 0x1f, 0xfe, 0x00,
0x01, 0xff, 0xe0, 0x00, 0x1f, 0xfe, 0x00, 0xff, 0xf0, 0x00, 0x07, 0xff, 0x00, 0x07, 0xff, 0x80,
0x00, 0x3f, 0xfc, 0x00, 0x3f, 0xf8, 0x00, 0x03, 0xff, 0xc0, 0x0f, 0xfe, 0x00, 0x00, 0xff, 0xf0,
0x00, 0x1f, 0xfe, 0x00, 0x00, 0xff, 0xe0, 0x3f, 0xfc, 0x00, 0x01, 0xff, 0xe0, 0x00, 0x07, 0xff,
0x00, 0x00, 0x7f, 0xf0, 0x1f, 0xf0, 0x00, 0x07, 0xff, 0x80, 0x00, 0x03, 0xff, 0xc0, 0x00, 0x1f,
0xf0, 0x0f, 0xe0, 0x00, 0x0f, 0xff, 0x00, 0x00, 0x00, 0xff, 0xe0, 0x00, 0x0f, 0xe0, 0x03, 0xc0,
0x00, 0x3f, 0xfc, 0x00, 0x00, 0x00, 0x7f, 0xf8, 0x00, 0x03, 0x80, 0x01, 0x00, 0x00, 0x7f, 0xf8,
0x00, 0x00, 0x00, 0x1f, 0xfc, 0x00, 0x01, 0x00, 0x00, 0x00, 0x01, 0xff, 0xe0, 0x00, 0x00, 0x00,
0x0f, 0xff, 0x00, 0x00, 0x00, 0x00, 0x00, 0x03, 0xff, 0xc0, 0x00, 0x00, 0x00, 0x03, 0xff, 0x80,
0x00, 0x00, 0x00, 0x00, 0x0f, 0xff, 0x00, 0x00, 0x00, 0x00, 0x01, 0xff, 0xe0, 0x00, 0x00, 0x00,
0x00, 0x1f, 0xfe, 0x00, 0x00, 0x00, 0x00, 0x00, 0x7f, 0xf0, 0x00, 0x00, 0x00, 0x00, 0x7f, 0xf8,
0x00, 0x00, 0x00, 0x00, 0x00, 0x3f, 0xfc, 0x00, 0x00, 0x00, 0x00, 0xff, 0xf0, 0x00, 0x00, 0x00,
0x00, 0x00, 0x0f, 0xfe, 0x00, 0x00, 0x00, 0x03, 0xff, 0xc0, 0x00, 0x00, 0x00, 0x00, 0x00, 0x07,
0xff, 0x80, 0x00, 0x00, 0x07, 0xff, 0x80, 0x00, 0x00, 0x00, 0x00, 0x00, 0x01, 0xff, 0xc0, 0x00,
0x00, 0x1f, 0xfe, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0xff, 0xf0, 0x00, 0x00, 0x3f, 0xfc,
0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x3f, 0xf8, 0x00, 0x00, 0xff, 0xf0, 0x00, 0x00, 0x00,
0x00, 0x00, 0x00, 0x00, 0x1f, 0xfe, 0x00, 0x01, 0xff, 0xc0, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00,
0x00, 0x07, 0xff, 0x00, 0x07, 0xff, 0x80, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x03, 0xff,
0xc0, 0x0f, 0xff, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0xff, 0xe0, 0x3f, 0xfc,
0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x7f, 0xf0, 0x1f, 0xf0, 0x00, 0x00, 0x00,
0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x1f, 0xf0, 0x0f, 0xe0, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00,
0x00, 0x00, 0x00, 0x0f, 0xe0, 0x03, 0xc0, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00,
0x03, 0x80, 0x01, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x01, 0x00, 0x00,
0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00,
0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00,
0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00,
0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00
};
const unsigned char down [] PROGMEM = {
// 'down, 105x64px
0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00,
0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00,
0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00,
0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00,
0x00, 0x00, 0x40, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x40, 0x00, 0xe0,
0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x01, 0xe0, 0x03, 0xf8, 0x00, 0x00, 0x00,
0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x03, 0xf0, 0x0f, 0xfc, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00,
0x00, 0x00, 0x00, 0x07, 0xf0, 0x0f, 0xff, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00,
0x1f, 0xf0, 0x03, 0xff, 0x80, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x7f, 0xf0, 0x01,
0xff, 0xe0, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0xff, 0xf0, 0x00, 0x7f, 0xf0, 0x00,
0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x01, 0xff, 0xc0, 0x00, 0x3f, 0xfc, 0x00, 0x00, 0x00, 0x00,
0x00, 0x00, 0x00, 0x07, 0xff, 0x80, 0x00, 0x0f, 0xfe, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00,
0x1f, 0xfe, 0x00, 0x00, 0x07, 0xff, 0x80, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x3f, 0xfc, 0x00,
0x00, 0x01, 0xff, 0xc0, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0xff, 0xf0, 0x00, 0x00, 0x00, 0xff,
0xf0, 0x00, 0x00, 0x00, 0x00, 0x00, 0x01, 0xff, 0xe0, 0x00, 0x00, 0x00, 0x3f, 0xf8, 0x00, 0x00,
0x00, 0x00, 0x00, 0x07, 0xff, 0x80, 0x00, 0x00, 0x00, 0x1f, 0xfe, 0x00, 0x00, 0x00, 0x00, 0x00,
0x0f, 0xff, 0x00, 0x00, 0x00, 0x00, 0x07, 0xff, 0x00, 0x00, 0x00, 0x00, 0x00, 0x3f, 0xfc, 0x00,
0x00, 0x00, 0x00, 0x03, 0xff, 0xc0, 0x00, 0x00, 0x00, 0x00, 0x7f, 0xf8, 0x00, 0x00, 0x00, 0x00,
0x00, 0xff, 0xe0, 0x00, 0x00, 0x00, 0x01, 0xff, 0xe0, 0x00, 0x00, 0x00, 0x00, 0x00, 0x7f, 0xf8,
0x00, 0x00, 0x00, 0x03, 0xff, 0xc0, 0x00, 0x00, 0x00, 0x40, 0x00, 0x1f, 0xfc, 0x00, 0x00, 0x00,
0x0f, 0xff, 0x00, 0x00, 0x40, 0x00, 0xe0, 0x00, 0x0f, 0xff, 0x00, 0x00, 0x00, 0x1f, 0xfe, 0x00,
0x01, 0xe0, 0x03, 0xf8, 0x00, 0x03, 0xff, 0x80, 0x00, 0x00, 0x7f, 0xf8, 0x00, 0x03, 0xf0, 0x0f,
0xfc, 0x00, 0x01, 0xff, 0xe0, 0x00, 0x00, 0xff, 0xf0, 0x00, 0x07, 0xf0, 0x0f, 0xff, 0x00, 0x00,
0x7f, 0xf0, 0x00, 0x03, 0xff, 0xc0, 0x00, 0x1f, 0xf0, 0x03, 0xff, 0x80, 0x00, 0x3f, 0xfc, 0x00,
0x07, 0xff, 0x80, 0x00, 0x3f, 0xf0, 0x01, 0xff, 0xe0, 0x00, 0x0f, 0xfe, 0x00, 0x1f, 0xfe, 0x00,
0x00, 0xff, 0xf0, 0x00, 0x7f, 0xf0, 0x00, 0x07, 0xff, 0x80, 0x3f, 0xfc, 0x00, 0x03, 0xff, 0xc0,
0x00, 0x3f, 0xfc, 0x00, 0x01, 0xff, 0xc0, 0xff, 0xf0, 0x00, 0x07, 0xff, 0x80, 0x00, 0x0f, 0xfe,
0x00, 0x00, 0xff, 0xf1, 0xff, 0xe0, 0x00, 0x1f, 0xfe, 0x00, 0x00, 0x07, 0xff, 0x80, 0x00, 0x3f,
0xff, 0xff, 0x80, 0x00, 0x3f, 0xfc, 0x00, 0x00, 0x01, 0xff, 0xc0, 0x00, 0x1f, 0xff, 0xff, 0x00,
0x00, 0xff, 0xf0, 0x00, 0x00, 0x00, 0xff, 0xf0, 0x00, 0x07, 0xff, 0xfc, 0x00, 0x01, 0xff, 0xe0,
0x00, 0x00, 0x00, 0x3f, 0xf8, 0x00, 0x03, 0xff, 0xf8, 0x00, 0x07, 0xff, 0x80, 0x00, 0x00, 0x00,
0x1f, 0xfe, 0x00, 0x00, 0xff, 0xe0, 0x00, 0x0f, 0xff, 0x00, 0x00, 0x00, 0x00, 0x07, 0xff, 0x00,
0x00, 0x7f, 0xc0, 0x00, 0x3f, 0xfc, 0x00, 0x00, 0x00, 0x00, 0x03, 0xff, 0xc0, 0x00, 0x1f, 0x00,
0x00, 0x7f, 0xf8, 0x00, 0x00, 0x00, 0x00, 0x00, 0xff, 0xe0, 0x00, 0x0e, 0x00, 0x01, 0xff, 0xe0,
0x00, 0x00, 0x00, 0x00, 0x00, 0x7f, 0xf8, 0x00, 0x00, 0x00, 0x03, 0xff, 0xc0, 0x00, 0x00, 0x00,
0x00, 0x00, 0x1f, 0xfc, 0x00, 0x00, 0x00, 0x0f, 0xff, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x0f,
0xff, 0x00, 0x00, 0x00, 0x1f, 0xfe, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x03, 0xff, 0x80, 0x00,
0x00, 0x7f, 0xf8, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x01, 0xff, 0xe0, 0x00, 0x00, 0xff, 0xf0,
0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x7f, 0xf0, 0x00, 0x03, 0xff, 0xc0, 0x00, 0x00, 0x00,
0x00, 0x00, 0x00, 0x00, 0x3f, 0xfc, 0x00, 0x07, 0xff, 0x80, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00,
0x00, 0x0f, 0xfe, 0x00, 0x1f, 0xfe, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x07, 0xff,
0x80, 0x3f, 0xfc, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x01, 0xff, 0xc0, 0xff, 0xf0,
0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0xff, 0xf1, 0xff, 0xe0, 0x00, 0x00, 0x00,
0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x3f, 0xff, 0xff, 0x80, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00,
0x00, 0x00, 0x00, 0x1f, 0xff, 0xff, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00,
0x07, 0xff, 0xfc, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x03, 0xff, 0xf8,
0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0xff, 0xe0, 0x00, 0x00, 0x00,
0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x7f, 0xc0, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00,
0x00, 0x00, 0x00, 0x00, 0x00, 0x1f, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00,
0x00, 0x00, 0x0e, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00,
0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00,
0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00
};
const unsigned char uparrow [] PROGMEM = {
0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00,
0x00, 0x0e, 0x00, 0x00, 0x00, 0x00, 0x1f, 0x00, 0x00, 0x00, 0x00, 0x3f, 0x80, 0x00, 0x00, 0x00,
0xff, 0xc0, 0x00, 0x00, 0x01, 0xff, 0xf0, 0x00, 0x00, 0x03, 0xff, 0xf8, 0x00, 0x00, 0x07, 0xff,
0xfc, 0x00, 0x00, 0x1f, 0xff, 0xff, 0x00, 0x00, 0x3f, 0xff, 0xff, 0x80, 0x00, 0xff, 0xff, 0xff,
0xc0, 0x01, 0xff, 0xff, 0xff, 0xf0, 0x03, 0xff, 0xff, 0xff, 0xf8, 0x03, 0xff, 0xff, 0xff, 0xf8,
0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00
};
const unsigned char downarrow [] PROGMEM = {
0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x1f, 0xff, 0xff, 0xff, 0xc0, 0x1f,
0xff, 0xff, 0xff, 0xc0, 0x0f, 0xff, 0xff, 0xff, 0x80, 0x03, 0xff, 0xff, 0xff, 0x00, 0x01, 0xff,
0xff, 0xfc, 0x00, 0x00, 0xff, 0xff, 0xf8, 0x00, 0x00, 0x3f, 0xff, 0xe0, 0x00, 0x00, 0x1f, 0xff,
0xc0, 0x00, 0x00, 0x0f, 0xff, 0x80, 0x00, 0x00, 0x03, 0xff, 0x00, 0x00, 0x00, 0x01, 0xfc, 0x00,
0x00, 0x00, 0x00, 0xf8, 0x00, 0x00, 0x00, 0x00, 0x70, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00,
0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00
};
const unsigned char dooropen [] PROGMEM = {
0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x0f, 0xff, 0xff, 0xc0, 0x1f, 0xff, 0xff, 0xe0,
0x3f, 0xff, 0xff, 0xe0, 0x3f, 0xff, 0xff, 0xf0, 0x38, 0x00, 0x00, 0xf0, 0x38, 0x00, 0x00, 0xf0,
0x38, 0x00, 0x00, 0xf0, 0x38, 0x04, 0x80, 0xf0, 0x38, 0x1c, 0xc0, 0xf0, 0x38, 0x3c, 0xf0, 0xf0,
0x38, 0x7c, 0xf8, 0xf0, 0x39, 0xfc, 0xfc, 0xf0, 0x3b, 0xfc, 0xfe, 0xf0, 0x3b, 0xfc, 0xfe, 0xf0,
0x38, 0xfc, 0xfc, 0xf0, 0x38, 0x7c, 0xf8, 0xf0, 0x38, 0x3c, 0xf0, 0xf0, 0x38, 0x1c, 0xc0, 0xf0,
0x38, 0x04, 0x80, 0xf0, 0x38, 0x00, 0x00, 0xf0, 0x38, 0x00, 0x00, 0xf0, 0x38, 0x00, 0x00, 0xf0,
0x3c, 0x00, 0x00, 0xf0, 0x3f, 0xff, 0xff, 0xe0, 0x1f, 0xff, 0xff, 0xe0, 0x1f, 0xff, 0xff, 0xc0,
0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00
};
const unsigned char closedoor [] PROGMEM = {
0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00,
0x0f, 0xff, 0xff, 0xc0, 0x0f, 0xff, 0xff, 0xc0, 0x08, 0x00, 0x00, 0x40, 0x0a, 0x00, 0x03, 0x40,
0x0b, 0x80, 0x07, 0x40, 0x0b, 0xc0, 0x0f, 0x40, 0x0b, 0xe0, 0x1f, 0x40, 0x0b, 0xf0, 0x3f, 0x40,
0x0b, 0xf8, 0x7f, 0x40, 0x0b, 0xfc, 0xff, 0x40, 0x0b, 0xff, 0xff, 0x40, 0x0b, 0xff, 0xff, 0x40,
0x0b, 0xfe, 0xff, 0x40, 0x0b, 0xf8, 0x7f, 0x40, 0x0b, 0xf0, 0x3f, 0x40, 0x0b, 0xe0, 0x1f, 0x40,
0x0b, 0xc0, 0x0f, 0x40, 0x0b, 0x80, 0x07, 0x40, 0x0b, 0x00, 0x03, 0x40, 0x08, 0x00, 0x01, 0x40,
0x0f, 0xff, 0xff, 0xc0, 0x0f, 0xff, 0xff, 0xc0, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00,
0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00
};
void setup(){
Serial.begin(9600);
if (!APDS.begin()) {
Serial.println("Error initializing APDS9960 sensor!");
}
Serial.println("Detecting gestures ...");
display.begin(SH1106_SWITCHCAPVCC, 0x3C);
display.setTextSize(2);
display.setTextColor(WHITE);
display.clearDisplay();
display.display();
home1();
}
void loop() {
display.clearDisplay();
if (APDS.gestureAvailable()) {
int gesture = APDS.readGesture();
switch (gesture) {
case GESTURE_UP:
Serial.println("Detected UP gesture");
display.clearDisplay();
floornum ++;
home1();
break;
case GESTURE_DOWN:
Serial.println("Detected DOWN gesture");
display.clearDisplay();
floornum --;
home1();
break;
case GESTURE_LEFT:
Serial.println("Detected LEFT gesture");
display.clearDisplay();
start();
break;
case GESTURE_RIGHT:
Serial.println("Detected RIGHT gesture");
display.clearDisplay();
home1();
break;
default:
break;
}
}
}
void home1()
{
display.setCursor(101,23);
display.println(floornum);
display.drawBitmap(23, 0, uparrow, 40, 18, WHITE);
display.drawBitmap(26, 46, downarrow, 40, 18, WHITE);
display.drawBitmap(0, 15, dooropen, 29, 30, WHITE);
display.drawBitmap(60, 15, closedoor, 29, 30, WHITE);
display.display();
}
void start()
{
while(floornum > currentfloor){
Serial.println("going UP ");
currentfloor++;
display.drawBitmap(0, 0, up, 100, 64, WHITE);
display.setCursor(101,23);
display.println(currentfloor);
display.display();
display.clearDisplay();
delay(2000);
}
while(floornum < currentfloor){
Serial.println("going Down ");
currentfloor--;
display.drawBitmap(0, 0, down, 100, 64, WHITE);
display.setCursor(101,23);
display.println(currentfloor);
display.display();
display.clearDisplay();
delay(2000);
}
if(floornum== currentfloor){
Serial.println("Reached");
display.clearDisplay();
home1();
Serial.print(currentfloor);
}
}
Comments
I used the code given above
I used the code given above and it says low memory available. This is causing the OLED to switch off in between. How do I fix this?
Hallo sir, can you please upload full specifications of components.