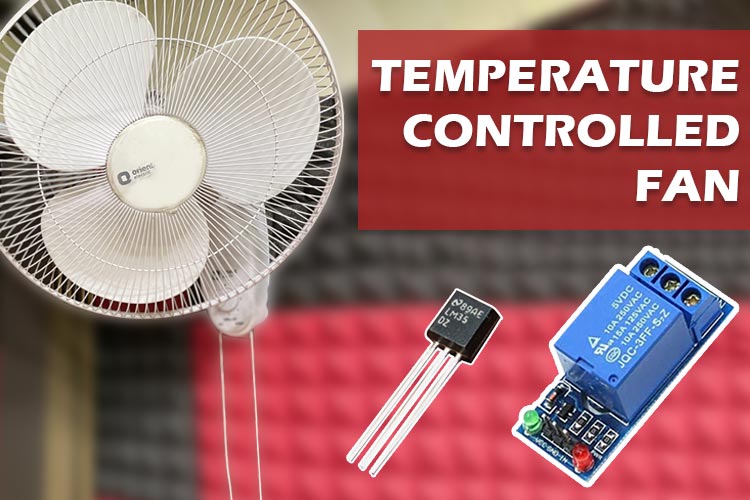
In today's fast-paced world, convenience is key. Dealing with the temperature fluctuations in your living space can be quite a hassle. For those who live in regions where the afternoons can be sweltering hot and the nights chillingly cold, constantly adjusting your wall fan can be annoying. However, with a little bit of DIY ingenuity and the help of some electronic components, you can transform your ordinary electric fan into an automatic one. In this temperature controlled fan project, we'll guide you through the process of converting your Temperature Controlled Fan using an Arduino Uno, LM35 temperature sensor, and a relay.
In this project we will be controlling an AC fan based on room temperature, previously we have built many similar projects like a simple automatic temperature controlled fan, a Temperature Based Fan Speed Controller using Arduino, IoT based Fan speed control, AC Fan Speed control using Smart phone you can also check them out if you are interested.
How to build a Temperature Controlled Fan using Arduino and LM35?
We will be building this temp controlled fan step by step. We will first interface the LM35 sensor with Arduino UNO to measure the surrounding temperature and relay to control the fan. We will then upload the conditional code in Arduino and check the output.
Components to Make a Temperature Controlled Fan
- Arduino
- Usb cable
- LM35 temperature sensor
- 5V single channel Relay
- Jumper wires
- Breadboard
- An AC Fan
LM35 Temperature Sensor Pinout
The LM35 is a temperature sensor integrated circuit that is widely used to measure temperature in electronic circuits and various applications. It is known for its simplicity and accuracy, making it a popular choice for temperature sensing in many projects. The LM35 is designed to provide a linear analog output voltage that is directly proportional to the temperature it senses.
The LM35 temperature sensor has 3 pins –VCC, GND and Analog Output Pin. Each of these pins has a separate function which is mentioned below.
VCC Pin : This is the power pin of the sensor which can be connected to a power supply which is between 4V and 32V.
GND Pin : The gounrd pin is connected to the ground of the circuit.
OUT Pin : It gives analog output with a 10 bit ADC which means the value will be between 0-1024 and the output voltage on this pin is directly proportional to the temperature.
5V Single Channel Relay Pinout
A 5V relay refers to an electromagnetic relay that operates with a 5-volt control voltage and has a single switching channel. This type of relay is commonly used in various electronic and electrical circuits to control high-power devices or loads using a low-power signal, typically from a microcontroller or other digital control source.
A single channel relay is a cost effective method to control high voltage high current load using a low voltage, low current load. It is used to control loads such as motors, fans, lamps and other similar AC loads
VCC: Supplies power to the module. The input voltage is 5V
GND: Serves as the common ground.
IN: The control pin for managing the relay's output. It is where we give “ON” or “OFF” signal from the microcontroller.
COM:This is the common PIN of the relay module.
NC: Normally connected to COM, unless the relay is activated to disconnect.
NO: Normally open, switches to COM when the relay is activated.
Note: The pin sequence might vary in some relay modules, so refer to the PCB annotations or datasheet for correct connections.
Temperature Controlled Fan Circuit Diagram
The complete circuit to build the temperature controlled fan using arduino and lm35 project is given below, we have used fritzing software for making most of the circuit diagrams. The fan and the plug were later added using photoshop. The diagram consists of an arduino,a relay, an lm35 sensor, an AC fan, and a plug.
The temperature controlled fan circuit is very simple. The LM35 sensor has 3 pins VCC, GND and Analog output pin. The VCC pin will be connected to the 5V pin of Arduino. The GND pin will be connected to the GND pin and the Analog output pin will be connected to the A0 pin of the Arduino.
The relay pins also have 3 pins - VCC, GND and Digital Input pin. The VCC will be connected to the 5V pin of Arduino. The GND pin will be connected to the GND pin and the Digital Input pin of Arduino will be connected to pin 2 of Arduino.
Working of Automatic Temperature Controlled Fan
The described project is a temperature control system that utilizes an LM35 temperature sensor, an Arduino microcontroller, and a relay to regulate an AC fan based on the ambient temperature. The LM35 sensor plays a crucial role in the system by continuously monitoring the temperature of the surrounding environment. It provides an analog output voltage that is directly proportional to the temperature it senses. This analog value is then sent to the Arduino for processing. The Arduino takes this input, converts it into degrees Celsius, and compares it to a predefined threshold value, such as 40 degrees Celsius.
In the first scenario, if the temperature reading from the LM35 exceeds the specified threshold (40 degrees Celsius in this case), the Arduino triggers the relay to turn the AC fan "ON." This action provides a cooling mechanism to maintain the temperature within the desired range. In the second scenario, if the temperature remains below the threshold, the Arduino refrains from sending any signal to the relay, thereby keeping the fan "OFF." This automation ensures that the fan operates only when necessary, conserving energy and ensuring the environment remains at a comfortable temperature.
Temperature Controlled Fan using Arduino Code Explanation
The complete Arduino code for Temperature controlled fan is given at the bottom of this page. You can also get the code from CircuitDigest Github page or from the link given below.
the code explanation of the Temperature controlled Fan project is as follows
const int lm35_pin = A0; // LM35 output pin const int relay_pin = 2; // Relay control pin (change to the appropriate pin)
These two lines declare two integer constants. lm35_pin is assigned the value A0, which is often used to represent an analog input pin on an Arduino. relay_pin is assigned the value 2, which is typically used to specify a digital output pin.
void setup() { Serial.begin(9600); pinMode(relay_pin, OUTPUT); // Set the relay pin as an output digitalWrite(relay_pin, LOW); // Turn off the relay (fan) }
The setup() function is a standard Arduino function that is called once when the program starts. In this function:
Serial.begin(9600); initializes serial communication at a baud rate of 9600 bits per second. This is used for sending data to the serial monitor for debugging and monitoring.
pinMode(relay_pin, OUTPUT); configures the relay_pin as an output, which means it will be used to control a device (likely a fan) by sending digital signals.
digitalWrite(relay_pin, LOW); sets the relay_pin to LOW, which means the relay is turned off initially.
void loop() { int temp_adc_val; float temp_val; temp_adc_val = analogRead(lm35_pin); // Read temperature temp_val = (temp_adc_val * 4.88); // Convert ADC value to equivalent voltage temp_val = (temp_val / 10); // LM35 gives an output of 10mV/°C Serial.print("Temperature = "); Serial.print(temp_val); Serial.println(" Degree Celsius"); if (temp_val < 40) { Serial.println("Fan off"); digitalWrite(relay_pin, LOW); // Turn off the relay (fan) } else { Serial.println("Fan on"); digitalWrite(relay_pin, HIGH); // Turn on the relay (fan) } }
The loop() function is another standard Arduino function that runs continuously after the setup() function. In this function:
int temp_adc_val; and float temp_val; declare integer and floating-point variables for storing temperature-related values.
temp_adc_val = analogRead(lm35_pin); reads the analog voltage from the LM35 temperature sensor connected to the lm35_pin (analog input A0). The LM35 sensor provides an analog voltage proportional to the temperature.
temp_val = (temp_adc_val * 4.88); scales the analog reading to obtain the voltage in millivolts. This assumes that the LM35 provides 10 mV per degree Celsius.
temp_val = (temp_val / 10); converts the voltage to the temperature in degrees Celsius by dividing by 10.
Several Serial.print and Serial.println statements are used to display the temperature value and a message in the Serial Monitor for monitoring.
An if statement checks if the temperature (temp_val) is less than 40 degrees Celsius. If it is, it prints "Fan off" and sets the relay_pin to LOW, turning off the relay (likely connected to a fan). If the temperature is greater than or equal to 40 degrees Celsius, it prints "Fan on" and sets the relay_pin to HIGH, turning on the relay (fan). This code is essentially controlling the fan based on the temperature readings from the LM35 sensor.
The code continuously loops, reading the temperature, and controlling the fan based on the temperature threshold specified in the if statement.
Temperature Controlled Fan using Arduino Project Demonstration
So, as you can see. As the temperature of LM35 rises above 40, the fan turns “ON” and as the temperature reduces below that threshold, it turns “OFF”.
Converting your manual electric fan into an automatic one is a simple yet awesome way to enhance your comfort and convenience and have some fun. We hope this temperature controlled fan using arduino project report has been informative and helpful in your quest for a more comfortable living space.
Complete Project Code
//Temperature Controlled Fan using Arduino and Lm35 Code
//by Circuirdigest
const int lm35_pin = A0; // LM35 output pin
const int relay_pin = 2; // Relay control pin (change to the appropriate pin)
void setup() {
Serial.begin(9600);
pinMode(relay_pin, OUTPUT); // Set the relay pin as an output
digitalWrite(relay_pin, LOW); // Turn off the relay (fan)
}
void loop() {
int temp_adc_val;
float temp_val;
temp_adc_val = analogRead(lm35_pin); // Read temperature
temp_val = (temp_adc_val * 4.88); // Convert ADC value to equivalent voltage
temp_val = (temp_val / 10); // LM35 gives an output of 10mV/°C
Serial.print("Temperature = ");
Serial.print(temp_val);
Serial.println(" Degree Celsius");
if (temp_val < 40) {
Serial.println("Fan off");
digitalWrite(relay_pin, LOW); // Turn off the relay (fan)
} else {
Serial.println("Fan on");
digitalWrite(relay_pin, HIGH); // Turn on the relay (fan)
}
}