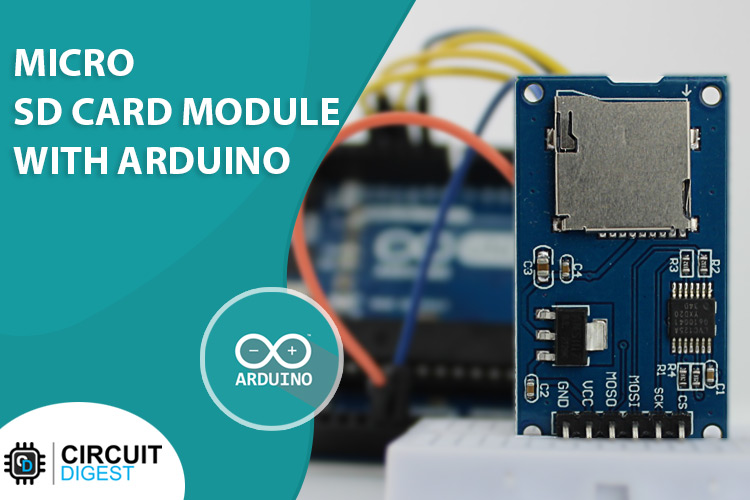
If you are an embedded engineer and working in the electronic industry, every once in a while there will be situations where you need to log and store a huge amount of data that the internal memory of an Arduino can handle, examples could be like any logger project like battery energy logger, Temperature logger or GPS Tracker. The solution to this problem is to use an SD card or micro sd card that packs gigabytes of data and its size is smaller than a one rupee coin. So in this tutorial, we decided to interface the SD Card module with Arduino and we will let you know all the details. So without further ado let's get right into it.
Micro SD Card Module Pinout
The Micro SD Card module has 6 pins; those are GND, VCC, MISO, MOSI, SCK, and CS. All the pins of this sensor module are digital, except VCC and Ground. The Pinout of a Micro SD Card Module is shown below:
GND is the ground pin of the micro sd card module and it should be connected to the ground pin of the Arduino.
VCC is the power supply pin of the micro sd card module that can be connected to 5V or 3.3V of the supply.
MISO Stands for Master In Slave Out. This is the SPI data out from the SD Card Module.
MOSI Stands for Master Out Slave In. This is the input pin of the SD Card Module.
SCK Stands for Serial Clock as the name implies it is the data synchronization pulse generated by the Arduino.
CS Stands for Chip Select, this pin can be controlled by the Arduino to enable or disable the module.
Micro SD Card Module – Parts
This is a cheap and easy-to-use sensor that can be used for many different applications. This sensor can be used to read SD card data with microcontroller. The parts marking of the SD Card Module is shown below.
If you take a close look at the Micro SD Card module, there is not much on the PCB itself. There are only three components that are significant, first is the Micro SD Card Holder Itself. This holder makes it easy for us to swap between different SD card modules. The second most important thing is the level shifter IC as the SD card module runs only on 3.3V and it has a maximum operating voltage of 3.6V so if we directly connect the SD card to 5V it will definitely kill the SD card. Also, the module has an onboard ultra-low dropout regulator that will convert the voltage from 5V to 3.3V. That is also why this module can operate on 3.3V power.
Commonly Asked Questions about Micro SD Card Module
Which format is best for an SD card?
When formatting SD cards your best choice is to format them using exFAT.
Do SD cards have firmware?
SD cards are preprogrammed with firmware. Firmware contains the instructions in ROM (read-only memory) for the device and enables the device to "boot up."
Does formatting SD cards shorten life?
The simple and straightforward answer to this question is yes it shortens the life of the SD Card. Format goes through the entire card, clears the data, and marks the blocks as available. Since cards have a finite amount of read/write, formatting can shorten the life of a card.
What is an exFAT SD card?
exFAT (Extensible File Allocation Table) is a file system introduced by Microsoft in 2006 and optimized for flash memory such as USB flash drives and SD cards. exFAT was proprietary until 28 August 2019, when Microsoft published its specification. Microsoft owns patents on several elements of its design.
Circuit Diagram for Micro SD Card Module
The module is made with very generic and readily available components. The Schematic Diagram of the Micro SD Card Module is shown below.
As you can see in the above schematic, we have the Micro SD Card connector which is a push-out type connector and the connector is connected to a logic level shifter IC. The maximum operating voltage of the module is 3.6V so the logic level shifter IC becomes very important. To power the SD card and the logic level converter, we are using a LM1117 LDO which is why this module can work with both 3.3V and 5V logic levels. The connector JP1 at the bottom of the schematic represents the connector at the bottom of the micro SD card module.
Arduino Micro SD Card Module Circuit Connection Diagram
Now that we have completely understood how a Micro SD Card Module works, we can connect all the required wires to the Arduino and write the code to get all the data out from the sensor. The Connection Diagram of the Micro SD Card Module with Arduino is shown below-
Connecting the Micro SD Card module to the Arduino UNO is very simple we just need to connect the SPI lines that is SCK, MISO, and MOSI, to the Arduinos SPI line that is SCK(D13), MOSI(D12), and MISO(D11) and if there are multiple devices connected on the SPI bus then we also need to connect the CS line to the Arduino. Other than that the VCC and Ground are used to power the device.
Preparing the Micro SD Card Module
Before inserting the SD Card into the SD card reader module, you need to properly format the card before you can actually work with it, otherwise, you would have problems because the SD card reader module can only read FAT16 or FAT32 file systems. If your sd card is new then chances are that the card is factory formatted, that may also not work because a pre-formatted card comes with a FAT file system, either way, it's better to format the old card to reduce issues during operation.
Arduino Code for Interfacing Micro SD Card Module with Arduino
The Arduino to read and write data from the SD card module is shown below. The code is very simple and easy to understand. The code checks if there exists a file name “data_log.txt” if the file exists then it proceeds to write the given information onto the SD card and if not, the code will create the file in the SD card and then write the data onto the sd card. We can later open the file and check the contents if it's written or not.
We start our code by defining the SD library and we also declare the chipSelect pin of the module, next we define a file type variable and name that myFile.
#include <SD.h> const int chipSelect = 10; File myFile;
Next, we have our setup function; in the setup function, we initialize the serial for debugging. We also check if the SD card is connected properly to the SPI pins of the Arduino.
// Open serial communications and wait for port to open: Serial.begin(9600); // wait for Serial Monitor to connect. Needed for native USB port boards only: while (!Serial);
Next, we have made a function named “check_and_create_file()”. In that function, we check if data_log.txt file exists in the SD card or not, if the file exists, we will proceed with our code and if not, then we will create the file. To create a file on the SD card you just need to open and close it very rapidly without a delay and the file will be created.
void check_and_create_file() { Serial.print("Initializing SD card..."); /*Check if the SD card exist or not*/ if (!SD.begin(chipSelect)) { Serial.println("initialization failed!"); while (1); } Serial.println("initialization done."); if (SD.exists("data_log.txt")) Serial.println("data_log.txt exists."); else { Serial.println("data_log.txt doesn't exist."); /* open a new file and immediately close it: this will create a new file */ Serial.println("Creating data_log.txt..."); myFile = SD.open("data_log.txt", FILE_WRITE); myFile.close(); /* Now Check again if the file exists in the SD card or not */ if (SD.exists("data_log.txt")) Serial.println("data_log.txt exists."); else Serial.println("data_log.txt doesn't exist."); } }
Next, we will write some text to the SD card and we will also read that text back into the serial monitor window. This way we will know that the text was written successfully on the SD card. We have made a function named read_write_sd_text() which does this automatically. Just call this function and you are done.
Inside the function, we open a
void read_write_sd_text() { myFile = SD.open("data_log.txt", FILE_WRITE); // if the file opened okay, write to it: if (myFile) { Serial.print("Writing to data_log.txt..."); myFile.println("Testing Data Write 1, 2, 3."); // close the file: myFile.close(); Serial.println("done."); } else { // if the file didn't open, print an error: Serial.println("error opening data_log.txt"); } // re-open the file for reading: myFile = SD.open("data_log.txt"); if (myFile) { Serial.println("data_log.txt:"); // read from the file until there's nothing else in it: while (myFile.available()) { Serial.write(myFile.read()); } // close the file: myFile.close(); } else { // if the file didn't open, print an error: Serial.println("error opening data_log.txt"); } }
This marks the end of our code section and we can move to another section of the article.
Working of the Micro SD Card Module
The gif below shows how the SD Card module works. We wrote the arduino code so that after the arduino initializes the code will check if it can find a SD card or not. If it finds an SD card it will check if the text file exists or not if the file exists the code will continue, if not the code will simply create a new text file and once that is done it will write testing 1, 2, 3. Inside the text file. Next the code will read the text file and print the data inside the text into the serial monitor window.
Troubleshooting Micro SD Card Module
- Check if the power supply to the module is working properly or not. Check whether the onboard LM1117-3.3V voltage regulator is working properly or not.
- Format the Micro SD card before inserting the card into the SD card module.
- Sometimes quick format in windows systems does not work, so you need to format the card using regular format method or you can use the Windows CMD to format the micro sd card.
- If you are using an old micro sd card make sure that the contact of the SD card is clean.
Projects using Arduino and Micro SD Card Module
If you are searching for PIC microcontroller based projects, this project could be what you are looking for because in this project we have used a PIC18F46K22 and SD Card module to build an example that can save text data onto an SD Card Module.
If you are searching the internet for a project on data logger then this project could be for you because in this project we have interfaced a SD Card module with Arduino and stored Temperature, Humidity, and Time on a SD Card module.
If you are interested in building yourself a digital infrared thermometer then this project is for you. In this project, we have not only built a thermometer but we have also used a SD Card Module to store the data in a SD card module so that we could recall the data if needed.
Supporting Files
Complete Project Code
#include <SD.h>
const int chipSelect = 10;
File myFile;
void setup() {
// Open serial communications and wait for port to open:
Serial.begin(9600);
// wait for Serial Monitor to connect. Needed for native USB port boards only:
while (!Serial);
check_and_create_file();
write_text();
}
void loop() {
// nothing happens after setup finishes.
}
void check_and_create_file()
{
Serial.print("Initializing SD card...");
/*Check if the SD card exist or not*/
if (!SD.begin(chipSelect)) {
Serial.println("initialization failed!");
while (1);
}
Serial.println("initialization done.");
if (SD.exists("data_log.txt"))
Serial.println("data_log.txt exists.");
else
{
Serial.println("data_log.txt doesn't exist.");
/* open a new file and immediately close it:
this will create a new file */
Serial.println("Creating data_log.txt...");
myFile = SD.open("data_log.txt", FILE_WRITE);
myFile.close();
/* Now Chec agin if the file exists in
the SD card or not */
if (SD.exists("data_log.txt"))
Serial.println("data_log.txt exists.");
else
Serial.println("data_log.txt doesn't exist.");
}
}
void write_text()
{
myFile = SD.open("data_log.txt", FILE_WRITE);
// if the file opened okay, write to it:
if (myFile) {
Serial.print("Writing to data_log.txt...");
myFile.println("testing 1, 2, 3.");
// close the file:
myFile.close();
Serial.println("done.");
} else {
// if the file didn't open, print an error:
Serial.println("error opening data_log.txt");
}
// re-open the file for reading:
myFile = SD.open("data_log.txt");
if (myFile) {
Serial.println("data_log.txt:");
// read from the file until there's nothing else in it:
while (myFile.available()) {
Serial.write(myFile.read());
}
// close the file:
myFile.close();
} else {
// if the file didn't open, print an error:
Serial.println("error opening data_log.txt");
}
}