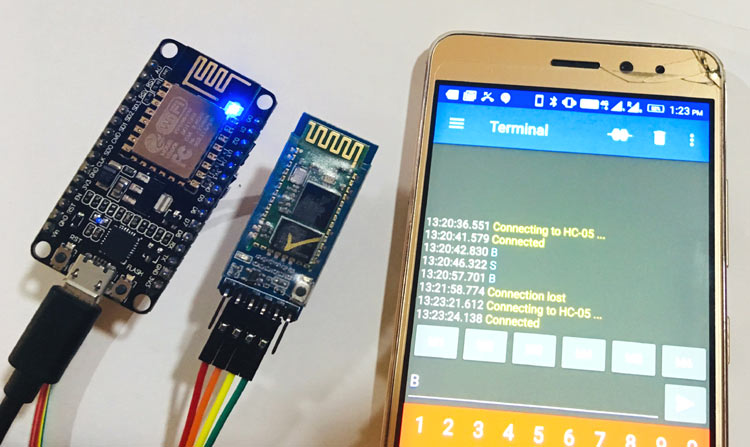
Now a days, the Bluetooth has become integral part of digital devices and it comes inbuilt in most of the devices such as Smartphone, Laptop, PC, Camera, Watches, Fitness Bands and many more. Bluetooth has always been dominating protocol in the wireless communication ever since it was discovered. Although Bluetooth Technology is fundamentally a cable replacement system but it also leverages a universal bridge to existing data networks and an ad hoc connection mechanism for number of devices in various configurations. We have used Bluetooth module HC05 and HC06 with many other microcontrollers to make them communicate wirelessly:
- Interfacing Bluetooth HC-05 with STM32F103C8 Blue Pill: Controlling LED
- Interfacing HC-05 Bluetooth module with AVR Microcontroller
- Android Controlled Robot using 8051 Microcontroller
- Controlling Raspberry Pi GPIO using Android App over Bluetooth
- Bluetooth Controlled Toy Car using Arduino
Today we will interface HC-05 Bluetooth Module with popular Wi-Fi module ESP8266 and control an LED wirelessly by sending commands via Bluetooth. This LED can be replaced by a Relay and an AC appliance to build a Home Automation Application.
Components Required
Hardware:
- NodeMCU ESP8266
- HC-05 Bluetooth Module
Software:
- Arduino IDE
- Serial Bluetooth Terminal (Android App): To Monitor Bluetooth Data on Smart Phone.
ESP8266 HC-05 Bluetooth Module Circuit Diagram
Circuit diagram to connect Bluetooth module HC-05 with NodeMCU ESP8266 is very simple and shown below:
An external Bluetooth module is needed with ESP8266 as it doesn’t have inbuilt Bluetooth like ESP32. ESP32 has inbuilt Bluetooth Low Energy (BLE) and Classic Bluetooth, on which we have previously covered few tutorials:
- ESP32 BLE Server - GATT Service for Battery Level Indication
- ESP32 BLE Client – Connecting to Fitness Band to Trigger a Bulb
- How to Use Serial Bluetooth in ESP32
HC-05 Bluetooth Module
HC-05 is a serial Bluetooth module. It can be configured using AT commands. It can work in three different configurations (Master, Slave, Loop back). In our project we will be using it as a slave. The features of HC-05 module includes,
- Typical -80dBm sensitivity.
- Default baud rate: 9600bps , 8 data bits , 1 stop bit , no parity.
- Auto-pairing pin code: “1234” or “0000” default pin code.
- It has 6 pins.
- Vcc and Gnd pins are used for powering the HC-05.
- Tx and Rx pins are used for communicating with the microcontroller.
- Enable pin for activating the HC-05 module. when it is low , the module is disabled
- State pin acts status indicator. When it is not paired/connected with any other Bluetooth device, LED flashes continuously. When it is connected/paired with any other Bluetooth device, then the LED flashes with the constant delay of 2 seconds.
To learn more about Bluetooth module go through our other Bluetooth related projects.
Using Serial Bluetooth Terminal (Android App)
Using this app is very easy and require few steps. The screenshots are given below with steps. Just pair the HC-05 with Smartphone. The default code is ‘0000’ or ‘1234’ but Mostly ‘1234’ works.
- Firstly download and install the the app Then go to devices to find HC-05 Module. Select HC-05 Module searched on the app. If not found then check if HC-05 is properly powered.
- After clicking the HC-05 it will get connected. Now type any message in the message box and send it. It will get printed on the Arduino Serial Monitor.
Programming NodeMCU ESP8266 to interface Bluetooth
For programming the NodeMCU ESP8266 using ArduinoIDE, just plug it from Laptop or PC using Micro USB Cable and open Arduino IDE. For this tutorial, the hardware serial and software serial will be used. The hardware serial will be used to read and write data to Arduino Serial Monitor and Sofware Serial will be used communicate with HC-05. As always complete code and Demo Video is given at the end of the tutorial.
Initially, include the Software Serial library since it will be used in this tutorial.
#include <SoftwareSerial.h>
Define the RX and TX pin for software serial communication, also define the led pin connected to NodeMCU. We are using internal LED of NodeMCU which is at Pin D4.
SoftwareSerial btSerial(D2, D3); // RX, int led = D4;
Start the Software and Hardware Serial at 9600 baud rate. Set Led Pin as output. Print some welcome and debug message.
Serial.begin(9600); btSerial.begin(9600); pinMode(D4, OUTPUT); Serial.println("Started...");
Firstly, read from the Bluetooth module and define a case that if Bluetooth Module Receives “B” from Phone then Start Blinking Led connected to D4 of NodeMCU else if it receives “S” then stop blinking led. We are not using delay() here. But Arduino ‘millis’ will be used not to hamper the performance of Arduino.
if (btSerial.available() > 0) { char data = btSerial.read(); switch (data) { case 'B': ledB = "blink"; break; case 'S': ledB = "stop"; break; default: break; } }
The millis is defined and set the delay at 500ms i.e. the led will blink after every 500ms. Also you can configure the led delay by changing the value of ‘interval’. The led state will be toggled.
unsigned long currentMillis = millis();
if (ledB == "blink") { Serial.println("blinking started"); if (currentMillis - previousMillis >= interval) { previousMillis = currentMillis; if (ledState == LOW) { ledState = HIGH; } else { ledState = LOW; } digitalWrite(led, ledState); } }
And this will finish the programming NodeMCU to Blink LED wirelessly using Bluetooth. You can also change the program to do different tasks with LED like the LED can be replaced by a Relay with AC appliance to make Bluetooth Home automation project. In case of any doubt or suggestion then please reach to our forum or comment below.
Complete Project Code
/* HC-05 interfacing with NodeMCU ESP8266
Author: Circuit Digest(circuitdigest.com)
*/
#include <SoftwareSerial.h>
SoftwareSerial btSerial(D2, D3); // Rx,Tx
int led = D4; // led also the internal led of NodemCU
int ledState = LOW; // led state to toggle
String ledB = "";
unsigned long previousMillis = 0; // millis instaed of delay
const long interval = 500; // blink after ecery 500ms
void setup() {
delay(1000);
Serial.begin(9600);
btSerial.begin(9600); // bluetooth module baudrate
pinMode(D4, OUTPUT);
Serial.println("Started...");
}
void loop() {
if (btSerial.available() > 0) { // check if bluetooth module sends some data to esp8266
char data = btSerial.read(); // read the data from HC-05
switch (data)
{
case 'B': // if receive data is 'B'
ledB = "blink"; // write the string
break;
case 'S': // if receive data is 'S'
ledB = "stop";
break;
default:
break;
}
}
unsigned long currentMillis = millis();
if (ledB == "blink") { // if received data is 'B' the start blinking
Serial.println("blinking started");
if (currentMillis - previousMillis >= interval) {
previousMillis = currentMillis;
if (ledState == LOW) {
ledState = HIGH;
} else {
ledState = LOW;
}
digitalWrite(led, ledState);
}
}
}
Comments
Is it possible to set this bodule to 7 data bits.
Can I combine the code of Bluetooth condition into blynk code for nodemcu?