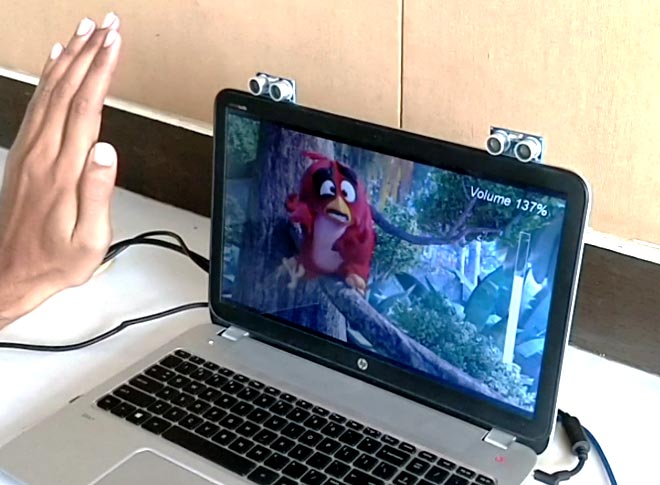
Recently Gesture controlled Laptops or computers are getting very famous. This technique is called Leap motion which enables us to control certain functions on our computer/Laptop by simply waving our hand in front of it. It is very cool and fun to do it, but these laptops are really priced very high. So in this project let us try building our own Gesture control Laptop/Computer by combining the Power of Arduino and Python.
We will use two Ultrasonic sensors to determine the position of our hand and control a media player (VLC) based on the position. I have used this for demonstration, but once you have understood the project, you can do anything by just changing few lines of code and control your favorite application in your favorite way.
Pre-requisites:
We have already covered few projects which combines Arduino with Python. So I assume that you have already installed Python and its serial library and have successfully tried out few basic projects like blinking LED. If not, don’t worry you can fall back to this Arduino-Python Led Controlling tutorial and get along with it. So make sure you have installed Python and pyserial library before proceeding.
Concept behind the project:
The concept behind the project is very simple. We will place two Ultrasonic (US) sensors on top of our monitor and will read the distance between the monitor and our hand using Arduino, based on this value of distance we will perform certain actions. To perform actions on our computer we use Python pyautogui library. The commands from Arduino are sent to the computer through serial port (USB). This data will be then read by python which is running on the computer and based on the read data an action will be performed.
Circuit Diagram:
To control the PC with Hand Gestures, just connect the two Ultrasonic sensors with Arduino. We know US sensor work with 5V and hence they are powered by the on board Voltage regulator of Arduino. The Arduino can be connected to the PC/Laptop for powering the module and also for Serial communication. Once the connections are done place them on your monitor as shown below. I have used a double side tape to stick it on my monitor but you can use your own creativity. After securing it in a place we can proceed with the Programming.
Programming your Arduino:
The Arduino should be programmed to read the distance of hand from the US sensor. The complete program is given at the end of this page; just below I have given the explanation for the program. If you are new to Ultrasonic sensor, just go through Arduino & Ultrasonic Sensor Based Distance Measurement.
By reading the value of distance we can arrive at certain actions to be controlled with gestures, for example in this program I have programmed 5 actions as a demo.
Action 1: When both the hands are placed up before the sensor at a particular far distance then the video in VLC player should Play/Pause.
Action 2: When right hand is placed up before the sensor at a particular far distance then the video should Fast Forward one step.
Action 3: When left hand is placed up before the sensor at a particular far distance then the video should Rewind one step.
Action 4: When right hand is placed up before the sensor at a particular near distance and then if moved towards the sensor the video should fast forward and if moved away the video should Rewind.
Action 5: When left hand is placed up before the sensor at a particular near distance and then if moved towards the sensor the volume of video should increase and if moved away the volume should Decrease.
Let us see how the program is written to perform the above actions. So, like all programs we start with defining the I/O pins as shown below. The two US sensors are connected to Digital pins 2,3,4 and 5 and are powered by +5V pin. The trigger pins are output pin and Echo pins are input pins.
The Serial communication between Arduino and python takes places at a baud rate of 9600.
const int trigger1 = 2; //Trigger pin of 1st Sesnor const int echo1 = 3; //Echo pin of 1st Sesnor const int trigger2 = 4; //Trigger pin of 2nd Sesnor const int echo2 = 5;//Echo pin of 2nd Sesnor void setup() { Serial.begin(9600); pinMode(trigger1, OUTPUT); pinMode(echo1, INPUT); pinMode(trigger2, OUTPUT); pinMode(echo2, INPUT); }
We need to calculate the distance between the Sensor and the hand each time before concluding on any action. So we have to do it many times, which means this code should be used as a function. We have written a function named calculate_distance() which will return us the distance between the sensor and the hand.
/*###Function to calculate distance###*/ void calculate_distance(int trigger, int echo) { digitalWrite(trigger, LOW); delayMicroseconds(2); digitalWrite(trigger, HIGH); delayMicroseconds(10); digitalWrite(trigger, LOW); time_taken = pulseIn(echo, HIGH); dist= time_taken*0.034/2; if (dist>50) dist = 50; }
Inside our main loop we check for the value of distance and perform the actions mentioned above. Before that we use two variables distL and distR which gets updated with current distance value.
calculate_distance(trigger1,echo1); distL =dist; //get distance of left sensor calculate_distance(trigger2,echo2); distR =dist; //get distance of right sensor
Since we know the distance between both the sensors, we can now compare it with predefined values and arrive at certain actions. For example if both the hands are placed at a distance of 40 mc then we play/pause the video. Here the word “Play/Pause” will be sent out through serial port
if ((distL >40 && distR>40) && (distL <50 && distR<50)) //Detect both hands {Serial.println("Play/Pause"); delay (500);}
If the Right hand alone is placed before the module then we fast forward the video by one step and if it is left hand we rewind by one step. Based on the action, here the word “Rewind” or “Forward” will be sent out through serial port
if ((distL >40 && distL<50) && (distR ==50)) //Detect Left Hand {Serial.println("Rewind"); delay (500);} if ((distR >40 && distR<50) && (distL ==50)) //Detect Right Hand {Serial.println("Forward"); delay (500);}
Foe detailed control of volume and track we use a different methodology so as to prevent false triggers. To control the volume we have to place the left hand approx. At a distance of 15 cm , then you can either move it towards the sensor to decrease the volume of move it away from the sensor to increase the volume. The code for the same is shown below. Based on the action, here the word “Vup” or “Vdown” will be sent out through serial port
//Lock Left - Control Mode if (distL>=13 && distL<=17) { delay(100); //Hand Hold Time calculate_distance(trigger1,echo1); distL =dist; if (distL>=13 && distL<=17) { Serial.println("Left Locked"); while(distL<=40) { calculate_distance(trigger1,echo1); distL =dist; if (distL<10) //Hand pushed in {Serial.println ("Vup"); delay (300);} if (distL>20) //Hand pulled out {Serial.println ("Vdown"); delay (300);} } } }
We can use the same method for the right side sensor also, to control the track of the video. That is if we move the right hand towards the sensor it will fast forward the movie and if you move it away from the sensor it will rewind the movie. Based on the action, here the word “Rewind” or “Forward” will be sent out through serial port
You can now read over the complete code for this gesture controlled PC given at the end of the page and try understating it as an whole and then copy it to your Arduino IDE.
Programming your Python:
The python program for this project is very simple. We just have to establish a serial communication with Arduino through the correct baud rate and then perform some basic keyboard actions. The first step with python would be to install the pyautogui module. Make sure you follow this step because the program will not work without pyautogui module.
Installing pyautogui module for windows:
Follow the below steps to install pyautogui for windows. If you are using other platforms the steps will also be more or less similar. Make sure your computer/Laptop is connected to internet and proceed with steps below
Step 1: Open Windows Command prompt and change the directory to the folder where you have installed python. By default the command should be
cd C:\Python27
Step 2: Inside your python directory use the command python –m pip install –upgrade pip to upgrade your pip. Pip is a tool in python which helps us to install python modules easily. Once this module is upgraded (as shown in picture below) proceed to next step.
python –m pip install –upgrade pip
Step 3: Use the command “python –m pip install pyautogui” to install the pyautogui module. Once the process is successful you should see a screen something similar to this below.
python –m pip install –upgrade pip
Now that the pyautogui module and pyserial module (installed in previous tutorial) is successful installed with the python, we can proceed with the python program. The complete python code is given at the end of the tutorial but the explanation for the same is as follows.
Let us import all the three required modules for this project. They are pyautogui, serial python and time.
import serial #Serial imported for Serial communication import time #Required to use delay functions import pyautogui
Next we establish connection with the Arduino through COM port. In my computer the Arduino is connected to COM 18. Use device manager to find to which COM port your Arduino is connected to and correct the following line accordingly.
ArduinoSerial = serial.Serial('com18',9600) #Create Serial port object called arduinoSerialData time.sleep(2) #wait for 2 seconds for the communication to get established
Inside the infinite while loop, we repeatedly listen to the COM port and compare the key words with any pre-defied works and make key board presses accordingly.
while 1: incoming = str (ArduinoSerial.readline()) #read the serial data and print it as line print incoming if 'Play/Pause' in incoming: pyautogui.typewrite(['space'], 0.2) if 'Rewind' in incoming: pyautogui.hotkey('ctrl', 'left') if 'Forward' in incoming: pyautogui.hotkey('ctrl', 'right') if 'Vup' in incoming: pyautogui.hotkey('ctrl', 'down') if 'Vdown' in incoming: pyautogui.hotkey('ctrl', 'up')
As you can see, to press a key we simply have to use the command “pyautogui.typewrite(['space'], 0.2)” which will press the key space for 0.2sec. If you need hot keys like ctrl+S then you can use the hot key command “pyautogui.hotkey('ctrl', 's')”.
I have used these combinations because they work on VLC media player you can tweak them in any way you like to create your own applications to control anything in computer with gestures.
Gesture Controlled Computer in Action:
Make the connections as defined above and upload the Arduino code on your Arduino board. Then use the python script below and launch the program on your laptop/computer.
Now you can play any movie on your computer using the VLC media player and use your hand to control the movie as shown in the video given below.
Hope you understood the project and enjoyed playing with it. This is just a demo and you can use your creativity to build a lot more cool gesture controlled stuff around this. Let me know if this was useful and what you will create using this in the comment section and I will be happy to know it.
Complete Project Code
Arduino Code:
/*
* Program for gesture control VLC Player
* Controlled uisng Python
* Code by B.Aswinth Raj
* Dated: 11-10-2017
* Website: www.circuitdigest.com
*/
const int trigger1 = 2; //Trigger pin of 1st Sesnor
const int echo1 = 3; //Echo pin of 1st Sesnor
const int trigger2 = 4; //Trigger pin of 2nd Sesnor
const int echo2 = 5;//Echo pin of 2nd Sesnor
long time_taken;
int dist,distL,distR;
void setup() {
Serial.begin(9600);
pinMode(trigger1, OUTPUT);
pinMode(echo1, INPUT);
pinMode(trigger2, OUTPUT);
pinMode(echo2, INPUT);
}
/*###Function to calculate distance###*/
void calculate_distance(int trigger, int echo)
{
digitalWrite(trigger, LOW);
delayMicroseconds(2);
digitalWrite(trigger, HIGH);
delayMicroseconds(10);
digitalWrite(trigger, LOW);
time_taken = pulseIn(echo, HIGH);
dist= time_taken*0.034/2;
if (dist>50)
dist = 50;
}
void loop() { //infinite loopy
calculate_distance(trigger1,echo1);
distL =dist; //get distance of left sensor
calculate_distance(trigger2,echo2);
distR =dist; //get distance of right sensor
//Uncomment for debudding
/*Serial.print("L=");
Serial.println(distL);
Serial.print("R=");
Serial.println(distR);
*/
//Pause Modes -Hold
if ((distL >40 && distR>40) && (distL <50 && distR<50)) //Detect both hands
{Serial.println("Play/Pause"); delay (500);}
calculate_distance(trigger1,echo1);
distL =dist;
calculate_distance(trigger2,echo2);
distR =dist;
//Control Modes
//Lock Left - Control Mode
if (distL>=13 && distL<=17)
{
delay(100); //Hand Hold Time
calculate_distance(trigger1,echo1);
distL =dist;
if (distL>=13 && distL<=17)
{
Serial.println("Left Locked");
while(distL<=40)
{
calculate_distance(trigger1,echo1);
distL =dist;
if (distL<10) //Hand pushed in
{Serial.println ("Vup"); delay (300);}
if (distL>20) //Hand pulled out
{Serial.println ("Vdown"); delay (300);}
}
}
}
//Lock Right - Control Mode
if (distR>=13 && distR<=17)
{
delay(100); //Hand Hold Time
calculate_distance(trigger2,echo2);
distR =dist;
if (distR>=13 && distR<=17)
{
Serial.println("Right Locked");
while(distR<=40)
{
calculate_distance(trigger2,echo2);
distR =dist;
if (distR<10) //Right hand pushed in
{Serial.println ("Rewind"); delay (300);}
if (distR>20) //Right hand pulled out
{Serial.println ("Forward"); delay (300);}
}
}
}
delay(200);
}
Python Code:
import serial #Serial imported for Serial communication
import time #Required to use delay functions
import pyautogui
ArduinoSerial = serial.Serial('com18',9600) #Create Serial port object called arduinoSerialData
time.sleep(2) #wait for 2 seconds for the communication to get established
while 1:
incoming = str (ArduinoSerial.readline()) #read the serial data and print it as line
print incoming
if 'Play/Pause' in incoming:
pyautogui.typewrite(['space'], 0.2)
if 'Rewind' in incoming:
pyautogui.hotkey('ctrl', 'left')
if 'Forward' in incoming:
pyautogui.hotkey('ctrl', 'right')
if 'Vup' in incoming:
pyautogui.hotkey('ctrl', 'down')
if 'Vdown' in incoming:
pyautogui.hotkey('ctrl', 'up')
incoming = "";
Comments
How do I decrease the volume
What are the specific materials needed for this project?
sensors are not working with python GUI if we work sensor with Arduino only it works in serial monitor but not with VLC player
You cannot control VLC player without getting your Python to work. What error are you facing while trying to run the python code?
In which software should I type Python code..
You have to read this tutorial
https://circuitdigest.com/microcontroller-projects/arduino-python-tutor…
this will explain how to use python with Arduino and will also answer your question
Is it possible to just use Arduino and copy and paste the code?
Please help me i send the next message
sketch_dec11a:19: error: expected initializer before 'void'
void calculate_distance(int trigger, int echo)
G:\Arduino Projects\Arduino program\sketch_dec11a\sketch_dec11a.ino: In function 'void loop()':
sketch_dec11a:34: error: 'distL' was not declared in this scope
distL =dist; //get distance of left sensor
G:\Arduino Projects\Arduino program\sketch_dec11a\sketch_dec11a.ino: At global scope:
sketch_dec11a:69: error: expected constructor, destructor, or type conversion before '(' token
delay(200); // put your main code here, to run repeatedly:
exit status 1
expected initializer before 'void'
This report would have more information with
"Show verbose output during compilation"
option enabled in File -> Preferences.
I couldn't run the python code and rest off the process. I stuck in after installing pyautogui module.
Hi friend,
can I have the same code for python 3.5 please ?
thanks
Is anyone in this forum who tried the project and succeded.
Sir, how would Arduino software recognize VLC media player?
Arduino cannot recognise VLC player. We are using Python here. Python along with Arduino can do woders. Read the full tutorial to understand how it is done
HI!
if i can code the program in c++ will it work?
ur code is not at all working
I want to use this project as a graduation project. Can you help me if you have a problem?
please give me entire project with detail
After installing pyautogui it is showing as "no such option as -u" in command prompt
Venkat Harish, I ran into the same problem. You need two hyphens before the upgrade argument.
Instead of this: python –m pip install –upgrade pip
Use this: python -m pip install --upgrade pip
after installing pyautogui it is showing as "no such option as -u" in cmd prompt. how to overcome that problem
i can't run a python program . what should i do to overcome that error?
how to connect between arduino and python
I got everything to work. I already uploaded the arduino code and got python to work but when I try to test it with VLC it doesn't work. What could be causing my problem?
how to run this project
Thank You....It is working
I am trying to working with this project. All are ok but at last I can't run the python program. So It suck me. Plz help if you have succeeded
bro..go to arduino app and select tools(available in header below the app name)and then select board(know ur board example arduino uno or nano etc) and back then select port(below the board option) which is like com3,com12,com13 etc know which serial is connected .for example if ur arduino port is com3 then in python code there is a line which is serial.Serial('com3',9600)..this com3 should be same. then dump the audrino code to board quickly run the python code ..i am also facing this error and now its working fine.
yeah.. u selected the Arduino board and dumped the code .
coming to python..,, i have installed the python 3.6.4 and installed the dependencies.
now tell me how to test whether the project is working or not?
after the code is being run in background, should we launch the vlc player?
plz reply....
python indicating this error "'module' object has no attribute 'Serial'"
If we want to control ppt with hand gestures what changes we must do?
The arduino and hardware will be the same. You have to change the python code for the respective keys
hello sir ,iam struck with the circuit please some one help me
Done all the same installation as mention in given link but Output is not getting only... none of the gesture is not working...in python shell output is getting as forward rewind but in VLC video is not forwarded or rewind ?
If pyton script is responding to your actions, then it means your project is 90% complete. Try playing the movie in full screen, also close the shell window of python before playing the movie. Just launch the code play the movie and try it
My program is perfect with both arduino and Python both have been executed but there is an issue with the port I.e. it doesn't allow one to overlap each other type of. And secondly even after correctly getting the output at arduino monitor there is no changes in the video player please help!
Boss i just love your idea. Never thought like this. I already have all this accessories. 3 distance sensors can make it even more accurate.
I will make its gesture detection and AI more accurate I.A. I am imaging more fun with playing games like this. and moving next previous pics in slide show with swipe gesture etc.
Thanks for the article.
while uploading program to board, it gives this message: Arduino: 1.8.5 (Windows 7), Board: "Arduino/Genuino Uno"
Sketch uses 3616 bytes (11%) of program storage space. Maximum is 32256 bytes.
Global variables use 258 bytes (12%) of dynamic memory, leaving 1790 bytes for local variables. Maximum is 2048 bytes.
avrdude: stk500_recv(): programmer is not responding
avrdude: stk500_getsync() attempt 1 of 10: not in sync: resp=0x76
avrdude: stk500_recv(): programmer is not responding
avrdude: stk500_getsync() attempt 2 of 10: not in sync: resp=0x76
avrdude: stk500_recv(): programmer is not responding
avrdude: stk500_getsync() attempt 3 of 10: not in sync: resp=0x76
avrdude: stk500_recv(): programmer is not responding
avrdude: stk500_getsync() attempt 4 of 10: not in sync: resp=0x76
avrdude: stk500_recv(): programmer is not responding
avrdude: stk500_getsync() attempt 5 of 10: not in sync: resp=0x76
avrdude: stk500_recv(): programmer is not responding
avrdude: stk500_getsync() attempt 6 of 10: not in sync: resp=0x76
avrdude: stk500_recv(): programmer is not responding
avrdude: stk500_getsync() attempt 7 of 10: not in sync: resp=0x76
avrdude: stk500_recv(): programmer is not responding
avrdude: stk500_getsync() attempt 8 of 10: not in sync: resp=0x76
avrdude: stk500_recv(): programmer is not responding
avrdude: stk500_getsync() attempt 9 of 10: not in sync: resp=0x76
avrdude: stk500_recv(): programmer is not responding
avrdude: stk500_getsync() attempt 10 of 10: not in sync: resp=0x76
Problem uploading to board. See http://www.arduino.cc/en/Guide/Troubleshooting#upload for suggestions.
This report would have more information with
"Show verbose output during compilation"
option enabled in File -> Preferences.
please help me to get rid of it
I'm getting this error after running the python code. please help
Traceback (most recent call last):
File "C:/Users/Tanmay Devlekar/Desktop/mp.py", line 5, in <module>
ArduinoSerial = serial.Serial('com3',9600) #Create Serial port object called arduinoSerialData
File "C:\Python27\lib\site-packages\serial\serialwin32.py", line 38, in __init__
SerialBase.__init__(self, *args, **kwargs)
File "C:\Python27\lib\site-packages\serial\serialutil.py", line 282, in __init__
self.open()
File "C:\Python27\lib\site-packages\serial\serialwin32.py", line 66, in open
raise SerialException("could not open port %r: %r" % (self.portstr, ctypes.WinError()))
SerialException: could not open port 'com3': WindowsError(5, 'Access is denied.')
>>>
have you checked if you have used the correct COM port?
Traceback (most recent call last):
File "C:/Python27/gc2.py", line 4, in <module>
Arduino_Serial = serial.Serial('com12',9600) # Initialize serial and Create Serial port object called Arduino_Serial
File "C:/Python27\serial\serialwin32.py", line 31, in __init__
super(Serial, self).__init__(*args, **kwargs)
File "C:/Python27\serial\serialutil.py", line 240, in __init__
self.open()
File "C:/Python27\serial\serialwin32.py", line 62, in open
raise SerialException("could not open port {!r}: {!r}".format(self.portstr, ctypes.WinError()))
SerialException: could not open port 'com12': WindowsError(2, 'The system cannot find the file specified.')
>>>
Respected sir Eeven i am facing the error.
if you have debugged it please let me know how to do it
hope you will reply as soon as possible.
Regards
PUNEET CHOPADE
Each and everything is working perfect.................:p
just tell me that after running the python script , what we've to do? Shall we play the VLC or we have to do anything else?
I get an error in python code in this line :
incoming = str(ArduinoSerial.readline())
Thanks the project is working good .
Could you help us in controlling the gallery using the same principle.
Sir can you please tell me which algorithm you are using for this project...Thanks
Hi, I am a newbie. How did to connect two ultrasonic sensors 5v pins in Arduino uno? As arduino uno has only one 5v pin, can anyone help? please share some details and picture if possible...
Code is working , but result is printed on serial monitor , video is not operated as it should be according to code.I have use code in C.
I have uploaded the code and compiled the python code but nothing happens and power and orange light are on on the board.
Check if the python code is receiving data from the arduino, i selected the wrong COM port and when corrected I got it working again!!
Can i also use this for powerpoint presentation control?
can I used with only one ultrasonic sensor
python –m pip install –upgrade pip
wene i use the code in cmd it show me errer say
pythone can't open file 'um':[Errno 2]no such file or diroctory
can i use this hand gesture mechanism as computer mouse
in other words can use this gesture to perform mouse function
eg. open window, close window
everything that the mouse can do
How we can get the access of vocal media player?please explain
Traceback (most recent call last):
File "C:\Users\Venkatesu\AppData\Local\Programs\Python\Python36-32\gesture.py", line 1, in <module>
import serial #Serial imported for Serial communication
ModuleNotFoundError: No module named 'serial'
You should install pyserial on your computer before you complie the program. Follow the tutorial to check how to install pyserial
can that be use for all? I mean can that function on dragging files / clicking google or softwares or anything in the laptop that normally a mouse and keyboard can do? thank you
Yes you can do all that in your python code but its hard performing those actions with US sensor alone
Code is running correctly in the python script but just play/pause gestures are working on VLC not forward, rewind,vup,vdown
i got everything working.....except "vol down" and "rewind" are not working please help me!!!
yeah!! I just solved the problem i was facing in rewinding and vol down.......
BTW, there is problem in Python programme .Nothing serious u just have written-
if 'Vup' in incoming:
pyautogui.hotkey('ctrl', 'down')
if 'Vdown' in incoming:
pyautogui.hotkey('ctrl', 'up')
-it should be- 'Vup' for ('ctrl', 'up') and 'Vdown' for ('ctrl,down')
sir every thing is working properly
except the play/pause it is not working what do i do now
I installed Pyserial and Pyautogui and when I try to run the python code, I have this error:
Traceback (most recent call last):
File "C:/1/3.py", line 3, in <module>
import pyautogui
File "C:\Python27\lib\site-packages\pyautogui\__init__.py", line 84, in <module>
import pyscreeze
SyntaxError: 'return' with argument inside generator (__init__.py, line 168)
What is wrong? Help
Try import pyautogui on your shell window and you should not get any error messages
Hi Aswinth I am getting the same error, can you please tell how to import pyautogui, I coudn't find it
Hello Aswinth sir, I have tried to import pyautogui as well as pyscreeze. I found no error as follows:
>>> import pyautogui
>>> import pyscreeze
>>>
But when I tried to run the code following error occurs:
Traceback (most recent call last):
File "C:/Python27/Gesture.py", line 6, in <module>
import pyautogui # add pyautogui library for programmatically controlling the mouse and keyboard.
File "C:\Python27\lib\site-packages\pyautogui\__init__.py", line 84, in <module>
import pyscreeze
SyntaxError: 'return' with argument inside generator (__init__.py, line 168)
Some one has suggested that use python 3 instead of python2 but python 3 does not make any folder like python27 so that we can'nt install pyautogui in that. If have any suggestion for installing pyautogui in python3 please guide me.
Everyone is talking about the error but no one has sollutions.
I have tried to run the codes in Windiws 7 (32 bit) as wel as (64 bit). But the error is same.
I got the solution!!.
Install Python3.7 and intall pyseries in C:\program files\Python37-32 and also pyautogui in the same. and the run the code. It works.
If need any help, one can ask on [email protected]
hi Elena,
same error for me what have u done?
I just moved from Python 2 to 3 version. It seems to be a bug with pyautogui on the old version. With 3 it is fine.
Can you please guide me how to shift the python 2 to python 3. I am trying to run the code in 3 but we have installed pyautogui is installed in C:\python27.
How to use python3 as we have installed pyautogui and pyserial in python 2.6.7
Hi Elena ! can you please guide me how to install pyautogui in python 3?
my email id is [email protected]
Please share the steps or link.
hello sir ,
me too get the same problem like
Traceback (most recent call last):
File "C:/Python27/handgestures.py", line 4, in <module>
import pyautogui
File "C:\Python27\lib\site-packages\pyautogui\__init__.py", line 84, in <module>
import pyscreeze
SyntaxError: 'return' with argument inside generator (__init__.py, line 168)
>>>
i tried to import pyautogui in shell window . it shows no error.
what to do?
Looks like the error does occur with Python 2 try Python 3
I have run the code in python2. I am getting the following error:
Traceback (most recent call last):
File "C:\Python27\HandGesture.py", line 3, in <module>
import pyautogui
File "C:\Python27\lib\site-packages\pyautogui\__init__.py", line 84, in <module>
import pyscreeze
SyntaxError: 'return' with argument inside generator (__init__.py, line 168)
>>> import pyscreeze
>>> import pyautogui
>>>
Python 2.7.14 (v2.7.14:84471935ed, Sep 16 2017, 20:19:30) [MSC v.1500 32 bit (Intel)] on win32
Type "copyright", "credits" or "license()" for more information.
>>> import pyautogui
Traceback (most recent call last):
File "<pyshell#0>", line 1, in <module>
import pyautogui
File "C:\Python27\lib\site-packages\pyautogui\__init__.py", line 80, in <module>
import pyscreeze
SyntaxError: 'return' with argument inside generator (__init__.py, line 168)
>>>
please help
Lalchand
Mar 23, 2019 reply
I have run the code in python2. I am getting the following error:
Traceback (most recent call last):
File "C:\Python27\HandGesture.py", line 3, in
import pyautogui
File "C:\Python27\lib\site-packages\pyautogui\__init__.py", line 84, in
import pyscreeze
SyntaxError: 'return' with argument inside generator (__init__.py, line 168)
Please help
I have run the code in python2. I am getting the following error:
Traceback (most recent call last):
File "C:\Python27\HandGesture.py", line 3, in
import pyautogui
File "C:\Python27\lib\site-packages\pyautogui\__init__.py", line 84, in
import pyscreeze
SyntaxError: 'return' with argument inside generator (__init__.py, line 168)
Please help
i need help with installing pyautogui.
error: complete output from command python setup.py egg_info:
Can anyone tell me which algorithm is used here!!?
great video big thank you