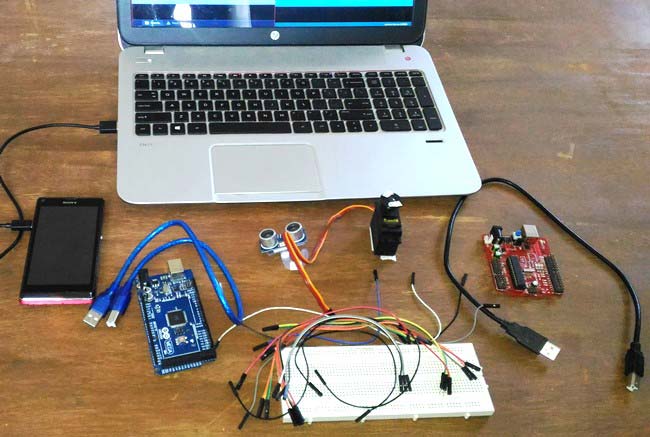
This is an interesting project in which we explore the power of an Arduino and Android to create a Surveillance device which uses Arduino and Ultra Sonic Sensor to broadcast the information to a mobile application (Android) using Bluetooth.
Safety and Security has been our primary concern since ages. Installing a security camera that has night mode with tilt and pan option will burn a big hole on our pockets. Hence let us make an economic device which does almost the same but without any video features.
This device senses objects with the help of Ultrasonic Sensor and hence can work even during night times. Also we are mounting the US (Ultra Sonic) sensor over a servo motor, this servo motor can be either be set to rotate automatically to scan the area or can be rotated manually using our Mobile app, so that we can focus the ultrasonic sensor in our required direction and sense the objects present over there. All the information sensed by the US sensor will be broadcasted to our Smart phone using Bluetooth Module (HC-05). So it will work like a Sonar or a Radar.
Interesting right?? .... Let us see what we would require to do this project.
Requirements:
Hardware:
- A +5V power supply ( I am using my Arduino (another) board for power supply)
- Arduino Mega (You can use anything from pro mini to Yun)
- Servo Motor (any rating)
- Bluetooth Module (HC-05)
- Ultra Sonic Sensor (HC-SR04)
- Breadboard (not mandatory)
- Connecting wires
- Android mobile
- Computer for programming
Software:
- Arduino Software
- Android SDK
- Processing Android (To create mobile application)
Once we are ready with our materials, let us start building the hardware. I have split this tutorial into Arduino Part and the Processing Part for easy understanding. People who are new to processing need not fear much because the complete code is given at the end of the tutorial which can be used as such.
Downloading and Installing Softwares:
The Arduino IDE can be installed from here. Download the software according to your OS and install it. The Arduino IDE will require a driver to communicate with your Arduino Hardware. This driver should get installed automatically once you connect your board with your computer. Try uploading a blink program from examples to make sure you Arduino is up and running.
The Processing IDE can be installed from here. Processing is an excellent open source application which can be used for many things, it has various modes. In “Java Mode” we can create windows computer applications ( .EXE files) and in “Android mode” we can create Android mobile Applications ( .APK files) it also has other modes like “Python mode” where you can write you python programs. This tutorial will not cover the basics of Processing, hence if you want learn java programming or processing head on to this great YouTube channel here.
Arduino Hardware part and Circuit Diagram:
This project involves a lot of components like the Servo Motor, Bluetooth Module, Ultrasonic Sensor etc. Hence if you are an absolute beginner then it would be recommended to start with some basic tutorial which involves these components and then come back here. Check out our various projects on Servo Motor, Bluetooth Module and Ultrasonic Sensor here.
All components are not powered by the Arduino itself because, the servo motor, Bluetooth module and US sensor altogether draws a lot of current which the Arduino will not be able to source. Hence it is strictly advisable to use any external +5V supply. If you do not have an external +5V supply at your reach, you can share the components between two Arduino boards as I have done. I have connected the Servos power rails to another Arduino board (red colour) and connected the Bluetooth module HC-05 and Ultrasonic sensor HC-SR04 to the Arduino mega. CAUTION: Powering up all these modules using one Arduino board will fry up the Arduino voltage regulator.
Connection diagram for this Arduino Based Sonar Project is given below:
Once the connections are made, mount the US sensor onto your Servo motor as shown below:
I have used a small plastic piece that was in my junk and a double side tape to mount the sensor. You can come up with your own idea to do the same. There are also servo holders available in market which can be used for the same purpose.
Once the Servo is mounted and the Connections are given, it should look something like this.
Follow the schematics on top if get any connections wrong. Now let us start programming the Arduino Mega using the Arduino IDE.
Arduino Software Part:
We have to write our code so that we can calculate the distance between an object and Ultra Sonic sensor and send it to our mobile application. We also have to write code for our servo motor to sweep and also get controlled from the data received by the Bluetooth module. But don’t worry the program is lot simpler than you can image, thanks to Arduino and its libraries. The complete code is given below in the code section.
Below function is used to make the servo motor automatically sweep from left to right (170 to 10) and again from right to left (10 to170). The two for loops are used to achieve the same. The function us() is called inside both functions to calculate the distance in between the sensor and the object and broadcast it to the Bluetooth. A delay of 50 ms is given to make the servo rotate slowly. The slower the motor rotates the accurate your readings become.
//**Function for servo to sweep**// void servofun() { Serial.println("Sweeping"); //for debugging for(posc = 10;posc <= 170;posc++) // Using 10 to 170 degree is safe than 0 to 180 because some servo might not be operational at extreme angels { servo.write(posc); // set the position of servo motor delay(50); us(); //measure the distance of objects sing the US sensor } for(posc = 170;posc >= 10;posc--) { servo.write(posc); delay(50); us(); //measure the distance of objects sing the US sensor } Serial.println ("Scan Complete"); //for debugging flag=0; } //**End of Servo sweeping function**//
As said earlier the servo motor can also be controlled manually from the smart phone. You simply swipe right to make the motor move right and swipe left to make the motor move left. The above function is used to achieve the same. The angel of the servo motor will be directly be received by the Bluetooth module and stored in the variable BluetoothData, then the servo is position in that particular angel by using the line servo.write(BluetoothData).
//**Function to control Servo manually**// void manualservo() { us(); // Get value from user and control the servo if (Blueboy.available()) { BluetoothData=Blueboy.read(); Serial.println(BluetoothData); servo.write(BluetoothData); Serial.println("Written"); if (BluetoothData == 'p') { flag=0; } } } //__End of manual control function__//
The distance present before the object will be calculated by below function. It works with a simple formulae that Speed = Distance/time. Since we know the speed of the US wave and the time taken the distance can be calculated using the above formulae.
//**Function to measure the distance**// void us() { int duration, distance; digitalWrite(trigPin, HIGH); delayMicroseconds(1000); digitalWrite(trigPin, LOW); duration = pulseIn(echoPin, HIGH); distance = (duration/2) / 29.1; // Calculates the distance from the sensor if (distance<200 && distance >0) Blueboy.write(distance); } //__End of distance measuring function__//
If you have any doubts in the program, feel free to use the comment section for your quires. So, once we are ready with our code we can straight away dump the code into our hardware. But the surveillance device will not start working till it is connected to the Android Application. Also check the Video at the end for complete working.
Android Mobile Application for Ultrasonic Radar:
If you do not want to make your own application and instead just want to install the same application used in this tutorial you can follow the steps below.
1. You can directly download the APK file from the below link. This APK file is made for Android version 4.4.2 and above (Kitkat an above). Extract the APK file from the zip file.
Android Application for Ultrasonic Radar
2. Transfer the .Apk file from your computer to your mobile phone.
3. Enable installing application from Unknown sources in your android settings.
4. Install the application.
If successfully installed, you will find the application named “Zelobt” installed on your phone as shown below:
If you have installed this APK, then you can skip the below part and jump to the next section.
Programming your own Application using Processing:
Either you can use the .APK file given above or you can build your own app using Processing as explained here. With little knowledge on programming it is also very easy to write your own code for your android application. However if you are just beginning then it is not advisable to start with this code since its a bit high than the beginner level.
This program uses two libraries namely, the “Ketai library” and the “ControlP5 library”. The ketai library is used to control all the hardware present inside our mobile phone. Things like you phones battery level, proximity sensor values, accelerometer sensor values, Bluetooth control options etc. can be easily accessed by this library. In this program we use this library to establish a communication between the phones Bluetooth and the Arduino Bluetooth (HC-05). The “ControlP5 library” is used to plot graphs for our radar system.
The complete android program is attached, you can download it from here.
CAUTION: Do not forget to install the above mentioned libraries and do not copy paste the code part alone, because the code imports images from data folder which altogether is given in above attachment. Hence download and use only that.
Once you are done with the coding part and have successfully compiled it you can directly connect your mobile phone to your computer through data cable and click on play button to dumb the application onto your mobile phone. Also check our other Processing Projects: Ping Pong Game using Arduino and Smart Phone Controlled FM Radio using Processing.
Working Explanation:
Now, we are ready with our hardware and the software part. Power up your hardware and pair your mobile to the Bluetooth module. Once paired open your “Zelobt” application that we just installed and now wait for a second and you should notice your Bluetooth module (HC-05) automatically getting connected with your smart phone. Once the connection is established you will get the following screen:
You can notice that it says connected to: Device name (hardware address) on the top of the screen. It also displays the current angel of the servo motor and the distance between the US sensor. A blue graph is also plotted on the red background based on the measured distance. The closer the object gets the more the taller the blue area gets. The graph measured when some objects are placed near is also shown in the second figure above.
As said earlier you can also control your servo motor from your mobile app. To do these, simply click on stop button. This will stop your servo from sweeping automatically. You can also find a circular wheel at the bottom of the screen which when swiped will rotate in clock or anti clock wise direction. By swiping this wheel you can also make your servo motor turn in that particular direction. The wheel and the graph updated when swiped are shown in the picture below.
Arduino Code is given below and the APK file for android Application is here. The working of the complete project is shown in the video below. Hope you understood the project. If you have any quires, kindly use the comment section below.
Complete Project Code
/*
* DIY-RADAR
*
* US Sensor
* trigpin >> 4
* echopin >> 5
*
* Servo motor
* signal pin >> 9
*
* Hc-05
* tx >> 10
* Rx >> 11
*
* WARNING
* Do not power up all your modules to a single Arduino the current drawn will fry your arduino board. Use separate supply.
*
*/
//*Include the required header files**//
#include <Servo.h>
#include <SoftwareSerial.h>
//__End of including headers__//
//**Defining pins for US sensor**//
#define trigPin 4
#define echoPin 5
///__End of defaniton__//
SoftwareSerial Blueboy(10, 11); //Naming our Bluetooth module as Blueboy and defiing the RX and TX pins as 10 and 11
Servo servo; //Initializing a servo object called servo
//**Global variabel declarations**//
int BluetoothData;
int posc = 0;
int flag=10;
//__End of global variable declartion__//
void setup() //Runce only once
{
servo.attach(9); //Servo is connected to pin 9
pinMode(trigPin, OUTPUT); //trigpin of US sensor is output
pinMode(echoPin, INPUT); //echopin of US sensor is Input
Serial.begin(38400); //Serial monitor is started at 38400 baud rate for debugging
Blueboy.begin(9600); //Bluetooth module works at 9600 baudrate
Blueboy.println("Blueboy is active"); //Conformation from Bluetooth
}
void loop() //The infinite loop
{
//**Program to start or stop the Survilance devide**//
if (Blueboy.available())
{
Serial.println("Incoming"); //for debugging
BluetoothData=Blueboy.read(); //read data from bluetooth
Serial.println(BluetoothData); //for debugging
if (BluetoothData == 'p') //if the mobile app has sent a 'p'
{
flag=0; //play the device in auto mode
}
if (BluetoothData == 's') //if the mobile app has sent a 's'
{
flag=1; //stop the device and enter manual mode
}
Serial.println(flag); //for debugging
}
if (flag==0)
servofun(); //Servo sweeps on own
if (flag==1)
manualservo(); //Manual sweeping
}
//_End of loop program__//
//**Function for servo to sweep**//
void servofun()
{
Serial.println("Sweeping"); //for debugging
for(posc = 10;posc <= 170;posc++) // Using 10 to 170 degree is safe than 0 to 180 because some servo might not be operational at extreme angels
{
servo.write(posc); // set the position of servo motor
delay(50);
us(); //measure the distance of objects sing the US sensor
}
for(posc = 170;posc >= 10;posc--)
{
servo.write(posc);
delay(50);
us(); //measure the distance of objects sing the US sensor
}
Serial.println ("Scan Complete"); //for debugging
flag=0;
}
//**End of Servo sweeping function**//
//**Function to control Servo manually**//
void manualservo()
{
us();
// Get value from user and control the servo
if (Blueboy.available())
{
BluetoothData=Blueboy.read();
Serial.println(BluetoothData);
servo.write(BluetoothData);
Serial.println("Written");
if (BluetoothData == 'p')
{
flag=0;
}
}
}
//__End of manual control function__//
//**Function to measure the distance**//
void us()
{
int duration, distance;
digitalWrite(trigPin, HIGH);
delayMicroseconds(1000);
digitalWrite(trigPin, LOW);
duration = pulseIn(echoPin, HIGH);
distance = (duration/2) / 29.1; // Calculates the distance from the sensor
if (distance<200 && distance >0)
Blueboy.write(distance);
}
//__End of distance measuring function__//
Comments
You can soon expect projects on ESP8266 and GSM. Stay tuned....
And thanks again for your kind words..
I have simply uploaded the code in arduino and downloaded the app.
No use of orocessing software and have not downloaded it yet.
Rx -> 11 of arduino uno
Tx -> 10 of arduino Uno
No response change in the app.
Kindly help.
Hi Ayush,
The problem is likely because you there was no bluetooth connection between your phone and Arduino. Did you pair the Bluetooth module with phone?
Is the module named "HC-05" or "HC-06"?
send a screen shot of the app to help you better
sir i am beginner but i saw your project and get a lot knowledge and please advise me from where should l i i start and please help me because i want master in electronics technologies fields
Hi channa,
I am glad that you liked the project. If you are completely new to electronics start with some basic projects. I cannot suggest you any projects without knowing our skill sets. So the best way is to evaluate yourself. Making a cool looking project with Arduino will not make you a master in electronics.
Start with the basics, electronics is a vast filed find your filed and master it. There are lots of small projects here which involves some basic training on some particular filed in electronics. Learn the concept behind the project and try building something on your own. Making your own projects will eventually make you master a topic.
ALL THE BEST.
Hey Aswinth,
This is really a good project and thanks for your explanation in detail. I would rather like to know whether we can connect two sensors placed in different locations and get the data from both sensors in the same app at once, using USB cable instead to Bluetooth module ?. Your response will be appreciated. Thanks
Hi Anuradha,
Yes it is very much possible. But, I am not sure if you can do it through USB cable. Actually, things will be more complicated for reading data through Data cable rather than using a Bluetooth port.
Anyway, If I were you, I would use two Bluetooth modules acting as slave to send from two US sensor kept at different location to a master Bluetooth module. This master Bluetooth module then can communicate with your phone.
OR
If you are okay with wired communications you can keep these sensor at different location and wire then up, to a single Arduino Board from which the data can be broadcasted to you mobile phone.
Thanks
In my condition, my arduino is arduino UNO
and my bluetooth connection is HC-06
Can we do the same thing like this project?
Yes Habib, follow the same circuit and code and you should get it working
There is a logic difference between Bluetooth module and Arduino i.e 3.3 v and 5v . Is there a need of logic convertors ?
Yes Darshil,
There is a logic difference, adding a potential divider will make things smooth. But I have run it without any such devider and it works fine. However it is recomended to add one.
I have uploaded the code as it is given in the last of the article. But I am not able to get the output. Can you please tell me which line is the actual start of the code ? Is there a need to write US sensor pins , Bluetooth module pin and servo motor pin as written in the start of the code ? Please help me .
i have copied the code as it is as given above from your site, but while i compile the code the shows me a compilation error, why is this so? can you please help me out.
What do you mean by compilation error? Paste he error report here so that I can guide you
How much longer distance it can measure from obstacles to radar??
disculpa intento conectarme con un modulo de bluetooth hc-06 y si se vincula, pero abro la aplicacion y no se conecta. me podias ayudar por favor
Verifique si HC-06 funciona correctamente. Use alguna otra aplicación de Android como Bluetoothterminal para verificar si está obteniendo algún dato.
I can't connect to the programme with bluetooth. Could you please send the link of it again? Or do you have any suggestion ?
Sir i had connected all the componets and installed your app but it is not connecting to bluetooth module i checked my bluetooth module with other app it is okay but with your app its not working my android verson is,7.0 can you plz help me
The android application is only paring with the device named "HC-06" ... I renamed the module to HC-06 and its working now
can i use arduino uno
Can i add extra PIR sensor to this project , so that it can also detect motion of the object. If there is motion, then let LED glow
Hello B.Aswinth Raj,
Could you modify your code in order to make the my.Chart middle radar processed image with image from here: "youtube.com/watch?v=RSAj_ydbg48.
You have the processing code here: "drive.google.com/file/d/0B27c2BryUv06R3BXV3VUUVRzMXM/view""
I need this project but i can't make the code to run.
Here is my address [email protected]
I have hco5 module and it is paired with phone.
Works with other apps but not from zealotbt app.
Program uploaded as it is and connection as per ckt diagram.
Please help me out
Can i use arduino uno for this project
can we use arduino uno
Please reply me with the link of the software which i can modify the android application.
does not able connect to the bluethooth module with the application
Assalam.O.Alikum ,
Sir,
I want to make a radar with is wireless comunication with android and also i use this as a obstacle avioding robot with another three US one US on Servo motor. when it find any obstacle in its way is stop there and is US with Servo Motor start rotating to left and right. Where their is no obstacle in its way in robot goes there. And the other US are connected to with the angle of 60 and the second with the angle of 120 if obstacle comes in its way its also aviod this and goes in oposite direction
For this i can make 3 programs.
One with sensor with servo Obstacle avioding robot.
Second with 2 other US Obstacle avioding robot.
And the third is a radar with wire comunication.
Now your code help me fo wireless comunication. But how i combine these codes please help me
The app doesn't work....not able to connect
A very nice collection of projects, so I would like to thank and respect everyone who participated in these projects
And please in the next times publish projects about GSM & ESP8266WiFi