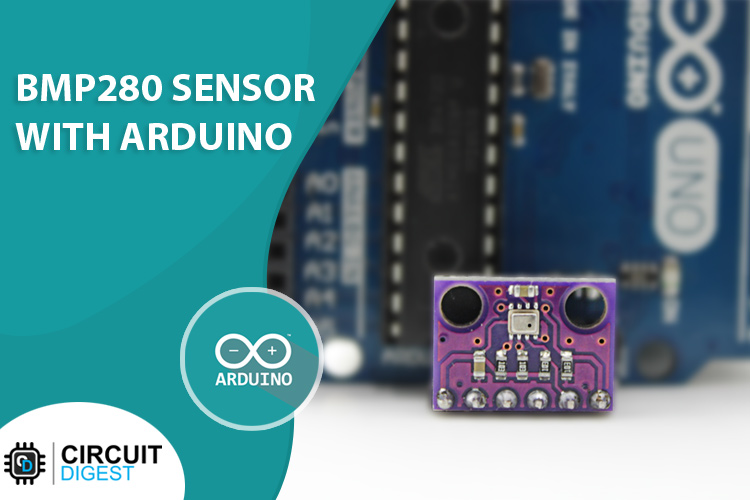
In many of our previous articles we have used many different types of temperature and humidity sensors like DHT22, DHT11, DS18b20 and LM35 to measure environmental parameters. In every one of those articles there's one thing common, none of those sensors can measure barometric pressure. So in today's article we wanted to interface the BMP280 with an Arduino and measure barometric pressure. Why BMP280 you ask? Because this sensor costs low and it only provides both temperature and barometer readings, and as pressure changes with altitude we can also use it as an altimeter with ±1 meter accuracy. But if you want to get all the weather data in one package there exists another sensor named BME280 that provides Temperature, Humidity, Barometric Pressure, and Altitude but as you can probably imagine it comes with a high cost.
BMP280 Digital Pressure Sensor Pinout
The BMP280 Digital Pressure Sensor module has 6 pins VCC, GND, SCL, SDA, CSB, and SDO. all the pins of this sensor module are digital, except VCC and Ground. The Pinout of the BMP280 barometric Pressure and Temperature Sensor is shown below:
VCC is the power supply pin of the BMP280 that can be connected to 3.3V or 5V of the supply. But note that the analog output will vary depending upon the provided supply voltage.
Ground is the ground pin of the Sesnor and it should be connected to the ground pin of the Arduino.
SCL stands for Serial Clock the master device pulses this pin at a regular interval to generate clock signal for communication.
SDA stands for Serial Data, through this pin data exchange happen between two devices.
CSB is the chip select pin of the module, if you are communicating with the device with SPI you can use this pin to communicate to select one if multiple devices are connected in the same bus.
SDO is the Serial Data out pin of the module. An output signal on a device where data is sent out to another SPI device.
BMP280 VS BME280 - What is the difference and which one is the Best?
If you search for weather sensor on the internet you will came across some affordable sensors among those there are two popular sensors, namely the BMP280 and the BME280, the name of the two modules sounds pretty similar, but there exists some key differences and in this section of the article we will discuss about that.
The major difference between the BME280 and the BMP280 sensor is that the BME280 sensor can measure Humidity while the BMP280 cannot measure humidity. Other than that there is not much difference between two devices and most parameters of the sensor remain the same.
BMP280 Digital Pressure Sensor Module – Parts
The manufacturer made this sensor so that anybody with basic knowledge in electronics can work with this sensor, so the module only requires very few readily available parts like a few resistors and two filter capacitors for stable operation. This is a MEMS based sensor and can detect sudden changes in barometric pressure and temperature. The parts marking of the sensor is shown below.
This sensor has six pins altogether, among those two are power pins which are VCC and GND. And the other four pins are for data and clock signals which are 5V tolerant. As you can see in the above image this board has to filter capacitors both of which are 100nF capacitor one capacitor is used to filter the analog power line and another one is used to power the digital power line. Other than that we have three pullup resistors for SCL, SDA, and SDO and one pulldown Resistor for SDI, with all these parts the BMP280 sensor is made.
Commonly Asked Questions about BMP280 Digital Pressure and Temperature Sensor Module
Can BMP280 measure Altitude?
This sensor can measure barometric pressure and temperature with very good accuracy. Because pressure changes with altitude we can also use it as an altimeter with ±1 meter accuracy!
What is the use of BMP280?
The BMP280 sensor is used to find the Digital Pressure and Temperature, whereas BME280 can be used to measure digital pressure, temperature, and altitude.
Can BMP280 measure humidity?
The BMP280 can only measure temperature and air pressure, while the BME280 can measure humidity in addition to temperature and air pressure.
What is the temperature range of the BMP280 sensor in which it remains operational?
The temperature range and the operating range of this sensor is exactly the same : -40 … +85 °C. That means the temperature of the sensor should be within that range for stable operation.
BMP280 Sensor Module Circuit Diagram
The Schematic Diagram of the BMP280 Temperature and Pressure Sensor is shown below and as you can see it's very simple to understand.
The BMP280 has two separate power supply pins VDD is the main power supply for all internal analog and digital functional blocks and VDDIO is a separate power supply pin, used for the supply of the digital interface. For those two pins two 100nF decoupling capacitors are used to filter the DC power supply line. Other than that the SCL, SDA, and the CSB line needs to be pulled up with a 10K resistor according to the datasheet of the device. On the other hand the SDO line needs to be pulled down for this sensor to work properly.
BMP280 Pressure Sensor with Arduino UNO – Connection Diagram
Now that we completely understand how the BMP280 Sensor works we can connect all the required wires to the Arduino UNO board, and in this section of the article, we will discuss just that!
In the above figure, the connection diagram of the BMP280 sensor with Arduino is shown we are using the I2C bus to connect the sensor to the Arduino for that we have connected the power pins of the Arduino board to the power pins of the BMP280 module and we have connected the SCL and SDA pins of the module to the SCL and SDA pin of the Arduino board.
The practical test hardware is shown below.
Arduino Code for Interfacing BMP280 Sensor Module with Arduino
The code for interfacing the BMP280 Sensor to Arduino is shown below. The code is very simple and easy to understand because we are using the Adafruit BMP280 library that makes the coding process very easy. So before we start the coding process we need to install the library. To do that go to the Manage Library section of the arduino and search for BMP280. You will get the Adafruit BMP280 library just click install and you will be prompted to install all the dependencies just click on install all button. And the library should get installed.
Now we can start our coding process. We initialize our code by declaring all the required libraries and we also make an instance of the Adafruit_BMP280 class. We also define the address of the module in a macro named BMP280_ADDRESS.
#include <Wire.h> #include <SPI.h> #include <Adafruit_BMP280.h> #define BMP280_ADDRESS 0x76 Adafruit_BMP280 bmp; // I2C
Next we have our setup() function, in the setup function as always we initialize the serial for debugging. We also defined a status variable to check if the begin method of the bmp instance was able to communicate with the module or not. If the communication process returns unsuccessful we print some debug message on the serial monitor window else we continue with the setup.
void setup() { Serial.begin(9600); while ( !Serial ) delay(100); // wait for native usb Serial.println(F("BMP280 test")); unsigned status; status = bmp.begin(BMP280_ADDRESS); if (!status) { Serial.println(F("Could not find a valid BMP280 sensor, check wiring or " "try a different address!")); Serial.print("SensorID was: 0x"); Serial.println(bmp.sensorID(),16); Serial.print(" ID of 0xFF probably means a bad address, a BMP 180 or BMP 085\n"); Serial.print(" ID of 0x56-0x58 represents a BMP 280,\n"); Serial.print(" ID of 0x60 represents a BME 280.\n"); Serial.print(" ID of 0x61 represents a BME 680.\n"); while (1) delay(10); }
If the communication process with the module returns a successful message we setup the important parameters of the library, they are Operating Mode, Temperature Oversampling, Pressure oversampling, Filtering, and Standby time. And with that we finish our setup function.
/* Default settings from the datasheet. */ bmp.setSampling(Adafruit_BMP280::MODE_NORMAL, /* Operating Mode. */ Adafruit_BMP280::SAMPLING_X2, /* Temp. oversampling */ Adafruit_BMP280::SAMPLING_X16, /* Pressure oversampling */ Adafruit_BMP280::FILTER_X16, /* Filtering. */ Adafruit_BMP280::STANDBY_MS_500); /* Standby time. */ }
Next we have our loop function. In the loop function we just call the readTemperature(), readPressure(), and readAltitude(1013.25) instances of the bmp class and print the data onto the serial monitor window. And that marks the end of our code portion.
void loop() { Serial.print(F("Temperature = ")); Serial.print(bmp.readTemperature()); Serial.println(" *C"); Serial.print(F("Pressure = ")); Serial.print(bmp.readPressure()); Serial.println(" Pa"); Serial.print(F("Approx altitude = ")); Serial.print(bmp.readAltitude(1013.25)); /* Adjusted to local forecast! */ Serial.println(" m"); Serial.println(); delay(2000); }
Working of the BMP280 Pressure Sensor Module
The gif down below shows the BMP280 Temperature and Pressure sensor in working condition. We are demonstrating the working of this sensor with temperature because we cannot change the surrounding pressure so we made a simple setup to show you the sensor is working properly.
BMP280 Sensor Not Working? - Here is what you should do
- The most common problem for BMP280 sensors is: Could not find a valid BMP280 sensor, check wiring!, if you are having this issue it might be that you're not using the correct I2C address. The sensor address of the BMP280 is (0x76). You need to define that in the code for proper operation, or you can use the I2C Scanner sketch to find the sensor address.
- Check if the VCC and the ground of the sensor is connected to VCC and ground of the Arduino.
- Check if the SCL is connected to A5 pin and SDA is connected to the SDA pin on the Arduino Board.
- Check your breadboard and jumper wires , if the jumper wires gets damaged that can sometimes cause problems.
Projects using BMP280 Pressure Sensor Module
If you are trying to build your own weather station then you can take a look at this project because in this project we have used the popular raspberry pi to build a simple weather station that uses the BMP280 sensor to get the barometric pressure.
In this tutorial, we discussed how to use BMP280 sensor with Arduino. Here you can find circuit connection, Arduino code, and a step-by-step guide.
If you are looking for a cheap and simple weather monitoring system then look no further than this, because in this project we have used the popular DHT11, BME180 and the nodeMCU board to build a simple web-server based weather station.
Supporting Files
Complete Project Code
/***************************************************************************
This is a library for the BMP280 humidity, temperature & pressure sensor
Designed specifically to work with the Adafruit BMP280 Breakout
----> http://www.adafruit.com/products/2651
These sensors use I2C or SPI to communicate, 2 or 4 pins are required
to interface.
Adafruit invests time and resources providing this open source code,
please support Adafruit andopen-source hardware by purchasing products
from Adafruit!
Written by Limor Fried & Kevin Townsend for Adafruit Industries.
BSD license, all text above must be included in any redistribution
***************************************************************************/
#include <Wire.h>
#include <SPI.h>
#include <Adafruit_BMP280.h>
#define BMP280_ADDRESS 0x76
Adafruit_BMP280 bmp; // I2C
void setup() {
Serial.begin(9600);
while ( !Serial ) delay(100); // wait for native usb
Serial.println(F("BMP280 test"));
unsigned status;
status = bmp.begin(BMP280_ADDRESS);
if (!status) {
Serial.println(F("Could not find a valid BMP280 sensor, check wiring or "
"try a different address!"));
Serial.print("SensorID was: 0x"); Serial.println(bmp.sensorID(),16);
Serial.print(" ID of 0xFF probably means a bad address, a BMP 180 or BMP 085\n");
Serial.print(" ID of 0x56-0x58 represents a BMP 280,\n");
Serial.print(" ID of 0x60 represents a BME 280.\n");
Serial.print(" ID of 0x61 represents a BME 680.\n");
while (1) delay(10);
}
/* Default settings from datasheet. */
bmp.setSampling(Adafruit_BMP280::MODE_NORMAL, /* Operating Mode. */
Adafruit_BMP280::SAMPLING_X2, /* Temp. oversampling */
Adafruit_BMP280::SAMPLING_X16, /* Pressure oversampling */
Adafruit_BMP280::FILTER_X16, /* Filtering. */
Adafruit_BMP280::STANDBY_MS_500); /* Standby time. */
}
void loop() {
Serial.print(F("Temperature = "));
Serial.print(bmp.readTemperature());
Serial.println(" *C");
Serial.print(F("Pressure = "));
Serial.print(bmp.readPressure());
Serial.println(" Pa");
Serial.print(F("Approx altitude = "));
Serial.print(bmp.readAltitude(1013.25)); /* Adjusted to local forecast! */
Serial.println(" m");
Serial.println();
delay(2000);
}
Your wiring is i2c, you did not introduce spi wiring to uno