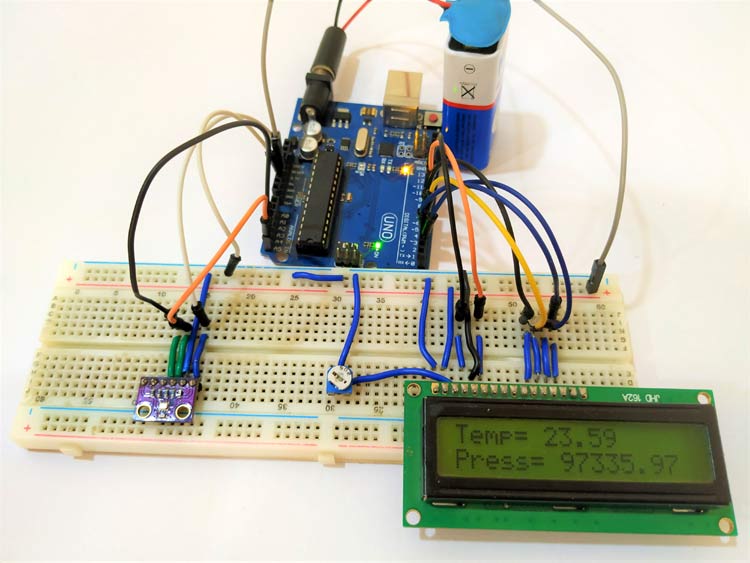
If you want to build your own temperature monitoring system or to measure the altitude of your drone or simply want to measure the atmospheric pressure in your area, then one of the best module for you to use in your project is the BMP280 Pressure sensor module. BMP280 is absolute pressure and temperature monitoring sensor which is the upgraded version of BMP085, BMP180, BMP183 sensors. Why is it called an upgraded version? It will be discussed in the following sections. We have already used the older version BMP180 with Arduino in one of our previous tutorials.
BMP280 sensor module can be used along with microcontrollers like Arduino, PIC, AVR, etc. For this project we are going to use Arduino Uno with BMP280 along with an LCD 16x2 display module, to display values of temperature and pressure. Before interfacing the BMP280 with Arduino, we need to download the BMP280 Arduino library, which is developed by Adafruit. Click on this Adafruit BMP280 library link to open the respective Github page and add the header file to your Arduino IDE.
Components Required
- Arduino
- BMP280
- Connecting Wires
- Bread Board
- LCD- 16x2
BMP280 Pressure Sensor Module:
The BMP280 sensor module works with the minimum voltage (VDD) of 1.71V, whereas the previous version sensor modules work on 1.8V (VDD). When it comes to current consumption BMP280 consumes 2.7uA, whereas the BMP180 consumes 12uA, and BMP183 and BMP085 consume 5uA each. The BMP280 also supports new filter modes. The BMP280 sensor module supports I2c, and SPI protocols, whereas the remaining sensor supports either I2c or SPI. The BMP280 sensor module has an accuracy of ±0.12 hPa, which is equivalent to ±1 m difference in altitude. Due to these key features, it is mostly used in various applications. The BMP sensor consists of a Pressure sensing element, Humidity sensing element and Temperature sensing element which are further connected to Pressure front-end, Humidity front-end, and temperature front-end. These front end IC’s are sensitivity analog amplifiers that are used in the amplification of small signals. The output of this analog front-end IC’s is fed to ADC as an input signal. In this the analog values are converted to digital voltage and this voltage is fed to the logic circuits for further interface with the outside world.
The BMP280 sensor module consists of three power modes sleep mode, forced mode, and Normal Mode. In sleep mode, no measurements are performed and power consumption is at a minimum. In forced mode, a single measurement is performed according to the selected measurement and filter options. Normal mode continuously cycles between measurement and standby period, and the cycles time period will be defined by Tstandby. The current in the standby mode is slightly higher than the sleep mode.
Circuit diagram to interface BMP280 with Arduino:
The circuit diagram to connect the Arduino with the BMP280 sensor and the LCD is shown below. If you are completely new to Arduino and LCD, then you can check this Arduino LCD tutorial to understand how to use Arduino with LCD displays.
The VCC and GND pins of the sensor are connected to the 3v3 and GND pins of the Arduino. The SCL and SDA pins of the sensor are connected to the A5 and A4 of the Arduino board. The LCD connections are as follows
LCD Pin Name |
Arduino Pin |
VSS and RW |
GND |
RS |
D9 |
E |
D8 |
D4, D5, D6, D7 |
D5,D4,D3,D2 |
Arduino Program to Interface BMP280 with Arduino:
The complete BMP280 Arduino code can be found at the bottom of this page which can be uploaded directly to your Arduino board. The explanation of the same is given below
These libraries are included for enabling the special functions. The #include <Adafruit_BMP280.h> header files we can directly read the values coming from the sensor. The #include <Wire.h> header is helpful in using I2C communication. The #include <LiquidCrystal.h> header is used to access the special function of LCD like lcd.print(), Lcd.setCursor(), etc. these header files can be downloaded using the link which is given above. The downloaded file will be in zip format. Now open Arduino select Sketch>include library>Add.zip library. Now add the downloaded file.
#include <Wire.h> #include <SPI.h> #include <Adafruit_BMP280.h> #include <LiquidCrystal.h>
Creating on object BMP for Adafruit_BMP280. An object file is created to access special functions.
Adafruit_BMP280 bmp; // I2C
Setting the pins of the Arduino to communicate with the LCD. Using these pins data will be transferred.
LiquidCrystal LCD(9, 8, 5, 4, 3, 2);
Initializing the LCD and Serial Communication.
void setup() { lcd.begin(16,2); Serial.begin(9600); Serial.println(F("BMP280 test")); lcd.print("Welcome to "); lcd.setCursor(0,1); lcd.print("CIRCUIT DIGEST"); delay(1000); lcd.clear(); if (!bmp.begin()) { Serial.println(F("Could not find a valid BMP280 sensor, check the wiring!")); while (1); }
This function works when the initializing of the bmp object is failed.
/* Default settings from datasheet. */ bmp.setSampling(Adafruit_BMP280::MODE_NORMAL, /* Operating Mode. */ Adafruit_BMP280::SAMPLING_X2, /* Temp. oversampling */ Adafruit_BMP280::SAMPLING_X16, /* Pressure oversampling */ Adafruit_BMP280::FILTER_X16, /* Filtering. */ Adafruit_BMP280::STANDBY_MS_500); /* Standby time. */ }
This part of the code prints the temperature on serial monitor and is for debugging purposes.
void loop() { Serial.print(F("Temperature = ")); Serial.print(bmp.readTemperature()); Serial.println(" *C");
The function bmp.readPressure and bmp.readTemprature are used to invoke special functions and return the temperature and pressure values.
lcd.print(bmp.readTemperature()); lcd.print(bmp.readPressure());
Working of Arduino BMP280 Pressure Sensor Interfacing Project
The functions bmp.readTemprature() and bmp.readPressure() are used to return the temperature and pressure values. These functions are a group of statements which perform a special task, in our case to return temperature and pressure files. These functions are invoked using bmp.readTemprature() and bmp.readPressure() functions. The lcd.setCursor sets the cursor of the LCD to the required position on the screen. The lcd.print command prints the data from the position set by the programmer. If there is no position set for the LCD by default it takes (0,0) as the initial position, and continuous printing the data. The next data takes the position of the next column, and the procedure continues until it reaches the end of the row and shifts to the next row.
The BMP280 can be is used in Flying toys, mobile phones, tablets, PCs, GPS devices, Portable health care devices, home weather stations, etc. By following this procedure and using header files and some special functions, we can easily interface BMP280 with the Arduino. The complete working can also be found in the video given below, hope you enjoyed this BMP280 Arduino tutorial and learned something useful. If you have any question leave them in the comment section below or use the forums for other technical questions.
Complete Project Code
#include <Wire.h>
#include <SPI.h>
#include <Adafruit_BMP280.h>
#include <LiquidCrystal.h>
Adafruit_BMP280 bmp; // I2C
//Adafruit_BMP280 bmp(BMP_CS); // hardware SPI
//Adafruit_BMP280 bmp(BMP_CS, BMP_MOSI, BMP_MISO, BMP_SCK);
LiquidCrystal lcd(9, 8, 5, 4, 3, 2);
void setup() {
lcd.begin(16,2);
Serial.begin(9600);
Serial.println(F("BMP280 test"));
lcd.print("Welcome to ");
lcd.setCursor(0,1);
lcd.print("CIRCUIT DIGEST");
delay(1000);
lcd.clear();
if (!bmp.begin()) {
Serial.println(F("Could not find a valid BMP280 sensor, check wiring!"));
while (1);
}
/* Default settings from datasheet. */
bmp.setSampling(Adafruit_BMP280::MODE_NORMAL, /* Operating Mode. */
Adafruit_BMP280::SAMPLING_X2, /* Temp. oversampling */
Adafruit_BMP280::SAMPLING_X16, /* Pressure oversampling */
Adafruit_BMP280::FILTER_X16, /* Filtering. */
Adafruit_BMP280::STANDBY_MS_500); /* Standby time. */
}
void loop() {
Serial.print(F("Temperature = "));
Serial.print(bmp.readTemperature());
Serial.println(" *C");
lcd.setCursor(0,0);
lcd.print("Temp= ");
lcd.print(bmp.readTemperature());
Serial.print(F("Pressure = "));
Serial.print(bmp.readPressure());
Serial.println(" Pa");
lcd.setCursor(0,1);
lcd.print("Press= ");
lcd.print(bmp.readPressure());
Serial.print(F("Approx altitude = "));
Serial.print(bmp.readAltitude(1018)); /* Adjusted to local forecast! */
Serial.println(" m");
Serial.println();
delay(2000);
}