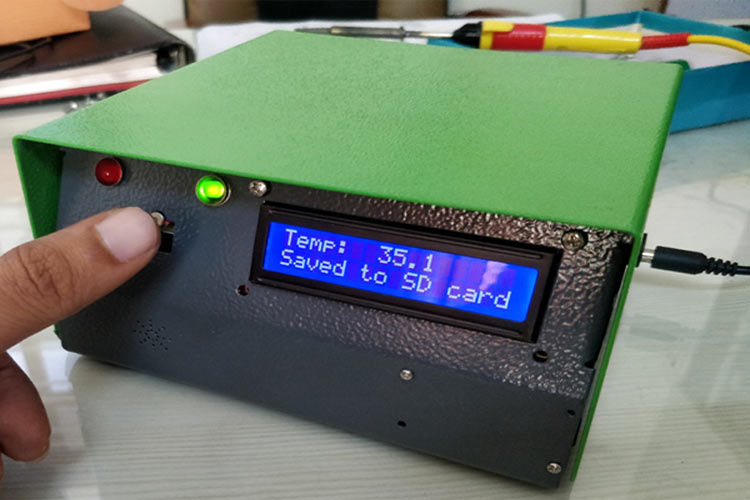
Scanning temperature at the entry gates of office, malls, shopping centers, etc. has become the “new normal” to tackle the current Covid19 pandemic. While it is possible to use a handheld IR thermometer to measure the temperature of each person entering the gate and note it down manually on a notebook, it is simply not the most effective way. We have already designed a Mobile Phone based smart IR Thermometer that can record the temperature of a person with a photograph and save it in an Excel file that can be shared on WhatsApp or Gmail. It has a lot of useful features but again this device also needs human intervention to measure the temperature of each individual.
So, in this project, we will design a wall mounted IR Thermometer using Arduino, that can be simply fixed on a wall and left turned on. Every induvial entering the premises can scan their temperature by stepping forward to this IR temperature sensor and it will measure the temperature of the individual and display it on the LCD. Apart from that, it also logs the time and temperature of the employee/visitor in excel format on an SD card. It is also very easy to add an RFID reader or a barcode scanner to this project to detect the employee's name and log the temperature and time against his name. This way, the device can also act as an attendance system since the time is also logged. Interesting right!! So let's get started….
Materials Required for Wall Mount IR Thermometer
- Arduino nano
- MLX90615
- TCRT5000
- DS3231 RTC module
- 16*2 LCD
- SD Card Module
- Buzzer
- Red LED
- Green LED
- Perf Board
- 1K, 4.7K, 10K Resistors
- 10K Potentiometer
- DC Barrel Jack
- 12V 1A DC Power Adapter
- LED Mount brackets
- Metal enclosure and screws
Wall Mounted Digital IR Thermometer Circuit Diagram
The complete schematic for Digital IR thermometer is given below. It might look like a complex circuit but most of them are simple interfacing circuits.
MLX90615 Non-Contact IR Temperature Sensor: The most important component in the circuit is the contactless Temperature sensor MLX90615. We have previously used the same sensor in our Smart Phone IR Thermometer project. The sensor works on the principle that all hot objects (including humans) emit IR rays and the intensity of the IR rays will be proportional to the temperature of the object. So, the sensor measures the temperature of the object by measuring the IR rays emitted from the object. The MLX90615 is a digital IR temperature sensor, meaning it has its own internal circuit to measure IR light from an object and directly convert it to a temperature value. This output temperature can be read using I2C communication, the pinout of MLX90615 is shown below.
TCRT5000 IR proximity Sensor: The next sensor we have on our device is the TCRT5000 IR proximity sensor. This sensor is used to detect if a person has placed their hand in front of the sensor. It is a simple proximity sensor that we have used in many projects previously like the Remote controlled Arduino Car that we built earlier. But in this project, we have used it a bit more efficiently to tackle the sunlight problem with IR sensor.
The TCRT5000 being an IR sensor can be falsely triggered by sunlight because the sun rays also consist of IR rays. To avoid the false triggering due to sunlight, we have connected the IR receiver LED to the analog port of Arduino and IR emitter to a digital pin. This way we can measure the IR signal received by the IR receiver when the IR emitter is turned on and again we can measure it when the IR emitter is turned off. Taking a difference between these two values will help us find the noise in the environment and thus avoid false triggering.
DS3231 RTC module: The DS3231 RTC module is used to keep track of the time and date. The RTC module has its own inbuilt battery so the device will remember the time even when powered is turned off. However, we have to set the correct time before we use it for the first time. We will not be discussing on how to do that in this article. DS3231 also communicated with I2C pins and hence is connected to the same pins of the MLX90615. However, both the modules have a different I2C address and hence we need not worry about the signals interfering.
SD card module: The SD card module is used to connect an SD card with our Arduino microcontroller. It is used to save the value of the temperature and time of the scan in a text file. The module works with SPI communication protocol and hence we have connected it to the SPI pins of Arduino as shown above.
16x2 LCD Display: The LCD display is used to display the value of temperature and time. It is a simple 16x2 alphanumeric LCD display that we have used in many other projects.
LED and Buzzer: Finally, we have the LEDs and Buzzer for output indication. The buzzer will beep once along with the green LED if the temperature is normal and the buzzer will beep multiple times along with the Red LED to indicate a high temperature.
As you can see there is nothing very complex with the circuit diagram, we have just directly connected the module with Arduino. And apart from that, we have few resistors for the LED and buzzer to act as current limiting resistors and the other 4.7k ohm resistors on the I2C bus is used to pull-up resistors. We also have a potentiometer connected to the LCD to adjust the contrast level of the LCD.
Building the Circuit on Perf Board
As you can see from the main image, the idea was to use this circuit inside a sheet metal enclosure. So the complete circuit shown above is soldered onto a perf board. Make sure to use wires to leave enough distance to mount the LCD, LED, Sensor, and Buzzers. I have used two perf boards, one for placing Arduino nano, DS3231, and all passive components and other for the two sensors (MLX90615 and TCRT500), based on your mounting enclosure, you can solder it in a different way. My perf board soldered to LCD and sensor module is shown below.
Installing the Required Libraries for MLX90615, DS3231, and SD Card
Now that the hardware is ready, its time to start programming our IR digital thermometer. The complete code for this project is given at the end of this page, but before we start, it is important to add the required libraries to your Arduino IDE. Make sure you add the right libraries from the below link else the code will throw errors when compiled.
Programming Arduino for Wall Mounted Thermometer
After adding the required libraries, you can directly upload the code given at the bottom of this page to check if the circuit is working. If you want to know how the code works, then keep reading...
Like always, we begin the program by adding the required header files and defining the pin names.
#define Buzz 5 #define Green 3 #define Red 4 #include <Wire.h> #include "DS3231.h" #include "MLX90615.h" #include <LiquidCrystal.h> #include <SPI.h> //Library for SPI communication (Pre-Loaded into Arduino) #include <SD.h> //Library for SD card (Pre-Loaded into Arduino)
Then we have two main global variables that have to be adjusted based on your application. The first variable is “error_correction” which is the value of temperature added to the original value from the MLX90615 sensor. Even though the MLX90615 sensor is factory calibrated, I noticed that the values from it were very low than original values, so I had to add the error_correction value to get reliable readings. I used my handheld meter to calibrate for these values. After calibration though, I found the sensor was very reliable in all ranges. The next variable is the Range_sensitivity, this value is used to determine how close the person should get before triggering our device. I have used a value of 200 but you can decrease this value if you want to increase the range.
float error_correction = 4; //add this to actual value int Range_sensitivity = 200; //Decrease this value to increase range
Moving on with our code, we have the Initialize SD Card function which is used to open SPI communication with SD card and create a file inside the SD card called “Temp_Log.text”. Then we will also open this file and write the heading for log. Here we are logging Date, Time, and Temperature so we name the heading accordingly. This function will be called only once when during the setup function execution.
void Initialize_SDcard() { // see if the card is present and can be initialized: if (!SD.begin(chipSelect)) { Serial.println("Card failed, or not present"); // don't do anything more: return; } // open the file. note that only one file can be open at a time, // so you have to close this one before opening another. File dataFile = SD.open("Temp_Log.txt", FILE_WRITE); // if the file is available, write to it: if (dataFile) { dataFile.println("Date,Time,Temperature"); //Write the first row of the excel file dataFile.close(); } }
Then we have the write SD card function which is used to log the actual values into the text file that we just created. This function will be called every time when a new reading is taken. The format of the logging will be comma-separated like “Date,Time,Temperature”, an example log will be something like “19.08.2020,10:45:17,35.6”. It might be a bit difficult to read but this format is called comma-separated values and it can be easily opened and understood by an excel while. Using an SD card for Data Logging is very similar to the Arduino Data Logger Project that we build earlier.
void Write_SDcard() { // open the file. note that only one file can be open at a time, // so you have to close this one before opening another. File dataFile = SD.open("Temp_Log.txt", FILE_WRITE); // if the file is available, write to it: if (dataFile) { dataFile.print(rtc.getDateStr()); //Store date on SD card dataFile.print(","); //Move to next column using a "," dataFile.print(rtc.getTimeStr()); //Store date on SD card dataFile.print(","); //Move to next column using a "," dataFile.print(temperature); //Store date on SD card dataFile.println(); //End of Row move to next row dataFile.close(); //Close the file } else Serial.println("OOPS!! SD card writing failed"); }
Proceeding further with the code, we have the void setup function where we initialize the device. We just display some intro message on the LCD and also initialize the SD card ready for use. There is nothing very serious to explain here.
void setup() { Serial.begin(9600); rtc.begin(); lcd.begin(16, 2); lcd.print("Temp. Scanner"); lcd.setCursor(0,1); lcd.print("CircuitDigest"); pinMode(2,OUTPUT); pinMode(Buzz,OUTPUT); pinMode(Red,OUTPUT); pinMode(Green,OUTPUT); mlx.begin(); digitalWrite(Buzz,LOW); digitalWrite(Red,LOW); digitalWrite(Green,LOW); Initialize_SDcard(); }
Next, inside the void loop function, our code also gets the current time and date and updates it on the LCD. Then we turn on digital pin 2 which is connected to the IR emitter LED and perform analog read on in A7 to which the IR receiver LED is connected. Then we again repeat this with the IR led turned off. This helps us to measure the Noise and Noise+Signal value from the IR sensor. Then we only have to subtract Noise value from the Noise+Signal value to get the value of Signal.
lcd.setCursor(0,1); lcd.print("Date: "); lcd.print(rtc.getDateStr()); digitalWrite(2,HIGH); // Turn on IR LED delayMicroseconds(500); // Forward rise time of IR LED Noise_P_Signal=analogRead(A7); // Read value from A0 => noise+signal digitalWrite(2,LOW); // Turn off IR LED delayMicroseconds(500); // Fall time of IR LED Noise=analogRead(A7); // Read value from A0 => noise only Signal = Noise - Noise_P_Signal;
The signal value will tell us how close the person is to the TCRT5000 IR sensor without the influence of the sunlight around the person. Then by comparing this signal value and noise value, we will trigger our thermometer to read the temperature value and also store it in the SD card. If the temperature is normal the Green Led will turn on and if the temperature is high the Red LED will turn on.
if (Signal>Range_sensitivity && Noise >500) //dec. signal to increase rage { digitalWrite(Buzz,HIGH); if (trigger == true) Serial.println("start") digitalWrite(2,LOW); //Turn off IR sensor to avoid interferance. for (int i=1; i<=3; i++) { temperature = (mlx.get_object_temp()) + error_correction; Serial.println(temperature,1); delay(150); } digitalWrite(Buzz,LOW); lcd.clear(); lcd.setCursor(0,0); lcd.print("Temp: "); lcd.print(temperature,1); lcd.setCursor(0,1); lcd.print("Saved to SD card"); Write_SDcard();
Wall Mount Thermometer Enclosure – Assembling
After testing the hardware and code, we can assemble our project in a permanent enclosure to install it in a facility. We have designed and fabricated a sheet metal enclosure for this purpose. The design was made considering the size of the LCD display, LEDs, and other components used in the project. Then the design was taken to a laser cutting shop to cut and bend the sheet metal according to design. Finally, it was given for a powder coating to improve the aesthetic look and cover the metal parts.
After receiving the casing, we simply have to mount the LCD, DC connector, and LES in the respective slots. Make sure the electronics part is well insulated from the metal enclosure. The above image shows the bottom and top part of the enclosure with the electronics assembled. And after the assembly was completed, the enclosure looked something like this below.
Wall Mounted Digital IR Thermometer Testing
After the assembling is done, it is time to test the device. We used an external 12V 1A adapter to power the device and mounted the device on the wall.
As you can see by default device will display time and date. If a person steps in front of the device sensor or shows his hand, it will read the temperature of the person and display it on the screen as shown in the below picture taken during testing. It will also store the same value with time and date on the SD card.
At the end of the day when all the temperature reading has been taken, the SD card can be removed from the device using the slot on the side. Just connect this SD card to a computer and you will find a file called Temp_Log.text with all the temperature values taken with the device. My recorded file that was created during testing is shown below.
As you can see, the values are not easy to read on a notepad like this. So it is always better to open the file using Microsoft Excel. Just open it as a CSV file and use a comma as a separator. The excel file will open our data something like this.
The complete working of the project can also be found in the video linked below. Hope you enjoyed the project and found it interesting to build your own. If you have any questions, please leave them in the comment section below. You can also write all your technical questions on forums to get them answered or to start a discussion.
Complete Project Code
/*Wall Mounted Temperature Scanner with SD Logging
* Website: circuitdigest.com
* Code by: B.Aswinth Raj
*/
float error_correction = 4; //add this to actual value
int Range_sensitivity = 200; //Decrease this value to increase range
#define Buzz 5
#define Green 3
#define Red 4
#include <Wire.h>
#include "DS3231.h"
#include "MLX90615.h"
#include <LiquidCrystal.h>
#include <SPI.h> //Library for SPI communication (Pre-Loaded into Arduino)
#include <SD.h> //Library for SD card (Pre-Loaded into Arduino)
DS3231 rtc(SDA, SCL); //RTC is connected via I2C
LiquidCrystal lcd(9, 8, 14, 15, 16, 17); // initialize the library with the numbers of the interface pins
MLX90615 mlx = MLX90615();
const int chipSelect = 10; //SD card CS pin connected to pin 4 of Arduino
int Noise;
int Signal;
int Noise_P_Signal;
boolean trigger = true;
float temperature;
float pvs_temperature;
void Initialize_SDcard()
{
// see if the card is present and can be initialized:
if (!SD.begin(chipSelect)) {
Serial.println("Card failed, or not present");
// don't do anything more:
return;
}
// open the file. note that only one file can be open at a time,
// so you have to close this one before opening another.
File dataFile = SD.open("Temp_Log.txt", FILE_WRITE);
// if the file is available, write to it:
if (dataFile) {
dataFile.println("Date,Time,Temperature"); //Write the first row of the excel file
dataFile.close();
}
}
void Write_SDcard()
{
// open the file. note that only one file can be open at a time,
// so you have to close this one before opening another.
File dataFile = SD.open("Temp_Log.txt", FILE_WRITE);
// if the file is available, write to it:
if (dataFile) {
dataFile.print(rtc.getDateStr()); //Store date on SD card
dataFile.print(","); //Move to next column using a ","
dataFile.print(rtc.getTimeStr()); //Store date on SD card
dataFile.print(","); //Move to next column using a ","
dataFile.print(temperature); //Store date on SD card
dataFile.println(); //End of Row move to next row
dataFile.close(); //Close the file
}
else
Serial.println("OOPS!! SD card writing failed");
}
void setup() {
Serial.begin(9600);
rtc.begin();
lcd.begin(16, 2);
lcd.print("Temp. Scanner");
lcd.setCursor(0,1);
lcd.print("CircuitDigest");
pinMode(2,OUTPUT);
pinMode(Buzz,OUTPUT);
pinMode(Red,OUTPUT);
pinMode(Green,OUTPUT);
mlx.begin();
digitalWrite(Buzz,LOW);
digitalWrite(Red,LOW);
digitalWrite(Green,LOW);
Initialize_SDcard();
}
void loop() {
lcd.setCursor(0,0);
lcd.print("Time: ");
lcd.print(rtc.getTimeStr());
lcd.setCursor(0,1);
lcd.print("Date: ");
lcd.print(rtc.getDateStr());
digitalWrite(2,HIGH); // Turn on IR LED
delayMicroseconds(500); // Forward rise time of IR LED
Noise_P_Signal=analogRead(A7); // Read value from A0 => noise+signal
digitalWrite(2,LOW); // Turn off IR LED
delayMicroseconds(500); // Fall time of IR LED
Noise=analogRead(A7); // Read value from A0 => noise only
Signal = Noise - Noise_P_Signal;
if (Signal>Range_sensitivity && Noise >500) //dec. signal to increase rage
{
digitalWrite(Buzz,HIGH);
if (trigger == true)
Serial.println("start");
digitalWrite(2,LOW); //Turn off IR sensor to avoid interferance.
for (int i=1; i<=3; i++)
{
temperature = (mlx.get_object_temp()) + error_correction;
Serial.println(temperature,1);
delay(150);
}
digitalWrite(Buzz,LOW);
lcd.clear();
lcd.setCursor(0,0);
lcd.print("Temp: ");
lcd.print(temperature,1);
lcd.setCursor(0,1);
lcd.print("Saved to SD card");
Write_SDcard();
if (temperature>38)
{
digitalWrite(Red,HIGH);
digitalWrite(Buzz,HIGH);
delay (5000);
}
else
{
digitalWrite(Green,HIGH);
delay (1000);
}
trigger = false;
}
else
{
delay(100);
trigger = true;
digitalWrite(13,LOW);
Serial.println("position_error");
}
digitalWrite(Red,LOW);
digitalWrite(Buzz,LOW);
digitalWrite(Green,LOW);
}
Comments
did you solve your problem?
did you solve your problem? because i have the same problem.
You should have used wring library
Try getting the library from here
https://github.com/CircuitDigest/Smart-Contactless-Thermometer-Arduino-…
Error in MLX90615 library
Hi! I'm also getting an error in the Library file of MLX90615.
I have downloaded the file for, gitHub also, as per your link, but the problem still remains!!
The error is: no matching function for call to 'MLX90615::MLX90615()'
Also, will it be possible to add a switch/button to change the error_correction value(s) for (1)Forehead & (2)Back of Hand option!!
Please help.
Thanks.
Bro Can you please tell me
Bro Can you please tell me that the error which you were getting has been resolved or not?
what the solution of this
what the solution of this problem " no matching function for call to 'MLX90615::MLX90615()' "??
because i have same problem.
Hello
Hello
I am an electrical student and i am new to Arduino stuff. I have a question i think you will find it stupid but i'll ask it anyway. As i searched about Arduino Nano i found that the A pins are analogue input so why did you connect from A0 to A3 pins to the LCD screen? The only explanation that i have is that you are using them as outputs to feed data to the LCD screen. So now i am confused are these inputs or outputs or both?
I read the code carefully and couldn't find an explanation so if anyone can explain this to me it would appreciate it very much.
Oh nevermind. I found the
Oh nevermind. I found the answer.
Thanks anyways.
Can you please send a file of
Can you please send a file of the fixed to my email @brendankariithi@icloud.com? I've been having the same problems but I have not found a solution.
If i use MLX90614 instead of
If i use MLX90614 instead of MLX90615, will it work? What changes do i need to make?
I getting compile error even after installing all libraries. Please provide me a sol
ution.