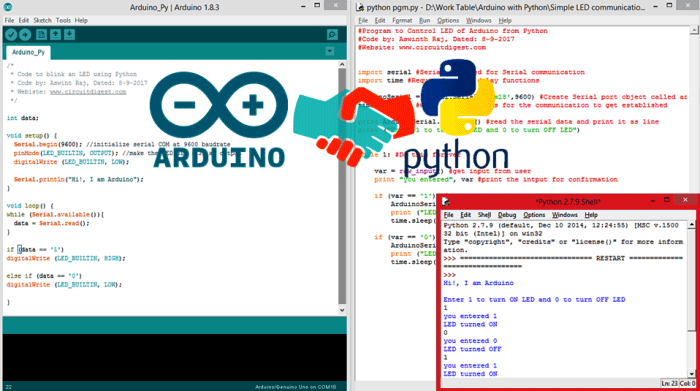
Arduino has always been a powerful and an easy to use learning/developing platform when it comes to open source hardware development. In today’s modern world, every hardware is powered by a high-level general purpose programming language to make it more effective and user friendly. One such language is Python. Python is an interpreted, object-oriented, high-level programming language with dynamic semantics with high-level built in data structures, combined with dynamic typing and dynamic binding, make it very attractive for Rapid Application Development.
Combining the power of Arduino and Python will open doors to lots of possibilities since python has an increased productivity with its ability to interact with other platforms like openCV, Matlab etc.. So in this tutorial we will learn how we can install python on our computer and how to use it with Arduino for toggling the on-board LED of Arduino.
So, Let’s get started....
Materials Required:
- Arduino Uno (or any Arduino Boards)
- Computer with Internet connection
Installing Python on your Computer:
Obviously the first step in this tutorial would be installing Python on our computer. The steps mentioned below are applicable only for windows users running either 32-bit or 64-bit OS. The installation procedure for MAC and Linux is different.
- Click on 32-bit Python-2.7.9 and this will install the 32-bit Python IDLE on your Computer. Do not download the 64-bit version or updated versions since they do not provide support for our Arduino Libraries. Even if your Computer is operating on 64-bit you can use 32-bit Python itself.
- Open the downloaded exe file and follow through the instruction. Do not change the directory in which the python is getting installed. It will be C:\Python27 by default and leave it as such.
- While the installation takes place you might get a warning from your anti-virus (if any) in that case click on allow.
That is it!, python is successfully installed on our computer. You can verify it by searching for “Python IDLE” in Windows search box and opening it.
When opened you should get the following screen. This window is called the Python Shell and we will refer to it as “Python shell” from now.
This screen is called the Python Shell. You can directly code in here and get the output on the same screen or create a new file and write the program there and verify the program here. We will later get into the details of creating a python program, for now let us check if python is working.
To do so, simply type “print (1+1)” and press enter. You should see the result getting printed as shown below.
Getting PySerial in Python:
The next step is to install pyserial. PySerial is a Python API module which is used to read and write serial data to Arduino or any other Microcontroller.
Click on Pyserial Windows to download PySerial. The resulting download will be a exe file which can be directly installed. Do not change any setting while installing. Leave it to the default directory and default settings.
Now, let us check if PySerial is installed properly. To do this, open Python Shell again and type in
import serial. If the library was successfully installed then you should not get any error messages as shown in the picture below. If you get any errors post them on the comment section and we will try resolving it.
This tutorial assumes that you are familiar with Arduino and have experience in uploading projects to Arduino. So let us directly jump into our Python program. If you are a beginner with Arduino check our Arduino Projects and start from LED Blinking with Arduino.
Our First Arduino Python Program:
As said earlier we will be controlling the in-built Arduino board LED using Python script. Let us start with the Arduino code.
Program for Arduino:
The complete program for this Arduino python tutorial is given at the end of this page. Read further to know how it works.
Inside the setup function we initialize the serial communication at 9600 baud rate and declare that we will be using the built in led as output and turn it low during program start. We have also sent a welcome message to python via serial print as shown below:
void setup() { Serial.begin(9600); //initialize serial COM at 9600 baudrate pinMode(LED_BUILTIN, OUTPUT); //make the LED pin (13) as output digitalWrite (LED_BUILTIN, LOW); Serial.println("Hi!, I am Arduino"); }
Inside the loop function, we read whatever the data that is coming in serially and assigning the value to the variable “data”. Now based on the value of this variable (“data”) we toggle the built in led as shown below.
void loop() { while (Serial.available()){ data = Serial.read(); } if (data == '1') digitalWrite (LED_BUILTIN, HIGH); else if (data == '0') digitalWrite (LED_BUILTIN, LOW); }
Program for Python:
The complete python program for this tutorial is given at the end of this page. Read further to know how to write and use the same.
- Open your Python Shell (Python IDLE) and click File->New
- This will open a new text file where you can type in your program.
- Before we type anything lets save the file, by Ctrl+S. Type in any name and click on save. This will automatically save you file in “.py” extension.
- Now, type in the program or paste the python code given at the end of this page. The explanation for the same is given below and finally run the program.
In our program the first step would be to import the serial and time library. The serial library as said earlier will be used to read and write serial data and the time library will be used to create delays in our program. These two libraries can be imported in our program using the following two lines:
import serial #Serial imported for Serial communication import time #Required to use delay functions
The next step would be to initialize a serial object using our serial library. In this program we have named our serial object as “ArduinoSerial”. In this line we have to mention the name of the COM port to which our Arduino is connected and at what baud rate it is operating as shown below.
ArduinoSerial = serial.Serial('com18',9600)
Note: It is very important to mention the correct COM port name. It can found by using the Device manager on your computer.
As soon the serial object is initialized we should hold the program for two seconds for the Serial communication to be established. This can be done by using the below line:
time.sleep(2)
Now we can read or write anything from/to our Arduino Board.
The following line will read anything coming from Arduino and will print it on the shell window
print ArduinoSerial.readline()
You can also assign the value to a variable and use it for computations.
The following line will write the value of the parameter to Arduino Board.
ArduinoSerial.write('1')
This line will write ‘1’ to the Arduino. You can send anything from decimals to strings using the same line.
Now, getting back to our program, inside the infinite while loop, we have the following lines
var = raw_input() #get input from user print "you entered", var #print the input for confirmation if (var == '1'): #if the value is 1 ArduinoSerial.write('1') #send 1 print ("LED turned ON") time.sleep(1) if (var == '0'): #if the value is 0 ArduinoSerial.write('0') #send 0 print ("LED turned OFF") time.sleep(1)
The line var=raw_input will get any value that is typed in the Shell script and assign that value to the variable var.
Later, if the value is 1 it will print ‘1’ serially to Arduino and if 0 it will print ‘0’ serially to Arduino. The code in our Arduino Program (discussed above) we will toggle the LED based on the received value.
Once the complete program is done your script should look something like this below
Now click on Run -> Run Module or press F5 this might ask you to save the program and then will launch it.
Controlling LED with Python and Arduino:
The working of this project is pretty straight forward. Upload the program to your Arduino and verify it is connected to the same COM port as mentioned in the python program. Then Launch the Python program as mentioned above.
This will launch a python shell script as shown below. The window on the left is the shell window showing the output and the window on the right is the script showing the program.
As you can see the string “Hi!, I am Arduino” entered in the Arduino program is received by the Python and displayed on its shell window.
When the shell window asks to enter values, we can enter either 0 or 1. If we send 1 the LED on the Arduino Board will turn ON and if we send 0 the LED on our Arduino Board will turn OFF. Showing a successfully connection between our Arduino Program and Python.
There are two program given below, one to be uploaded and run from Arduino and second is to be run from Python Shell in Windows.
Hope you understood the project and were able to get it working. If not, post your problem in the comment below and I will be happy to help you out. In our next project we will learn what else can be done cool with Python and Arduino by exploring deep into other python modules like Vpython, gamepython etc. Until then stay tuned....
Program for Arduino:
/*
* Code to blink an LED using Python
* Code by: Aswint Raj, Dated: 8-9-2017
* Webiste: www.circuitdigest.com
*/
int data;
void setup() {
Serial.begin(9600); //initialize serial COM at 9600 baudrate
pinMode(LED_BUILTIN, OUTPUT); //make the LED pin (13) as output
digitalWrite (LED_BUILTIN, LOW);
Serial.println("Hi!, I am Arduino");
}
void loop() {
while (Serial.available()){
data = Serial.read();
}
if (data == '1')
digitalWrite (LED_BUILTIN, HIGH);
else if (data == '0')
digitalWrite (LED_BUILTIN, LOW);
}
Python Program for Windows:
#Program to Control LED of Arduino from Python
#Code by: Aswinth Raj, Dated: 8-9-2017
#Website: www.circuitdigest.com
import serial #Serial imported for Serial communication
import time #Required to use delay functions
ArduinoSerial = serial.Serial('com18',9600) #Create Serial port object called arduinoSerialData
time.sleep(2) #wait for 2 secounds for the communication to get established
print ArduinoSerial.readline() #read the serial data and print it as line
print ("Enter 1 to turn ON LED and 0 to turn OFF LED")
while 1: #Do this forever
var = raw_input() #get input from user
print "you entered", var #print the intput for confirmation
if (var == '1'): #if the value is 1
ArduinoSerial.write('1') #send 1
print ("LED turned ON")
time.sleep(1)
if (var == '0'): #if the value is 0
ArduinoSerial.write('0') #send 0
print ("LED turned OFF")
time.sleep(1)
Comments
You get this error because
You get this error because you should missed adding the serial librarey to your Python code.
This line "import serial" should have been deleted by mistake in your code.
you dont have the module
You just don't have the module installed in your Python library........To install it open up your command prompt and type "pip install pyserial" in the python directory of your computer. Hope that solves your problem.
Python 2.7.9 (default, Dec 10
Python 2.7.9 (default, Dec 10 2014, 12:24:55) [MSC v.1500 32 bit (Intel)] on win32
Type "copyright", "credits" or "license()" for more information.
>>> ================================ RESTART ================================
>>>
Traceback (most recent call last):
File "C:\Python27\arduino_py\my_1st_project.py", line 4, in <module>
ArduinoSerial = serial.Serial('com18',9600) #Create Serial port object called arduinoSerialData
File "C:\Python27\lib\site-packages\serial\serialwin32.py", line 38, in __init__
SerialBase.__init__(self, *args, **kwargs)
File "C:\Python27\lib\site-packages\serial\serialutil.py", line 282, in __init__
self.open()
File "C:\Python27\lib\site-packages\serial\serialwin32.py", line 66, in open
raise SerialException("could not open port %r: %r" % (self.portstr, ctypes.WinError()))
SerialException: could not open port 'com18': WindowsError(2, 'The system cannot find the file specified.')
>>>
When I run the Python, I get this error.
How to rectify this error?Please help me in solving this....
same error what you have mentioned
not working properly
not working properly
>>> import serial
Traceback (most recent call last):
File "<pyshell#1>", line 1, in <module>
import serial
ImportError: No module named serial
>>>
Please click on this link and
Please click on this link and add the pyserial librarey
https://pypi.python.org/packages/47/c9/7802e11ab388ad1539de716649add8bb…
This should solve the problem
No, you still get this error
No, you still get this error even when pyserial has been added.
I've fixed my problem already
I've fixed my problem already. I'm sorry for my carelessness. My problem is putting wrong com port.
Glad you got it working!!
Glad you got it working!!
All the best
Python to Arduino
I don't get any errors but nothing prints out. I'm running python 3.5.2. I did add the parenthesis to all five print statements. See example of one of them below.
print(ArduinoSerial.readline())
Python to Arduino
I got it working by changing the following lines.
ArduinoSerial = serial.Serial('com18',9600)
to
ArduinoSerial = serial.Serial('com18',9600, timeout=0)
var = raw_input() #get input from user
to
var = input() #get input from user
ArduinoSerial.write('1') & ArduinoSerial.write('0')
to
ArduinoSerial.write(bytes(var.encode('ascii')))
Traceback (most recent call
Traceback (most recent call last):
File "<pyshell#0>", line 1, in <module>
import serial
File "C:\Users\Lenovo\AppData\Local\Programs\Python\Python35-32\lib\site-packages\serial\__init__.py", line 19, in <module>
from serial.serialwin32 import *
File "C:\Users\Lenovo\AppData\Local\Programs\Python\Python35-32\lib\site-packages\serial\serialwin32.py", line 12, in <module>
from serial import win32
File "C:\Users\Lenovo\AppData\Local\Programs\Python\Python35-32\lib\site-packages\serial\win32.py", line 196
MAXDWORD = 4294967295L # Variable c_uint
^
SyntaxError: invalid syntax
how could I solve this error
Check if you have installed
Check if you have installed the correct serialpy librarey. Also try a basic serial program to check if it is working
Superb what a perfect and
Superb what a perfect and clear explanation. I have a doubt that , the output shown in python shell is what output of the controller. For example we say if we interface LCD with arduino, the output of the python shell will be displayed in LCD?? or the output of arduino serial monitor will be displayed??.
Error message
>>> ArduinoSerial = serial.Serial('com',9600)
Traceback (most recent call last):
File "<pyshell#18>", line 1, in <module>
ArduinoSerial = serial.Serial('com',9600)
File "C:\Python27\lib\site-packages\serial\serialwin32.py", line 38, in __init__
SerialBase.__init__(self, *args, **kwargs)
File "C:\Python27\lib\site-packages\serial\serialutil.py", line 282, in __init__
self.open()
File "C:\Python27\lib\site-packages\serial\serialwin32.py", line 66, in open
raise SerialException("could not open port %r: %r" % (self.portstr, ctypes.WinError()))
SerialException: could not open port 'com': WindowsError(2, 'The system cannot find the file specified.')
pls help me
I am not able to get the
I am not able to get the output on python shell. When i run the phython code it only shows Hi! I am arduino press 1 or 0, after entering the value nothing is happening. plzz help.
This means that your Arduino
This means that your Arduino is not responding to the commands sent from python. Try opening your Arduino Serial monitor directly and press 1 or 0 to check if the LED is toggled
After i run Python i recive
After i run Python i recive this message :
Python 2.7.9 (default, Dec 10 2014, 12:24:55) [MSC v.1500 32 bit (Intel)] on win32
Type "copyright", "credits" or "license()" for more information.
>>> import serial
>>> ================================ RESTART ================================
>>>
Traceback (most recent call last):
File "G:\Arduino Projects\Python\LED.py", line 7, in <module>
ArduinoSerial=serial.Serial('com3',9600) #Create Serial port object called arduinoSerialData
File "C:\Python27\lib\site-packages\serial\serialwin32.py", line 38, in __init__
SerialBase.__init__(self, *args, **kwargs)
File "C:\Python27\lib\site-packages\serial\serialutil.py", line 282, in __init__
self.open()
File "C:\Python27\lib\site-packages\serial\serialwin32.py", line 66, in open
raise SerialException("could not open port %r: %r" % (self.portstr, ctypes.WinError()))
SerialException: could not open port 'com3': WindowsError(2, 'The system cannot find the file specified.')
I think your PySerial
I think your PySerial librarey was not installed properly. "import serial" should not throw you an error
Hello, please help me how can
Hello, please help me how can i find the problem here
void setup() {
Serial.begin(9600); //initialize serial COM at 9600 baudrate
pinMode(LED_BUILTIN, OUTPUT); //make the LED pin (13) as output
digitalWrite (LED_BUILTIN, LOW);
Serial.println("Hi!, I am Arduino");
}
void loop() {
while (Serial.available()){
data = Serial.read();
}
if (data == '1')
digitalWrite (LED_BUILTIN, HIGH);
else if (data == '0')
digitalWrite (LED_BUILTIN, LOW);
}
Arduino: 1.8.4 (Windows 7), Board: "Arduino/Genuino Uno"
Sketch uses 1826 bytes (5%) of program storage space. Maximum is 32256 bytes.
Global variables use 208 bytes (10%) of dynamic memory, leaving 1840 bytes for local variables. Maximum is 2048 bytes.
avrdude: ser_open(): can't open device "\\.\COM1": The system cannot find the file specified.
Problem uploading to board. See http://www.arduino.cc/en/Guide/Troubleshooting#upload for suggestions.
This report would have more information with
"Show verbose output during compilation"
option enabled in File -> Preferences.
I think it is mostly likely a
I think it is mostly likely a driver problem. Did you try few example programs of arduino? are they working?
Hello sir,
Hello sir,
That dosen't work, for example the blinking the external LED. I recived the nextmessage,
Arduino: 1.8.5 (Windows 7), Board: "Arduino/Genuino Uno"
Sketch uses 928 bytes (2%) of program storage space. Maximum is 32256 bytes.
Global variables use 9 bytes (0%) of dynamic memory, leaving 2039 bytes for local variables. Maximum is 2048 bytes.
avrdude: ser_open(): can't open device "\\.\COM1": The system cannot find the file specified.
"can't open device "\\.\COM1"
"can't open device "\\.\COM1"
This error indicates that your Arduino IDE was not able to communicate with your COM 1.
1. Use device manager and check to which COM port you have connected the Arduino and menton the same on you IDE
2. Make sure you have closed the Serial monitor of Arduino (if opened pvsly) before uploading the code
Whenever I try to run module,
Whenever I try to run module, it keeps showing "Invalid Syntax" and highlighted ArduinoSerial of print ArduinoSerial.readline()... For Arduino it is working fine and able to read 1 & 0. Any idea on how I can solve this issue?
Post the complete error
Post the complete error report so as to help you!
I am sorry,i have rectified
I am sorry,i have rectified my error in the code .The error is due to my carelessness.Thank you
python problem
Traceback (most recent call last):
File "<pyshell#1>", line 1, in <module>
import serial
ImportError: No module named serial
I installed pyserial-2.7
I installed pyserial-2.7.win32.exe
But when i called import serial have error
>>> import serial
Traceback (most recent call last):
File "<stdin>", line 1, in <module>
File "C:\Users\Thi Nguyen\AppData\Local\Programs\Python\Python36-32\lib\site-packages\serial\__init__.py", line 19, in <module>
from serial.serialwin32 import *
File "C:\Users\Thi Nguyen\AppData\Local\Programs\Python\Python36-32\lib\site-packages\serial\serialwin32.py", line 12, in <module>
from serial import win32
File "C:\Users\Thi Nguyen\AppData\Local\Programs\Python\Python36-32\lib\site-packages\serial\win32.py", line 196
MAXDWORD = 4294967295L # Variable c_uint
^
SyntaxError: invalid syntax
Please advise me !
i have this error message :
i have this error message : File "<tmp 1>", line 5
print ArduinoSerial.readline()
^
SyntaxError: invalid syntax
....
Traceback (most recent call
Traceback (most recent call last):
File "<pyshell#3>", line 1, in <module>
import serial
ImportError: No module named serial
You did not install the
You did not install the serial library properly, follow the instruction above to install it correctly
Error opening serial port 'COM5'. (Port busy)
hello sir,
I encountered a problem. When I opened the python shell to execute the program, I typed it in the python shell.
'1' or '0', "Error opening serial port 'COM5'. (Port busy)" appears in rduino. I use anaconda enviroment.
I hope you can help me, thank you very much.
Error opening serial port
Error opening serial port 'COM5'. (Port busy)
This is because the COM5 port is already being accessed by some other application. Most likely you should have left the serial monitor of the Arduino IDE open. Close it and try again
syntax Error
i am getting syntax error and ArduinoSerial is getting highlighted, can you please help??
Serial pport object is not getting created.
Whenever I try to run module, it keeps showing "Invalid Syntax" and highlighted ArduinoSerial of print ArduinoSerial.readline()... For Arduino it is working fine and able to read 1 & 0.
So I tried to execute single line in shell. Then I found that there is no Attribute named Serial in module serial. And I get this error.
>>> ArduinoSerial=serial.Serial('com8',9600)
Traceback (most recent call last):
File "<pyshell#7>", line 1, in <module>
ArduinoSerial=serial.Serial('com8',9600)
AttributeError: module 'serial' has no attribute 'Serial'
>>>
Help me to rectify this error.
thanks
>>> import serial
>>> import serial
Traceback (most recent call last):
File "<pyshell#2>", line 1, in <module>
import serial
File "C:\Users\Vidya Rao\AppData\Local\Programs\Python\Python36\lib\site-packages\serial\__init__.py", line 19, in <module>
from serial.serialwin32 import *
File "C:\Users\Vidya Rao\AppData\Local\Programs\Python\Python36\lib\site-packages\serial\serialwin32.py", line 12, in <module>
from serial import win32
File "C:\Users\Vidya Rao\AppData\Local\Programs\Python\Python36\lib\site-packages\serial\win32.py", line 196
MAXDWORD = 4294967295L # Variable c_uint
^
SyntaxError: invalid syntax
When trying to run the python
When trying to run the python code this is what I get:
Traceback (most recent call last):
File "C:/Python27/FirstProject.py", line 9, in <module>
ArduinoSerial = serial.Serial('com6',9600) #Create Serial port object called arduinoSerialData
File "C:\Python27\lib\site-packages\serial\serialwin32.py", line 38, in __init__
SerialBase.__init__(self, *args, **kwargs)
File "C:\Python27\lib\site-packages\serial\serialutil.py", line 282, in __init__
self.open()
File "C:\Python27\lib\site-packages\serial\serialwin32.py", line 66, in open
raise SerialException("could not open port %r: %r" % (self.portstr, ctypes.WinError()))
SerialException: could not open port 'com6': WindowsError(5, 'Access is denied.')
It is because you have
It is because you have selected the wrong COM port. Go to device managae and check to which ur board is connected to and use the on program. It should ne COM 6
regarding my project
hi
I am doing Arduino based project, in my project m using the python programming(laptop) to communicate with the Arduino Ethernet shield to control the fan and bulb by using the relay. I want to use the wifi to send and receive the signal from pc to Arduino and Arduino to pc. could you help me and tell me how can I do this.
what kind of thing I have to use for the bidirectional communication between the pc and the Arduino through the internet.
Error rising during importing serial module
Traceback (most recent call last):
File "<stdin>", line 1, in <module>
File "C:\Users\NCTC\AppData\Local\Programs\Python\Python36-32\lib\site-packages\serial\__init__.py", line 19, in <module>
from serial.serialwin32 import *
File "C:\Users\NCTC\AppData\Local\Programs\Python\Python36-32\lib\site-packages\serial\serialwin32.py", line 12, in <module>
from serial import win32
File "C:\Users\NCTC\AppData\Local\Programs\Python\Python36-32\lib\site-packages\serial\win32.py", line 196
MAXDWORD = 4294967295L # Variable c_uint
^
SyntaxError: invalid syntax
I receive this error whenever I try to upload this on my system and import eriale module
Did you include the python
Did you include the python serial library?
error
Hello sir, for me its showing below errors,
Traceback (most recent call last):
File "C:/Python27/python.py", line 8, in <module>
ArduinoSerial = serial.Serial('com3',9600) #Create Serial port object called arduinoSerialData
File "C:\Python27\lib\site-packages\serial\serialwin32.py", line 38, in __init__
SerialBase.__init__(self, *args, **kwargs)
File "C:\Python27\lib\site-packages\serial\serialutil.py", line 282, in __init__
self.open()
File "C:\Python27\lib\site-packages\serial\serialwin32.py", line 66, in open
raise SerialException("could not open port %r: %r" % (self.portstr, ctypes.WinError()))
SerialException: could not open port 'com3': WindowsError(5, 'Access is denied.')
>>>
error
Hello sir, for me its showing below errors,
Traceback (most recent call last):
File "C:/Python27/python.py", line 8, in <module>
ArduinoSerial = serial.Serial('com3',9600) #Create Serial port object called arduinoSerialData
File "C:\Python27\lib\site-packages\serial\serialwin32.py", line 38, in __init__
SerialBase.__init__(self, *args, **kwargs)
File "C:\Python27\lib\site-packages\serial\serialutil.py", line 282, in __init__
self.open()
File "C:\Python27\lib\site-packages\serial\serialwin32.py", line 66, in open
raise SerialException("could not open port %r: %r" % (self.portstr, ctypes.WinError()))
SerialException: could not open port 'com3': WindowsError(5, 'Access is denied.')
>>>
Thanks!! I got it correct....
Thanks!! I got it correct.....its working.
arduino libraries supporting 64-bit version or updated versions
Hey I had a question regarding the support for python's 64-bit version or updated versions with the arduino libraries. As i have to install pynput which is used by the latest updated version of python for my project. Can you please help me with the same?
What is your question?
What is your question?
Which platform are you trying to install this for? Windows?
print ArduinoSerial.readline()
Great tutorial right here. Thanks.
My codes is working properly, except i cannot seem to get to print the Serial.println("Hi!, I am Arduino"); into my shell.
what could be the problem?
Hi there.
Hi there.
I slightly changed your code so that it could be used in Python 3.7.
import serial #for Serial communication
import time #for delay functions
arduino = serial.Serial('com4',9600) #Create Serial port object called arduinoSerialData
time.sleep(2) #wait for 2 secounds for the communication to get established
print (arduino.readline()) #read the serial data and print it as line
print ("Enter 1 to get LED ON & 0 to get OFF")
while 1: #Do this in loop
#line below changed in python 3
var=input("entered 1 or 0 ?") #get input from user.
if (var == '1'): #if the value is 1
arduino.write(var.encode()) #send 1 .this line changed in python 3.
print ("LED turned ON")
time.sleep(1)
elif (var == '0'): #if the value is 0
arduino.write(var.encode()) #send 0. this line changed in python 3.
print ("LED turned OFF")
time.sleep(1)
Indented error
Showing error:
"Expected an indented block"
Below is the code:
import serial
import time
ArduinoSerial= serial.Serial('COM4', 9600)
print ArduinoSerial.readline()
print ("Enter 1 to turn led on and 0 to turn led off")
while 1:
var = raw_input ()
print ("you entered", var)
if (var == '1'):
ArduinoSerial.write('1')
print ("LED torned ON")
time.sleep(1)
if (var == '0'):
ArduinoSerial.write('0')
print ("LED turned OFF")
well all have got different
well all have got different but i got this please reply fast
import serial
Traceback (most recent call last):
File "<pyshell#0>", line 1, in <module>
import serial
File "C:\Users\Dell\AppData\Local\Programs\Python\Python37-32\lib\site-packages\serial\__init__.py", line 19, in <module>
from serial.serialwin32 import *
File "C:\Users\Dell\AppData\Local\Programs\Python\Python37-32\lib\site-packages\serial\serialwin32.py", line 12, in <module>
from serial import win32
File "C:\Users\Dell\AppData\Local\Programs\Python\Python37-32\lib\site-packages\serial\win32.py", line 196
MAXDWORD = 4294967295L # Variable c_uint
^
Traceback (most recent call
Traceback (most recent call last):
File "<pyshell#0>", line 1, in <module>
import serial
File "C:\Users\mendo\AppData\Local\Programs\Python\Python310\lib\site-packages\serial\__init__.py", line 19, in <module>
from serial.serialwin32 import *
File "C:\Users\mendo\AppData\Local\Programs\Python\Python310\lib\site-packages\serial\serialwin32.py", line 12, in <module>
from serial import win32
File "C:\Users\mendo\AppData\Local\Programs\Python\Python310\lib\site-packages\serial\win32.py", line 196
MAXDWORD = 4294967295L # Variable c_uint
help plz
Hey Thank You For Posting the
Hey Thank You For Posting the Project, It Was Really Cool
It was working great , After clearing all the errors
I'm using Python 3.10.0 Version
Errors i faced:
*I got Syntax error dialogue box ,
*missing parentheses in call to 'print' ... dialogue box"
*and import serial - Error
Here are the changes i made
From the Python Code :
import serial #Serial imported for Serial communication
import time #Required to use delay functions
ArduinoSerial = serial.Serial('COM3', 9600) #Create Serial port object called arduinoSerialData
time.sleep(2) #wait for 2 secounds for the communication to get established
print (ArduinoSerial.readline()) #read the serial data and print it as line
print ("Enter 1 to turn ON LED and 0 to turn OFF LED")
while 1: #Do this forever
var = input() #get input from user
print ("you entered", var) #print the intput for confirmation
if (var == '1'): #if the value is 1
ArduinoSerial.write('1'.encode()) #send 1
print ("LED turned ON")
time.sleep(1)
if (var == '0'): #if the value is 0
ArduinoSerial.write('0'.encode()) #send 0
print ("LED turned OFF")
time.sleep(1)
*there are no changes in Arduino Code
Python 2.7.9 (default, Dec 10 2014, 12:24:55) [MSC v.1500 32 bit (Intel)] on win32
Type "copyright", "credits" or "license()" for more information.
>>> print (1+1)
2
>>> import serial
Traceback (most recent call last):
File "<pyshell#1>", line 1, in <module>
import serial
ImportError: No module named serial
>>>