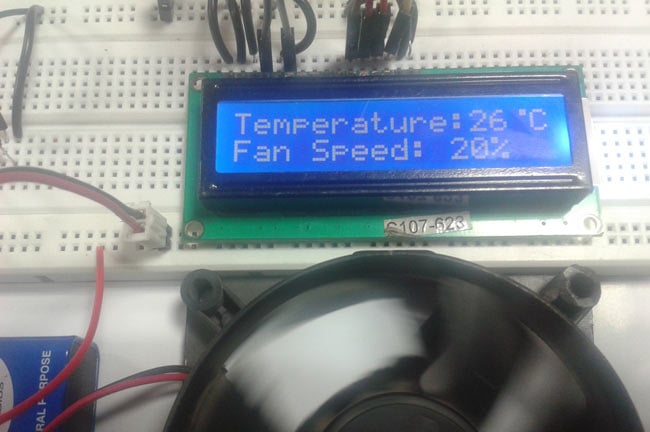
In this arduino based project, we are going to build a temperature-controlled fan using Arduino. With this circuit, we will be able to adjust the fan speed in our home or office according to the room temperature and also show the temperature and fan speed changes on a 16x2 LCD display. To do this we will be using an Arduino UNO Board, LCD, DHT11 sensor Module, and DC fan that is controlled by using PWM. Let's discuss more on this is done so that you can build one on your own. We have also built a project to perform Automatic AC temperature control, you can also check that out if you are intrested.
Components Required
The following are the materials required to perform a temperature-based fan speed control using Arduino. Most of the components should be easily available in your local hardware shop
-
Arduino UNO
-
DHT11 sensor
-
DC Fan
-
2n2222 transistor
-
9 volt battery
-
16x2 LCD
-
1K resistor
-
Connecting wires
Arduino Fan Speed Control using Temperature Sesnor
This project consists of three sections. One senses the temperature by using humidity and temperature sensor namely DHT11. The second section reads the dht11 sensor module’s output and extracts temperature value into a suitable number in Celsius scale and control the fan speed by using PWM. And last part of system shows humidity and temperature on LCD and Fan driver.
Here in this project, we have used a sensor module namely DHT11 that already has discuss our previous project namely “Humidity and Temperature Measurement using Arduino”. Here we have only used this DHT sensor for sensing temperature, and then programmed our arduino according to the requirements.
Working on this project is very simple. We have created PWM at pwm pin of arduino and applied it at base terminal of the transistor. Then transistor creates a voltage according to the PWM input.
Fan speed and PWM values and duty cycles values are showing in given table
Temperature |
Duty Cycle |
PWM Value |
Fan Speed |
Less 26 |
0% |
0 |
Off |
26 |
20 % |
51 |
20% |
27 |
40% |
102 |
40% |
28 |
60% |
153 |
60% |
29 |
80% |
204 |
80% |
Greater 29 |
100% |
255 |
100% |
What is PWM? PWM is a technique by using we can control the voltage or power. To understand it more simply, if you are applying 5 volt for driving a motor then motor will moving with some speed, now if we reduces applied voltage by 2 means we apply 3 volts to motor then motor speed also decreases. This concept is used in the project to control the voltage using PWM. (To understand more about PWM, check this circuit: 1 Watt LED Dimmer)
The main game of PWM is digital pulse with some duty cycle and this duty cycle is responsible for controlling the speed or voltage.
Suppose we have a pule with duty cycle 50% that means it will give half of voltage that we apply.
Formula for duty cycle given below:
Duty Cycle= Ton/T
Where T= total time or Ton+Toff
And Ton= On time of pulse (means 1 )
And Toff= Off time of pulse (means 0)
Arduino Temperature Controlled Fan Circuit Diagram
Connections of this temperature controlled fan circuit is very simple, here a liquid crystal display is used for displaying temperature and Fan speed Status. LCD is directly connected to Arduino in 4-bit mode (Check this tutorial for more details: LCD Interfacing with Arduino Uno). Pins of LCD namely RS, EN, D4, D5, D6 and D7 are connected to Arduino digital pin number 7, 6, 5, 4, 3 and 2. And a DHT11 sensor module is also connected to digital pin 12 of Arduino. Digital pin 9 is used for controlling fan speed through the transistor.
If you are looking for something simple and more cost-effective you can check out the temperature controlled LED using LM35 and Temperature controlled Automatic AC switch projects, both of them are very easy to built and does not need a microcontroller.
Arduino Code for temperature-controlled fan
First, we include the library for LCD and DHT sensor and then define pin for lcd, dht sensor and for fan.
Then initialize all the things in setup loop. And in loop by using dht function reads DHT sensor and then using some dht functions we extract temperature and display these on LCD.
After this we compare the temperature with pre define temperature digit and then generate PWM according to the temperature value.
For generating PWM we have used “analogWrite(pin, PWM value)” fuction in 8 bit. Mean if PWM value is equivalent of analog value. So if we need to generate 20% of duty cycle then we pass 255/5 value as PWM in “analogWrite” Function.
Complete Project Code
#include<dht.h> // Including library for dht
#include<LiquidCrystal.h>
LiquidCrystal lcd(7, 6, 5, 4, 3, 2);
#define dht_dpin 12
dht DHT;
#define pwm 9
byte degree[8] =
{
0b00011,
0b00011,
0b00000,
0b00000,
0b00000,
0b00000,
0b00000,
0b00000
};
void setup()
{
lcd.begin(16, 2);
lcd.createChar(1, degree);
lcd.clear();
lcd.print(" Fan Speed ");
lcd.setCursor(0,1);
lcd.print(" Controlling ");
delay(2000);
analogWrite(pwm, 255);
lcd.clear();
lcd.print("Circuit Digest ");
delay(2000);
}
void loop()
{
DHT.read11(dht_dpin);
int temp=DHT.temperature;
lcd.setCursor(0,0);
lcd.print("Temperature:");
lcd.print(temp); // Printing temperature on LCD
lcd.write(1);
lcd.print("C");
lcd.setCursor(0,1);
if(temp <26 )
{
analogWrite(9,0);
lcd.print("Fan OFF ");
delay(100);
}
else if(temp==26)
{
analogWrite(pwm, 51);
lcd.print("Fan Speed: 20% ");
delay(100);
}
else if(temp==27)
{
analogWrite(pwm, 102);
lcd.print("Fan Speed: 40% ");
delay(100);
}
else if(temp==28)
{
analogWrite(pwm, 153);
lcd.print("Fan Speed: 60% ");
delay(100);
}
else if(temp==29)
{
analogWrite(pwm, 204);
lcd.print("Fan Speed: 80% ");
delay(100);
}
else if(temp>29)
{
analogWrite(pwm, 255);
lcd.print("Fan Speed: 100% ");
delay(100);
}
delay(3000);
}
Comments
something wrong with dht library
arduino ide is showing compilation error due to the dht library. kindly specify the library used in your program code as dht.h is not a valid library
Please install the DHT
Please install the DHT library properly, and make sure that dht.h file is there in the folder. Remember that header file name is case sensitive, if file in the DHT library folder is DHT.h then use DHT.h not dht.h
i already change dht.h to DHT
i already change dht.h to DHT.h but i got another error:
pwn_fan.ino:6:1: error: 'dht' does not name a type
pwn_fan.ino: In function 'void loop()':
pwn_fan.ino:39:6: error: expected unqualified-id before '.' token
pwn_fan.ino:40:15: error: expected primary-expression before '.' token
pwn_fan.ino:87:14: error: expected '}' at end of input
Error compiling.
how can i fix this? can you help me please... thanks in advance
how do you rectified your error ,please tell me,i am doing that
how do you rectified your error ,please tell me,i am doing that project
Code is working fine, please
Code is working fine, please install dht library properly. Download DHT library from here and and install it using this Tutorial: Installing Additional Arduino Libraries
regarding project
bro please give me the code and all detail how to do pleass
arduino code
plz send me rectified code . i m doing this project . i requst you plz send me code which is edited by you
hello, i want to ask. how did
hello, i want to ask. how did you show the data on digital oscilloscope? is it the interface that allow us to manipulate the temperature setting or anything like that? thank you
pls could you modify the
pls could you modify the codes if a heater is added to the system in other to activate if temp is low. thanks
Compling.....
There is no problem in compiling the code. You need to add DHT library in arduono ide library folder.
Thank you
the fan still running in same speed
I've already run your codes without any error... but the fan spinning at the same speed even though in different temperature. could you help me with the problem please... this technique is so crucial to my project. thank you
hello.. i have problem.. in
hello.. i have problem.. in my proteus, does not have DHT11. How to put that sensor in my proteus library. anyone can help me?
Proteus 8 supports the DHT
Proteus 8 supports the DHT library, no need to add. For Proteus 7 you can find the DHT library on Internet and can add.
Cannot Compile
hallo, by the way thank you for your shared
i have a problem when i want to compile the source code, arduino says 'dht' was not name a type
what should i do?
please your advice
thank you anyway
Please read all the above
Please read all the above comments carefully, and install the DHT library properly, and make sure that dht.h file is there in the folder. Remember that header file name is case sensitive, if file in the DHT library folder is DHT.h then use DHT.h not dht.h
Garbage value
Display shows garbage value and fan does not rotate
connection and program written as per given
And compiled and uploaded properly
Use well regulated Power
Use well regulated Power supply for your circuit for better results.
when i compile, it shows me
when i compile, it shows me this error:
'class DHT' has no member named 'read11'
can someone tell me what does it mean?
Add dht11 library for arduino
Add dht11 library for arduino in arduino ide.
then try again.
best of luck
FAN connection
it terms of the diagram connection for the fan, is everything correct does there need a ground added to the fan as mention in the first comment or is everything correct
Circuit is correct, used
Circuit is correct, used Motor is DC motor and its Ground is connected to negative terminal of Battery through Transistor 2N2222
friend this connection is for
friend this connection is for servo ,motor u need to connect fan and wen u connect fan it is nessery to give ground to the ground in auredino if u do dis ur project wil run sucessfully and it will work properly
can you please send me full
can you please send me full code of this project
Please explain the resistor parts
Please can you explain the resistor parts? To where the two resistors are exactly connecting to? It's not very clear for me and I need to do this as my project so please help?
Please check the connections
Please check the connections in Circuit Diagram.
Install the DHT library
Install the DHT library properly, go through the above comments to install DHT library properly.
Pls give the link to install
Pls give the link to install dht library
Instead of using a battery,
Instead of using a battery, can I use a 12V power adapter and have the ground from the fan into ground on my board, and the 12v power running through the 2n2222 transistor and the power from fan into the other end of the 2n2222 transistor?
You need to check the
You need to check the datasheet of all the components for their power and current rating before using 12v Adapter, it may burn some components.
Are there any alternative for
Are there any alternative for 9v Battery ? i find it quite pricey haha
Thanks . Will AA batteries do ?
Although the 9v batteries are
Although the 9v batteries are very cheap, but you can use 4 or 6 AA batteries for simple DC fan.
will the be a difference if I
will the be a difference if I use lm35 as my sensor instead of DHT11 ......I use the exact circuit l
Yes you can, but you need to
Yes you can, but you need to change the Code accordingly, check this Project to interface LM35 to Arduino: Arduino Based Digital Thermometer
Sir , I'm having a problem I
Sir , I'm having a problem I tried to compile the code but there was an error "LiquidCrystal" does not name a type I hope you could help me here thank you
You may have forgotten to
You may have forgotten to include the LiquidCrystal.h library, please check and always use latest version of Arduino IDE.
I got an error stating - " no
I got an error stating - " no matching function for call to 'DHT::DHT()' "
Please help me out.
I did all my connections
I did all my connections correct but not printing and not able to control
Instead of DHT11. Is it
Instead of DHT11. Is it possible to use DHT22. I have read in many articles, DHT22 have more accuracy. So I guess DHT22 will be more useful. If it is possible to use, will be there any change in coding and other electronic components used in the circuit ??
Yes you can use DHT22 with
Yes you can use DHT22 with this circuit. It is recommended to check its datasheet for its pins and power requirement.
fan speed not controlling
i did all the things same as said but my fan moves at constant speed.....it doesn't even switches off when temperature falls below the limit and its speed doesn't increases when temperature increases...
PLEASE HELP..
Please someone send me your
Please someone send me your runnable code?
thanks
Nice work, exactly what I was
Nice work, exactly what I was looking for. But I wonder how anybody got it to work.
First I had a bunch of error on arduino, dht errors. So I used that library for dht11 http://playground.arduino.cc/Main/DHT11Lib
then LiquidCrystal lcd(7, 6, 5, 4, 3, 2); should be LiquidCrystal lcd(2, 3, 4, 5, 6, 7);
and #define dht_dpin 12 ==> #define dht11pin 12
and dht DHT; ==> dht11 DHT11;
and
DHT.read11(dht_dpin);
int temp=DHT.temperature;
should be
DHT11.read(dht11pin);
int temp=DHT11.temperature;
this is it for the code
and then the fan circuit should be grounded to an arduino analogue ground (from the battery minus to arduino GRND, just under +5V).
After all that change it's working thank you
I have facing an error to
I have facing an error to dht11 DHT11; I get an error no matching function for call to 'DHT11::DHT11()'
Most Helpful Comment Here!!
Thanks a lot!
It's only after I read your comment I was able to get my fan to stop spinning at a constant speed for all temp.
Great project,very useful.
So yeah, if your error is that fan is spinning at constant speed connect negative of fan to collector of 2222 ,negative of 9V to emitter and a wire from emitter to GND line of arduino.
DHT libraries
New to this environment and struggling with finding the right combination of library and code statements. The constant appears to be 'dht' does not name a type. The library you refer to does not appear in the library manager - updated, renamed or absorbed into a larger one ?
I was hoping this 'Temperature controlled Fan' would be working on my Solar furnace by now but this code problem has held me up 2 long and frustrating days.....
Any more specific direction on this library/code would be appreciated.
We are doing this project,
We are doing this project,.while running this code we are getting an error like (fatal error:dht.h: No such file or directory.
Compilation terminated
automatic fan speed
there is no interfacing to the fan/motor .. pls help me guys..
please help me in code i got
please help me in code i got error in dht library .so please send the entire correct code as early as possible.
simulation
In proteus it shows these errors: 1.cannot find model file 'DHTXX.MDF'
2.Missing interface model 'DHTXXITF' IN U1 #P
3. PIN 'DATA' is not modelled
4. PIN 'VDD' is not modelled
5. PIN 'GND' is not modelled
Please please help me to solve all these errors and run simulation of this project.
It's working! But I don't
It's working! But I don't understand meaning of those byte degree values in the code. Plz explain me==>
byte degree[8] =
{
0b00011,
0b00011,
0b00000,
0b00000,
0b00000,
0b00000,
0b00000,
0b00000
};
Compilation error with dht header
As Sai vasavi mentioned above, even I'm getting the same error:dht.h: No such file or directory. Compilation terminated. Please send the exact link of the library and executable code asap as it is to be submitted tomorrow.
:D phew!! Kudos... it worked
:D phew!! Kudos... it worked finally. No changes required in the code. Purely depends on installing dht library.
Thanks guys.
Where did you get that
Where did you get that library? Please tell me how you installed that library? This is for my project. Thanks for your help :)
what is the voltage of the DC
what is the voltage of the DC fan used in this project? If the voltage of fan is 5 v , and used battery 9v, so we must use voltage regulator or not?
Can i know how volt of dc fan
Can i know how volt of dc fan, 12v or 5v.
hi. please help me. what does
hi. please help me. what does byte degree coding means? i dont get it
i dont know the meaning of the error ;please help me
Arduino: 1.8.1 (Windows 8.1), Board: "Arduino/Genuino Uno"
C:\Users\Debasish Kalita\Documents\Arduino\random\random.ino:1:50: fatal error: dht.h: No such file or directory
#include<dht.h> // Including library for dht
^
compilation terminated.
exit status 1
Error compiling for board Arduino/Genuino Uno.
This report would have more information with
"Show verbose output during compilation"
option enabled in File -> Preferences.
Download and add dht.g
Download and add dht.g library from Github to ArduinoIDE
if i am using DHT22 sensor
if i am using DHT22 sensor instead of DHT11 then what will be the change in the code....please solve my problem
what is the volatge of the dc
what is the volatge of the dc fan used? 5v or 9v?
Please how to fix this error
Please how to fix this error
#include <Adafruit_Sensor.h>
^
compilation terminated.
exit status 1
Error compiling for board Arduino/Genuino Uno
error
i get this error:
Arduino: 1.8.5 (Mac OS X), Board: "Arduino/Genuino Uno"
In file included from /Users/DanyaalT/Documents/Arduino/libraries/DHT-sensor-library-master/DHT_U.cpp:22:0:
/Users/DanyaalT/Documents/Arduino/libraries/DHT-sensor-library-master/DHT_U.h:25:29: fatal error: Adafruit_Sensor.h: No such file or directory
#include <Adafruit_Sensor.h>
^
compilation terminated.
exit status 1
Error compiling for board Arduino/Genuino Uno.
This report would have more information with
"Show verbose output during compilation"
option enabled in File -> Preferences.
what can i do
You did not add the adafruit
You did not add the adafruit_sensor library to your Arduino IDE correctly.. Try adding it again follow the tutorial to know how it is done
display doesn't show any
display doesn't show any value rather contains only box.please need urgent suggestion.Is the motor is 9v dc
The diagram shown is wrong.
The diagram shown is wrong. To make it work (according to written code), you have to connect LCD pins like this :
(LCD pin) - (Arduino Digital pin)
RS - pin 7
En - pin 6
D4 - pin 5
D5 - pin 4
D6 - pin 3
D7 - pin 2
Now. You need to add “dht library” to work with DHT11 sensor. To do this, follow this steps :
1. Download https://github.com/RobTillaart/Arduino/archive/master.zip
2. Copy the Arduino-master/libraries/DHTstable subfolder of the downloaded file to the libraries subfolder of your sketchbook folder. You can find the location of your sketchbook folder at File > Preferences > Sketchbook location in the Arduino IDE.
This means : after downloading the .zip file, at first extract it. You’ll find a folder named “Arduino-master”. Go inside this folder. Inside this “Arduino-master” folder, there is another subfolder called DHTstable. Copy this ‘DHTstable” folder to your “Sketchbook location” , which default is : This pc/Documents/Arduino. If you can’t find it, open your Arduino IDE, then check : File > Preferences > Sketchbook location .
Now, in documents, go to “Arduino” folder. Then go inside “libraries” subfolder. Then paste “DHTstable” folder there.
There you go, you’ve completed your library setup. Now compile the code, you won’t have any error.
I’ve found this solution on : https://arduino.stackexchange.com/questions/44898/dht-h-library-not-being-imported
One last thing : Connect 2N2222 transistor properly. After constructing the whole circuit, Just “connect a wire between ‘Battery’s Ground point’ to ‘Arduino’s ground point’ in breadboard, which’ll make then a common ground”. Otherwise, your circuit will be open, & the fan won’t work.
I tried to explain step by step, hope this’ll help u guys.
Thank you.
Thank a ton Ishtiaque
Thank a ton Ishtiaque
It should be useful for people here
The fan keeps rotating at a
The fan keeps rotating at a constant speed, done all the things mentioned above pls help
About Code
Hii @Clyde ,, your query is excatly correct, fan keeps rotaing at constant speed. It means Battery supply is directly given to fan.
Means there is no use of code for changing fan speed.
please help us
No it does not mean battery
No it does not mean battery is connected directly fan. As you can see there is a switching transistor in-between the fan and battery. If your fan does not reduce speed it means the transistor is not switching.
Try turning off the fan completly to check if the transistor is working
Please reply, Code run but proteus shows 'can't find DHTXX. MDF
Please anyone reply
I have added dht file in library of arduino and also for proteus, so finally code successfully run in arduino compiler but proteus shows following two error
* cannot find model file 'DHTXX. MDF
* Simulation failed due to net list linker error
i followed the program...but
i followed the program...but why the temperature is not accurate?
48 degree celcius in my room....
Code error
Even though i have installed the DHT library files,there are lots of error while compiling the program please do correct the program and upload it .
can you please send me full
can you please send me full code of this project
The full code of this
The full code of this projects is already given in this page at the bottom of the explanation
Arduino: 1.8.5 (Windows Store
Arduino: 1.8.5 (Windows Store 1.8.10.0) (Windows 10), Board: "Arduino/Genuino Uno"
dht11 DHT11;
^
In function 'void loop()':
sketch_aug17b:34: error: request for member 'read' in '11', which is of non-class type 'int'
DHT11.read(DHT11pin);
^
sketch_aug17b:34: error: 'DHT11pin' was not declared in this scope
DHT11.read(DHT11pin);
^
sketch_aug17b:35: error: request for member 'temperature' in '11', which is of non-class type 'int'
int temp=DHT11.temperature;
^
'dht11' does not name a type
Any suggestions for a solution?
Multiple motors
Dear Saddam, thanks for the great share. It is working perfect. Just wanted to know how many identical items can I connect to this PWM ? Or how can I run multiple PWM circuits for multiple fans ? Thanks
It depends on how long you
It depends on how long you are running the wires and how much current is being drawn. If you powering multiple devices an Op-Amp buffer circuit is recommended
3 fans
I want to control the speed of 3 fans (Not seperately, they will all run same speed regarding the enviroment temperature) They are all 12v 0,30A. Can I connect all 3 to the same PWM circuit ? In other words how many amperes can I run through the circuit ? Thanks
Diagram mistake is that there is no ground in fan motor circuit