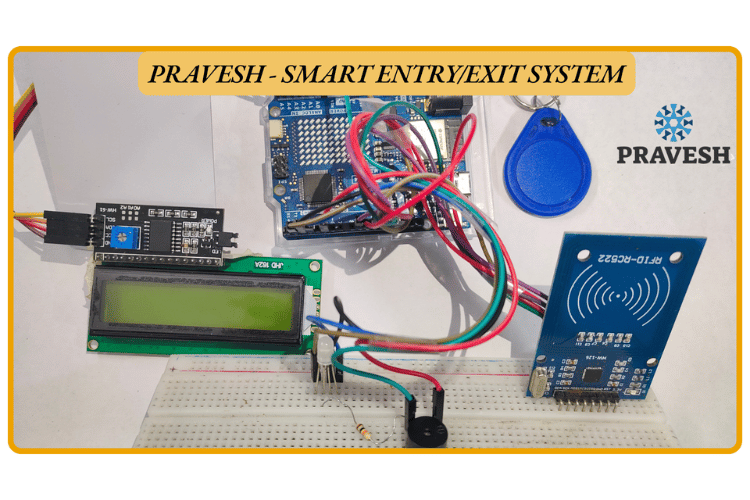
PRAVESH is an RFID-enabled Smart Entry-Exit System designed to replace manual logs with a seamless digital solution. Using RFID cards, students can quickly and accurately log their entry and exit, reducing delays and improving accountability. Built on the Arduino UNO R4 Wi-Fi Board, PRAVESH integrates with campus infrastructure, providing real-time tracking, automated email notifications, and secure database storage. If you are looking to build something simple using RFID, you can also check out how to make an RFID Door Lock System using Arduino.
Impact Statement
PRAVESH is an innovative RFID-enabled Smart Entry-Exit System developed to streamline entry and exit processes, aimed at replacing the current manual logbook method, which is time-consuming and prone to errors. By using RFID cards, students experience quick, hassle-free access without the need for manual entries, reducing delays and promoting accountability. The administration benefits significantly, with PRAVESH enabling paperless operations, efficient record management and real-time reporting Built with the Arduino UNO R4 Wi-Fi microcontroller, which provides internet connectivity, and a custom frontend interface that gets updated in real time with the database, PRAVESH logs each student’s entry and exit with details such as time, date, and personal information. The system also triggers an automated form email for visit purposes. We take pride in PRAVESH’s potential to modernize campus operations, improve security, and greatly enhance convenience for students.
Components Required
- Arduino Uno R4 WiFi Board
- RC522 RFID Card Reader Module 13.56MHz
- RFID 13.56MHz Card and 13.56MHz RFID IC Key Tag
- RGB Led
- 5V Passive Buzzer
- LCD1602 Parallel LCD Display with IIC/I2C interface
- IIC/I2C Serial Interface Adapter Module
- 10K Ohm Resistor
- Jumper Wires
- Bread Board
- Type-C USB Cable
Key Features
Streamlined Access: Fast, error-free RFID-based entry/exit system.
Enhanced Accountability: Automated logging provides accurate, real-time data.
Efficient and Modernized: Integrates with campus systems for seamless operation.
Eco-Friendly: Automated emails reduce paperwork, offering a sustainable solution.
Navigate to this YouTube Video for Full Demonstration of the Project
Circuit Diagram Explanation
Next, let's look at the connections used in this project. The circuit diagram is straightforward.
For Power Input,
I am currently using my laptop which is connected using a usb cable. We can also use a 2S Li-ion battery pack, which provides an average voltage of 7.4V. This is connected to the Vin pin and ground of the Arduino UNO R4 WiFi Board.
RFID Scanner Module Connections
SDA -> Arduino pin 10
SCK -> Arduino pin 13
MOSI -> Arduino pin 11
MISO -> Arduino pin 12
Reset -> Arduino pin 9
Power -> 3.3V on Arduino
Ground -> ground of Arduino
Buzzer:
Shared ground with the RGB LED.
Buzzer pin -> Arduino pin 5
RGB LED:
Red -> Arduino pin 3
Blue -> Arduino pin 2
Green -> Arduino pin 1
Ground with resistor in between.
LCD Display (I2C Interface)
SDA -> Arduino pin A4
SCL -> Arduino pin A5
VCC -> 5V output from Arduino
Ground -> Common ground with Arduino
Arduino Code Explanation
For Better Explanation let's split the code into three parts: setup, loop, and supporting functions.
Setup and Initialization:
This segment initializes the RFID reader, LEDs, buzzer, LCD, and connects to WiFi. It also displays the initial connection status on the LCD.
#include <WiFiS3.h>
#include <ArduinoHttpClient.h>
#include <SPI.h>
#include <MFRC522.h>
#include <Wire.h>
#include <LiquidCrystal_I2C.h>// Define constants
#define RED_LED_PIN 1
#define GREEN_LED_PIN 2
#define BLUE_LED_PIN 3
#define BUZZER_PIN 5 // Define the buzzer pinString URL = "http://192.168.104.160/rfidattendance/test_data.php";
const char* ssid = "kk"; // Your WiFi network name
const char* password = "12345678"; // Your WiFi password#define RST_PIN 9
#define SS_PIN 10
MFRC522 rfid(SS_PIN, RST_PIN); // Create MFRC522 instance
WiFiClient wifiClient; // Create WiFiClient instance
HttpClient client = HttpClient(wifiClient, "192.168.104.160", 80); // Use IP and port for the server
// Initialize LCD with I2C address (0x27 is common, but check your LCD's documentation)
LiquidCrystal_I2C lcd(0x27, 16, 2); // 16 columns, 2 rows
void setup() {
Serial.begin(115200);
SPI.begin();
rfid.PCD_Init(); // Initialize MFRC522
Serial.println("RFID Reader initialized.");
// Initialize LED pins
pinMode(RED_LED_PIN, OUTPUT);
pinMode(GREEN_LED_PIN, OUTPUT);
pinMode(BLUE_LED_PIN, OUTPUT);
pinMode(BUZZER_PIN, OUTPUT); // Initialize buzzer pin
// Initialize LEDs and buzzer to off
digitalWrite(RED_LED_PIN, HIGH);
digitalWrite(GREEN_LED_PIN, LOW);
digitalWrite(BLUE_LED_PIN, LOW);
digitalWrite(BUZZER_PIN, LOW);
// Initialize LCD
lcd.init();
lcd.backlight(); // Turn on LCD backlight
lcd.clear();
// Display initial message
lcd.setCursor(0, 0);
lcd.print("WiFi Connecting..");
// Connect to WiFi
WiFi.begin(ssid, password);
while (WiFi.status() != WL_CONNECTED) {
delay(1000);
}
Serial.println("nConnected to WiFi!");
Serial.print("IP Address: ");
Serial.println(WiFi.localIP());
lcd.clear();
lcd.setCursor(0, 0);
lcd.print("WiFi Connected");
delay(2000); // Wait before starting the loop
}
Wi-Fi and RFID Status Check:
This part checks Wi-Fi connectivity and prompts the user to scan their RFID card. The LCD displays appropriate messages, and LEDs indicate WiFi status.
void loop() {
if (WiFi.status() != WL_CONNECTED) {
// If not connected to Wi-Fi, display "WiFi not connected"
lcd.clear();
lcd.setCursor(0, 0);
lcd.print("WiFi not connected");
digitalWrite(RED_LED_PIN, HIGH); // Red LED on
digitalWrite(GREEN_LED_PIN, LOW);
digitalWrite(BLUE_LED_PIN, LOW);
return;
} else {
// If connected to Wi-Fi, display "Scan your card"
lcd.clear();
lcd.setCursor(0, 0);
lcd.print("Scan your card");
digitalWrite(RED_LED_PIN, LOW);
digitalWrite(GREEN_LED_PIN, HIGH); // Green LED on
digitalWrite(BLUE_LED_PIN, LOW);
}
RFID Scanning and UID Transmission:
This segment reads the RFID UID, displays a "Wait" message on the LCD, and sends the UID to the server. It handles server response and updates the LCD and LEDs accordingly.
// Look for new RFID tags
if (!rfid.PICC_IsNewCardPresent() || !rfid.PICC_ReadCardSerial()) {
return;
}// Read UID
String uid = "";
for (byte i = 0; i < rfid.uid.size; i++) {
if (rfid.uid.uidByte[i] < 0x10) {
uid += "0"; // Add leading zero if needed
}
uid += String(rfid.uid.uidByte[i], HEX);
}// Print the UID
Serial.print("UID tag: ");
Serial.println(uid);
// Display "Wait" on LCD and turn on blue LED and buzzer when tag is read
lcd.clear();
lcd.setCursor(0, 0);
lcd.print("Wait");
digitalWrite(BLUE_LED_PIN, HIGH); // Blue LED on
digitalWrite(BUZZER_PIN, HIGH); // Buzzer on
// Send UID to server
String postData = "uid=" + uid;
client.beginRequest();
client.post("/rfidattendance/test_data.php");
client.sendHeader("Content-Type", "application/x-www-form-urlencoded");
client.sendHeader("Content-Length", postData.length());
client.beginBody();
client.print(postData);
client.endRequest();
int statusCode = client.responseStatusCode();
String response = client.responseBody();
Serial.print("Status code: ");
Serial.println(statusCode);
Serial.print("Response: ");
Serial.println(response);
Serial.println("------------------------------------------");
// After sending UID, turn off blue LED, buzzer, and display "Thank you"
digitalWrite(BLUE_LED_PIN, LOW);
digitalWrite(BUZZER_PIN, LOW);
digitalWrite(GREEN_LED_PIN, HIGH); // Green LED back on
lcd.clear();
lcd.setCursor(0, 0);
lcd.print("Thank you");
delay(2000); // Show "Thank you" for 2 seconds
// Display "Scan your card" again
lcd.clear();
lcd.setCursor(0, 0);
lcd.print("Scan your card");
// Halt PICC
rfid.PICC_HaltA();
// Stop encryption on PCD
rfid.PCD_StopCrypto1();
delay(5000); // Short delay to prevent rapid switching
}
Working Demonstration
Below, you can see the working images of the project
Not Connected to Wi-Fi (LED is Red), the screen shows “WiFi Connecting”.
Connect to Wi-Fi, screen displays "Wi-Fi Connected".
Connected to Wi-Fi (LED Turning Green signifying the system is ready to be used), also screen displays “Scan your card”.
RFID Scanned and sending UID to the server (Screen Showing “Wait”, signifying wait time before tapping the next rfid card). On successful scanning the screen shows "Thank You" for confirmation.
RFID Scanned Successfully, display shows "Scan Your Card" signifying the system is ready to be used again.
Working of the RFID Wi-Fi Access Control System
This project functions as an RFID-based access control system that uses an Arduino Uno R4 Wi-Fi to connect to a server, send card UID, and provide real-time feedback using LEDs, display and a buzzer. The system operates as follows:
Setup and Initialization:
Upon powering on, the Arduino Uno R4 Wi-Fi connects to the configured Wi-Fi network. If connected successfully, the green LED turns on and the display shows “Scan your card” to indicate network readiness. Meanwhile, the RFID reader (MFRC522) and other output components are also initialized.
Wi-Fi Status Check:
In each loop iteration, the Arduino Uno R4 Wi-Fi checks its Wi-Fi connection status. If disconnected, the red LED lights up to signal this, also the screen shows “WiFi connecting..”. Once Wi-Fi reconnects, the red LED turns off and the green LED turns back on, the screen now shows “WiFi connected”.
RFID Tag Detection:
The RFID reader continuously scans for new RFID tags. When a tag is detected, the reader retrieves the UID, a unique identifier for each tag. This UID is converted to a string for easy transmission.
Visual and Audio Feedback:
Upon detecting an RFID tag, the system lights up the LED and activates the buzzer, also the screen changes to “wait” indicating wait time between two rfid scans. This provides an immediate indication to the user that their tag has been scanned.
Data Transmission to Server:
The UID is sent to the server using an HTTP POST request. The server URL is predefined, and the UID data is posted to the endpoint /rfidattendancet/test_data.php. The server response status and body are printed to the Serial Monitor for debugging and confirmation.
Completion and Reset:
After the UID is successfully sent, the system changes the “wait” display to “scan your card”, readying the system for the next scan. The RFID reader halts communication with the card to conserve power until the next tag is detected.
Email functionality:
During the Data Transmission to Server step , the UID is also matched to the email of respective person and an form is sent to the registered email id of the person with the reason for visit and return time estimate thing , after the user fills that and submits the form , it automatically updates at the database .
The form which will open from the link in the mail sent is:
Delay and Repeat:
A short delay prevents the system from reading the same card multiple times too quickly. The system then loops back to check for Wi-Fi status and new RFID tags, maintaining continuous operation.
Custom Frontend Development
We have crafted a sleek and intuitive frontend using HTML and CSS, ensuring a visually appealing website that not only meets the aesthetic needs but also serves the functional requirements for managing the system. The frontend is designed to provide ease of access and streamline the administration process.
To store and manage data, we integrated a MySQL database that holds user and log information securely. The backend communication with the MySQL database is handled efficiently using PHP, ensuring smooth handling of POST requests from the Arduino UNO R4 Wi-Fi board and seamless data retrieval.
The frontend consists of the following key pages:
Login Page
This page serves as the authentication gateway, ensuring that only authorized personnel can access sensitive data. Users can log in using their admin email ID and password, providing a secure and controlled access point to the system.
Users Page
This page displays a list of all users currently registered in the system. The user details shown include:
User ID
UID
Name
Email ID
The Users Page allows administrators to quickly view the status of all registered users.
Manage Users Page
This page enables administrators to perform key user management tasks:
Adding New Users: Easily register new users with necessary details.
Updating Existing User Details: Modify user information, including:
ID
Name
Gender
S. No.
Email ID
Removing Users: Administrators can remove users from the system as needed.
Users Log Page
This page presents a comprehensive log of student entry and exit details. The information displayed includes:
User ID
Card UID
Name
Gender
Date and Time of Entry/Exit
Reason for Visit
Estimated Time of Return
The Users Log Page offers administrators an easy-to-navigate interface to monitor all student movements in real-time.
Export to Excel Page
The Export to Excel page provides the ability to download Users Log Page data in an Excel (.xlsx) format. Administrators can filter logs based on date and time, allowing for custom export of specific periods for further analysis or record-keeping.
With this frontend setup, we have ensured a well-structured, user-friendly, and secure interface for managing and accessing data, making the administration process smoother and more efficient.
Click on the GitHub Image to view or download code