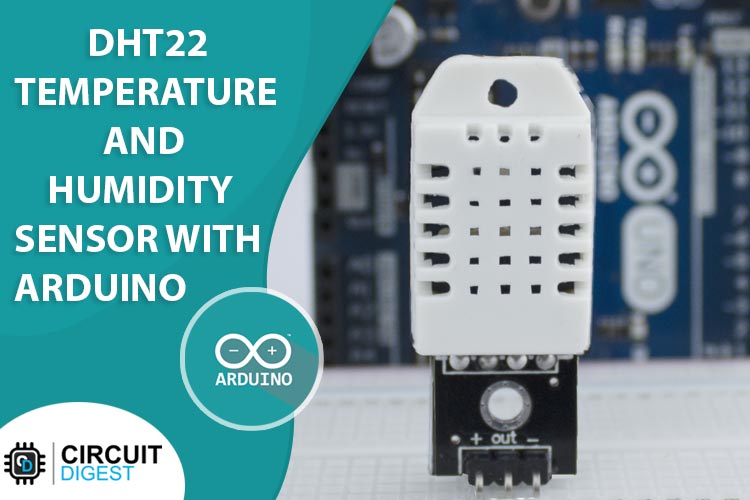
The DHT22 temperature and humidity sensor is a versatile and cost-effective sensor used to measure ambient temperature and humidity for a wide range of applications. It is based on a digital signal output and can provide high-accuracy measurements with a resolution of 0.1 degrees Celsius for temperature and 0.1% for humidity. The sensor uses a capacitive humidity sensing element and a thermistor to measure the humidity and temperature respectively. The DHT22 sensor is also relatively low power and can operate on a voltage range of 3.3V to 5V, making it suitable for battery-powered projects. Additionally, the sensor comes with long-term stability and high reliability, this makes it a perfect choice for various applications such as HVAC, weather stations, and indoor air quality monitoring systems. So, in this tutorial we have decided to interface DHT22 with Arduino UNO and in the process we will let you know all the details, so without further ado let's get right into it.
DHT11 Sensor is very much similar to DHT22 and we have used DHT11 sensor with many other microcontrollers to build applications like:
- Interfacing DHT11 Temperature and Humidity Sensor with Raspberry Pi
- MATLAB Data Logging, Analysis and Visualization: Plotting DHT11 Sensor readings on MATLAB
- Automatic AC Temperature Controller using Arduino, DHT11 and IR Blaster
- IoT Weather Station using NodeMCU: Monitoring Humidity, Temperature and Pressure over Internet
DHT22 Temperature and Humidity Sensor Module
The DHT22 module has a total of 3 pins. And the DHT22 bare bone sensor has 4 pins. If we consider the module among three pins two of which are power pins, and one is a data pin. If we are looking at the 4-pin sensor the extra pin is a NC pin, and it has no functions. The pin diagram of both the module and the sensor is shown below.
DHT22 Module Pinout
The diagram below shows the pinout of the DHT22 module.
DATA Data Pin for 1-Wire Communication
GND Ground Pin of the Module, Connects to the Ground pin of the Arduino.
VCC Supply Pin of the Module.
Not Used In this sensor, this pin is not used.
Parts Marking of the DHT22 Sensor Module
Aside from the sensor, the DHT22 module comprises only two components on the PCB. a pullup resistor and a decoupling capacitor, the parts marking of the DHT22 module is shown below.
Circuit Diagram of DHT22 Module
The complete schematic diagram of the DHT22 Temperature and Humidity Sensor Module is shown below:
The DHT22 module's schematic diagram is shown above. As previously stated, the board has only a few members. The VCC and GND pins are linked directly to the DHT22, and a pullup resistor is attached to the DATA pin. Tantalum and multilayer capacitors offer enough filtering. As a power indication, and in some PCBs, you can find an indicator LED, but for most of the board the LED is not present.
Commonly Asked Questions about DHT22 Sensor Module
Q. What is DHT22 in a nutshell?
The DHT22 is the more expensive version of the DHT11 sensor which obviously has better specifications. Its temperature measuring range is from -40 to +125 degrees Celsius with +-0.5 degrees accuracy, while the DHT11 temperature range is from 0 to 50 degrees Celsius with +-2 degrees accuracy.
Q. Is DHT22 analog or digital?
The DHT-22 (also named as AM2302) is a digital-output relative humidity and temperature sensor.
Q. Is the DHT22 waterproof?
No. It’s not waterproof.
Q. What’s the sampling rate of a DHT11 sensor?
DHT22 has a sampling rate of 1Hz.
Q. What protocol does DHT22 use?
The DHT22 sensor uses a proprietary single-bus communication protocol that can send and receive data with calculated timing pulses.
How Does the DHT22 Works?
If you are using an original DHT22 sensor then it will have a NTC thermistor and the sensor module inside it, but most of the sensors that you can find in the market are mostly non genuine parts and inside that you will find a small sensor that you can see in the image below.
The humidity sensing component consists of a moisture-holding substrate sandwiched in between two electrodes. When the substrate absorbs water content, the resistance between the two electrodes decreases. The change in resistance between the two electrodes is proportional to the relative humidity. Higher relative humidity decreases the resistance between the electrodes, while lower relative humidity increases the resistance between the electrodes. This change in resistance is measured with the onboard MCU’s ADC and the relative humidity is calculated.
Each DHT22 element is strictly calibrated in the laboratory which is extremely accurate in humidity calibration. The calibration coefficients are stored as programs in the OTP memory, which are used by the sensor’s internal signal detecting process.
DHT22 Single-bus Communication Protocol
A single bus communication protocol is used to communicate with the DHT22 and microcontroller. A sample data takes about 4ms to run down. This data consists of decimal and integer parts. The total data is 40 bit long and its is MSB format. The Data format is as follows: 8bit integral RH data + 8bit decimal RH data + 8bit integral T data + 8bit decimal T data + 8bit checksum. If the data transmission is right, the check-sum should be the last 8bit of "8bit integral RH data + 8bit decimal RH data + 8bit integral T data + 8bit decimal T data".
When the MCU sends the start signal DHT changes from low power mode to running mode, and dumps all the 40-bit data to the microcontroller, the microcontroller reads the data and calculates the temperature and humidity from the binary data.
The image above shows how the data communication works with the microcontroller and the DHT22.
Circuit Diagram for Interfacing DHT22 Sensor with Arduino
Now that we have completely understood how a DHT22 Sensor works, we can connect all the required wires to Arduino and write the code to get all the data out from the sensor. The following image shows the circuit diagram for interfacing the DHT22 sensor module with Arduino.
Connections are simple and only require three wires. Connect the VCC and GND of the module to the 5V and GND pins of the Arduino. Then connect the DATA pin to Arduino’s digital pin 2. We communicate with DHT22 through this pin.
Arduino DHT22 Code for Interfacing the Sensor Module
Now let’s look at the code for interfacing the DHT22 sensor. For that first install the Adafruit’s DHT sensor library and Adafruit Unified Sensor Driver through the library manager. Then create a blank sketch and paste the code at the end of this article into it.
#include <Wire.h> #include "DHT.h" #include <Adafruit_GFX.h> #include <Adafruit_SSD1306.h>
In the start, we have included all the necessary libraries and defined the sensor type as DHT22 and the sensor pin as digital pin 2. And then created an instance for the DHT library.
#define DHTTYPE DHT22 Adafruit_SSD1306 display = Adafruit_SSD1306(128, 64, &Wire, -1); unsigned long delayTime; uint8_t DHTPin = 2; DHT dht(DHTPin, DHTTYPE); float Temperature; float Humidity; float Temp_Fahrenheit;
Next, we have our setup() function, in the setup function we initialize the serial for debugging, we initialize the DHT and we also initialize the display. We also set the text size and make the display color white.
void setup() { Serial.begin(115200); pinMode(DHTPin, INPUT); dht.begin(); display.begin(SSD1306_SWITCHCAPVCC, 0x3C); display.display(); delay(100); display.clearDisplay(); display.display(); display.setTextSize(1.75); display.setTextColor(WHITE); }
Next, we have our loop function, in the loop function we get the humidity, we get the temperature and we get the temperature in Celsius and Fahrenheit.
void loop(){ Humidity = dht.readHumidity(); // Read temperature as Celsius (the default) Temperature = dht.readTemperature(); // Read temperature as Fahrenheit (isFahrenheit = true) Temp_Fahrenheit = dht.readTemperature(true); // Check if any reads failed and exit early (to try again). if (isnan(Humidity) || isnan(Temperature) || isnan(Temp_Fahrenheit)) { Serial.println(F("Failed to read from DHT sensor!")); return; } }
Next, we print the data on the serial monitor and OLED
Serial.print(F("Humidity: ")); Serial.print(Humidity); Serial.print(F("% Temperature: ")); Serial.print(Temperature); Serial.print(F("°C ")); Serial.print(Temp_Fahrenheit); Serial.println(F("°F ")); display.setCursor(0, 0); display.clearDisplay(); display.setTextSize(1); display.setCursor(0, 0); display.print("Temperature: "); display.setTextSize(2); display.setCursor(0, 10); display.print(Temperature); display.print(" "); display.setTextSize(1); display.cp437(true); display.write(167); display.setTextSize(2); display.print("C"); display.setTextSize(1); display.setCursor(0, 35); display.print("Humidity: "); display.setTextSize(2); display.setCursor(0, 45); display.print(Humidity); display.print(" %"); display.display(); delay(1000); }
Projects using DHT22 Sensor and Arduino
In our previous attempts, there were some interesting projects done with the DHT22 sensor. If you want to know more about those topics, links are given below.
In this project, we are going to build a temperature-controlled fan using Arduino. With this circuit, we will be able to adjust the fan speed in our home or office according to the room temperature and also show the temperature and fan speed changes on a 16x2 LCD display.
If you are a python developer and looking for a way to use python with ESP8266 or ESP32 then this project is for you, because in this project we have not only used python with ESP but we have also used a DHT22 as an example to send data through the mess.
In this project, we are going to make a small Automatic Temperature Control Circuit that could minimize the electricity charges by varying the AC temperature automatically based on the Room's temperature.
Complete Project Code
#include <Wire.h>
#include "DHT.h"
#include <Adafruit_GFX.h>
#include <Adafruit_SSD1306.h>
void setup() {
Serial.begin(115200);
pinMode(DHTPin, INPUT);
dht.begin();
display.begin(SSD1306_SWITCHCAPVCC, 0x3C);
display.display();
delay(100);
display.clearDisplay();
display.display();
display.setTextSize(1.75);
display.setTextColor(WHITE);
}
void loop(){
Humidity = dht.readHumidity();
// Read temperature as Celsius (the default)
Temperature = dht.readTemperature();
// Read temperature as Fahrenheit (isFahrenheit = true)
Temp_Fahrenheit = dht.readTemperature(true);
// Check if any reads failed and exit early (to try again).
if (isnan(Humidity) || isnan(Temperature) || isnan(Temp_Fahrenheit)) {
Serial.println(F("Failed to read from DHT sensor!"));
return;
}
Serial.print(F("Humidity: "));
Serial.print(Humidity);
Serial.print(F("% Temperature: "));
Serial.print(Temperature);
Serial.print(F("°C "));
Serial.print(Temp_Fahrenheit);
Serial.println(F("°F "));
display.setCursor(0, 0);
display.clearDisplay();
display.setTextSize(1);
display.setCursor(0, 0);
display.print("Temperature: ");
display.setTextSize(2);
display.setCursor(0, 10);
display.print(Temperature);
display.print(" ");
display.setTextSize(1);
display.cp437(true);
display.write(167);
display.setTextSize(2);
display.print("C");
display.setTextSize(1);
display.setCursor(0, 35);
display.print("Humidity: ");
display.setTextSize(2);
display.setCursor(0, 45);
display.print(Humidity);
display.print(" %");
display.display();
delay(1000);
}