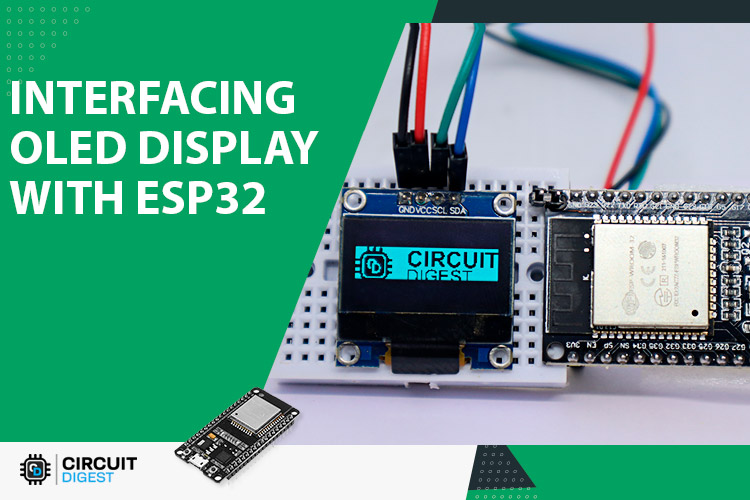
As we know that visual aids like displays are inevitable in any Human Machine Interfacing. They are in every electronics nowadays, from simple toys to smartphones, computers, and even critical lifesaving medical devices. The displays will make it easier for the device to convey all of the information it has to provide to the user. If you look at displays, there are multiple types of displays available in the market. From basic seven-segment displays to advanced AMOLED displays. In this tutorial, we are going to look into such a type of display, which is known as OLED or Organic Light Emitting Diode display.
OLED Display Module Pinout
The OLED module we are going to use leverages I2C for communication with the microcontroller. You can also find similar displays with either I2C or SPI interface or even with both in the same PCB. The module we are using has 4 pins in total. And the pinout of a OLED Display Module is shown below-
GND Ground connection for the module. Connect to the GND pin of the ESP32 Devkit.
VCC Provides power for the module. Connect to the 3.3V pin of the ESP32 Devkit.
SCL Serial Clock pin. Used for providing clock pulse for I2C Communication.
SDA Serial Data pin. Used for transferring Data through I2C communication.
Keep in mind that some display module in the market comes with the pin positions swapped. So always check the pin labeling on the silkscreen before making the connections. Some modules come with a 3.3V regulator on boards and some don’t. If your module doesn’t have this regulator onboard, use the module only with 3.3V devices. Otherwise, you may end up damaging them. If it has the regulator with the marking 662K you can use it with 5V devices.
OLED Module Parts
The below image shows the components on the OLED display module PCB.
The XC6206P332 Voltage regulator steps down the input voltage to 3.3V. The inclusion of this voltage regulator allows us to interface the OLED module to even 5.5V microcontrollers or circuits. We can also set the OLED module I2C address by changing the position of the address select resistor. IIC default address is 0x78 (0x3C in 7 -bit) and can be changed to 0x7A (0x3D in 7 -bit).
Circuit Diagram for 0.96” OLED Module
The Schematic diagram for the OLED module is given below. The circuit consists of bare minimum components.
As you can see the board contains very few components. Most of them are complimentary resistors and capacitors which are necessary for the SSD1306 display controller in the OLED panel. Other components include the 3.3V voltage regulator (XC6206P332), pullup resistors for the I2C line, and the address select resistor.
Commonly Asked Questions when using OLED Display with ESP32
Q. What is an OLED display module?
OLED is an Organic Light-Emitting Diode. OLED Display is a self-light-emitting technology composed of a thin, multi-layered organic film placed between an anode and cathode. In contrast to LCD technology, OLED does not require a backlight.
Q. What is the display controller used in these OLED modules?
SSD1306 and SH1106 are the most commonly used display controllers in the small OLED modules.
Q. What type of protocol do OLED modules use?
The OLED modules support I2C or SPI protocols.
Q. What are the commonly available OLED display sizes and resolutions?
Even though OLED displays are available in different sizes and resolutions, the most commonly used ones are 0.96” and 1.3” modules with 128x64 Dpi resolution. Modules with a resolution of 128x32 are also common in the DIY community.
ESP32 OLED Module Interfacing Connection Diagram
The following image shows how to connect the OLED module with ESP32 Devkit.
Since we are using the OLED module with the I2C protocol, we only need 4 wires for the connections. The VCC and GND pins are connected to the 3.3V and GND pins of the ESP32 Devkit respectively. The SCL pin is connected to the GPIO22 and the SDA pin to the GIPO21.
Arduino Code for Interfacing OLED Display with ESP32
As the connections are made, let’s look at the coding part. To make the interfacing easier we need to install two Arduino libraries Adafruit’s SSD1306 library and Adafruit GFX Library. To install them navigate to the Sketch > Include Library > Manage Libraries. Wait for the Library Manager to download the libraries index and update the list of installed libraries. Then search for the specific library and install it.
Testing the OLED Display
To test and make sure the display is working fine, first make the connections as per the connection diagram provided. Once it’s done, open the example program by navigating to File > Examples > Adafruit SSD1306 >ssd1306_128x64_i2c. Once the example file is opened change the address of the OLED to 0x3C. Then compile and upload the program to the ESP32. The result will be as below-
Arduino Code with Basic Text and Graphics Functions
To learn the basic functions, copy and paste the following code to the Arduino IDE and upload it to the ESP32. The result is as below-
#include <Wire.h> #include <Adafruit_GFX.h> #include <Adafruit_SSD1306.h> #define SCREEN_WIDTH 128 // OLED display width, in pixels #define SCREEN_HEIGHT 64 // OLED display height, in pixels // Declaration for an SSD1306 display connected to I2C (SDA, SCL pins) #define OLED_RESET -1 // Reset pin # (or -1 if sharing Arduino resetpin) Adafruit_SSD1306 display(SCREEN_WIDTH, SCREEN_HEIGHT, &Wire, OLED_RESET); void setup() { Serial.begin(115200); // SSD1306_SWITCHCAPVCC = generate display voltage from 3.3V internally if (!display.begin(SSD1306_SWITCHCAPVCC, 0x3C)) { Serial.println(F("SSD1306 allocation failed")); for (;;); // Don't proceed, loop forever } // Clear the buffer. display.clearDisplay(); // Display Text display.setTextSize(1); display.setTextColor(WHITE); display.setCursor(0, 28); display.println("Hello world!"); display.display(); delay(2000); display.clearDisplay(); // Display Inverted Text display.setTextColor(BLACK, WHITE); // 'inverted' text display.setCursor(0, 28); display.println("Hello world!"); display.display(); delay(2000); display.clearDisplay(); // Changing Font Size display.setTextColor(WHITE); display.setCursor(0, 24); display.setTextSize(2); display.println("Hello!"); display.display(); delay(2000); display.clearDisplay(); // Display Numbers display.setTextSize(1); display.setCursor(0, 28); display.println(123456789); display.display(); delay(2000); display.clearDisplay(); // Specifying Base For Numbers display.setCursor(0, 28); display.print("0x"); display.print(0xFF, HEX); display.print("(HEX) = "); display.print(0xFF, DEC); display.println("(DEC)"); display.display(); delay(2000); display.clearDisplay(); // Display ASCII Characters display.setCursor(0, 24); display.setTextSize(2); display.write(1); display.display(); delay(2000); display.clearDisplay(); // Scroll full screen display.setCursor(0, 0); display.setTextSize(1); display.println("Full"); display.println("screen"); display.println("scrolling!"); display.display(); display.startscrollright(0x00, 0x07); delay(4500); display.stopscroll(); delay(1000); display.startscrollleft(0x00, 0x07); delay(4500); display.stopscroll(); delay(1000); display.startscrolldiagright(0x00, 0x07); delay(4500); display.startscrolldiagleft(0x00, 0x07); delay(4500); display.stopscroll(); display.clearDisplay(); //draw rectangle display.setTextSize(1); display.setTextColor(WHITE); display.setCursor(0, 0); display.println("Rectangle"); display.drawRect(0, 15, 60, 40, WHITE); display.display(); delay(2000); display.clearDisplay(); //draw filled rectangle display.setCursor(0, 0); display.println("Filled Rectangle"); display.fillRect(0, 15, 60, 40, WHITE); display.display(); delay(2000); display.clearDisplay(); //draw rectangle with rounded corners display.setCursor(0, 0); display.println("Round Rectangle"); display.drawRoundRect(0, 15, 60, 40, 8, WHITE); display.display(); delay(2000); display.clearDisplay(); //draw filled rectangle with rounded corners display.setCursor(0, 0); display.println("Filled Round Rectangle"); display.fillRoundRect(0, 15, 60, 40, 8, WHITE); display.display(); delay(2000); display.clearDisplay(); //draw circle display.setCursor(0, 0); display.println("Circle"); display.drawCircle(20, 35, 20, WHITE); display.display(); delay(2000); display.clearDisplay(); //draw filled circle display.setCursor(0, 0); display.println("Filled Circle"); display.fillCircle(20, 35, 20, WHITE); display.display(); delay(2000); display.clearDisplay(); //draw triangle display.setCursor(0, 0); display.println("Triangle"); display.drawTriangle(30, 15, 0, 60, 60, 60, WHITE); display.display(); delay(2000); display.clearDisplay(); //draw filled triangle display.setCursor(0, 0); display.println("Filled Triangle"); display.fillTriangle(30, 15, 0, 60, 60, 60, WHITE); display.display(); delay(2000); display.clearDisplay(); } void loop() {}
Now let’s look at the code. As usual at the starting, we need to include all the necessary libraries and we must declare any global variable.
#include <Wire.h> #include <Adafruit_GFX.h> #include <Adafruit_SSD1306.h>
In the above section, we have included the wire.h library because we are going to use the I2C protocol and also included the Adafruit libraries necessary for the communication.
#define SCREEN_WIDTH 128 // OLED display width, in pixels #define SCREEN_HEIGHT 64 // OLED display height, in pixels #define OLED_RESET 4 // Reset pin # (or -1 if sharing Arduino reset pin) #define SCREEN_ADDRESS 0x3C ///< See datasheet for Address; 0x3D for 128x64, 0x3C for 128x32 Adafruit_SSD1306 display(SCREEN_WIDTH, SCREEN_HEIGHT, &Wire, OLED_RESET);
Here we have defined the screen resolution as SCREEN_WIDTH and SCREEN_HIGHT. And since we don’t use the reset pin, we declared its value to be -1 so that the library won’t miss behaving. Next, we have changed the OLED address to 0x3C. Change this according to your display module. If you are not sure you can use the I2C scanner example to identify the module’s I2C address. Then we created an instance called to display to use all along with the program. We will use this instance to access all the Adafruit GFX library features.
void setup() { Serial.begin(115200); // SSD1306_SWITCHCAPVCC = generate display voltage from 3.3V internally if (!display.begin(SSD1306_SWITCHCAPVCC, 0x3C)) { Serial.println(F("SSD1306 allocation failed")); for (;;); // Don't proceed, loop forever } // Clear the buffer. display.clearDisplay();
At the start of the setup function, we have initialized the serial communication and initialized the Adafruit GFX library too. Then we cleared the display buffer with the help clearDisplay function. This will clear any data that’s on the display if the display is not reset previously. We will be using this function all along the code to clear anything on the display. Now let’s look at each section and what’s their functions.
Displaying Text
// Display Text display.setTextSize(1); display.setTextColor(WHITE); display.setCursor(0, 28); display.println("Hello world!"); display.display(); delay(2000); display.clearDisplay();
The above section will print the word Hello world! to the OLED display. Before printing the text, we have called a few other functions. The display.setTextSize(size) will set the text size, the display.setTextColor(colour) will set the text colour to white, and finally the display.setCursor(x,y) sets the cursor to the position that corresponds to the x, y coordinates we have provided. Once the print function is called we will call the display.display() that will tell the display controller to display the buffer content on the screen. The display won’t show what we have transferred to the buffer unless this function is called.
Displaying Inverted Text
// Display Inverted Text display.setTextColor(BLACK, WHITE); // 'inverted' text display.setCursor(0, 28); display.println("Hello world!"); display.display(); delay(2000); display.clearDisplay();
In this section, we have used the setTextcolor function to declare the text color as well as the text background color. This will print the text in black color with a white text background.
Increase Text Size
// Changing Font Size display.setTextColor(WHITE); display.setCursor(0, 24); display.setTextSize(2); display.println("Hello!"); display.display(); delay(2000); display.clearDisplay();
This section demonstrates how to change the text size. You can see that the text size doubled when we increased the value we passed to the setTextSize function.
Displaying Numbers
// Display Numbers display.setTextSize(1); display.setCursor(0, 28); display.println(123456789); display.display(); delay(2000); display.clearDisplay();
This is also similar to displaying a text. You can simply use the print or println function to print the value to the display.
Displaying a Base Encoded Number
// Specifying Base For Numbers display.setCursor(0, 28); display.print("0x"); display.print(0xFF, HEX); display.print("(HEX) = "); display.print(0xFF, DEC); display.println("(DEC)"); display.display(); delay(2000); display.clearDisplay();
This will help you print any numbers as it is. For example, if you want to print a Hex value, you can do that by simply mentioning the base within the print or println function. The format is as follows print(value, base).
Displaying Glyph or ASCII Characters
// Display ASCII Characters display.setCursor(0, 24); display.setTextSize(2); display.write(1); display.display(); delay(2000); display.clearDisplay();
The font files not only contain the alphanumeric characters but also small symbols or graphical characters too. To display them we can use the write function. You can display any ASCII charter in your font file by just writing the ASCII code for that specific character. In the example, we have printed the smiley face which has the ASCII code 0x01. Below is the ASCII character table for the default font used with the Adafruit GFX library. You can use your own font files and they may contain different Glyphs with different ASCII codes.
Scrolling
// Scroll full screen display.setCursor(0, 0); display.setTextSize(1); display.println("Full"); display.println("screen"); display.println("scrolling!"); display.display(); display.startscrollright(0x00, 0x07); delay(4500); display.stopscroll(); delay(1000); display.startscrollleft(0x00, 0x07); delay(4500); display.stopscroll(); delay(1000); display.startscrolldiagright(0x00, 0x07); delay(4500); display.startscrolldiagleft(0x00, 0x07); delay(4500); display.stopscroll(); display.clearDisplay();
Just like in the character LCDs we can also scroll text or even the entire screen with the Adafruit GFX library. The startscrollright and startscrollleft functions will scroll the display to right or left respectively. The values within the functions are the start and end page numbers. The current values in the code will scroll the entire display i.e. the whole 8 pages.
Drawing Rectangles
//draw rectangle display.setTextSize(1); display.setTextColor(WHITE); display.setCursor(0, 0); display.println("Rectangle"); display.drawRect(0, 15, 60, 40, WHITE); display.display(); delay(2000); display.clearDisplay();
The function is simple. You will need to specify the coordinate of the top left corner and the width, height, and color of the rectangle as follows drawRect(x, y, width, height, colour).
Drawing Filled Rectangles
//draw filled rectangle display.setCursor(0, 0); display.println("Filled Rectangle"); display.fillRect(0, 15, 60, 40, WHITE); display.display(); delay(2000); display.clearDisplay();
The function is similar to the drawRect function, the only difference is that it will fill up the rectangle. You will need to specify the coordinate of the top left corner and the width, height, and color of the rectangle as follows fillRect(x, y, width, height, colour).
Drawing Rectangles with Rounded Corners
//draw rectangle with rounded corners display.setCursor(0, 0) display.println("Round Rectangle"); display.drawRoundRect(0, 15, 60, 40, 8, WHITE); display.display(); delay(2000); display.clearDisplay();
The function is also similar to the drawRect function. You will need to specify the coordinate of the top left corner and the width, height, radius of the corner, and color of the rectangle as follows drawRoundRect (x, y, width, height, radius, colour).
Drawing Filled Rectangles with Rounded Corners
//draw filled rectangle with rounded corners display.setCursor(0, 0); display.println("Filled Round Rectangle"); display.fillRoundRect(0, 15, 60, 40, 8, WHITE); display.display(); delay(2000); display.clearDisplay();
The function is also similar to the drawRoundRect function, the only difference is that it will fill up the rounded rectangle. You will need to specify the coordinate of the top left corner and the width, height, radius of the corner, and color of the rectangle as follows fillRoundRect (x, y, width, height, radius, colour).
Drawing Circle
//draw circle display.setCursor(0, 0); display.println("Circle"); display.drawCircle(20, 35, 20, WHITE); display.display(); delay(2000); display.clearDisplay();
To draw a circle we can use the drawCircle function. This function requires three parameters – the x and y coordinates for the center point and the radius of the circle. The function format is drawCircle(x, y, Radius).
Drawing Filled Circle
//draw filled circle display.setCursor(0, 0); display.println("Filled Circle"); display.fillCircle(20, 35, 20, WHITE); display.display(); delay(2000); display.clearDisplay();
To draw a filled circle, we can use the fillCircle function. This function requires three parameters – the x and y coordinates for the centre point and the radius of the circle. The function format is fillCircle(x, y, Radius).
Drawing Triangle
//draw triangle display.setCursor(0, 0); display.println("Triangle"); display.drawTriangle(30, 15, 0, 60, 60, 60, WHITE); display.display(); delay(2000); display.clearDisplay();
To draw a triangle, the drawTriangle function requires a full set of seven parameters: the X, and Y coordinates for three corner points defining the triangle, followed by the colour. The format is drawTriangle(x1, y1, x2, y2, x3, y3, Colour).
Drawing Filled Triangle
//draw filled triangle display.setCursor(0, 0); display.println("Filled Triangle"); display.fillTriangle(30, 15, 0, 60, 60, 60, WHITE); display.display(); delay(2000); display.clearDisplay();
Similar to the drawTriangle function, the fillTriangle function also requires a full set of seven parameters: the X, and Y coordinates for three corner points defining the triangle, followed by the color. The format is fillTriangle(x1, y1, x2, y2, x3, y3, Colour).
Displaying Image on OLED Display
To display an image, first, you need to make sure its resolution is within the OLED size. For our example, we will need to resize any image that we are going to use to a resolution of 128x64 pixels. After that, we will need to convert this to a hex data array so that we can store this image in the code and can recall it whenever is needed. There are multiple ways to do all these and I’m going to show you the easiest of them. For that go to the Image2cpp website and upload your picture.
Set the canvas type size to 128 x 64. You can resize the image using the scaling option. Change all other settings until you are satisfied with the preview. Once you are done with all the changes select Arduino code as Code output format and click on generate code button. The corresponding array containing image data will be displayed below. Copy this code and past it to the global variable section of the code. Once done use the array name with the drawBitmap(x, y, array_name, Width, Hieght, Colour) function. Here is the whole code for displaying the image. Change the image array according to you to display your favorite image.
#include <Wire.h> #include <Adafruit_GFX.h> #include <Adafruit_SSD1306.h> #define SCREEN_WIDTH 128 // OLED display width, in pixels #define SCREEN_HEIGHT 64 // OLED display height, in pixels // Declaration for an SSD1306 display connected to I2C (SDA, SCL pins) #define OLED_RESET -1 // Reset pin # (or -1 if sharing Arduino reset pin) Adafruit_SSD1306 display(SCREEN_WIDTH, SCREEN_HEIGHT, &Wire, OLED_RESET); #define NUMFLAKES 10 // Number of snowflakes in the animation example #define LOGO_HEIGHT 64 #define LOGO_WIDTH 128 // 'CD_new_Logo', 128x64px static const unsigned char PROGMEM logo [] PROGMEM = { 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0xff, 0xcf, 0x3d, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xce, 0x38, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xce, 0x38, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xc6, 0x38, 0xff, 0xff, 0xff, 0xc0, 0x7c, 0x70, 0x03, 0xf8, 0x0f, 0x8f, 0xc7, 0x10, 0x00, 0xff, 0x80, 0x00, 0xff, 0xff, 0xff, 0x80, 0x3c, 0x70, 0x01, 0xf0, 0x07, 0x8f, 0xc7, 0x10, 0x00, 0xfe, 0x00, 0x00, 0x3f, 0xff, 0xff, 0x0e, 0x1c, 0x71, 0xf1, 0xe1, 0xc3, 0x8f, 0xc7, 0x1f, 0x8f, 0xfc, 0x00, 0x00, 0x0f, 0xff, 0xfe, 0x1f, 0x1c, 0x71, 0xf0, 0xc3, 0xe3, 0x8f, 0xc7, 0x1f, 0x8f, 0xf8, 0xff, 0xff, 0x8f, 0xff, 0xfe, 0x3f, 0xfc, 0x71, 0xf8, 0xc7, 0xff, 0x8f, 0xc7, 0x1f, 0x8f, 0xf9, 0xc0, 0x00, 0xc7, 0xff, 0xfe, 0x3f, 0xfc, 0x71, 0xf1, 0xc7, 0xff, 0x8f, 0xc7, 0x1f, 0x8f, 0xf1, 0x80, 0x00, 0x67, 0xff, 0xfe, 0x3f, 0xfc, 0x70, 0x01, 0xc7, 0xff, 0x8f, 0xc7, 0x1f, 0x8f, 0xf1, 0x00, 0x00, 0x63, 0xff, 0xfe, 0x3f, 0xfc, 0x70, 0x03, 0xc7, 0xff, 0x8f, 0xc7, 0x1f, 0x8f, 0x03, 0x0f, 0x80, 0x20, 0x7f, 0xfe, 0x3f, 0xfc, 0x70, 0x0f, 0xc7, 0xff, 0x8f, 0xc7, 0x1f, 0x8f, 0x03, 0x1f, 0xc0, 0x20, 0x7f, 0xfe, 0x3f, 0xbc, 0x71, 0xc3, 0xc7, 0xf3, 0x8f, 0xc7, 0x1f, 0x8f, 0xf3, 0x18, 0xe0, 0x23, 0xff, 0xfe, 0x3f, 0x1c, 0x71, 0xe3, 0xc3, 0xe3, 0x8f, 0xcf, 0x1f, 0x8f, 0xf3, 0x18, 0xe0, 0x27, 0xff, 0xff, 0x0e, 0x1c, 0x71, 0xe1, 0xe1, 0xc3, 0xc7, 0x8f, 0x1f, 0x8f, 0xf3, 0x39, 0xf8, 0x27, 0xff, 0xff, 0x00, 0x3c, 0x71, 0xf0, 0xf0, 0x07, 0xc0, 0x0f, 0x1f, 0x8f, 0x03, 0x19, 0x8c, 0x20, 0x7f, 0xff, 0xc0, 0x7c, 0x71, 0xf8, 0xf8, 0x0f, 0xe0, 0x1f, 0x1f, 0x8f, 0x03, 0x19, 0x84, 0x20, 0x7f, 0xff, 0xf1, 0xff, 0xff, 0xff, 0xfe, 0x3f, 0xf8, 0x7f, 0xff, 0xff, 0x03, 0x1d, 0x86, 0x20, 0x7f, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xf3, 0x0d, 0x86, 0x27, 0xff, 0xff, 0xff, 0xff, 0xf7, 0xff, 0xff, 0xdf, 0xff, 0xff, 0xff, 0xff, 0xf3, 0x01, 0x84, 0x27, 0xff, 0xfe, 0x03, 0xef, 0x80, 0xe0, 0x1e, 0x03, 0x00, 0x7f, 0xff, 0xff, 0xf3, 0x01, 0x8c, 0x23, 0xff, 0xfe, 0xf9, 0xef, 0x3e, 0x67, 0xfc, 0xf3, 0xf7, 0xff, 0xff, 0xff, 0x03, 0x01, 0xfc, 0x20, 0x7f, 0xfe, 0xfc, 0xee, 0x7e, 0x67, 0xfc, 0xfb, 0xf7, 0xff, 0xff, 0xff, 0x03, 0x01, 0xf0, 0x20, 0x7f, 0xfe, 0xfe, 0xee, 0xff, 0xe7, 0xfc, 0xff, 0xf7, 0xff, 0xff, 0xff, 0xf1, 0x00, 0x00, 0x63, 0xff, 0xfe, 0xfe, 0xee, 0xff, 0xe0, 0x1e, 0x0f, 0xf7, 0xff, 0xff, 0xff, 0xf9, 0x80, 0x00, 0x67, 0xff, 0xfe, 0xfe, 0xee, 0xf0, 0x60, 0x1f, 0x83, 0xf7, 0xff, 0xff, 0xff, 0xf9, 0xc0, 0x01, 0xc7, 0xff, 0xfe, 0xfe, 0xee, 0xf8, 0x67, 0xff, 0xf1, 0xf7, 0xff, 0xff, 0xff, 0xf8, 0x7f, 0xff, 0x8f, 0xff, 0xfe, 0xfc, 0xee, 0x7f, 0x67, 0xfd, 0xf9, 0xf7, 0xff, 0xff, 0xff, 0xfc, 0x00, 0x00, 0x1f, 0xff, 0xfe, 0xfc, 0xee, 0x7e, 0x67, 0xfc, 0xf9, 0xf7, 0xff, 0xff, 0xff, 0xff, 0x00, 0x00, 0x3f, 0xff, 0xfe, 0x71, 0xef, 0x1c, 0x67, 0xfc, 0x73, 0xf7, 0xff, 0xff, 0xff, 0xff, 0xc6, 0x38, 0xff, 0xff, 0xfe, 0x03, 0xef, 0x81, 0xe0, 0x0f, 0x07, 0xf7, 0xff, 0xff, 0xff, 0xff, 0xc6, 0x38, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xce, 0x38, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xce, 0x38, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xcf, 0x3d, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00 }; void setup() { Serial.begin(115200); // SSD1306_SWITCHCAPVCC = generate display voltage from 3.3V internally if (!display.begin(SSD1306_SWITCHCAPVCC, 0x3C)) { Serial.println(F("SSD1306 allocation failed")); for (;;); // Don't proceed, loop forever } // Clear the buffer display.clearDisplay(); display.drawBitmap(0, 0, logo, LOGO_WIDTH,LOGO_HEIGHT,WHITE); display.display(); } void loop() { delay(1); }
And here is the result.
Projects Using ESP32 and OLED Display Module
There are some interesting projects done with the ESP32 and OLED display. If you want to know more about those topics, links are given below.
In this tutorial, you will learn about Real Time Clock (RTC) and its interfacing with the ESP32 and OLED display. Also we explained how to build digital real time clock with ESP32 and OLED Display
We build Internet clock without using RTC module. This is more accurate as compare to RTC clock. ESP32 is a Wi-Fi module and can be easily connected to the internet so we will use NTP and UDP Protocol to fetch Time from the internet using Wi-Fi.
We build a Biometric Attendance System using ESP32 and OLED Display Module which can store the attendance records in google sheets.
Supporting Files
#include <Wire.h> #include <Adafruit_GFX.h> #include <Adafruit_SSD1306.h> #define SCREEN_WIDTH 128 // OLED display width, in pixels #define SCREEN_HEIGHT 64 // OLED display height, in pixels // Declaration for an SSD1306 display connected to I2C (SDA, SCL pins) #define OLED_RESET -1 // Reset pin # (or -1 if sharing Arduino reset pin) Adafruit_SSD1306 display(SCREEN_WIDTH, SCREEN_HEIGHT, &Wire, OLED_RESET); void setup() { Serial.begin(115200); // SSD1306_SWITCHCAPVCC = generate display voltage from 3.3V internally if (!display.begin(SSD1306_SWITCHCAPVCC, 0x3C)) { Serial.println(F("SSD1306 allocation failed")); for (;;); // Don't proceed, loop forever } // Clear the buffer. display.clearDisplay(); // Display Text display.setTextSize(1); display.setTextColor(WHITE); display.setCursor(0, 28); display.println("Hello world!"); display.display(); delay(2000); display.clearDisplay(); // Display Inverted Text display.setTextColor(BLACK, WHITE); // 'inverted' text display.setCursor(0, 28); display.println("Hello world!"); display.display(); delay(2000); display.clearDisplay(); // Changing Font Size display.setTextColor(WHITE); display.setCursor(0, 24); display.setTextSize(2); display.println("Hello!"); display.display(); delay(2000); display.clearDisplay(); // Display Numbers display.setTextSize(1); display.setCursor(0, 28); display.println(123456789); display.display(); delay(2000); display.clearDisplay(); // Specifying Base For Numbers display.setCursor(0, 28); display.print("0x"); display.print(0xFF, HEX); display.print("(HEX) = "); display.print(0xFF, DEC); display.println("(DEC)"); display.display(); delay(2000); display.clearDisplay(); // Display ASCII Characters display.setCursor(0, 24); display.setTextSize(2); display.write(1); display.display(); delay(2000); display.clearDisplay(); // Scroll full screen display.setCursor(0, 0); display.setTextSize(1); display.println("Full"); display.println("screen"); display.println("scrolling!"); display.display(); display.startscrollright(0x00, 0x07); delay(4500); display.stopscroll(); delay(1000); display.startscrollleft(0x00, 0x07); delay(4500); display.stopscroll(); delay(1000); display.startscrolldiagright(0x00, 0x07); delay(4500); display.startscrolldiagleft(0x00, 0x07); delay(4500); display.stopscroll(); display.clearDisplay(); //draw rectangle display.setTextSize(1); display.setTextColor(WHITE); display.setCursor(0, 0); display.println("Rectangle"); display.drawRect(0, 15, 60, 40, WHITE); display.display(); delay(2000); display.clearDisplay(); //draw filled rectangle display.setTextSize(1); display.setTextColor(WHITE); display.setCursor(0, 0); display.println("Filled Rectangle"); display.fillRect(0, 15, 60, 40, WHITE); display.display(); delay(2000); display.clearDisplay(); //draw rectangle with rounded corners display.setTextSize(1); display.setTextColor(WHITE); display.setCursor(0, 0); display.println("Round Rectangle"); display.drawRoundRect(0, 15, 60, 40, 8, WHITE); display.display(); delay(2000); display.clearDisplay(); //draw filled rectangle with rounded corners display.setTextSize(1); display.setTextColor(WHITE); display.setCursor(0, 0); display.println("Filled Round Rectangl"); display.fillRoundRect(0, 15, 60, 40, 8, WHITE); display.display(); delay(2000); display.clearDisplay(); //draw circle display.setTextSize(1); display.setTextColor(WHITE); display.setCursor(0, 0); display.println("Circle"); display.drawCircle(20, 35, 20, WHITE); display.display(); delay(2000); display.clearDisplay(); //draw filled circle display.setTextSize(1); display.setTextColor(WHITE); display.setCursor(0, 0); display.println("Filled Circle"); display.fillCircle(20, 35, 20, WHITE); display.display(); delay(2000); display.clearDisplay(); //draw triangle display.setTextSize(1); display.setTextColor(WHITE); display.setCursor(0, 0); display.println("Triangle"); display.drawTriangle(30, 15, 0, 60, 60, 60, WHITE); display.display(); delay(2000); display.clearDisplay(); //draw filled triangle display.setTextSize(1); display.setTextColor(WHITE); display.setCursor(0, 0); display.println("Filled Triangle"); display.fillTriangle(30, 15, 0, 60, 60, 60, WHITE); display.display(); delay(2000); display.clearDisplay(); } void loop() {}
......................................................................................................................
#include <SPI.h> #include <Wire.h> #include <Adafruit_GFX.h> #include <Adafruit_SSD1306.h> #define SCREEN_WIDTH 128 // OLED display width, in pixels #define SCREEN_HEIGHT 64 // OLED display height, in pixels // Declaration for an SSD1306 display connected to I2C (SDA, SCL pins) #define OLED_RESET -1 // Reset pin # (or -1 if sharing Arduino reset pin) Adafruit_SSD1306 display(SCREEN_WIDTH, SCREEN_HEIGHT, &Wire, OLED_RESET); #define NUMFLAKES 10 // Number of snowflakes in the animation example #define LOGO_HEIGHT 64 #define LOGO_WIDTH 128 // 'CD_new_Logo', 128x64px static const unsigned char PROGMEM logo [] PROGMEM = { 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0xff, 0xcf, 0x3d, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xce, 0x38, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xce, 0x38, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xc6, 0x38, 0xff, 0xff, 0xff, 0xc0, 0x7c, 0x70, 0x03, 0xf8, 0x0f, 0x8f, 0xc7, 0x10, 0x00, 0xff, 0x80, 0x00, 0xff, 0xff, 0xff, 0x80, 0x3c, 0x70, 0x01, 0xf0, 0x07, 0x8f, 0xc7, 0x10, 0x00, 0xfe, 0x00, 0x00, 0x3f, 0xff, 0xff, 0x0e, 0x1c, 0x71, 0xf1, 0xe1, 0xc3, 0x8f, 0xc7, 0x1f, 0x8f, 0xfc, 0x00, 0x00, 0x0f, 0xff, 0xfe, 0x1f, 0x1c, 0x71, 0xf0, 0xc3, 0xe3, 0x8f, 0xc7, 0x1f, 0x8f, 0xf8, 0xff, 0xff, 0x8f, 0xff, 0xfe, 0x3f, 0xfc, 0x71, 0xf8, 0xc7, 0xff, 0x8f, 0xc7, 0x1f, 0x8f, 0xf9, 0xc0, 0x00, 0xc7, 0xff, 0xfe, 0x3f, 0xfc, 0x71, 0xf1, 0xc7, 0xff, 0x8f, 0xc7, 0x1f, 0x8f, 0xf1, 0x80, 0x00, 0x67, 0xff, 0xfe, 0x3f, 0xfc, 0x70, 0x01, 0xc7, 0xff, 0x8f, 0xc7, 0x1f, 0x8f, 0xf1, 0x00, 0x00, 0x63, 0xff, 0xfe, 0x3f, 0xfc, 0x70, 0x03, 0xc7, 0xff, 0x8f, 0xc7, 0x1f, 0x8f, 0x03, 0x0f, 0x80, 0x20, 0x7f, 0xfe, 0x3f, 0xfc, 0x70, 0x0f, 0xc7, 0xff, 0x8f, 0xc7, 0x1f, 0x8f, 0x03, 0x1f, 0xc0, 0x20, 0x7f, 0xfe, 0x3f, 0xbc, 0x71, 0xc3, 0xc7, 0xf3, 0x8f, 0xc7, 0x1f, 0x8f, 0xf3, 0x18, 0xe0, 0x23, 0xff, 0xfe, 0x3f, 0x1c, 0x71, 0xe3, 0xc3, 0xe3, 0x8f, 0xcf, 0x1f, 0x8f, 0xf3, 0x18, 0xe0, 0x27, 0xff, 0xff, 0x0e, 0x1c, 0x71, 0xe1, 0xe1, 0xc3, 0xc7, 0x8f, 0x1f, 0x8f, 0xf3, 0x39, 0xf8, 0x27, 0xff, 0xff, 0x00, 0x3c, 0x71, 0xf0, 0xf0, 0x07, 0xc0, 0x0f, 0x1f, 0x8f, 0x03, 0x19, 0x8c, 0x20, 0x7f, 0xff, 0xc0, 0x7c, 0x71, 0xf8, 0xf8, 0x0f, 0xe0, 0x1f, 0x1f, 0x8f, 0x03, 0x19, 0x84, 0x20, 0x7f, 0xff, 0xf1, 0xff, 0xff, 0xff, 0xfe, 0x3f, 0xf8, 0x7f, 0xff, 0xff, 0x03, 0x1d, 0x86, 0x20, 0x7f, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xf3, 0x0d, 0x86, 0x27, 0xff, 0xff, 0xff, 0xff, 0xf7, 0xff, 0xff, 0xdf, 0xff, 0xff, 0xff, 0xff, 0xf3, 0x01, 0x84, 0x27, 0xff, 0xfe, 0x03, 0xef, 0x80, 0xe0, 0x1e, 0x03, 0x00, 0x7f, 0xff, 0xff, 0xf3, 0x01, 0x8c, 0x23, 0xff, 0xfe, 0xf9, 0xef, 0x3e, 0x67, 0xfc, 0xf3, 0xf7, 0xff, 0xff, 0xff, 0x03, 0x01, 0xfc, 0x20, 0x7f, 0xfe, 0xfc, 0xee, 0x7e, 0x67, 0xfc, 0xfb, 0xf7, 0xff, 0xff, 0xff, 0x03, 0x01, 0xf0, 0x20, 0x7f, 0xfe, 0xfe, 0xee, 0xff, 0xe7, 0xfc, 0xff, 0xf7, 0xff, 0xff, 0xff, 0xf1, 0x00, 0x00, 0x63, 0xff, 0xfe, 0xfe, 0xee, 0xff, 0xe0, 0x1e, 0x0f, 0xf7, 0xff, 0xff, 0xff, 0xf9, 0x80, 0x00, 0x67, 0xff, 0xfe, 0xfe, 0xee, 0xf0, 0x60, 0x1f, 0x83, 0xf7, 0xff, 0xff, 0xff, 0xf9, 0xc0, 0x01, 0xc7, 0xff, 0xfe, 0xfe, 0xee, 0xf8, 0x67, 0xff, 0xf1, 0xf7, 0xff, 0xff, 0xff, 0xf8, 0x7f, 0xff, 0x8f, 0xff, 0xfe, 0xfc, 0xee, 0x7f, 0x67, 0xfd, 0xf9, 0xf7, 0xff, 0xff, 0xff, 0xfc, 0x00, 0x00, 0x1f, 0xff, 0xfe, 0xfc, 0xee, 0x7e, 0x67, 0xfc, 0xf9, 0xf7, 0xff, 0xff, 0xff, 0xff, 0x00, 0x00, 0x3f, 0xff, 0xfe, 0x71, 0xef, 0x1c, 0x67, 0xfc, 0x73, 0xf7, 0xff, 0xff, 0xff, 0xff, 0xc6, 0x38, 0xff, 0xff, 0xfe, 0x03, 0xef, 0x81, 0xe0, 0x0f, 0x07, 0xf7, 0xff, 0xff, 0xff, 0xff, 0xc6, 0x38, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xce, 0x38, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xce, 0x38, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xcf, 0x3d, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00 }; void setup() { Serial.begin(115200); // SSD1306_SWITCHCAPVCC = generate display voltage from 3.3V internally if (!display.begin(SSD1306_SWITCHCAPVCC, 0x3C)) { Serial.println(F("SSD1306 allocation failed")); for (;;); // Don't proceed, loop forever } // Clear the buffer display.clearDisplay(); display.drawBitmap(0, 0, logo, LOGO_WIDTH,LOGO_HEIGHT,WHITE); display.display(); } void loop() { delay(1); }