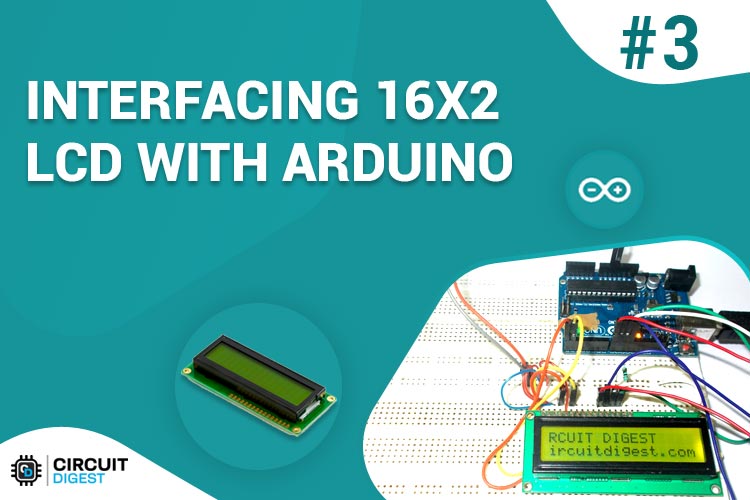
To establish a good communication between human world and machine world, display units play an important role. And so they are an important part of embedded systems. Display units - big or small, work on the same basic principle. Besides complex display units like graphic displays and 3D dispays, one must know working with simple displays like 16x1 and 16x2 units. The 16x1 display unit will have 16 characters and are in one line. The 16x2 LCD will have 32 characters in total 16in 1st line and another 16 in 2nd line. Here one must understand that in each character there are 5x10=50 pixels so to display one character all 50 pixels must work together. But we need not to worry about that because there is another controller (HD44780) in the display unit which does the job of controlling the pixels. (you can see it in LCD unit, it is the black eye at the back ).
In this tutorial, we are going to interface a 16x2 LCD with ARDUINO UNO. Unlike normal development boards interfacing an LCD to an ARDUINO is quite easy. Here we don’t have to worry about data sending and receiving. We just have to define the pin numbers and it will be ready to display data on LCD.
Note: We updated this tutorial and added some more additional information along with a step-by-step guide to interface 16x2 LCD with Arduino. You can follow the below link for an updated tutorial.
This tutorial provides Step-by-step guide, Circuit Diagram, and Complete code to learn how to Interface 16x2 LCD with Arduino
Components Required
Hardware: ARDUINO UNO, power supply (5v), JHD_162ALCD(16x2LCD), 100uF capacitor.
Software: Arduino IDE (Arduino nightly).
Circuit Diagram and Explanation
In 16x2 LCD there are 16 pins over all if there is a back light, if there is no back light there will be 14 pins. One can power or leave the back light pins. Now in the 14 pins there are 8 data pins (7-14 or D0-D7), 2 power supply pins (1&2 or VSS&VDD or GND&+5v), 3rd pin for contrast control (VEE-controls how thick the characters should be shown), and 3 control pins (RS&RW&E).
In the circuit, you can observe I have only took two control pins, this gives the flexibility. The contrast bit and READ/WRITE are not often used so they can be shorted to ground. This puts LCD in highest contrast and read mode. We just need to control ENABLE and RS pins to send characters and data accordingly.
The connections which are done for LCD are given below:
PIN1 or VSS to ground
PIN2 or VDD or VCC to +5v power
PIN3 or VEE to ground (gives maximum contrast best for a beginner)
PIN4 or RS (Register Selection) to PIN0 of ARDUINO UNO
PIN5 or RW (Read/Write) to ground (puts LCD in read mode eases the communication for user)
PIN6 or E (Enable) to PIN1 of ARDUINO UNO
PIN11 or D4 to PIN8 of ARDUINO UNO
PIN12 or D5 to PIN9 of ARDUINO UNO
PIN13 or D6 to PIN10 of ARDUINO UNO
PIN14 or D7 to PIN11 of ARDUINO UNO
The ARDUINO IDE allows the user to use LCD in 4 bit mode. This type of communication enables the user to decrease the pin usage on ARDUINO, unlike other the ARDUINO need not to be programmed separately for using it in 4 it mode because by default the ARDUINO is set up to communicate in 4 bit mode. In the circuit you can see we have used 4bit communication (D4-D7).
So from mere observation from above table we are connecting 6 pins of LCD to controller in which 4 pins are data pins and 2 pins for control.
The above figure shows the circuit diagram of 16x2 LCD connected to ARDUINO UNO.
Working
To interface a LCD to the ARDUINO UNO, we need to know a few things.
|
As by the above table we only need to look at these four lines for establishing a communication between an ARDUINO and LCD.
First we need to enable the header file (‘#include <LiquidCrystal.h>’), this header file has instructions written in it, which enables the user to interface an LCD to UNO in 4 bit mode without any fuzz. With this header file we need not have to send data to LCD bit by bit, this will all be taken care of and we don’t have to write a program for sending data or a command to LCD bit by bit.
Second we need to tell the board which type of LCD we are using here. Since we have so many different types of LCD (like 20x4, 16x2, 16x1 etc.). Here we are going to interface a 16x2 LCD to the UNO so we get ‘lcd.begin(16, 2);’. For 16x1 we get ‘lcd.begin(16, 1);’.
In this instruction we are going to tell the board where we connected the pins. The pins which are connected need to be represented in order as “RS, En, D4, D5, D6, D7”. These pins are to be represented correctly. Since we have connected RS to PIN0 and so on as show in the circuit diagram, we represent the pin number to board as “LiquidCrystal lcd(0, 1, 8, 9, 10, 11);”. The data which needs to be displayed in LCD should be written as “ cd.print("hello, world!");”. With this command the LCD displays ‘hello, world!’.
As you can see we need not to worry about any thing else, we just have to initialize and the UNO will be ready to display data. We don’t have to write a program loop to send the data BYTE by BYTE here.
The way of communication between LCD and UNO is explained step by step in C code given below:
Complete Project Code
#include <LiquidCrystal.h>
// initialize the library with the numbers of the interface pins
LiquidCrystal lcd(0, 1, 8, 9, 10, 11); /// REGISTER SELECT PIN,ENABLE PIN,D4 PIN,D5 PIN, D6 PIN, D7 PIN
void setup()
{
// set up the LCD's number of columns and rows:
lcd.begin(16, 2);
}
void loop()
{
// set the cursor to column 0, line 1
lcd.print(" CIRCUIT DIGEST");//print name
lcd.setCursor(0, 1); // set the cursor to column 0, line 2
lcd.print("www.circuitdigest.com");//print name
delay(750);//delay of 0.75sec
lcd.scrollDisplayLeft();//shifting data on LCD
lcd.setCursor(0, 0);// set the cursor to column 0, line1
}
Comments
We have lot of GSM with
We have lot of GSM with Arduino projects, check them to get it properly interfaced.
All black cursors
My display shows only black squares. The text appears however if I look from an angle. What should I do? Thx.
It turns out that a pot was
It turns out that a pot was needed on gnd-v0-vss in order to adjust contrast.
LCD interface successful
Thanks for sharing the code and interfacing diagram.
I have included a 2.2k resistor between Vo (pin 3 of LCD Display) and ground to eliminate black squares.
information
is posible to to give 5v supply vcc lcd using Ardiuno but im only using usb port of Ardiuno
Is it possible to connect LED
Is it possible to connect LED display with Arduino
i dont have bread board so
i dont have bread board so how can i connect LCD to aurdino board??
arduino+gas sensor+16/2 gas sensor
i need a code and circuit diagram to detect gas by gas sensor and display the gas percentage in lcd
by the way in my code i have involve buzzer too.when gas value exceeds 15 my buzzer have to run.plz i need it quickly
Arduino interface to 16 x 2 LCD
Who says you can't teach an old dinosaur new tricks ?? I've been searching for a simple solution for getting data written to this LCD, and you have the best and simplest solution. I' working on an RPM counter where I need to output the data, so now I can do this.... Most of the other tutorials didn't mention the pins 0 and 1 for interfacing ??
You did an excellent job...
Interfacing 16x2 LCD with Arduino
This article was a great help. I recently came by a number of Data Image CM2020 16*2 displays. Turns out they have the identical interface pins. Now I can use them.
LCD SYSTEM
I am working on LCD with AT89C52 as interface. After my construction, I am finding it difficult to program the system to display my intended data.
How can I remove those black
How can I remove those black squares without using any potentiometer ?
100uF capacitor
Excellent it was very informing tutorial.
one thing though I did not see the 100uF capacitor on the connection image a resistor is there. thanks in advance
I want to display traffic information such as stop like dat..
We r planned to use lcd with traffic lights