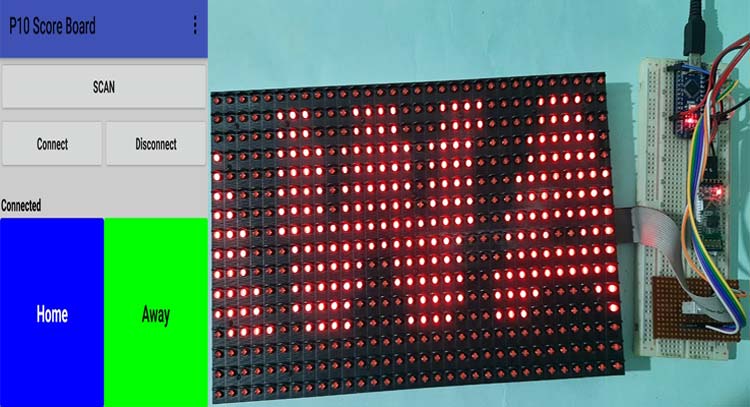
An electronic scoreboard is one of the most important gadgets anybody can have during any sports tournament. Old manual scoreboard using conventional methods are very time consuming and Error-prone, hence a computerized scoreboard becomes necessary where the display unit needs to be changed in real-time. This is why in this project, we will be building a Bluetooth controlled wireless scoreboard in which we can change the score on the board just by using an android application. The brain of this project is an Arduino Nano, and for the display part, we will be using a P10 LED matrix to show the score remotely in real-time.
The P10 LED Display Matrix
A P10 LED Matrix Display is the best way available to make a LED advertisement board for outdoor or indoor use. This panel has a total of 512 high brightness LEDs mounted on a plastic housing designed for best display results. It also comes with an IP65 rating for waterproofing making it perfect for outdoor use. With this, you can make a big LED signboard by combining any number of such panels in any row and column structure.
Our module has a size of 32*16, which means that there are 32 LEDs in each row and 16 LEDs in each column. So, there is a total of 512 LEDs present in each led signboard. Other than that, it has an IP65 rating for waterproofing, it can be powered by a single 5V power source, it has a very wide viewing angle, and the brightness can go up to 4500 nits. So, you will be able to see it clearly in brought daylight. Previously we have also used this P10 Display with Arduino to build a simple LED Board.
Pin Description of P10 LED Matrix:
This LED display board uses a 10-pin mail header for input and output connection, in this section, we have described all the necessary pins of this module. Also, you can see there is an external 5V connector in the middle of the module which is used to connect the external power to the board.
- Enable: This pin is used to control the brightness of the LED panel, by giving a PWM pulse to it.
- A, B: These are called multiplex select pins. They take digital input to select any multiplex rows.
- Shift clock (CLK), Store clock (SCLK), and Data: These are the normal shift register control pins. Here a shift register 74HC595 is used.
Interfacing P10 LED Display Module to Arduino:
Connecting the P10 matrix display module to Arduino is a very simple process, in our circuit, we configured pin 9 of the Arduino as Enable pin, Pin 6 as Pin A, Pin 7 as pin B, Pin 13 is the CLK, Pin 8 is the SCLK, Pin 11 is the DATA, and finally Pin GND is the GND pin for the module and Arduino, a complete table below explain the pin configuration clearly.
P10 LED Module |
Arduino UNO |
ENABLE |
9 |
A |
6 |
B |
7 |
CLK |
13 |
SCLK |
8 |
DATA |
11 |
GND |
GND |
Note: Connect the power terminal of the P10 module to an external 5V power source, because 512 LEDs will consume a lot of power. It is recommended to connect a 5V, 3 Amp DC power supply to a single unit of P10 LED module. If you are planning to connect more numbers module, then increase your SMPS capacity accordingly.
Components Required for Arduino Scoreboard
As this is a very simple project, the components requirements are very generic, a list of required components are shown below, you should be able to find all of the listed material in your local hobby store.
- Arduino Nano
- P10 LED matrix display
- Breadboard
- 5V, 3 AMP SMPS
- HC-05 Bluetooth Module
- Connecting Wires
Circuit Diagram for Arduino Scoreboard
The Schematic for the Arduino LED Scoreboard is shown below as this project is very simple, I have used the popular software fritzing to develop the schematic.
The working of the circuit is very simple, we have an Android application and a Bluetooth module, to successfully communicate with the Bluetooth module, you have to pair the HC-05 module with the android application. Once we are connected, we can send the string that we want to display, once the string is sent, Arduino will process the string and convert it to a signal that the internal 74HC595 shift resistor can understand, after the data is sent to the shift resistor, its ready to display.
Arduino Scoreboard Code Explanation
After the successful completion of the hardware setup, now it’s time for the programming of Arduino Nano. The stepwise description of the code is given below. Also, you can get the complete Arduino Scoreboard code at the bottom of this Tutorial.
First of all, we need to include all the libraries. We have used the DMD.h Library in order to control the P10 led display. You can download and include it from the given GitHub link. After that, you need to include the TimerOne.h Library, which will be used for interrupt programming in our code.
There are many fronts available in this library, we have used “Arial_black_16” for this project.
#include <SPI.h> #include <DMD.h> #include <TimerOne.h> #include "SystemFont5x7.h" #include "Arial_black_16.h"
In the next step, the number of rows and columns are defined for our LED matrix board. We have used only one module in this project, so both ROW value and COLUMN value can be defined as 1.
#define ROW 1 #define COLUMN 1 #define FONT Arial_Black_16 DMD led_module (ROW, COLUMN);
After that, all the variables used in the code are defined. A character variable is used to receive serial data from Android App, two integer values are used to store scores, and an array is defined which stores the final data to be displayed on the Matrix.
char input; int a = 0, b = 0; int flag = 0; char cstr1[50];
A Function scan_module() is defined, which continuously checks for any incoming data from Arduino Nano through the SPI. If yes, then it will trigger an interrupt for doing certain events as defined by the user in the program.
void scan_module() { led_module.scanDisplayBySPI(); }
Inside setup(), the timer is initialized, and the interrupt is attached to the function scan_module, which was discussed earlier. Initially, the screen was cleared using the function clear screen(true), which means all the pixels are defined as OFF.
In the setup, serial communication was also enabled using function Serial.begin(9600) where 9600 is the baud rate for Bluetooth communication.
void setup() { Serial.begin(9600); Timer1.initialize(2000); Timer1.attachInterrupt(scan_module); led_module.clearScreen( true ); }
Here, the serial data availability is checked, if there is valid data is coming from Arduino or not. The received data from the App is stored in a variable.
if(Serial.available() > 0) { flag = 0; input = Serial.read();
Then, the received value was compared with the predefined variable. Here, in the Android application, two buttons are taken to select the scores for both teams. When button 1 is pressed, Character ‘a’ is transmitted to Arduino and when button2 is pressed, Character ‘b’ is transmitted to Arduino. Hence, in this section, this data is matched, and if matched, then the respective score values are incremented as shown in the code.
if (input == 'a' && flag == 0) { flag = 1; a++; } else if (input == 'b' && flag == 0) { flag = 1; b++; } else;
Then, the received data is converted into a character Array, as the P10 matrix function is only capable of displaying character data type. This is why all variables are converted and concatenated into a character array.
(String("HOME:")+String(a) + String(" - ") + String("AWAY:")+String(b)).toCharArray(cstr1, 50);
Then, to display information in the module, the font is selected using the selection() function. Then drawMarquee() function is used to display the desired information on the P10 board.
led_module.selectFont(FONT); led_module.drawMarquee(cstr1,50, (32 * ROW), 0);
Finally, as we need a scrolling message display, I have written a code to shift our whole message from Right to Left directions using a certain period.
long start = millis(); long timming = start; boolean flag = false; while (!flag) { if ((timming + 30) < millis()) { flag = led_module.stepMarquee(-1, 0); timming = millis(); } }
This marks the end of our coding process. And now it’s ready for uploading.
Smartphone Controlled Scoreboard - Testing
After uploading code to Arduino, it’s time to test the project. Before that, the android application needs to be installed on our smartphone. You can download the P10 Score Board application from the given link. Once installed, open the app and the home screen should look like the below image.
Click on the SCAN button to add the Bluetooth module with App. This will show the list of paired Bluetooth devices of the phone. If you haven’t paired the HC-05 Bluetooth module before, pair the module using your phone’s Bluetooth setting and then do this step. The screen will look like shown:
Then, from the list, click on “HC-05” as this is the name of our Bluetooth module used here. After clicking on it, it will show connected on the screen. Then we can proceed with the scoreboard.
Click on any button between “Home” & “Away” as shown in the App. If the Home button is selected, the score of Home will be incremented in the P10 display. Similarly, if the Away button is selected, the score of Away will be incremented. The below image shows how the final screen looks.
I hope you liked the project and learned something new, if you have any other questions regarding the project, feel free to comment down below or you can ask your question in our forum.
Complete Project Code
#include <SPI.h>
#include <DMD.h>
#include <TimerOne.h>
#include "SystemFont5x7.h"
#include "Arial_black_16.h"
#define ROW 1
#define COLUMN 1
#define FONT Arial_Black_16
char input;
int a = 0, b = 0;
int flag = 0;
char cstr1[50];
DMD led_module(ROW, COLUMN);
void scan_module()
{
led_module.scanDisplayBySPI();
}
void setup()
{
Serial.begin(9600);
Timer1.initialize(2000);
Timer1.attachInterrupt(scan_module);
led_module.clearScreen( true );
}
void loop()
{
if(Serial.available() > 0)
{
flag = 0;
input = Serial.read();
if (input == 'a' && flag == 0)
{
flag = 1;
a++;
}
else if (input == 'b' && flag == 0)
{
flag = 1;
b++;
}
else;
}
(String("HOME:")+String(a) + String(" - ") + String("AWAY:")+String(b)).toCharArray(cstr1, 50);
led_module.selectFont(FONT);
led_module.drawMarquee(cstr1,50, (32 * ROW), 0);
long start = millis();
long timming = start;
boolean flag = false;
while (!flag)
{
if ((timming + 30) < millis())
{
flag = led_module.stepMarquee(-1, 0);
timming = millis();
}
}
}
Comments
Before that, the android
Before that, the android application needs to be installed on our smartphone. You can download the P10 Score Board application from the given link. Once installed, open the app and the home screen should look like the below image.
There is no link for android apps. above Please proved the link to download or give the file location from which we can get.
Thanks
If I wanted to insert two
If I wanted to insert two team names instead of Home and Away name then what changes i have to make?
Please provide the smartphone aps link as there is no link availablre.