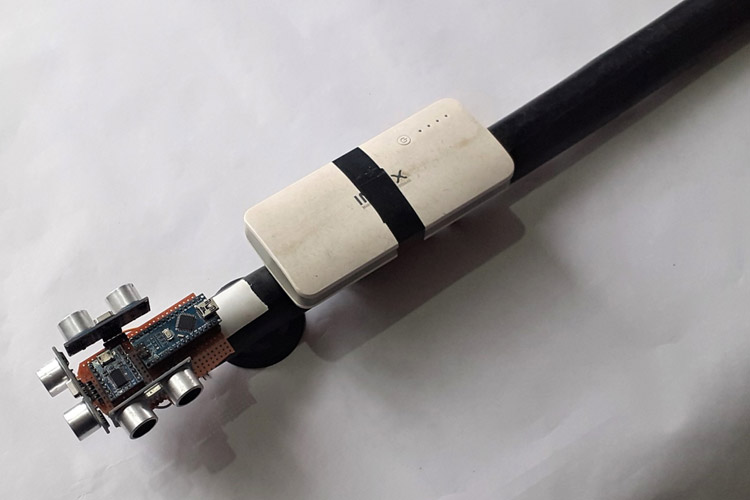
Obstacle detection is one of the major concerns for a blind person. Presented here is a smart blind stick using Arduino Uno and Ultrasonic sensors. The primary goal of this project is to assist blind people to walk with ease and to alert them whenever their walking path is obstructed by other objects or people. As a warning signal, a Voice module is connected in the circuit, that gives a voice warning according to the direction of the object, for example, if the object is in Left then it will say ‘Careful Object in Left’.
This Smart stick will have three Ultrasonic sensors connected in left, right, and center to sense distance from any obstacle, a JQ6500 Voice Sound Module to give warning signal, and a 9V battery to power the setup.
Components Required for Smart Blind Stick
- Arduino Nano
- 3× Ultrasonic Sensor
- JQ6500 Voice Sound Module
- 8Ω Speaker
- 1 KΩ Resistor
JQ6500 Voice Sound Module
JQ6500 Voice Sound Module is perfect for broadcasting a message on the speaker like a fire alarm, train, and bus alert systems, business hall prompts, equipment failure alarms, etc. It can decode the MP3, hardcoded MP3, or WMV format files into the audible voice format. It comes with 24 bits Digital-to-Analog Converter with a dynamic range of 90dB and supports 8 / 11.025 / 12/16 / 22.05 / 24/32 / 44.1 / 48 kHz sampling rate. The MP3 files can be controlled with buttons, or via a serial communications protocol.
Uploading MP3 files to the onboard Memory:
The MP3 files to the on-board memory of JQ6500 can be uploaded using a Windows computer (Mac or Linux users you will need to use the JQ6500-28p and an SD Card). Steps for the same are given below:
- Connect the board to your computer over the Mini USB connector.
- A new “CD-ROM” drive will appear on your computer, double click on that and open the application named ‘MusicDownload.exe’.
- Some JQ6500-16P devices do not have the MusicDownload.exe installed on them, if you can’t find the MusicDownload.exe program; you can download this zip file that contains it.
- The application is in the Chinese language, so to upload the files, open the .exe file and then click the second tab from the left.
- Then click on the file selector tab that is in the right corner.
- A file selector will open, select all the mp3 files you wish to upload click the Open button on the file selector
- Click back to the first tab and then click the button to upload the files
Smart Blind Stick Circuit Diagram
The complete circuit diagram for Smart Blind Stick is shown below. It is pretty simple as we only need to connect three ultrasonic sensors and one JQ6500 voice module.
The complete board is powered by a 9V battery. The brain of the circuit is Arduino Nano. Three Ultrasonic sensors are used for obstacle detection in the left, right, and front of the stick. Two of the four pins of these three sensors, namely VCC & GND, are connected to Arduino’s 5V and GND. The remaining two pins–TRIG & ECHO are connected to Arduino as follows.
Ultrasonic Sensor (Left) |
Arduino Nano |
Trig |
D6 |
Echo |
D7 |
Ultrasonic Sensor (Right) |
Arduino Nano |
Trig |
D2 |
Echo |
D3 |
Ultrasonic Sensor (Centre) |
Arduino Nano |
Trig |
D4 |
Echo |
D5 |
The JQ5600 MP3 module is a 3.3V logic module, so you can’t connect it directly to the Arduino’s IO pins, but it is fine to be powered from the Arduino’s 5V power line. RX and TX pins of the MP3 module are connected to digital pins 9 and 8 of the Arduino Nano. A 1kΩ resistor is put in between the Arduino digital pin 9 to MP3 module RX to drop the voltage down from the Arduino’s 5V.
Arduino Program for Smart Blind Stick
Once we are ready with our hardware, we can connect the Arduino to our computer and start programming. The complete code for this project is given at the bottom of this page; you can upload it directly to your Arduino board. However, if you are curious to know how the code works, read further.
The code uses JQ6500_Serial.h and SoftwareSerial.h libraries. SoftwareSerial library comes pre-install with Arduino IDE while the JQ6500 Serial library can be installed from the given link.
As usual, start the code by including all the required libraries and defining all the pins used in this project.
#include <Arduino.h> #include <SoftwareSerial.h> #include <JQ6500_Serial.h> JQ6500_Serial mp3(8,9); int left_trigPin = 6; int left_echoPin = 7; int right_trigPin = 2; int right_echoPin = 3; int center_trigPin = 4; int center_echoPin = 5;
Then inside the setup() function, initialize Input Output pins. In our program, the Trigger pins of all three sensors are the Output device and the Echo pins are the Input device. We also initialize the serial monitor and JQ6500 voice module.
pinMode(left_trigPin,OUTPUT); pinMode(left_echoPin,INPUT); pinMode(right_trigPin,OUTPUT); pinMode(right_echoPin,INPUT); pinMode(center_trigPin,OUTPUT); pinMode(center_echoPin,INPUT); Serial.begin(115200); mp3.begin(9600); mp3.reset();
Inside the main loop we are reading all three sensors' data, i.e. left, right, and center. We begin with reading the sensor data of the Ultrasonic sensor for distance, and then calculate the distance using the time taken between triggering and Received ECHO. The formula for calculating distance is given below:
digitalWrite (left_trigPin, HIGH); delayMicroseconds (10); digitalWrite (left_trigPin, LOW); duration = pulseIn (left_echoPin, HIGH); distance = (duration/2) / 29.1;
There will be no warning if the measured distance is more than 50cm. But, if it is less than 50cm the Voice module will be triggered to play the voice warning.
if (distance < 50) { Serial.print("Left Distance"); Serial.print(distance); mp3.playFileByIndexNumber(2); }
The same logic is used for all three sensors. The program can be easily adapted for your application by changing the value which we use to compare. You use the serial monitor to debug if a false alarm is trigger.
Testing the Smart Arduino Blind Stick
Finally, it’s time to test our blind stick Arduino project. Make sure the connections are done as per the circuit diagram and the program is successfully uploaded. Now, power the setup using a 9V battery and you should start to see results. Move the blind walking stick closer to the object and you will notice the voice warning according to the direction of the object. For example, if the object is in Left, then it will say ‘Careful Object in Left’.
The complete working of this Smart Arduino blind stick is shown in the video given at the end of this page.
Complete Project Code
#include <Arduino.h>
#include <SoftwareSerial.h>
#include <JQ6500_Serial.h>
JQ6500_Serial mp3(8,9);
int left_trigPin = 7;
int left_echoPin = 6;
int right_trigPin = 2;
int right_echoPin = 3;
int center_trigPin = 4;
int center_echoPin = 5;
void setup() {
pinMode(left_trigPin,OUTPUT);
pinMode(left_echoPin,INPUT);
pinMode(right_trigPin,OUTPUT);
pinMode(right_echoPin,INPUT);
pinMode(center_trigPin,OUTPUT);
pinMode(center_echoPin,INPUT);
Serial.begin(115200);
mp3.begin(9600);
mp3.reset();
mp3.setVolume(50);
mp3.setLoopMode(MP3_LOOP_NONE);
}
void loop(){
left();
right();
center();
}
void left(){
delay(500);// reading will be taken after ....miliseconds
Serial.println("\n");
int duration, distance;
digitalWrite (left_trigPin, HIGH);
delayMicroseconds (10);
digitalWrite (left_trigPin, LOW);
duration = pulseIn (left_echoPin, HIGH);
distance = (duration/2) / 29.1;
//distance= duration*0.034/2;
if (distance < 30) { // Change the number for long or short distances.
Serial.print("Left Distance");
Serial.print(distance);
mp3.playFileByIndexNumber(2);
}
}
void right(){
delay(500);// reading will be taken after ....miliseconds
Serial.println("\n");
int duration, distance;
digitalWrite (right_trigPin, HIGH);
delayMicroseconds (10);
digitalWrite (right_trigPin, LOW);
duration = pulseIn (right_echoPin, HIGH);
distance = (duration/2) / 29.1;
if (distance <30) { // Change the number for long or short distances.
Serial.print("Right Distance");
Serial.print(distance);
mp3.playFileByIndexNumber(3);
}
}
void center(){
delay(500);// reading will be taken after ....miliseconds
Serial.println("\n");
int duration, distance;
digitalWrite (center_trigPin, HIGH);
delayMicroseconds (10);
digitalWrite (center_trigPin, LOW);
duration = pulseIn (center_echoPin, HIGH);
distance = (duration/2) / 29.1;
if (distance <30) { // Change the number for long or short distances.
Serial.print("Center Distance");
Serial.print(distance);
mp3.playFileByIndexNumber(1);
}
}
Comments
Seriously it is so important
Seriously it is so important plz reply me plzz
I think there is a problem
I think there is a problem with library version that you are using. Try the below code:
#include
#include
#include
// Create the mp3 module object,
// Arduino Pin 8 is connected to TX of the JQ6500
// Arduino Pin 9 is connected to one end of a 1k resistor,
// the other end of the 1k resistor is connected to RX of the JQ6500
// If your Arduino is 3v3 powered, you can omit the 1k series resistor
SoftwareSerial mySerial(8, 9);
JQ6500_Serial mp3(mySerial);
void setup() {
mySerial.begin(9600);
mp3.reset();
mp3.setVolume(20);
mp3.setLoopMode(MP3_LOOP_ALL);
mp3.play();
}
void loop() {
// Do nothing, it's already playing and looping :-)
}
Sorry, it's not posting the
Sorry, it's not posting the library files. First include the Arduino.h, SoftwareSerial.h, JQ6500_Serial.h libraries with the above code and check if it works or not. If it doesnt work try changing the software serial library version.
not able to upload the code
not able to upload the code to aurdino nano ????
Remove all the connections,
Remove all the connections, then upload the code after that make all connections
I am compile code show error
I am compile code show error please solve it is important for me
Arduino: 1.8.16 (Windows Store 1.8.51.0) (Windows 10), Board: "Arduino Nano, ATmega328P"
sketch_oct14c:2:9: error: #include expects "FILENAME" or <FILENAME>
#include
^
sketch_oct14c:3:9: error: #include expects "FILENAME" or <FILENAME>
#include
^
exit status 1
#include expects "FILENAME" or <FILENAME>
This report would have more information with
"Show verbose output during compilation"
option enabled in File -> Preferences.
When I connect JQ6500 it
When I connect JQ6500 it shown me like a USB drive in File manager and when I click the file Music Download the application opened and I select my require files but when I press Update button nothing it happens and progress bar is not shown any working. Kindly guide me whats wrong with it. Thanks
An error was showed while i am verifying the code please reply with correct code its urgent
error is Shown Below
no matching function for call to 'JQ6500_Serial::JQ6500_Serial(int, int)'
thanks
regrads
vamsi