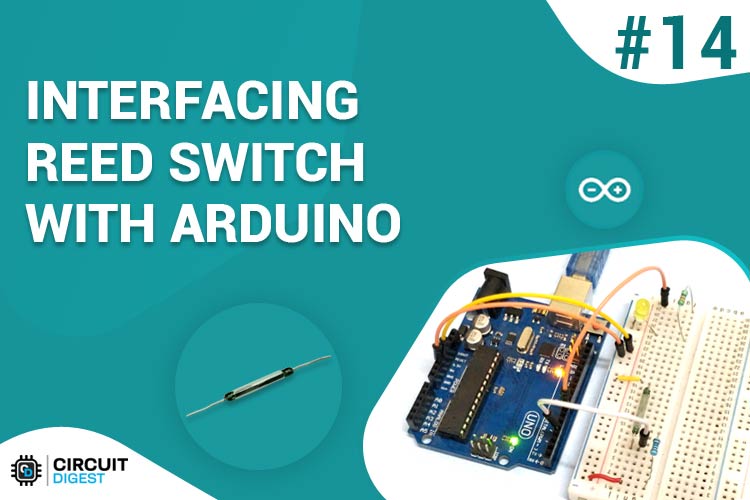
Reed switch is used in many of the real-life applications such as magnetic door switch, laptops, smartphones etc. In this article, we learn about Reed Switch and guide you to Interface a Reed Switch with Arduino.
Reed Switch
Reed switch is basically an electrical switch which is operated when a magnetic field is brought near to it. It was invented by W. B. Ellwood in 1936 at bell laboratories. It is made up of two small metal pieces kept inside a glass tube under vacuum. In a typical reed switch two metal pieces will be made of a ferromagnetic material and covered with rhodium or ruthenium to give them long life. The switch will be activated when there is a presence of magnetic field around the switch.
The glass enclosure of the two metal pieces protect them from dirt, dust and other particles. Reed switch can be operated in any environment such as environment where flammable gas is present or environment where corrosion would affect open switch contacts.
There are two types of reed switch.
- Normally open reed switch
- Normally closed reed switch
In normally open reed switch, switch is open in the absence of magnetic field and it is closed in the presence of magnetic field. Under the presence of magnetic field, two metal contacts inside the glass tube attract each other to make contact.
In normally closed reed switch, switch is closed in the absence of magnetic field and it is open in the presence of magnetic field.
Applications of Reed switch
- Used in telephone exchange
- In laptops to put the screen on sleep if the lid is closed
- Used in window and door sensors in burglar alarm system
Components Required
- Arduino Uno
- Reed switch
- Resistors
- LED
- Magnet
- Connecting wires
Arduino Reed Switch Circuit Diagram
Working of Reed Switch with Arduino
Arduino Uno is a open source microcontroller board based on ATmega328p microcontroller. It has 14 digital pins (out of which 6 pins can be used as PWM outputs), 6 analog inputs, on board voltage regulators etc. Arduino Uno has 32KB of flash memory, 2KB of SRAM and 1KB of EEPROM. It operates at the clock frequency of 16MHz. Arduino Uno supports Serial, I2C, SPI communication for communicating with other devices. The table below shows the technical specification of Arduino Uno.
Microcontroller |
ATmega328p |
Operating voltage |
5V |
Input Voltage |
7-12V (recommended) |
Digital I/O pins |
14 |
Analog pins |
6 |
Flash memory |
32KB |
SRAM |
2KB |
EEPROM |
1KB |
Clock speed |
16MHz |
To interface reed switch with Arduino we need to build a voltage divider circuit as shown in the figure below. Vo is +5V when the switch is open and 0V when the switch is closed. We are using a normally open reed switch in this project. Switch is closed in the presence of magnetic field and it is open in the absence of magnetic field.
Code explanation
The complete code for this Arduino reed switch project is given at the end of this article. The code is split into small meaningful chunks and explained below.
In this part of the code we have to define pins on which Reed switch and LED which is connected to Arduino. Reed switch is connected to digital pin 4 of Arduino and LED is connected to digital pin 7 of Arduino through a current limiting resistor. The variable “reed_status” is used to hold the status of reed switch.
int LED = 7; int reed_switch = 4; int reed_status;
In this part of the code, we have to set status of pins on which LED and reed switch is connected. Pin number 4 is set as input and pin number 7 is set as output.
void setup() { pinMode(LED, OUTPUT); pinMode(reed_switch, INPUT); }
Next, we have to read the status of reed switch. If it is equal to 1, switch is open and LED is turned off. If it is equal to 0, switch is closed and we have to turn on LED. This process is repeated every second. This task is accomplished with this part of the code below.
void loop() { reed_status = digitalRead(reed_switch); if (reed_status == 1) digitalWrite(LED, LOW); else digitalWrite(LED, HIGH); delay(1000); }
So as you have seen its very easy to use Reed Switch with Arduino.
Complete Project Code
int LED = 7;
int reed_switch = 4;
int reed_status;
void setup()
{
pinMode(LED, OUTPUT);
pinMode(reed_switch, INPUT);
}
void loop()
{
reed_status = digitalRead(reed_switch);
if (reed_status == 1)
digitalWrite(LED, LOW);
else
digitalWrite(LED, HIGH);
delay(1000);
}
Comments
This program needs to do a bit more ...
This is a simple program and perhaps a good example of the "basics" for a beginner to start with, but if we just wanted to turn the LED off and on from the switch we really don't need an Arduino to do that. Perhaps you can add some more programming so the reed switch could be the same as used for a burglar alarm and placed on a door and the Arduino could send an email, a text (SMS) to a cell phone or perhaps a Windows text message (broadcast) to a computer to alert that someone opened the door?
Yeah point agreed. But still
Yeah point agreed. But still I think once we interface with Arduino and learn how to read the sensor everything that you state can be done
This tutorial perhaps
This tutorial perhaps represents the first step in a complex system- for example, I'm using a reed switch to activate a custom pattern on a strip of WS2812 LEDs whenever my sliding pantry door is opened. The strip is controlled by an Arduino NANO running the FastLED library, making it easy and fun to write all kinds of custom patterns (every 100th door opening results in a surprise rainbow pattern, for example. :).
interfacing reedswitch with arduino
The schematic of the reedswitch shows a pullup resistor for the digital i/o. This is NOT a voltage divider circuit.
interfacing reedswitch with arduino
You could also use the function internal pullup. There is no need for an external resistor..
how can we reprogram fm microcontroller