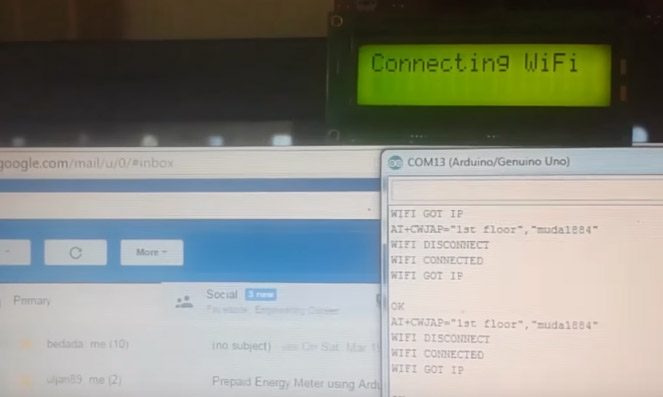
We are moving towards the World of Internet of Things (IoT). This technology plays a very important role in the Electronics and Embedded system. Sending an Email from any Microcontroller or Embedded system is very basic thing, which is required in IoT. So in this article, we will learn “How to send an Email using Wi-Fi and Arduino”.
For sending Email via Wi-Fi module and Arduino, first of all we need to have an Email account. So user can create email account at smtp2go.com. After signup, remember your new email address and password.
You can also learn How to Call and Message using Arduino.
We can understand the whole process in below Steps:
Step 1: First arrange all the required Components.
- Arduino UNO
- ESP8266 Wi-Fi module
- USB Cable
- Laptop
- 16x2 LCD (optional)
- 10K POT (optional)
- Power supply
- Connecting wires
Wi-Fi Module ESP8266:
Step 2: In this step we will connect ESP8266 Wi-Fi module with Arduino and give it power supply from 3.3v pin of Arduino.
Step 3: In this step, we need to sign-up for Email address and Password (smtp2go.com). smtp2go.com provides the email services, to send the emails using outgoing email server.
Step 4: We will need Username and Password in base64 encoded format with utf-8 character set. For converting the Email and Password in base64 encoded format use below given link. Keep the encoded username and password handy, we need it in our program to login at smtp2go.com.
Step 5: Now start writing code for sending Email. ‘Programming Explanation’ and Code is given at the end.
Circuit Explanation:
Circuit is very simple, for this project we only need Arduino and ESP8266 Wi-Fi module. A 16x2 LCD is also connected for displaying the status messages. This LCD is optional. ESP8266’s Vcc and GND pins are directly connected to 3.3V and GND of Arduino and CH_PD is also connected with 3.3V.
Tx and Rx pins of ESP8266 are directly connected to pin 2 and 3 of Arduino. And Pin 2 of Arduino is also shorted with Tx pin (Pin 1) of Arduino. This pin is shorted for displaying response of ESP8266 directly on Serial monitor of Arduino. Software Serial Library is used to allow serial communication on pin 2 and 3 of Arduino.
Note: For watching the response of ESP8266 on Serial Monitor, don’t initialise the Serial.begin(9600) function.
Programming Explanation:
1. In programming part first we include libraries and define pins for LCD & software serial communication. By default Pin 0 and 1 of Arduino are used for serial communication but by using SoftwareSerial library, we can allow serial communication on other digital pins of the Arduino.
2. void connect_wifi(String cmd, int t) Function is defined to Connect the Wi-Fi module to the Internet.
void connect_wifi(String cmd, int t) { int temp=0,i=0; while(1) { Serial1.println(cmd); while(Serial1.available()) { if(Serial1.find("OK")) ... .... .... ... .....
3. After it connect module to SMTP server by using given commands:
lcd.print("Configuring Email.."); Serial1.println("AT+CIPSTART=4,\"TCP\",\"mail.smtp2go.com\",2525"); delay(2000); Serial1.println("AT+CIPSEND=4,20"); delay(2000); Serial1.println("EHLO 192.168.1.123"); delay(2000); Serial1.println("AT+CIPSEND=4,12");
Note: Many email servers will not accept email from a non-commercial, dhcp-issued ip address.
4. Now we will try to Login at smtp2go.co, using the base64 encoded User name and password, which we have derived in Step 4 above.
lcd.print("Try To Login....."); Serial1.println("AUTH LOGIN"); delay(2000); Serial1.println("AT+CIPSEND=4,30"); delay(2000); Serial1.println("c2FkZGFtNDIwMUBnbWFpbC5jb20="); //base64 encoded username delay(2000); Serial1.println("AT+CIPSEND=4,18"); delay(2000); Serial1.println("Y2lyY3VpdDQyMDE="); //base64 encoded password
5. After this, enter your main message that user want to send. And send this email by ending line (sending) ‘.’
Serial1.println("Testing Success"); delay(2000); Serial1.println("AT+CIPSEND=4,3"); delay(2000); Serial1.println('.'); delay(10000); Serial1.println("AT+CIPSEND=4,6"); delay(2000); Serial1.println("QUIT"); ... ..... .......
Other functions and Code are self-explanatory and easy to understand.
Complete Project Code
#include<LiquidCrystal.h>
LiquidCrystal lcd(14,15,16,17,18,19);
#include <SoftwareSerial.h>
SoftwareSerial Serial1(2, 3); // RX, TX
boolean No_IP=false;
String IP="";
void check4IP(int t1)
{
int t2=millis();
Serial1.flush();
while(t2+t1>millis())
{
while(Serial1.available()>0)
{
if(Serial1.find("WIFI GOT IP"))
{
No_IP=true;
}
}
}
}
void get_ip()
{
IP="";
char ch=0;
while(1)
{
Serial1.println("AT+CIFSR");
while(Serial1.available()>0)
{
if(Serial1.find("STAIP,"))
{
delay(1000);
Serial.print("IP Address:");
while(Serial1.available()>0)
{
ch=Serial1.read();
if(ch=='+')
break;
IP+=ch;
}
}
if(ch=='+')
break;
}
if(ch=='+')
break;
delay(1000);
}
Serial.print(IP);
Serial.print("Port:");
Serial.println(80);
}
void connect_wifi(String cmd, int t)
{
int temp=0,i=0;
while(1)
{
Serial1.println(cmd);
while(Serial1.available())
{
if(Serial1.find("OK"))
i=8;
}
delay(t);
if(i>5)
break;
i++;
}
if(i==8)
Serial.println("OK");
else
Serial.println("Error");
}
void setup()
{
Serial1.begin(9600);
// Serial.begin(9600);
lcd.begin(16,2);
lcd.print("Sending Email by");
lcd.setCursor(0,1);
lcd.print(" Arduino & WIFI ");
delay(2000);
lcd.clear();
lcd.print(" Circuit Digest ");
delay(2000);
lcd.clear();
lcd.print("Finding ESP8266");
connect_wifi("AT",100);
connect_wifi("ATE1",100);
lcd.clear();
lcd.print("Connected");
delay(1000);
connect_wifi("AT+CWMODE=3",100);
connect_wifi("AT+CWQAP",100);
connect_wifi("AT+RST",5000);
lcd.clear();
lcd.print("Connecting WiFi");
check4IP(5000);
if(!No_IP)
{
Serial.println("Connecting Wifi....");
connect_wifi("AT+CWJAP=\"1st floor\",\"muda1884\"",7000); //provide your WiFi username and password here
}
else
{
}
lcd.clear();
lcd.print("WIFI Connected...");
Serial.println("Wifi Connected");
delay(1000);
lcd.clear();
lcd.print("Getting IP Add.");
Serial.println("Getting IP Address....");
get_ip();
delay(1000);
lcd.clear();
lcd.print("IP:");
lcd.print(IP);
lcd.setCursor(0,1);
lcd.print("PORT: 80");
connect_wifi("AT+CIPMUX=1",100);
connect_wifi("AT+CIPSERVER=1,80",100);
delay(2000);
lcd.clear();
lcd.print("Configuring Email..");
Serial1.println("AT+CIPSTART=4,\"TCP\",\"mail.smtp2go.com\",2525");
delay(2000);
Serial1.println("AT+CIPSEND=4,20");
delay(2000);
Serial1.println("EHLO 192.168.1.123");
delay(2000);
Serial1.println("AT+CIPSEND=4,12");
delay(2000);
lcd.clear();
lcd.print("Try To Login.....");
Serial1.println("AUTH LOGIN");
delay(2000);
Serial1.println("AT+CIPSEND=4,30");
delay(2000);
Serial1.println("c2FkZGFtNDIwMUBnbWFpbC5jb20="); //base64 encoded username
delay(2000);
Serial1.println("AT+CIPSEND=4,18");
delay(2000);
Serial1.println("Y2lyY3VpdDQyMDE="); //base64 encoded password
lcd.clear();
lcd.print("Login Success");
delay(2000);
Serial1.println("AT+CIPSEND=4,34");
delay(2000);
Serial1.println("MAIL FROM:<saddam4201@ gmail.com>"); // use your email address
delay(2000);
Serial1.println("AT+CIPSEND=4,32");
delay(2000);
lcd.clear();
lcd.print("Seniding Email 2");
lcd.setCursor(0,1);
lcd.print("Saddam4201@ gmail");
Serial1.println("RCPT To:<saddam4201@ gmail.com>");
delay(2000);
Serial1.println("AT+CIPSEND=4,6");
delay(2000);
Serial1.println("DATA");
delay(2000);
Serial1.println("AT+CIPSEND=4,24");
delay(2000);
Serial1.println("Testing Success");
delay(2000);
Serial1.println("AT+CIPSEND=4,3");
delay(2000);
Serial1.println('.');
delay(10000);
Serial1.println("AT+CIPSEND=4,6");
delay(2000);
Serial1.println("QUIT");
delay(2000);
lcd.clear();
lcd.print("Email Sent...");
}
void loop()
{
}
Comments
hello saddam hsmtp3go is very
hello saddam hsmtp3go is very important to open the account sir
What problem are you facing?
What problem are you facing? Please follow the tutorial carefully.
Arduino Uno+ESP8266
Hi,
I am beginner for embedded systems and working on IoT. I have interfaced arduino uno with ESP8266 wifi module and 16*2 LCD. Now i have to type text in textbox of HTML page and hen i click on submit button ,it should get displayed on LCD.
Please reply
Thanks in advance
Hi, my LCD ago marked 'GET
Hi, my LCD ago marked 'GET WIFI IP "and the program runs in a loop by giving me the IP address of the wireless card or something else. Why?
Have you connected to your Wi
Have you connected to your Wi Fi, remember to replace your wifi's username and password, here in the line:
connect_wifi("AT+CWJAP=\"1st floor\",\"muda1884\"",7000);
Hyy Maddy...
Hyy Maddy...
I don't know why code is showing error in the line connect_wifi("AT+CWJAP=\"1st floor\",\"muda1884\"",7000);
Here my code is connect_wifi("Android AP_2704",kavita123); telling "my password" was not declared in this scope.
Please help me out.
Problem
I have this problem and I am not getting correct.
"
[...]
> IPSEND=4,34
busy s...
Recv 18 bytes
SEND OK
+IPD,4,35:535 Incorrect authentication data
MAIL FROM:<fklbonfim@gmail.com>
ERROR
AT+CIPSEND=4,32
OK
> T+CIPSEND=4,6
busy s...
Recv 32 bytes
SEND OK
+IPD,4,26:500 unrecognized command
AT+CIPSEND=4,24
OK
> END=4,3
busy s...
Recv 24 bytes
SEND OK
+IPD,4,26:500 unrecognized command
AT+CIPSEND=4,6
OK
>
Recv 6 bytes
SEND OK
+IPD,4,26:500 unrecognized command"
Hi!!! How did you solve this
Hi!!! How did you solve this problem?
how to watch on the serial monitor?
Hi!
Having fun working this through. I don't have an LCD, and you mention "Note: For watching the response of ESP8266 on Serial Monitor, don’t initialise the Serial.begin(9600) function.". Can someone explain how to do that in the code?
MANY thanks!
Means you should not use
Means you should not use default Rx and Tx pins (0 and 1) of Arduino for serial communication, instead use 2 and 3 pin as Rx Tx using SoftwareSerial.h Library and short the Pin 1 and 2 to get the response on Serial Monitor as done in this tutorial.
Hi,
Hi,
thanks for writing this tutorial. But i got a problem.
Im always stuck on "Getting IP Add."
The eps is visible in my router overview and also got an ip adress.
AT+CIFSR
192.168.4.1
192.168.178.48
OK
but it wont stop asking for a ip adress.
What am i doing wrong?
Thank you sir.. I can confirm
Thank you sir.. I can confirm that this is working. Simple and easy..just follow the steps. I set it up to sent me an email when there is an alarm trigger at home.I wrote the commands in an progmem array and used a for function to call them so they dont use ram.The only problem is that the email applications dont refresh/synchronise on smartphones to often.
Problem
Very goog job ......BUT....
if i give the AT command by hand for the '.' (DOT, for terminate the text of the email) in the serial monitor works and send me an email
BUT if i put this in the code doesn't work see below....please help me thanks a lot
Serial1.println("AT+CIPSEND=3");
delay(2000);
Serial1.println('.');
Looping the same output
OK
AT+CIFSR
192.168.4.1
192.168.1.3
OK
AT+CIFSR
192.168.4.1
192.168.1.3
What should I do??
Trouble Shooting
I get this in my serial monitor:
ón't use rtc mem data
rl�‚slÌÿ
Ai-Thinker Technology Co.,Ltd.
invalid
WIFI CONNECTED
WIFI GOT IP
AT+CWJAP="Redmi","efdfdf"
WIFI DISCONNECT
AT+CWJAP="Redmi","efdfdf"
busy p...
AT+CWJAP="Redmi","efdfdf"
busy p...
+CWJAP:1
FAIL
AT+CWJAP="Redmi","efdfdf"
WIFI CONNECTED
WIFI GOT IP
OK
AT+CWJAP="Redmi","efdfdf"
WIFI DISCONNECT
AT+CIFSR
busy p...
AT+CIFSR
busy p...
AT+CIFSR
busy p...
AT+CIFSR
busy p...
AT+CIFSR
busy p...
+CWJAP:1
FAIL
AT+CIFSR
+CIFSR:APIP,"192.168.4.1"
+CIFSR:APMAC,"1a:fe:34:99:87:c7"
+CIFSR:STAIP,"0.0.0.0"
+CIFSR:STAMAC,"18:fe:34:99:87:c7"
and thereafter just the following text repeatedly, with no more progress.
OK
AT+CIFSR
+CIFSR:APIP,"192.168.4.1"
+CIFSR:APMAC,"1a:fe:34:99:87:c7"
+CIFSR:STAIP,"0.0.0.0"
+CIFSR:STAMAC,"18:fe:34:99:87:c7"
OK
AT+CIFSR
+CIFSR:APIP,"192.168.4.1"
+CIFSR:APMAC,"1a:fe:34:99:87:c7"
+CIFSR:STAIP,"0.0.0.0"
+CIFSR:STAMAC,"18:fe:34:99:87:c7"
...
What should I do?
username and password
hi saddam ,i dont know where i put my username and password in this:
connect_wifi("AT+CWJAP=\"1st floor\",\"muda1884\"",7000);
1st floor is user name and
1st floor is user name and muda1884 is password, replace them with yours.
Program error
I am getting some error in SoftwareSerial Serial1 saying conflicting declaration ''SoftwareSerial Serial1'
I am using arduino mega 2560
help
hello mr saddam
thank for your info
i have tried set the arduino to send distance from ultrasonic sensor to webpage
but at first i would like to check if my ESP8266 coneccted properly
i have used your code but when i run it i got thoese on the computer secree :
AT
AT
AT
AT
AT
AT
AT
ATE1
ATE1
ATE1
ATE1
ATE1
ATE1
ATE1
AT+CWMODE=3
AT+CWMODE=3
AT+CWMODE=3
AT+CWMODE=3
AT+CWMODE=3
AT+CWMODE=3
AT+CWMODE=3
AT+CWQAP
AT+CWQAP
AT+CWQAP
AT+CWQAP
AT+CWQAP
AT+CWQAP
AT+CWQAP
AT+RST
AT+RST
AT+RST
AT+RST
AT+RST
AT+RST
AT+RST
can you please let me know the reason and how to solve this or anyone can guide me on that ?
You may need to adjust the
You may need to adjust the Baud Rate according to your ESP, we have lot projects using ESP with Arduino check here: http://circuitdigest.com/search/node/esp8266%20and%20arduino and learn to interface ESP with Arduino
send email
Hello mr saddam thank for your info
i have this problem
+IPD,4,182:250-mail.smtp2go.com Hello 192.168.1.123 [187.163.212.60]
250-SIZE 52428800
250-8BITMIME
250-DSN
250-PIPELINING
250-AUTH CRAM-MD5 PLAIN LOGIN
250-STARTTLS
250-PRDR
250 HELP
AT+CIPSEND=4,12
OK
>
Recv 12 bytes
SEND OK
+IPD,4,18:334 VXNlcm5hbWU6
AT+CIPSEND=4,30
OK
>
Recv 30 bytes
SEND OK
+IPD,4,18:334 UGFzc3dvcmQ6
AT+CIPSEND=4,18
OK
>
Recv 18 bytes
SEND OK
+IPD,4,30:235 Authentication succeeded
AT+CIPSEND=4,34
OK
> T+CIPSEND=4,32
busy s...
Recv 34 bytes
SEND OK
+IPD,4,8:250 OK
RCPT To:<ggbathory@gmail.com>
ERROR
AT+CIPSEND=4,6
OK
>
Recv 6 bytes
SEND OK
+IPD,4,26:500 unrecognized command
AT+CIPSEND=4,24
OK
> END=4,3
busy s...
Recv 24 bytes
SEND OK
+IPD,4,26:500 unrecognized command
.
ERROR
AT+CIPSEND=4,6
OK
>
Recv 6 bytes
SEND OK
+IPD,4,26:500 unrecognized command
Connection
Sir I found your tutorial very helpful. I have encountered a slight problem. When i send the EHLO command i dont get any response. Is there any need for port forwarding before i make this connection? Or is it something else?
Thank you.
make call using esp8266
hello ,
your privious projects were really awesome, i m done with this project can u please make a tutorial on how to make a call using esp8266.
it will be very helpfull to explore IOT more.
Should I use SMTP user or App
Should I use SMTP user or App user of smtp2go??
I'm using encoded SMTP user but I get auth error:
250-SIZE 52428800
250-8BITMIME
250-DSN
250-PIPELINING
250-AUTH CRAM-MD5 PLAIN LOGIN
250-STARTTLS
250-PRDR
250 HELP
AUTH LOGIN
ERROR
AT+CIPSEND=4,30
Also in Serial1.println("EHLO 192.168.1.123");
What's that IP address?
not obtaining ip
Sending Email by
Arduino & WIFI
Mayank
Finding ESP8266
OK
OK
Connected
OK
OK
OK
Connecting WIFI()
Connecting Wifi....
OK
Wifi Connected
Getting IP Address.
it is not obtaining any ip. plzz help me to fix this
what is the use of shorting
what is the use of shorting serial1 port -2 to serial port 1 i.e port 2 to port 1
esp8266
iam doing a mini project on security system USING(ardino uno , ultrasonic sensor , buzzer, esp8266 wifi module ) (i need to get message to mobile from the urdino uno while somthing is dittected ) how to i procead i completed with decting and now i need to get message any help is usefull
Thanks
got error IP
Hi Mr Maddy..how to fix this?it display
Wifi Connected
ERROR
Getting IP Address....
ERROR
AT+CIFSR
+CIFSR:APIP,"192.168.4.1"
+CIFSR:APMAC,"1a:fe:34:f2:79:5a"
+CIFSR:STAIP,"192.168.43.116"
+CIFSR:STAMAC,"18:fe:34:f2:79:5a"
transform to5100 shield
Would it be possible to make this work for a ethernet shield?
I need it for a greenhouse hobby.
Can you please help me i am a absoluut beginner..
i have looked to change it my self but i am stuck .
Thanks Frank
Two subtle mistakes in the program
delay(2000);
Serial1.println("MAIL FROM:<saddam4201@ gmail.com>"); // there is a space after @
delay(2000);
Serial1.println("AT+CIPSEND=4,32");
delay(2000);
lcd.clear();
lcd.print("Seniding Email 2");
lcd.setCursor(0,1);
lcd.print("Saddam4201@ gmail");
Serial1.println("RCPT To:<saddam4201@ gmail.com>"); // there is a space after @
delay(2000);
Serial1.println("Testing Success");
Your Code works Easy
I just used part of your code to send email from a ATWINC1500 on a Adafruit Feather M0.
it works well. I did not know SMPT protocol or that it would be easy to do until I read your explanation.
get_ip address and !NO_IP
get_ip address is problem not accepting local ip addres
So, I'm getting this
So, I'm getting this
cmlxdWV0aG9tQGdtYWlsLmNvbQ==
ERROR
AT+CIPSEND=4,17
OK
> AT+CIPSEND=4,32
busy s...
Anjd I believe that chains other error messages I get after that. What should I do?
The RCPT TO line is giving me
The RCPT TO line is giving me an error instead of SEND OK, what could that be?
Very good project but now we
Very good project but now we have a full equiped ES8266, no need to have an Arduino UNO.
Anybody has the sketck to send a mail with only ESP8266 ?
Thanks in advance
Regards
Alain
IP addressing
I'm not getting IP addressing, after IP addressing message not getting IP address. So please help me
void get_ip()
{
IP=" ";
char ch=0;
while(1)
{
Serial1.println("AT+CIFSR");
while(Serial1.available()>0)
{
if(Serial1.find("STAIP,"))
{
delay(1000);
Serial.print("IP Address:");
while(Serial1.available()>0)
{
ch=Serial1.read();
if(ch=='+')
break;
IP+=ch;
}
}
if(ch=='+')
break;
}
if(ch=='+')
break;
delay(1000);
}
lcd.clear();
lcd.print(IP);
lcd.setCursor(0,1);
lcd.print("Port: 80");
Serial.print(IP);
Serial.print("Port:");
Serial.println(80);
delay(1000);
}
OUTPUT:
OK
OK
OK
OK
OK
Connecting Wifi....
OK
Wifi Connected
Getting IP Address....
same problem
I to have same problem i to didnt get the ip address,i have same program code
Related to project
Hey I have bolt in which will do module is present so can I use bolt with arudino to make this project if u solve my I am very thankful
Everything is working perfect
Everything is working perfect except sending the mail. Although it is being displayed in the Serial Monitor that the email is sent but it has not.
#include <SoftwareSerial.h>
SoftwareSerial esp8266(6,7); // RX, TX
boolean No_IP=false;
String IP="";
String cmd="",str="";
void check4IP(int t1)
{
int t2=millis();
esp8266.flush();
while(t2+t1>millis())
{
while(esp8266.available()>0)
{
if(esp8266.find("WIFI GOT IP"))
{
No_IP=true;
}
}
}
}
void get_ip()
{
IP="";
char ch=0;
while(1)
{
esp8266.println("AT+CIFSR");
while(esp8266.available()>0)
{
if(esp8266.find("STAIP,"))
{
delay(1000);
Serial.print("IP Address:");
while(esp8266.available()>0)
{
ch=esp8266.read();
if(ch=='+')
break;
IP+=ch;
}
}
if(ch=='+')
break;
}
if(ch=='+')
break;
delay(1000);
}
Serial.print(IP);
Serial.print("Port:");
Serial.println(80);
}
void connect_wifi(String cmd, int t)
{
int temp=0,i=0;
while(1)
{
esp8266.println(cmd);
while(esp8266.available())
{
if(esp8266.find("OK"))
i=8;
}
delay(t);
if(i>5)
break;
i++;
}
if(i==8)
Serial.println("OK");
else
Serial.println("Error");
}
void setup()
{
esp8266.begin(9600);
Serial.begin(9600);
// lcd.begin(16,2);
Serial.println("Sending Email by");
delay(1000);
// lcd.setCursor(0,1);
Serial.println(" Arduino & WIFI ");
delay(2000);
// lcd.clear();
// lcd.print(" Circuit Digest ");
// delay(2000);
// lcd.clear();
Serial.print("Finding ESP8266");
connect_wifi("AT",100);
connect_wifi("ATE1",100);
//lcd.clear();
//lcd.print("Connected");
delay(1000);
connect_wifi("AT+CWMODE=3",100);
connect_wifi("AT+CWQAP",100);
connect_wifi("AT+RST",5000);
//lcd.clear();
Serial.print("Connecting WiFi");
check4IP(5000);
if(!No_IP)
{
Serial.println("Connecting Wifi....");
connect_wifi("AT+CWJAP=\"TP-LINK_PIUMIU\",\"piumiu123#\"",7000); //provide your WiFi username and password here
}
else
{
}
//lcd.clear();
//lcd.print("WIFI Connected...");
Serial.println("Wifi Connected");
delay(1000);
//lcd.clear();
//lcd.print("Getting IP Add.");
Serial.println("Getting IP Address....");
get_ip();
delay(1000);
// lcd.clear();
// lcd.print("IP:");
// lcd.print(IP);
// lcd.setCursor(0,1);
// lcd.print("PORT: 80");
connect_wifi("AT+CIPMUX=1",100);
connect_wifi("AT+CIPSERVER=1,80",100);
delay(2000);
//lcd.clear();
Serial.print("Configuring Email..");
esp8266.println("AT+CIPSTART=4,\"TCP\",\"mail.smtp2go.com\",2525");
delay(2000);
esp8266.println("AT+CIPSEND=4,20");
delay(2000);
esp8266.println("EHLO 192.168.0.104");
delay(2000);
esp8266.println("AT+CIPSEND=4,12");
delay(2000);
//lcd.clear();
Serial.print("Try To Login.....");
esp8266.println("AUTH LOGIN");
delay(2000);
esp8266.println("AT+CIPSEND=4,34");
delay(2000);
esp8266.println("Zm9yZXN0Z3VtcC44M0BnbWFpbC5jb20="); //base64 encoded username
delay(2000);
esp8266.println("AT+CIPSEND=4,18");
delay(2000);
esp8266.println("cGl1bWVvd2FtcmE="); //base64 encoded password
//lcd.clear();
Serial.print("Login Success");
delay(2000);
//str="MAIL FROM:<mishtudeep@gmail.com>";
//cmd = "AT+CIPSEND=4,";
//cmd += String(str.length());
esp8266.println("AT+CIPSEND=4,36");
delay(2000);
esp8266.println("MAIL FROM:<forestgump.83@gmail.com>"); // use your email address
delay(2000);
esp8266.println("AT+CIPSEND=4,32");
delay(2000);
//lcd.clear();
Serial.println("Seniding Email to");
//lcd.setCursor(0,1);
Serial.println("sree060796@gmail.com");
esp8266.println("RCPT To:<sree060796@gmail.com>");
delay(2000);
esp8266.println("AT+CIPSEND=4,6");
delay(2000);
esp8266.println("DATA");
delay(2000);
esp8266.println("AT+CIPSEND=4,17");
delay(2000);
esp8266.println("Testing Success");
delay(2000);
esp8266.println("AT+CIPSEND=4,3");
delay(2000);
esp8266.println('.');
delay(10000);
esp8266.println("AT+CIPSEND=4,6");
delay(2000);
esp8266.println("QUIT");
delay(2000);
//lcd.clear();
Serial.println("Email Sent...");
}
void loop()
{
}
Check if you have entered the
Check if you have entered the correct SSID and password in the program
Email is not received
Hi,
Thanks for this tutoriel
I made an account in smtp2go and I use AT commands to send an email with my arduino uno and my esp8266 module
All steps are successfully done, but when I check my gmail account, I cannot find any email (even in Spams)
Is this issue related to only gmail or there is another problem ?
hi i have done the connection
hi i have done the connection according to ur circuit only and i have added my base encoded paswword and email id but still when i upload the code the initial commands for 9600 bps is not shown in the serial terminal
SMTP2GO not available
when I try to create an account at the SMTP2GO site it says that it is not available in my country..are there any other alternatives which have a very close functionality to this one?
Hi, nice project.
Hi, nice project.
Well, i've been trying to make this work for a few days, but i got this problem. Everything works fine until this point.
+IPD,4,25:501 Invalid base64 data
AT+CIPSEND=4,17
OK
>
Recv 17 bytes
SEND OK
+IPD,4,26:500 unrecognized command
AT+CIPSEND=4,3
OK
>
Recv 3 bytes
SEND OK
+IPD,4,26:500 unrecognized command
AT+CIPSEND=4,6
Do you know what it could be?
Thank you a lot
Hi,
Hi,
I am unable to load the code from arduino ide to esp8266
Sketch uses 232,505 bytes (53%) of program storage space. Maximum is 434,160 bytes.
Global variables use 32,504 bytes (39%) of dynamic memory, leaving 49,416 bytes for local variables. Maximum is 81,920 bytes.
warning: espcomm_sync failed
error: espcomm_open failed
error: espcomm_upload_mem failed
error: espcomm_upload_mem failed
Need URGENT Help, someone can directly contact me on msyukahn94@gmail.com
Please HELP here...
hi syukran. i having the same
hi syukran. i having the same problem too.. have you solved the problem? If yes can you share me how to solve it?
Configure Arduino IDE
Guys you have to prepare the Arduino IDE before you can compile the ESP8266 programs on Arduino IDE.
This tutorial https://circuitdigest.com/microcontroller-projects/programming-esp8266-… help
Can I email a photo in this process?
Thank you so much for such a useful project demonstration.Now,using this exact process,can I email a photo stored in my sd card attached to the arduino board?
If yes,please give related information....Thanks a lot....
No, Arduino is not powerful
No, Arduino is not powerful enough to process pictures
Hi i am everything is working
Hi i am everything is working until this point:
+IPD,4,25:501 Invalid base64 data
MAIL FROM:<jayjames.tyson @gmaill.com>
ERROR
AT+CIPSEND=4,32
what can i do to fix it?
Serial1.println("EHLO 192
Serial1.println("EHLO 192.168.1.123");
What is this IP address ??
IS it network Ip or esp8266 ip?
Hello Saddam,
Thank you for posting this project.
I'm new to the ESP8266 and ARDUINO UNO, Can you please share the step by step procedure followed by you during the project.
Thanks in advance