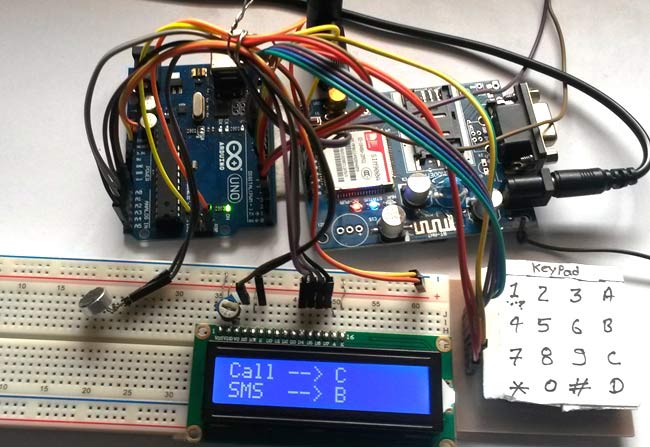
Sometimes people find it difficult to use the GSM Module for its basic functions like calling, texting etc., specifically with the Microcontrollers. So here we are going to build a Simple Mobile Phone using Arduino, in which GSM Module is used to Make the Call, answer the Call, send SMS, and read SMS, and also this Arduino phone has Mic and Speaker to talk over this Phone. This project will also serve as a proper interfacing of GSM Module with Arduino, with all the Code needed to operate any Phone’s basic functions.
Components Required:
- Arduino Uno
- GSM Module SIM900
- 16x2 LCD
- 4x4 Keypad
- Breadboard or PCB
- Connecting jumper wire
- Power supply
- Speaker
- MIC
- SIM Card
Working Explanation:
In this Arduino Mobile Phone Project, we have used Arduino Uno to control whole system’s features and interfacing all the components in this system. A 4x4 Alphanumeric Keypad is used for taking all kind of inputs like: Enter mobile number, type messages, make a call, receive a call, send SMS, read SMS etc. GSM Module is used to communicate with the network for calling and messaging purpose. We have also interfaced a MIC and a Speaker for Voice Call and Ring sound and a 16x2 LCD is used for showing messages, instructions and alerts.
Alphanumeric is a method to enter numbers and alphabets both by using same keypad. In this method, we have interfaced 4x4 keypad with Arduino and written Code for accepting alphabets too, check the Code in Code section below.
Working of this project is easy. All the features will be performed by Using Alphanumeric Keypad. Check the Full code and a Demo Video below to properly understand the process. Here we are going to explain all the four features of the projects below.
Explaining Four Features of Arduino Mobile Phone:
1. Make a Call:
To make a call by using our Arduino based Phone, we have to press ‘C’ and then need to enter the Mobile Number on which we want to make a call. Number will be entered by using alphanumeric keypad. After entering the number we again need to press ‘C’. Now Arduino will process for connecting the call to the entered number by using AT command:
ATDxxxxxxxxxx; <Enter> where xxxxxxxxx is entered Mobile Number.
2. Receive a Call:
Receiving a call is very easy. When someone is calling to your system SIM number, which is there in GSM Module, then your system will show ‘Incoming…’ message over the LCD with incoming number of caller. Now we just need to Press ‘A’ to attend this call. When we press ‘A’, Arduino will send given command to GSM Module:
ATA <enter>
3. Send SMS:
When we want to send a SMS using our Arduino based Mobile Phone, then we need to Press ‘B’. Now System will ask for Recipient Number, means ‘to whom’ we want to send SMS. After entering the number we need to press ‘D’ and now LCD asks for message. Now we need to type the message, like we enter in normal mobile, by using keypad and then after entering the message we need to press ‘D’ to send SMS. To Send SMS Arduino sends given command:
AT+CMGF=1 <enter> AT+CMGS=”xxxxxxxxxx” <enter> where: xxxxxxxxxx is entered mobile number
And send 26 to GSM to send SMS.
4. Receive and Read SMS:
This feature is also simple. In this, GSM will receive SMS and stores it in SIM card. And Arduino continuously monitors the received SMS indication over UART. We just need to Press ‘D’, to read the SMS, when we see the New Message symbol (like a envelope: See the Video at the end) on the LCD. Below is the SMS Received indication displayed on the Serial port is:
+CMTI: “SM” <SMS stored location> +CMTI: “SM”,6 Where 6 is message location where it stored in SIM card.
When Arduino gets this ‘SMS received’ indication then it extracts SMS storing location and sends command to GSM to read the received SMS. And show a ‘New Message Symbol’ over the LCD.
AT+CMGR=<SMS stored location><enter> AT+CMGR=6
Now GSM sends stored message to Arduino and then Arduino extract main SMS and display it over the LCD and then after reading this SMS Arduino Clear the ‘New SMS symbol’ from the LCD.
Note: There is no coding for MIC and Speaker.
Check the Full code and a Demo Video below to properly understand the process.
Circuit Diagram and Explanation:
Circuit Diagram of this for interfacing GSM SIM900 and Arduino is given above. 16x2 LCD pins RS, EN, D4, D5, D6 and D7 are connected with pin number 14, 15, 16, 17, 18 and 19 of Arduino respectively. GSM Module’s Rx and Tx pins are directly connected with Arduino’s pin D3 and D2 respectively (Ground of Arduino and GSM must be connected with each other). 4x4 keypad Row pins R1, R2, R3, R4 are directly linked to pin number 11,10, 9, 8 of Arduino and Colum pins of keypad C1, C2, C3 are linked with pin number 7, 6, 5, 4 of Arduino. MIC is directly connected at mic+ and mic- of GSM Module and Speaker is directly connected at SP+ and SP- pins for GSM Module.
Programming Explanation:
Programming part of this project is little complex for beginners. In this code we have used keypad library #include <Keypad.h> for interfacing simple keypad for entering numbers. And for entering alphabets with the same keypad, we have created function void alfakey(). Means we have made every key multi functioning and we can enter any character or integer by using only 10 keys.
Like if we press key 2 (abc2), it will show ‘a’ and if we presses it again then it will replace ‘a’ to ‘b’ and if again we press three times then it will show ‘c’ at same place in LCD. If we wait for some time after pressing key, cursor will automatic move to next position in LCD. Now we can enter next char or number. The same procedure is applied for other keys.
#include <Keypad.h> const byte ROWS = 4; //four rows const byte COLS = 4; //four columns char hexaKeys[ROWS][COLS] = { {'1','2','3','A'}, {'4','5','6','B'}, {'7','8','9','C'}, {'*','0','#','D'} }; byte rowPins[ROWS] = {11, 10, 9, 8}; //connect to the row pinouts of the keypad byte colPins[COLS] = {7, 6, 5, 4}; //connect to the column pinouts of the keypad //initialize an instance of class NewKeypad Keypad customKeypad = Keypad( makeKeymap(hexaKeys), rowPins, colPins, ROWS, COLS);
void alfakey() { int x=0,y=0; int num=0; while(1) { lcd.cursor(); char key=customKeypad.getKey(); if(key) { if(key=='1') { num=0; lcd.setCursor(x,y); .... ..... ........ ....
Apart from operating keypad, we have created many other functions like void call() for calling feature of Phone, void sms() for messaging feature, void lcd_status() for display LCD status void gsm_init() for initializing the GSM Module etc. Check below all other function related to make & receive Call and send & read SMS using GSM Module and Arduino. All the functions are self-explanatory and understandable.
Complete Project Code
#include <SoftwareSerial.h>
SoftwareSerial Serial1(2, 3); // RX, TX
#include<LiquidCrystal.h>
LiquidCrystal lcd(14,15,16,17,18,19);
byte back[8] =
{
0b00000,
0b00000,
0b11111,
0b10101,
0b11011,
0b11111,
0b00000,
0b00000
};
String number="";
String msg="";
String instr="";
String str_sms="";
String str1="";
int ring=0;
int i=0,temp=0;
int sms_flag=0;
char sms_num[3];
int rec_read=0;
int temp1=0;
#include <Keypad.h>
const byte ROWS = 4; //four rows
const byte COLS = 4; //four columns
char hexaKeys[ROWS][COLS] =
{
{'1','2','3','A'},
{'4','5','6','B'},
{'7','8','9','C'},
{'*','0','#','D'}
};
byte rowPins[ROWS] = {11, 10, 9, 8}; //connect to the row pinouts of the keypad
byte colPins[COLS] = {7, 6, 5, 4}; //connect to the column pinouts of the keypad
//initialize an instance of class NewKeypad
Keypad customKeypad = Keypad( makeKeymap(hexaKeys), rowPins, colPins, ROWS, COLS);
String ch="1,.?!@abc2def3ghi4jkl5mno6pqrs7tuv8wxyz90 ";
void setup()
{
Serial1.begin(9600);
lcd.begin(16,2);
lcd.createChar(1, back);
lcd.print("Simple Mobile ");
lcd.setCursor(0,1);
lcd.print("System Ready..");
delay(1000);
gsm_init();
lcd.clear();
lcd.print("System Ready");
delay(2000);
}
void loop()
{
serialEvent();
if(sms_flag==1)
{
lcd.clear();
lcd.print("New Message");
int ind=instr.indexOf("+CMTI: \"SM\",");
ind+=12;
int k=0;
lcd.setCursor(0,1);
lcd.print(ind);
while(1)
{
while(instr[ind]!= 0x0D)
{
sms_num[k++]=instr[ind++];
}
break;
}
ind=0;
sms_flag=0;
lcd.setCursor(0,1);
lcd.print("Read SMS --> D");
delay(4000);
instr="";
rec_read=1;
temp1=1;
i=0;
}
if(ring == 1)
{
number="";
int loc=instr.indexOf("+CLIP: \"");
if(loc > 0)
{
number+=instr.substring(loc+8,loc+13+8);
}
lcd.setCursor(0,0);
lcd.print("Incomming... ");
lcd.setCursor(0,1);
lcd.print(number);
instr="";
i=0;
}
else
{
serialEvent();
lcd.setCursor(0,0);
lcd.print("Call --> C ");
lcd.setCursor(0,1);
lcd.print("SMS --> B ");
if(rec_read==1)
{
lcd.write(1);
lcd.print(" ");
}
else
lcd.print(" ");
}
char key=customKeypad.getKey();
if(key)
{
if(key== 'A')
{
if(ring==1)
{
Serial1.println("ATA");
delay(5000);
}
}
else if(key=='C')
{
call();
}
else if(key=='B')
{
sms();
}
else if(key == 'D' && temp1==1)
{
rec_read=0;
lcd.clear();
lcd.print("Please wait...");
Serial1.print("AT+CMGR=");
Serial1.println(sms_num);
int sms_read_flag=1;
str_sms="";
while(sms_read_flag)
{
while(Serial1.available()>0)
{
char ch=Serial1.read();
str_sms+=ch;
if(str_sms.indexOf("OK")>0)
{
sms_read_flag=0;
//break;
}
}
}
int l1=str_sms.indexOf("\"\r\n");
int l2=str_sms.indexOf("OK");
String sms=str_sms.substring(l1+3,l2-4);
lcd.clear();
lcd.print(sms);
delay(5000);
}
delay(1000);
}
}
void call()
{
number="";
lcd.clear();
lcd.print("After Enter No.");
lcd.setCursor(0,1);
lcd.print("Press C to Call");
delay(2000);
lcd.clear();
lcd.print("Enter Number:");
lcd.setCursor(0,1);
while(1)
{
serialEvent();
char key=customKeypad.getKey();
if(key)
{
if(key=='C')
{
lcd.clear();
lcd.print("Calling........");
lcd.setCursor(0,1);
lcd.print(number);
Serial1.print("ATD");
Serial1.print(number);
Serial1.println(";");
long stime=millis()+5000;
int ans=1;
while(ans==1)
{
while(Serial1.available()>0)
{
if(Serial1.find("OK"))
{
lcd.clear();
lcd.print("Ringing....");
int l=0;
str1="";
while(ans==1)
{
while(Serial1.available()>0)
{
char ch=Serial1.read();
str1+=ch;
if(str1.indexOf("NO CARRIER")>0)
{
lcd.clear();
lcd.print("Call End");
delay(2000);
ans=0;
return;
}
}
char key=customKeypad.getKey();
if(key == 'D')
{
lcd.clear();
lcd.print("Call End");
delay(2000);
ans=0;
return;
}
if(ans==0)
break;
}
}
}
}
}
else
{
number+=key;
lcd.print(key);
}
}
}
}
void sms()
{
lcd.clear();
lcd.print("Initilising SMS");
Serial1.println("AT+CMGF=1");
delay(400);
lcd.clear();
lcd.print("After Enter No.");
lcd.setCursor(0,1);
lcd.print("Press D ");
delay(2000);
lcd.clear();
lcd.print("Enter Rcpt No.:");
lcd.setCursor(0,1);
Serial1.print("AT+CMGS=\"");
while(1)
{
serialEvent();
char key=customKeypad.getKey();
if(key)
{
if(key=='D')
{
//number+='"';
Serial1.println("\"");
break;
}
else
{
//number+=key;
Serial1.print(key);
lcd.print(key);
}
}
}
lcd.clear();
lcd.print("After Enter MSG ");
lcd.setCursor(0,1);
lcd.print("Press D to Send ");
delay(2000);
lcd.clear();
lcd.print("Enter Your Msg");
delay(1000);
lcd.clear();
lcd.setCursor(0,0);
alfakey();
}
void alfakey()
{
int x=0,y=0;
int num=0;
while(1)
{
lcd.cursor();
char key=customKeypad.getKey();
if(key)
{
if(key=='1')
{
num=0;
lcd.setCursor(x,y);
lcd.print(ch[num]);
for(int i=0;i<3000;i++)
{
lcd.noCursor();
char key=customKeypad.getKey();
if(key=='1')
{
num++;
if(num>5)
num=0;
lcd.setCursor(x,y);
lcd.print(ch[num]);
i=0;
delay(200);
}
}
x++;
if(x>15)
{
x=0;
y++;
y%=2;
}
msg+=ch[num];
}
else if(key=='2')
{
num=6;
lcd.setCursor(x,y);
lcd.print(ch[num]);
for(int i=0;i<3000;i++)
{
lcd.noCursor();
char key=customKeypad.getKey();
if(key=='2')
{
num++;
if(num>9)
num=6;
lcd.setCursor(x,y);
lcd.print(ch[num]);
i=0;
delay(200);
}
}
x++;
if(x>15)
{
x=0;
y++;
y%=2;
}
msg+=ch[num];
}
else if(key=='3')
{
num=10;
lcd.setCursor(x,y);
lcd.print(ch[num]);
for(int i=0;i<3000;i++)
{
lcd.noCursor();
char key=customKeypad.getKey();
if(key=='3')
{
num++;
if(num>13)
num=10;
lcd.setCursor(x,y);
lcd.print(ch[num]);
i=0;
delay(200);
}
}
x++;
if(x>15)
{
x=0;
y++;
y%=2;
}
msg+=ch[num];
}
else if(key=='4')
{
num=14;
lcd.setCursor(x,y);
lcd.print(ch[num]);
for(int i=0;i<3000;i++)
{
lcd.noCursor();
char key=customKeypad.getKey();
if(key=='4')
{
num++;
if(num>17)
num=14;
lcd.setCursor(x,y);
lcd.print(ch[num]);
i=0;
delay(200);
}
}
x++;
if(x>15)
{
x=0;
y++;
y%=2;
}
msg+=ch[num];
}
else if(key=='5')
{
num=18;
lcd.setCursor(x,y);
lcd.print(ch[num]);
for(int i=0;i<3000;i++)
{
lcd.noCursor();
char key=customKeypad.getKey();
if(key=='5')
{
num++;
if(num>21)
num=18;
lcd.setCursor(x,y);
lcd.print(ch[num]);
i=0;
delay(200);
}
}
x++;
if(x>15)
{
x=0;
y++;
y%=2;
}
msg+=ch[num];
}
else if(key=='6')
{
num=22;
lcd.setCursor(x,y);
lcd.print(ch[num]);
for(int i=0;i<3000;i++)
{
lcd.noCursor();
char key=customKeypad.getKey();
if(key=='6')
{
num++;
if(num>25)
num=22;
lcd.setCursor(x,y);
lcd.print(ch[num]);
i=0;
delay(200);
}
}
x++;
if(x>15)
{
x=0;
y++;
y%=2;
}
msg+=ch[num];
}
else if(key=='7')
{
num=26;
lcd.setCursor(x,y);
lcd.print(ch[num]);
for(int i=0;i<3000;i++)
{
lcd.noCursor();
char key=customKeypad.getKey();
if(key=='7')
{
num++;
if(num>30)
num=26;
lcd.setCursor(x,y);
lcd.print(ch[num]);
i=0;
delay(200);
}
}
x++;
if(x>15)
{
x=0;
y++;
y%=2;
}
msg+=ch[num];
}
else if(key=='8')
{
num=31;
lcd.setCursor(x,y);
lcd.print(ch[num]);
for(int i=0;i<3000;i++)
{
lcd.noCursor();
char key=customKeypad.getKey();
if(key=='8')
{
num++;
if(num>34)
num=31;
lcd.setCursor(x,y);
lcd.print(ch[num]);
i=0;
delay(200);
}
}
x++;
if(x>15)
{
x=0;
y++;
y%=2;
}
msg+=ch[num];
}
else if(key=='9')
{
num=35;
lcd.setCursor(x,y);
lcd.print(ch[num]);
for(int i=0;i<3000;i++)
{
lcd.noCursor();
char key=customKeypad.getKey();
if(key=='9')
{
num++;
if(num>39)
num=35;
lcd.setCursor(x,y);
lcd.print(ch[num]);
i=0;
delay(200);
}
}
x++;
if(x>15)
{
x=0;
y++;
y%=2;
}
msg+=ch[num];
}
else if(key=='0')
{
num=40;
lcd.setCursor(x,y);
lcd.print(ch[num]);
for(int i=0;i<3000;i++)
{
lcd.noCursor();
char key=customKeypad.getKey();
if(key=='0')
{
num++;
if(num>41)
num=40;
lcd.setCursor(x,y);
lcd.print(ch[num]);
i=0;
delay(200);
}
}
x++;
if(x>15)
{
x=0;
y++;
y%=2;
}
msg+=ch[num];
}
else if(key=='D')
{
lcd.clear();
lcd.print("Sending SMS....");
// Serial1.print("AT+CMGS=");
// Serial1.print(number);
// delay(2000);
Serial1.print(msg);
Serial1.write(26);
delay(5000);
lcd.clear();
lcd.print("SMS Sent to");
lcd.setCursor(0,1);
lcd.print(number);
delay(2000);
number="";
break;
}
}
}
}
void send_data(String message)
{
Serial1.println(message);
delay(200);
}
void send_sms()
{
Serial1.write(26);
}
void lcd_status()
{
lcd.setCursor(2,1);
lcd.print("Message Sent");
delay(2000);
//lcd.setCursor()
//lcd.print("")
//return;
}
void back_button()
{
//lcd.setCursor(0,15);
}
void ok_button()
{
lcd.setCursor(0,4);
lcd.print("OK");
}
void call_button()
{
lcd.setCursor(0,4);
lcd.print("CALL");
}
void sms_button()
{
lcd.setCursor(0,13);
lcd.print("SMS");
}
void gsm_init()
{
lcd.clear();
lcd.print("Finding Module..");
boolean at_flag=1;
while(at_flag)
{
Serial1.println("AT");
while(Serial1.available()>0)
{
if(Serial1.find("OK"))
at_flag=0;
}
delay(1000);
}
lcd.clear();
lcd.print("Module Connected..");
delay(1000);
lcd.clear();
lcd.print("Disabling ECHO");
boolean echo_flag=1;
while(echo_flag)
{
Serial1.println("ATE1");
while(Serial1.available()>0)
{
if(Serial1.find("OK"))
echo_flag=0;
}
delay(1000);
}
lcd.clear();
lcd.print("Echo OFF");
delay(1000);
lcd.clear();
lcd.print("Finding Network..");
boolean net_flag=1;
while(net_flag)
{
Serial1.println("AT+CPIN?");
while(Serial1.available()>0)
{
if(Serial1.find("+CPIN: READY"))
net_flag=0;
}
delay(1000);
}
lcd.clear();
lcd.print("Network Found..");
delay(1000);
lcd.clear();
}
void serialEvent()
{
while(Serial1.available())
{
char ch=Serial1.read();
instr+=ch;
i++;
if(instr[i-4] == 'R' && instr[i-3] == 'I' && instr[i-2] == 'N' && instr[i-1] == 'G' )
{
ring=1;
}
if(instr.indexOf("NO CARRIER")>=0)
{
ring=0;
i=0;
}
if(instr.indexOf("+CMTI: \"SM\"")>=0)
{
sms_flag=1;
}
}
}
Comments
after initializing the display shows only one message "finding module" and there is no further process being done by the circuit. please give proper solution.
when i compiling the code it show,
error compiling for arduino uno board
what i do sir
what type of speaker u used sir ?
Looks good.
after initializing the display shows only one message "finding module.." and there is no further process being done by the circuit. please give proper solution.
what's next? please
Tell me the command code for displaying messages through LCD via gsm900A...pls rply sir as soon as possible
Actually I made this project all worked properly after wards means after working for a day It stopped working in the sense the LCD is giving garbage value after call -c n message display
dear i need your help as admin is not replying i made this project but unfortunatly it leaves me with finding module kindly help
when i compile it it shows error compiling
Hi sir when i compiling the code it show,
error compiling for arduino uno board
what i do sir
all the functions are working except the sms , i am not recieving the sms frm my mobile to lcd,
void serialEvent()
{
while(Serial1.available())
{
char ch=Serial1.read();
instr+=ch;
i++;
if(instr[i-4] == 'R' && instr[i-3] == 'I' && instr[i-2] == 'N' && instr[i-1] == 'G' )
{
ring=1;
}
if(instr.indexOf("NO CARRIER")>=0)
{
ring=0;
i=0;
}
if(instr.indexOf("+CMTI: \"SM\"")>=0)
{
sms_flag=1;
}
}
}
my serial monitor is not recieving this indication from serial,
please help as soon as possible
My code is showing errors:
'gsm_init() was not declared in this scope'
I need help
hello,
have you find your problem, i have the same but i don't see the solution
hey guys please help me ... My project GSM based intilay GMS modem send me msg but when i send comond to refil my LIquid tank it will not work..
When Gsm send me msg then i send a msg to again gsm it will not accept my msg request
String inputString="";
String num;
int trigPin=52;
int echoPin=53;
long duration,distance;
int motor=8;
int a;
int maxiumRange=50;
String destinationNumber="+923312775616";
void setup() { // void Setup Start
Serial.begin(9600);
pinMode(motor,OUTPUT);
pinMode(trigPin,OUTPUT);
pinMode(echoPin,INPUT);
LCD.begin(16,2);
LCD.setCursor(0,0);
LCD.print("WELCOME");
gsm.begin(9600);
gsm.println("AT+CMGF=1"); //FOR TEXT MODE
gsm.println("AT+CNMI=2,2,0,0,0"); //DIRECT MODE NO NEED TO SAVE INCOMING MESSAGES
delay(2000);
Serial.print("Hello Iam Conneted With Arduino"); //GSM conneted to arduino
delay(2000);
LCD.clear();
LCD.setCursor(0,0);
LCD.print("Liquid Level:");
} //void Setup Close
void loop() { //LOOP start 1
digitalWrite(trigPin,LOW);
delayMicroseconds (2000);
digitalWrite(trigPin,HIGH);
delayMicroseconds (15);
digitalWrite(trigPin,LOW);
delayMicroseconds(10);
duration= pulseIn(echoPin,HIGH);
distance= duration*0.034/2;
LCD.setCursor(0,1);
LCD.print(" ");
LCD.setCursor(0,1);
LCD.print(distance);
LCD.println("=CM");
delay(500);
if (distance >=10){ // if condtion start 1
gsm.print("AT+CMGF=1\r"); // AT command to send SMS message to subsriber
delay(1000);
gsm.println("AT+CMGS= \""+destinationNumber+"\""); //Serial.println("AT + CMGS = \"+923041806419\""); // recipient's mobile number, in international format
delay(1000);
gsm.println("your Tank is Empty Do you Want To refill "); // message to send
delay(1000);
gsm.println((char)26); // End AT command with a ^Z, ASCII code 26
delay(1000);
gsm.println();
delay(10000);
if(gsm.available()){ //Coming MSG from DestionNumber Owner if2
char inChar = gsm.read();
if (inChar =='+') { // if3
num=gsm.readStringUntil(':');
if(num=="CMT"){ //if4
gsm.readStringUntil(',');
gsm.readStringUntil('"');
gsm.readStringUntil('#');
inputString=gsm.readStringUntil('$');
Serial.println(inputString);
if(inputString=="motoron"){ // Motor On code if5
digitalWrite(motor,HIGH);
delay(100);
}
hi, i want to know how many calls at the most can i do with sim900? maybe 100? is posible to do 500 calls?
The number of calls is infinite. As long as you have a valid sim with a valid balance to make calls. You can make calls using the GSM module
i had my compilation but it is showing compilation error if u got output for this project with that code plzz send me the code to my email:
my mail id :[email protected] plzz send me as soon as possible.
How to make gsm based irrigation system using 8051 microcontroller in sms sending programs
You should use the USART of 8051, the below link would be a good place to start with
https://circuitdigest.com/microcontroller-projects/gsm-module-interfaci…
hello , I couldn't find half of the components in Proteus . could you upload your .DNS file or something
The components used in this circuit diagram is self made, so no wonder you dint find them on your machine. Why do you need to re-create the circuit diagram, you cannot simulate it. Just use it as a guidance to build your project
The components used in this circuit diagram is self made, so no wonder you dint find them on your machine. Why do you need to re-create the circuit diagram, you cannot simulate it. Just use it as a guidance to build your project
sir the code does compile in arduino software ,please send me commplete ,copiled code in my email
In sim900 module we insert bsnl sim and given 5V supply, then status and network signal led is blinking. but when we interfaced with the aurdino then the network and status signal is not blinking. what we have check now????
its not a true
tmhary to incoming call py num hi nai thk ,,, mble mien kuch or hai or display py kuch orr
after initializing the display shows only one message "finding module" and there is no further process being done by the circuit. please give proper solution.
it's doing like that when ARDUINO can't communicate with SIM900 over serial. Check the baudrate of the module(by using a serial to USB converter try at 9600 with command "AT" -> you should receive "OK" from the module, if you don't receive "ok" check with baud 115200 which is usually default). After you find out the baud you can change it either in sim module(command "AT+IPR=9600" instead of 9600 you can put the wanted baudrate) either in code(search in code after "9600" and replace it as needed). If the baud it's identic in the module and in the code and still not working check your connections (TX, RX)
the project i have done and is working but the text that i receive i can only view the first characters and not more, i would like to get help to view the whole text when i receive a text more than 16 characters
the project i have done and is working but the text that i receive i can only view the first characters and not more, i would like to get help to view the whole text when i receive a text more than 16 characters
Looks like a strange error the charector that you are getting is it random or the exact 1st charector of your message. Can you show us a sample>?
Thanks i was succesful in troubleshooting the error by adding these line of code
lcd.clear();
lcd.print(sms.substring(16,32));
lcd.setCursor(0,1);
lcd.print(sms.substring(16,32));
delay(5000);
}
now when I send a text like "hello" , i receive text a text i sent before like "maoni" plus which i sent now so i receive "maonihello" can you help me check out the codes
#include <SoftwareSerial.h>
SoftwareSerial Serial1(2, 3); // RX, TX
#include<LiquidCrystal.h>
LiquidCrystal lcd(14,15,16,17,18,19);
byte back[8] =
{
0b00000,
0b00000,
0b11111,
0b10101,
0b11011,
0b11111,
0b00000,
0b00000
};
String number="";
String msg="";
String instr="";
String str_sms="";
String str1="";
int ring=0;
int i=0,temp=0;
int sms_flag=0;
char sms_num[3];
int rec_read=0;
int temp1=0;
#include <Keypad.h>
const byte ROWS = 4; //four rows
const byte COLS = 4; //four columns
char hexaKeys[ROWS][COLS] =
{
{'1','2','3','A'},
{'4','5','6','B'},
{'7','8','9','C'},
{'*','0','#','D'}
};
byte rowPins[ROWS] = {11, 10, 9, 8}; //connect to the row pinouts of the keypad
byte colPins[COLS] = {7, 6, 5, 4}; //connect to the column pinouts of the keypad
//initialize an instance of class NewKeypad
Keypad customKeypad = Keypad( makeKeymap(hexaKeys), rowPins, colPins, ROWS, COLS);
String ch="1,.?!@abc2def3ghi4jkl5mno6pqrs7tuv8wxyz90 ";
void setup()
{
Serial1.begin(9600);
lcd.begin(16,2);
lcd.createChar(1, back);
lcd.print("Simple Mobile ");
lcd.setCursor(0,1);
lcd.print("System Ready..");
delay(1000);
gsm_init();
lcd.clear();
lcd.print("System Ready");
delay(2000);
}
void loop()
{
serialEvent();
if(sms_flag==1)
{
lcd.clear();
lcd.print("New Message");
int ind=instr.indexOf("+CMTI: \"SM\",");
ind+=12;
int k=0;
lcd.setCursor(0,1);
lcd.print(ind);
while(1)
{
while(instr[ind]!= 0x0D)
{
sms_num[k++]=instr[ind++];
}
break;
}
ind=0;
sms_flag=0;
lcd.setCursor(0,1);
lcd.print("Read SMS --> D");
delay(4000);
instr="";
rec_read=1;
temp1=1;
i=0;
}
if(ring == 1)
{
/* number="";
int loc=instr.indexOf("+CLIP: \"");
if(loc > 0)
{
number+=instr.substring(loc+8,loc+13+8);
}
lcd.setCursor(0,0);
lcd.print("Incomming... ");
lcd.setCursor(0,1);
lcd.print(number);
instr="";
i=0;*/
}
else
{
serialEvent();
lcd.setCursor(0,0);
lcd.print("read --> D ");
lcd.setCursor(0,1);
lcd.print("SMS --> B ");
if(rec_read==1)
{
lcd.write(1);
lcd.print(" ");
}
else
lcd.print(" ");
}
char key=customKeypad.getKey();
if(key)
{
if(key== 'A')
{
// if(ring==1)
// {
// Serial1.println("ATA");
// delay(5000);
// }
lcd.setCursor(0, 0);
lcd.print("Key A not in use");
}
else if(key=='C')
{
// call();
lcd.setCursor(0, 0);
lcd.print("Key C not in use");
}
else if(key=='B')
{
sms();
}
else if(key == 'D' && temp1==1)
{
rec_read=0;
lcd.clear();
lcd.print("Please wait...");
Serial1.print("AT+CMGR=");
Serial1.println(sms_num);
int sms_read_flag=1;
str_sms="";
while(sms_read_flag)
{
while(Serial1.available()>0)
{
char ch=Serial1.read();
str_sms+=ch;
if(str_sms.indexOf("OK")>0)
{
sms_read_flag=0;
//break;
}
}
}
int l1=str_sms.indexOf("\"\r\n");
int l2=str_sms.indexOf("OK");
String sms=str_sms.substring(l1+3,l2-4);
lcd.clear();
lcd.print(sms.substring(16,32));
lcd.setCursor(0,1);
lcd.print(sms.substring(16,32));
delay(5000);
}
delay(1000);
}
}
void sms()
{
lcd.clear();
lcd.print("Initilising SMS");
Serial1.println("AT+CMGF=1");
delay(400);
Serial1.print("AT+CMGS=\"+255687166982\"\r");
lcd.clear();
lcd.print("After Enter MSG ");
lcd.setCursor(0,1);
lcd.print("Press D to Send ");
delay(2000);
lcd.clear();
lcd.print("Enter Your Msg");
delay(1000);
lcd.clear();
lcd.setCursor(0,0);
alfakey();
}
void alfakey()
{
int x=0,y=0;
int num=0;
while(1)
{
lcd.cursor();
char key=customKeypad.getKey();
if(key)
{
if(key=='1')
{
num=0;
lcd.setCursor(x,y);
lcd.print(ch[num]);
for(int i=0;i<3000;i++)
{
lcd.noCursor();
char key=customKeypad.getKey();
if(key=='1')
{
num++;
if(num>5)
num=0;
lcd.setCursor(x,y);
lcd.print(ch[num]);
i=0;
delay(200);
}
}
x++;
if(x>15)
{
x=0;
y++;
y%=2;
}
msg+=ch[num];
}
else if(key=='2')
{
num=6;
lcd.setCursor(x,y);
lcd.print(ch[num]);
for(int i=0;i<3000;i++)
{
lcd.noCursor();
char key=customKeypad.getKey();
if(key=='2')
{
num++;
if(num>9)
num=6;
lcd.setCursor(x,y);
lcd.print(ch[num]);
i=0;
delay(200);
}
}
x++;
if(x>15)
{
x=0;
y++;
y%=2;
}
msg+=ch[num];
}
else if(key=='3')
{
num=10;
lcd.setCursor(x,y);
lcd.print(ch[num]);
for(int i=0;i<3000;i++)
{
lcd.noCursor();
char key=customKeypad.getKey();
if(key=='3')
{
num++;
if(num>13)
num=10;
lcd.setCursor(x,y);
lcd.print(ch[num]);
i=0;
delay(200);
}
}
x++;
if(x>15)
{
x=0;
y++;
y%=2;
}
msg+=ch[num];
}
else if(key=='4')
{
num=14;
lcd.setCursor(x,y);
lcd.print(ch[num]);
for(int i=0;i<3000;i++)
{
lcd.noCursor();
char key=customKeypad.getKey();
if(key=='4')
{
num++;
if(num>17)
num=14;
lcd.setCursor(x,y);
lcd.print(ch[num]);
i=0;
delay(200);
}
}
x++;
if(x>15)
{
x=0;
y++;
y%=2;
}
msg+=ch[num];
}
else if(key=='5')
{
num=18;
lcd.setCursor(x,y);
lcd.print(ch[num]);
for(int i=0;i<3000;i++)
{
lcd.noCursor();
char key=customKeypad.getKey();
if(key=='5')
{
num++;
if(num>21)
num=18;
lcd.setCursor(x,y);
lcd.print(ch[num]);
i=0;
delay(200);
}
}
x++;
if(x>15)
{
x=0;
y++;
y%=2;
}
msg+=ch[num];
}
else if(key=='6')
{
num=22;
lcd.setCursor(x,y);
lcd.print(ch[num]);
for(int i=0;i<3000;i++)
{
lcd.noCursor();
char key=customKeypad.getKey();
if(key=='6')
{
num++;
if(num>25)
num=22;
lcd.setCursor(x,y);
lcd.print(ch[num]);
i=0;
delay(200);
}
}
x++;
if(x>15)
{
x=0;
y++;
y%=2;
}
msg+=ch[num];
}
else if(key=='7')
{
num=26;
lcd.setCursor(x,y);
lcd.print(ch[num]);
for(int i=0;i<3000;i++)
{
lcd.noCursor();
char key=customKeypad.getKey();
if(key=='7')
{
num++;
if(num>30)
num=26;
lcd.setCursor(x,y);
lcd.print(ch[num]);
i=0;
delay(200);
}
}
x++;
if(x>15)
{
x=0;
y++;
y%=2;
}
msg+=ch[num];
}
else if(key=='8')
{
num=31;
lcd.setCursor(x,y);
lcd.print(ch[num]);
for(int i=0;i<3000;i++)
{
lcd.noCursor();
char key=customKeypad.getKey();
if(key=='8')
{
num++;
if(num>34)
num=31;
lcd.setCursor(x,y);
lcd.print(ch[num]);
i=0;
delay(200);
}
}
x++;
if(x>15)
{
x=0;
y++;
y%=2;
}
msg+=ch[num];
}
else if(key=='9')
{
num=35;
lcd.setCursor(x,y);
lcd.print(ch[num]);
for(int i=0;i<3000;i++)
{
lcd.noCursor();
char key=customKeypad.getKey();
if(key=='9')
{
num++;
if(num>39)
num=35;
lcd.setCursor(x,y);
lcd.print(ch[num]);
i=0;
delay(200);
}
}
x++;
if(x>15)
{
x=0;
y++;
y%=2;
}
msg+=ch[num];
}
else if(key=='0')
{
num=40;
lcd.setCursor(x,y);
lcd.print(ch[num]);
for(int i=0;i<3000;i++)
{
lcd.noCursor();
char key=customKeypad.getKey();
if(key=='0')
{
num++;
if(num>41)
num=40;
lcd.setCursor(x,y);
lcd.print(ch[num]);
i=0;
delay(200);
}
}
x++;
if(x>15)
{
x=0;
y++;
y%=2;
}
msg+=ch[num];
}
else if(key=='D')
{
lcd.clear();
lcd.print("Sending SMS....");
// Serial1.print("AT+CMGS=");
// Serial1.print(number);
// delay(2000);
Serial1.print(msg);
Serial1.write(26);
delay(5000);
lcd.clear();
lcd.print("SMS Sent to");
lcd.setCursor(0,1);
lcd.print(number);
delay(2000);
number="0687166552";
break;
}
}
}
}
void send_data(String message)
{
Serial1.println(message);
delay(200);
}
void send_sms()
{
Serial1.write(26);
}
void lcd_status()
{
lcd.setCursor(2,1);
lcd.print("Message Sent");
delay(2000);
//lcd.setCursor()
//lcd.print("")
//return;
}
void ok_button()
{
lcd.setCursor(0,4);
lcd.print("OK");
}
void sms_button()
{
lcd.setCursor(0,13);
lcd.print("SMS");
}
void gsm_init()
{
lcd.clear();
lcd.print("Finding Module..");
boolean at_flag=1;
while(at_flag)
{
Serial1.println("AT");
while(Serial1.available()>0)
{
if(Serial1.find("OK"))
at_flag=0;
}
delay(1000);
}
lcd.clear();
lcd.print("Module Connected..");
delay(1000);
lcd.clear();
lcd.print("Disabling ECHO");
boolean echo_flag=1;
while(echo_flag)
{
Serial1.println("ATE1");
while(Serial1.available()>0)
{
if(Serial1.find("OK"))
echo_flag=0;
}
delay(1000);
}
lcd.clear();
lcd.print("Echo OFF");
delay(1000);
lcd.clear();
lcd.print("Finding Network..");
boolean net_flag=1;
while(net_flag)
{
Serial1.println("AT+CPIN?");
while(Serial1.available()>0)
{
if(Serial1.find("+CPIN: READY"))
net_flag=0;
}
delay(1000);
}
lcd.clear();
lcd.print("Network Found..");
delay(1000);
lcd.clear();
}
void serialEvent()
{
while(Serial1.available())
{
char ch=Serial1.read();
instr+=ch;
i++;
if(instr[i-4] == 'R' && instr[i-3] == 'I' && instr[i-2] == 'N' && instr[i-1] == 'G' )
{
ring=1;
}
if(instr.indexOf("NO CARRIER")>=0)
{
ring=0;
i=0;
}
if(instr.indexOf("+CMTI: \"SM\"")>=0)
{
sms_flag=1;
}
}
}
Thanks i was succesful in troubleshooting the error by adding these line of code
lcd.clear();
lcd.print(sms.substring(16,32));
lcd.setCursor(0,1);
lcd.print(sms.substring(16,32));
delay(5000);
}
now when I send a text like "hello" , i receive text a text i sent before like "maoni" plus which i sent now so i receive "maonihello" can you help me check out the codes
#include <SoftwareSerial.h>
SoftwareSerial Serial1(2, 3); // RX, TX
#include<LiquidCrystal.h>
LiquidCrystal lcd(14,15,16,17,18,19);
byte back[8] =
{
0b00000,
0b00000,
0b11111,
0b10101,
0b11011,
0b11111,
0b00000,
0b00000
};
String number="";
String msg="";
String instr="";
String str_sms="";
String str1="";
int ring=0;
int i=0,temp=0;
int sms_flag=0;
char sms_num[3];
int rec_read=0;
int temp1=0;
#include <Keypad.h>
const byte ROWS = 4; //four rows
const byte COLS = 4; //four columns
char hexaKeys[ROWS][COLS] =
{
{'1','2','3','A'},
{'4','5','6','B'},
{'7','8','9','C'},
{'*','0','#','D'}
};
byte rowPins[ROWS] = {11, 10, 9, 8}; //connect to the row pinouts of the keypad
byte colPins[COLS] = {7, 6, 5, 4}; //connect to the column pinouts of the keypad
//initialize an instance of class NewKeypad
Keypad customKeypad = Keypad( makeKeymap(hexaKeys), rowPins, colPins, ROWS, COLS);
String ch="1,.?!@abc2def3ghi4jkl5mno6pqrs7tuv8wxyz90 ";
void setup()
{
Serial1.begin(9600);
lcd.begin(16,2);
lcd.createChar(1, back);
lcd.print("Simple Mobile ");
lcd.setCursor(0,1);
lcd.print("System Ready..");
delay(1000);
gsm_init();
lcd.clear();
lcd.print("System Ready");
delay(2000);
}
void loop()
{
serialEvent();
if(sms_flag==1)
{
lcd.clear();
lcd.print("New Message");
int ind=instr.indexOf("+CMTI: \"SM\",");
ind+=12;
int k=0;
lcd.setCursor(0,1);
lcd.print(ind);
while(1)
{
while(instr[ind]!= 0x0D)
{
sms_num[k++]=instr[ind++];
}
break;
}
ind=0;
sms_flag=0;
lcd.setCursor(0,1);
lcd.print("Read SMS --> D");
delay(4000);
instr="";
rec_read=1;
temp1=1;
i=0;
}
if(ring == 1)
{
/* number="";
int loc=instr.indexOf("+CLIP: \"");
if(loc > 0)
{
number+=instr.substring(loc+8,loc+13+8);
}
lcd.setCursor(0,0);
lcd.print("Incomming... ");
lcd.setCursor(0,1);
lcd.print(number);
instr="";
i=0;*/
}
else
{
serialEvent();
lcd.setCursor(0,0);
lcd.print("read --> D ");
lcd.setCursor(0,1);
lcd.print("SMS --> B ");
if(rec_read==1)
{
lcd.write(1);
lcd.print(" ");
}
else
lcd.print(" ");
}
char key=customKeypad.getKey();
if(key)
{
if(key== 'A')
{
// if(ring==1)
// {
// Serial1.println("ATA");
// delay(5000);
// }
lcd.setCursor(0, 0);
lcd.print("Key A not in use");
}
else if(key=='C')
{
// call();
lcd.setCursor(0, 0);
lcd.print("Key C not in use");
}
else if(key=='B')
{
sms();
}
else if(key == 'D' && temp1==1)
{
rec_read=0;
lcd.clear();
lcd.print("Please wait...");
Serial1.print("AT+CMGR=");
Serial1.println(sms_num);
int sms_read_flag=1;
str_sms="";
while(sms_read_flag)
{
while(Serial1.available()>0)
{
char ch=Serial1.read();
str_sms+=ch;
if(str_sms.indexOf("OK")>0)
{
sms_read_flag=0;
//break;
}
}
}
int l1=str_sms.indexOf("\"\r\n");
int l2=str_sms.indexOf("OK");
String sms=str_sms.substring(l1+3,l2-4);
lcd.clear();
lcd.print(sms.substring(16,32));
lcd.setCursor(0,1);
lcd.print(sms.substring(16,32));
delay(5000);
}
delay(1000);
}
}
void sms()
{
lcd.clear();
lcd.print("Initilising SMS");
Serial1.println("AT+CMGF=1");
delay(400);
Serial1.print("AT+CMGS=\"+255687166982\"\r");
lcd.clear();
lcd.print("After Enter MSG ");
lcd.setCursor(0,1);
lcd.print("Press D to Send ");
delay(2000);
lcd.clear();
lcd.print("Enter Your Msg");
delay(1000);
lcd.clear();
lcd.setCursor(0,0);
alfakey();
}
void alfakey()
{
int x=0,y=0;
int num=0;
while(1)
{
lcd.cursor();
char key=customKeypad.getKey();
if(key)
{
if(key=='1')
{
num=0;
lcd.setCursor(x,y);
lcd.print(ch[num]);
for(int i=0;i<3000;i++)
{
lcd.noCursor();
char key=customKeypad.getKey();
if(key=='1')
{
num++;
if(num>5)
num=0;
lcd.setCursor(x,y);
lcd.print(ch[num]);
i=0;
delay(200);
}
}
x++;
if(x>15)
{
x=0;
y++;
y%=2;
}
msg+=ch[num];
}
else if(key=='2')
{
num=6;
lcd.setCursor(x,y);
lcd.print(ch[num]);
for(int i=0;i<3000;i++)
{
lcd.noCursor();
char key=customKeypad.getKey();
if(key=='2')
{
num++;
if(num>9)
num=6;
lcd.setCursor(x,y);
lcd.print(ch[num]);
i=0;
delay(200);
}
}
x++;
if(x>15)
{
x=0;
y++;
y%=2;
}
msg+=ch[num];
}
else if(key=='3')
{
num=10;
lcd.setCursor(x,y);
lcd.print(ch[num]);
for(int i=0;i<3000;i++)
{
lcd.noCursor();
char key=customKeypad.getKey();
if(key=='3')
{
num++;
if(num>13)
num=10;
lcd.setCursor(x,y);
lcd.print(ch[num]);
i=0;
delay(200);
}
}
x++;
if(x>15)
{
x=0;
y++;
y%=2;
}
msg+=ch[num];
}
else if(key=='4')
{
num=14;
lcd.setCursor(x,y);
lcd.print(ch[num]);
for(int i=0;i<3000;i++)
{
lcd.noCursor();
char key=customKeypad.getKey();
if(key=='4')
{
num++;
if(num>17)
num=14;
lcd.setCursor(x,y);
lcd.print(ch[num]);
i=0;
delay(200);
}
}
x++;
if(x>15)
{
x=0;
y++;
y%=2;
}
msg+=ch[num];
}
else if(key=='5')
{
num=18;
lcd.setCursor(x,y);
lcd.print(ch[num]);
for(int i=0;i<3000;i++)
{
lcd.noCursor();
char key=customKeypad.getKey();
if(key=='5')
{
num++;
if(num>21)
num=18;
lcd.setCursor(x,y);
lcd.print(ch[num]);
i=0;
delay(200);
}
}
x++;
if(x>15)
{
x=0;
y++;
y%=2;
}
msg+=ch[num];
}
else if(key=='6')
{
num=22;
lcd.setCursor(x,y);
lcd.print(ch[num]);
for(int i=0;i<3000;i++)
{
lcd.noCursor();
char key=customKeypad.getKey();
if(key=='6')
{
num++;
if(num>25)
num=22;
lcd.setCursor(x,y);
lcd.print(ch[num]);
i=0;
delay(200);
}
}
x++;
if(x>15)
{
x=0;
y++;
y%=2;
}
msg+=ch[num];
}
else if(key=='7')
{
num=26;
lcd.setCursor(x,y);
lcd.print(ch[num]);
for(int i=0;i<3000;i++)
{
lcd.noCursor();
char key=customKeypad.getKey();
if(key=='7')
{
num++;
if(num>30)
num=26;
lcd.setCursor(x,y);
lcd.print(ch[num]);
i=0;
delay(200);
}
}
x++;
if(x>15)
{
x=0;
y++;
y%=2;
}
msg+=ch[num];
}
else if(key=='8')
{
num=31;
lcd.setCursor(x,y);
lcd.print(ch[num]);
for(int i=0;i<3000;i++)
{
lcd.noCursor();
char key=customKeypad.getKey();
if(key=='8')
{
num++;
if(num>34)
num=31;
lcd.setCursor(x,y);
lcd.print(ch[num]);
i=0;
delay(200);
}
}
x++;
if(x>15)
{
x=0;
y++;
y%=2;
}
msg+=ch[num];
}
else if(key=='9')
{
num=35;
lcd.setCursor(x,y);
lcd.print(ch[num]);
for(int i=0;i<3000;i++)
{
lcd.noCursor();
char key=customKeypad.getKey();
if(key=='9')
{
num++;
if(num>39)
num=35;
lcd.setCursor(x,y);
lcd.print(ch[num]);
i=0;
delay(200);
}
}
x++;
if(x>15)
{
x=0;
y++;
y%=2;
}
msg+=ch[num];
}
else if(key=='0')
{
num=40;
lcd.setCursor(x,y);
lcd.print(ch[num]);
for(int i=0;i<3000;i++)
{
lcd.noCursor();
char key=customKeypad.getKey();
if(key=='0')
{
num++;
if(num>41)
num=40;
lcd.setCursor(x,y);
lcd.print(ch[num]);
i=0;
delay(200);
}
}
x++;
if(x>15)
{
x=0;
y++;
y%=2;
}
msg+=ch[num];
}
else if(key=='D')
{
lcd.clear();
lcd.print("Sending SMS....");
// Serial1.print("AT+CMGS=");
// Serial1.print(number);
// delay(2000);
Serial1.print(msg);
Serial1.write(26);
delay(5000);
lcd.clear();
lcd.print("SMS Sent to");
lcd.setCursor(0,1);
lcd.print(number);
delay(2000);
number="0687166552";
break;
}
}
}
}
void send_data(String message)
{
Serial1.println(message);
delay(200);
}
void send_sms()
{
Serial1.write(26);
}
void lcd_status()
{
lcd.setCursor(2,1);
lcd.print("Message Sent");
delay(2000);
//lcd.setCursor()
//lcd.print("")
//return;
}
void ok_button()
{
lcd.setCursor(0,4);
lcd.print("OK");
}
void sms_button()
{
lcd.setCursor(0,13);
lcd.print("SMS");
}
void gsm_init()
{
lcd.clear();
lcd.print("Finding Module..");
boolean at_flag=1;
while(at_flag)
{
Serial1.println("AT");
while(Serial1.available()>0)
{
if(Serial1.find("OK"))
at_flag=0;
}
delay(1000);
}
lcd.clear();
lcd.print("Module Connected..");
delay(1000);
lcd.clear();
lcd.print("Disabling ECHO");
boolean echo_flag=1;
while(echo_flag)
{
Serial1.println("ATE1");
while(Serial1.available()>0)
{
if(Serial1.find("OK"))
echo_flag=0;
}
delay(1000);
}
lcd.clear();
lcd.print("Echo OFF");
delay(1000);
lcd.clear();
lcd.print("Finding Network..");
boolean net_flag=1;
while(net_flag)
{
Serial1.println("AT+CPIN?");
while(Serial1.available()>0)
{
if(Serial1.find("+CPIN: READY"))
net_flag=0;
}
delay(1000);
}
lcd.clear();
lcd.print("Network Found..");
delay(1000);
lcd.clear();
}
void serialEvent()
{
while(Serial1.available())
{
char ch=Serial1.read();
instr+=ch;
i++;
if(instr[i-4] == 'R' && instr[i-3] == 'I' && instr[i-2] == 'N' && instr[i-1] == 'G' )
{
ring=1;
}
if(instr.indexOf("NO CARRIER")>=0)
{
ring=0;
i=0;
}
if(instr.indexOf("+CMTI: \"SM\"")>=0)
{
sms_flag=1;
}
}
}
I need help doing this project because when i compile it everything is good connect and appears strange caracters on the lcd, please help, email me: [email protected]
can you make a certain button for deleting, like that for case of space?
exit status 1 Keypad.h: No such file or directory
Hi
Calls ok and recieves ok but dosn't seem to hang up on call end from either Party.
Serial1.print("ATH"); needed somewhere ????
only way to end call is hard reset.
Also would be nice to have a back space (del) key in case of mistake.
Am i missing somthing ? may have better look through code.
Arduino: 1.6.4 (Windows 8.1), Board: "Arduino Duemilanove or Diecimila, ATmega328"
mobilefull2.ino: In function 'void setup()':
mobilefull2:51: error: 'gsm_init' was not declared in this scope
mobilefull2.ino: In function 'void loop()':
mobilefull2:58: error: 'serialEvent' was not declared in this scope
mobilefull2:135: error: 'sms' was not declared in this scope
mobilefull2.ino: In function 'void call()':
mobilefull2:183: error: 'serialEvent' was not declared in this scope
'gsm_init' was not declared in this scope
mobilefull2.ino: In function 'void setup()':
mobilefull2:51: error: 'gsm_init' was not declared in this scope
mobilefull2.ino: In function 'void loop()':
mobilefull2:58: error: 'serialEvent' was not declared in this scope
mobilefull2:135: error: 'sms' was not declared in this scope
mobilefull2.ino: In function 'void call()':
mobilefull2:183: error: 'serialEvent' was not declared in this scope
'gsm_init' was not declared in this scope
This report would have more information with
"Show verbose output during compilation"
enabled in File > Preferences.