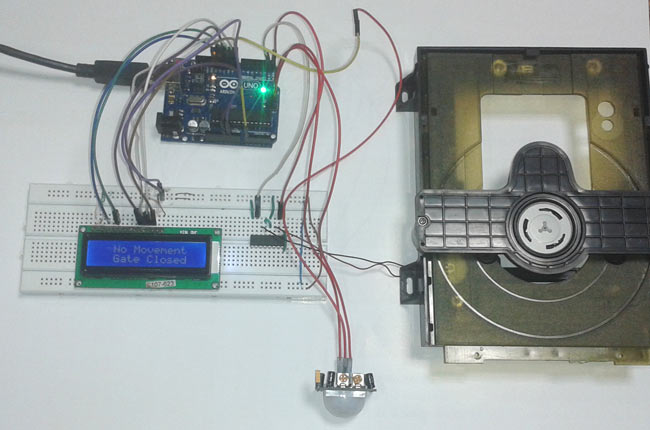
You must have seen automatic door openers in shopping malls and other commercial buildings. They open the door when someone comes near the entrance and close it after sometime. A number of technologies are available to make such kinds of systems like PIR sensors, Radar sensors, Laser sensors, Infrared sensors, etc. In this arduino based project, we have tried to replicate the same system by using a PIR sensor.
It uses a motion-detecting sensor (PIR sensor) to open or close the door which detects the infrared energy omitted from human's body. When someone comes in front of the door, the infrared energy detected by the sensor changes and it triggers the sensor to open the door whenever someone approaches the door. The signal is further sent to arduino uno that controls the door.
Circuit Components
- Arduino UNO
- 16x2 LCD
- PIR Sensor
- Connecting wires
- Bread board
- 1 k resistor
- Power supply
- Motor driver
- CD case (DVD Troly)
PIR Sensor
PIR sensor detects any change in heat, and whenever it detects any change, its output PIN becomes HIGH. They are also referred as Pyroelectric or IR motion sensors.
Here we should note that every object emits some amount of infrared when heated. Human also emits infrared because of body heat. PIR sensors can detect small amount of variation in infrared. Whenever an object passes through the sensor range, it produces infrared because of the friction between air and object, and get caught by PIR.
The main component of PIR sensor is Pyroelectric sensor shown in figure (rectangular crystal behind the plastic cap). Along with BISS0001 ("Micro Power PIR Motion Detector IC"), some resistors, capacitors and other components used to build PIR sensor. BISS0001 IC take the input from sensor and does processing to make the output pin HIGH or LOW accordingly.
Pyroelectric sensor divide in two halves, when there is no motion, both halves remain in same state, means both senses the same level of infrared. As soon as somebody enters in first half, the infrared level of one half becomes greater than other, and this causes PIRs to react and makes the output pin high.
Pyroelectric sensor is covered by a plastic cap, which has array of many Fresnel Lens inside. These lenses are curved in such a manner so that sensor can cover a wide range.
Circuit Diagram and Explanation
Connections for arduino based door opener circuit are shown in the above diagram. Here a PIR sensor is used for sensing human motion which has three terminals Vcc, GND and Dout. Dout is directly connected to pin number 14 (A0) of arduino uno. A 16x2 LCD is used for displaying the status. RS, EN pins of LCD connected to 13 and 12 of arduino and data pins D0-D7 are connected to arduino digital pin numbers 11, 10, 9, 8. RW is directly connected to ground. L293D motor driver is connected to arduino pin 0 and 1 for opening and closing the gate. Here in circuit we have used a motor for gate.
Programming Explanation
The concept used here for programming is very simple. In program we have only used digital input output.
DigitalRead is used for reading output of PIR sensor.
After that, if PIR sensor senses any motion then program sends an command to open gate, stop gate, closing gate and stop gate.
See below the complete code for arduino based automatic door opener.
Complete Project Code
#include <LiquidCrystal.h>
LiquidCrystal lcd(13, 12, 11, 10, 9, 8);
#define PIR_sensor 14
#define m11 0
#define m12 1
void setup()
{
lcd.begin(16, 2);
pinMode(m11, OUTPUT);
pinMode(m12, OUTPUT);
pinMode(PIR_sensor, INPUT);
lcd.print(" Automatic ");
lcd.setCursor(0,1);
lcd.print(" Door Opener ");
delay(3000);
lcd.clear();
lcd.print("CIRCUIT DEGEST ");
delay(2000);
}
void loop()
{
if(digitalRead(PIR_sensor))
{
lcd.setCursor(0,0);
lcd.print("Movement Detected");
lcd.setCursor(0, 1);
lcd.print(" Gate Opened ");
digitalWrite(m11, HIGH); // gate opening
digitalWrite(m12, LOW);
delay(1000);
digitalWrite(m11, LOW); // gate stop for a while
digitalWrite(m12, LOW);
delay(1000);
lcd.clear();
lcd.print(" Gate Closed ");
digitalWrite(m11, LOW); // gate closing
digitalWrite(m12, HIGH);
delay(1000);
digitalWrite(m11, LOW); // gate closed
digitalWrite(m12, LOW);
delay(1000);
}
else
{
lcd.setCursor(0,0);
lcd.print(" No Movement ");
lcd.setCursor(0,1);
lcd.print(" Gate Closed ");
digitalWrite(m11, LOW);
digitalWrite(m12, LOW);
}
}
Comments
it is very simple project
it is very simple project .pls dont use aurdino board .
don't do ur projects only based on concept
just try to do with compactness and simple cost ,reliabled one .
I want make it so I require
I want make it so I require help from it...
Great job. I want to do this
Great job. I want to do this same project, so my questions are what programming language did you used and how were you able to put the code inside the ardino uno. I would really appreciate your help and guidance. Thanks.
In order to write the code ,
In order to write the code , you need to download arduino onto your computer . Go to the arduino official website and download it . then copy the code .
Arduino simply supports C/C++
Arduino simply supports C/C++ language, and Arduino language itself derived from C/C++. Means it supports all the C++'s OOPS concept, operators, conditions statements etc. And there is a Arduino IDE, which is used to verify and upload the code to the Arduino, you just need to connect Arduino board to the PC using USB. Arduino IDE (Arduino Nightly) can be downloaded from here: https://www.arduino.cc/en/Main/Software
can we use ir sensor instead
can we use ir sensor instead of pir sensor??
These are just the variable
These are just the variable name, used to define PIN 0 and 1 of Arduino, you can use any name.
check the code in void_loop()
check the code in void_loop() function, motor rotate in one direction to Open the gate and then rotate in another direction to Close the gate.
what type of ic use in this project please tell me
what type of ic use in this project please tell me
What motor did you use?
Could you please enlarge a little on the type of motor you used? Geared, stepper, servo, etc., and also its specifications?
How can I connectthe L293D on
How can I connectthe L293D on the motor? My dvd troly is from an actual dvd and I cant figure out how to connect it because it has 4 pins
You need to find out the two
You need to find out the two wires, which are connected to rotating motor of DVD tray.
If I use ultrasonic sensor
If I use ultrasonic sensor instead of PIR sensor,which parts should I change of the program? Also could you tell the kind of the motor used?
check this for Ultrasonic
check this for Ultrasonic sensor interfacing: Arduino Based Distance Measurement using Ultrasonic Sensor
LCD only displaying black boxes
I tried all possible solutions. Nothing works :(
As tharun said nothing works
As tharun said nothing works for now. Same program,same proteus connections,same materials,but there is a mistake which I do not understand. There is power on the LCD,but the sentences are not seen. Motor rotates almost independent from the PIR sensor :/ ıf there is a extra code or something else,please uniform us
my pir sensor is getting high
my pir sensor is getting high no matter how far i go from it...it is written somewhere in the internet that the range of a pir sensor is 15 - 20 feet..can you please tell me what to do...
Learn about PIR sensor and
Learn about PIR sensor and how to adjust its distance range here : PIR Sensor
hello.can u plz tell me how
hello.can u plz tell me how to connect a 12v dc motor to it using relay
You can check our Home
You can check our Home automation projects to Interface Relay with Arduino, then connect DC motor to Relay.
what is the function of the
what is the function of the ICSP pin in arduino uno
We have used DVD Trolley from
We have used DVD Trolley from the computer here, but you can use simple DC motor, attached to gate. You can choose the DC Motor accordingly and need to provide the power supply accordingly.
actually i am thinking of a
actually i am thinking of a project where i am using barrier instead of door and when vehicle will come nearer then pir sensor will work n open up the barrier. now i want to achieve linear motion in vertical plane (as of a bollard) can u guide how i can achieve it. i would appreciate ur help....
I want to add a counter for
I want to add a counter for how many people are enter /out in the door? is it possible in this project? how? plz let me know if its possible
Check this Project :
Check this Project : Automatic Room Light Controller with Bidirectional Visitor Counter
TX keeps flashing
I've done this project left out the LCD added a motor driver and servo motor but can't get it to run the TX keeps flashing for some reason any ideas
What dother I do
I can't get this to work at all
I don't have the screen attached sour tried with the code the same and changed to delete it limes of code related to screen
Doesn't work
I changed motors I added a stepper motor
Changed code again to include stepper code still don't work
Changed it all back again and it runs full sequence with out the regard for timing
You should use simple DC
You should use simple DC motors with the given code, servo and stepper wont work with this code. Also adjust the sensitivity of PIR sensor.
about simulation software
dear brother i want to try this project simulation on proteus but i did't find some equipment like arduino uno,pir sensor u use in this simulation
You need to install Libraries
You need to install Libraries for them in Proteus.
Connection with motor or Cd drive !
How i connect wire and motor with CD drive ?
CD Drive itself has the DC
CD Drive itself has the DC motor, you don't need to connect any additional motor. You need to find connections to this CD drive motor.
If I connect with dc motor
If I connect with dc motor and battery,should I change the code?,and what's power supply use?
This project already using a
This project already using a 9v Battery, and you can take power supply for motor from Arduino (connected to computer) or a 9v battery should work.
can i replace cd case with
can i replace cd case with the door(build manually) that connected with dc motor? is the code still the same?
Yes, the code will remain the
Yes, the code will remain the same.
can i know how to design
can i know how to design correctly this circuit diagram by using proteus..
Circuit images
Can u please upload or mail me he actual circuit images from different angles ... since i am new to this and cant do it by the graphical circuit diagram ... and i have seen a few differences in the first pic and the one in the video... please help
pir sensor
just a quick question i'm not sure if anyone asked this but what about using 2 pir sensors
Does arduino work for high moters
I am making an door open close system for a real gate which works on high voltage moters do arduino is capable of that much of volt at list a fans moter
You can use Relay to trigger
You can use Relay to trigger High voltage AC motors. Learn about relay here.
I didn't get how you connect
I didn't get how you connect the cd rom with the motor driver...please let me know the connection of cd drive to motor driver
you need to find only two
you need to find only two wires which leads to CD drives Motor.
Sir I made this project but
Sir I made this project but the sensor is not working. Door automatically opens after some delay. Plz solve this issue I've tried every possible solution.
power supplly
sir where should we give power supply.. like 9v battery so that i can carry to my clg
What can i do to the code to
What can i do to the code to stop the door from opening and closing when the sensor is still detecting?
I am interested about this
I am interested about this project.But i have a small doubt about this ,I can't find a perfect schematic on this project.could you guys help me out
Schematic is already given
Schematic is already given the in Article, please check.
Double Sensor
Can I connect 2 sensors to the same pins and still perform as intended?
This is so that the door can open from both sides.
thanks
Mechanical technology
whats the name of small device in right side of lcd in breed board....
good project
i wanna try this project ...may you send the circuit diagram
about project and connections
hello,
we are using PIR sensor, Arduino, cd disc drive for this project.will you please, help me how to connect because I tried it with LCD but couldn't complete this project.
bluetooth sensor
instead of PIR sensor, we will use bluetooth sensor because we will make a face detection application and then we will have to connect it with bluetooth. any suggestions?
Wow nice idea, I think it is
Wow nice idea, I think it is very much possible! But, what will you use to do face detection? If I were you i would use openCV with Arduino thorugh serial COM. How are you thinking to do it.
problems with pir sensing stability
in my project the the pir is not getting stable, the error occurs with pir is its sensitivity,in my case i have used arduino code to run pir but the pir is continious sensing the motion even when there is no movement ahead of the pir.
How i can remove this erro??????
The problem could either be
The problem could either be with the module or code. So check the module separelty by using an LED to it output pin and check if it is working properly then proceed with arduino.
for code in c language
i want the code of this project in c language.
WHY my Motor keep moving non stop ?
please help me my motor keep move clockwise and anticlockwise non-stop is this code problem ?
i want to implement this on
i want to implement this on LABVIEW plz suggest me..
which DC motor is connected
which DC motor is connected (voltage)....???
I think its a normal motor
I think its a normal motor and it operates in +5V as shown in the circuit diagram
Project is very efficient...
Project is very efficient....can you please tell me what cd disk have u used ....can we get that piece online
If I change pir sensor to ir
If I change pir sensor to ir sensor.does code same????
how can we use if we want
how can we use if we want connect with servomotor in order to interface hydraulic sysytem
Servo motors can be easily
Servo motors can be easily interfaced with Arduino. You can refer the link below
https://circuitdigest.com/microcontroller-projects/arduino-servo-motor-…
Sir what is the power source
Sir what is the power source which We give hear if it is a battery what is the rating of battery
hello, i was wondering where
hello, i was wondering where did you connect your power supply ?
Just power your arduino with
Just power your arduino with the programming cable and the whole project will be powered.
If you want to use a battery then use a 9V battery with its negative to ground and positive to Vin.
motor speed
How can i lower the speed of my dc motor, and reduce the time of the motor spinning
thanks :)