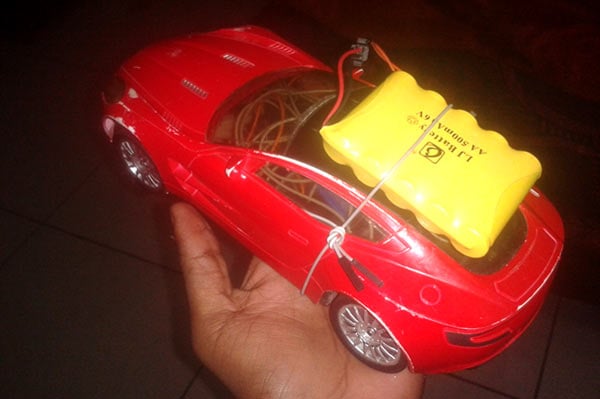
After developing few popular robotic projects like line follower robot, edge avoiding robot, DTMF robot, gesture controlled robot, etc. in this project we are going to develop a bluetooth controlled robo car. Here we used a Bluetooth module to control the car, and it is also an android based application.
Components
- Arduino UNO
- DC Motors
- Bluetooth module HC-05
- Motor Driver L293D
- 9 Volt Battery and 6 volt battery
- Battery Connector
- Toy Car
Bluetooth controlled car is controlled by using Android mobile phone instead of any other method like buttons, gesture etc. Here only needs to touch button in android phone to control the car in forward, backwardd, left and right directions. So here android phone is used as transmitting device and Bluetooth module placed in car is used as receiver. Android phone will transmit command using its in-built Bluetooth to car so that it can move in the required direction like moving forward, reverse, turning left, turning right and stop.
Bluetooth Module
HC Bluetooth module consists two things one is Bluetooth serial interface module and a Bluetooth adaptor. Bluetooth serial module is used for converting serial port to Bluetooth.
How to operate Bluetooth module?
You can directly use the Bluetooth module after purchasing from market, because there is no need to change any setting of Bluetooth module. Default baud rate of new Bluetooth module is 9600 bps. You just need to connect rx and tx to controller or serial converter and give 5 volt dc regulated power supply to module.
Bluetooth module has two modes one is master mode and second one is slave mode. User can set either mode by using some AT commands. Even user can set module’s setting by using AT command. Here is some commands uses are given:
First of all user need to enter AT mode with 38400 bps baud rate by pressing EN button at Bluetooth module or by giving HIGH level at EN pin. Note: all commands should ends with \r\n (0x0d and 0x0a) or ENTER KEY from keyboard.
After it if you send AT to module then module will respond with OK
AT → Test Command
AT+ROLE=0 → Slave Mode select
AT+ROLE=1 → Master Mode select
AT+NAME=xyz → Set Bluetooth Name
AT+PSWD=xyz → Set Password
AT+UART=<value1>,<value2>,<value3> → set Baud rate
Eg. AT+UART=9600,0,0
Pin Description of accelerometer
- STATE → Open
- Rx → Serial receiving pin
- Tx → Serial transmitting pin
- GND → ground
- Vcc → +5volt dc
- EN → to enter in AT mode
Working Explanation
In this project we have used a toy car for demonstration. Here we have selected a RF toy car with moving left right steering feature. After buying this car we have replaced its RF circuit with our Arduino circuit. This car have two dc motors at its front and rear side. Front side motor is used for giving direction to car means turning left or right side (like real car steering feature). And rear side motor is used for driving the car in forward and backward direction. A Bluetooth module is used to receive command from android phone and Arduino UNO is used for controlling the whole system.
Bluetooth controlled car moves according to button touched in the android Bluetooth mobile app. To run this project first we need to download Bluetooth app form Google play store. We can use any Bluetooth app that supporting or can send data. Here are some apps' name that might work correctly.
- Bluetooth Spp pro
- Bluetooth controller
After installing app you need to open it and then search Bluetooth device and select desired Bluetooth device. And then configure keys. Here in this project we have used Bluetooth controller app.
- Download and install Bluetooth Controller.
- Turned ON mobile Bluetooth.
- Now open Bluetooth controller app
- Press scan
- Select desired Bluetooth device
- Now set keys by pressing set buttons on screen. To set keys we need to press ‘set button’ and set key according to picture given below:
After setting keys press ok.
When we touch forward button in Bluetooth controller app then car start moving in forward direction and moving continues forward until next command comes.
When we touch backward button in Bluetooth controller app then car start moving in reverse direction and moving continues reverse until next command comes.
When we touch left button in Bluetooth controller app then car start moving in left direction and moving continues left until next command comes. In this condition front side motor turns front side wheels in left direction and rear motor runs in forward direction.
When we touch right button in Bluetooth controller app then car start moving in right direction and moving continues right until next command comes. In this condition front side motor turns front side wheels in right direction and rear motor runs in forward direction.
And by touching stop button we can stop the car.
Circuit Diagram and Explanation
Circuit diagram for bluetooth controlled car is shown in above figure. A Motor driver is connected to arduino to run the car. Motor driver’s input pins 2, 7, 10 and 15 are connected to arduino's digital pin number 12, 11, 10 and 9 respectively. Here we have used two DC motors to driver car in which one motor is connected at output pin of motor driver 3 and 6 and another motor is connected at 11 and 14. A 6 volt Battery is also used to power the motor driver for driving motors. Bluetooth module’s rx and tx pins are directly connected at tx and rx of Arduino. And vcc and ground pin of Bluetooth module is connected at +5 volt and gnd of Arduino. And a 9 volt battery is used for power the circuit at Arduino’s Vin pin
Program Explanation
In program first of all we have defined output pins for motors.
#define m11 11 // rear motor #define m12 12 #define m21 10 // front motor #define m22 9
And then in setup, we gave directions to pin.
void setup() { Serial.begin(9600); pinMode(m11, OUTPUT); pinMode(m12, OUTPUT); pinMode(m21, OUTPUT); pinMode(m22, OUTPUT); }
After this we read input by using serial communication form Bluetooth module and perform the operation accordingly.
void loop() { while(Serial.available()) { char ch=Serial.read(); str[i++]=ch; if(str[i-1]=='1') { Serial.println("Forward"); forward(); i=0; } else if(str[i-1]=='2') { Serial.println("Left"); right(); i=0; } else if(str[i-1]=='3') { Serial.println("Right"); left(); i=0; }
Then we have created functions for different directions of car. There are five conditions for this Bluetooth controlled car which are used to give the directions:
Touched button in Bluetooth controller app |
Output for front side motor to give direction |
Output for rear side motor to move forward or reverse direction |
|||
Button |
M11 |
M12 |
M21 |
M22 |
Direction |
Stop |
0 |
0 |
0 |
0 |
Stop |
Forward |
0 |
0 |
0 |
1 |
Forward |
Backward |
0 |
0 |
1 |
0 |
Backward |
Right |
1 |
0 |
0 |
1 |
Right |
left |
0 |
1 |
0 |
1 |
Left |
Complete Project Code
#define m11 11 // rear motor
#define m12 12
#define m21 10 // front motor
#define m22 9
char str[2],i;
void forward()
{
digitalWrite(m11, LOW);
digitalWrite(m12, LOW);
digitalWrite(m21, HIGH);
digitalWrite(m22, LOW);
}
void backward()
{
digitalWrite(m11, LOW);
digitalWrite(m12, LOW);
digitalWrite(m21, LOW);
digitalWrite(m22, HIGH);
}
void left()
{
digitalWrite(m11, HIGH);
digitalWrite(m12, LOW);
delay(100);
digitalWrite(m21, HIGH);
digitalWrite(m22, LOW);
}
void right()
{
digitalWrite(m11, LOW);
digitalWrite(m12, HIGH);
delay(100);
digitalWrite(m21, HIGH);
digitalWrite(m22, LOW);
}
void Stop()
{
digitalWrite(m11, LOW);
digitalWrite(m12, LOW);
digitalWrite(m21, LOW);
digitalWrite(m22, LOW);
}
void setup()
{
Serial.begin(9600);
pinMode(m11, OUTPUT);
pinMode(m12, OUTPUT);
pinMode(m21, OUTPUT);
pinMode(m22, OUTPUT);
}
void loop()
{
while(Serial.available())
{
char ch=Serial.read();
str[i++]=ch;
if(str[i-1]=='1')
{
Serial.println("Forward");
forward();
i=0;
}
else if(str[i-1]=='2')
{
Serial.println("Left");
right();
i=0;
}
else if(str[i-1]=='3')
{
Serial.println("Right");
left();
i=0;
}
else if(str[i-1]=='4')
{
Serial.println("Backward");
backward();
i=0;
}
else if(str[i-1]=='5')
{
Serial.println("Stop");
Stop();
i=0;
}
delay(100);
}
}
Comments
Not working
well i tried it but the car is not moving.. the module is connected and the app is running but the car is not moving.. please help me
nice guys , your web is good
nice guys , your web is good to learn for me
ardunio robot
Nice work. I want help. I go to make ardunio Bluetooth control robot of 2 DC motor so plz give me a code for it. Plz help me. Thanking u
Significance of the Study
what is the significance of the study and what is the physics behind it?
i gave 12 v power supply to
i gave 12 v power supply to arduino and l293d separately but not working help plzz
You can use one 12v power
You can use one 12v power supply to power both the arduino and motors, but I think power is not the problem in your circuit.
possible solution
Have you made the ground common by connecting the negative supply of both the battery with the ground pin of arduino????
Bluetooth module car
Can i use l298n motor driver ic in your circuit. Is it working?
yes you can, but l298 is used
yes you can, but l298 is used where we need to draw high current (2A) and thats why we need heat sink with it.
information and query
SIR,
i use L298D for this circuit is it okay?
i give 9V supply to both Arduino and L293D is it okay?
And most important thing is please Provide me the whole and complete code to run this car.
the BT app require any programming?
please reply me as soon as possible
Can i use wifi module in
Can i use wifi module in place of bluetooth
code not able to upload
after connecting bluetooth n arduino RX n TX pins resp the program is not uploading what to do
regard to interfacing of blutooth module
I follow your program but all commands are not working correctly what should i do for that
robot maker
Sir! How shud I use the servo motor to make the connection of arm with the same app controller?
bluetooth controlled car with obstacle avoidance ability
Hey There,
I was wondering if you could give me more details on the project and perhaps offer suggestions on how to add an obstacle avoidance capability... I was thinking of interfacing the system with a proximity sensor designed to alert the user and stop the wheels. Any suggestions?
Hi there
Hi there
the the module is converted to AT command mode and responding the AT commands but it is still not working in the project the app detects the module but the motors are not turning on I have used 12v power for the arduino and for dc motors but it is not working the module connects to a device the led blinks after 2 sec of intervals I am using 16x2 LCD to show that the module is detected or not. I have edit the LCD prints in void forward,backward,left,right and stop I have edit the LCD prints in void setup and in void loop I have edit two prints in void loop() below is the prints code in void loop()
void loop()
{
lcd.begin(16,2);
while(Serial.available())
lcd.setCursor(0,0);
lcd.print("Connected");
delay(1000);
{
char ch=Serial.read();
lcd.clear();
lcd.setCursor(0,0);
lcd.print("Responding");
}
the first print is displaying but the second print is not displaying.
please reply me soon as possible
hi, i have problem in verifying
Hi, in verifying the code, i have a message that it is , After serial println left it tells me this ' right' was not declared in this scope
Please provide type proper
Please provide type proper code.It has so many Errors.
wrong code
in the above code, the forward function makes only one wheel to move.that makes the body of the car to turn on to left or right side but doesn't move front ....please correct the code
Blueetooth module
We have connected all the circuits code has been dumped.But the car is not moving
Should we give any commands or coding to the bluetooth module?
should we give any code for the app? Suggest me the best app
Did your pair your Bluetooth
Did your pair your Bluetooth with Arduino?
application of this project
would you please inform me about the application of that project I had made this but I want to known about its application kindly mention this
Bluetooth controlled robot using arduino
Sir ...I need a Whole Documentation and format about this Project (Bluetooth controlled robot using arduino)....I'm not Getting No idea What to put in document and what to eliminate ...plz sir help me
I want to know about directing finite range
Sir...please give me the way..how can I control the robo car such a way that(i.e 10 m forward,2m left,65 degree angle and etc)
nice