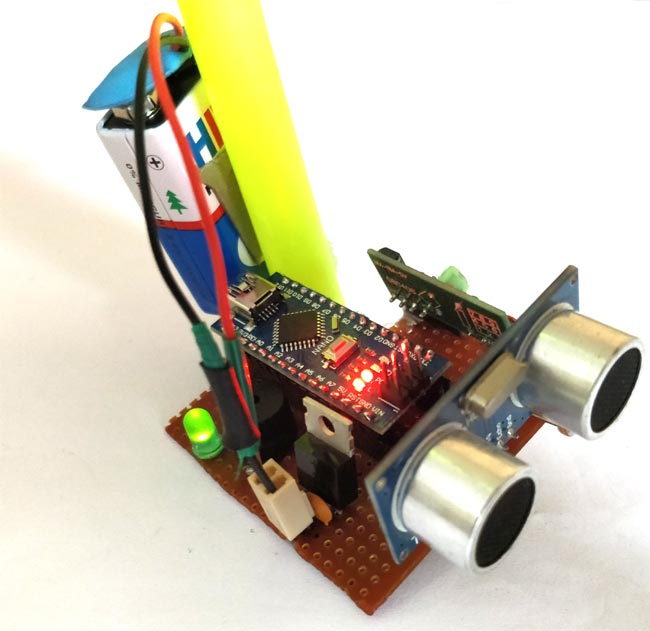
Ever heard of Hugh Herr? He is a famous American rock climber who has shattered the limitations of his disabilities; he is a strong believer that technology could help disabled persons to live a normal life. In one of his TED talk Herr said “Humans are not disabled. A person can never be broken. Our built environment, our technologies, is broken and disabled. We the people need not accept our limitations, but can transfer disability through technological Innovation”. These were not just words but he lived his life to them, today he uses Prosthetic legs and claims to live to normal life. So yes, technology can indeed neutralize human disability; with this in mind let us use some simple devlopment boards and sensors to build an ultrasonic blind walking stick using Arduino that could perform more than just a stick for visually impaired persons.
This Smart stick will have an Ultrasonic sensor to sense distance from any obstacle, LDR to sense lighting conditions and a RF remote using which the blind man could remotely locate his stick. All the feedbacks will be given to the blind man through a Buzzer. Of course you can use a vibrator motor in place of Buzzer and advance a lot more using your creativity. If you want a simple easy to build version of this blindstick you can also check out our Smart Blind Stick project report where we have used only a nano board and ultrasonic sensor without any RF modules.
Materials Required:
- Arduino Nano (Any version will work)
- Ultrasonic Sensor HC-SR04
- LDR
- Buzzer and LED
- 7805
- 433MHz RF transmitter and receiver
- Resistors
- Capacitors
- Push button
- Perf board
- Soldering Kit
- 9V batteries
You can buy all the required components for this smart blind stick project from here.
Blind Stick Circuit Diagram:
This Arduino Smart Blind Stick Project requires two separate circuits. One is the main circuit which will be mounted on the blind man’s stick. The other is a small remote RF transmitter circuit which will be used to locate the main circuit. The main board’s circuit diagram to build a blind stick using ultrasonic sensor is shown below:
As we can see an Arduino Nano is used to control all the sensors, but you can also build this Smart blind stick using arduino uno but following the same pinouts and program. The complete board is powered by a 9V battery which is regulated to +5V using a 7805 Voltage regulator. The Ultrasonic sensor is powered by 5V and the trigger and Echo pin is connected to Arduino nano pin 3 and 2 as shown above. The LDR is connected with a resistor of value 10K to form a Potential divider and the difference in voltage is read by Arduino ADC pin A1. The ADC pin A0 is used to read the signal from RF receiver. The output of the board is given by the Buzzer which is connected to pin 12.
The RF remote circuit is shown below. Its working is also further explained.
I have used a small hack to make this RF remote control circuit to work. Normally while using this 433 MHz RF module requires an Encoder and Decoder or two MCU to work, like in our previous RF Transmitter and Receiver circuit we used the HT12D and HT12E, decoder and encoder IC respectively. But, in our application we just need the receiver to detect if the transmitter is sending some signals. So the Data pin of the transmitter is connected to Ground or Vcc of the supply.
The data pin of the receiver is passed through an RC filter and then given to the Arduino as shown below. Now, whenever the button is pressed the Receiver output some constant ADC value repeatedly. This repetition cannot be observed when the button is not pressed. So we write the Arduino program to check for repeated values to detect if the button is pressed. So that is how a Blind person can track his stick. You can check here: how RF transmitter and receiver work.
I have used a perf board to solder all the connections so that it gets intact with the stick. But, you can also make them on a breadboard. These are the boards that I made for this blind stick project using arduino.
Arduino Program for Smart Blind Stick:
Once we are ready with our hardware, we can connect the Arduino to our Computer and start programming. The complete code used for this page can be found at the bottom of this page, you can upload it directly to your Arduino board. However, if you are curious to know how the code works read further.
Like all programs we start with void setup() to initialise Input Output pins. In our program the Buzzer and Trigger pin is an Output device and the Echo pin is an Input device. We also initialise the serial monitor for debugging.
void setup() { Serial.begin(9600); pinMode(Buzz,OUTPUT); digitalWrite(Buzz,LOW); pinMode(trigger, OUTPUT); pinMode(echo, INPUT); }
Inside the main loop we are reading all the sensors data. We begin with reading the sensor data of Ultrasonic sensor for distance, LDR for light intensity and RF signal to check if the button is pressed. All these data is saved in a variable as shown below for future use.
calculate_distance(trigger,echo); Signal = analogRead(Remote); Intens = analogRead(Light);
We start with checking for the Remote signal. We use a variable called similar_count to check how many times the same values are being repeated from the RF receiver. This repetition will occur only when the button is pressed. So we trigger the Remote pressed alarm if the count exceeds a value of 100.
//Check if Remote is pressed int temp = analogRead(Remote); similar_count=0; while (Signal==temp) { Signal = analogRead(Remote); similar_count++; } //If remote pressed if (similar_count<100) { Serial.print(similar_count); Serial.println("Remote Pressed"); digitalWrite(Buzz,HIGH);delay(3000);digitalWrite(Buzz,LOW); }
You can also check it on Serial Monitor on your computer:
Next we check for the intensity of light around the blind man. If the LDR gives a value of less than 200 it is assumed to be very dark and we give him the warning through buzzer with a specific tone of delay with 200ms. If the intensity is very bright that is more than 800 then also we give a warning with another tone. The alarm tone and intensity can be easily varied by changing the respective value in the below code.
//If very dark if (Intens<200) { Serial.print(Intens); Serial.println("Bright Light"); digitalWrite(Buzz,HIGH);delay(200);digitalWrite(Buzz,LOW);delay(200);digitalWrite(Buzz,HIGH);delay(200);digitalWrite(Buzz,LOW);delay(200); delay(500); } //If very bright if (Intens>800) { Serial.print(Intens); Serial.println("Low Light"); digitalWrite(Buzz,HIGH);delay(500);digitalWrite(Buzz,LOW);delay(500);digitalWrite(Buzz,HIGH);delay(500);digitalWrite(Buzz,LOW);delay(500); }
Finally, we start measuring the distance from any obstacle. There will be no alarm if the measured distance is more than 50cm. But, if it is less than 50cm the alarm will start by beeping the buzzer. As the object gets closer to the buzzer the beeping interval will also decrease. The closer the object is the faster the buzzer will beep. This can be done by creating a delay that is proportional to the distance measured. Since the delay () in Arduino cannot accept variables we have to use a for loop which loop based on the measured distance as shown below.
if (dist<50) { Serial.print(dist); Serial.println("Object Alert"); digitalWrite(Buzz,HIGH); for (int i=dist; i>0; i--) delay(10); digitalWrite(Buzz,LOW); for (int i=dist; i>0; i--) delay(10); }
Learn more about measuring the distance using Ultrasonic sensor and Arduino.
The program can be easily adapted for your application by changing the value which we use to compare. You use the serial monitor to debug if a false alarm is trigger. If you have any problem you can use the comment section below to post your questions
Arduino Blind Stick in Action:
Finally it’s time to test our blind stick arduino project. Make sure the connections are done as per the circuit diagram and the program is successfully uploaded. Now, power both the circuits using a 9V battery and you should start to see results. Move the Ultra Sonic sensor closer to object and you will notice the Buzzer beeping and this beeping frequency increases as the stick goes closer to object. If the LDR is covered in dark or if there is too much light the buzzer will beep. If everything is normal the buzzer will not beep.
When you press the button on the remote the buzzer will give a long beep. The complete working of this Smart Stick for the blind using Arduino is shown in the Video given at the end of this page. I also use a small stick to mount the complete assembly you can use a larger one or an actual blind stick and put it in action.
If your buzzer is always beeping it means the alarm is being false triggered. You can open the serial monitor to check for the parameters and check which is falling in critical and adjust that. As always you can post your problem in the comment section to get help. Hope you understood the project and enjoyed building something.
Complete Project Code
/*
* Program for Blind Man Stick
* Code by B.Aswinth Raj
* Dated: 03-11-2017
* Website: www.circuitdigest.com
*/
const int trigger = 3; //Trigger pin of 1st Sesnor
const int echo = 2; //Echo pin of 1st Sesnor
const int Buzz = 13; //Echo pin of 1st Sesnor
const int Remote = A0; //Echo pin of 1st Sesnor
const int Light = A1; //Echo pin of 1st Sesnor
long time_taken;
int dist;
int Signal;
int Intens;
int similar_count;
void setup() {
Serial.begin(9600);
pinMode(Buzz,OUTPUT);
digitalWrite(Buzz,LOW);
pinMode(trigger, OUTPUT);
pinMode(echo, INPUT);
}
/*###Function to calculate distance###*/
void calculate_distance(int trigger, int echo)
{
digitalWrite(trigger, LOW);
delayMicroseconds(2);
digitalWrite(trigger, HIGH);
delayMicroseconds(10);
digitalWrite(trigger, LOW);
time_taken = pulseIn(echo, HIGH);
dist= time_taken*0.034/2;
if (dist>300)
dist=300;
}
void loop() { //infinite loopy
calculate_distance(trigger,echo);
Signal = analogRead(Remote);
Intens = analogRead(Light);
//Check if Remote is pressed
int temp = analogRead(Remote);
similar_count=0;
while (Signal==temp)
{
Signal = analogRead(Remote);
similar_count++;
}
//If remote pressed
if (similar_count<100)
{
Serial.print(similar_count); Serial.println("Remote Pressed");
digitalWrite(Buzz,HIGH);delay(3000);digitalWrite(Buzz,LOW);
}
//If very dark
if (Intens<200)
{
Serial.print(Intens); Serial.println("Bright Light");
digitalWrite(Buzz,HIGH);delay(200);digitalWrite(Buzz,LOW);delay(200);digitalWrite(Buzz,HIGH);delay(200);
digitalWrite(Buzz,LOW);delay(200);
delay(500);
}
//If very bright
if (Intens>800)
{
Serial.print(Intens); Serial.println("Low Light");
digitalWrite(Buzz,HIGH);delay(500);digitalWrite(Buzz,LOW);delay(500);digitalWrite(Buzz,HIGH);delay(500);
digitalWrite(Buzz,LOW);delay(500);
}
if (dist<50)
{
Serial.print(dist); Serial.println("Object Alert");
digitalWrite(Buzz,HIGH);
for (int i=dist; i>0; i--)
delay(10);
digitalWrite(Buzz,LOW);
for (int i=dist; i>0; i--)
delay(10);
}
//Serial.print("dist=");
//Serial.println(dist);
//Serial.print("Similar_count=");
//Serial.println(similar_count);
//Serial.print("Intens=");
//Serial.println(Intens);
}
Comments
Having the same issue
I was also having the same issue , did you resolve it somehow?
I have the same problem.
I have the same problem. Buzzer is beeping and serial monitor show " Remote pressed " even if I am doing nothing.
May someone help me?
Alter your code to remove
Alter your code to remove remote option. Check if everything else is working. Then tweak your RC values to get the Remote working properly
We removed the remote option
We removed the remote option but the problem is same .
It’s still beeping. Can you give the alter code.
Hi Areeba!
Hi Areeba!
What is your problem? If You remove remote control option, everything - according to the diagram - work correctly. Just remember to change the code from D13 to D12 to which the buzzer is connected.
const int trigPin=3;
const int trigPin=3;
const int echoPin=2;
const int motorPin=7;
const int buzzerPin=12;
long time_taken;
int dist;
int Signal;
int Intens;
int similar_count;
void calculate_distance(int trigPin, int echoPin)
{
Serial.begin(9600);
digitalWrite(trigPin, LOW);
delayMicroseconds(2);
digitalWrite(trigPin, HIGH);
delayMicroseconds(10);
digitalWrite(trigPin, LOW);
time_taken = pulseIn(echoPin, HIGH);
dist= time_taken*0.034/2;
if (dist>300)
dist=300;
}
void setup()
{
pinMode(buzzerPin,OUTPUT);
pinMode(trigPin, OUTPUT);
pinMode(echoPin, INPUT);
pinMode(motorPin, OUTPUT);
digitalWrite(buzzerPin,LOW);
}
void loop()
{
calculate_distance(trigPin,echoPin);
if (dist<50)
{
Serial.print(dist); Serial.println("Object Alert");
digitalWrite(buzzerPin,HIGH);
for (int i=dist; i>0; i--)
delay(10);
digitalWrite(buzzerPin,LOW);
for (int i=dist; i>0; i--)
delay(10);
}
}
Changed from d13 to d12.I used this code in my arduino uno. Its still beeping.
RC values to get remote working
What exact RC values has to be connected for proper working of remote. It is being detected continuously.
continous beeping and wrong output
can u send me the codes without rf receiver
or the corrected code
pls send codes as soon as possible
Im facing the same problem
Im facing the same problem the buzzer is beeping itself.. Have u figured out the problem?? If so please help me.
Yes it does, but you should
Yes it does, but you should change the trigger point values since it will change based on your hardware
Sensors used to help blind persons
I want codings about to give instructions to a blind person in such a way that it tells move right there is a object in front of you and take left there is a tree in front of you.And suggest name for that project.
Circuit issue
Sir, sorry to disturb you but,the receiver and transmitter sections of this smart blind stick on which I'm working on made me to do this,as .. I'm done with proper dumping of code u mentioned in this page and all connection stuff but I'm getting output
What problem are you facing?
What problem are you facing? What is your Serial monitor Outputing?
Where can I find rf receiver
Where can I find rf receiver in proteus ??? By which name ??
We want to use wifi in our
We want to use wifi in our project.whenever obstacle is found then it should be detected by circuit. when wifi chip recieves signals then phone should vibrate. can any one help please.....
can we use a speaker of
can we use a speaker of instead buzzer that speak the blind person to go whether left or right
Regrading project
I want all the components values (like resistor aridino ect... Plz any 1 helps me
Gourish the resistor values
Gourish the resistor values are mentioned in the circuit diagram above
smart blind stick using aurdino
How can we use speaker replace of buzzer or vibrator they can giva a instruction to a person.....
Just remove the buzzer and
Just remove the buzzer and replace it with a vibrator. It will vibrate every time the buzzer made sound.
If you want to make pre-defined patterns in vibration you have to change the code, which is also very easy
RC values to get remote working
Can anyone give the exact RC values for operation of remote.
You can either calculate the
You can either calculate the value of RC which is heptic or experiment your own. A value of 5.7k and 0.1uF would be a good value to start with
RC values of rf module
Hi Aisha! The project is working well without adding the remote code in it. Could you please suggest some values for RC. Or should I make some changes in the code to make the remote work?
Can I use aurdiuno uno rather
Can I use aurdiuno uno rather than the aurdiuno Nano if yes what changes have to done.
Smart blind stick using arduino
Why is the blind man using a remote-control to make buzzer sound in the receiver? I presume the receiver with the ultrasonic is hanging on his chest for example. What is the purpose of signaling by remote control?
My remote is not working
My remote is not working please help me
Where we have to write the
Where we have to write the code ??
How is it working since it is not connected to pc or laptop ?
Can anyone tell which circuit
Can anyone tell which circuit designing software I should use to draw and run the circuit please
You cannot simulate this
You cannot simulate this circuit completely , here the software Proteus is used
am Livus from RWANDA
am Livus from RWANDA
can anyone plz help me to make the code of remote control work because the buzzer is always beeping and the serial monitor display that the remote is pressed and similar_count value is always 0.
The code given here cannot
The code given here cannot support the circuit..overall it ws a bad experience referring to this.
Nano me programme work nhi Kar rha h
Sir Aapne jase bataya h wase hi mane thik thik connection kare h par Aapka programme work nhi Kar rha h ultra sonic sensor or nano board bilkul thik h mane nano or ultra sonic sensor 2 pec manga rakhe h sir isme koi liabray to nhi add Karni padegi plz reply bsir
Need Help
Hey Good Morning i just wanted to ask some question about the circuit could you please at least give a tutorial how did you solder the pins and to be specific could you please a tutorial make a full tutorial how did you created it. Please it's very urgent sorry to tell this but we cannot leave or were not going to graduate if we don't solve or created this robot thank you so much i hope you receive this.
distance program is not working
sir, i want to ask why its always say 0object alert, even though i already put an object very very close to the ultrasonic sensor?? please reply sir, its urgent, thank you.
Regarding Arduino
Through Arduino Uno implement same project with same code?
sir i have a problem on the
sir i have a problem on the output. it keep on displaying object alert even though i don't put any object below 50cm. and it doesn't also beep. please help me solve this problem sir. thank you
It is because the ultrasonic
It is because the ultrasonic sensor is not working properly. Try using the serial monitor to check what values your us sensor is giving
verify problem
Sketch uses 3914 bytes (12%) of program storage space. Maximum is 32256 bytes.
Global variables use 246 bytes (12%) of dynamic memory, leaving 1802 bytes for local variables. Maximum is 2048 bytes
Smart stick for blind person
Is this used for detecting living things also.. or if not wht type of sensor or any thing we can used for it..???
The sensor used here is
The sensor used here is ultrasonic sensor, it will detect ever obstacle including living beings
doubt regrding smart blind stick
sir , i want to add google navigations or google maps to the smart blind stick is it possible to add .if it is can u help the code .
Where we connect the led with
Where we connect the led with this circuit
Hello sir can you help with
Hello sir can you help with the Remote Pressed Issue, Buzzer keeps beeping even if im doing nothing. Thanks
Open the serial monitor and
Open the serial monitor and check what actually makes the Buzzer to beep. If it is the RF Remote problem try disabling it in the program and check. I think is it better to use Encoder and Decoder on both sides of the RF modules to make it reliable
Smart Blind Stick
Hi is anyone can sned me the complete picture of the circuit? I am very new and I am confused why there is a capacitor in the circuit diagram while in the list of components is not. Please help me. I need this for my project. Thank you
I mean another way of wiring
I mean another way of wiring the circuit diagram that can be easily understood to a beginner like me. Thanks I really need your help asap.
const int trigPin=3;
const int trigPin=3;
const int echoPin=2;
const int motorPin=7;
const int buzzerPin=12;
long time_taken;
int dist;
int Signal;
int Intens;
int similar_count;
void calculate_distance(int trigPin, int echoPin)
{
Serial.begin(9600);
digitalWrite(trigPin, LOW);
delayMicroseconds(2);
digitalWrite(trigPin, HIGH);
delayMicroseconds(10);
digitalWrite(trigPin, LOW);
time_taken = pulseIn(echoPin, HIGH);
dist= time_taken*0.034/2;
if (dist>300)
dist=300;
}
void setup()
{
pinMode(buzzerPin,OUTPUT);
pinMode(trigPin, OUTPUT);
pinMode(echoPin, INPUT);
pinMode(motorPin, OUTPUT);
digitalWrite(buzzerPin,LOW);
}
void loop()
{
calculate_distance(trigPin,echoPin);
if (dist<50)
{
Serial.print(dist); Serial.println("Object Alert");
digitalWrite(buzzerPin,HIGH);
for (int i=dist; i>0; i--)
delay(10);
digitalWrite(buzzerPin,LOW);
for (int i=dist; i>0; i--)
delay(10);
}
}
I used this code in my arduino uno. The buzzer is still beeping. What should i do?
What is the purpose of buzzer
What is the purpose of buzzer beep for very bright ldr detection. I understand low light intensity to warn of night .
though to s blind man both affect nothing . As he can't see.
the buzzer is always beeping and the serial monitor is like that
object alert
remote pressed
bright light
and its keep like this. what's the problem can you help me please?
and i am using an UNO arduino