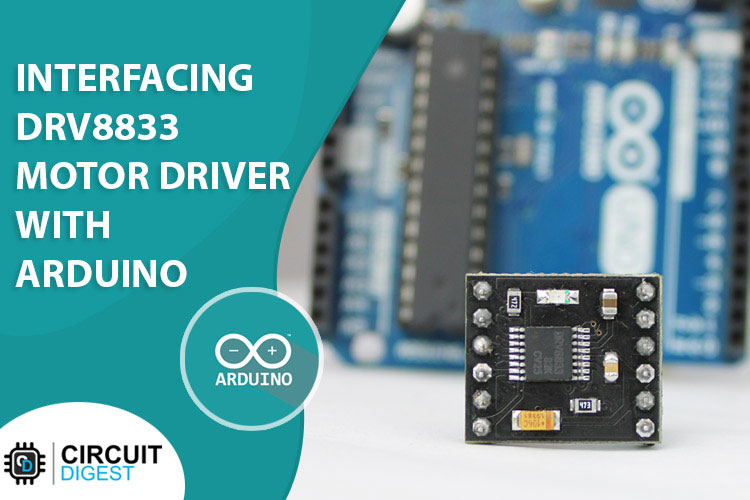
If you are interested in building robots then it is certain that you need to learn how to control the speed and direction of a DC motor, and in one of our previous tutorials, we did just that using the popular L293D Motor Driver IC and ESP32. But there exists another IC named DRV8833 that also provides dual drive motor driver solution and it's cheaper than the L293D IC. So, in this project, we will be Interfacing the DRV8833 motor driver IC with Arduino and in the way, we will know all the details about this IC. Here we have used Arduino Pro Mini, however any Arduino model can be used to built this project.
Controlling DC motors with Microcontrollers
In order to get complete control of the motor we need to take control of the speed and direction of the motor, and to do that we need to use Pulse Width Modulation or PWM Technique to control the speed and we are going to use the internal H-bridge to control the direction of the motor. We previously did a basic DC motor control using Arduino and PWM technique.
PWM - Motor Speed Control
When we are talking about a DC motor to change the speed of the DC motor, we need to change the amplitude of the input voltage that is applied to the motor. A common technique to do that is PWM (Pulse Width Modulation). In PWM the applied voltage is adjusted by sending a series of pulses, so the output voltage is proportional pulse width generated by the microcontroller that is also known as duty cycle. We have explained Pulse width modulation in detail earlier. Also check more PWM based projects with other microcontrollers by following the link.
The higher the duty cycle, the higher the average voltage applied to the DC motor (resulting in higher speed) and the shorter the duty cycle, the lower the average voltage applied to the DC motor (resulting in lower speed).
H-Bridge motor Direction Control
To change the direction of rotation of a DC motor you need to change the polarity of the supply. A common technique to do that is by using an H-bridge motor driver. An H-bridge motor driver consists of four switches (usually MOSFETs) arranged in a certain formation and the motor is connected to the center of the arrangement to form the H-like structure,
By Closing/enabling two opposite switches we can change the direction of the flowing current thus changing the direction of rotation.
DRV8833 Motor Driver IC
The DRV8833 is a dual channel motor drive IC capable of driving two bidirectional motors or a single stepper motor. The output driver block of each H-bridge consists of N-channel power MOSFETs configured as an H- bridge to drive the motor windings. Each H-bridge includes circuitry to regulate or limit the winding current. This IC is also designed to drive inductive loads such as relays, solenoids, DC, and bipolar stepping motors also.
The internal structure of the DRV8833 motor drive IC is shown above and it's taken from the DRV8833 dataset of the device. The device integrates two NMOS H-bridges and current regulation circuitry. The DRV8833 can be powered with a supply voltage from 2.7 to 10.8 V and can provide an output current up to 1.5-A RMS. The device also includes a low-power sleep mode, which lets the system save power when not driving the motor.
DRV8833 Motor Driver Module Pinout
The pinout of the DRV8833 Motor Driver Module is shown below. The DRV8833 module has a total of 12 pins that can be connected to the microcontrollers and motor.
EEP this is the sleep pin for the module. Logic high to enable device, logic low to enter low-power sleep mode and reset all
OUT(1-2) Outpu1 and Output2 of the interna H-Bridge A.
OUT(3-4) Outpu1 and Output2 of the internal H-Bridge B.
IN(1-2) Input1 and Input2 of the internal H-Bridge A.
IN(3-4) Input3 and Input4 of the internal H-Bridge B.
ULT Fault pin of the IC. Logic low when in fault condition (overtemperature, overcurrent)
VCC Supply pin of the IC. Max OPerating Voltage 11.8V.
GND Ground pin of the IC connects it to supply Ground.
DRV8833 Motor Driver IC Module Parts
The parts marking of the DRV8823 IC is shown below, the module is made with generic components and in this action that is shown below.
As you can see in the image above, in the middle of the module we have our DRV8833 IC and there are mainly three bypass capacitors on the board, a 10uF tantalum capacitor for input power. A 2.2uF capacitor for the VINT Pin and a 0.1uF capacitor for the VCP Pin. and finally, we have the onboard power indicator with its 4.7K Current limiting resistor.
Schematic Diagram of the DRB8833 Module
The internal schematic diagram of the DRV8833 module is shown below, the circuit diagram of the module is very simple as it has very few components on the PCB.
As you can see in the schematic diagram the connection for all the bypass capacitors with the IC is shown, we have also shown the connection for the pin header and beside the header you can see the name on the pins in the PCB. On the PCB there is also an LED with current Limiting Resistor as indicator.
Commonly Asked Questions about DRV8833 Motor Driver IC
What is the difference between L293D and DRV8833?
The L293D and the DRV8833 both are motor driver ICs, but they have differences. Both the ICs have a maximum current of 1.5A but the operating voltage of the DRV8833 IC is 11.8V whereas the operating voltage of L293D is 40V.
Can I run the stepper motor with DRV8833?
Yes, the DRV8833 IC can drive stepper motors. But micro stepping is not possible with this IC for that you will be needing a dedicated driver IC.
Can I connect 4 motors to DRV8833?
The DRV8833 is designed to drive two DC motors. If you want to drive 4 motors, it may still be feasible if you sacrifice direction control.
Simple Circuit Diagram: DRV8833 Interfacing with Arduino
The schematic diagram of the DRV8833 Module interfacing with Arduino is shown below.
In the schematic we are Interfacing DRV8833 with Arduino Pro Mini, and as per the diagram we have connected pins 9,6,5,3 to the IN1, IN2, IN3, IN4 pin of the module and the output pins are connected to the to motors. A switch is connected to pin 10 of the Arduino Pro Mini; this switch is used to change the direction of rotation. To control the speed of the motors we have two potentiometers and we are powering the circuit with an 8.4V battery. Finally, there is a switch to control the direction of the motors. The Hardware setup of the Module is shown below.
Arduino Code for Interfacing DRV8833 Motor Driver IC with Arduino
Complete code for Arduino DC motor speed control using PWM is given below, here we are explaining the code in detail.
Now that we have a clear understanding of how the DRV8833 Module works we can write some code to control the speed and direction of the motor.
We start our code by defining all the pins for motor driver and the switches.
#define mode_pin 10 #define IN1_PIN 9 #define IN4_PIN 6 #define IN3_PIN 5 #define IN4_PIN 3
Next, we have our setup function, in the setup function we make the PWM pins as outputs and the switch pins as input, and we set all the pins low.
void setup() { pinMode(IN1_PIN, OUTPUT); pinMode(IN4_PIN, OUTPUT); pinMode(IN3_PIN, OUTPUT); pinMode(IN4_PIN, OUTPUT); pinMode(mode_pin, INPUT); digitalWrite(IN1_PIN, LOW); digitalWrite(IN4_PIN, LOW); digitalWrite(IN3_PIN, LOW); digitalWrite(IN4_PIN, LOW); }
Next, we have our loop function. In the loop function we check the switch position if the switch is at the high state we rotate the motor clockwise and if the state of switch is low we rotate the motor counter clockwise.
void loop() { int sensorValue = analogRead(A0); int sensorValue1 = analogRead(A1); if (digitalRead(mode_pin) == LOW) { digitalWrite(IN3_PIN, LOW); analogWrite(IN4_PIN, sensorValue); digitalWrite(IN1_PIN, LOW); analogWrite(IN4_PIN, sensorValue1); } if (digitalRead(mode_pin) == HIGH) { digitalWrite(IN4_PIN, LOW); analogWrite(IN3_PIN, sensorValue); digitalWrite(IN4_PIN, LOW); analogWrite(IN1_PIN, sensorValue1); } }
We also use the analogRead(), and analogWrite() function to read the ADC value and write that value as PWM signal.
Projects Using Motor Driver IC and Arduino
Looking for a cool little raspberry pi project online? Then search no more because in this project we have built a raspberry pi-based motor drive hat that can be used to drive any motor that consumes 1.5A peak current.
Looking for a 8051 based motor controller which is easy to use and simple to make and cost less than any other microcontroller based solution, then you can check this project out, because in this project we have interfaced a 8051 microcontroller to build a PWM based motor driver system.
If you are looking to build a robot car then this project is for you, because in this project we have built an android phone and used it to drive a robot.
If you are looking for some interesting projects online then this project is for you, because in this project we have used a BLDC motor to make a fidget spinner that can be used to understand how it works and how to interact with it.
#define mode_pin 10
#define IN1_PIN 9
#define IN4_PIN 6
#define IN3_PIN 5
#define IN4_PIN 3
void setup() {
pinMode(IN1_PIN, OUTPUT);
pinMode(IN4_PIN, OUTPUT);
pinMode(IN3_PIN, OUTPUT);
pinMode(IN4_PIN, OUTPUT);
pinMode(mode_pin, INPUT);
digitalWrite(IN1_PIN, LOW);
digitalWrite(IN4_PIN, LOW);
digitalWrite(IN3_PIN, LOW);
digitalWrite(IN4_PIN, LOW);
}
void loop() {
int sensorValue = analogRead(A0);
int sensorValue1 = analogRead(A1);
if (digitalRead(mode_pin) == LOW)
{
digitalWrite(IN3_PIN, LOW);
analogWrite(IN4_PIN, sensorValue);
digitalWrite(IN1_PIN, LOW);
analogWrite(IN4_PIN, sensorValue1);
}
if (digitalRead(mode_pin) == HIGH)
{
digitalWrite(IN4_PIN, LOW);
analogWrite(IN3_PIN, sensorValue);
digitalWrite(IN4_PIN, LOW);
analogWrite(IN1_PIN, sensorValue1);
}
}
Comments
Hi, I've yet to see how the…
Hi,
I've yet to see how the DRV8833 can be used to control a Nema 17HS4023 stepper. I can't find an example anywhere, does this mean it doesn't work for steppers ?
Hello I was wanting to see if you could help me out I was using your code it's working alright but for some reason when I start turning the potentiometer about the 15% mark there is a small spot where it just stops moving it just stands still then when I continue it starts going again and then there is another spot about the halfway mark where it also does it again and then it just continues going again and then just continues until the end if you could help me out i would really appreciate it