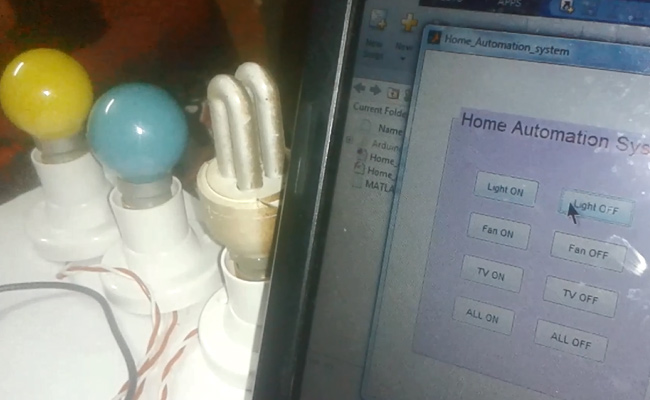
We all are familiar with the word ‘Automation’, where the human interaction is minimal and things can be controlled automatically or remotely. Home automation is very popular and demanding concept in the field of Electronics, and we are also making our best efforts to make this concept easily understandable and manageable as Electronics Projects. We have previously developed several types of Home Automation Projects with a working Video and Code, please check:
- DTMF Based Home Automation
- GSM Based Home Automation using Arduino
- PC Controlled Home Automation using Arduino
- Bluetooth Controlled Home Automation using 8051
- IR Remote Controlled Home Automation using Arduino
And in this project, we are going to build our next home automation project using MATLAB and Arduino, which is GUI Based Home Automation System Using Arduino and MATLAB
Components:
- Arduino UNO
- USB Cable
- ULN2003
- Relay 5 volt
- Bulb with holder
- Connecting wires
- Laptop
- Power supply
- PVT
Working Explanation:
In this project, we are using MATLAB with Arduino to control the Home appliances, through a Graphical User Interface in Computer. Here we have used wired communication for sending data from computer (MATLAB) to Arduino. In computer side, we have used GUI in MATLAB to create some buttons for controlling home appliances. For communication between Arduino and MATLAB, we first need to install the “MATLAB and Simulink Support for Arduino” or “Arduino IO Package”. To do so follow the below steps or check the video below:
- Download the Arduino IO Package from here. You need to Sign up before download.
- Then Burn/upload the adioe.pde file to the Arduino using Arduino IDE. This adioe.pde file can be found in Arduino IO Package – ArduinoIO\pde\adioe\adioe.pde
- Then open the MATLAB software, go through the Arduino IO folder, open the install_arduino.m file and Run it in Matlab. You will see a message of “Arduino folders added to the path” in command window of MATLAB, means MATLAB path is updated to Arduino folders.
That’s how we make the Arduino, communicate with MATLAB. Above method is suitable for “MATLAB R2013b or earlier versions”, if you are using the higher version of MATLAB (like R2015b or R2016a), you can directly click on Add-ons Tab in MATLAB and then click “Get Hardware Support Packages”, from where you can install Arduino packages for MATLAB.
After installing files, now you can create a GUI for Home Automation Project. Basically in GUI, we are creating Push Buttons for controlling the home appliances from computer. Buttons can be created by going into “Graphical User Interface” in “New” menu in MATLAB. Further we can set the name and colours of these buttons, we have created 8 buttons, in which six to ON and OFF three home appliances and two buttons to ON and OFF all the appliances simultaneously.
Now after creating the buttons, when you click on Run button in that GUI window, it will ask you to save this GUI file (with extension .fig), also known as ‘fig file’. As soon you saved the file, it will automatically create a code file (with extension .m), also known as ‘M file’ (see below screen shot), where you can put the Code (given in Code section below). You can download the GUI file and Code file for this project from here: Home_Automation_system.fig and Home_Automation_system.m (right click and select Save link as...), or you can create them yourself like we have explained.
After coding you can now finally Run the .m file from the code window, you will see “Attempting connection..” in the command window. Then a “Arduino successfully connected” message appears, if everything goes well. And finally you will see previously created GUI (buttons) in GUI window, from where you can control the home appliances by just clicking on the buttons in your Computer. Make sure that Arduino is connected to Arduino via USB cable. Here in this project we have used 3 bulbs for demonstration, which indicates Fan, Light and TV.
Working of the whole project, from installing the Arduino MATLAB support package to Turn On or OFF the appliance, can be understood at the Video at the end.
Circuit Explanation:
Circuit of this project is very easy. Here we have used an Arduino UNO board and Relay Driver ULN2003 for driving relays. Three 5 volt SPDT Relays are connected to Arduino pin number 3, 4 and 5, through relay driver ULN2003, for controlling LIGHT, FAN and TV respectively.
Programming Explanation:
When we press any button from the GUI window then it sends some commands to Arduino and then Arduino do that operation. After installing Arduino MATLAB IO support package, we can access Arduino from the MATLAB by using the same Arduino functions, with some little variation, like:
For making a pin HIGH in Arduino we write code as digitalWrite(pin, HIGH)
In MATLAB we will use this function with the help of an object or variable like,
a.digitalWrite(pin, HIGH);
and likewise so on.
Before doing this we have to initialise variable like this:
a = Arduino(‘COM1’); // change COM1 according to your port
In this project, there is no Arduino code except the Arduino MATLAB support package code or file. As explained earlier that code file (.m file) is automatically generated while saving the GUI file (.fig file). There is already some code prewritten in .m file. Basically these are Callback functions for Push buttons, means we can define what should happen on clicking on these Push Buttons.
In MATLAB code, first we initialise serial port and make it an object by using a variable. And then we can start programming like Arduino using the variable.
clear ar; global ar; ar=arduino('COM13'); ar.pinMode(3, 'OUTPUT'); ar.pinMode(4, 'OUTPUT'); ar.pinMode(5, 'OUTPUT'); ar.pinMode(13, 'OUTPUT');
In the call back function of each button, we have written the related code for On or OFF the respective Home Appliances, connected to Arduino via Relay. Like for example, Callback function for Light ON is given below:
function light_on_Callback(hObject, eventdata, handles) % hObject handle to light_on (see GCBO) % eventdata reserved - to be defined in a future version of MATLAB % handles structure with handles and user data (see GUIDATA) global ar; ar.digitalWrite(3, 1); ar.digitalWrite(13, 1);
Likewise we can write the code in the Callback functions of all the buttons, to control the other connected Home Appliances, check the full MATLAB Code below (.m file).
Complete Project Code
function varargout = Home_Automation_system(varargin)
% HOME_AUTOMATION_SYSTEM MATLAB code for Home_Automation_system.fig
% HOME_AUTOMATION_SYSTEM, by itself, creates a new HOME_AUTOMATION_SYSTEM or raises the existing
% singleton*.
%
% H = HOME_AUTOMATION_SYSTEM returns the handle to a new HOME_AUTOMATION_SYSTEM or the handle to
% the existing singleton*.
%
% HOME_AUTOMATION_SYSTEM('CALLBACK',hObject,eventData,handles,...) calls the local
% function named CALLBACK in HOME_AUTOMATION_SYSTEM.M with the given input arguments.
%
% HOME_AUTOMATION_SYSTEM('Property','Value',...) creates a new HOME_AUTOMATION_SYSTEM or raises the
% existing singleton*. Starting from the left, property value pairs are
% applied to the GUI before Home_Automation_system_OpeningFcn gets called. An
% unrecognized property name or invalid value makes property application
% stop. All inputs are passed to Home_Automation_system_OpeningFcn via varargin.
%
% *See GUI Options on GUIDE's Tools menu. Choose "GUI allows only one
% instance to run (singleton)".
%
% See also: GUIDE, GUIDATA, GUIHANDLES
% Edit the above text to modify the response to help Home_Automation_system
% Last Modified by GUIDE v2.5 28-Feb-2016 01:59:17
% Begin initialization code - DO NOT EDIT
gui_Singleton = 1;
gui_State = struct('gui_Name', mfilename, ...
'gui_Singleton', gui_Singleton, ...
'gui_OpeningFcn', @Home_Automation_system_OpeningFcn, ...
'gui_OutputFcn', @Home_Automation_system_OutputFcn, ...
'gui_LayoutFcn', [] , ...
'gui_Callback', []);
if nargin && ischar(varargin{1})
gui_State.gui_Callback = str2func(varargin{1});
end
if nargout
[varargout{1:nargout}] = gui_mainfcn(gui_State, varargin{:});
else
gui_mainfcn(gui_State, varargin{:});
end
% End initialization code - DO NOT EDIT
% --- Executes just before Home_Automation_system is made visible.
function Home_Automation_system_OpeningFcn(hObject, eventdata, handles, varargin)
% This function has no output args, see OutputFcn.
% hObject handle to figure
% eventdata reserved - to be defined in a future version of MATLAB
% handles structure with handles and user data (see GUIDATA)
% varargin command line arguments to Home_Automation_system (see VARARGIN)
% Choose default command line output for Home_Automation_system
handles.output = hObject;
% Update handles structure
guidata(hObject, handles);
clear ar;
global ar;
ar=arduino('COM13');
ar.pinMode(3, 'OUTPUT');
ar.pinMode(4, 'OUTPUT');
ar.pinMode(5, 'OUTPUT');
ar.pinMode(13, 'OUTPUT');
% UIWAIT makes Home_Automation_system wait for user response (see UIRESUME)
% uiwait(handles.figure1);
% --- Outputs from this function are returned to the command line.
function varargout = Home_Automation_system_OutputFcn(hObject, eventdata, handles)
% varargout cell array for returning output args (see VARARGOUT);
% hObject handle to figure
% eventdata reserved - to be defined in a future version of MATLAB
% handles structure with handles and user data (see GUIDATA)
% Get default command line output from handles structure
varargout{1} = handles.output;
% --- Executes on button press in light_on.
function light_on_Callback(hObject, eventdata, handles)
% hObject handle to light_on (see GCBO)
% eventdata reserved - to be defined in a future version of MATLAB
% handles structure with handles and user data (see GUIDATA)
global ar;
ar.digitalWrite(3, 1);
ar.digitalWrite(13, 1);
% --- Executes on button press in light_off.
function light_off_Callback(hObject, eventdata, handles)
% hObject handle to light_off (see GCBO)
% eventdata reserved - to be defined in a future version of MATLAB
% handles structure with handles and user data (see GUIDATA)
global ar;
ar.digitalWrite(3, 0);
ar.digitalWrite(13, 0);
% --- Executes on button press in fan_on.
function fan_on_Callback(hObject, eventdata, handles)
% hObject handle to fan_on (see GCBO)
% eventdata reserved - to be defined in a future version of MATLAB
% handles structure with handles and user data (see GUIDATA)
global ar;
ar.digitalWrite(4, 1);
% --- Executes on button press in fan_off.
function fan_off_Callback(hObject, eventdata, handles)
% hObject handle to fan_off (see GCBO)
% eventdata reserved - to be defined in a future version of MATLAB
% handles structure with handles and user data (see GUIDATA)
global ar;
ar.digitalWrite(4, 0);
% --- Executes on button press in tv_on.
function tv_on_Callback(hObject, eventdata, handles)
% hObject handle to tv_on (see GCBO)
% eventdata reserved - to be defined in a future version of MATLAB
% handles structure with handles and user data (see GUIDATA)
global ar;
ar.digitalWrite(5, 1);
% --- Executes on button press in tv_off.
function tv_off_Callback(hObject, eventdata, handles)
% hObject handle to tv_off (see GCBO)
% eventdata reserved - to be defined in a future version of MATLAB
% handles structure with handles and user data (see GUIDATA)
global ar;
ar.digitalWrite(5, 0);
% --- Executes on button press in all_on.
function all_on_Callback(hObject, eventdata, handles)
% hObject handle to all_on (see GCBO)
% eventdata reserved - to be defined in a future version of MATLAB
% handles structure with handles and user data (see GUIDATA)
global ar;
ar.digitalWrite(3, 1);
ar.digitalWrite(4, 1);
ar.digitalWrite(5, 1);
% --- Executes on button press in all_off.
function all_off_Callback(hObject, eventdata, handles)
% hObject handle to all_off (see GCBO)
% eventdata reserved - to be defined in a future version of MATLAB
% handles structure with handles and user data (see GUIDATA)
global ar;
ar.digitalWrite(3, 0);
ar.digitalWrite(4, 0);
ar.digitalWrite(5, 0);
Comments
linking arduino pushbuttons to gui pushbutons
Dear Saddam,
I have been searching for weeks on a way to link between Arduino and GUI
I have created a GUI of two push buttons and an edit box.When i click one of the buttons it would increments and the other would decrements..I need to connect these two buttons to two pushbuttons on Arduino, that is when I push the button of Arduino it would increment or decrement in GUI.
I would really really appreciate your help.
Thank you
final year project
Pls can you give me the matlab gui file because i need it and i am not familiar with matlab coding
Link to Download the GUI file
Link to Download the GUI file (.fig) is already given in Working Explanation, with the name Home_Automation_system.fig. Right click on that link and Click on "Save link as..."
I am getting this error
Error using HOME_AUTOMATION_SYSTEM>HOME_AUTOMATION_SYSTEM_OutputFcn (line 77)
Failed to open serial port COM4 to communicate with board Uno. Make sure there is no other MATLAB
arduino object for this board. For troubleshooting, see Arduino Hardware Troubleshooting.
Error in gui_mainfcn (line 264)
feval(gui_State.gui_OutputFcn, gui_hFigure, [], gui_Handles);
Error in HOME_AUTOMATION_SYSTEM (line 42)
gui_mainfcn(gui_State, varargin{:});
I am getting this error while running this program please help
Facing the same problem
Heyy, how did you fix this error
Please revert back asap
Thankyou
what is the pvt written in components required
I would like to know what do you mean by PVT in components required.
PVT is terminal block with
PVT is terminal block with screw on relay module block.
error after running code
after showing aurdino connected successfully ..when we click on push buttons its working properly.but after some period of time its showing an error of .."Error while evaluating uicontrol Callback"..and all the push buttons are been disabled..please sort it out
where did you guys used pvt?
where did you guys used pvt? what is it mean and its significance?
making GUI for home automation using power line comunication
please any one help that who can i send the GUI signal from one arduino to another arduino using power line communication.......
I can't run the Home
I can't run the Home_Automation_system.m code. Can I ask for a help???
I says
I says
Error using Home_Automation_system>Home_Automation_system_OpeningFcn (line 64)
Cannot find Arduino hardware on port COM11. Make sure Arduino hardware is properly plugged in. Otherwise, please specify both port and
board type. For more information, see arduino function reference page.
Error in gui_mainfcn (line 220)
feval(gui_State.gui_OpeningFcn, gui_hFigure, [], guidata(gui_hFigure), varargin{:});
Error in Home_Automation_system (line 42)
gui_mainfcn(gui_State, varargin{:});
What to do??? Please help me. My port is COM11 but it did'nt run. Can you help me please??? Thank you
Check in device manager if
Check in device manager if your board is discovered
Facing the same problem
Heyy, how did you fix this error
Please revert back asap
Thankyouu
Quick enquiry
please can you give the component name/specification of the component having the relays. Or you build it yourself?
Suitable ... interested in using