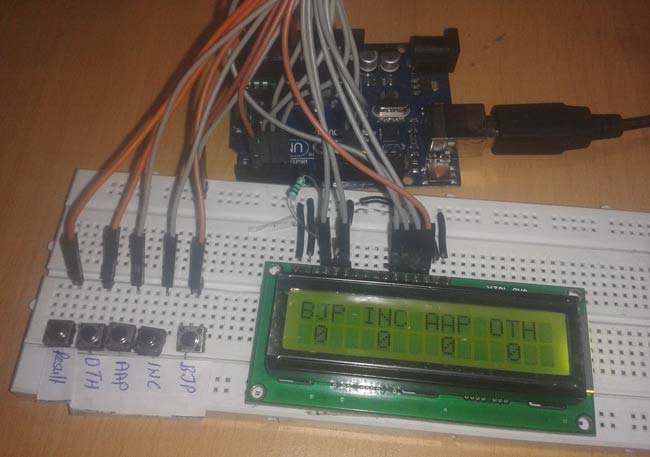
We all are quite familiar with voting machines, even we have covered few other electronic voting machine projects previously using RFID with 8051, AVR microcontroller, and Raspberry Pi. In this project, we have used the arduino uno board to build a simple electronic voting machine. If you are looking for a similar Fingerprint based biometric voting machine project, you can check the link.
Components
- Arduino Uno
- 16x2 LCD
- Push button
- Bread board
- Power
- Connecting wires
Arduino Electronic Voting Machine Circuit Diagram and Working
In this project we have used four push buttons for four different candidates. We can increase the number of candidate but for better understanding we have limited it to four. When any voter press any of four button then respecting voting value will increment by one each time. After whole voting we will press result button to see the results. As the "result" button is pressed, arduino calculates the total votes of each candidate and show it on LCD display.
Circuit of this project is quite easy which contains Arduino, push buttons and LCD. Arduino controls the complete processes like reading button, incrementing vote value, generating result and sending vote and result to LCD. Here we have added five buttons in which first button is for BJP, second for INC, third is for AAP, forth is for OTH means others and last button is used for calculating or displaying results.
The five push buttons are directly connected with pin 15-19(A1-A5) of Arduino with respect to ground. A 16x2 LCD is connected with arduino in 4-bit mode. Control pin RS, RW and En are directly connected to arduino pin 12, GND and 11. And data pin D4-D7 is connected to pins 5, 4, 3 and 2 of arduino.
Arduino EVM Code Description
First of all we include header and define pins for LCD and than initialize some variables and pin for taking candidate's voting input means switch.
After it, initialize the LCD and give direction to input-output pins.
and then make pullup the input pin by software.
In code we have used digital read function to read Button pressed.
And then displaying voting on the LCD with the candidate party’s Name.
Complete Project Code
//Arduino based EVM Code
#include<LiquidCrystal.h>
LiquidCrystal lcd(12, 11, 5, 4, 3, 2);
#define sw1 15
#define sw2 16
#define sw3 17
#define sw4 18
#define sw5 19
int vote1=0;
int vote2=0;
int vote3=0;
int vote4=0;
void setup()
{
pinMode(sw1, INPUT);
pinMode(sw2,INPUT);
pinMode(sw3,INPUT);
pinMode(sw4,INPUT);
pinMode(sw5,INPUT);
lcd.begin(16, 2);
lcd.print("Voting Machine");
lcd.setCursor(0,1);
lcd.print("Circuit Digest");
delay(3000);
digitalWrite(sw1, HIGH);
digitalWrite(sw2, HIGH);
digitalWrite(sw3, HIGH);
digitalWrite(sw4, HIGH);
digitalWrite(sw5, HIGH);
lcd.clear();
lcd.setCursor(0,0);
lcd.print("BJP");
lcd.setCursor(4,0);
lcd.print("INC");
lcd.setCursor(8,0);
lcd.print("AAP");
lcd.setCursor(12,0);
lcd.print("OTH");
}
void loop()
{
lcd.setCursor(0,0);
lcd.print("BJP");
lcd.setCursor(1,1);
lcd.print(vote1);
lcd.setCursor(4,0);
lcd.print("INC");
lcd.setCursor(5,1);
lcd.print(vote2);
lcd.setCursor(8,0);
lcd.print("AAP");
lcd.setCursor(9,1);
lcd.print(vote3);
lcd.setCursor(12,0);
lcd.print("OTH");
lcd.setCursor(13,1);
lcd.print(vote4);
if(digitalRead(sw1)==0)
vote1++;
while(digitalRead(sw1)==0);
if(digitalRead(sw2)==0)
vote2++;
while(digitalRead(sw2)==0);
if(digitalRead(sw3)==0)
vote3++;
while(digitalRead(sw3)==0);
if(digitalRead(sw4)==0)
vote4++;
while(digitalRead(sw4)==0);
if(digitalRead(sw5)==0)
{
int vote=vote1+vote2+vote3+vote4;
if(vote)
{
if((vote1 > vote2 && vote1 > vote3 && vote1 > vote4))
{
lcd.clear();
lcd.print("BJP Wins");
delay(2000);
lcd.clear();
}
else if((vote2 > vote1 && vote2 > vote3 && vote2 > vote4))
{
lcd.clear();
lcd.print("INC Wins");
delay(2000);
lcd.clear();
}
else if((vote3 > vote1 && vote3 > vote2 && vote3 > vote4))
{
lcd.clear();
lcd.print("AAP Wins");
delay(2000);
lcd.clear();
}
else if(vote4 > vote1 && vote4 > vote2 && vote4 > vote3)
{
lcd.setCursor(0,0);
lcd.clear();
lcd.print("OTH Wins");
delay(2000);
lcd.clear();
}
else if(vote4 > vote1 && vote4 > vote2 && vote4 > vote3)
{
lcd.setCursor(0,0);
lcd.clear();
lcd.print("OTH Wins");
delay(2000);
lcd.clear();
}
else
{
lcd.clear();
lcd.print(" Tie Up Or ");
lcd.setCursor(0,1);
lcd.print(" No Result ");
delay(1000);
lcd.clear();
}
}
else
{
lcd.clear();
lcd.print("No Voting....");
delay(1000);
lcd.clear();
}
vote1=0;vote2=0;vote3=0;vote4=0,vote=0;
lcd.clear();
}
}
Comments
Arduino uno software
Will u please help me out that
Which software is being used to write program in arduino uno?????
Arduino IDE software (Arduino
Arduino IDE software (Arduino Nightly : https://www.arduino.cc/en/Main/Software) is used to write, verify and upload/burn the code into Arduino.
It doesn't store the result
It doesn't store the result so if power suppyly is lost the memory erases.... You can use eeprom of the arduino using eeprom.h library to store the result
Where does pin 2 and 16 of
Where does pin 2 and 16 of LCD are connected.
It is the symbol of Power
It is the symbol of Power supply positive terminal or Vcc.
how to avoid the pressing the
how to avoid the pressing the switch more than one times by a person
Either we can delay in
Either we can delay in between two continuous button pressing or for more accurate solution we can use finger print sensor.
How much power supply
How much power supply does.it.need to work
Hi !can u help me?
Hi !can u help me?
When I am doing coding in ardino it showing code error .can u tell me why ?
sir I am getting the error in
sir I am getting the error in the program as "stray '\302' in program" so what should I do and where are the 16 and 2 pin connected ?
I tried this but nothing is
I tried this but nothing is getting displayed on the lcd .
check you LCD connection and
check you LCD connection and also the contrast level of the LCD
No of votes.
How much votes can it save? I mean how many votes can be saved on arduino's internal eeprom. I am using Arduino Uno which has 1024 bytes of eeprom.
i am doing a project on
i am doing a project on fingure print voting can you please send me code for that!!
Can you help me in addition with the recognition of finger print? If the same finger print is discovered and i will display error?