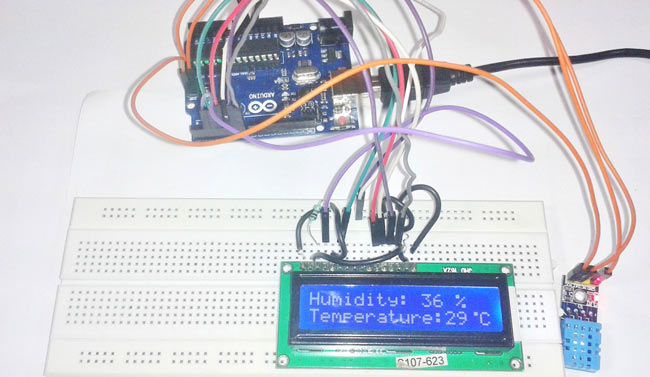
Humidity and temperature are common parameters to measure environmental conditions. In this Arduino based project we are going to measure ambient temperature and humidity and display it on a 16x2 LCD screen. A combined temperature and himidity sensor DHT11 is used with Arduino uno to develop this Celsius scale thermometer and percentage scale humidity measurement project. In one of my previous project, I have also developed a digital thermometer using temperature sensor LM35.
This project consists of three sections - one senses the humidity and temperature by using humidity and temperature sensor DHT11. The second section reads the DHTsensor module’s output and extracts temperature and humidity values into a suitable number in percentage and Celsius scale. And the third part of the system displays humidity and temperature on LCD.
Working of this project is based on single wire serial communication. First arduino send a start signal to DHT module and then DHT gives a response signal containing temperature and humidity data. Arduino collect and extract in two parts one is humidity and second is temperature and then send them to 16x2 LCD.
Here in this project we have used a sensor module namely DHT11. This module features a humidity and temperature complex with a calibrated digital signal output means DHT11 sensor module is a combined module for sensing humidity and temperature which gives a calibrated digital output signal. DHT11 gives us very precise value of humidity and temperature and ensures high reliability and long term stability. This sensor has a resistive type humidity measurement component and NTC type temperature measurement component with an 8-bit microcontroller inbuilt which has a fast response and cost effective and available in 4-pin single row package.
DHT11 module works on serial communication i.e. single wire communication. This module sends data in form of pulse train of specific time period. Before sending data to arduino it needs some initialize command with a time delay. And the whole process time is about 4ms. A complete data transmission is of 40-bit and data format of this process is given below:
8-bit integral RH data + 8-bit decimal RH data + 8-bit integral T data + 8-bit decimal T data + 8-bit check sum.
Complete Process
First of all arduino sends a high to low start signal to DHT11 with 18µs delay to ensure DHT’s detection. And then arduino pull-up the data line and wait for 20-40µs for DHT’s response. Once DHT detects starts signal, it will send a low voltage level response signal to arduino of time delay about 80µs. And then DHT controller pull up the data line and keeps it for 80µs for DHT’s arranging of sending data.
When data bus is at low voltage level it means that DHT11 is sending response signal. Once it is done, DHT again makes data line pull-up for 80µs for preparing data transmission.
Data format that is sending by DHT to arduino for every bit begins with 50µs low voltage level and length of high voltage level signal determines whether data bit is “0” or “1”.
One important thing is to make sure pull up resistor value because if we are placing DHT sensor at <20 meter distance, 5k pull up resistor is recommended. If placing DHT at longer the 20 meter then use appropriate value pull up resistor.
Circuit Diagram and Explanation
A liquid crystal display is used for displaying temperature and humidity which is directly connected to arduino in 4-bit mode. Pins of LCD namely RS, EN, D4, D5, D6 and D7 are connected to arduino digital pin number 2, 3, 4, 5, 6 and 7. And a DHT11 sensor module is also connected to digital pin 12 of arduino with a 5k pull-up resistor.
Programming Description
In programming, we are going to use pre-built libraries for DHT11 sensor and LCD display module.
Then we haved defined pins for LCD and DHT sensor and initialized all the things in setup. Then in a loop by using dht function reads DHT sensor and then using some dht functions we extract humidity and temperature and display them on LCD.
Here degree symbol is created by using custom character method.
Complete Project Code
#include<dht.h> // Including library for dht
#include<LiquidCrystal.h>
LiquidCrystal lcd(2, 3, 4, 5, 6, 7);
#define dht_dpin 12
dht DHT;
byte degree[8] =
{
0b00011,
0b00011,
0b00000,
0b00000,
0b00000,
0b00000,
0b00000,
0b00000
};
void setup()
{
lcd.begin(16, 2);
lcd.createChar(1, degree);
lcd.clear();
lcd.print(" Humidity ");
lcd.setCursor(0,1);
lcd.print(" Measurement ");
delay(2000);
lcd.clear();
lcd.print("Circuit Digest ");
delay(2000);
}
void loop()
{
DHT.read11(dht_dpin);
lcd.setCursor(0,0);
lcd.print("Humidity: ");
lcd.print(DHT.humidity); // printing Humidity on LCD
lcd.print(" %");
lcd.setCursor(0,1);
lcd.print("Temperature:");
lcd.print(DHT.temperature); // Printing temperature on LCD
lcd.write(1);
lcd.print("C");
delay(500);
}
Comments
You can clearly see the parts
You can clearly see the parts used, Arduino Uno, 16X2 LCD and DHT11 Temperature and humidity sensor. Proteus doesn't come with Arduino installed, you need to add Arduino Library in Proteus. Library folder can be found at Proteus 8 Professional\Data\LIBRARY
but the created arduino doesn
but the created arduino doesn't have a ground nor vcc ?
How I can add dht11.h file
How I can add dht11.h file because this header file is not in my library.?
Download DHT library from
Download DHT library from here and and install it using this Tutorial: Installing Additional Arduino Libraries
Add DHT library in arduino
Add DHT library in arduino IDE and then try again....
How to download dht11
How to download dht11 function in arduino?
Can I directly run this
Can I directly run this project without testing in Proteus?
Download DHT library from
Download DHT library from here and and install it using this Tutorial: Installing Additional Arduino Libraries. Yes you can it run directly.
nothing iis clear.....
nothing iis clear......everything is blank!!!!!!
wat to do?????????????
can any one send me the
can any one send me the Proteus Simulation of this project ?
My proteus arduino library
I downloaded peoteus arduino library but it doesn't have 5v,3v,GND and VIN pinouts. What should i do ?
its showing up errors even
its showing up errors even after adding libraries. the error message is :
Arduino: 1.8.1 (Windows 7), Board: "Arduino/Genuino Uno"
sketch_jan11a:5: error: no matching function for call to 'DHT::DHT()'
DHT dht;
^
C:\Users\user\Desktop\sketch_jan11a\sketch_jan11a\sketch_jan11a.ino:5:5: note: candidates are:
In file included from C:\Users\user\Desktop\sketch_jan11a\sketch_jan11a\sketch_jan11a.ino:1:0:
C:\Users\user\Documents\Arduino\libraries\DHT-sensor-library-master/DHT.h:40:4: note: DHT::DHT(uint8_t, uint8_t, uint8_t)
DHT(uint8_t pin, uint8_t type, uint8_t count=6);
^
C:\Users\user\Documents\Arduino\libraries\DHT-sensor-library-master/DHT.h:40:4: note: candidate expects 3 arguments, 0 provided
C:\Users\user\Documents\Arduino\libraries\DHT-sensor-library-master/DHT.h:38:7: note: constexpr DHT::DHT(const DHT&)
class DHT {
^
C:\Users\user\Documents\Arduino\libraries\DHT-sensor-library-master/DHT.h:38:7: note: candidate expects 1 argument, 0 provided
C:\Users\user\Documents\Arduino\libraries\DHT-sensor-library-master/DHT.h:38:7: note: constexpr DHT::DHT(DHT&&)
C:\Users\user\Documents\Arduino\libraries\DHT-sensor-library-master/DHT.h:38:7: note: candidate expects 1 argument, 0 provided
C:\Users\user\Desktop\sketch_jan11a\sketch_jan11a\sketch_jan11a.ino: In function 'void loop()':
sketch_jan11a:32: error: 'class DHT' has no member named 'read11'
dht.read11(dht_dpin);
^
sketch_jan11a:35: error: 'class DHT' has no member named 'humidity'
lcd.print(dht.humidity); // printing Humidity on LCD
^
sketch_jan11a:39: error: 'class DHT' has no member named 'temperature'
lcd.print(dht.temperature); // Printing temperature on LCD
^
exit status 1
no matching function for call to 'DHT::DHT()'
This report would have more information with
"Show verbose output during compilation"
option enabled in File -> Preferences.
code error
I tried to use the lower version 1.0.0 nut still give error : 'dht' does not name a type !!
LCD display Humidity: -999.0% and Temperature: -999
On lcd there is no display of readings of the temp and humidity instead it is showing
Humidity: -999.0
Temperature: -999 like this.
What is the mistake can anyone tell??
hi. i want to know name of the parts that you use because the are not same as mine. what proteus you used? i also have a problem regarding the arduino libraries in proteus. i could'nt find the arduino's part in proteus library. i've check that my proteus 8 does'nt have the library folder after installed. please help me. thank you