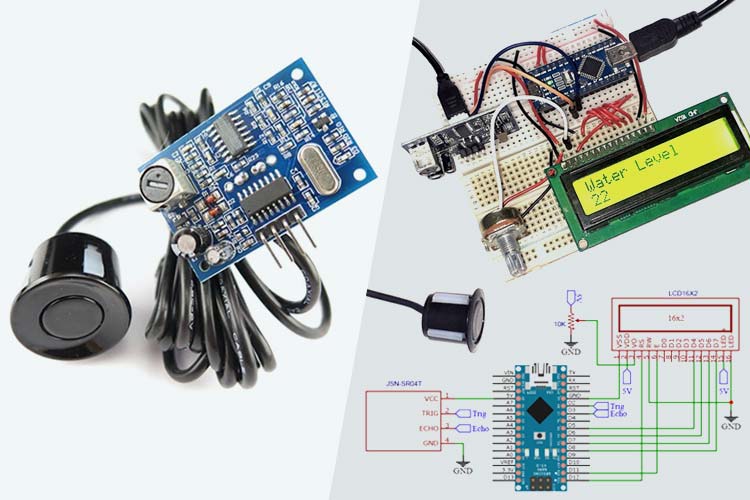
Ultrasonic sensor, also known as a SONAR sensor is an electronic device that is used to measure the distance of a target object by emitting ultrasonic sound waves. Apart from distance measurement, it is also used for object detection and obstacle avoidance robots. Previously, we used an HC-SR04 Ultrasonic sensor with Arduino and other microcontrollers for distance measurement and other applications. This sensor is easy to use and pretty inexpensive. But it has some downsides. One of them is that these sensors can’t be used in applications that involve water and harsh environments.
In this tutorial, we are going to discuss a new Waterproof ultrasonic sensor named JSN SR-04T. Throughout this tutorial, we will learn how the JSN SR-04T Waterproof Ultrasonic Sensor works, what are its features and specifications and how to interface it with the Arduino Nano.
Components Required
- Arduino Nano
- JSN SR-04T Waterproof Ultrasonic Sensor
- 16*2 LCD Display
- Breadboard
- Jumper Wires
JSN SR-04T Waterproof Ultrasonic Sensor
JSN-SR0T4 is a waterproof ultrasonic distance measurement sensor module that can provide 25cm-450cm non-contact distance measurements, with a ranging accuracy of up to 2mm. This sensor module comes in two separate parts, one being the transducer, which is the sensing element and the other being the control board. It is very similar to the ultrasonic sensors which are found in car bumpers.
JSN SR-04T sensor module has an industrial-grade integrated ultrasonic sensing probe design, waterproof type, stable performance, and high precision. It can be used in horizontal ranging, obstacle avoidance, automated control, monitoring of objects and their movement, traffic control, security, and artificial intelligence, and other applications.
JSN SR-04T Pinout:
Pin No. |
Pin Name |
Description |
1 |
5V |
Positive supply pin of the sensor |
2 |
Trig |
Input pin of the sensor. This pin has to be kept high for 10μs to initialize measurement by sending ultrasonic waves. |
3 |
Echo |
Output pin sensor. This pin goes high for a period that will be equal to the time taken for the ultrasonic wave to return to the sensor. |
4 |
Gnd |
This pin is connected to the Ground of the system. |
The JSN SR-04T sensor module is similar to HC-SR04, but it brings some improvements compared to HC-SR04:
- Unlike HC-SR04, JSN SR-04T doesn’t have the transducer soldered onto the PCB. Instead, it’s connected with a pretty long external cable (2.5 Meters) so, you can put the sensing element far away from all the control circuitry.
- The sensor itself is encased in a waterproof enclosure, which might be useful if you want to locate it outside in harsher environments.
With all these advantages, it has some disadvantages too. The minimum distance this sensor can measure is 20cm compared to the 2cm of the HC-SR04. The reason behind this is that JSN SR-04T has only one transducer. It uses the same transducer to both transmit and receive the signal so it needs time to switch from one mode to another. This is why the JSN SR-04T sensor can't measure below 20cm but HC-SR04 can because it has a separate transmitter and receiver.
Specifications & Features of JSN SR-04T Waterproof Ultrasonic Sensor:
- Working voltage: DC 5V
- Static working current: 5mA
- Working current: 30mA
- Acoustic emission frequency:40KHz
- Operating Range: 25cm to 4.5m
- Cable Length: 2.5 meter
Interfacing JSN SR-04T with Arduino
The complete circuit diagram for Interfacing Waterproof Ultrasonic Sensor JSN SR-04T with Arduino is given below:
That’s pretty much the wiring. Both modules are powered with GND and 5V, Trigger and Echo pins of ultrasonic sensor are connected to D2 and D3 of Arduino. Complete LCD connections are given in the table below.
LCD |
Arduino |
VSS |
GND |
VDD |
5V |
VO |
Potentiometer |
RS |
D12 |
RW |
GND |
E |
D11 |
D4 |
D4 |
D5 |
D5 |
D6 |
D6 |
D7 |
D7 |
A |
5V |
K |
GND |
My hardware looked like this:
Programming Arduino for Waterproof Ultrasonic Sensor
Complete code for Interfacing Waterproof Ultrasonic Sensor JSN SR-04T to Arduino can be found at the end of the page. Here, I am explaining some important parts of the program.
As usual, start the code by including all the required libraries. Here, we are only using LiquidCrystal.h library. It is used for controlling the LCD module using an Arduino board with the help of built-in methods defined inside the library. This library can be used for both the 4-bit mode and 8-bit mode wiring of LCD.
#include<LiquidCrystal.h>
Then in the next line, define the pins of Arduino that are interfaced to LCD
LiquidCrystal lcd(12, 11, 5, 6, 7, 8);
Then define the trigger pin and echo for the ultrasonic sensor. We have connected the trigger pin to the D2 pin of Arduino and the echo pin to the D3 pin of the Arduino.
#define ECHOPIN 3 #define TRIGPIN 2
Inside the setup() function, define the trigger pin as an output and the echo pin as an Input and also start the serial communication at 9600 for showing the results on the serial monitor and initialize the interface to the LCD screen and specifies the dimensions.
void setup() { Serial.begin(9600); lcd.begin(16, 2); pinMode(ECHOPIN,INPUT_PULLUP); pinMode(TRIGPIN, OUTPUT); digitalWrite(ECHOPIN, HIGH); }
In the loop, first set trigger pin to LOW State for 2 µs to make sure that the trigger pin is clear. Then set the trigger pin high for 15 us to send an ultrasonic wave. We will use the pulseIn() function to read the travel time and store that value into the variable “duration”. In the end, we will print the value of the distance on the Serial Monitor.
void loop() { digitalWrite(TRIGPIN, LOW); delayMicroseconds(2); digitalWrite(TRIGPIN, HIGH); delayMicroseconds(15); digitalWrite(TRIGPIN, LOW); int distance = pulseIn(ECHOPIN, HIGH, 26000); distance=distance/58; Serial.print(distance);
Testing the Waterproof Ultrasonic Sensor JSN SR-04T
Once your code and hardware are ready, connect Arduino to the laptop and upload the code. After that, open the serial monitor at a baud rate of 9600, and put something in front of the sensor. Calculated distance will be displayed on LCD display.
Complete working video and code are given below. If you have any queries regarding this project, drop them in the comment section below.
Complete Project Code
#include<LiquidCrystal.h>
LiquidCrystal lcd(12, 11, 4, 5, 6, 7); // sets the interfacing pins
#define ECHOPIN 3
#define TRIGPIN 2
void setup() {
Serial.begin(9600);
lcd.begin(16, 2); // initializes the 16x2 LCD
pinMode(ECHOPIN,INPUT_PULLUP);
pinMode(TRIGPIN, OUTPUT);
digitalWrite(ECHOPIN, HIGH);
}
void loop() {
lcd.clear();
digitalWrite(TRIGPIN, LOW);
delayMicroseconds(2);
digitalWrite(TRIGPIN, HIGH);
delayMicroseconds(15);
digitalWrite(TRIGPIN, LOW);
int distance1 = pulseIn(ECHOPIN, HIGH, 26000);
int distance=distance1/58;
Serial.print(distance);
Serial.println(" cm");
lcd.setCursor(0,0); //sets the cursor at row 0 column 0
lcd.print("Water Level"); // prints 16x2 LCD MODULE
lcd.setCursor(0,1);
lcd.print(distance);
delay(500);
}
Comments
Thank you for this great tutorial - very helpful.
Please correct the wiring: In the schematic you have LCD D4-D7 connected to Ardu D5-D8, In the table it is correct. In the text the code
LiquidCrystal lcd(12, 11, 5, 6, 7, 8);
needs to read
LiquidCrystal lcd(12, 11, 4, 5, 6, 7);
The attached code is correct.
All the best.
GOOD EVENING. THANKS FOR THE GOOD WORK YOU ARE DOING, PRESENTING PROJECTS WE CAN TRY TO REPRODUCE. PLEASE I URGENTLY NEED JSN SR - 40 T WATERPROOF ULTRASONIC SENSOR AND OTHER COMPONENTS TO HELP ME TRY OIT THIS PROJECT. I ALSO NEED TO KNOW THE COST.