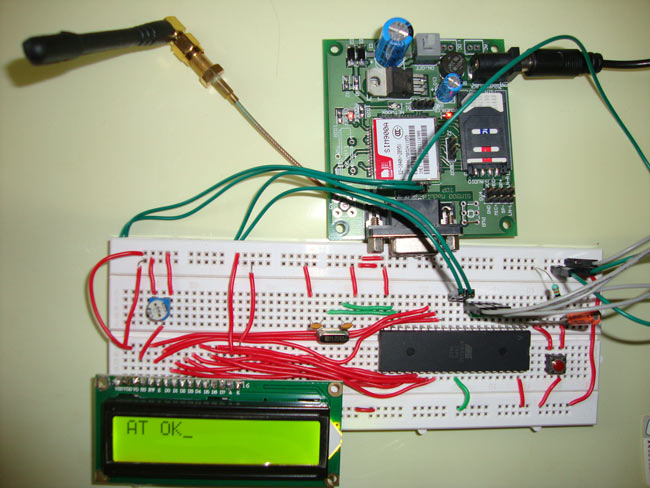
GSM module is used in many communication devices which are based on GSM (Global System for Mobile Communications) technology. It is used to interact with GSM network using a computer. GSM module only understands AT commands, and can respond accordingly. The most basic command is “AT”, if GSM respond OK then it is working good otherwise it respond with “ERROR”. There are various AT commands like ATA for answer a call, ATD to dial a call, AT+CMGR to read the message, AT+CMGS to send the sms etc. AT commands should be followed by Carriage return i.e. \r (0D in hex), like “AT+CMGS\r”. We can use GSM module using these commands.
GSM Interfacing with 8051
Instead of using PC, we can use microcontrollers to interact with GSM module and LCD to get the response from GSM module. So we are going to interface GSM with a 8051 microcontroller (AT89S52). It’s very easy to interface GSM with 8051, we just need to send AT commands from microcontroller and receive response from GSM and display it on LCD. We can use microcontroller’s serial port to communicate with GSM, means using PIN 10 (RXD) and 11 (TXD).
First we need to connect LCD to 8051, you can learn this from here: LCD Interfacing with 8051 Microcontroller. Then we need to connect GSM module to 8051, now here we should pay some attention. First you need to check that whether your GSM module is capable of working at TTL logic or it can only work with RS232. Basically if your module has RX and TX (with GND) Pins on board then it can work on TTL logic. And If it don’t have any RX,TX pins and only have a RS232 port (serial port with 9) then you need to use MAX232 IC to connect serial port to the microcontroller. Basically MAX232 used to convert serial data into TTL logic because Microcontroller can only work on TTL logic. But if GSM module has RX, TX pins then you don’t need to use MAX232 or any serial converter, you can directly connect RX of GSM to TX (PIN 11) of 8051 and TX of GSM to RX (PIN 10) of 8051. In our case I have used SIM900A module and it has RX, TX pins so I haven’t used MAX232.
Circuit Diagram for GSM interfacing with AT89S52 microcontroller is shown in above figure. Now after the connection, we just need to write program to send AT commands to GSM and receive its response on LCD. There are many AT commands as described above, but our scope of this article is just to interface GSM with 8051, so we are just going to send command “AT” followed by “\r” (0D in hex). This will give us a response “OK”. But you can extend this program to use all the facilities of GSM.
Code explanation
Besides all the LCD related functions, here we have used Serial port and timer mode register (TMOD). You can learn about LCD functions and other code by going through our 8051 projects section, here I am explaining about serial communication related code functions:
GSM_init() function:
This function is use to set the Baudrate for microcontroller. Baudrate is nothing but the Bits/second transmitted or received. And we need to match the baudrate of 8051 to the Baud rate of GSM module i.e. 9600. We have used the Timer 1 in Mode 2 (8-bit auto-reload mode) by setting the TMOD register to 0X20 and Higher byte of Timer 1(TH1) to 0XFD to get the baud rate of 9600. Also SCON register is used to set the mode of serial communication, we have used Mode1 (8-bit UART) with receiving enabled.
GSM_write Function:
SBUF (serial buffer special function register) is used for serial communication, whenever we want to send any byte to serial device we put that byte in SBUF register, when the complete byte has been sent then TI bit is set by hardware. We need to reset it for sending next byte. It’s a flag that indicates that byte has been sent successfully. TI is the second bit of SCON register. We have sent “AT” using this function.
GSM_read function:
Same as sending, whenever we receive any byte from external device that byte is put in SBUF register, we just need to read it. And whenever the complete byte has been received RI bit is set by hardware. We need to reset it for receiving next byte. RI is the first bit of SCON register. We have read response “OK” using this function.
Complete Project Code
#include<reg52.h>
#define display_port P2 //Data pins connected to port 2 on microcontroller
sbit rs = P3^2; //RS pin connected to pin 2 of port 3
sbit rw = P3^3; // RW pin connected to pin 3 of port 3
sbit e = P3^4; //E pin connected to pin 4 of port 3
int k;
unsigned char str[26];
void GSM_init() // serial port initialization
{
TMOD=0x20; // Timer 1 selected, Mode 2(8-bit auto-reload mode)
TH1=0xfd; // 9600 baudrate
SCON=0x50; // Mode 1(8-bit UART), receiving enabled
TR1=1; // Start timer
}
void msdelay(unsigned int time) // Function for creating delay in milliseconds.
{
unsigned m,n ;
for(m=0;m<time;m++)
for(n=0;n<1275;n++);
}
void lcd_cmd(unsigned char command) //Function to send command instruction to LCD
{
display_port = command;
rs= 0;
rw=0;
e=1;
msdelay(1);
e=0;
}
void lcd_data(unsigned char disp_data) //Function to send display data to LCD
{
display_port = disp_data;
rs= 1;
rw=0;
e=1;
msdelay(1);
e=0;
}
void lcd_init() //Function to prepare the LCD and get it ready
{
lcd_cmd(0x38); // for using 2 lines and 5X7 matrix of LCD
msdelay(10);
lcd_cmd(0x0F); // turn display ON, cursor blinking
msdelay(10);
lcd_cmd(0x01); //clear screen
msdelay(10);
lcd_cmd(0x80); // bring cursor to beginning of first line
msdelay(10);
}
void lcd_string(unsigned char *str) // Function to display string on LCD
{
int i=0;
while(str[i]!='\0')
{
lcd_data(str[i]);
i++;
msdelay(10);
if(i==15) lcd_cmd(0xc2);
}
return;
}
void GSM_write(unsigned char ch) // Function to send commands to GSM
{
SBUF=ch; // Put byte in SBUF to send to GSM
while(TI==0); //wait until the byte trasmission
TI=0; //clear TI to send next byte.
}
void GSM_read() // Function to read the response from GSM
{
while(RI==0); // Wait until the byte received
str[k]=SBUF; //storing byte in str array
RI=0; //clear RI to receive next byte
}
void main()
{
k=0;
lcd_init();
GSM_init();
msdelay(200);
lcd_string("Interfacing GSM with 8051");
msdelay(200);
lcd_cmd(0x01); // Clear LCD screen
msdelay(10);
GSM_write('A'); // Sending 'A' to GSM module
lcd_data('A');
msdelay(1);
GSM_write('T'); // Sending 'T' to GSM module
lcd_data('T');
msdelay(1);
GSM_write(0x0d); // Sending carriage return to GSM module
msdelay(50);
while(1)
{
GSM_read();
if(str[k-1]=='O' && str[k]=='K'){
lcd_data(0x20); // Write 'Space'
lcd_data(str[k-1]);
lcd_data(str[k]);
break;
}
k=k+1;
}
}
Comments
GSM module
no ok is displayed on my lcd. can you please help me with this
@Vineet and @Nithesh: Which
@Vineet and @Nithesh: Which GSM module are you using? You may need to use RS232 as explained in the Project.
I am using SIM900A module but
I am using SIM900A module but I can see only AT and there is no OK displayed. How will I know if the AT has reached the gsm module..? the gsm module's pcb I am using has a max232 embedded inside. pls help
nice job ...need to info.
nice job ...need to info. about sms sending and reaceving
Do I to attach an antenna to
Do I to attach an antenna to the SIM 900 module ?
Antenna comes with the GSM
Antenna comes with the GSM module, you need to attach.
Not getting OK
LCD get only AT not OK, sim900A GSM works fine on hyperterminal on PC but read unwanted text on lcd when i write code to read actual reply from gsm. please help me.
OK is not being displayed
OK is not being displayed because of the statement 'k=0'...k should be 1.
I am not getting OK. Pl help
I am not getting OK. Pl help me. I am using 8051 and gsm SIM900A
ABOUT PROJECT
i am not getting ok lcd screen can please help me?
You need to setup the network
You need to setup the network in gsm module.Once you insert your sim and power led is on give a long press to power button in gsm module.N/W led while start to blink indicating the network from your sim is established.Once it starts to blink leave the power button.MAKE SURE YOU DUMP THE CODE TO 8051 ONCE BEFORE CONNECTING RX AND TX WIRE.ONCE YOU DUMP DO CONNECTION.
STM32
I am working on my graduation project. So I have to interface a gsm modem with a stm32 ... But before I have to read and write in a usb key with micro usb card stm32. I use for this the keil and cubemx . I wrote a code. There is no error. but when I build it . I do not find the text file in the usb key. Any idea?
Software used to make schematic
Hi, wanna to know which software is used to make such a schematic, thanks
I think the software used
I think the software used here ISIS Proteus
How to send SMS amd make a call using 8051
Hi
you haven't explain about how to send message and make a call using 8051 MCU with GSM.
thank you for vidio