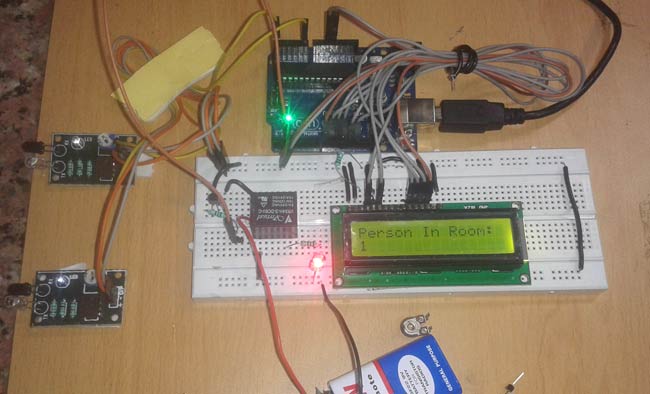
Often we see visitor counters at stadium, mall, offices, class rooms etc. How they count the people and turn ON or OFF the light when nobody is inside? Today we are here with automatic room light controller project with bidirectional visitor counter by using Arduino Uno. It is very interesting project for hobbyists and students for fun as well as learning.
Components
- Arduino UNO
- Relay (5v)
- Resisters
- IR Sensor module
- 16x2 LCD display
- Bread Board
- Connecting Wires
- Led
- BC547 Transistor
The project of “Digital visitor counter” is based on the interfacing of some components such as sensors, motors etc. with arduino microcontroller. This counter can count people in both directions. This circuit can be used to count the number of persons entering a hall/mall/home/office in the entrance gate and it can count the number of persons leaving the hall by decrementing the count at same gate or exit gate and it depends upon sensor placement in mall/hall. It can also be used at gates of parking areas and other public places.
This project is divided in four parts: sensors, controller, counter display and gate. The sensor would observe an interruption and provide an input to the controller which would run the counter increment or decrement depending on entering or exiting of the person. And counting is displayed on a 16x2 LCD through the controller.
When any one enters in the room, IR sensor will get interrupted by the object then other sensor will not work because we have added a delay for a while.
Circuit Explanation
There are some sections of whole visitor counter circuit that are sensor section, control section, display section and driver section.
Sensor section: In this section we have used two IR sensor modules which contain IR diodes, potentiometer, Comparator (Op-Amp) and LED’s. Potentiometer is used for setting reference voltage at comparator’s one terminal and IR sensors sense the object or person and provide a change in voltage at comparator’s second terminal. Then comparator compares both voltages and generates a digital signal at output. Here in this circuit we have used two comparators for two sensors. LM358 is used as comparator. LM358 has inbuilt two low noise Op-amp.
Control Section: Arduino UNO is used for controlling whole the process of this visitor counter project. The outputs of comparators are connected to digital pin number 14 and 19 of arduino. Arduino read these signals and send commands to relay driver circuit to drive the relay for light bulb controlling. If you find any difficulty in working with relay, check out this tutorial on arduino relay control to learn more about operating relay with Arduino.
Display section: Display section contains a 16x2 LCD. This section will display the counted number of people and light status when no one will in the room.
Relay Driver section: Relay driver section consist a BC547 transistor and a 5 volt relay for controlling the light bulb. Transistor is used to drive the relay because arduino does not supply enough voltage and current to drive relay. So we added a relay driver circuit to get enough voltage and current for relay. Arduino sends commands to this relay driver transistor and then light bulb will turn on/off accordingly.
Visitor Counter Circuit Diagram
The outputs of IR Sensor Modules are directly connected to arduino digital pin number 14(A0) and 19(A5). And Relay driver transistor at digital pin 2. LCD is connected in 4 bit mode. RS and EN pin of LCD is directly connected at 13 and 12. Data pin of LCD D4-D7 is also directly connected to arduino at D11-D8 respectively. Rest of connections are shown in the below circuit diagram.
Code Explanation
First we have included library for LCD and defined pin for the same. And also defined input output pin for sensors and ralay.
Then given direction to input output pin and initialized LCD in setup loop.
In loop function we read sensors input and increment or decrement the counting depending upon enter or exit operation. And also check for zero condition. Zero condition means no one in the room. If zero condition is true then arduino turn off the bulb by deactivating the relay through transistor.
And if zero condition is false then arduino turns on the light. Here is two functions for enter and exit.
Complete Project Code
#include<LiquidCrystal.h>
LiquidCrystal lcd(13,12,11,10,9,8);
#define in 14
#define out 19
#define relay 2
int count=0;
void IN()
{
count++;
lcd.clear();
lcd.print("Person In Room:");
lcd.setCursor(0,1);
lcd.print(count);
delay(1000);
}
void OUT()
{
count--;
lcd.clear();
lcd.print("Person In Room:");
lcd.setCursor(0,1);
lcd.print(count);
delay(1000);
}
void setup()
{
lcd.begin(16,2);
lcd.print("Visitor Counter");
delay(2000);
pinMode(in, INPUT);
pinMode(out, INPUT);
pinMode(relay, OUTPUT);
lcd.clear();
lcd.print("Person In Room:");
lcd.setCursor(0,1);
lcd.print(count);
}
void loop()
{
if(digitalRead(in))
IN();
if(digitalRead(out))
OUT();
if(count<=0)
{
lcd.clear();
digitalWrite(relay, LOW);
lcd.clear();
lcd.print("Nobody In Room");
lcd.setCursor(0,1);
lcd.print("Light Is Off");
delay(200);
}
else
digitalWrite(relay, HIGH);
}
Comments
Sir, how will the code change
Sir, how will the code change accordingly if I use microcontroller 8051 in place of aurdino board.please suggest me if there is modification needed on this program.
did this project work i want
did this project work i want the complete data of this project
Updatet version
hey i created a ne verison ofyour code just place it into your exciting code ..it will count the people WARNING the pins i used for the sensors ar 3 and 4 . 3 is the one you pass first wen you go in :
boolean sensor1 = false ;
boolean sensor2 = false ;
int count = 0 ;
void setup() {
// put your setup code here, to run once:
pinMode(3, INPUT);
pinMode(4, INPUT);
pinMode(2, OUTPUT);
}
void loop() {
// put your main code here, to run repeatedly:
delay (200);
sensor();
if (sensor1 == true) {
S1();
}
//if (sensor2 == true){
// S2();
//}
}
void sensor() {
if (digitalRead(3) == HIGH) {
sensor1 = true;
}
else if (digitalRead(3) == LOW) {
sensor1 = false;
}
if (digitalRead(4) == HIGH) {
sensor2 = true;
}
else if (digitalRead(4) == LOW) {
sensor2 = false;
}
else {
loop();}
}
void S1() {
while (sensor1 == true && sensor2 == false) {
sensor();
}
if (sensor1 == false && sensor2 == false) {
}
else if (sensor1 == true && sensor2 == true) {
S1S2();
}
else {
loop();
}
}
void S1S2() {
while (sensor1 == true && sensor2 == true) {
sensor();
}
if (sensor1 == false && sensor2 == true){
S2F();
}
else if (sensor1 == true && sensor2 == false){
S1();
}
else {
loop();
}
}
void S2F() {
while (sensor2 == true && sensor1 == false){
sensor();
}
if (sensor2 == false && sensor1 == false){
count = count + 1;
loop();
}
else if (sensor1 == true && sensor2 == true){
S1S2();
}
else {
loop();
}
}
void S2() {
while (sensor2 == true && sensor1 == false) {
sensor();
}
if (sensor1 == false && sensor2 == false) {
}
else if (sensor1 == true && sensor2 == true) {
S2S1();
}
else {
loop();
}
}
void S2S1() {
while (sensor2 == true && sensor1 == true) {
sensor();
}
if (sensor2 == false && sensor1 == true){
S1F();
}
else if (sensor2 == true && sensor1 == false){
S2();
}
else {
loop();
}
}
void S1F() {
while (sensor1 == true && sensor2 == false){
sensor();
}
if (sensor1 == false && sensor2 == false){
count = count - 1;
loop();
}
else if (sensor2 == true && sensor1 == true){
S1S2();
}
else {
loop();
}
}
same circuit is sufficient for your program??
do the same circuit sufficient for your new program???
Sir what exactly does your
Sir what exactly does your modified code do? And how did you implement it physically like did you place two sensors at the same door at some distance to track the count? Also, can the sensors be deployed vertically on the ceiling instead of horizontally?
And how did you implement it
And how did you implement it physically like did you place two sensors at the same door at some distance to track the count?
No the code is already fully functional. You just have to build it on your own
Also, can the sensors be deployed vertically on the ceiling instead of horizontally?
Yes you can mount it vertically also it will work. But it will not suit the application
i need your help man
i am aren't able to understand your code . please can you add the fuctionality of lcd so that the counted person can be displayed on lcd . please i neeed it as early as possible because i am giong to sumbit my project within this week at uni
Where is the program for LCD
Where is the program for LCD display
if two persons enter at the
if two persons enter at the time, can this circuit count the correct number.
10 in the program
pls check and send the full program and step by step process in cricuit diagram how to fix in everything
IN RELAY NO OR NC WHICH IS
IN RELAY NO OR NC WHICH IS GIVEN TO GROUND PLEASE GIVE ME REPLY URGENTLY
Hi, wonderful project idea
Hi, wonderful project idea and demonstration, I have bought all the required components, however I wish to use LED or an array of LEDs in stead of 220v AC bulb, what should I do to make it work? Like in your video I see a relay is attached to the breadboard, however I am bit confused about LED, should I directly connect LED in place of bulb(bad idea?) or relay?
Learn More about how to use
@Andrews @BRAHMAREDDY @Abhishek: Learn More about how to use relay here.
hello guys
hello guys
is there is any way to set arduino to count when the sensor didnt get any signal
I mean exactly On the contrary of this program when the IR module didnt get any IR signal instead of getting signal ?
please help
problem in the connection of relay with load.
hi....am ajit ...i almost done this project according to your above details...but unfortunately there is a problem.i did not able connect the relay with load ....plz give some idea about it.
Please read all the previous
Please read all the previous comments before asking questions, we might have answered them already.
Thanks
If you copied and pasted the
If you copied and pasted the code that is provided in this project and had a problem where the counter increases without any object passing through the ir module and when it passes the counter stope adjust the code like this:
if(digitalRead(in))
IN();
if(digitalRead(out))
OUT();
// will be like this:
while(digitalRead(in) == LOW){
IN();
break;}
if(digitalRead(out) == LOW){
OUT();
break;}
// define in as pin 3 and out as pin 4 on digital pins
thank you for this project
thank you for this project sir. but i have a little bit of problem the counting that I saw is not stable it increases the original count and decreases immediately in every second..how will i fix it sir..thanks in advance...
Concern abount your project
do you know whatvis the maximum range og this ir sensors?
Normally the range of IR LED
Normally the range of IR LED is 2 meter, but it can be increased by using Lens.
visitor counter using pic
Can you please give me a suggestion for how to make the same code for pic?
can you provide me a code and
can you provide me a code and circuit diagram for atmega16
this program is not working its show error in compilation ????
this program is not working its show error in compilation ????
bi-directional visitor counter
I have created a new version on this project for my first semester final project. Intially idea i get from this web then worked on it finally i made it . My version is more sensible and i have used direct incidence of ir sensor . These direct incidence sensor could be made at home very easily . if any body want any help regarding this project he may ask.
request code for automatic room light controller using arduino
Sir,
As you expressed to help us,please provide your modified arduino code for automatic room light controller using arduino and seven segment display.
thanks in advance. may god bless you
Sir I dont have IR sensor in
Sir I dont have IR sensor in protues simulation
hello guys
i want to use 3 relays in a program to control more lights but i have used one relay and i want u help me to add them in program help me please
the program is below
#include<LiquidCrystal.h>
LiquidCrystal lcd(13,12,11,10,9,8);
#define in 14
#define out 19
#define relay 2
int count=0;
void IN()
{
count++;
lcd.clear();
lcd.print("Person In Room:");
lcd.setCursor(0,1);
lcd.print(count);
delay(1000);
}
void OUT()
{
count--;
lcd.clear();
lcd.print("Person In Room:");
lcd.setCursor(0,1);
lcd.print(count);
delay(1000);
}
void setup()
{
lcd.begin(16,2);
lcd.print("Visitor Counter");
delay(2000);
pinMode(in, INPUT);
pinMode(out, INPUT);
pinMode(relay, OUTPUT);
lcd.clear();
lcd.print("Person In Room:");
lcd.setCursor(0,1);
lcd.print(count);
}
void loop()
{
if(digitalRead(in))
IN();
if(digitalRead(out))
OUT();
if(count<=0)
{
lcd.clear();
digitalWrite(relay, LOW);
lcd.clear();
lcd.print("Nobody In Room");
lcd.setCursor(0,1);
lcd.print("Light Is Off");
delay(200);
}
else
digitalWrite(relay, HIGH);
}
circuit diagram
please send me interfacing assemble video of this project
regarding change of sensor
Sir I am in need of using the sensor range for about 3-10 ft for sensing range. So I have decided to use ultrasonic sensor for that range of measurement. Can i use ultrasonic sensor for this project? if not can you suggest me any other sensor?
while doing this experiment
while doing this experiment the problem that i am facing is in my lcd continuously no. of person is getting changed in absence of any input.
That means when i bring my hands near to the either of the sensors it performs according to the porgram but the moment i am removing my hand(that means there is nothing to be sensed) lcd starts showing an infinite loop of 0 and 1 and it continues untill i again bring my hand near sensor.
please give me the solution.
code doubt
i have checked the connections .. they are correct..
when i run the code , the screen displays 'no. of persons in the room',
but when i give an input like move my hand across the sensor, the screen goes blank and comes back on when i remove my hand..
plz tell me what to do..
i have a week before i have to submit the project and its too late to change it.
i appreciate all the help i can get.
Hi. You've shown the
Hi. You've shown the connection of Sensors Output to Analog pins of Arduino. But in code, you've used digitalRead. Shouldn't it be analogRead because its connected to Analog pins. Thanks
I did it and it works very
I did it and it works very well.
But the sensors you used are small range detector.
How much Your sensors range can detect?
Please give the whole code
Please give the whole code for this project.
what are the softwares used
what are the softwares used in this project
regarding code
hello admin is this the correct code or we need to alter something??????
reply soon plz
Sensor counting - urgent
I am new to Arduino. I have given the same circuit connection and uploaded the program. but the counter is changing between 0 and 1 automatically. Where i have done mistake. how to find?
The Red led on my sensor
The Red led on my sensor module is always on standby. when we put our hands near the module the LED BLINKS AND AGAIN TURN ON. Some times the number of persons simply goes on increasing or decreasing. I doubt it is not working according to the sensor input.
Please look into my problem, I want to submit the project within 2 days.
code not working
please send me the corrected code which worked for you.
The red colour led of my ir
The red colour led of my ir sensor module is always turned on, and when i move my hands infront the led blinks and again turn on. what change should i make in coding. Here is my coding
#include<LiquidCrystal.h>
LiquidCrystal lcd(13,12,11,10,9,8);
#define in 14
#define out 19
#define relay 2
int count=0;
void IN()
{
count++;
lcd.clear();
lcd.print("Person In Room:");
lcd.setCursor(0,1);
lcd.print(count);
delay(1000);
}
void OUT()
{
count--;
lcd.clear();
lcd.print("Person In Room:");
lcd.setCursor(0,1);
lcd.print(count);
delay(1000);
}
void setup()
{
lcd.begin(16,2);
lcd.print("Visitor Counter");
delay(2000);
pinMode(in, INPUT);
pinMode(out, INPUT);
pinMode(relay, OUTPUT);
lcd.clear();
lcd.print("Person In Room:");
lcd.setCursor(0,1);
lcd.print(count);
}
void loop()
{
if(digitalRead(in))
IN();
if(digitalRead(out))
OUT();
if(count<=0)
{
lcd.clear();
digitalWrite(relay, LOW);
lcd.clear();
lcd.print("Nobody In Room");
lcd.setCursor(0,1);
lcd.print("Light Is Off");
delay(200);
}
else
digitalWrite(relay, HIGH);
}
Automatic Room Light Controller with Bidirectional Visitor Count
Output light keeps blinking even when there is no count... please help
I have connected a Led
I have connected a Led instead of the Relay but the Led Keeps blinking even when there is no count.
When the count is one or more the Led blinks in a fast rate. Please help
The count keeps varying ...
The count keeps varying ... When the count is 1 the arduino count keeps varying between 1 and 0 . So the output relay keeps turning ON and OFF. Same thing occurs when the count is two the count keeps varying between 2 and 1
Please help!!
Hi Praneeth, the problem is
Hi Praneeth, the problem is most likely because your IR module is making a false trigger. Use the Serial monitor to check which creates a false trigger. Maybe a pull down resistor or 10K to the signal pin or IR module should solve the problem
Can we add PIR Sensor along
Can we add PIR Sensor along with IR sensor to this project. IR sensor for counting the persons and PIR Sensor for turning on the light on particular area in a large area/hall. So can we use PIR And IR sensor in this project. If it is possible can you explain me how can we do it.
Problem solved
Thank you Aisha, Your idea was the solution, where the threshold of the IR input was crossing continuously. Adding 10 K resistor in series with the IR sensor worked.
Thanks again
by the way great project and thanks for everyone contributing to this.
Sir , i need the entire
Sir , i need the entire circuit of above showed video of automatic room light controller.
Will u please say about the maximum distance provided between 2 ir modules.
And also gave the connection to the 5v relay .
I am waiting for ur reply
please is the IR the same
please is the IR the same thing with IR proximity sensor? i did search it online in our local electronic store that is what the are giving to me
Yes khalees you can also use
Yes khalees you can also use an IR proximity sensor module it will work like the same
further development doubts
how can i send the data of no. of persons in the room using mobile phone?
how can i add a limit for no. of persons to enter into the room and also voice alarm if count exceeds?
Please reply me as soon as possible
Use a bluetooth module like
Use a bluetooth module like HC-05 and send the data over to your phone. You can record a voice alarm audio into a SD card and play it from arduino when ever the count exceeds a particular value
bidirectional visitor counter basic interview questions
How to count bidirectional.......I.e. I count 12 then how to reduce 11 if I exit .....Because same LDR used to in or out.......
They are not LDR, it is IR
They are not LDR, it is IR sensor. Read the complete explanation patiently.
The project could count bi-directional because the author has used two IR sensor. So based on the sequence we can tell is the person is entering the room or exiting the room
The code count the also
The code count the also update the person in negative value so try this code.
#include<LiquidCrystal.h>
LiquidCrystal lcd(13,12,11,10,9,8);
#define in 14
#define out 19
#define relay 2
int count=0;
void IN()
{
count++;
lcd.clear();
lcd.print("Person In Room:");
lcd.setCursor(0,1);
lcd.print(count);
delay(1000);
}
void OUT()
{
count--;
lcd.clear();
lcd.print("Person In Room:");
lcd.setCursor(0,1);
lcd.print(count);
delay(1000);
}
void setup()
{
lcd.begin(16,2);
lcd.print("Visitor Counter");
delay(2000);
pinMode(in, INPUT);
pinMode(out, INPUT);
pinMode(relay, OUTPUT);
lcd.clear();
lcd.print("Person In Room:");
lcd.setCursor(0,1);
lcd.print(count);
}
void loop()
{
if(digitalRead(in))
IN();
if(digitalRead(out))
OUT();
if(count<=0)
{
count=0;
lcd.clear();
digitalWrite(relay, LOW);
lcd.clear();
lcd.print("Nobody In Room");
lcd.setCursor(0,1);
lcd.print("Light Is Off");
delay(200);
}
else
digitalWrite(relay, HIGH);
}
MULTIPLE LED'S
my problem is... when (<=1) person enters the room , one led should lights up. when (<=2) person enters the room , two led should lights up...
can anyone plz provide me code over this.......
Problem in LCD display
I have trouble in output in LCD display plz advice me connection properly .
And give me proper correct Codeing
Plz help me sir
LCD DISPLAY PROBLEM
My lcd display is not getting output it is seen blank.not a seen count or any output in lcd display pls help me what is solution of this.
Problem in Lcd diplay output
Problem in Lcd diplay output.nothing getting cursor in lcd.
Check your connection, may be
Check your connection, may be the contrast of the LCD is not set properly. Many people get it wrong the first time
I made this project but there
I made this project but there some prblm...relay is not operated automatically ...and major prblm is that if we run the code then LCD will shows everything but there fluctuation in counting 1st count 1 and if are goes 2nd time it will show 2 but then 1 2 1 2 fluctuates please send perfect code ....i have a very less time ...to submit my project ...
LCD not showing count
I've made all proper connections and even the LED's and sensors are working perfectly, but the LCD doesn't show anything even though it is ON.
wrong circuit diagram
You have connected those input of ir sensor to analog pins in circuit diagram but according to your code explanation it should be connected to digital pins
Components list
Sir Send me the total components list with there values and how many components are need.
Can you post a video about
Can you post a video about all the connections in detail ?
Code modifications made by me
This is my first Arduino project attempt. I've tried to reduce some part of the code to eliminate the LCD display part. Can anyone tell if the following code is correct?
#define in 14
#define out 19
#define relay 2
int count=0;
void IN()
{
count++;
delay(1000);
}
void OUT()
{
count--;
delay(1000);
}
void setup()
{
pinMode(in, INPUT);
pinMode(out, INPUT);
pinMode(relay, OUTPUT);
}
void loop()
{
if(digitalRead(in))
IN();
if(digitalRead(out))
OUT();
if(count<=0)
digitalWrite(relay, LOW);
else
digitalWrite(relay, HIGH);
}
related to lcd couldnot show any thing
sir the project making this cant be work
lcd show blank screen
bulb continously on and
sensors did not work properly
sir please help me immediately
Pls i need help modifying the
Pls i need help modifying the code. is there a way to make the relay and break the relay such as activating a push button switch to control RF light source
if(count<=0)
{
count=0;
lcd.clear();
digitalWrite(relay, HIGH); Then low again to simulate a push control.
else
digitalWrite(relay, HIGH); Then low again to simulate a push control.
thanks
Request to get the "correct" source code
Sir pls send me the "correct" or updated source code for counting the people. I try the source code that mentions in the previous source code but it won't works.
The issue shows counting the people 0 and 1 before count the people
sir, if you have video abot
sir, if you have video abot how to connect the circuit. kindly please send. arn24680@gmail.com
Sir plz send me correct code ,no ic arduino uno