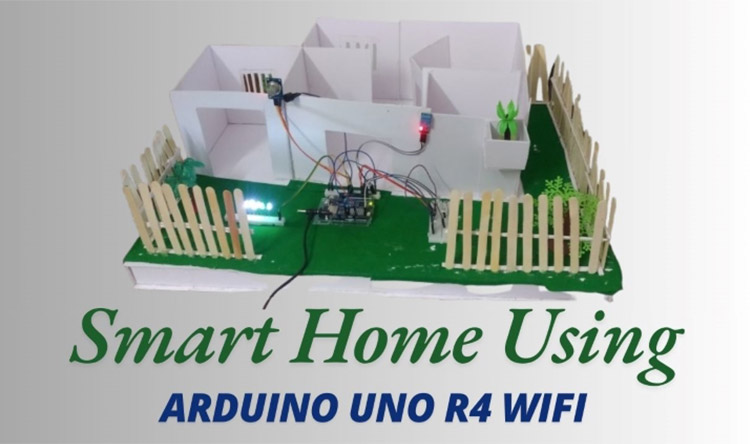
Our Smart Home Using Arduino Uno R4 WiFi project is designed for home safety and convenience, integrating temperature, humidity, light, and gas monitoring. Using sensors like DHT11/DHT22, LDR, and MQ-2 gas sensor, the system detects environmental changes and alerts users through LEDs and a piezo buzzer when thresholds are exceeded, such as harmful gas levels or insufficient lighting.
Motivated by the need for a cost-effective, accessible smart home solution, this setup enables remote monitoring via Arduino Uno R4 WiFi, allowing users to respond promptly to any issues. We’re particularly proud of its adaptability: users can start with essential functions and expand based on household needs, making it a scalable, modular solution that adds significant value to home safety and comfort.
Impact Statement
Our Smart Home Using Arduino Uno R4 WiFi project is designed for home safety and convenience, integrating temperature, humidity, light, and gas monitoring. Using sensors like DHT11/DHT22, LDR, and MQ-2 gas sensor, the system detects environmental changes and alerts users through LEDs and a piezo buzzer when thresholds are exceeded, such as harmful gas levels or insufficient lighting.
Motivated by the need for a cost-effective, accessible smart home solution, this setup enables remote monitoring via Arduino Uno R4 WiFi, allowing users to respond promptly to any issues.
We’re particularly proud of its adaptability: users can start with essential functions and expand based on household needs, making it a scalable, modular solution that adds significant value to home safety and comfort.
Project Description
This smart home automation project is designed to enhance convenience, safety, and energy efficiency in everyday living spaces. Built using an Arduino R4 WiFi module and various sensors, the system provides real-time monitoring and remote control via the Blynk app. This project is a contribution to the Circuit Digest competition, and the Arduino R4 WiFi was sourced from DigiKey, adding reliable connectivity and functionality.
Key Features
Temperature and Humidity Monitoring: The system uses a DHT11 sensor to measure and report temperature and humidity levels. This data is sent to the Blynk app, enabling remote monitoring to ensure comfortable and stable room conditions, even from a distance.
Automatic Lighting Control: With an LDR (Light Dependent Resistor) sensor, the system automatically adjusts the brightness of garden lights based on ambient lighting. This ensures energy savings during daylight hours and efficient illumination at night, which is ideal for outdoor spaces.
Gas Detection and Alert System: Equipped with an MQ2 gas sensor, the system can detect smoke or gas leaks and immediately sound an alarm via a buzzer. This feature is designed to enhance safety, especially in areas prone to gas leakages, such as kitchens.
LED Matrix Display for Notifications: The LED matrix can display custom scrolling text, providing a versatile way to show alerts, environmental conditions, or greetings. It adds a layer of personalization and functionality, making it a unique feature in the automation system.
Remote Control And Monitoring Via Blynk App
Through the Blynk app, users can control individual lights, monitor sensor readings in real time, and receive alerts if hazardous gas levels are detected. This remote functionality makes the system flexible and accessible, even when users are away from home.
Components Used
1. Arduino Uno R4 WiFi
2. DHT11 sensor (temperature and humidity)
3. LDR (Light Dependent Resistor) (for light sensing)
4. MQ-2 gas sensor (for smoke/gas detection)
5. piezo Buzzer
6. LEDs (for indication)
7. Resistors (as needed)
8. Breadboard and jumper wires
9. Power supply
To see the full demonstration video, click on the YouTube Video below.
Circuit Diagram With Explanation
Let's go through the circuit setup for our home automation project step by step.
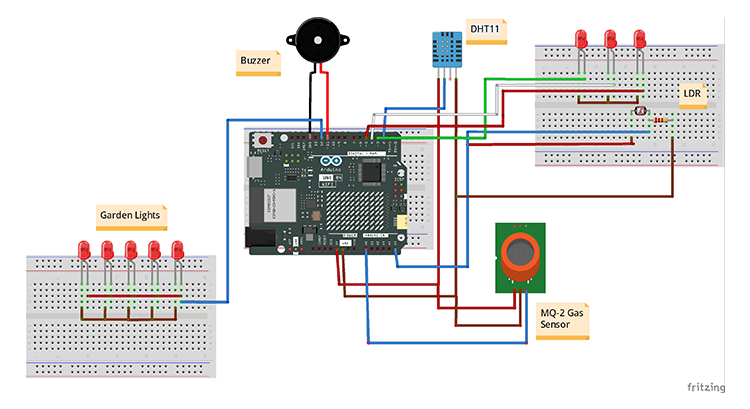
This project connects various sensors and outputs to the Arduino R4 WiFi, which acts as the “brain” of the system. Here’s a breakdown of each component and its connections:
1.Arduino R4 WiFi:
This is the main controller of the project. It’s connected to the internet via WiFi, allowing us to control and monitor the system remotely using the Blynk app.
2.DHT11 Temperature and Humidity Sensor:
This sensor measures temperature and humidity in the environment. It has three pins:
VCC: Connect this to the 5V pin on the Arduino.
GND: Connect this to the ground (GND) pin on the Arduino.
Data Pin: Connect the data pin to digital pin D2 on the Arduino. This pin reads the temperature and humidity data, which we then send to the Blynk app.
3.LDR Sensor (Light Dependent Resistor):
The LDR measures light levels, allowing the system to control garden lighting automatically.
The LDR is connected to analog input pin A5 on the Arduino, where it measures the light level and adjusts the garden light brightness accordingly.
4.MQ2 Gas Sensor:
This sensor detects the presence of gases like smoke or LPG, helping to increase home safety.
It has three main pins:
VCC: Connect to 5V.
GND: Connect to ground.
Analog Output Pin: Connect to A0 on the Arduino. This pin sends a signal to the Arduino if gas is detected, activating the alert system.
5.LED Lights (Green, White, Red):
These LEDs serve as indicators for various statuses, and each one is connected to a different digital pin:
Green LED: Connected to pin D3.
White LED: Connected to pin D4.
Red LED: Connected to pin D5.
By sending signals to these pins, the Arduino can turn each LED on or off, which we can also control remotely through the app.
6.Garden Light:
The garden light is connected to D12 on the Arduino and is controlled automatically by the LDR sensor to save energy and provide lighting only when needed.
7.Buzzer:
The buzzer serves as an alarm in case of gas detection.
Connect the buzzer’s positive pin to digital pin D11 and the negative pin to ground. The Arduino activates the buzzer if it detects a high gas level, warning everyone nearby.
8.LED Matrix:
The LED matrix is a small display that can show scrolling messages, like project information or sensor alerts.
This is controlled by the Arduino through specific communication pins, which vary based on your exact model. It’s a fun way to make the system interactive and visually informative.
9.Power and Ground Connections:
To keep everything running, The system is powered by a DC adapter (5V-9V) and a lithium battery (3.7V).
Smart Home Using Arduino Uno R4 WiFi Code Explanation
This code automates various devices in a smart home setup using the Arduino R4, the Blynk app, and several sensors. We’ll go through each section to explain how it all works together.
1. Libraries and Essential Definitions
#define BLYNK_PRINT Serial
#define BLYNK_TEMPLATE_ID "..."
#define BLYNK_TEMPLATE_NAME "Arduino R4"
#define BLYNK_AUTH_TOKEN "..."
#include <SPI.h>
#include <WiFiS3.h>
#include <BlynkSimpleWifi.h>
#include <DHT.h>
#include "ArduinoGraphics.h"
#include "Arduino_LED_Matrix.h"
ArduinoLEDMatrix matrix;
DHT dht(2, DHT11);
Blynk: This library enables remote control of devices and monitoring of sensors through the Blynk mobile app.
WiFiS3: This library allows the Arduino R4 to connect to WiFi, making it possible to send data and control devices remotely.
DHT: This library is for interfacing with the DHT11 sensor to read temperature and humidity.
ArduinoLEDMatrix: This controls the LED matrix to display messages or alerts.
2. WiFi Credentials and Pin Setup
char ssid[] = "RKranjan";
char pass[] = "********";
#define GREEN_LIGHT 3
#define WHITE_LIGHT 4
#define RED_LIGHT 5
#define LDR_SENSOR A5
#define GARDEN_LIGHT 12
#define MQ2_PIN A0
#define BUZZER_PIN 11
WiFi Credentials: These are used to connect the Arduino to your WiFi network, ensuring internet access for remote control via Blynk.
Pin Setup: Each component, such as the lights, sensors, and buzzer, is assigned to specific pins on the Arduino.
3. Setting Up Virtual Pins for Blynk
#define VIRTUAL_PIN_TEMPERATURE V1
#define VIRTUAL_PIN_HUMIDITY V2
float sensorValue;
Virtual Pins: Virtual pins are used to send data (temperature and humidity) from the Arduino to the Blynk app.
4. setup() Function
void setup() {
Serial.begin(115200);
pinMode(GREEN_LIGHT, OUTPUT);
pinMode(WHITE_LIGHT, OUTPUT);
pinMode(RED_LIGHT, OUTPUT);
pinMode(GARDEN_LIGHT, OUTPUT);
dht.begin();
WiFi.begin(ssid, pass);
Blynk.config(BLYNK_AUTH_TOKEN);
matrix.begin();
matrix.beginDraw();
matrix.stroke(0xFFFFFFFF);
matrix.textScrollSpeed(100);
matrix.textFont(Font_4x6);
matrix.beginText(0, 1, 0xFFFFFF);
matrix.println(" UNO r4 ");
matrix.endText(SCROLL_LEFT);
matrix.endDraw();
}
Initializes serial communication, pin modes, sensors, and connects the Arduino to WiFi and the Blynk app.
Displays a welcome message on the LED matrix.
5. loop() Function
void loop() {
if (WiFi.status() == WL_CONNECTED && !Blynk.connected()) {
Blynk.connect(2000);
}
if (Blynk.connected()) {
Blynk.run();
}
readDHTSensor();
updateGardenLight();
readGasSensor();
scrollMatrixText();
}
This is the main loop that continuously checks for internet connectivity, updates sensor data, controls devices, and updates the display.
6. Temperature and Humidity Monitoring readDHTSensor()
void readDHTSensor() {
static unsigned long lastReadTime = 0;
if (millis() - lastReadTime > 2000) {
lastReadTime = millis();
float humidity = dht.readHumidity();
float temperature = dht.readTemperature();
if (!isnan(humidity) && !isnan(temperature)) {
Serial.print("Temperature: ");
Serial.print(temperature);
Serial.print(" °C, Humidity: ");
Serial.println(humidity);
Blynk.virtualWrite(VIRTUAL_PIN_TEMPERATURE, temperature);
Blynk.virtualWrite(VIRTUAL_PIN_HUMIDITY, humidity);
} else {
Serial.println("Failed to read from DHT sensor!");
}
}
}
Reads the DHT11 sensor every 2 seconds to get temperature and humidity.
If readings are successful, they’re sent to the Blynk app and displayed in the Serial Monitor.
If unsuccessful, it shows an error message.
7. Garden Light Control updateGardenLight()
void updateGardenLight() {
int adc_val = 255 - (analogRead(LDR_SENSOR) / 4);
analogWrite(GARDEN_LIGHT, adc_val);
}
Light Control Based on LDR: Checks the light level around the garden light. If it’s dark, the garden light turns on; if it’s bright, it dims or turns off.
LDR Sensor Reading: Measures light intensity, so the garden light can automatically adjust its brightness based on the surrounding light.
8. Gas Sensor for Smoke Detection readGasSensor()
void readGasSensor() {
sensorValue = analogRead(MQ2_PIN);
Serial.print("Gas Sensor Value: ");
Serial.print(sensorValue);
if (sensorValue > 200) {
Serial.print(" | Smoke detected!");
playEmergencySiren();
} else {
noTone(BUZZER_PIN);
}
Serial.println("");
}
Gas Sensor: Monitors air quality. If smoke or dangerous gas levels exceed 200, it triggers an alert.
Emergency Alert: If smoke is detected, it calls playEmergencySiren() to sound an alarm.
9. Emergency Siren playEmergencySiren()
void playEmergencySiren() {
for (int freq = LOW_FREQ; freq <= HIGH_FREQ; freq += 10) {
tone(BUZZER_PIN, freq);
delayMicroseconds(FADE_TIME * 1000);
}
delayMicroseconds(HOLD_TIME * 1000);
for (int freq = HIGH_FREQ; freq >= LOW_FREQ; freq -= 10) {
tone(BUZZER_PIN, freq);
delayMicroseconds(FADE_TIME * 1000);
}
delayMicroseconds(HOLD_TIME * 1000);
}
Creates a siren sound pattern by gradually changing frequencies to alert the user of a potential emergency.
The tone() function is used to create varying frequencies, mimicking a siren, which continues until the gas levels return to normal.
10. Scrolling Text on Matrix scrollMatrixText()
void scrollMatrixText() {
static unsigned long lastScrollTime = 0;
if (millis() - lastScrollTime > 500) {
lastScrollTime = millis();
matrix.beginDraw();
matrix.stroke(0xFFFFFFFF);
matrix.textScrollSpeed(50);
matrix.textFont(Font_5x7);
matrix.beginText(0, 1, 0xFFFFFF);
matrix.println(" Scrolling text! ");
matrix.endText(SCROLL_LEFT);
matrix.endDraw();
}
}
Scrolls a message across the LED matrix.
Displays project title on the LED matrix every 500 milliseconds, creating a dynamic visual effect.
11. Remote Light Control with Blynk (BLYNK_WRITE)
BLYNK_WRITE(V0) {
digitalWrite(GREEN_LIGHT, param.asInt());
}
BLYNK_WRITE(V4) {
digitalWrite(WHITE_LIGHT, param.asInt());
}
BLYNK_WRITE(V3) {
digitalWrite(RED_LIGHT, param.asInt());
}
Lights remotely controlled through the Blynk app and webDashboard.
Users can turn on or off each light by toggling buttons on the Blynk app, which sends signals to the Arduino to control the respective lights.
Demonstration Of The IoT-Enabled Home Automation System
1. Hardware Setup
Arrange the components as shown in the circuit diagram, ensuring that each sensor and light is connected to the correct pins.
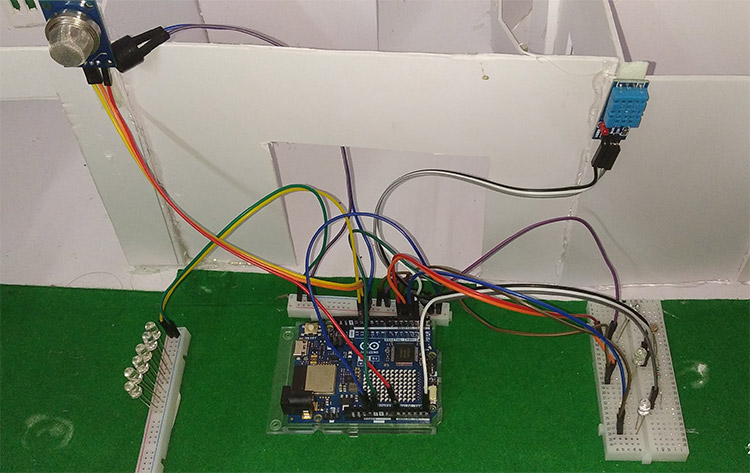
2. Connecting To The Blynk App
Blynk Setup Steps:
1. Open Blynk App and create a new project.
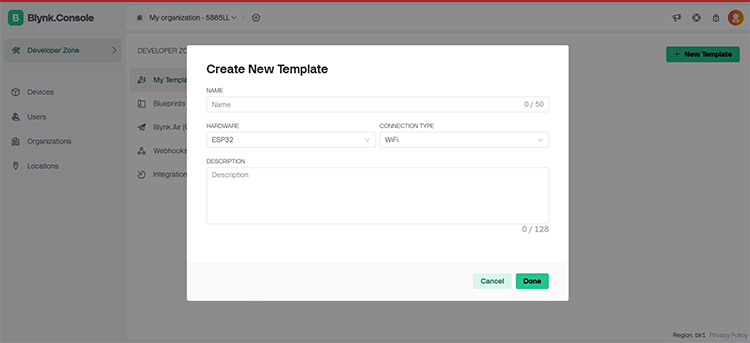
2. Select Arduino R4 WiFi as the board.
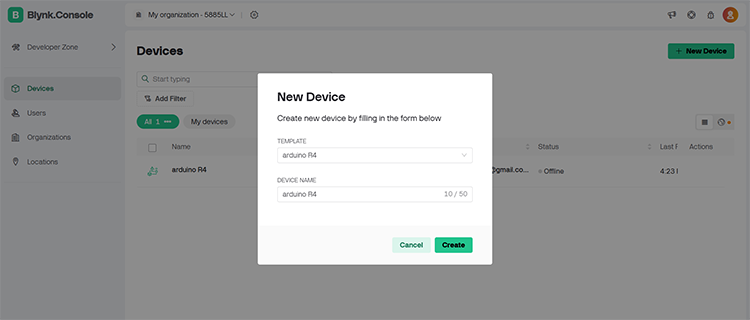
3. Add Widgets for temperature, humidity, and light control.
4. Assign Virtual Pins in the app to match those defined in the code (e.g., V1 for temperature, V0 for lights).
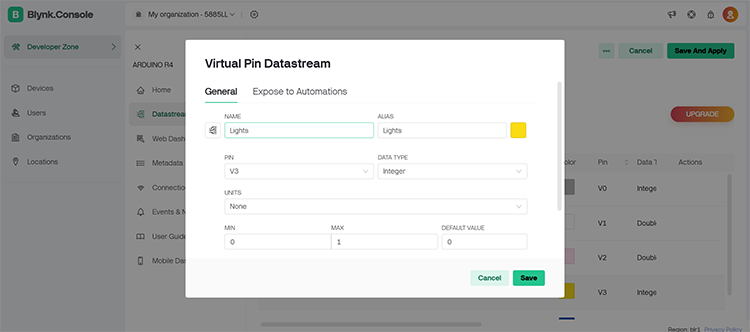
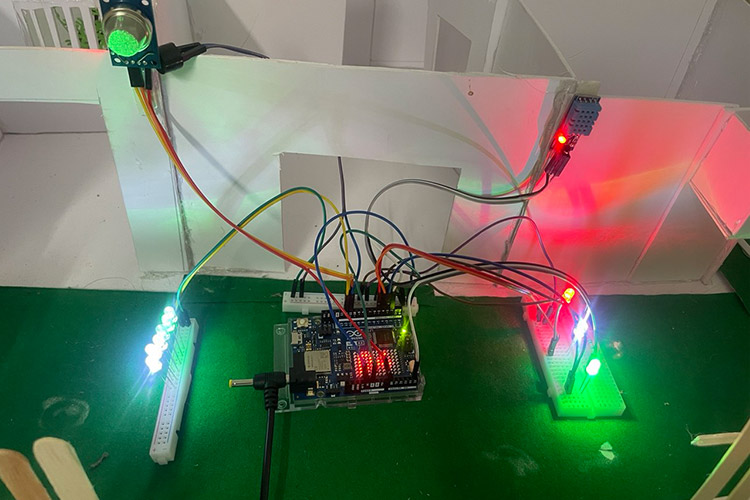
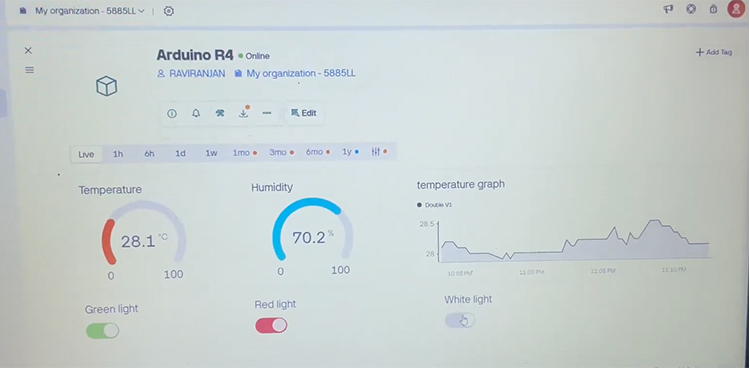
Complete Project Code
#define BLYNK_PRINT Serial // Define Blynk print for Serial Monitor output
#define BLYNK_TEMPLATE_ID "**********" // Blynk template ID
#define BLYNK_TEMPLATE_NAME "Arduino R4" // Blynk template name
#define BLYNK_AUTH_TOKEN "qrxKXXIYXy_7C9dWLfqRs7-ETsPwn60w" // Blynk authorization token
#include // Include SPI library
#include // Include WiFi library for S3
#include // Include Blynk library for WiFi
#include // Include DHT sensor library
#include "ArduinoGraphics.h" // Include graphics library for Arduino
#include "Arduino_LED_Matrix.h" // Include LED matrix library
ArduinoLEDMatrix matrix; // Create an instance of the LED matrix
DHT dht(2, DHT11); // Create a DHT sensor instance on pin 2
char ssid[] = "RKranjan"; // WiFi SSID
char pass[] = "********"; // WiFi password
#define GREEN_LIGHT 3 // Define pin for green light
#define WHITE_LIGHT 4 // Define pin for white light
#define RED_LIGHT 5 // Define pin for red light
#define LDR_SENSOR A5 // Define pin for LDR sensor
#define GARDEN_LIGHT 12 // Define pin for garden light
#define MQ2_PIN A0 // Define pin for MQ2 gas sensor
#define BUZZER_PIN 11 // Define pin for buzzer
const int LOW_FREQ = 600; // Define low frequency for the buzzer
const int HIGH_FREQ = 1200; // Define high frequency for the buzzer
const int FADE_TIME = 10; // Define fade time for frequency change
const int HOLD_TIME = 200; // Define hold time for frequency
#define VIRTUAL_PIN_TEMPERATURE V1 // Define virtual pin for temperature
#define VIRTUAL_PIN_HUMIDITY V2 // Define virtual pin for humidity
float sensorValue; // Variable to store sensor value
void setup() {
Serial.begin(115200); // Start serial communication at 115200 baud rate
pinMode(GREEN_LIGHT, OUTPUT); // Set green light pin as output
pinMode(WHITE_LIGHT, OUTPUT); // Set white light pin as output
pinMode(RED_LIGHT, OUTPUT); // Set red light pin as output
pinMode(GARDEN_LIGHT, OUTPUT); // Set garden light pin as output
dht.begin(); // Initialize DHT sensor
WiFi.begin(ssid, pass); // Start WiFi connection
Blynk.config(BLYNK_AUTH_TOKEN); // Configure Blynk without blocking
matrix.begin(); // Initialize LED matrix
matrix.beginDraw(); // Begin drawing on the matrix
matrix.stroke(0xFFFFFFFF); // Set stroke color to white
matrix.textScrollSpeed(100); // Set text scroll speed
matrix.textFont(Font_4x6); // Set font for text
matrix.beginText(0, 1, 0xFFFFFF); // Begin text at position (0,1) with white color
matrix.println(" UNO r4 "); // Print text to matrix
matrix.endText(SCROLL_LEFT); // End text drawing and scroll left
matrix.endDraw(); // Finish drawing on the matrix
}
void loop() {
// Attempt to connect to Blynk without blocking
if (WiFi.status() == WL_CONNECTED && !Blynk.connected()) {
Blynk.connect(2000); // Attempt to reconnect to Blynk with a 2-second timeout
}
if (Blynk.connected()) {
Blynk.run(); // Run Blynk tasks only if connected
}
// Continue running other functions regardless of Blynk connection status
readDHTSensor(); // Read temperature and humidity from DHT sensor
updateGardenLight(); // Adjust garden light based on LDR sensor
readGasSensor(); // Read value from gas sensor
scrollMatrixText(); // Scroll text on LED matrix
}
// DHT Sensor Reading
void readDHTSensor() {
static unsigned long lastReadTime = 0; // Variable to store the last read time
if (millis() - lastReadTime > 2000) { // Read sensor every 2 seconds
lastReadTime = millis();
float humidity = dht.readHumidity(); // Read humidity
float temperature = dht.readTemperature(); // Read temperature
if (!isnan(humidity) && !isnan(temperature)) { // Check for valid readings
Serial.print("Temperature: ");
Serial.print(temperature);
Serial.print(" °C, Humidity: ");
Serial.println(humidity);
Blynk.virtualWrite(VIRTUAL_PIN_TEMPERATURE, temperature); // Send temperature to Blynk
Blynk.virtualWrite(VIRTUAL_PIN_HUMIDITY, humidity); // Send humidity to Blynk
} else {
Serial.println("Failed to read from DHT sensor!"); // Print error message
}
}
}
// LDR Sensor Control
void updateGardenLight() {
int adc_val = 255 - (analogRead(LDR_SENSOR) / 4); // Read LDR value and calculate garden light value
analogWrite(GARDEN_LIGHT, adc_val); // Set garden light brightness
}
// Gas Sensor Reading
void readGasSensor() {
sensorValue = analogRead(MQ2_PIN); // Read value from gas sensor
Serial.print("Gas Sensor Value: ");
Serial.print(sensorValue);
if (sensorValue > 200) { // Check if smoke is detected
Serial.print(" | Smoke detected!"); // Print smoke detection message
playEmergencySiren(); // Play emergency siren sound
} else {
noTone(BUZZER_PIN); // Stop the buzzer if no smoke
}
Serial.println(""); // Print newline
}
// Emergency Siren Sound
void playEmergencySiren() {
for (int freq = LOW_FREQ; freq <= HIGH_FREQ; freq += 10) { // Increase frequency
tone(BUZZER_PIN, freq); // Play sound at current frequency
delayMicroseconds(FADE_TIME * 1000); // Wait for fade time
}
delayMicroseconds(HOLD_TIME * 1000); // Hold the high frequency
for (int freq = HIGH_FREQ; freq >= LOW_FREQ; freq -= 10) { // Decrease frequency
tone(BUZZER_PIN, freq); // Play sound at current frequency
delayMicroseconds(FADE_TIME * 1000); // Wait for fade time
}
delayMicroseconds(HOLD_TIME * 1000); // Hold the low frequency
}
// Matrix Text Scrolling
void scrollMatrixText() {
static unsigned long lastScrollTime = 0; // Variable to store the last scroll time
if (millis() - lastScrollTime > 500) { // Scroll text every 500 milliseconds
lastScrollTime = millis();
matrix.beginDraw(); // Begin drawing on the matrix
matrix.stroke(0xFFFFFFFF); // Set stroke color to white
matrix.textScrollSpeed(50); // Set text scroll speed
matrix.textFont(Font_5x7); // Set font for text
matrix.beginText(0, 1, 0xFFFFFF); // Begin text at position (0,1) with white color
matrix.println(" Scrolling text! "); // Print scrolling text
matrix.endText(SCROLL_LEFT); // End text drawing and scroll left
matrix.endDraw(); // Finish drawing on the matrix
}
// Blynk Button Controls
BLYNK_WRITE(V0) {
digitalWrite(GREEN_LIGHT, param.asInt()); // Control green light based on Blynk button state
}
BLYNK_WRITE(V4) {
digitalWrite(WHITE_LIGHT, param.asInt()); // Control white light based on Blynk button state
}
BLYNK_WRITE(V3) {
digitalWrite(RED_LIGHT, param.asInt()); // Control red light based on Blynk button state
}