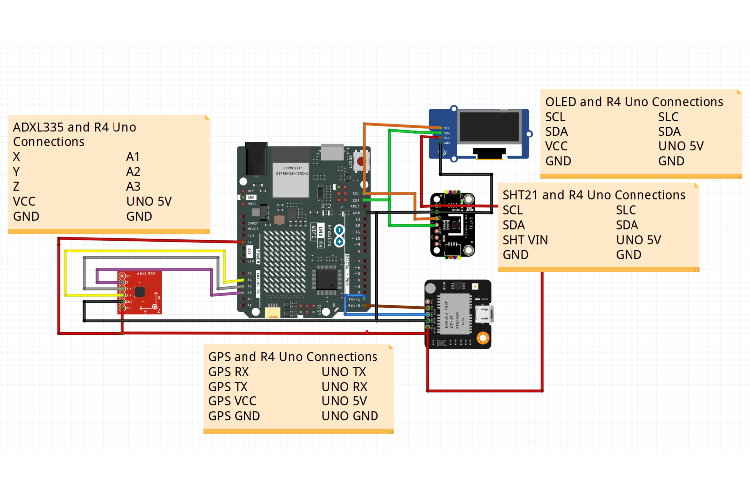
This project aims to empower users to lead a healthier and safer lifestyle.
Next, let's look at the connections used in this project. The sensor SHT21 and OLED display use an I2C bus, the GPS sensor uses UART and the accelerometer sensors use analog pins.
The connections are done in that manner where SHT21 and OLED are using the same I2C because they have different slave addresses. The GPS uses UART (bottom right) and the accelerometer is using 3 analog pins for X, Y and Z axis (left side of the circuit diagram)
Components Required
Input Sensors:
1. Temperature and Humidity - SHT21
2. Location Tracking - GT-U7
3. Accelerometer - ADXL335
Output Sensor
This Circuit Diagram is Created Using Fritzing
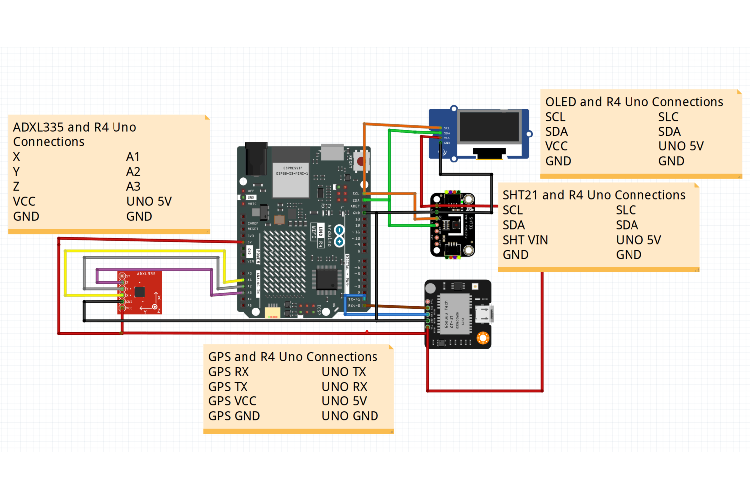
Working:
1.GPS
Global Positioning System is a satellite-based radio navigation system. There are many satellites revolving around the Earth. The positioning of the satellites is done in such a manner that there are 4 of them visible in the sky from any location on Earth.
The GPS module that we are using works as a receiver that listens to the radio signals that are transmitted by those satellites. The GPS receiver module receives the broadcasting signals and is capable of estimating its relative distance from all four satellites. The method used to determine is called trilateration which is all done using the GPS receiver. The reason it is called trilateration is because the location is determined by latitude, longitude, and altitude which can be received by 3 satellites and the fourth one allows the GPS module to have more precise information.
The receiver we are using works on a standard called NEMA. NEMA is the National Marine Electronics Association which was formed in 1957 by a group of electronics dealers to create better communications with manufacturers. This much like other standards reduced the confusion for different parts and everyone can follow much like ASCII and HEX created for computers.
GPS receiver manufactured today uses the NEMA data format standard which allows the engineers to easily write firmware or software for various GPS modules keeping the code the same. All the data is encoded in this data format, which has be to received using UART.
To read this data, we use onboard UART of Arduino Uno R4 WiFi. To have meaningful data, the GPS receiver has to be locked with more than 3 satellites to provide the location information. Once the receiver is locked, we get the data in formats starting from $GPGGA, $GPGLL, $GPRMC.
This NEMA standard starting with different messages has different data encoded like latitude, longitude, speed, time, altitude, azimuth, number of satellite connections, etc.
We will understand this in more detail with an example shown below from our GPS Receiver:
2. Temperature and Humidity Sensor - SHT21
This sensor works on a communication interface called I2C. I2C is a synchronous multi-controller, single-ended, serial communication bus interface invented by Philips Semiconductor in 1982. The working principle for this bus is, that it uses a master and slave setup. A user can connect multiple slaves with a single master. Each slave is identified with a unique slave address provided in the part's datasheet.
We will interface our SHT21 module using I2C and a predefined library to read the temperature and humidity.
3. Accelerometer - ADXL335
Accelerometers are widely used in motion sensing or tilt sensing applications. It is used for image and video stabilization, sports, and health monitoring devices. It works on the principle of gravitational force exertion over a particular axis. This force is then measured in plus or munis g form and filtered to provide us acceleration readings for all three axes.
We will use this sensor to detect the steps or pedometer application by developing an algorithm based on the largest acceleration change provided by one of the three axes. The sensor will be calibrated and vector square root will be taken and compared with the average acceleration to count the step.
Code Explanation:
We will test each one of the components individually to ensure the correctness of it. Once, we have an understanding that the system works then we will integrate the system.
Part 1: Testing The GPS Sensor
GT-U7 GPS receiver uses NEMA standard to receive and decode the data into latitude, longitude, and altitude. There are many of other information as well but we will first focus on the ones we need.
To receive the data, connect the GPS module as per the circuit diagram mentioned above. Although there is a little change, for testing purposes I will use the software serial library to enable an extra serial than the hardware serial provided by UNO
Code: GPS Test
#include <TinyGPSPlus.h>
#include <SoftwareSerial.h>
static const int RXPin = 4, TXPin = 3;
static const uint32_t GPSBaud = 9600;
// The TinyGPSPlus object
TinyGPSPlus gps;
// The serial connection to the GPS device
SoftwareSerial ss(RXPin, TXPin);
void setup()
{
Serial.begin(115200);
ss.begin(GPSBaud);
}
void loop()
{
// This sketch displays information every time a new sentence is correctly encoded.
while (ss.available() > 0)
if (gps.encode(ss.read()))
displayInfo();
if (millis() > 5000 && gps.charsProcessed() < 10)
{
Serial.println(F("No GPS detected: check wiring."));
while(true);
}
}
void displayInfo()
{
Serial.print(F("Location: "));
if (gps.location.isValid())
{
Serial.print(gps.location.lat(), 6);
Serial.print(F(","));
Serial.print(gps.location.lng(), 6);
}
else
{
Serial.print(F("INVALID"));
}
Serial.print(F(" Date/Time: "));
if (gps.date.isValid())
{
Serial.print(gps.date.month());
Serial.print(F("/"));
Serial.print(gps.date.day());
Serial.print(F("/"));
Serial.print(gps.date.year());
}
else
{
Serial.print(F("INVALID"));
}
Serial.print(F(" "));
if (gps.time.isValid())
{
if (gps.time.hour() < 10) Serial.print(F("0"));
Serial.print(gps.time.hour());
Serial.print(F(":"));
if (gps.time.minute() < 10) Serial.print(F("0"));
Serial.print(gps.time.minute());
Serial.print(F(":"));
if (gps.time.second() < 10) Serial.print(F("0"));
Serial.print(gps.time.second());
Serial.print(F("."));
if (gps.time.centisecond() < 10) Serial.print(F("0"));
Serial.print(gps.time.centisecond());
}
else
{
Serial.print(F("INVALID"));
}
Serial.println();
}
Output:
Part 2: Testing The Temperature and Humidity Sensor - SHT21
Since this sensor uses an I2C bus as an OLED display. We have to first ensure the slave addresses are not the same.
Code:
#include <SHT21.h> // include SHT21 library
SHT21 sht;
float temp; // variable to store temperature
float humidity; // variable to store hemidity
void setup() {
Wire.begin(); // begin Wire(I2C)
Serial.begin(9600); // begin Serial
}
void loop() {
temp = sht.getTemperature(); // get temp from SHT
humidity = sht.getHumidity(); // get temp from SHT
Serial.print("Temp: "); // print readings
Serial.print(temp);
Serial.print("t Humidity: ");
Serial.println(humidity);
delay(85); // min delay for 14bit temp reading is 85ms
}
Output:
Part 3: Testing The Accelerometer Sensor - ADXL 335
ADXL335 data is read through onboard 10-bit ADC's
Code:
const int xpin = A1; // x-axis of the accelerometer
const int ypin = A2; // y-axis
const int zpin = A3; // z-axis (only on 3-axis models)
void setup() {
// initialize the serial communications:
Serial.begin(9600);
// Provide ground and power by using the analog inputs as normal digital pins.
// This makes it possible to directly connect the breakout board to the
// Arduino. If you use the normal 5V and GND pins on the Arduino,
// you can remove these lines.
pinMode(groundpin, OUTPUT);
pinMode(powerpin, OUTPUT);
digitalWrite(groundpin, LOW);
digitalWrite(powerpin, HIGH);
}
void loop() {
// print the sensor values:
Serial.print(analogRead(xpin));
// print a tab between values:
Serial.print("t");
Serial.print(analogRead(ypin));
// print a tab between values:
Serial.print("t");
Serial.print(analogRead(zpin));
Serial.println();
// delay before next reading:
delay(100);
}
Output:
This smartwatch is designed to revolutionize personal health and safety by providing real-time data on location, and activity levels like temperature and humidity. With its advanced tracking capabilities, users can:
Monitor their health: Accurately track daily steps, distance, and calories burned to maintain a healthy lifestyle.
Stay safe: Use GPS tracking for peace of mind during outdoor activities and emergencies.
Make informed decisions: Access real-time body temperature and humidity data to plan activities and protect themselves from extreme weather conditions.
Enhance overall well-being: Gain valuable insights into their health and habits, leading to a more balanced and fulfilling life.
This can create an awareness of being outdoors with a better quality of life journey.