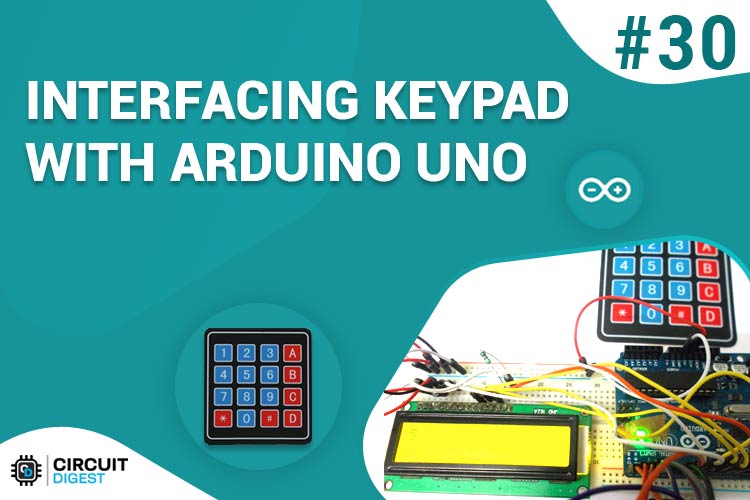
In this tutorial we are going to interface a 4x4 (16 key) keypad with ARDUINO UNO. We all know keypad is one of the most important input devices used in electronics engineering. Keypad is the easiest and the cheapest way to give commands or instructions to an electronic system. Whenever a key is pressed in keypad module the Arduino Uno detects it and shoes the corresponding key on 16x2 LCD. [Also check: Keypad Interfacing with 8051 Microcontroller]
Components Required
Hardware: ARDUINO UNO, power supply (5v), 100uF capacitor , buttons (two pieces), 1KΩ resistor (two pieces), 4x4 Keypad Module, LED, JHD_162ALCD (16x2LCD).
Software: arduino IDE (Arduino nightly).
Circuit Diagram and Working Explanation
In 16x2 LCD there are 16 pins over all if there is a back light, if there is no back light there will be 14 pins. One can power or leave the back light pins. Now in the 14 pins there are 8 data pins (7-14 or D0-D7), 2 power supply pins (1&2 or VSS&VDD or GND&+5v), 3rd pin for contrast control (VEE-controls how thick the characters should be shown) and 3 control pins (RS&RW&E).
In the Arduino uno keypad interfacing circuit, you can observe that I have only took two control pins. This give the flexibility of better understanding, the contrast bit and READ/WRITE are not often used so they can be shorted to ground. This puts LCD in highest contrast and read mode. We just need to control ENABLE and RS pins to send characters and data accordingly. [Also check: 16x2 LCD Interfacing with Arduino Uno]
The connections which are done for LCD are given below:
PIN1 or VSS to ground
PIN2 or VDD or VCC to +5v power
PIN3 or VEE to ground (gives maximum contrast best for a beginner)
PIN4 or RS (Register Selection) to PIN8 of ARDUINO UNO
PIN5 or RW (Read/Write) to ground (puts LCD in read mode eases the communication for user)
PIN6 or E (Enable) to PIN9 of ARDUINO UNO
PIN11 or D4 to PIN10 of ARDUINO UNO
PIN12 or D5 to PIN11 of ARDUINO UNO
PIN13 or D6 to PIN12 of ARDUINO UNO
PIN14 or D7 to PIN13 of ARDUINO UNO
We are going to connect the keypad module between pins 0-7 of Arduino Uno, as shown in the circuit diagram. All the eight pins of keypad module are connected accordingly.
Now for setting up a connection between Keypad Module and UNO, we need to get the keypad library from the ARDUINO website. After that we can directly call the header file. We will discuss each command below.
// Define the Keymap
{'1','2','3','A'}, {'4','5','6','B'}, {'7','8','9','C'}, {'#','0','*','D'} }; // Connect keypad ROW0, ROW1, ROW2 and ROW3 to these Arduino pins.
// Connect keypad COL0, COL1 and COL2 to these Arduino pins.
// Create the Keypad
|
First we will call the header file which enables user to access all keypad commands. We will write each key of keypad in matrix, for the UNO to understand the key press.
Telling the Uno which pins are used to connect the keypad module.
Telling the UNO to map each key on press.
Getting the UNO mapped key in to a memory.
The working of Arduino Uno Keypad Interface is best explained in C code given below.
Complete Project Code
#include <LiquidCrystal.h>
// initialize the library with the numbers of the interface pins
LiquidCrystal lcd(8, 9, 10, 11, 12, 13);//RS,EN,D4,D5,D6,D7
#include <Keypad.h>//header for keypad commands enabling
const byte ROWS = 4; // Four rows
const byte COLS = 4; // Three columns
// Define the Keymap
char keys[ROWS][COLS] = {
{'1','2','3','A'},
{'4','5','6','B'},
{'7','8','9','C'},
{'#','0','*','D'}
};
// Connect keypad ROW0, ROW1, ROW2 and ROW3 to these Arduino pins.
byte rowPins[ROWS] = { 0, 1, 2, 3 };
// Connect keypad COL0, COL1 and COL2 to these Arduino pins.
byte colPins[COLS] = { 4, 5, 6, 7 };
// Create the Keypad
Keypad kpd = Keypad( makeKeymap(keys), rowPins, colPins, ROWS, COLS );
void setup()
{
for(int k=8;k<14;k++)
{
pinMode(k,OUTPUT);//pins 8-14 are enabled as output
}
lcd.begin(16, 2);//initializing LCD
}
void loop()
{
char key = kpd.getKey(); //storing pressed key value in a char
if (key != NO_KEY)
{
lcd.print(key); //showing pressed character on LCD
}
}
Comments
Update ARDUINO IDE software, you might have a older version.
include keypad.h library to this code !! you won't get that error
what arduino version you used sir
can i get the code of password protection along with encrypted messeges?
I just need help on my project I need a programme for water level controller that uses keypad to type required level and usession ultrasonic sensor to monitor level of water as well LCD to display and water pump to pump required water
Check this one: Automatic Water Level Indicator and Controller using Arduino and add a keypad to enter required water level.
I need the code for interfacing keypad with arduino and p10 led display. Please help me
When I execute the code it will show keypad does not name a type.. Please solve my problem ASAP.