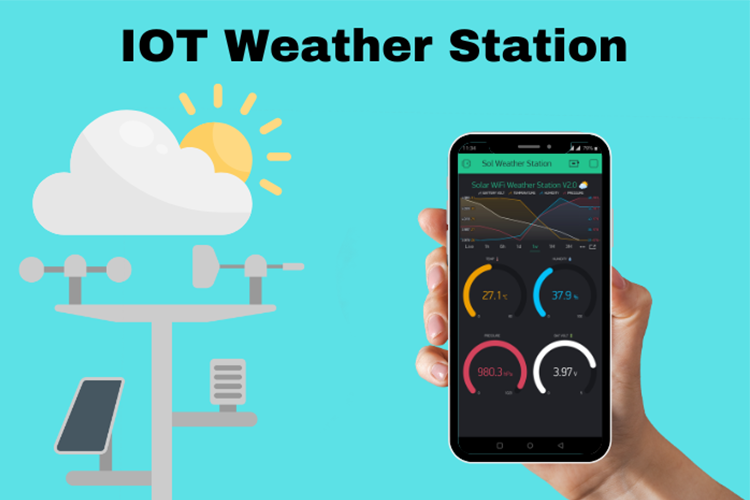
Project Explanation
This project showcases an IoT Weather Station built using the Arduino Uno R4 Wi-Fi, designed to monitor and report various environmental parameters. Utilizing multiple sensors—including the DHT22 for temperature and humidity, the BMP180 for atmospheric pressure, an anemometer for wind speed, a wind vane for wind direction, and a rain gauge for measuring rainfall—this station provides comprehensive weather data. All collected data is uploaded in real-time to the Blynk app, enabling remote monitoring.
Impact Statement
Increased Accessibility: With user-friendly apps like Blynk, anyone can easily access weather data. This helps people make better decisions about farming, outdoor events, and safety during storms.
Cost-Effective: Using affordable components like sensors and Arduino boards keeps the project within a low budget. This makes it possible for schools and small communities to set up their own weather stations without spending too much.
Customizable and Scalable: The system can be easily adjusted to fit different needs. Users can add more sensors or change settings based on their specific requirements. It can also grow over time as more features are added.
Remote Access: With WiFi capabilities, users can check weather data from anywhere. This is particularly useful for farmers and emergency responders who need up-to-date information to make important decisions.
Research and Education: This project provides a hands-on learning experience for students. It encourages interest in science and technology by allowing them to explore how weather works and how to use technology to measure it.
Components Used
Arduino UNO R4 WiFi - (X1)
Reed Switch - (X10)
DHT22 - (X1)
BMP180 - (X1)
3D Printed Parts
Wires
PVC Pipe
PVC Pipe T - Joint (X1)
PVC Pipe L - Joint (X2)
Electronics Enclosure
5x8x2.5 Bearings (X4)
Watch the full demonstration by clicking on the YouTube video below :
Circuit Diagram Explanation
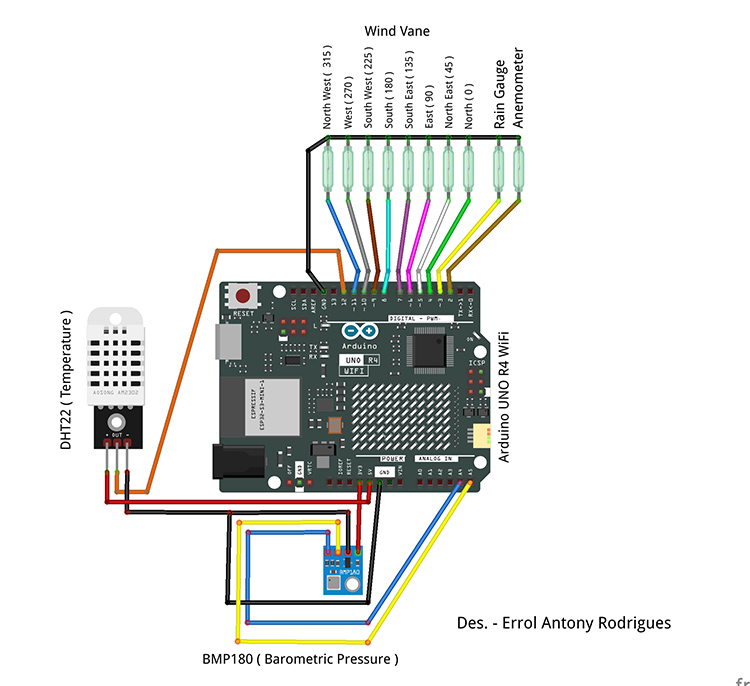
1.DHT22
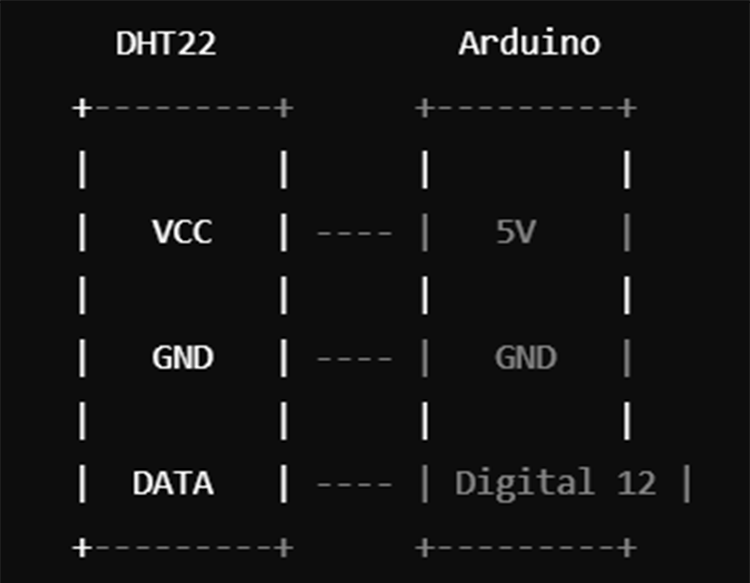
DHT22 VCC → Arduino 5V
DHT22 GND → Arduino GND
DHT22 DATA → Arduino Digital Pin 12
2.BMP180
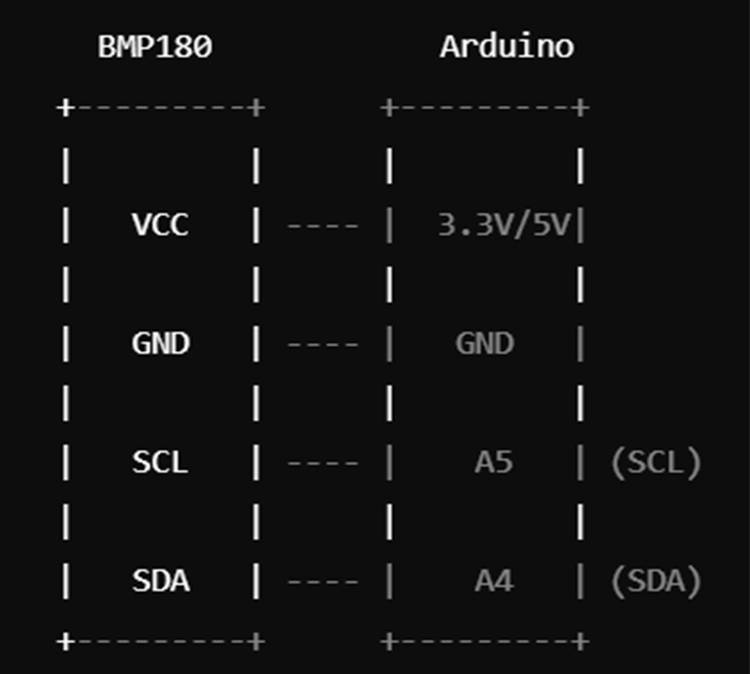
BMP180 VCC → Arduino 3.3V
BMP180 GND → Arduino GND
BMP180 SCL → Arduino A5 (SCL)
BMP180 SDA → Arduino A4 (SDA)
3.Anemometer
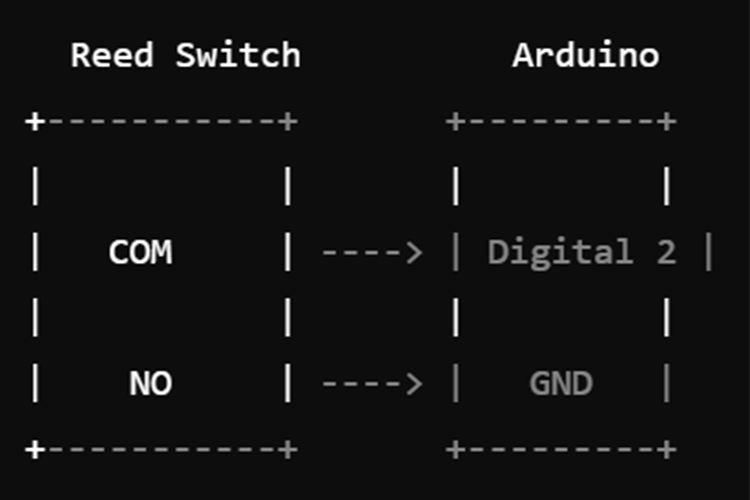
Reed Switch COM → Arduino Digital Pin 2
Reed Switch NO → Arduino GND
4.Rain Gauge
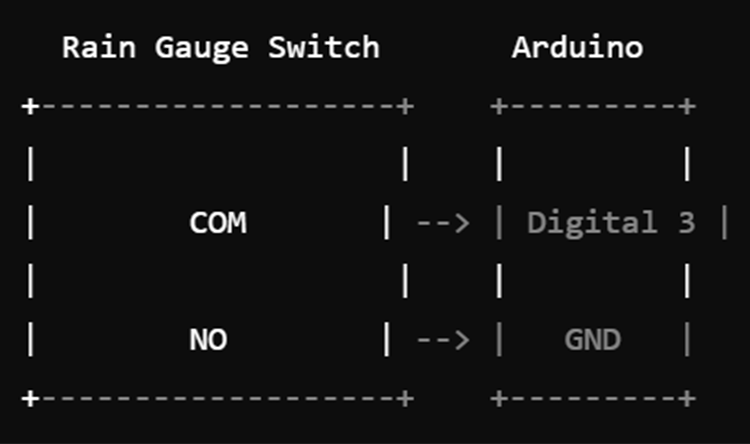
Rain Gauge COM → Arduino Digital Pin 3
Rain Gauge NO → Arduino GND
5.Wind Vane
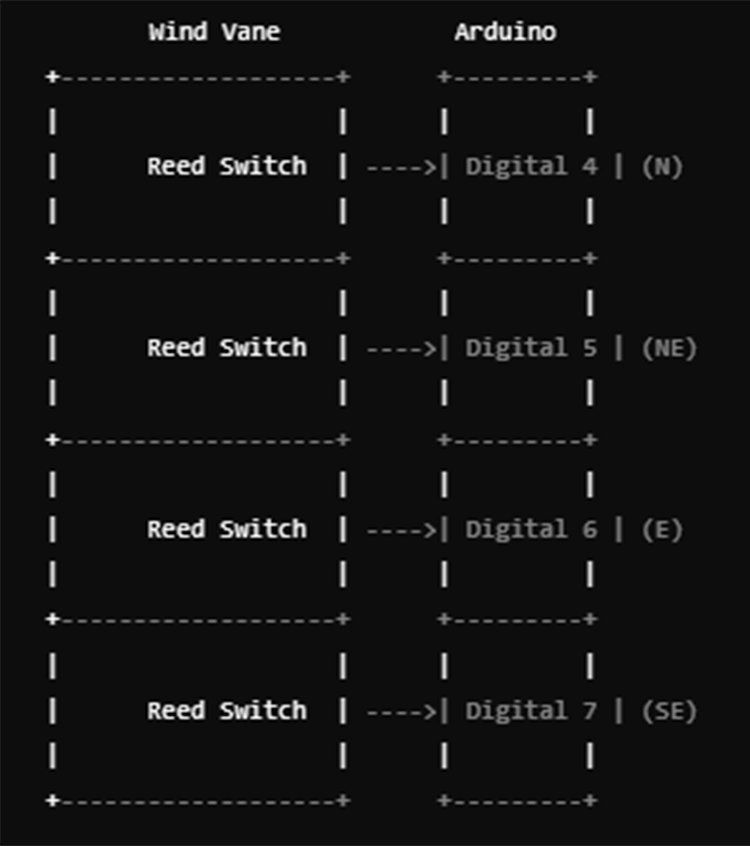
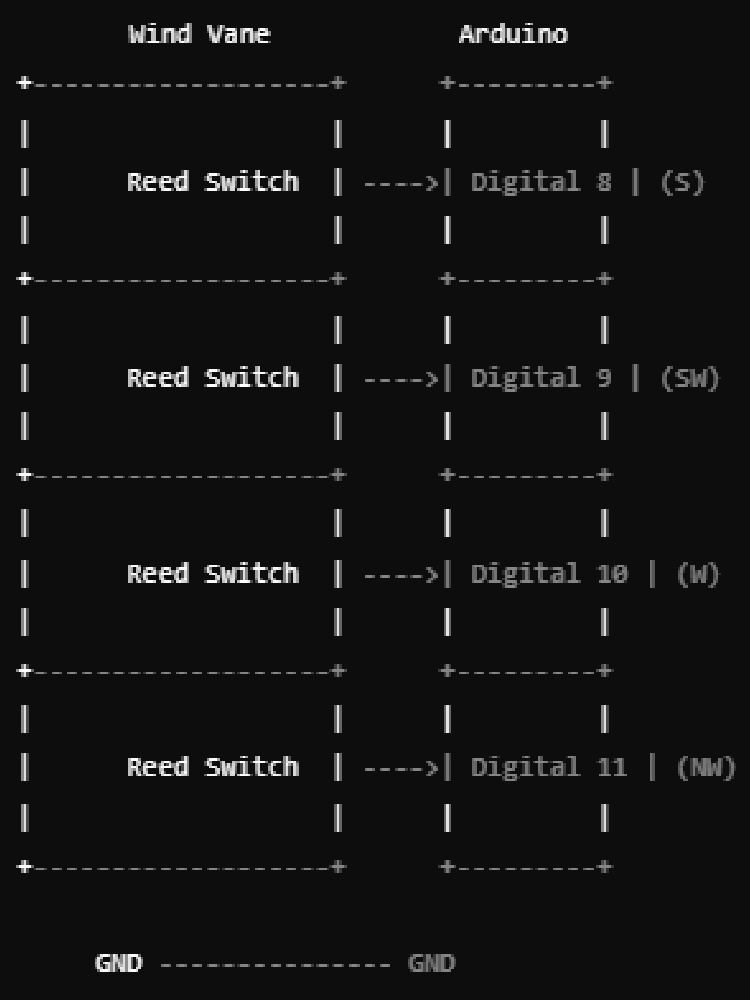
Connect each reed switch to is designated pin from (4 to 11)
Connect the other side of each switch to Arduino GND
Code Explanation
1.Blynk Configuration

Blynk Setup: These lines define identifiers for your Blynk project. Blynk is a platform that allows you to control hardware remotely and visualize data.
2.Library Inclusions

DHT Sensor: Measures temperature and humidity.
BMP180 Sensor: Measures atmospheric pressure.
WiFi and Blynk Libraries: Enable the Arduino to connect to the internet and send data to the Blynk app.
3.Sensor Configuration

DHT Setup: Configures the DHT sensor on digital pin 12, specifying it as a DHT22 type.
BMP180 Setup: Initializes the BMP180 sensor object.
4.WiFi Configuration

WiFi Credentials: Stores the SSID and password for connecting to the WiFi network.
5.Sensor And Timer Variables
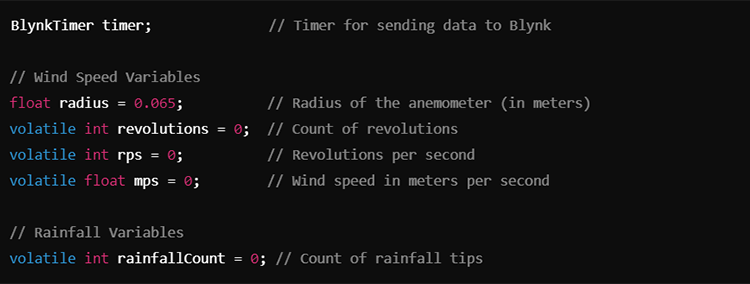
Wind Speed Calculation: Variables used for measuring the wind speed based on revolutions of the anemometer.
Rainfall Measurement: Variable for counting the number of tips from the rain gauge.
6.Setup Function
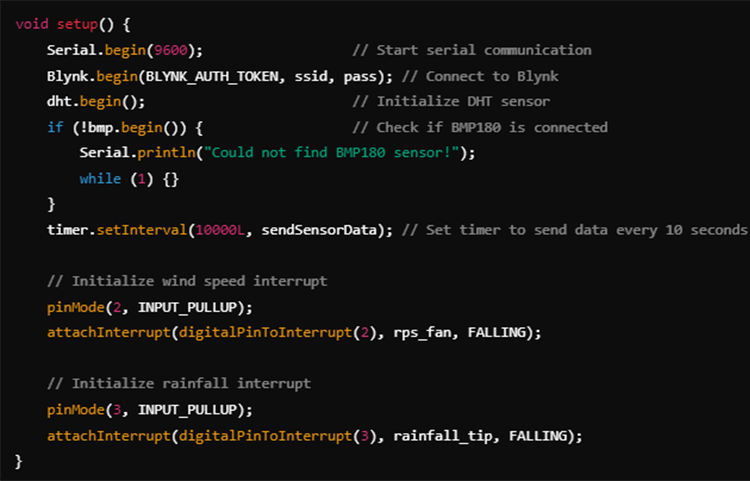
Initialization: Sets up serial communication, connects to Blynk, initializes sensors, and configures interrupts for the anemometer and rain gauge.
Error Handling: If the BMP180 isn’t found, the program halts.
7.Loop Function
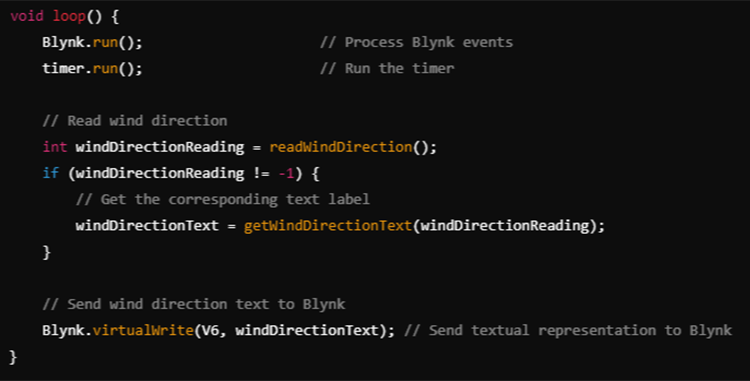
Continuous Operation: Processes Blynk events and reads wind direction. The wind direction text is sent to the Blynk app.
8.Sending Sensor Data
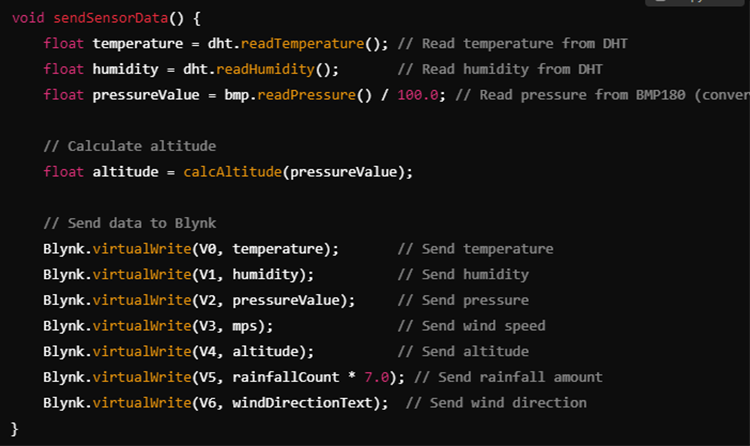
Data Collection: Reads data from the DHT and BMP180 sensors and sends the information to Blynk using virtual pins.
Rainfall Calculation: Converts the rainfall count into a measurable value before sending.
9.Reading Wind Direction

Direction Detection: Determines which reed switch is activated to identify the wind direction.
Calculations
1.Rainfall
The calculation of rainfall in a tipping bucket rain gauge typically involves counting the number of times the bucket tips over, as each tip represents a fixed volume of collected rainwater.
Mechanism: The rain gauge consists of a bucket that tips when a certain volume of rain (e.g., 0.2 mm) is collected. Each time the bucket tips, it sends a signal (usually a momentary closure of a switch or a reed switch)
Counting Tips: The Arduino counts these tips using an interrupt service routine (ISR). Each time the bucket tips, the ISR increments a counter.
Code breakdown
Variables:

ISR for Rain Gauge: This function is triggered by an interrupt when the rain gauge tips. It checks to avoid counting noise or false triggers using a debounce mechanism (ensuring a minimum time interval between counts).
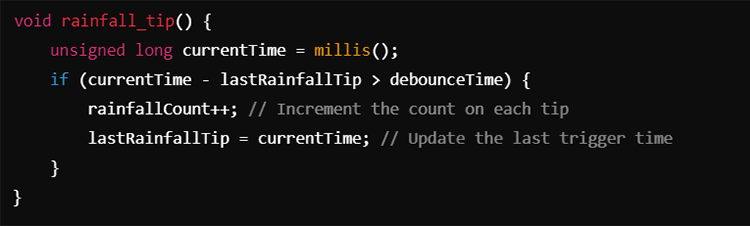
Calculating Rainfall Amount: In the sendSensorData function, the rainfall amount is calculated and sent to Blynk:
Here, 7.0
represents the volume of rain corresponding to one tip of the bucket. For example, if each tip of the bucket measures 7 mm of rainfall, then multiplying the number of tips by 7 gives the total rainfall in millimeters.

Summary
Each tip of the bucket represents a known volume of rain (e.g., 7 mm).
The Arduino counts the number of tips using an interrupt.
The total rainfall is calculated by multiplying the tip count by the volume represented by each tip.
2.Anemometer
Wind speed is typically calculated using an anemometer, which measures the rotational speed of cups or blades that catch the wind. In your Arduino project, the wind speed is calculated based on the number of revolutions of the anemometer over a specific time period.
Mechanism: An anemometer usually consists of rotating cups or blades. As the wind blows, it causes these cups to rotate. The speed of rotation is directly proportional to the wind speed.
Counting Revolutions: The Arduino counts the number of revolutions using an interrupt service routine (ISR). Each time the anemometer completes a full rotation, it triggers an interrupt, which increments a counter.
Code breakdown
Variables:

ISR for Anemometer: This function is triggered whenever the anemometer completes a rotation. The debounce mechanism ensures that only valid rotations are counted, preventing false triggers from noise.
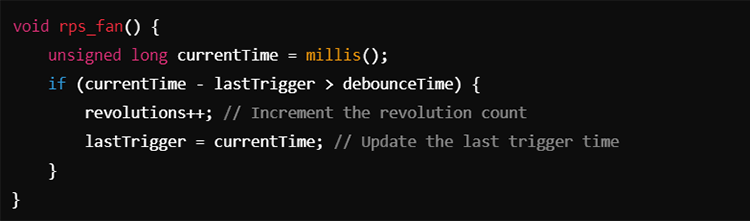
Calculating Wind Speed: The calculation occurs in the sendSensorData function
Revolutions Per Second (RPS): The number of revolutions counted in a 5-second interval is divided by 5 to get the RPS.
Wind Speed in Meters per Second (MPS): The formula used converts RPS to wind speed
MPS = RPS x (Circumference of the cup)
Circumference is calculated by - 2 x 3.1414 x radius
The factor of 60 is used to convert from minutes to seconds since RPS is revolutions per second.
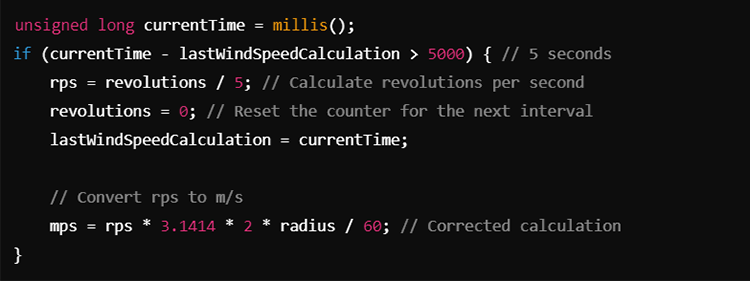
Summary
Each rotation of the anemometer is counted by the Arduino using interrupts.
The number of revolutions in a set time period (e.g., 5 seconds) is used to calculate the revolutions per second (RPS).
The RPS is converted to wind speed (meters per second) using the circumference of the anemometer cups.
3.Wind Vane
A wind vane, also known as a weather vane, is a device used to indicate the direction from which the wind is blowing. Here’s how it works, including its components and how it interfaces with an Arduino for readings.
The wind vane consists of a lightweight, rotatable component (the arrow) mounted on a vertical shaft. The arrow points in the direction that the wind is coming from.
When the wind blows, it pushes against the larger end of the vane, causing it to rotate around the shaft until it aligns with the wind direction.
Multiple reed switches are installed at specific positions corresponding to cardinal and intercardinal directions
North (0°)
North-East (45°)
East (90°)
South-East (135°)
South (180°)
South-West (225°)
West (270°)
North-West (315°)
When the wind vane turns and points in a particular direction, the corresponding reed switch closes, sending a signal to the Arduino. The Arduino detects which switch is activated and determines the wind direction based on the switch position.
Code Breakdown
In the Arduino code, you would have an array of reed switches corresponding to the wind directions:
When reading the wind direction, the Arduino checks the state of each reed switch:
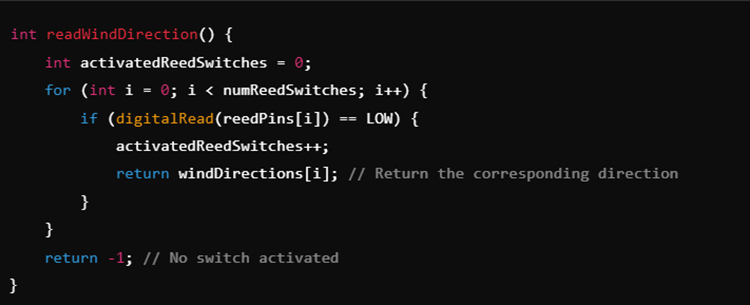
Summary
Wind Vane Operation: Uses aerodynamic principles to rotate and align with wind direction.
Integration with Arduino: Reed switches are used to detect the wind direction electronically.
Functionality: The system detects which reed switch is activated to determine the wind direction in degrees, allowing for accurate weather monitoring.
Working Pictures
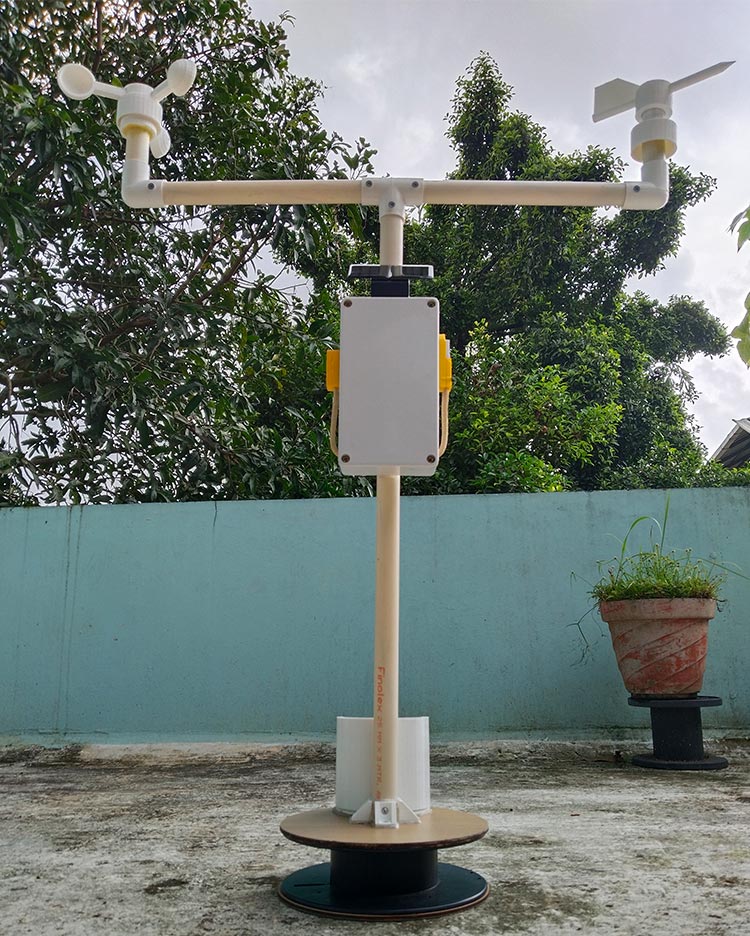
For all the 3D printing files and code, follow the GitHub link below: