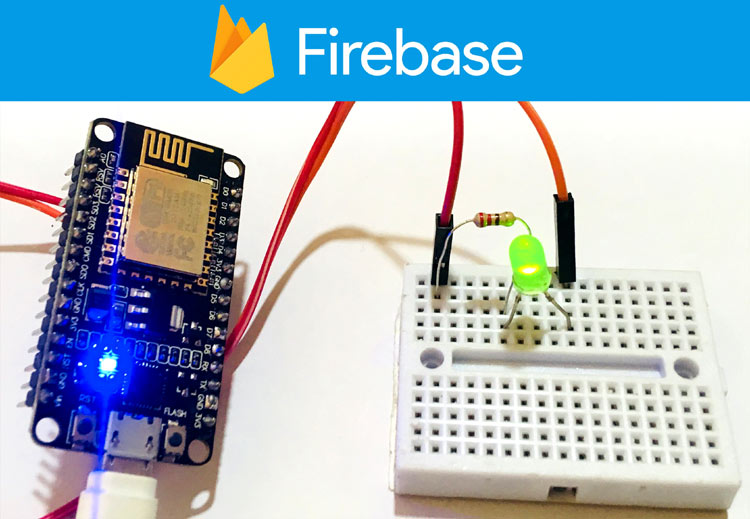
Have you ever thought of controlling any peripheral from anywhere in the world? Yes the IoT (Internet of things) has made this possible to control any appliance from anywhere and there are many IoT hardware and cloud platform are available to achieve this. We have covered many IoT applications in our previous tutorial. Today we will use Google Firebase cloud to control an LED using NodeMCU ESP8266. If you don’t know that what Firebase is, then I will try to tell in brief.
Firebase is Google’s database platform which is used to create, manage and modify data generated from any android application, web services, sensors etc. For more information on Firebase please visit the official website of Google Firebase.
To control an LED from Firebase, first we will set up the NodeMCU ESP8266 Wi-Fi Module and then set up Google Firebase.
Setting Up NodeMCU ESP8266 Wi-Fi Module
Setting up NodeMCU is very easy and it won’t take much time. This can be done in few simple components and simple steps.
Components required
- NodeMCU ESP8266(12E)
- Led
If you don’t have NodeMCU ESP8266(12E) then you can also use Generic ESP8266 module with Arduino UNO and Arduino IDE. To know how to program Generic ESP8266 module using Arduino UNO and Arduino IDE, just follow the link.
Circuit Diagram
Once you are all set with components please follow simple steps mentioned below:
- Connect NodeMCU with Computer.
- Open Arduino IDE.
- Goto ‘Tools’ and Select ‘Boards’.
- In board’s section, select ‘NodeMCU V1.0 (ESP-12E Module)’.
- Also select appropriate COM Port.
- After finding the “FIREBASE_HOST” and “FIREBASE_AUTH” following the steps mentioned below, edit this in sketch given below and upload.
If you are completely new to ESP8266 then start with Introduction of ESP8266 article.
Programming NodeMCU ESP8266 for using Google Firebase
Complete program with working Video is given at the end. Here we are explaining few important parts of the code.
Firstly include the libraries for using ESP8266 and firebase.
#include <ESP8266WiFi.h #include <FirebaseArduino.h
Download and install the libraries by following the below links:
https://github.com/FirebaseExtended/firebase-arduino/blob/master/src/Firebase.h
https://github.com/bblanchon/ArduinoJson
While compiling, if you get error that ArduinoJson.h library is not installed then please install it using link given above.
These two parameters are very important to communicate with firebase. Setting these parameters will enable the data exchange between and ESP8266 and firebase. To find these parameters for your project, follow the steps given in later section (Setting Up Firebase) below.
#define FIREBASE_HOST "your-project.firebaseio.com" // the project name address from firebase id #define FIREBASE_AUTH "06dEpqanFg***************qAwnQLwLI" // the secret key generated from firebase
After successfully finding the credentials, just replace in the above code.
Below parameters are used to connect to your home Wi-Fi network or the network that you setup. Replace SSID and password with your network SSID and password. Also internet is required in order to communicate with firebase.
#define WIFI_SSID "xxxxxxxxxxxxx" // input your home or public wifi name #define WIFI_PASSWORD "xxxxxxxxxxxxxx" //password of wifi ssid
This statement tries to connect with your entered Wi-Fi network.
WiFi.begin(WIFI_SSID, WIFI_PASSWORD); //try to connect with wifi
When connecting to Wi-Fi, if it doesn’t connect to network then just print(.) until it is not successfully connected.
while (WiFi.status() != WL_CONNECTED) { Serial.print("."); delay(500); }
This statement tries to connect with firebase server. If the host address and authorization key are correct then it will connect successfully
Firebase.begin(FIREBASE_HOST, FIREBASE_AUTH); // connect to firebase
This is the class provided by firebase library to send string to firebase server. To use more classes go to this link. Initially send one string to the home path. With the help of this we can change the status of LED.
Firebase.setString("LED_STATUS", "OFF"); //send initial string of led status
After sending one status string to firebase path, write this statement to get the status of LED from same path and save it to variable.
fireStatus = Firebase.getString("LED_STATUS"); // get ld status input from firebase
If received string is “ON” or “on” then just turn on built-in LED and externally connected LED.
if (fireStatus == "ON" || fireStatus == "on") { // compare the input of led status received from firebase Serial.println("Led Turned ON"); digitalWrite(LED_BUILTIN, HIGH); // make bultin led ON digitalWrite(led, HIGH); // make external led ON }
If received string is “OFF” or “off” then just turn off built-in LED and externally connected led.
else if (fireStatus == "OFF" || fireStatus == "off") { // compare the input of led status received from firebase Serial.println("Led Turned OFF"); digitalWrite(LED_BUILTIN, LOW); // make bultin led OFF digitalWrite(led, LOW); // make external led OFF }
If received string is not any of these then just ignore and print some error meesage.
else { Serial.println("Wrong Credential! Please send ON/OFF"); }
Setting Up Firebase Console
If you are using Firebase first time then you may take some time to setting it up. Have patience and follow these steps.
1. If you have Gmail id then you don’t need to Sign Up for firebase, if you don’t have Gmail id then Sign Up for one and then you can go to next step.
2. Open your browser and go to “firebase.google.com”
3. At the right top corner go to “Go to Console”
4. Click on “Add project”
5. Input your Project Name.
6. Accept the terms and condition, Create project and click on “Continue”
You have successfully created your project. Look for the Host Name and Authorization Key also known as Secret Key. For this, follow steps given below:
7. Go to Settings Icon(Gear Icon) and click on “Project Settings”
8. Now click on “Service Accounts”
9. You can see two options “Firebase admin SDK” and “Database Secrets”
10. Click on “Database Secrets”
11. Scroll on your project name and ”Show” option appears at right side of your project
12. Click on “Show” and now you can see secret key created for your project
13. Copy the secret key and save it to notepad. This is your “FIREBASE_AUTH” string which we have written in Arduino program above.
14. Now go to “Database” on left control bar and click on it
15. Scroll down and click on “Create Database”
16. Choose “Start in test mode” and click on “Enable”
17. Now your database is created and you will have to come to this section again to control LED
18. Now just above the database you can see
“https://your_project_name.firebaseio.com/”
19. Just copy “your_project_name.firebaseio.com” without any slash and https and save it again to notepad just you had saved for secret key
20. This is your “FIREBASE_HOST” string which we have written in Arduino program above
21. You can explore the firebase but let’s finish the tutorial first.
Now put “FIREBASE_HOST” and “FIREBASE_AUTH” in Arduino program and upload the sketch. And we are done with setting up both sections. Complete Arduino Program is given at the end.
Open the firebase database and write “ON” or “OFF” or “on” or “off” in “LED_STATUS” section to control LED from Google Firebase console.
Watch the Video below to understand the working of the project. We will send temperature and humidity data to firebase cloud in our next project.
/* Controlling LED using Firebase console by CircuitDigest(www.circuitdigest.com) */
#include <ESP8266WiFi.h> // esp8266 library
#include <FirebaseArduino.h> // firebase library
#define FIREBASE_HOST "your-project.firebaseio.com" // the project name address from firebase id
#define FIREBASE_AUTH "06dEpqanFg***************qAwnQLwLI" // the secret key generated from firebase
#define WIFI_SSID "xxxxxxxxxxxxx" // input your home or public wifi name
#define WIFI_PASSWORD "xxxxxxxxxxxxxx" //password of wifi ssid
String fireStatus = ""; // led status received from firebase
int led = D3; // for external led
void setup() {
Serial.begin(9600);
delay(1000);
pinMode(LED_BUILTIN, OUTPUT);
pinMode(led, OUTPUT);
WiFi.begin(WIFI_SSID, WIFI_PASSWORD); //try to connect with wifi
Serial.print("Connecting to ");
Serial.print(WIFI_SSID);
while (WiFi.status() != WL_CONNECTED) {
Serial.print(".");
delay(500);
}
Serial.println();
Serial.print("Connected to ");
Serial.println(WIFI_SSID);
Serial.print("IP Address is : ");
Serial.println(WiFi.localIP()); //print local IP address
Firebase.begin(FIREBASE_HOST, FIREBASE_AUTH); // connect to firebase
Firebase.setString("LED_STATUS", "OFF"); //send initial string of led status
}
void loop() {
fireStatus = Firebase.getString("LED_STATUS"); // get ld status input from firebase
if (fireStatus == "ON") { // compare the input of led status received from firebase
Serial.println("Led Turned ON");
digitalWrite(LED_BUILTIN, LOW); // make bultin led ON
digitalWrite(led, HIGH); // make external led ON
}
else if (fireStatus == "OFF") { // compare the input of led status received from firebase
Serial.println("Led Turned OFF");
digitalWrite(LED_BUILTIN, HIGH); // make bultin led OFF
digitalWrite(led, LOW); // make external led OFF
}
else {
Serial.println("Wrong Credential! Please send ON/OFF");
}
}
Comments
Error
I've folowed the steps but I'm getting an errpr like
Arduino: 1.8.11 Hourly Build 2019/09/30 10:12 (Mac OS X), Board: "NodeMCU 1.0 (ESP-12E Module), 80 MHz, Flash, Legacy (new can return nullptr), All SSL ciphers (most compatible), 4MB (FS:2MB OTA:~1019KB), 2, v2 Lower Memory, Disabled, None, Only Sketch, 115200"
In file included from /Users/bhargav/Documents/Arduino/libraries/firebase-arduino-master/src/Firebase.h:30:0,
from /Users/bhargav/Documents/Arduino/libraries/firebase-arduino-master/src/FirebaseArduino.h:22,
from /Users/bhargav/Desktop/FireBaseCntrl/FireBaseCntrl.ino:3:
/Users/bhargav/Documents/Arduino/libraries/firebase-arduino-master/src/FirebaseObject.h:109:11: error: StaticJsonBuffer is a class from ArduinoJson 5. Please see arduinojson.org/upgrade to learn how to upgrade your program to ArduinoJson version 6
std::shared_ptr<StaticJsonBuffer<FIREBASE_JSONBUFFER_SIZE>> buffer_;
^
In file included from /Users/bhargav/Documents/Arduino/libraries/firebase-arduino-master/src/FirebaseArduino.h:22:0,
from /Users/bhargav/Desktop/FireBaseCntrl/FireBaseCntrl.ino:3:
/Users/bhargav/Documents/Arduino/libraries/firebase-arduino-master/src/Firebase.h:86:11: error: StaticJsonBuffer is a class from ArduinoJson 5. Please see arduinojson.org/upgrade to learn how to upgrade your program to ArduinoJson version 6
std::shared_ptr<StaticJsonBuffer<FIREBASE_JSONBUFFER_SIZE>> buffer_;
^
Multiple libraries were found for "ESP8266WiFi.h"
Used: /Users/bhargav/Library/Arduino15/packages/esp8266/hardware/esp8266/2.6.3/libraries/ESP8266WiFi
Multiple libraries were found for "FirebaseArduino.h"
Used: /Users/bhargav/Documents/Arduino/libraries/firebase-arduino-master
Multiple libraries were found for "ArduinoJson.h"
Used: /Users/bhargav/Documents/Arduino/libraries/ArduinoJson
Not used: /Users/bhargav/Documents/Arduino/libraries/ArduinoJson-6.x
Multiple libraries were found for "ESP8266HTTPClient.h"
Used: /Users/bhargav/Library/Arduino15/packages/esp8266/hardware/esp8266/2.6.3/libraries/ESP8266HTTPClient
exit status 1
Error compiling for board NodeMCU 1.0 (ESP-12E Module).
This report would have more information with
"Show verbose output during compilation"
option enabled in File -> Preferences
remove multiple libraries and
remove multiple libraries and install arduinojason library old version 5.13.2
I HAVE DONE AS PER TUTPRIAL
I HAVE DONE AS PER TUTPRIAL BUT WHEN I AM SENDING ON OR OF COMMAND FROM FIRE BASE LED IS NOT RESPONDING.
Hello,
Sir I have done all the above steps as it is in this tutorial, but my realtime database is still showing <myproject:"NULL"> instead of LED_STATUS, kindly guide me through