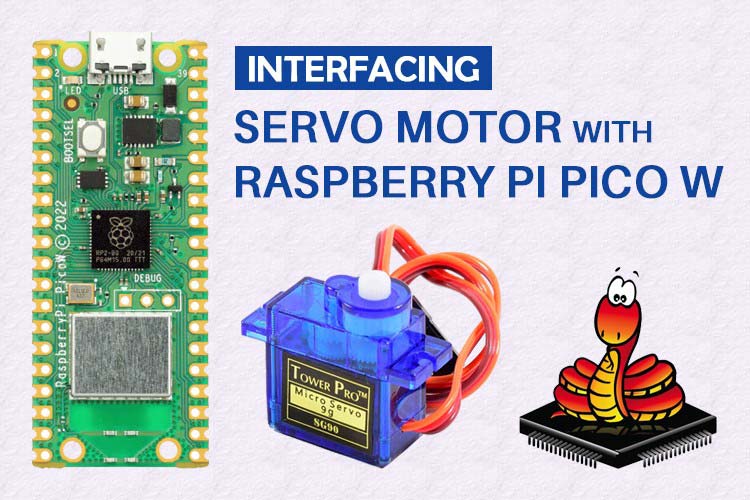
This blog explores interfacing a servo motor with the Raspberry Pi Pico W using MicroPython. The Pico W is an affordable and compact microcontroller board based on the RP2040 chip, ideal for embedded systems and IoT projects. MicroPython, a lightweight implementation of Python, provides a user-friendly environment for microcontroller programming. By following the steps outlined, readers will learn how to connect a servo motor to the Pico W, write MicroPython code for controlling its movement, and gain the skills to integrate precise motor control into their own projects. Let's dive in!
Servo Motor Pinout
Servo motor has 3 pins
VCC (+5V or +6V): Supplies voltage to the servo motor.
GND Completes the electrical circuit.
Control Signal (PWM): Receives position control signals via PWM.
Working of a Servo Motor
In the context of a servo motor, pulse width modulation (PWM) is used to control the position or angle of the motor's output shaft. The servo motor receives a PWM signal as an input, where the duration of the high state (the "on" time) within each PWM period determines the desired position.
Typically, the PWM signal has a fixed frequency, often around 50 Hz, with a duty cycle ranging from 5% to 10% representing the minimum angle and 10% to 20% representing the maximum angle of the servo motor. For example, if the PWM signal has a period of 20 ms, a duty cycle of 5% would result in a pulse width of 1 ms, corresponding to the minimum angle position, while a duty cycle of 10% would result in a pulse width of 2 ms, representing the maximum angle position.
The servo motor's control circuitry interprets the PWM signal and compares the pulse width with its current position feedback. Based on this comparison, the control circuitry adjusts the power supplied to the motor, causing it to rotate and move the output shaft towards the desired position. The servo motor's feedback mechanism continuously monitors the position and provides feedback to the control circuitry, allowing for precise adjustments to reach and maintain the desired angle.
By adjusting the duty cycle of the PWM signal, you can control the position of the servo motor's output shaft. This enables you to precisely position the motor in a wide range of angles, making servo motors a popular choice for applications that require accurate and controlled motion, such as robotic arms, RC vehicles, and automated systems.
Common questions about Servo Motors
What are the typical applications of servo motors?
Servo motors are widely used in applications that require precise control of position or angle. Common applications include robotics, RC vehicles, industrial automation, camera gimbals, 3D printers, CNC machines, and robotic arms.
Can servo motors rotate continuously?
Most servo motors are designed for limited-range motion rather than continuous rotation. However, there are specific servo motor models called "continuous rotation servos" that can rotate continuously in either direction, allowing for continuous motion control.
Can I power a servo motor directly from a microcontroller?
It is generally not recommended to power a servo motor directly from a microcontroller because servo motors often require higher current than what a microcontroller's output pin can provide. Instead, it is best to power the servo motor using an external power supply (e.g., batteries, DC power adapter) and connect the control signal wire to the microcontroller.
Circuit Diagram of Pico W with Servo motors
Connect the power wire of the servo motor to the 3.3V pin on the Raspberry Pi Pico W.
Connect the ground wire of the servo motor to any GND pin on the Raspberry Pi Pico W.
Connect the control signal wire of the servo motor to any GPIO pin on the Raspberry Pi Pico W. Note the GPIO pin number for later use.
Programming Raspberry Pi Pico W to Control Servo Motor
from time import sleep from machine import Pin from machine import PWM
The code imports necessary modules: sleep from the time module, Pin and PWM from the machine module.
pwm = PWM(Pin(0))
A PWM object is created with Pin(0) as the parameter. This associates the PWM object with GPIO Pin 0 on the microcontroller.
pwm.freq(50)
The frequency of the PWM signal is set to 50 Hz.
def setServoCycle(position): pwm.duty_u16(position) sleep(0.01)
This function, setServoCycle, is defined to set the duty cycle of the PWM signal. The position parameter is expected to be a value between 0 and 65535, representing the duty cycle range. The duty_u16() method of the PWM object is used to set the duty cycle based on the provided position. After setting the duty cycle, the function sleeps for 0.01 seconds.
while True: for pos in range(1000, 9000, 50): setServoCycle(pos) for pos in range(9000, 1000, -50): setServoCycle(pos)
This code segment runs an infinite loop. Within the loop, two for loops are used to iterate over a range of values. The first loop starts from 1000, increases by 50, and stops at 8950. It calls the setServoCycle() function with each value from the range to set the servo motor's position.
After that, the second for loop starts from 9000, decreases by 50, and stops at 1050. Again, the setServoCycle() function is called with each value from the range to set the servo motor's position.
This creates a back-and-forth motion of the servo motor, sweeping it from one extreme position to the other repeatedly.
Projects using Pico W
Real-Time Temperature and Humidity Monitoring Webserver with Raspberry Pi Pico W and DHT11 Sensor
Are you looking to create a smart and efficient real-time temperature and humidity monitoring system? Look no further! Our blog presents a comprehensive guide on building a cutting-edge web server using Raspberry Pi Pico W and the reliable DHT11 sensor. Discover how this DIY project can revolutionize your home or workspace by providing crucial environmental data at your fingertips.
Getting Started with Raspberry Pi Pico W and building a LED Webserver using Micropython
Welcome to the exciting world of Raspberry Pi Pico W and MicroPython! Our blog offers a perfect starting point for beginners and tech enthusiasts to dive into the world of embedded systems and web development. Learn how to unleash the power of Raspberry Pi Pico W and program it using the user-friendly MicroPython language. Follow our step-by-step guide to create a mesmerizing LED web server, where you can control LEDs remotely through a web interface.
Complete Project Code
from time import sleep
from machine import Pin
from machine import PWM
pwm = PWM(Pin(0 b))
pwm.freq(50)
#Function to set an angle
#The position is expected as a parameter
def setServoCycle (position):
pwm.duty_u16(position)
sleep(0.01)
while True:
for pos in range(1000,9000,50):
setServoCycle(pos)
for pos in range(9000,1000,-50):
setServoCycle(pos)