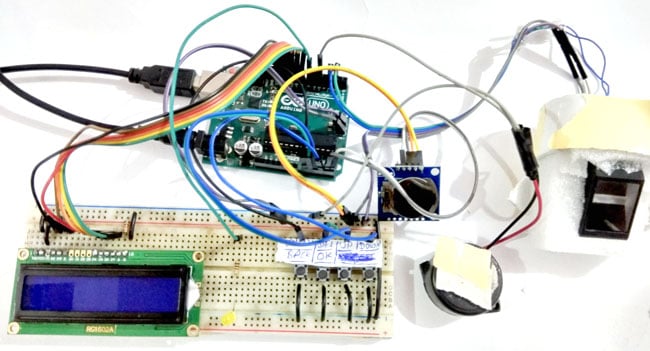
Attendance systems are commonly used systems to mark the presence in offices and schools. From manually marking the attendance in attendance registers to using high-tech applications and biometric systems, these systems have improved significantly. In our previous projects, we have covered few other electronic attendance system projects using RFID and AVR microcontroller, 8051 and raspberry Pi. In this project, we used fingerprint Module and Arduino to take and keep attendance data and records. By using fingerprint sensor, the system will become more secure for the users. Following sections explains technical details of making a fingerprint based biometric attendance system using Arduino.
Required Components
- Arduino -1
- Finger print module -1
- Push Button - 4
- LEDs -1
- 1K Resistor -2
- 2.2K resistor -1
- Power
- Connecting wires
- Box
- Buzzer -1
- 16x2 LCD -1
- Bread Board -1
- RTC Module -1
Project Description:
In this fingerprint attendance system circuit, we used Fingerprint Sensor module to authenticate a true person or employee by taking their finger input in the system. Here we are using 4 push buttons to enroll, Delete, UP/Down. ENROLL and DEL key has triple features. ENROLL key is used for enrollment of a new person into the system. So when the user wants to enroll new finger then he/she need to press ENROLL key then LCD asks for the ID, where user want to be store the finger print image. Now if at this time user does not want to proceed further then he/she can press ENROLL key again to go back. This time ENROLL key behave as Back key, i.e. ENROLL key has both enrollment and back function. Besides enroll key is also used to download attendance data over serial monitor. Similarly, DEL/OK key also has the same double function like when user enrolls new finger, then he/she need to select finger ID by using another two key namely UP and DOWN. Now user need to press DEL/OK key (this time this key behave like OK) to proceed with selected ID. And Del key is used for reset or delete data from EEPROM of Arduino.
FingerPrint module:
Fingerprint sensor module captures finger’s print image and then converts it into the equivalent template and saves them into its memory as per selected ID by Arduino. All the process is commanded by Arduino like taking an image of finger’s print, convert it into templates and storing as ID etc. You can check some more projects using fingerprint module:
Fingerprint based security system
Fingerprint based biometric voting machine
Here we have added a Yellow LED which indicates that fingerprint module is ready to take an image of the finger. A buzzer is also used for various indications. Arduino is the main component of this system it is responsible for control of the whole system.
Working of Fingerprint Based Attendance System
Working of this fingerprint attendance system project is fairly simple. First of all, the user needs to enroll fingerprints of the user with the help of push buttons. To do this, user need to press ENROLL key and then LCD asks for entering ID for the fingerprint to save it in memory by ID name. So now user needs to enter ID by using UP/DOWN keys. After selecting ID, user needs to press OK key (DEL key). Now LCD will ask to place finger over the fingerprint module. Now user needs to place his finger over finger print module and then the module takes finger image. Now the LCD will say to remove finger from fingerprint module, and again ask to place finger again. Now user needs to put his finger again and module takes an image and convert it into templates and stores it by selected ID into the finger print module’s memory. Now the user will be registered and he/she can feed attendance by putting their finger over fingerprint module.By the same method, all the users will be registered into the system.
Now if the user wants to remove or delete any of the stored ID or fingerprint, then he/she need to press DEL key. Once delete key is pressed LCD will ask to select ID that need to be deleted. Now user needs to select ID and press OK key (same DEL key). Now LCD will let you know that fingerprint has been deleted successfully.
How Attendance works in this Fingerprint Attendance System Project:
Whenever user place his finger over fingerprint module then fingerprint module captures finger image, and search if any ID is associated with this fingerprint in the system. If fingerprint ID is detected then LCD will show Attendance registered and in the same time buzzer will beep once and LED will turn off until the system is ready to take input again.
Along with the fingerprint module, we have also used an RTC module for Time and date. Time and date are running continuously in the system. So Arduino take time and date whenever a true user places his finger over fingerprint and save them in the EEPROM at the allotted slot of memory.
Here we have created 5 user space in this system for 30 days. By pressing the RESET button in Arduino and then immediately enroll key will be responsible for downloading attendance data over serial monitor from the Arduino EEPROM Memory.
Memory Management:
We have 1023 byte memory in Arduino UNO out of which we have 1018 byte to store data and we have taken 5 user attendance data for 30 days. And every attendance will record time and date so this becomes 7-byte data.
So total memory required is
5*30*7=1050 so here we need more 32 bytes
But if we will use 4 users then we required
4*30*7=840
Here we have done this project demonstration by taking 5 users memory. By this, we will not able to store 32 byte or 5 attendance records of the 5th user.
You may try it by 4 users by changing some lines in code. I have made the comments in the code where the changes are needed.
Circuit Diagram and Description for Fingerprint Attendance System Project
The circuit of this fingerprint based attendance system project, as shown in the above diagram is quite simple. It has Arduino for controlling all the process of the project, push button for enrolling, deleting, selecting IDs and for attendance, a buzzer for alerting, LEDs for indication and LCD to instruct user and showing the resultant messages.
As shown in the circuit diagram, a push button is directly connected to pin A0(ENROL), A1(DEL), A2(UP), A3(DOWN) of Arduino with respect to the ground And Yellow LED is connected at Digital pin D7 of Arduino with respect to ground through a 1k resistor. Fingerprint module’s Rx and Tx directly connected at Serial pin D2 and D3 (Software Serial) of Arduino. 5v supply is used for powering finger print module taken from Arduino board. A buzzer is also connected at pin A5. A 16x2 LCD is configured in 4-bit mode and its RS, EN, D4, D5, D6, and D7 are directly connected at Digital pin D13, D12, D11, D10,D9, and D8 of Arduino.
Code Explanation:
The fingerprint attendance system code for arduino is given in the subsequent sections. Although the code is explained well with comments, we are discussing here few important parts of the code. We used fingerprint library for interfacing finger print module with Arduino board.
First of all, we include the header file and defines input and output pin and define the macro and declared variables. After this, in setup function, we give direction to defined pin and initiate LCD and finger print module
After it, we have to write code for downloading attendance data.
void setup() { delay(1000); lcd.begin(16,2); Serial.begin(9600); pinMode(enroll, INPUT_PULLUP); pinMode(up, INPUT_PULLUP); pinMode(down, INPUT_PULLUP); pinMode(del, INPUT_PULLUP); pinMode(match, INPUT_PULLUP); pinMode(buzzer, OUTPUT); pinMode(indFinger, OUTPUT); digitalWrite(buzzer, LOW); if(digitalRead(enroll) == 0) { digitalWrite(buzzer, HIGH); delay(500); digitalWrite(buzzer, LOW); lcd.clear(); lcd.print("Please wait"); lcd.setCursor(0,1); lcd.print("Downloding Data");
Afterit, we have to write code for clearing attendance data from EEPROM.
if(digitalRead(del) == 0) { lcd.clear(); lcd.print("Please Wait"); lcd.setCursor(0,1); lcd.print("Reseting....."); for(int i=1000;i<1005;i++) EEPROM.write(i,0); for(int i=0;i<841;i++) EEPROM.write(i, 0xff); lcd.clear(); lcd.print("System Reset"); delay(1000); }
After it, we initiate finger print module, showing welcome message over LCD and also initeiated RTC module.
After it, in loop function, we have read RTC time and displayed it on LCD
void loop() { now = rtc.now(); lcd.setCursor(0,0); lcd.print("Time->"); lcd.print(now.hour(), DEC); lcd.print(':'); lcd.print(now.minute(), DEC); lcd.print(':'); lcd.print(now.second(), DEC); lcd.print(" "); lcd.setCursor(0,1); lcd.print("Date->"); lcd.print(now.day(), DEC); lcd.print('/'); lcd.print(now.month(), DEC); lcd.print('/'); lcd.print(now.year(), DEC);
After it, waiting for the finger print to take input and compare captured image ID with stored IDs. If amatch occurs then proceed with next step. And checking enroll del keys as well
int result=getFingerprintIDez(); if(result>0) { digitalWrite(indFinger, LOW); digitalWrite(buzzer, HIGH); delay(100); digitalWrite(buzzer, LOW); lcd.clear(); lcd.print("ID:"); lcd.print(result); lcd.setCursor(0,1); lcd.print("Please Wait...."); delay(1000); attendance(result); lcd.clear(); lcd.print("Attendance "); lcd.setCursor(0,1); lcd.print("Registed"); delay(1000); digitalWrite(indFinger, HIGH); return; }
Given void checkKeys() function is used for checking Enroll or DEL key is pressed or not and what to do if pressed. If the ENROL key pressed the Enroll() function is called and DEL key press then delete() function is called.
void delet() function is used for entering ID to be deleted and calling uint8_t deleteFingerprint(uint8_t id) function that will delete finger from records.
Given Function is used to taking finger print image and convert them into the template and save as well by selected ID into the finger print module memory.
uint8_t getFingerprintEnroll() { int p = -1; lcd.clear(); lcd.print("finger ID:"); lcd.print(id); lcd.setCursor(0,1); lcd.print("Place Finger"); delay(2000); while (p != FINGERPRINT_OK) { p = finger.getImage(); ..... ..... ....... ....
Given function is used for storing attendance time and date in the allotted slot of EEPROM
void attendance(int id) { int user=0,eepLoc=0; if(id == 1) { eepLoc=0; user=user1++; } else if(id == 2) { eepLoc=210; user=user2++; } else if(id == 3) .... .... .....
Given function is used to fetching data from EEPROM and send to serial monitor
void download(int eepIndex) { if(EEPROM.read(eepIndex) != 0xff) { Serial.print("T->"); if(EEPROM.read(eepIndex)<10) Serial.print('0'); Serial.print(EEPROM.read(eepIndex++)); Serial.print(':'); if(EEPROM.read(eepIndex)<10) Serial.print('0'); Serial.print(EEPROM.read(eepIndex++)); .... .... .....
Complete Project Code
#include<EEPROM.h>
#include<LiquidCrystal.h>
LiquidCrystal lcd(13,12,11,10,9,8);
#include <SoftwareSerial.h>
SoftwareSerial fingerPrint(2, 3);
#include <Wire.h>
#include "RTClib.h"
RTC_DS1307 rtc;
#include "Adafruit_Fingerprint.h"
uint8_t id;
Adafruit_Fingerprint finger = Adafruit_Fingerprint(&fingerPrint);
#define enroll 14
#define del 15
#define up 16
#define down 17
#define match 5
#define indFinger 7
#define buzzer 5
#define records 4 // 5 for 5 user
int user1,user2,user3,user4,user5;
DateTime now;
void setup()
{
delay(1000);
lcd.begin(16,2);
Serial.begin(9600);
pinMode(enroll, INPUT_PULLUP);
pinMode(up, INPUT_PULLUP);
pinMode(down, INPUT_PULLUP);
pinMode(del, INPUT_PULLUP);
pinMode(match, INPUT_PULLUP);
pinMode(buzzer, OUTPUT);
pinMode(indFinger, OUTPUT);
digitalWrite(buzzer, LOW);
if(digitalRead(enroll) == 0)
{
digitalWrite(buzzer, HIGH);
delay(500);
digitalWrite(buzzer, LOW);
lcd.clear();
lcd.print("Please wait");
lcd.setCursor(0,1);
lcd.print("Downloding Data");
Serial.println("Please wait");
Serial.println("Downloding Data..");
Serial.println();
Serial.print("S.No. ");
for(int i=0;i<records;i++)
{
digitalWrite(buzzer, HIGH);
delay(500);
digitalWrite(buzzer, LOW);
Serial.print(" User ID");
Serial.print(i+1);
Serial.print(" ");
}
Serial.println();
int eepIndex=0;
for(int i=0;i<30;i++)
{
if(i+1<10)
Serial.print('0');
Serial.print(i+1);
Serial.print(" ");
eepIndex=(i*7);
download(eepIndex);
eepIndex=(i*7)+210;
download(eepIndex);
eepIndex=(i*7)+420;
download(eepIndex);
eepIndex=(i*7)+630;
download(eepIndex);
// eepIndex=(i*7)+840; // 5th user
// download(eepIndex);
Serial.println();
}
}
if(digitalRead(del) == 0)
{
lcd.clear();
lcd.print("Please Wait");
lcd.setCursor(0,1);
lcd.print("Reseting.....");
for(int i=1000;i<1005;i++)
EEPROM.write(i,0);
for(int i=0;i<841;i++)
EEPROM.write(i, 0xff);
lcd.clear();
lcd.print("System Reset");
delay(1000);
}
lcd.clear();
lcd.print(" Attendance ");
lcd.setCursor(0,1);
lcd.print(" System ");
delay(2000);
lcd.clear();
lcd.print("Circuit Digest");
lcd.setCursor(0,1);
lcd.print("Saddam Khan");
delay(2000);
digitalWrite(buzzer, HIGH);
delay(500);
digitalWrite(buzzer, LOW);
for(int i=1000;i<1000+records;i++)
{
if(EEPROM.read(i) == 0xff)
EEPROM.write(i,0);
}
finger.begin(57600);
Serial.begin(9600);
lcd.clear();
lcd.print("Finding Module");
lcd.setCursor(0,1);
delay(1000);
if (finger.verifyPassword())
{
Serial.println("Found fingerprint sensor!");
lcd.clear();
lcd.print("Found Module ");
delay(1000);
}
else
{
Serial.println("Did not find fingerprint sensor :(");
lcd.clear();
lcd.print("module not Found");
lcd.setCursor(0,1);
lcd.print("Check Connections");
while (1);
}
if (! rtc.begin())
Serial.println("Couldn't find RTC");
// rtc.adjust(DateTime(F(__DATE__), F(__TIME__)));
if (! rtc.isrunning())
{
Serial.println("RTC is NOT running!");
// following line sets the RTC to the date & time this sketch was compiled
rtc.adjust(DateTime(F(__DATE__), F(__TIME__)));
// This line sets the RTC with an explicit date & time, for example to set
// January 21, 2014 at 3am you would call:
// rtc.adjust(DateTime(2014, 1, 21, 3, 0, 0));
}
lcd.setCursor(0,0);
lcd.print("Press Match to ");
lcd.setCursor(0,1);
lcd.print("Start System");
delay(2000);
user1=EEPROM.read(1000);
user2=EEPROM.read(1001);
user3=EEPROM.read(1002);
user4=EEPROM.read(1003);
user5=EEPROM.read(1004);
lcd.clear();
digitalWrite(indFinger, HIGH);
}
void loop()
{
now = rtc.now();
lcd.setCursor(0,0);
lcd.print("Time->");
lcd.print(now.hour(), DEC);
lcd.print(':');
lcd.print(now.minute(), DEC);
lcd.print(':');
lcd.print(now.second(), DEC);
lcd.print(" ");
lcd.setCursor(0,1);
lcd.print("Date->");
lcd.print(now.day(), DEC);
lcd.print('/');
lcd.print(now.month(), DEC);
lcd.print('/');
lcd.print(now.year(), DEC);
lcd.print(" ");
delay(500);
int result=getFingerprintIDez();
if(result>0)
{
digitalWrite(indFinger, LOW);
digitalWrite(buzzer, HIGH);
delay(100);
digitalWrite(buzzer, LOW);
lcd.clear();
lcd.print("ID:");
lcd.print(result);
lcd.setCursor(0,1);
lcd.print("Please Wait....");
delay(1000);
attendance(result);
lcd.clear();
lcd.print("Attendance ");
lcd.setCursor(0,1);
lcd.print("Registed");
delay(1000);
digitalWrite(indFinger, HIGH);
return;
}
checkKeys();
delay(300);
}
// dmyyhms - 7 bytes
void attendance(int id)
{
int user=0,eepLoc=0;
if(id == 1)
{
eepLoc=0;
user=user1++;
}
else if(id == 2)
{
eepLoc=210;
user=user2++;
}
else if(id == 3)
{
eepLoc=420;
user=user3++;
}
else if(id == 4)
{
eepLoc=630;
user=user4++;
}
/*else if(id == 5) // fifth user
{
eepLoc=840;
user=user5++;
}*/
else
return;
int eepIndex=(user*7)+eepLoc;
EEPROM.write(eepIndex++, now.hour());
EEPROM.write(eepIndex++, now.minute());
EEPROM.write(eepIndex++, now.second());
EEPROM.write(eepIndex++, now.day());
EEPROM.write(eepIndex++, now.month());
EEPROM.write(eepIndex++, now.year()>>8 );
EEPROM.write(eepIndex++, now.year());
EEPROM.write(1000,user1);
EEPROM.write(1001,user2);
EEPROM.write(1002,user3);
EEPROM.write(1003,user4);
// EEPROM.write(4,user5); // figth user
}
void checkKeys()
{
if(digitalRead(enroll) == 0)
{
lcd.clear();
lcd.print("Please Wait");
delay(1000);
while(digitalRead(enroll) == 0);
Enroll();
}
else if(digitalRead(del) == 0)
{
lcd.clear();
lcd.print("Please Wait");
delay(1000);
delet();
}
}
void Enroll()
{
int count=1;
lcd.clear();
lcd.print("Enter Finger ID:");
while(1)
{
lcd.setCursor(0,1);
lcd.print(count);
if(digitalRead(up) == 0)
{
count++;
if(count>records)
count=1;
delay(500);
}
else if(digitalRead(down) == 0)
{
count--;
if(count<1)
count=records;
delay(500);
}
else if(digitalRead(del) == 0)
{
id=count;
getFingerprintEnroll();
for(int i=0;i<records;i++)
{
if(EEPROM.read(i) != 0xff)
{
EEPROM.write(i, id);
break;
}
}
return;
}
else if(digitalRead(enroll) == 0)
{
return;
}
}
}
void delet()
{
int count=1;
lcd.clear();
lcd.print("Enter Finger ID");
while(1)
{
lcd.setCursor(0,1);
lcd.print(count);
if(digitalRead(up) == 0)
{
count++;
if(count>records)
count=1;
delay(500);
}
else if(digitalRead(down) == 0)
{
count--;
if(count<1)
count=records;
delay(500);
}
else if(digitalRead(del) == 0)
{
id=count;
deleteFingerprint(id);
for(int i=0;i<records;i++)
{
if(EEPROM.read(i) == id)
{
EEPROM.write(i, 0xff);
break;
}
}
return;
}
else if(digitalRead(enroll) == 0)
{
return;
}
}
}
uint8_t getFingerprintEnroll()
{
int p = -1;
lcd.clear();
lcd.print("finger ID:");
lcd.print(id);
lcd.setCursor(0,1);
lcd.print("Place Finger");
delay(2000);
while (p != FINGERPRINT_OK)
{
p = finger.getImage();
switch (p)
{
case FINGERPRINT_OK:
Serial.println("Image taken");
lcd.clear();
lcd.print("Image taken");
break;
case FINGERPRINT_NOFINGER:
Serial.println("No Finger");
lcd.clear();
lcd.print("No Finger");
break;
case FINGERPRINT_PACKETRECIEVEERR:
Serial.println("Communication error");
lcd.clear();
lcd.print("Comm Error");
break;
case FINGERPRINT_IMAGEFAIL:
Serial.println("Imaging error");
lcd.clear();
lcd.print("Imaging Error");
break;
default:
Serial.println("Unknown error");
lcd.clear();
lcd.print("Unknown Error");
break;
}
}
// OK success!
p = finger.image2Tz(1);
switch (p) {
case FINGERPRINT_OK:
Serial.println("Image converted");
lcd.clear();
lcd.print("Image converted");
break;
case FINGERPRINT_IMAGEMESS:
Serial.println("Image too messy");
lcd.clear();
lcd.print("Image too messy");
return p;
case FINGERPRINT_PACKETRECIEVEERR:
Serial.println("Communication error");
lcd.clear();
lcd.print("Comm Error");
return p;
case FINGERPRINT_FEATUREFAIL:
Serial.println("Could not find fingerprint features");
lcd.clear();
lcd.print("Feature Not Found");
return p;
case FINGERPRINT_INVALIDIMAGE:
Serial.println("Could not find fingerprint features");
lcd.clear();
lcd.print("Feature Not Found");
return p;
default:
Serial.println("Unknown error");
lcd.clear();
lcd.print("Unknown Error");
return p;
}
Serial.println("Remove finger");
lcd.clear();
lcd.print("Remove Finger");
delay(2000);
p = 0;
while (p != FINGERPRINT_NOFINGER) {
p = finger.getImage();
}
Serial.print("ID "); Serial.println(id);
p = -1;
Serial.println("Place same finger again");
lcd.clear();
lcd.print("Place Finger");
lcd.setCursor(0,1);
lcd.print(" Again");
while (p != FINGERPRINT_OK) {
p = finger.getImage();
switch (p) {
case FINGERPRINT_OK:
Serial.println("Image taken");
break;
case FINGERPRINT_NOFINGER:
Serial.print(".");
break;
case FINGERPRINT_PACKETRECIEVEERR:
Serial.println("Communication error");
break;
case FINGERPRINT_IMAGEFAIL:
Serial.println("Imaging error");
break;
default:
Serial.println("Unknown error");
return;
}
}
// OK success!
p = finger.image2Tz(2);
switch (p) {
case FINGERPRINT_OK:
Serial.println("Image converted");
break;
case FINGERPRINT_IMAGEMESS:
Serial.println("Image too messy");
return p;
case FINGERPRINT_PACKETRECIEVEERR:
Serial.println("Communication error");
return p;
case FINGERPRINT_FEATUREFAIL:
Serial.println("Could not find fingerprint features");
return p;
case FINGERPRINT_INVALIDIMAGE:
Serial.println("Could not find fingerprint features");
return p;
default:
Serial.println("Unknown error");
return p;
}
// OK converted!
Serial.print("Creating model for #"); Serial.println(id);
p = finger.createModel();
if (p == FINGERPRINT_OK) {
Serial.println("Prints matched!");
} else if (p == FINGERPRINT_PACKETRECIEVEERR) {
Serial.println("Communication error");
return p;
} else if (p == FINGERPRINT_ENROLLMISMATCH) {
Serial.println("Fingerprints did not match");
return p;
} else {
Serial.println("Unknown error");
return p;
}
Serial.print("ID "); Serial.println(id);
p = finger.storeModel(id);
if (p == FINGERPRINT_OK) {
Serial.println("Stored!");
lcd.clear();
lcd.print("Stored!");
delay(2000);
} else if (p == FINGERPRINT_PACKETRECIEVEERR) {
Serial.println("Communication error");
return p;
} else if (p == FINGERPRINT_BADLOCATION) {
Serial.println("Could not store in that location");
return p;
} else if (p == FINGERPRINT_FLASHERR) {
Serial.println("Error writing to flash");
return p;
}
else {
Serial.println("Unknown error");
return p;
}
}
int getFingerprintIDez()
{
uint8_t p = finger.getImage();
if (p != FINGERPRINT_OK)
return -1;
p = finger.image2Tz();
if (p != FINGERPRINT_OK)
return -1;
p = finger.fingerFastSearch();
if (p != FINGERPRINT_OK)
{
lcd.clear();
lcd.print("Finger Not Found");
lcd.setCursor(0,1);
lcd.print("Try Later");
delay(2000);
return -1;
}
// found a match!
Serial.print("Found ID #");
Serial.print(finger.fingerID);
return finger.fingerID;
}
uint8_t deleteFingerprint(uint8_t id)
{
uint8_t p = -1;
lcd.clear();
lcd.print("Please wait");
p = finger.deleteModel(id);
if (p == FINGERPRINT_OK)
{
Serial.println("Deleted!");
lcd.clear();
lcd.print("Figer Deleted");
lcd.setCursor(0,1);
lcd.print("Successfully");
delay(1000);
}
else
{
Serial.print("Something Wrong");
lcd.clear();
lcd.print("Something Wrong");
lcd.setCursor(0,1);
lcd.print("Try Again Later");
delay(2000);
return p;
}
}
void download(int eepIndex)
{
if(EEPROM.read(eepIndex) != 0xff)
{
Serial.print("T->");
if(EEPROM.read(eepIndex)<10)
Serial.print('0');
Serial.print(EEPROM.read(eepIndex++));
Serial.print(':');
if(EEPROM.read(eepIndex)<10)
Serial.print('0');
Serial.print(EEPROM.read(eepIndex++));
Serial.print(':');
if(EEPROM.read(eepIndex)<10)
Serial.print('0');
Serial.print(EEPROM.read(eepIndex++));
Serial.print(" D->");
if(EEPROM.read(eepIndex)<10)
Serial.print('0');
Serial.print(EEPROM.read(eepIndex++));
Serial.print('/');
if(EEPROM.read(eepIndex)<10)
Serial.print('0');
Serial.print(EEPROM.read(eepIndex++));
Serial.print('/');
Serial.print(EEPROM.read(eepIndex++)<<8 | EEPROM.read(eepIndex++));
}
else
{
Serial.print("---------------------------");
}
Serial.print(" ");
}
Comments
Hello community of circuit
Hello community of circuit digest
I have see your new posts those are amazing please send me the code of this
Project.
15 August
"Happy independence day"
God bless you all circuit digest communnity
i want to do this in my
i want to do this in my academic project,anyone help me?
Is it possible to introduce
Is it possible to introduce external storage like an sd card so that the system can accommodate more people to be enrolled in the system. If so please include in the circuit and also changes in the sketch(program)
same problem
for me also same problem.please if you know the solution then kindly send me reply through gmail
if you have the final working code please send me
if you have the final working code please send me it will be very helpful
Can I get the circuit diagram
Can I get the circuit diagram for fingerprint based biometric attendance system
Hi I'm select this project I
Hi I'm select this project I buy tha all components for this project and I extra security for password so I want circuit diagram and codeings so kindly request plz help me
Header Files
Hey did you ever get the header files? if so could you send them my way?
hey if you got the header files and code then please send me too
hey if you got the header files and code then please send me too it will be very helpfull
Biometric Attendance System
Please provide me the link to download the fingerprint module library for proteaus.
About this project
The code which u have given here, when I am trying to verify the code in arduino ide it shows me compilation error; error shown is:
compilation terminated.
exit status 1
Error compiling for board Arduino/Genuino Uno.
Can u plz help???
is ur error solver ?
is ur error solver ?
bcz i have the same error
store into SD card
Dear sir,
How can I store user data to SD card... can i get code please?
https://circuitdigest.com
https://circuitdigest.com/microcontroller-projects/arduino-data-logger-…
Use the link to learn how to store data to SD card. The code is also given in link
compiling error
sir send me the solution of the error code . how can i get complete running code
sir, why i got unknown error
sir, why i got unknown error when enroll data. the image converted then unknown error. pls help
what version of arduino
what version of arduino software that is compatible to upload the coding? kindly need your help asap. tq
Admin Mode
Hi,
Is there code with an Admin mode, where he/she can only add/delete ID's? Normal users will only be able to view and search.
How to add code to arduino
How to add code to arduino board after connecting to pc?can u pls explain me about!!!!!
Now by seeing your discription I got clarity with my PROJECT,it makes me to get into my work if u pls reply to my above questions.
Thanking you in anticipetion
How did you saved the
How did you saved the recordsn a software system?
i want to do this in my
i want to do this in my academic project,anyone help me?
need a help related this project
you are using fingerprint but i am using rfid tag and nodemcu plz help me i stuck in hardware
i dont know how to send data from nodemcu to desktop application or if you can help me to send it to visual studio else i will take care of it
thankyou hoping a positive reply from CIrcuitDigest
Sir i have typed and run the
Sir i have typed and run the code in arduino IDE but it throws an error
C:\Users\Arun\Desktop\exp1\exp2\exp2.ino: In function 'uint8_t getFingerprintEnroll()':
C:\Users\Arun\Desktop\exp1\exp2\exp2.ino:493:7: warning: return-statement with no value, in function returning 'uint8_t {aka unsigned char}' [-fpermissive]
return;
Can you help me please?
Getting the same error
Getting the same error
/home/maxyspark/Arduino/finger/finger.ino: In function 'uint8_t getFingerprintEnroll()':
/home/maxyspark/Arduino/finger/finger.ino:493:7: warning: return-statement with no value, in function returning 'uint8_t {aka unsigned char}' [-fpermissive]
return;
^
/home/maxyspark/Arduino/libraries/Adafruit_Fingerprint_Sensor_Library-1.1.1/Adafruit_Fingerprint.cpp: In member function 'uint8_t Adafruit_Fingerprint::setPassword(uint32_t)':
/home/maxyspark/Arduino/libraries/Adafruit_Fingerprint_Sensor_Library-1.1.1/Adafruit_Fingerprint.cpp:268:54: warning: narrowing conversion of '(password >> 24)' from 'uint32_t {aka long unsigned int}' to 'uint8_t {aka unsigned char}' inside { } [-Wnarrowing]
SEND_CMD_PACKET(FINGERPRINT_SETPASSWORD, (password >> 24), (password >> 16), (password >> 8), password);
^
/home/maxyspark/Arduino/libraries/Adafruit_Fingerprint_Sensor_Library-1.1.1/Adafruit_Fingerprint.cpp:33:21: note: in definition of macro 'GET_CMD_PACKET'
uint8_t data[] = {__VA_ARGS__}; \
^
/home/maxyspark/Arduino/libraries/Adafruit_Fingerprint_Sensor_Library-1.1.1/Adafruit_Fingerprint.cpp:268:3: note: in expansion of macro 'SEND_CMD_PACKET'
SEND_CMD_PACKET(FINGERPRINT_SETPASSWORD, (password >> 24), (password >> 16), (password >> 8), password);
^
/home/maxyspark/Arduino/libraries/Adafruit_Fingerprint_Sensor_Library-1.1.1/Adafruit_Fingerprint.cpp:268:72: warning: narrowing conversion of '(password >> 16)' from 'uint32_t {aka long unsigned int}' to 'uint8_t {aka unsigned char}' inside { } [-Wnarrowing]
SEND_CMD_PACKET(FINGERPRINT_SETPASSWORD, (password >> 24), (password >> 16), (password >> 8), password);
^
/home/maxyspark/Arduino/libraries/Adafruit_Fingerprint_Sensor_Library-1.1.1/Adafruit_Fingerprint.cpp:33:21: note: in definition of macro 'GET_CMD_PACKET'
uint8_t data[] = {__VA_ARGS__}; \
^
/home/maxyspark/Arduino/libraries/Adafruit_Fingerprint_Sensor_Library-1.1.1/Adafruit_Fingerprint.cpp:268:3: note: in expansion of macro 'SEND_CMD_PACKET'
SEND_CMD_PACKET(FINGERPRINT_SETPASSWORD, (password >> 24), (password >> 16), (password >> 8), password);
^
/home/maxyspark/Arduino/libraries/Adafruit_Fingerprint_Sensor_Library-1.1.1/Adafruit_Fingerprint.cpp:268:90: warning: narrowing conversion of '(password >> 8)' from 'uint32_t {aka long unsigned int}' to 'uint8_t {aka unsigned char}' inside { } [-Wnarrowing]
SEND_CMD_PACKET(FINGERPRINT_SETPASSWORD, (password >> 24), (password >> 16), (password >> 8), password);
^
/home/maxyspark/Arduino/libraries/Adafruit_Fingerprint_Sensor_Library-1.1.1/Adafruit_Fingerprint.cpp:33:21: note: in definition of macro 'GET_CMD_PACKET'
uint8_t data[] = {__VA_ARGS__}; \
^
/home/maxyspark/Arduino/libraries/Adafruit_Fingerprint_Sensor_Library-1.1.1/Adafruit_Fingerprint.cpp:268:3: note: in expansion of macro 'SEND_CMD_PACKET'
SEND_CMD_PACKET(FINGERPRINT_SETPASSWORD, (password >> 24), (password >> 16), (password >> 8), password);
^
/home/maxyspark/Arduino/libraries/Adafruit_Fingerprint_Sensor_Library-1.1.1/Adafruit_Fingerprint.cpp:33:32: warning: narrowing conversion of 'password' from 'uint32_t {aka long unsigned int}' to 'uint8_t {aka unsigned char}' inside { } [-Wnarrowing]
uint8_t data[] = {__VA_ARGS__}; \
^
/home/maxyspark/Arduino/libraries/Adafruit_Fingerprint_Sensor_Library-1.1.1/Adafruit_Fingerprint.cpp:39:30: note: in expansion of macro 'GET_CMD_PACKET'
#define SEND_CMD_PACKET(...) GET_CMD_PACKET(__VA_ARGS__); return packet.data[0];
^
/home/maxyspark/Arduino/libraries/Adafruit_Fingerprint_Sensor_Library-1.1.1/Adafruit_Fingerprint.cpp:268:3: note: in expansion of macro 'SEND_CMD_PACKET'
SEND_CMD_PACKET(FINGERPRINT_SETPASSWORD, (password >> 24), (password >> 16), (password >> 8), password);
I have the same error
I have the same error if you found the solution can you please send me the code it will be very helpfull its my college project and i have to submit this.Please kindly reply.
This is very helpful.
This is very helpful.
Could you make an attendance list system using RFID and Arduino?
little problem with fingerprint module
Hello
I used fingerprint module gt511c3 for this project and its not working with the code any solution for fixing this I've searched about this and some websites showing gt511c3 have seperate coding or something like that plzz help me to find any solution
Biomatric attendance system
Sir i am also making this type of project for my collage. Can u please tell me that ehich type of fingerprint sensor is best for this project or can i used normal usb fingerprint sensor for this priject suggest me cheap fingerprint sensor .
Code is not working
Sir i made this project and circuit connections are correct but when I'm running the code its is showing this message....
Sketch uses 13022 bytes (40%) of program storage space. Maximum is 32256 bytes.
Global variables use 1602 bytes (78%) of dynamic memory, leaving 446 bytes for local variables. Maximum is 2048 bytes.
Low memory available, stability problems may occur.
An error occurred while uploading the sketch
And after that LCD is showing nothing but only 16 lines. So it would be very helpful for me if u can help me through this problem.
sir i too have the same
sir i too have the same problem . plss help me to clear the error
Error in program
Undeclared error and many other error come su plz send me correct code plz sir plz
error--in--program
Sir, in this code there comes an error in line 405 .Why?
to clear the error
hello sir, i found errors in the above program like RTC_DS1307, void loop(){, void setup() {, if(! rtc.begin() ), if(! rtc.isrunning()), and now= rtc.now(); . sir please send me a copy of a correct program or tell me how to clear the errors in this program(send me the procedure on this). (sir please reply for this , its an emergency).
Intellegent one!
Sir, thank you. Please support me, since it is a best project. If you possible, just send the code and document of this project.
Sir please send the correct
Sir please send the correct code of this project
Sir I got one error
Sir I got one error
Adafruit_Fingerprint.h file not found in below line
#include<Adafruit_Fingerprint.h>
Old help me asap
Proteus library for r305
hello sir.. i am not able to find the proteus library for the fingerprint sensor module. can you please help me out of this? and it's too urgent.
There is no finger print
There is no finger print library available for Proteus then used here is custom build just to show you how the connections should be made. It cannot be simulated.
Project
My project is related to this project .
Its name is bike security system using fingerprint scanner / password and gsm module
Component -GSM module 900,fingerprint scanner 305,keypad ,buzzer ,arduno uno ,DC motor ,speaker , microphone ,etc.
So can you help me to interfacing and programming this project or give me hint , how to ready this project
hello!! please how can the
hello!! please how can the system be modified so that I can have a remote database and each time the system takes attendance, it should update the database remotely. I want a system that the database can be queried for students attendance record for any course at any given time or semester
how to feed data to webpage and sd card using arduino
do we need any other library file for ardunio other than adafruit for mega
Need help
How can fix the error like ....Arduino/libraries/Adafruit_Fingerprint_Sensor_Library-1.1.1/Adafruit_Fingerprint.cpp:268:3: note: in expansion of macro 'SEND_CMD_PACKET'
SEND_CMD_PACKET(FINGERPRINT_SETPASSWORD, (password >> 24), (password >> 16), (password >> 8), password);.
thank you for your help.
hey sir how do u doing i am
hey sir how do u doing i am doing this project also for my semister project and this is very helpful idea and i need to store mare students then i have to use the sd card and also thre is no fingerprint library for proteus do you have any idea about this if so please give me your hand.
Error
Sir I have Received this type of error
How Can I Solve this type of error plz
Board: "Arduino/Genuino Uno"
fatal error: RTClib.h: No such file or directory
#include <RTClib.h>
^
compilation terminated.
exit status 1
Error compiling for board Arduino/Genuino Uno.
arduino&protues
how can i get finger print&rtc module from protues for design?. plz help this is my semester project
Hello sir, in datasheet of
Hello sir, in datasheet of fingerprint sensor written that 1000 pictures can storage.
Now I want to know, can I use "id" number above 255. because 8 bit system.
Please help me.
N.B.: I used maximum 161 id And use SD card, GSM with it for 1 year attendance system.
sir i have this error please
sir i have this error please help me
Sketch uses 13150 bytes (40%) of program storage space. Maximum is 32256 bytes.
Global variables use 1624 bytes (79%) of dynamic memory, leaving 424 bytes for local variables. Maximum is 2048 bytes.
Low memory available, stability problems may occur.
Attendence system
First of all very interesting project.
i have successfully completed this project on 20 june 2019.
there were some issues in circuit diagram.
1) i got error "module not found" that i resolved by flipping the pins.
2) enroll and other buttons were not stable. i added pull-up resistors .
if anyone need help, contact me on asif5955iqbal@yahoo.com
Regards:
Asif Iqbal
PAKISTAN,LAHORE
why i multiply by 7 and then
why i multiply by 7 and then the result is added by 210?please help..
PLS HOW DO I INCREASE THE
PLS HOW DO I INCREASE THE NUMBER OF PEOLPE IT CAN CAPTURE.. CAN U SEND ME THE CODE TO USE
THANKS
PLS HOW DO I INCREASE THE
PLS HOW DO I INCREASE THE NUMBER OF PEOLPE IT CAN CAPTURE.. CAN U SEND ME THE CODE TO USE
THANKS
Hello sir, the code works…
Hello sir, the code works fine for me, but it stores sign in data only. Please help me provide the code for both sign in and sign out. Thanks you sir.
Sir is there any related circuit Aurduino controlled 8 players buzzer round..... If it is there then send me link plz.....