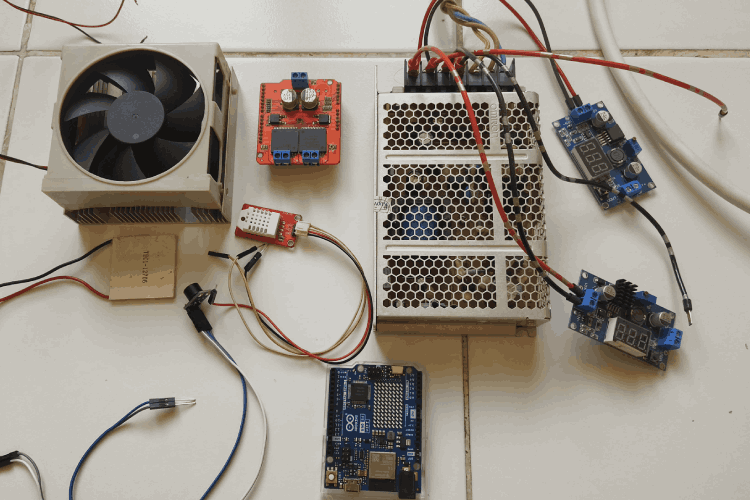
This method uses a heat pump to recycle the air used in drying, making it more energy efficient. This technology allows drying at lower temperatures and maintains the quality of the coffee beans. Heat pump drying is an efficient and environmentally friendly method because it reuses the heat generated to speed up drying. In this process, the heat pump functions to remove water vapor from the coffee beans by regulating the temperature and humidity in the drying chamber.
Heat Pump Drying Process Working Principle: Heat pumps work by absorbing heat from the environment and compressing it to a higher temperature, which is then used to circulate warm air into the drying chamber. This hot air is responsible for evaporating the water in the coffee beans.
Heat Recycling: This system recycles the hot air after the moisture has been removed, so that dry air with a stable temperature can be reused for drying. This reduces the energy requirement for continuously heating the air.
Temperature and Humidity Control: Heat pump technology allows for more precise control of temperature and humidity, usually at low temperatures (40–50°C). This lower temperature helps maintain the quality of the coffee's aroma and flavor and avoids damage to the beans.
Impact Statement
The project aims to monitor and control the post-harvest drying process of coffee beans, to reduce the water content in the coffee beans to a safe level, usually around 10-12%, so that the coffee beans can be stored for a long time without being damaged by microbial growth such as mold. The drying process is also important to maintain the quality of the taste and aroma of the coffee, because too high a water content can cause uncontrolled fermentation, destroying the desired taste characteristics.
Component Lists:
1. Arduino UNO R4 WiFi
2. Monster Motor Shield
3. Sensor DHT22
4. MLX90614 Infrared Thermometer
5. Fan 12V
6. Peltier
7. Omron Power Supply 24V 5A
8. Step Down
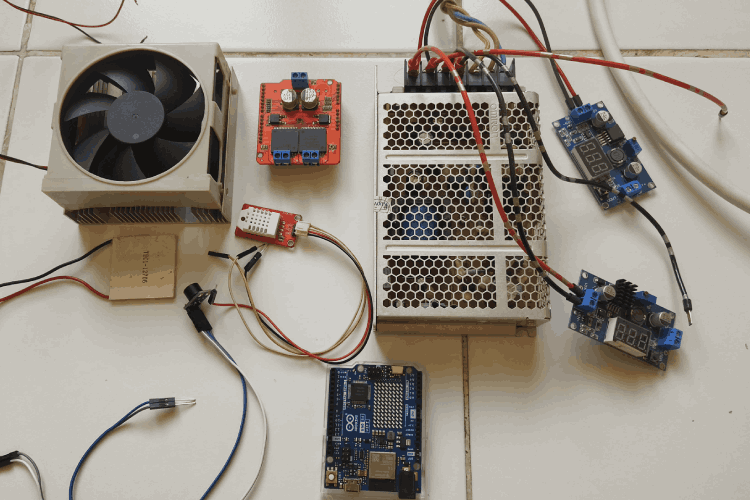
Above you can see the image of the selected components.
To see the full demonstration video, click on the YouTube Video below.
Circuit Diagram Explanation:
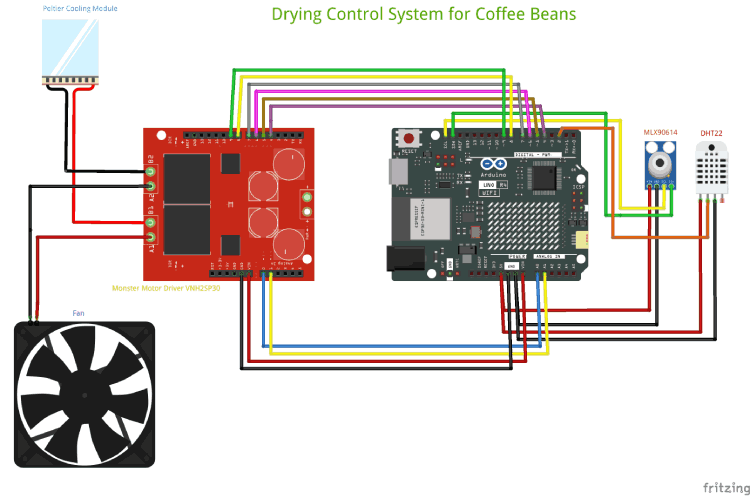
This circuit diagram represents a Drying Control System for Coffee Beans using an Arduino Uno R4 WiFi, a Monster Motor Driver (VNH2SP30), temperature and humidity sensors, a Peltier cooling module, and a fan.
Here’s a breakdown of each component and its connections:
Arduino Uno R4 WiFi: Acts as the central control unit for the drying system and reads data from sensors (temperature and humidity) and controls the fan and Peltier module through the motor driver.
DHT22 Sensor: Measures temperature and humidity of the drying environment and connected to the Arduino to provide real-time humidity and temperature readings, which help to control the drying process.
MLX90614 Sensor: An infrared temperature sensor used to measure the temperature of the coffee beans without contact and connected to the Arduino to give more accurate temperature readings of the beans themselves.
Monster Motor Driver (VNH2SP30): Controls the fan and peltier cooling module based on inputs from the Arduino. This driver allows the Arduino to handle higher power requirements for the fan and peltier module, which operate on higher currents than the Arduino can provide directly. This shield contains a pair of VNH2SP30 full-bridge motor drivers. This driver has a higher current tolerance in compare to its similar drivers. This shield can withstand up to 14A continuous current. Its power supply should be between 5.5 and 16 volts. One of the control pins of this module is PWM. This pin is connected to the MOSFET or transistor control pin (the gate or the base), and the longer the duty cycle, the more voltage will be across the fan and peltier.
Fan: Assists in air circulation for the drying process and connected to the motor driver, enabling speed and power control based on drying requirements.
Peltier Cooling Module: Cools the surrounding air if the drying environment gets too warm, helping to maintain an optimal drying temperature and connected to the motor driver, which allows the Arduino to control when it should be turned on or off based on the temperature readings from the sensors.
Connections:
Sensors (DHT22 and MLX90614): Connected to the Arduino via digital and analog pins, which allows the Arduino to read real-time environmental data.
Motor Driver (VNH2SP30): Connects the fan and Peltier module to the Arduino. Power and ground connections are made to provide a stable power supply. Signal connections to the Arduino allow it to control the fan and Peltier module based on sensor readings. The Arduino reads temperature and humidity data from the DHT22 and MLX90614 sensors. Based on these readings, it adjusts the fan and Peltier cooling module through the Monster Motor Driver, optimizing the drying conditions for coffee beans. This system aims to maintain a controlled drying environment by regulating airflow and temperature.
Drying Monitoring System for Coffee Beans Code:
The setup part initializes WiFi, MQTT PubsubClient, ArduinoJson, Adafruit_MLX90614, DHT Sensor.
#include <Arduino.h>
#include <WiFiS3.h>
#include "ArduinoJson.h"
#include "PubSubClient.h"
#include "Adafruit_Sensor.h"
#include "DHT.h"
#include "Adafruit_MLX90614.h"
#include <SPI.h>
#include "config.h"
void initialize_wifi();
void publish_data();
boolean reconnect();
void callback(char* topic, byte* payload, unsigned int length);
WiFiClient net;
PubSubClient mqttClient(net);
#define DHTPIN 2
#define DHTTYPE DHT22
DHT dht(DHTPIN, DHTTYPE);
Adafruit_MLX90614 obj_temp = Adafruit_MLX90614();
Includes and Defines: Includes necessary libraries and defines pin numbers for sensors MLX90614 and DHT.
Global function: Declares global function for the air humidity and temperature from DHT and object temperature from MLX90614.
Setup Function: Initializes WiFi, publisher data from MQTT, reconnect MQTT, and MQTT Callback.
The publisher function is used to send the data air temperature, humidity, and object temperature to the ThingsBoard Cloud.
void publish_data()
{
JsonDocument doc;
float t = dht.readTemperature();
float h = dht.readHumidity();
if (isnan(t) || isnan(h))
{
Serial.println("Failed to read sensor");
return;
}
float temperature_obj = obj_temp.readObjectTempC();
doc["temperature"] = t;
doc["humidity"] = h;
doc["object_temperature"] = temperature_obj;
serializeJsonPretty(doc, data);
long now = millis();
if (now - lastData > 15000) //updated sensor to the cloud every 15 seconds
{
lastData = now;
Serial.println(data);
mqttClient.publish(PUBLISH_TOPIC, data);
}
}
Loop function: continuously checks for looping MQTT and updates sensor data to the cloud every 15 seconds.
void loop() {
if (WiFi.status() != WL_CONNECTED)
{
initialize_wifi();
}
if (WiFi.status() == WL_CONNECTED && !mqttClient.connected())
{
long now = millis();
if (now - lastReconnectAttempt > 2000)
{
lastReconnectAttempt = now;
if (reconnect())
{
lastReconnectAttempt = 0;
}
}
} else
{
mqttClient.loop();
}
publish_data();
}
The configuration: config.h is used to configure mqtt broker,wifi ssid, wifi password, mqtt username, mqtt password, and topic publisher and subscriber.#ifndef _CONFIG_H
#define _CONFIG_H
#include <Arduino.h>
// Fill in the hostname of your IoT broker
#define SECRET_BROKER "demo.thingsboard.io"
#defineMQTT_PORT1883
#define SSID_WIFI ""
#define PASSWORD_WIFI ""
#defineTOKEN ""
#defineMQTT_USERNAME ""
#defineMQTT_PASSWORD ""
#define PUBLISH_TOPIC "v1/devices/me/telemetry"
#define SUBSCRIBE_TOPIC "v1/devices/me/rpc/request/+"
Working Demonstration of the "Drying Monitoring System for Coffee Beans Based on IoT"
In the picture, it is a setup for real-time monitoring of environmental parameters like temperature and humidity. On the screen, a dashboard displays live graphs showing the values of parameters such as "object temperature," "temperature," and "humidity." There’s a line chart on the left tracking changes over time, and a bar chart on the right showing current readings for each parameter in Thingsboard Cloud.
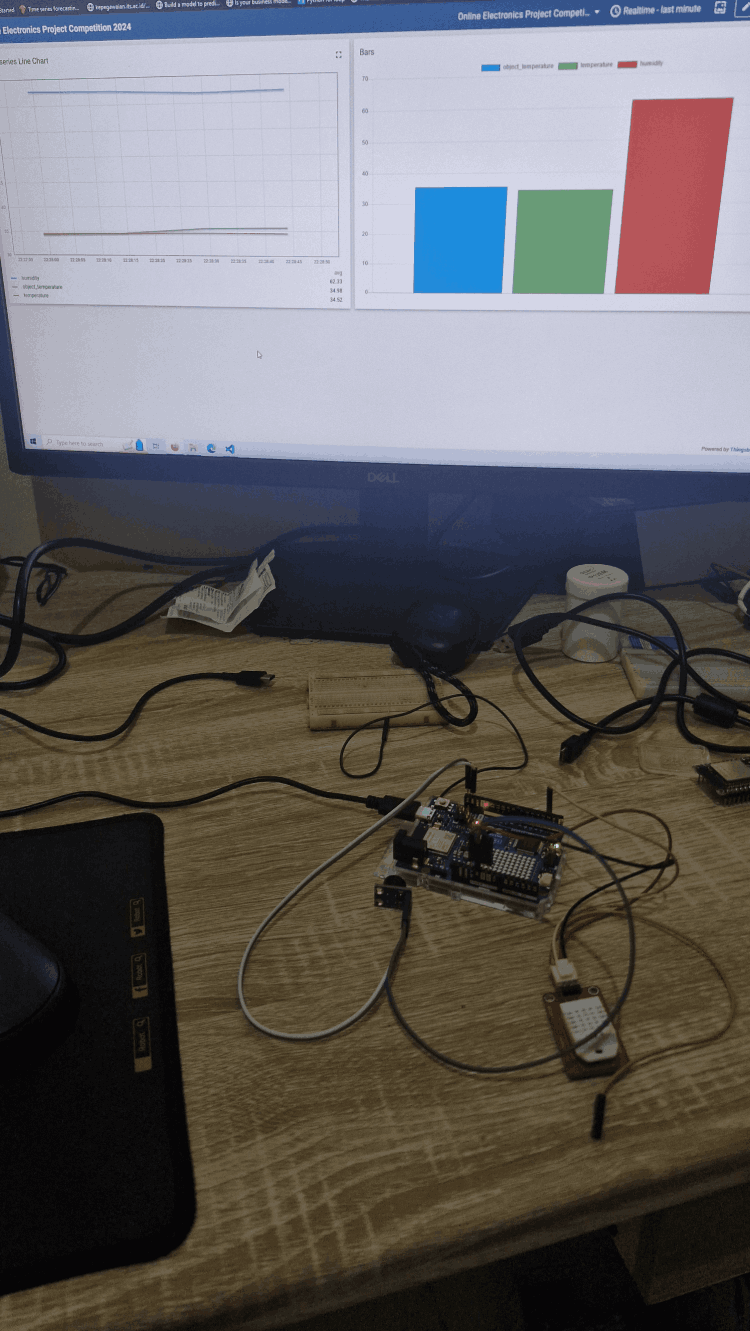
On the desk, there is an electronics setup connected to a microcontroller (Arduino UNO WiFi R4) with a sensor module attached, DHT22 for temperature and humidity, infrared MLX90614 for object temperature measurements.
The image shows a data visualization dashboard powered by Thingsboard, likely used for an electronics project in the 2024 Online Electronics Project Competition. This dashboard displays live environmental data, including humidity, object temperature, and ambient temperature, with values updated in real time.
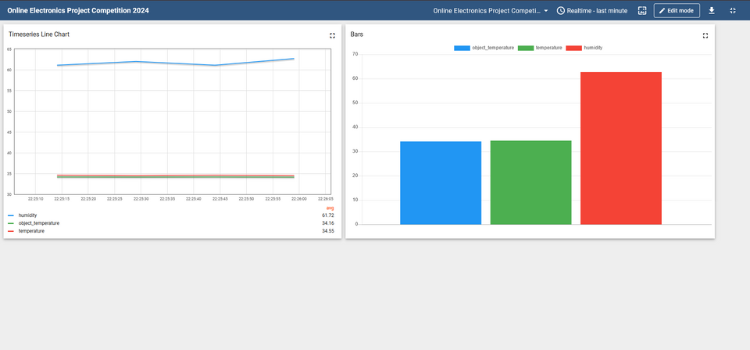
On the left side, a Timeseries Line Chart plots these parameters over time. The chart uses different colored lines to represent:
Humidity (in blue), which appears to have a relatively high value around 61.72 on average.
Object Temperature (in green), with an average of 34.16.
Temperature (in red), with an average of 34.55.
On the right side, a Bar Chart displays the current values of these parameters. The heights of the bars indicate:
Object Temperature at approximately 34.
Temperature at around 34.5.
Humidity at around 61.7, the highest among the three parameters.
This dashboard allows for easy real-time monitoring and comparison of these environmental factors, which are likely being gathered from connected IoT sensors as part of the project.
So, that’s it about the working of this project.
For all the code, follow the GitHub link below: