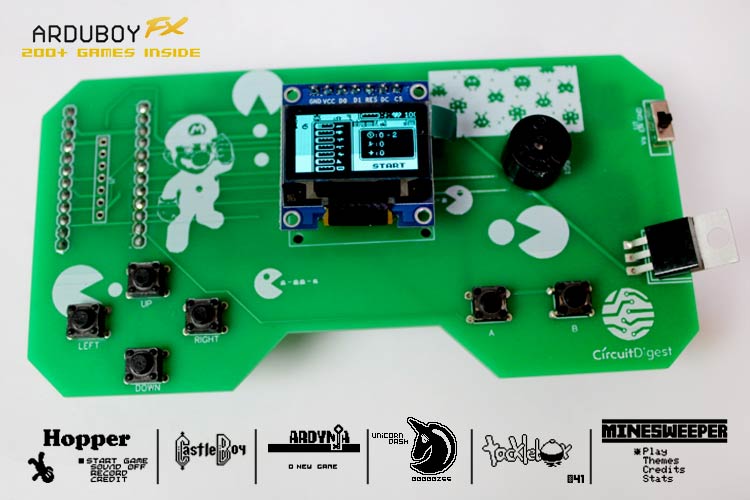
In this tutorial, we are going to build a handheld game console using Arduino Pro Micro, Arduboy package, and a handful of hardware. For those who don’t know, Arduboy is an 8-bit, credit-card-sized programmable gaming system inspired by Nintendo Gameboy. Arduboy is a game development system based on the popular open-source Arduino platform. It is really easy to learn, share, and play, allowing us to learn to code and create our games. The original Arduboy game package is based on an ATmega32U4 microcontroller and 128x64 Pixels serial OLED display. So, to build this project you should have an ATmega32u4-based Arduino microcontroller and a 6-pin SPI Based OLED display.
We have used NextPCB to fabricate the PCB boards for this project. NextPCB is an experienced PCB manufacturer specialized in the PCB manufacturing and assembly industry for more than 15 years. They provide high-quality industry-standard PCB with a very quick turnaround time, as low as 24 hours. They can also provide other turnkey solutions like component sourcing, PCB prototyping, quality testing, etc. We will explain more on how to use NextPCB later in this article, but for now, let’s discuss the design and working of our Handheld Game Console.
Components Required for Handheld Game Console
- 1x Arduino Pro Micro (5V)
- 1x OLED Display (SPI)
- 6x Tactile Push Button
- 1x Buzzer
- 1x 7805 Voltage Regulator
- 1x 4mm SPDT Slide Switch
- 2x 18650 Cell
- 1x 18650 Double Cell Battery Holder
Circuit Diagram for Arduino Handheld Game Console
The complete circuit diagram for building the Arduboy game clone is shown below. As this is a clone board, we used the same components as the original board. The schematic was drawn using EasyEDA. The circuit consists of an Arduino Pro Micro, SPI OLED Display Module, voltage regulator, and some push buttons.
This complete setup is powered by two 18650 cells connected in series. Output voltage from these cells will be around 7.4 so a 7805-voltage regulator is used to supply regulated 5V to Arduino pro micro and other modules. The complete connections are as follows:
Arduino Pro Micro |
OLED Display |
Vcc |
Vcc |
GND |
GND |
D15 |
SCL |
D16 |
SDA |
D4 |
DC |
D2 |
RES |
Arduino Pro Micro |
Push Buttons |
A0 |
UP |
A3 |
Down |
A1 |
Right |
A2 |
Left
|
D7 |
A |
D8 |
B |
Arduino Pro Micro |
Buzzer |
D5 |
Positive |
D6 |
GND |
Designing a PCB Arduboy
Now that we understand how the schematics work, we can proceed with building the PCB for our project. You can design the PCB using any PCB software of your choice. If you are a beginner who is just getting started in the world of PCB, we would recommend you to check out the getting started guide for PCB Designing. If you want to skip the design processes, you can also download the Gerber file of this Handheld Game Console project using the link below:
Download GERBER file for Arduboy
You can also check out the other PCB projects that we have previously built on Circuit Digest if you are interested. Now that our Design is ready, it's time to get them fabricated using the Gerber file. Getting your PCB fabricated from NextPCB is easy; simply follow the steps given below.
Step 1: Go to www.nextpcb.com sign up if this is your first time. Then, in the next window click on ‘Quote now’.
Step2: Now in the next window, upload the Gerber file, and fill out the other important details like dimensions of your PCB, the number of layers, and the number of PCBs you require.
In the next step, you have to select the build time, shipping country, and shipping method. The final cost of the PCB depends on the shipping country and shipping method.
The final step is Checkout. To make sure the process is smooth; NextPCB first verifies all the details and Gerber file before proceeding with the payment. This way, you can be sure that your PCB is fabrication-friendly and will reach you as committed.
Assembling our Handheld Game Console Board
After a few days, we received our PCB from NextPCB in a neat package and as you can see below, the PCB quality was good as always. The top layer and bottom layer were seamlessly done with proper visa and track spacing. The top layer and the bottom layer of the board are shown below.
After making sure that the tracks and footprints were correct, I proceeded with assembling the PCB. The completely soldered board looked like as shown in the image below:
Programming Arduino Pro Micro for Playing Arduboy-Homemade Games
Now to play the Arduboy games with Arduino Pro Micro, we first have to add the Arduboy Homemade package on Arduino IDE. This package includes all Arduboy libraries with support for alternate displays and wiring.
To install the Arduboy package, Open the Arduino IDE and then go to File > Preferences.
Then copy and paste the URL below in the Additional Boards Manager URLs box and click on OK
Then go to Tools > Boards > Board manager and in the text box type homemade or Arduboy homemade. Click on the Arduboy Homemade package and click the Install button.
With this, we have added the Arduboy package on Arduino IDE and now we can download and play our favorite Arduboy game on our Game Console. So, go ahead and download any game from Arduboy. After that, open up the .ino from the game folder. Then go to the Tools menu and make the selection as follows:
Board: Arduboy
Bootloader: Cathy3K
Core: Arduboy optimized core
After that, select the port to which Arduino micro is connected and hit upload.
Testing our Arduino based Gameboy
After uploading the code plug in the Arduino and 18650 cells and you should see the OLED display start up a demo of the game. Push button A is used to start and stop the game while Push button B is used for Attack.
I hope you enjoyed building this project. A complete working video is given below. If you have any questions, please leave them in the comment section.
Complete Project Code
#include "Globals.h"
#include "Draw.h"
#include "Sprites.h"
#include "Items.h"
itemsManager iM;
#include "Animations.h"
animationManager aM;
#include "Player.h"
playerTank pT;
#include "Projectiles.h"
projectileManager pM;
#include "Enemys.h"
enemyManager eM;
#include "Map.h"
mapManager mM;
#include "Spawn.h"
spawnManager sM;
#include "Buttons.h"
#include "Checks.h"
void setup() {
#ifdef USE_SERIAL
Serial.begin(57600);
Serial.println(F("MicroTank!"));
#endif
#ifdef ESP8266
ps2x.config_gamepad(PS2_CLK, PS2_CMD, PS2_SEL, PS2_DAT, false, false);
// this function must be called or the esp will crash with the first call
arduboy.setExternalButtonsHandler(getButtons);
#endif
arduboy.begin();
arduboy.setFrameRate(GAME_FRAMES);
// some start tone
sound.tone(200, 200, 300, 200);
arduboy.fillScreen(WHITE);
sM.init();
pT.init();
//eM.add(100, 30, ENEMY_MINE, 0);
//eM.add(100, 30, ENEMY_ROCKET, 0);
//arduboy.display();
//delay(60000);
}
void playMode() {
checkItemsCatch();
checkItemsSpawn();
checkEnemyChrush();
checkProjectiles();
mM.update();
sM.update();
pM.update();
iM.update();
eM.update();
aM.update();
pT.update();
checkSlowmo();
checkSpecialAttack();
}
void menuMode() {
mM.drawMap();
pT.drawTankShop();
}
void playGame() {
#ifdef DEBUG_FRAME_TIME
uint32_t start = millis();
#endif
prepareThings();
updateGameButtons();
if (isPlayMode) {
playMode();
} else {
menuMode();
}
// needs to be after the drawMap
pT.drawCornerInfo();
#ifdef DEBUG_FRAME_TIME
// pirnt time for a frame to screen
arduboy.fillRect(0, 0, 26, 7, BLACK);
//drawNumbers(0, 0, millis() - start);
//drawNumbers(0, 0, eM.count());
//drawNumbers(0, 0, sM.difficulty);
#endif
}
void loop() {
if (!(arduboy.nextFrame()))
return;
arduboy.pollButtons();
arduboy.fillScreen(WHITE);
if (isStartAnimation) {
playAnimation();
} else {
playGame();
}
arduboy.display();
gameFrames++;
}