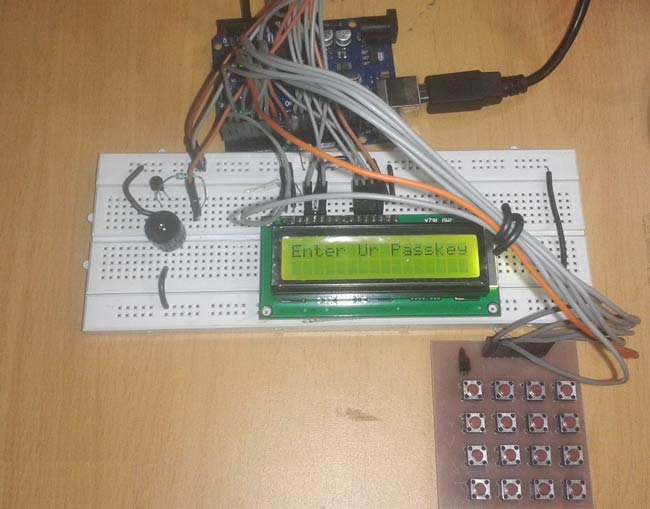
Security is a major concern in our day to day life, and digital locks have became an important part of these security systems. One such digital code lock is imitated in this project using arduino board and a matrix keypad.
Components
- Arduino
- Keypad Module
- Buzzer
- 16x2 LCD
- BC547 Transistor
- Resistor (1k)
- Bread board
- Power
- Connecting wires
In this circuit we have used multiplexing technique to interface keypad for input the password in the system. Here we are using 4x4 keypad which contains 16 key. If we want to use 16 keys then we need 16 pin for connection to arduino but in multiplexing technique we need to use only 8 pin for interfacing 16 keys. So that it is a smart way to interface a keypad module. [Also check: Keypad Interfacing with Arduino]
Multiplexing Technique: Multiplexing technique is a very efficient way to reduce number of pins used with the microcontroller for providing input or password or numbers. Basically this technique is used in two ways - one is row scanning and other one is colon scanning. But in this arduino based project we have used keypad library so we do not need to make any multiplexing code for this system. We only need to use keypad library for providing input.
Circuit Description
Circuit of this project is very simple which contains Arduino, keypad module, buzzer and LCD. Arduino controls the complete processes like taking password form keypad module, comparing passwords, driving buzzer and sending status to LCD display. Keypad is used for taking password. Buzzer is used for indications and LCD is used for displaying status or messages on it. Buzzer is driven by using a NPN transistor.
Keypad module’s Column pins are directly connected to pin 4, 5, 6, 7 and Row pins are connected to 3, 2, 1, 0 of arduino uno. A 16x2 LCD is connected with arduino in 4-bit mode. Control pin RS, RW and En are directly connected to arduino pin 13, GND and 12. And data pin D4-D7 is connected to pins 11, 10, 9 and 8 of arduino. And one buzzer is connected at pin 14(A1) of arduino through a BC547 NPN transistor.
Working
We have used inbuilt arduino’s EEPROM to save password, so when we run this circuit first time program read a garbage data from inbuilt arduino’s EEPROM and compare it with input password and give a message on LCD that is Access Denied because password does not match. For solving this problem we need to set a default password for the first time by using programming given below:
for(int j=0;j<4;j++) EEPROM.write(j, j+49);
lcd.print("Enter Ur Passkey:"); lcd.setCursor(0,1); for(int j=0;j<4;j++) pass[j]=EEPROM.read(j);
This will set password “1234” to EEPROM of Arduino.
After running it first time we need to remove this from program and again write the code in to the arduino and run. Now your system will run fine. And for your second time used password is now “1234”. Now you can change it by pressing # button and then enter your current password and then enter your new password.
When you will enter your password, system will compare your entered password with that password that is stored in EEPROM of arduino. If match is occurred then LCD will show “access granted” and if password is wrong then LCD will “Access Denied” and buzzer continuously beep for some time. And buzzer is also beep a single time whenever user will press any button from keypad.
Programming Description
In code we have used keypad library for interfacing keypad with arduino.
#include <Keypad.h> #include<LiquidCrystal.h> #include<EEPROM.h>
const byte ROWS = 4; //four rows const byte COLS = 4; //four columns char hexaKeys[ROWS][COLS] = { {'1','2','3','A'}, {'4','5','6','B'}, {'7','8','9','C'}, {'*','0','#','D'} }; byte rowPins[ROWS] = {3, 2, 1, 0}; //connect to the row pinouts of the keypad byte colPins[COLS] = {4, 5, 6, 7}; //connect to the column pinouts of the keypad //initialize an instance of class NewKeypad Keypad customKeypad = Keypad( makeKeymap(hexaKeys), rowPins, colPins, ROWS, COLS);
We have included LCD library for LCD interfacing and for interfacing EEPROM we have included library EEPROM.h., and then initialized variable and defined pins for components.
#define buzzer 15 LiquidCrystal lcd(13,12,11,10,9,8); char password[4]; char pass[4],pass1[4]; int i=0; char customKey=0;
And then we initialized LCD and give direction to pins in setup function
void setup() { lcd.begin(16,2); pinMode(led, OUTPUT); pinMode(buzzer, OUTPUT); pinMode(m11, OUTPUT); pinMode(m12, OUTPUT); lcd.print(" Electronic "); lcd.setCursor(0,1); lcd.print(" Keypad Lock "); delay(2000); lcd.clear(); lcd.print("Enter Ur Passkey:"); lcd.setCursor(0,1);
After this we read keypad in loop function
customKey = customKeypad.getKey(); if(customKey=='#') change(); if (customKey) { password[i++]=customKey; lcd.print(customKey); beep(); }
And then compare password with save password using string compare method.
if(i==4) { delay(200); for(int j=0;j<4;j++) pass[j]=EEPROM.read(j); if(!(strncmp(password, pass,4))) { digitalWrite(led, HIGH); beep(); lcd.clear(); lcd.print("Passkey Accepted"); delay(2000); lcd.setCursor(0,1); lcd.print("#.Change Passkey"); delay(2000); lcd.clear(); lcd.print("Enter Passkey:"); lcd.setCursor(0,1); i=0; digitalWrite(led, LOW); }
This is password change function and buzzer beep function
void change() { int j=0; lcd.clear(); lcd.print("UR Current Passk"); lcd.setCursor(0,1); while(j<4) { char key=customKeypad.getKey(); if(key) { pass1[j++]=key; lcd.print(key); void beep() { digitalWrite(buzzer, HIGH); delay(20); digitalWrite(buzzer, LOW); }
Complete Project Code
#include <Keypad.h>
#include<LiquidCrystal.h>
#include<EEPROM.h>
#define buzzer 15
LiquidCrystal lcd(13,12,11,10,9,8);
char password[4];
char pass[4],pass1[4];
int i=0;
char customKey=0;
const byte ROWS = 4; //four rows
const byte COLS = 4; //four columns
char hexaKeys[ROWS][COLS] = {
{'1','2','3','A'},
{'4','5','6','B'},
{'7','8','9','C'},
{'*','0','#','D'}
};
byte rowPins[ROWS] = {3, 2, 1, 0}; //connect to the row pinouts of the keypad
byte colPins[COLS] = {4, 5, 6, 7}; //connect to the column pinouts of the keypad
//initialize an instance of class NewKeypad
Keypad customKeypad = Keypad( makeKeymap(hexaKeys), rowPins, colPins, ROWS, COLS);
void setup()
{
lcd.begin(16,2);
pinMode(led, OUTPUT);
pinMode(buzzer, OUTPUT);
pinMode(m11, OUTPUT);
pinMode(m12, OUTPUT);
lcd.print(" Electronic ");
lcd.setCursor(0,1);
lcd.print(" Keypad Lock ");
delay(2000);
lcd.clear();
lcd.print("Enter Ur Passkey:");
lcd.setCursor(0,1);
for(int j=0;j<4;j++)
EEPROM.write(j, j+49);
for(int j=0;j<4;j++)
pass[j]=EEPROM.read(j);
}
void loop()
{
customKey = customKeypad.getKey();
if(customKey=='#')
change();
if (customKey)
{
password[i++]=customKey;
lcd.print(customKey);
beep();
}
if(i==4)
{
delay(200);
for(int j=0;j<4;j++)
pass[j]=EEPROM.read(j);
if(!(strncmp(password, pass,4)))
{
digitalWrite(led, HIGH);
beep();
lcd.clear();
lcd.print("Passkey Accepted");
delay(2000);
lcd.setCursor(0,1);
lcd.print("#.Change Passkey");
delay(2000);
lcd.clear();
lcd.print("Enter Passkey:");
lcd.setCursor(0,1);
i=0;
digitalWrite(led, LOW);
}
else
{
digitalWrite(buzzer, HIGH);
lcd.clear();
lcd.print("Access Denied...");
lcd.setCursor(0,1);
lcd.print("#.Change Passkey");
delay(2000);
lcd.clear();
lcd.print("Enter Passkey:");
lcd.setCursor(0,1);
i=0;
digitalWrite(buzzer, LOW);
}
}
}
void change()
{
int j=0;
lcd.clear();
lcd.print("UR Current Passk");
lcd.setCursor(0,1);
while(j<4)
{
char key=customKeypad.getKey();
if(key)
{
pass1[j++]=key;
lcd.print(key);
beep();
}
key=0;
}
delay(500);
if((strncmp(pass1, pass, 4)))
{
lcd.clear();
lcd.print("Wrong Passkey...");
lcd.setCursor(0,1);
lcd.print("Better Luck Again");
delay(1000);
}
else
{
j=0;
lcd.clear();
lcd.print("Enter New Passk:");
lcd.setCursor(0,1);
while(j<4)
{
char key=customKeypad.getKey();
if(key)
{
pass[j]=key;
lcd.print(key);
EEPROM.write(j,key);
j++;
beep();
}
}
lcd.print(" Done......");
delay(1000);
}
lcd.clear();
lcd.print("Enter Ur Passk:");
lcd.setCursor(0,1);
customKey=0;
}
void beep()
{
digitalWrite(buzzer, HIGH);
delay(20);
digitalWrite(buzzer, LOW);
}
Comments
When reset the password is default password.but we are the followers of yours need when we reset the changed password must be there
first upload the code
then remove these lines
for(int j=0;j<4;j++)
EEPROM.write(j, j+49);
the reupload it its work fine
can i know what is the m11 and m12 as output? what are those. i am new to arduino please help:)
compilation terminated.
exit status 1
Error compiling for board Arduino/Genuino Uno.
hello, please help. this is the error message i got when i tried verifying the full code on my arduino software
#include <Keypad.h>
this line is correct or not
because my arduino software
it show
compilation terminated
exit status 1
error compilation for board arduino/genuino uno
what is that mean?
You may need to install the Keypad Library, please visit this link: http://playground.arduino.cc/Code/Keypad
This is ok and have a problem what if someone pressed only 2 digit and left. How will you reset your system to execute from setup again
#include <Keypad.h>
this line is correct or not
because my arduino software
it show
how to encrypt the passkey
Dobrý den,
vyzkoušel jsem Váš návrh Digital Code Lock using Arduino,a funguje velice pěkně.
Bylo by možné, aby se v pracovním módu ukazovaly při vkládání pouze hvězdičky, aby na display nebylo vidět heslo? Je možné doplnit kód o zprovoznění pinMode(m11, OUTPUT);
pinMode(m12, OUTPUT); pinMode(led, OUTPUT);?
Jinak jsem nadšený z Vašeho řešení pomocí Arduino Uno a LCD, opravdu pěkné.
Radek
led was not declared in this scope
pinMode(led, OUTPUT);
pinMode(buzzer, OUTPUT);
pinMode(m11, OUTPUT);
pinMode(m12, OUTPUT);
whta sholud be here it is constatntly giving me errors plzzz help me plzzzzzzzzzzzzzzzzz
it shows error: 'EEPROM' was not declared in this scope. but i already import that library in these code but still it appears an error? how can i fix it ?
Hi Saddam!
Thanks for the tip. This is a great help. I will try to use EEPROM.h instead of Password.h
Thank you!
it is showing error dat" led is not declared".....wat shud i do now?????
nice bro,thanks
the right code after modification
#include <Keypad.h>
#include<LiquidCrystal.h>
#include<EEPROM.h>
#define buzzer 15
LiquidCrystal lcd(13,12,11,10,9,8);
char password[4];
char pass[4],pass1[4];
int i=0;
char customKey=0;
const byte ROWS = 4; //four rows
const byte COLS = 4; //four columns
char hexaKeys[ROWS][COLS] = {
{'1','2','3','A'},
{'4','5','6','B'},
{'7','8','9','C'},
{'*','0','#','D'}
};
byte rowPins[ROWS] = {3, 2, 1, 0}; //connect to the row pinouts of the keypad
byte colPins[COLS] = {4, 5, 6, 7}; //connect to the column pinouts of the keypad
//initialize an instance of class NewKeypad
Keypad customKeypad = Keypad( makeKeymap(hexaKeys), rowPins, colPins, ROWS, COLS);
void setup()
{
lcd.begin(16,2);
pinMode(buzzer, OUTPUT);
lcd.print(" Electronic ");
lcd.setCursor(0,1);
lcd.print(" Keypad Lock ");
delay(2000);
lcd.clear();
lcd.print("Enter Ur Passkey:");
lcd.setCursor(0,1);
for(int j=0;j<4;j++)
EEPROM.write(j, j+49);
for(int j=0;j<4;j++)
pass[j]=EEPROM.read(j);
}
void loop()
{
customKey = customKeypad.getKey();
if(customKey=='#')
change();
if (customKey)
{
password[i++]=customKey;
lcd.print(customKey);
beep();
}
if(i==4)
{
delay(200);
for(int j=0;j<4;j++)
pass[j]=EEPROM.read(j);
if(!(strncmp(password, pass,4)))
{
beep();
lcd.clear();
lcd.print("Passkey Accepted");
delay(2000);
lcd.setCursor(0,1);
lcd.print("#.Change Passkey");
delay(2000);
lcd.clear();
lcd.print("Enter Passkey:");
lcd.setCursor(0,1);
i=0;
}
else
{
digitalWrite(buzzer, HIGH);
lcd.clear();
lcd.print("Access Denied...");
lcd.setCursor(0,1);
lcd.print("#.Change Passkey");
delay(2000);
lcd.clear();
lcd.print("Enter Passkey:");
lcd.setCursor(0,1);
i=0;
digitalWrite(buzzer, LOW);
}
}
}
void change()
{
int j=0;
lcd.clear();
lcd.print("UR Current Passk");
lcd.setCursor(0,1);
while(j<4)
{
char key=customKeypad.getKey();
if(key)
{
pass1[j++]=key;
lcd.print(key);
beep();
}
key=0;
}
delay(500);
if((strncmp(pass1, pass, 4)))
{
lcd.clear();
lcd.print("Wrong Passkey...");
lcd.setCursor(0,1);
lcd.print("Better Luck Again");
delay(1000);
}
else
{
j=0;
lcd.clear();
lcd.print("Enter New Passk:");
lcd.setCursor(0,1);
while(j<4)
{
char key=customKeypad.getKey();
if(key)
{
pass[j]=key;
lcd.print(key);
EEPROM.write(j,key);
j++;
beep();
}
}
lcd.print(" Done......");
delay(1000);
}
lcd.clear();
lcd.print("Enter Ur Passk:");
lcd.setCursor(0,1);
customKey=0;
}
void beep()
{
digitalWrite(buzzer, HIGH);
delay(20);
digitalWrite(buzzer, LOW);
}
Excellent sujet , merci pour les explications///
Bonne continuation....
i am from somalia i want to design this project so can you help me i don't have any data about this project but i like to make this project thanx sir.
Thanks for posting this project. I've just completed it myself!
compilation terminated.
exit status 1
Error compiling for board Arduino/Genuino Uno.
how can i solve this error
Please the previous comment before posting, your question/issue might have answered already.
EEPROM.write(j, j+49);
may I know what value is j+49 is?
you write it in address "j" right??
ty for reply
I want to make this project can you give me the actual code with no errors ,and plz also tell it's some applications . Thank you
Anyone knows how to program pic16f877a my program is working on simulation mode but not on hardware any help plz...
https://circuitdigest.com/microcontroller-projects/led-blinking-with-pi…
Follow this tutorial and you will learn how to proceed with your Hardware in PIC
Hello, Dear
I am new and beginner in electronics. I want to build the above circuit, my proteus is not having the keypad shown here. I can only find the one with seven pins not eight. How can I get it?
Please help me!
Thank you!
what is use of transistor??? can't we connect buzzer without transistor???
Awesome work :)
code is showing error.
hi, i'm planning on making your digital code lock using arduino. i want to use a solenoid lock but i dont know the circuit diagram. can you help me? thanks