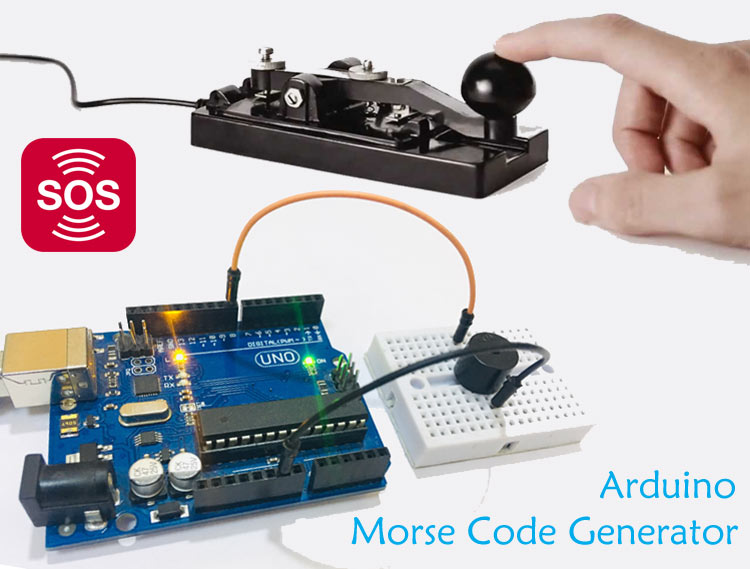
Morse code is a system of communication to encode any character in two different durations of signals called Dots and Dashes. Morse code is developed by Samuel F.B. and further used in telegraphy for transferring secret information. It was most used at the time of World War II. A Morse code can be performed by tapping, flashing light or writing. The Morse code is available in two versions, the original and the international morse code. In the international morse code, the original version is modified by removing spaces and designing the dashes in a specific length. The Morse code is available for encoding alphabets and numbers. It is mainly used in the radio and ocean communication and also a part of training for soldiers.
The language has always been the barrier for the Morse code, as it’s hard to perform the code for diacritic characters in other language. There are some famous words considered as important feature of Morse code like ‘SOS’. SOS full form is Save Our Souls created as a universal distress signal represents danger.
The below image shows the Morse code for the alphabets from A to Z.
Today in this tutorial we will build a Morse Code Translator using Arduino which will take any character as an input from serial monitor and convert it into Morse code equivalent beeps by buzzer.
Components Required
- Arduino Uno
- Buzzer
- Breadboard
- Jumper wires
Circuit Diagram
Connect the buzzer’s positive pin to the 8th pin of the Arudino UNO and the negative pin to the ground of Arduino. Upload the code using Arduino IDE and type the characters on the serial monitor to create the sound. A LCD can also be added with Arduino to display the dash and dots.
Programming Explanation
The complete code for Arduino Morse Code Generator is given at the end, here we are explaining the program to understand the working of the project.
The below code is used to receive the character string and then convert it into morse code.
char stringToMorseCode[] = "";
Then define the pin for the buzzer connected to the Arduino and the pitch for the sound generated by that buzzer. Then we are defining the length of dot and dash.
int audio8 = 8; // output audio on pin 8 int note = 1200; // music note/pitch int dotLen = 100; // length of the morse code 'dot' int dashLen = dotLen * 3; // length of the morse code 'dash'
In the void loop() function, if serial data is available, it will save into a variable indata. Then it reads the character one by one using command inData[index1]. The command variable.toUppercase() is used to change the lower case characters into uppercase. Then it creates sound according to every character.
void loop() { char inChar = 0; char inData[100] = ""; // data length of 6 characters String variable = ""; String variable1 = ""; int index1 = 0; if ( Serial.available() > 0 ) { while (Serial.available() > 0 && index1 < 100) { delay(100); inChar = Serial.read(); inData[index1] = inChar; index1++; inData[index1] = '\0'; } variable.toUpperCase(); for (byte i = 0 ; i < 100 ; i++) { variable.concat(String(inData[i])); } delay(20);
Below functions MorseDot and MorseDash are used to create the sound for dot and dash respectively.
void MorseDot() { tone(audio8, note, dotLen); // start playing a tone delay(dotLen); // hold in this position } void MorseDash() { tone(audio8, note, dashLen); // start playing a tone delay(dashLen); // hold in this position }
The GetChar function have the code for all the alphabets. So, whenever we type any alphabet, the respective morse code is taken from this function to create the particular sound.
void GetChar(char tmpChar) { switch (tmpChar) { case 'a': MorseDot(); delay(100); MorseDash(); delay(100); break; … … … default: break; } }
Now upload the code into Arduino using Arduino IDE and type any character into the serial monitor and hit the enter button to send the characters to the Arduino.
Here we have typed ‘SOS’ which is a universal distress signal, to create the sound for the same.
The demonstration for the same is given in the video below.
char stringToMorseCode[] = "";
int audio8 = 8; // output audio on pin 8
int note = 1200; // music note/pitch
int dotLen = 100; // length of the morse code 'dot'
int dashLen = dotLen * 3; // length of the morse code 'dash'
void setup() {
Serial.begin(9600);
}
void loop()
{
char inChar = 0;
char inData[100] = ""; // data length of 6 characters
String variable = "";
String variable1 = "";
int index1 = 0;
if ( Serial.available() > 0 ) { // Read from Rx from atmega16
while (Serial.available() > 0 && index1 < 100) // read till 6th character
{
delay(100);
inChar = Serial.read(); // start reading serilly and save to variable
inData[index1] = inChar;
index1++;
inData[index1] = '\0'; // Add a null at the end
}
variable.toUpperCase(); // convert to uppercase
for (byte i = 0 ; i < 100 ; i++) {
variable.concat(String(inData[i])); // concat strings
}
delay(20);
}
String stringToMorseCode = String(variable);
for (int i = 0; i < sizeof(stringToMorseCode) - 1; i++)
{
char tmpChar = stringToMorseCode[i];
tmpChar = toLowerCase(tmpChar);
GetChar(tmpChar);
}
}
void MorseDot()
{
tone(audio8, note, dotLen); // start playing a tone
delay(dotLen); // hold in this position
}
void MorseDash()
{
tone(audio8, note, dashLen); // start playing a tone
delay(dashLen); // hold in this position
}
void GetChar(char tmpChar)
{
switch (tmpChar) {
case 'a':
MorseDot();
delay(100);
MorseDash();
delay(100);
break;
case 'b':
MorseDash();
delay(100);
MorseDot();
delay(100);
MorseDot();
delay(100);
MorseDot();
delay(100);
break;
case 'c':
MorseDash();
delay(100);
MorseDot();
delay(100);
MorseDash();
delay(100);
MorseDot();
delay(100);
break;
case 'd':
MorseDash();
delay(100);
MorseDot();
delay(100);
MorseDot();
delay(100);
break;
case 'e':
MorseDot();
delay(100);
break;
case 'f':
MorseDot();
delay(100);
MorseDot();
delay(100);
MorseDash();
delay(100);
MorseDot();
delay(100);
break;
case 'g':
MorseDash();
delay(100);
MorseDash();
delay(100);
MorseDot();
delay(100);
break;
case 'h':
MorseDot();
delay(100);
MorseDot();
delay(100);
MorseDot();
delay(100);
MorseDot();
delay(100);
break;
case 'i':
MorseDot();
delay(100);
MorseDot();
delay(100);
break;
case 'j':
MorseDot();
delay(100);
MorseDash();
delay(100);
MorseDash();
delay(100);
MorseDash();
delay(100);
break;
case 'k':
MorseDash();
delay(100);
MorseDot();
delay(100);
MorseDash();
delay(100);
break;
case 'l':
MorseDot();
delay(100);
MorseDash();
delay(100);
MorseDot();
delay(100);
MorseDot();
delay(100);
break;
case 'm':
MorseDash();
delay(100);
MorseDash();
delay(100);
break;
case 'n':
MorseDash();
delay(100);
MorseDot();
delay(100);
break;
case 'o':
MorseDash();
delay(100);
MorseDash();
delay(100);
MorseDash();
delay(100);
break;
case 'p':
MorseDot();
delay(100);
MorseDash();
delay(100);
MorseDash();
delay(100);
MorseDot();
delay(100);
break;
case 'q':
MorseDash();
delay(100);
MorseDash();
delay(100);
MorseDot();
delay(100);
MorseDash();
delay(100);
break;
case 'r':
MorseDot();
delay(100);
MorseDash();
delay(100);
MorseDot();
delay(100);
break;
case 's':
MorseDot();
delay(100);
MorseDot();
delay(100);
MorseDot();
delay(100);
break;
case 't':
MorseDash();
delay(100);
break;
case 'u':
MorseDot();
delay(100);
MorseDot();
delay(100);
MorseDash();
delay(100);
break;
case 'v':
MorseDot();
delay(100);
MorseDot();
delay(100);
MorseDot();
delay(100);
MorseDash();
delay(100);
break;
case 'w':
MorseDot();
delay(100);
MorseDash();
delay(100);
MorseDash();
delay(100);
break;
case 'x':
MorseDash();
delay(100);
MorseDot();
delay(100);
MorseDot();
delay(100);
MorseDash();
delay(100);
break;
case 'y':
MorseDash();
delay(100);
MorseDot();
delay(100);
MorseDash();
delay(100);
MorseDash();
delay(100);
break;
case 'z':
MorseDash();
delay(100);
MorseDash();
delay(100);
MorseDot();
delay(100);
MorseDot();
delay(100);
break;
default:
break;
}
}
Hello, This project generate Morse code for anything you type in the COM window? Not just for SOS?